-
Notifications
You must be signed in to change notification settings - Fork 31
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #47 from ctk03272/master
안녕하세요 온도/습도 센서 보내드립니다.
- Loading branch information
Showing
4 changed files
with
167 additions
and
47 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,98 @@ | ||
|
||
Arduino 온/습도 센서 개발 | ||
============================== | ||
|
||
# Arduino 온/습도 센서 개발 | ||
|
||
|
||
* Arduino ESP 8266 에 DHT11 센서를 장착하여 온/습도 측정을 가능하게 한다. | ||
## 사용한 DHT11의 경우 아래와 같은 모양을 하고 있다. | ||
|
||
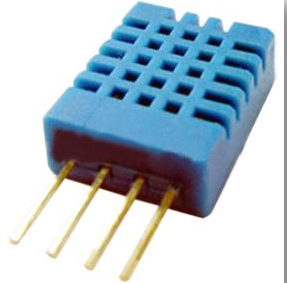 | ||
|
||
* 이 경우 왼쪽부터 전원, 데이터 OUT, 사용하지 않음, 그라운드의 순서이다. | ||
|
||
|
||
|
||
|
||
## 회로도의 경우 위와 같다. | ||
|
||
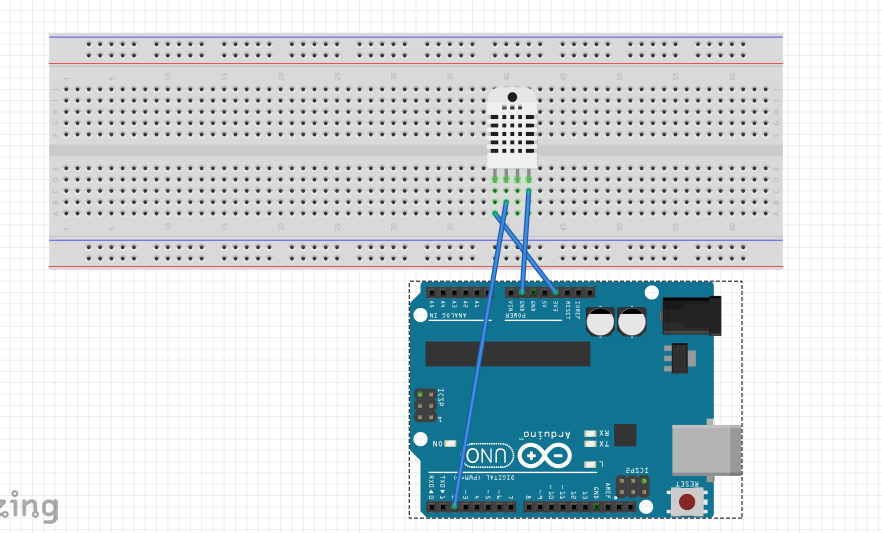 | ||
|
||
* 디지털 2번포트와 데이터 포트가 연결되어 있고, 전원은 3.3V 그리고 GND에 연결되어 있다. | ||
|
||
* 이런식으로 연결할 경우 출력으로 습도, 온도(섭씨, 화시), 습도를 고려한 체감온도가 출력되게 된다. | ||
|
||
## 코드의 경우 아래와 같다. | ||
|
||
// Example testing sketch for various DHT humidity/temperature sensors | ||
// Written by ladyada, public domain | ||
|
||
#include "DHT.h" | ||
|
||
#define DHTPIN 2 // what digital pin we're connected to | ||
|
||
// Uncomment whatever type you're using! | ||
#define DHTTYPE DHT11 // DHT 11 | ||
//#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321 | ||
//#define DHTTYPE DHT21 // DHT 21 (AM2301) | ||
|
||
// Connect pin 1 (on the left) of the sensor to +5V | ||
// NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1 | ||
// to 3.3V instead of 5V! | ||
// Connect pin 2 of the sensor to whatever your DHTPIN is | ||
// Connect pin 4 (on the right) of the sensor to GROUND | ||
// Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor | ||
|
||
// Initialize DHT sensor. | ||
// Note that older versions of this library took an optional third parameter to | ||
// tweak the timings for faster processors. This parameter is no longer needed | ||
// as the current DHT reading algorithm adjusts itself to work on faster procs. | ||
DHT dht(DHTPIN, DHTTYPE); | ||
|
||
void setup() { | ||
Serial.begin(9600); | ||
Serial.println("DHTxx test!"); | ||
|
||
dht.begin(); | ||
} | ||
|
||
void loop() { | ||
// Wait a few seconds between measurements. | ||
delay(2000); | ||
|
||
// Reading temperature or humidity takes about 250 milliseconds! | ||
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) | ||
float h = dht.readHumidity(); | ||
// Read temperature as Celsius (the default) | ||
float t = dht.readTemperature(); | ||
// Read temperature as Fahrenheit (isFahrenheit = true) | ||
float f = dht.readTemperature(true); | ||
|
||
// Check if any reads failed and exit early (to try again). | ||
if (isnan(h) || isnan(t) || isnan(f)) { | ||
Serial.println("Failed to read from DHT sensor!"); | ||
return; | ||
} | ||
|
||
// Compute heat index in Fahrenheit (the default) | ||
float hif = dht.computeHeatIndex(f, h); | ||
// Compute heat index in Celsius (isFahreheit = false) | ||
float hic = dht.computeHeatIndex(t, h, false); | ||
|
||
Serial.print("Humidity: "); | ||
Serial.print(h); | ||
Serial.print(" %\t"); | ||
Serial.print("Temperature: "); | ||
Serial.print(t); | ||
Serial.print(" *C "); | ||
Serial.print(f); | ||
Serial.print(" *F\t"); | ||
Serial.print("Heat index: "); | ||
Serial.print(hic); | ||
Serial.print(" *C "); | ||
Serial.print(hif); | ||
Serial.println(" *F"); | ||
} | ||
|
||
* 이 코드의 경우 DHT11, DHT21, DHT22 겸용이며 위의 코드에서 바꿔주게 될경우 각각의 기기의 대하여 사용할 수 있다. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,69 @@ | ||
// Example testing sketch for various DHT humidity/temperature sensors | ||
// Written by ladyada, public domain | ||
|
||
#include "DHT.h" | ||
|
||
#define DHTPIN 2 // what digital pin we're connected to | ||
|
||
// Uncomment whatever type you're using! | ||
#define DHTTYPE DHT11 // DHT 11 | ||
//#define DHTTYPE DHT22 // DHT 22 (AM2302), AM2321 | ||
//#define DHTTYPE DHT21 // DHT 21 (AM2301) | ||
|
||
// Connect pin 1 (on the left) of the sensor to +5V | ||
// NOTE: If using a board with 3.3V logic like an Arduino Due connect pin 1 | ||
// to 3.3V instead of 5V! | ||
// Connect pin 2 of the sensor to whatever your DHTPIN is | ||
// Connect pin 4 (on the right) of the sensor to GROUND | ||
// Connect a 10K resistor from pin 2 (data) to pin 1 (power) of the sensor | ||
|
||
// Initialize DHT sensor. | ||
// Note that older versions of this library took an optional third parameter to | ||
// tweak the timings for faster processors. This parameter is no longer needed | ||
// as the current DHT reading algorithm adjusts itself to work on faster procs. | ||
DHT dht(DHTPIN, DHTTYPE); | ||
|
||
void setup() { | ||
Serial.begin(9600); | ||
Serial.println("DHTxx test!"); | ||
|
||
dht.begin(); | ||
} | ||
|
||
void loop() { | ||
// Wait a few seconds between measurements. | ||
delay(2000); | ||
|
||
// Reading temperature or humidity takes about 250 milliseconds! | ||
// Sensor readings may also be up to 2 seconds 'old' (its a very slow sensor) | ||
float h = dht.readHumidity(); | ||
// Read temperature as Celsius (the default) | ||
float t = dht.readTemperature(); | ||
// Read temperature as Fahrenheit (isFahrenheit = true) | ||
float f = dht.readTemperature(true); | ||
|
||
// Check if any reads failed and exit early (to try again). | ||
if (isnan(h) || isnan(t) || isnan(f)) { | ||
Serial.println("Failed to read from DHT sensor!"); | ||
return; | ||
} | ||
|
||
// Compute heat index in Fahrenheit (the default) | ||
float hif = dht.computeHeatIndex(f, h); | ||
// Compute heat index in Celsius (isFahreheit = false) | ||
float hic = dht.computeHeatIndex(t, h, false); | ||
|
||
Serial.print("Humidity: "); | ||
Serial.print(h); | ||
Serial.print(" %\t"); | ||
Serial.print("Temperature: "); | ||
Serial.print(t); | ||
Serial.print(" *C "); | ||
Serial.print(f); | ||
Serial.print(" *F\t"); | ||
Serial.print("Heat index: "); | ||
Serial.print(hic); | ||
Serial.print(" *C "); | ||
Serial.print(hif); | ||
Serial.println(" *F"); | ||
} |
This file was deleted.
Oops, something went wrong.
This file was deleted.
Oops, something went wrong.