-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
use case for writing to .csv, sample classes for testing
- added a use case for writing schedule data to the .csv - new sample "HRSystem", "Employee" and "Main" classes to facilitate testing
- Loading branch information
1 parent
8bc2dcf
commit 6d14af3
Showing
14 changed files
with
157 additions
and
60 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,39 +1,7 @@ | ||
# Project Template | ||
# How to Run the Program | ||
|
||
This is a template repository for CSC 207 projects. | ||
This repository contains starter code for a gradle project. | ||
It also contains workflow documents that give instructions on how to manage your Github repository and how to use Github Projects for efficient collaboration. | ||
Since this feature is only the "Schedule" portion of the overall HR Management System, there are some dependencies on classes that are present throughout the entire system. | ||
There have been stand-in classes ("Employee" and "HRSystem") made to test this portion somewhat independently, as well as a "Main" class that can be run to simulate an active HR System that would open the Schedule interface. | ||
|
||
## Checklist For Your Project | ||
- [ ] Verify the correct settings for your project repository | ||
- [ ] Set up Github Projects | ||
- [ ] Create the implementation plan using issues and Github Projects | ||
- [ ] Create deveopment branches for your features | ||
- [ ] Use pull requests to merge finished features into main branch | ||
- [ ] Conduct code reviews | ||
|
||
**If your team has trouble with any of these steps, please ask on Piazza. For example, with how GitHub Classroom works, your team *may* not have permissions to do some of the first few steps, in which case we'll post alternative instructions as needed.** | ||
|
||
## Workflow Documents | ||
|
||
* Github Workflow: Please refer to the workflow that was introduced in the first lab. You should follow this when working on your code. The following document provides additional details too. | ||
|
||
* [Project Planning and Development Guide](project_plan_dev.md): This document helps you to understand how to create and maintain a project plan for your class project. **This document helps you to complete the Implementation Plan Milestone.** | ||
|
||
## Gradle Project | ||
Import this project into your Intellij editor. It should automatically recognise this as a gradle repository. | ||
The starter code was built using SDK version 11.0.1. Ensure that you are using this version for this project. (You can, of course, change the SDK version as per your requirement if your team has all agreed to use a different version) | ||
|
||
You have been provided with two starter files for demonstration: HelloWorld and HelloWorldTest. | ||
|
||
You will find HelloWorld in `src/main/java/tutorial` directory. Right click on the HelloWorld file and click on `Run HelloWorld.main()`. | ||
This should run the program and print on your console. | ||
|
||
You will find HelloWorldTest in `src/test/java/tutorial` directory. Right click on the HelloWorldTest file and click on `Run HelloWorldTest`. | ||
All tests should pass. Your team can remove this sample of how testing works once you start adding your project code to the repo. | ||
|
||
Moving forward, we expect you to maintain this project structure. You *should* use Gradle as the build environment, but it is fine if your team prefers to use something else -- just remove the gradle files and push your preferred project setup. Assuming you stick with Gradle, your source code should go into `src/main/java` (you can keep creating more subdirectories as per your project requirement). Every source class can auto-generate a test file for you. For example, open HelloWorld.java file and click on the `HelloWorld` variable as shown in the image below. You should see an option `Generate` and on clicking this your should see an option `Test`. Clicking on this will generate a JUnit test file for `HelloWorld` class. This was used to generate the `HelloWorldTest`. | ||
|
||
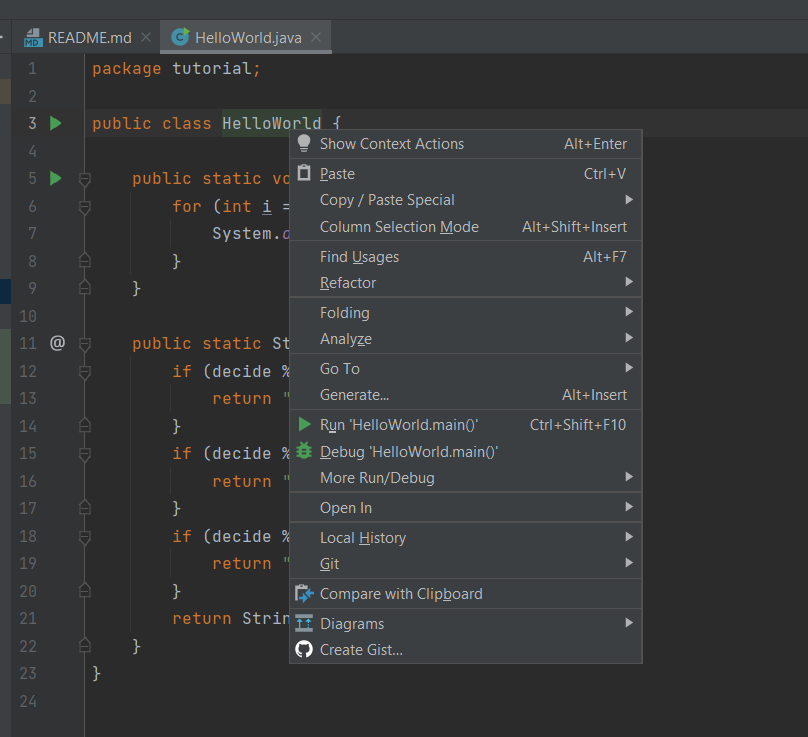 | ||
|
||
You can create another simple class and try generating a test for this class. | ||
A sample set of Employees has been hard-coded into the "Main" class in the "drivers" package, as well as a pre-set .csv file that matches the data. | ||
Run said class to test the scheduling feature. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
package drivers; | ||
|
||
import entities.Employee; | ||
import entities.HRSystem; | ||
import interfaceadapters.ScheduleController; | ||
import usecases.ScheduleFileReader; | ||
|
||
import java.io.IOException; | ||
import java.util.ArrayList; | ||
import java.util.List; | ||
|
||
public class Main { | ||
|
||
public static void main(String[] args) { | ||
try { | ||
String fileName = "ScheduleData.csv"; | ||
String currentWorkingDirectory = System.getProperty("user.dir"); | ||
String filePath = currentWorkingDirectory + "\\src\\main\\java\\resources\\" + fileName; | ||
List<String[]> data = ScheduleFileReader.readCSV(filePath); | ||
|
||
// Sample HRSystem | ||
ArrayList<Employee> employees = new ArrayList<>(); | ||
employees.add(new Employee("001", "name")); | ||
employees.add(new Employee("002", "name")); | ||
employees.add(new Employee("003", "name")); | ||
employees.add(new Employee("004", "name")); | ||
employees.add(new Employee("005", "name")); | ||
HRSystem hrSystem = new HRSystem(employees); | ||
|
||
ScheduleUI scheduleUI = new ScheduleUI(); | ||
|
||
ScheduleController scheduleController = new ScheduleController(hrSystem, scheduleUI, data); | ||
scheduleController.openUI(); | ||
} catch (IOException e) { | ||
System.out.println("Failed to open and read the schedule .csv file."); | ||
} | ||
|
||
} | ||
|
||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
package entities; | ||
|
||
public class Employee { | ||
|
||
private String id; | ||
private String name; | ||
|
||
public Employee(String id, String name) { | ||
this.id = id; | ||
this.name = name; | ||
} | ||
|
||
public String getId() { | ||
return this.id; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
package entities; | ||
|
||
import java.util.ArrayList; | ||
|
||
public class HRSystem { | ||
|
||
private ArrayList<Employee> employees; | ||
|
||
public HRSystem(ArrayList<Employee> employees) { | ||
this.employees = employees; | ||
} | ||
|
||
public ArrayList<Employee> getEmployees() { | ||
return this.employees; | ||
} | ||
|
||
private void addEmployee(Employee employee) { | ||
this.employees.add(employee); | ||
} | ||
|
||
private void removeEmployee(String id) { | ||
for (Employee employee : employees) { | ||
if (employee.getId() == id) { | ||
employees.remove(employee); | ||
return; | ||
} | ||
} | ||
} | ||
|
||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,4 +1,4 @@ | ||
"SHIFT: 9:00AM - 5:00PM" | ||
"001 002","001","001","001","001","001","001" | ||
"004","001 002","001","001","001","001","001" | ||
"SHIFT: 5:00PM - 11:00PM" | ||
"001 002","002","002","002","002","002","002" | ||
"001 002","002","002","002","002","002","002" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
package usecases; | ||
|
||
import java.io.FileWriter; | ||
import java.io.IOException; | ||
import java.util.ArrayList; | ||
|
||
public class ScheduleFileWriter { | ||
|
||
public static void writeCSV(String[][] data, String filePath) throws IOException { | ||
FileWriter writer = new FileWriter(filePath); | ||
ArrayList<String[]> parsedData = parseForCSV(data); | ||
for (String[] row : parsedData) { | ||
StringBuilder sb = new StringBuilder(); | ||
for (int i = 0; i < row.length; i++) { | ||
sb.append(row[i]); | ||
if (i < row.length - 1) { | ||
sb.append(","); | ||
} | ||
} | ||
sb.append(System.lineSeparator()); | ||
writer.write(sb.toString()); | ||
} | ||
writer.close(); | ||
} | ||
|
||
public static ArrayList<String[]> parseForCSV(String[][] data) { | ||
ArrayList<String[]> parsedData = new ArrayList<>(); | ||
for (String[] lst : data) { | ||
String shiftText = "\"SHIFT: " + lst[0] + "\""; | ||
parsedData.add(new String[]{shiftText}); | ||
String[] newLst = new String[lst.length - 1]; | ||
for (int i = 1; i < lst.length; i++) { | ||
newLst[i - 1] = "\"" + lst[i].replaceAll(",", "") + "\""; | ||
} | ||
parsedData.add(newLst); | ||
} | ||
return parsedData; | ||
} | ||
|
||
} |