-
-
Notifications
You must be signed in to change notification settings - Fork 108
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: new “extend” method for makeServicePlugin
## API ``` import { makeServicePlugin } from ‘feathers-vuex’ import { feathersClient } from ‘./feathers-client.js’ class Todo { /* truncated */ } makeServicePlugin({ Model: Todo, service: feathersClient.service(‘todos’), extend({ store, module }) { // Listen to other parts of the store store.watch(/* truncated */) return { state: {}, getters: {}, mutations: {}, actions: {} } } }) The old options for customizing the store are still fully functional. The `module` object in the options contains the default state, getters, mutations, and actions merged with the ones provided in the options. They are made available in case this is useful; however, they do not need to be returned `extend` method’s return object. The object returned from `extend` will be merged over the top of the ones in `module`. As a result of this change, the following options are now deprecated in the `makeServicePlugin` method: - state - getters - mutations - actions ## History and Relevance Up until now, `makeServicePlugin` and `makeAuthPlugin` have supported passing `state`, `getters`, `mutations`, and `actions` as keys in the options as shown here: 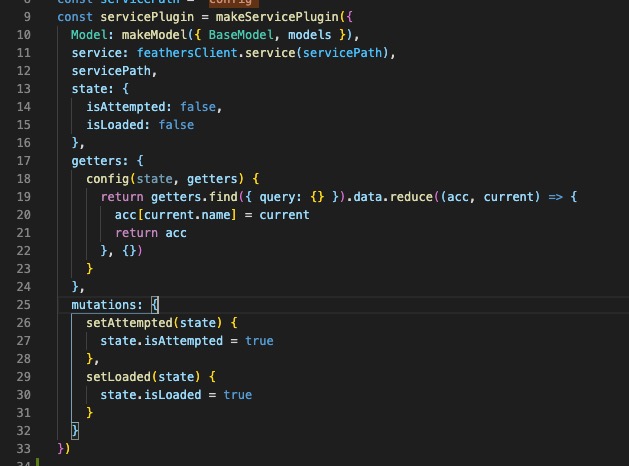 This is nice and clean until you need access to the `store` variable to access APIs like `store.watch` (when you want a clean way to define related logic between multiple vuex modules). This issue specifically showed up when I wanted a purely-logical, non-template-based method of watching for authentication from Auth0. Up until now, I have always used the “Gateway Component” pattern that has a conditional in it for if auth is present, then put the majority of the app logic in a child component. As shown in the above example, this extra logic can now live inside the same module as the rest of the service and vuex plugin definition.
- Loading branch information
1 parent
8d399fa
commit 4c8a627
Showing
5 changed files
with
285 additions
and
103 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.