diff --git a/docs/python_docs/python/tutorials/deploy/inference/image_classification_jetson.md b/docs/python_docs/python/tutorials/deploy/inference/image_classification_jetson.md
new file mode 100644
index 000000000000..5a697ca7960e
--- /dev/null
+++ b/docs/python_docs/python/tutorials/deploy/inference/image_classification_jetson.md
@@ -0,0 +1,117 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+# Image Classication using pretrained ResNet-50 model on Jetson module
+
+This tutorial shows how to install MXNet v1.6 with Jetson support and use it to deploy a pre-trained MXNet model for image classification on a Jetson module.
+
+## What's in this tutorial?
+
+This tutorial shows how to:
+
+1. Install MXNet v1.6 along with its dependencies on a Jetson module (This tutorial has been tested on Jetson Xavier AGX and Jetson Nano modules)
+
+2. Deploy a pre-trained MXNet model for image classifcation on the module
+
+## Who's this tutorial for?
+
+This tutorial would benefit developers working on Jetson modules implementing deep learning applications. It assumes that readers have a Jetson module setup with Jetpack installed, are familiar with the Jetson working environment and are somewhat familiar with deep learning using MXNet.
+
+## Prerequisites
+
+To complete this tutorial, you need:
+
+* A [Jetson module](https://developer.nvidia.com/embedded/develop/hardware) setup with [Jetpack 4.4](https://docs.nvidia.com/jetson/jetpack/release-notes/) installed using NVIDIA [SDK Manager](https://developer.nvidia.com/nvidia-sdk-manager)
+
+* An SSH connection to the module OR display and keyboard setup to directly open shell on the module
+
+* [Swapfile](https://help.ubuntu.com/community/SwapFaq) installed, especially on Jetson Nano for additional memory (increase memory if the inference script terminates with a `Killed` message)
+
+## Installing MXNet v1.6 with Jetson support
+
+To install MXNet with Jetson support, you can follow the [installation guide](https://mxnet.apache.org/get_started/jetson_setup) on MXNet official website.
+
+Alternatively, you can also directly install MXNet v1.6 wheel with Jetson support, hosted on a public s3 bucket. Here are the steps to install this wheel:
+
+*WARNING: this MXNet wheel is provided for your convenience but it contains packages that are not provided nor endorsed by the Apache Software Foundation.
+As such, they might contain software components with more restrictive licenses than the Apache License and you'll need to decide whether they are appropriate for your usage. Like all Apache Releases, the
+official Apache MXNet (incubating) releases consist of source code only and are found at https://mxnet.apache.org/get_started/download .*
+
+We start by installing MXNet dependencies
+```bash
+sudo apt-get update
+sudo apt-get install -y git build-essential libopenblas-dev libopencv-dev python3-pip
+sudo pip3 install -U pip
+```
+
+Then we download and install MXNet v1.6 wheel with Jetson support
+```bash
+wget https://mxnet-public.s3.us-east-2.amazonaws.com/install/jetson/1.6.0/mxnet_cu102-1.6.0-py2.py3-none-linux_aarch64.whl
+sudo pip3 install mxnet_cu102-1.6.0-py2.py3-none-linux_aarch64.whl
+```
+
+And we are done. You can test the installation now by importing mxnet from python3
+```bash
+>>> python3 -c 'import mxnet'
+```
+
+## Running a pre-trained ResNet-50 model on Jetson
+
+We are now ready to run a pre-trained model and run inference on a Jetson module. In this tutorial we are using ResNet-50 model trained on Imagenet dataset. We run the following classification script with either cpu/gpu context using python3.
+
+```python
+from mxnet import gluon
+import mxnet as mx
+
+# set context
+ctx = mx.gpu()
+
+# load pre-trained model
+net = gluon.model_zoo.vision.resnet50_v1(pretrained=True, ctx=ctx)
+net.hybridize(static_alloc=True, static_shape=True)
+
+# load labels
+lbl_path = gluon.utils.download('http://data.mxnet.io/models/imagenet/synset.txt')
+with open(lbl_path, 'r') as f:
+ labels = [l.rstrip() for l in f]
+
+# download and format image as (batch, RGB, width, height)
+img_path = gluon.utils.download('https://github.com/dmlc/web-data/blob/master/mxnet/doc/tutorials/python/predict_image/cat.jpg?raw=true')
+img = mx.image.imread(img_path)
+img = mx.image.imresize(img, 224, 224) # resize
+img = mx.image.color_normalize(img.astype(dtype='float32')/255,
+ mean=mx.nd.array([0.485, 0.456, 0.406]),
+ std=mx.nd.array([0.229, 0.224, 0.225])) # normalize
+img = img.transpose((2, 0, 1)) # channel first
+img = img.expand_dims(axis=0) # batchify
+img = img.as_in_context(ctx)
+
+prob = net(img).softmax() # predict and normalize output
+idx = prob.topk(k=5)[0] # get top 5 result
+for i in idx:
+ i = int(i.asscalar())
+ print('With prob = %.5f, it contains %s' % (prob[0,i].asscalar(), labels[i]))
+```
+
+After running the above script, you should get the following output showing the five classes that the image most relates to with probability:
+```bash
+With prob = 0.41940, it contains n02119789 kit fox, Vulpes macrotis
+With prob = 0.28096, it contains n02119022 red fox, Vulpes vulpes
+With prob = 0.06857, it contains n02124075 Egyptian cat
+With prob = 0.03046, it contains n02120505 grey fox, gray fox, Urocyon cinereoargenteus
+With prob = 0.02770, it contains n02441942 weasel
+```
diff --git a/docs/static_site/src/_includes/get_started/cloud/cpu.md b/docs/static_site/src/_includes/get_started/cloud/cpu.md
index 5415e96b6534..440582727b68 100644
--- a/docs/static_site/src/_includes/get_started/cloud/cpu.md
+++ b/docs/static_site/src/_includes/get_started/cloud/cpu.md
@@ -1,8 +1,16 @@
-MXNet should work on any cloud provider's CPU-only instances. Follow the Python pip install
-instructions,
-Docker instructions, or try the following preinstalled option.
+MXNet should work on any cloud provider's CPU-only instances. Follow the Python
+pip install instructions, Docker instructions, or try the following preinstalled
+option.
+
+WARNING: the following cloud provider packages are provided for your convenience
+but they point to packages that are *not* provided nor endorsed by the Apache
+Software Foundation. As such, they might contain software components with more
+restrictive licenses than the Apache License and you'll need to decide whether
+they are appropriate for your usage. Like all Apache Releases, the official
+Apache MXNet (incubating) releases consist of source code only and are found at
+the [Download page](https://mxnet.apache.org/get_started/download).
* **Amazon Web Services**
- [AWS Deep Learning AMI](https://aws.amazon.com/machine-learning/amis/) - Preinstalled
Conda environments
-for Python 2 or 3 with MXNet and MKL-DNN.
\ No newline at end of file
+for Python 2 or 3 with MXNet and MKL-DNN.
diff --git a/docs/static_site/src/_includes/get_started/cloud/gpu.md b/docs/static_site/src/_includes/get_started/cloud/gpu.md
index 725d96581776..8f64a3ac5cba 100644
--- a/docs/static_site/src/_includes/get_started/cloud/gpu.md
+++ b/docs/static_site/src/_includes/get_started/cloud/gpu.md
@@ -1,7 +1,14 @@
-MXNet is available on several cloud providers with GPU support. You can also find
-GPU/CPU-hybrid support for
-use cases like scalable inference, or even fractional GPU support with AWS Elastic
-Inference.
+MXNet is available on several cloud providers with GPU support. You can also
+find GPU/CPU-hybrid support for use cases like scalable inference, or even
+fractional GPU support with AWS Elastic Inference.
+
+WARNING: the following cloud provider packages are provided for your convenience
+but they point to packages that are *not* provided nor endorsed by the Apache
+Software Foundation. As such, they might contain software components with more
+restrictive licenses than the Apache License and you'll need to decide whether
+they are appropriate for your usage. Like all Apache Releases, the official
+Apache MXNet (incubating) releases consist of source code only and are found at
+the [Download page](https://mxnet.apache.org/get_started/download).
* **Alibaba**
- [NVIDIA
@@ -29,4 +36,4 @@ All NVIDIA VMs use the [NVIDIA MXNet Docker
container](https://ngc.nvidia.com/catalog/containers/nvidia:mxnet).
Follow the [container usage
instructions](https://ngc.nvidia.com/catalog/containers/nvidia:mxnet) found in
-[NVIDIA's container repository](https://ngc.nvidia.com/).
\ No newline at end of file
+[NVIDIA's container repository](https://ngc.nvidia.com/).
diff --git a/docs/static_site/src/_includes/get_started/get_started.html b/docs/static_site/src/_includes/get_started/get_started.html
index 772802fbaeb5..d8c7d3a42e5a 100644
--- a/docs/static_site/src/_includes/get_started/get_started.html
+++ b/docs/static_site/src/_includes/get_started/get_started.html
@@ -10,8 +10,10 @@
-
Installing MXNet
-
Indicate your preferred configuration. Then, follow the customized commands to install MXNet.
+
Platform and use-case specific instructions for using MXNet
+
+ Please indicate your preferred configuration below to see specific instructions.
+
+
-
-
- {% markdown %}{% include /get_started/macos/r/cpu.md %}{% endmarkdown %}
-
-
- {% markdown %}{% include /get_started/macos/r/gpu.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/macos/r/build-from-source.md %}{% endmarkdown %}
+
+
-
- {% markdown %}{% include /get_started/macos/scala/cpu.md %}{% endmarkdown %}
-
-
- {% markdown %}{% include /get_started/macos/scala/gpu.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/macos/scala/build-from-source.md %}{% endmarkdown %}
+
+
-
- {% markdown %}{% include /get_started/macos/clojure/cpu.md %}{% endmarkdown %}
-
-
- {% markdown %}{% include /get_started/macos/clojure/gpu.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/macos/clojure/build-from-source.md %}{% endmarkdown %}
+
-
- {% markdown %}{% include /get_started/macos/java/cpu.md %}{% endmarkdown %}
-
-
- {% markdown %}{% include /get_started/macos/java/gpu.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/macos/java/build-from-source.md %}{% endmarkdown %}
+
-
-
-
- {% markdown %}{% include /get_started/macos/julia/pkg.md %}{% endmarkdown %}
-
-
-
- {% markdown %}{% include /get_started/macos/julia/build-from-source.md %}{% endmarkdown %}
-
-
+
+ {% markdown %}{% include /get_started/macos/julia/build-from-source.md %}{% endmarkdown %}
+
-
- {% markdown %}{% include /get_started/macos/perl/perl.md %}{% endmarkdown %}
-
-
+
+ {% markdown %}{% include /get_started/macos/perl/build-from-source.md %}{% endmarkdown %}
+
+
-
- {% markdown %}{% include /get_started/macos/cpp/cpp.md %}{% endmarkdown %}
-
-
-
- For more installation options, refer to the MXNet macOS installation guide.
-
+
+ {% markdown %}{% include /get_started/macos/cpp/build-from-source.md %}{% endmarkdown %}
+
+
+
+
+
{% markdown %}{% include /get_started/windows/python/cpu/pip.md %}{% endmarkdown %}
-
- {% markdown %}{% include /get_started/windows/python/cpu/docker.md %}{% endmarkdown %}
-
-
{% markdown %}{% include /get_started/windows/python/cpu/build-from-source.md %}{% endmarkdown %}
-
-
+
+
+
+
{% markdown %}{% include /get_started/windows/python/gpu/pip.md %}{% endmarkdown %}
-
+
- {% markdown %}{% include /get_started/windows/python/gpu/build-from-source.md %}{% endmarkdown %}
-
-
-
-
+ {% markdown %}{% include /get_started/windows/python/gpu/build-from-source.md %}{% endmarkdown %}
+
+
+
+
-
-
- {% markdown %}{% include /get_started/windows/r/cpu.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/windows/r/build-from-source.md %}{% endmarkdown %}
+
+
-
- {% markdown %}{% include /get_started/windows/r/gpu.md %}{% endmarkdown %}
-
-
-
- {% markdown %}{% include /get_started/windows/scala/scala.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/windows/scala/build-from-source.md %}{% endmarkdown %}
+
+
-
- {% markdown %}{% include /get_started/windows/clojure/clojure.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/windows/clojure/build-from-source.md %}{% endmarkdown %}
+
+
-
- {% markdown %}{% include /get_started/windows/java/java.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/windows/java/build-from-source.md %}{% endmarkdown %}
+
-
-
- {% markdown %}{% include /get_started/windows/julia/pkg.md %}{% endmarkdown %}
-
-
- {% markdown %}{% include /get_started/windows/julia/build-from-source.md %}{% endmarkdown %}
-
-
+
+ {% markdown %}{% include /get_started/windows/julia/build-from-source.md %}{% endmarkdown %}
+
-
- {% markdown %}{% include /get_started/windows/perl/perl.md %}{% endmarkdown %}
-
+
+ {% markdown %}{% include /get_started/windows/perl/build-from-source.md %}{% endmarkdown %}
+
-
- {% markdown %}{% include /get_started/windows/cpp/cpp.md %}{% endmarkdown %}
-
-
-
- For more installation options, refer to the MXNet Windows installation guide.
-
+
+ {% markdown %}{% include /get_started/windows/cpp/build-from-source.md %}{% endmarkdown %}
+
+
+
diff --git a/docs/static_site/src/_includes/get_started/linux/clojure/cpu.md b/docs/static_site/src/_includes/get_started/linux/clojure/build-from-source.md
similarity index 100%
rename from docs/static_site/src/_includes/get_started/linux/clojure/cpu.md
rename to docs/static_site/src/_includes/get_started/linux/clojure/build-from-source.md
diff --git a/docs/static_site/src/_includes/get_started/linux/clojure/gpu.md b/docs/static_site/src/_includes/get_started/linux/clojure/gpu.md
deleted file mode 100644
index 26293f6e077f..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/clojure/gpu.md
+++ /dev/null
@@ -1,15 +0,0 @@
-You can use the Maven packages defined in the following dependency to include MXNet in your Clojure
-project. To maximize leverage, the Clojure package has been built on the existing Scala package. Please
-refer to the [MXNet-Scala setup guide]({{'/get_started/scala_setup'|relative_url}}) for a detailed set of instructions
-to help you with the setup process that is required to use the Clojure dependency.
-
-
-
-{% highlight html %}
-
- org.apache.mxnet.contrib.clojure
- clojure-mxnet-linux-gpu
-
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/linux/cpp/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/cpp/build-from-source.md
new file mode 100644
index 000000000000..d37cf77a3afc
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/linux/cpp/build-from-source.md
@@ -0,0 +1,2 @@
+To use the C++ package, build from source the `USE_CPP_PACKAGE=1` option. Please
+refer to the build from source instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/cpp/cpp.md b/docs/static_site/src/_includes/get_started/linux/cpp/cpp.md
deleted file mode 100644
index dc492d801262..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/cpp/cpp.md
+++ /dev/null
@@ -1,4 +0,0 @@
-To enable the C++ package, build from source using `make USE_CPP_PACKAGE=1`.
-Refer to the [MXNet C++ setup guide](/get_started/cpp_setup.html)
-for full instructions.
-
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/linux/java/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/java/build-from-source.md
new file mode 100644
index 000000000000..585cc3e41890
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/linux/java/build-from-source.md
@@ -0,0 +1,6 @@
+Previously available binaries distributed via Maven have been removed as they
+redistributed Category-X binaries in violation of Apache Software Foundation
+(ASF) policies.
+
+At this point in time, no third-party binary Java packages are available. Please
+follow the build from source instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/java/gpu.md b/docs/static_site/src/_includes/get_started/linux/java/gpu.md
deleted file mode 100644
index 6f6757f6e2ea..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/java/gpu.md
+++ /dev/null
@@ -1,17 +0,0 @@
-You can use the Maven packages defined in the following dependency to include MXNet in your Java
-project. The Java API is provided as a subset of the Scala API and is intended for inference only.
-Please refer to the MXNet-Java setup guide for a detailed set of
-instructions to help you with the setup process.
-
-
-
-
-
-{% highlight html %}
-
- org.apache.mxnet
- mxnet-full_2.11-linux-x86_64-gpu
- [1.5.0, )
-
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/linux/julia/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/julia/build-from-source.md
index 018aca9d7387..cef507527bfe 100644
--- a/docs/static_site/src/_includes/get_started/linux/julia/build-from-source.md
+++ b/docs/static_site/src/_includes/get_started/linux/julia/build-from-source.md
@@ -1,2 +1 @@
-Refer to the [Julia section of the MXNet Ubuntu installation guide](/get_started/ubuntu_setup#install-the-mxnet-package-for-julia).
-
+Please follow the build from source instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/julia/pkg.md b/docs/static_site/src/_includes/get_started/linux/julia/pkg.md
deleted file mode 100644
index 35971305ee2d..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/julia/pkg.md
+++ /dev/null
@@ -1,10 +0,0 @@
-Install a pinned version of MXNet like this:
-
-{% highlight julia %}
-]add MXNet#v1.5.0
-{% endhighlight %}
-
-Or directly install the latest release:
-{% highlight julia %}
-]add MXNet
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/linux/perl/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/perl/build-from-source.md
new file mode 100644
index 000000000000..cef507527bfe
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/linux/perl/build-from-source.md
@@ -0,0 +1 @@
+Please follow the build from source instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/perl/perl.md b/docs/static_site/src/_includes/get_started/linux/perl/perl.md
deleted file mode 100644
index 02d82e457726..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/perl/perl.md
+++ /dev/null
@@ -1 +0,0 @@
-Refer to the [Perl section of the MXNet Ubuntu installation guide](get_started/ubuntu_setup.html#install-the-mxnet-package-for-perl).
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/linux/python/cpu/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/python/cpu/build-from-source.md
index 4adf039739d5..cef507527bfe 100644
--- a/docs/static_site/src/_includes/get_started/linux/python/cpu/build-from-source.md
+++ b/docs/static_site/src/_includes/get_started/linux/python/cpu/build-from-source.md
@@ -1 +1 @@
-To build from source, refer to the [MXNet Ubuntu installation guide]({{'/get_started/ubuntu_setup' | relative_url}}).
+Please follow the build from source instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/python/cpu/docker.md b/docs/static_site/src/_includes/get_started/linux/python/cpu/docker.md
index 8f2c8eb143a4..7eab6d36dec2 100644
--- a/docs/static_site/src/_includes/get_started/linux/python/cpu/docker.md
+++ b/docs/static_site/src/_includes/get_started/linux/python/cpu/docker.md
@@ -1,22 +1,18 @@
-Docker images with *MXNet* are available at [DockerHub](https://hub.docker.com/r/mxnet/).
-
-**Step 1** Install Docker on your machine by following the [docker installation
-instructions](https://docs.docker.com/engine/installation/linux/ubuntu/#install-using-the-repository).
-
-*Note* - You can install Community Edition (CE) to get started with *MXNet*.
-
-**Step 2** [Optional] Post installation steps to manage Docker as a non-root user.
+WARNING: the following links and names of binary distributions are provided for
+your convenience but they point to packages that are *not* provided nor endorsed
+by the Apache Software Foundation. As such, they might contain software
+components with more restrictive licenses than the Apache License and you'll
+need to decide whether they are appropriate for your usage. Like all Apache
+Releases, the official Apache MXNet (incubating) releases consist of source code
+only and are found at
+the [Download page](https://mxnet.apache.org/get_started/download).
+
-Follow the four steps in this [docker
-documentation](https://docs.docker.com/engine/installation/linux/linux-postinstall/#manage-docker-as-a-non-root-user)
-to allow managing docker containers without *sudo*.
-
-If you skip this step, you need to use *sudo* each time you invoke Docker.
-
-**Step 3** Pull the MXNet docker image.
+Docker images with *MXNet* are available at [DockerHub](https://hub.docker.com/r/mxnet/).
+After you installed Docker on your machine, you can use them via:
{% highlight bash %}
-$ docker pull mxnet/python # Use sudo if you skip Step 2
+$ docker pull mxnet/python
{% endhighlight %}
You can list docker images to see if mxnet/python docker image pull was successful.
@@ -28,16 +24,4 @@ REPOSITORY TAG IMAGE ID CREATED SIZE
mxnet/python latest 00d026968b3c 3 weeks ago 1.41 GB
{% endhighlight %}
-Using the latest MXNet with [Intel MKL-DNN](https://github.com/intel/mkl-dnn) is
-recommended for the
-fastest inference speeds with MXNet.
-
-{% highlight bash %}
-$ docker pull mxnet/python:1.3.0_cpu_mkl # Use sudo if you skip Step 2
-$ docker images # Use sudo if you skip Step 2
-
-REPOSITORY TAG IMAGE ID CREATED SIZE
-mxnet/python 1.3.0_cpu_mkl deaf9bf61d29 4 days ago 678 MB
-{% endhighlight %}
-
-**Step 4** Validate the installation.
\ No newline at end of file
+You can then validate the installation.
diff --git a/docs/static_site/src/_includes/get_started/linux/python/cpu/pip.md b/docs/static_site/src/_includes/get_started/linux/python/cpu/pip.md
index 81db75ea9038..83e86047dc4f 100644
--- a/docs/static_site/src/_includes/get_started/linux/python/cpu/pip.md
+++ b/docs/static_site/src/_includes/get_started/linux/python/cpu/pip.md
@@ -1,8 +1,31 @@
+WARNING: the following PyPI package names are provided for your convenience but
+they point to packages that are *not* provided nor endorsed by the Apache
+Software Foundation. As such, they might contain software components with more
+restrictive licenses than the Apache License and you'll need to decide whether
+they are appropriate for your usage. Like all Apache Releases, the official
+Apache MXNet (incubating) releases consist of source code only and are found at
+the [Download page](https://mxnet.apache.org/get_started/download).
+
Run the following command:
-
+
+{% highlight bash %}
+pip install mxnet
+{% endhighlight %}
+
+MKL-DNN enabled pip packages are optimized for Intel hardware. You can find
+performance numbers
+in the
MXNet tuning guide.
+
+{% highlight bash %}
+pip install mxnet-mkl
+{% endhighlight %}
+
+
+
+
{% highlight bash %}
-$ pip install mxnet
+pip install mxnet==1.5.1
{% endhighlight %}
MKL-DNN enabled pip packages are optimized for Intel hardware. You can find
@@ -10,15 +33,15 @@ performance numbers
in the
MXNet tuning guide.
{% highlight bash %}
-$ pip install mxnet-mkl
+pip install mxnet-mkl==1.5.1
{% endhighlight %}
-
+
{% highlight bash %}
-$ pip install mxnet==1.4.1
+pip install mxnet==1.4.1
{% endhighlight %}
MKL-DNN enabled pip packages are optimized for Intel hardware. You can find
@@ -26,14 +49,14 @@ performance numbers
in the
MXNet tuning guide.
{% highlight bash %}
-$ pip install mxnet-mkl==1.4.1
+pip install mxnet-mkl==1.4.1
{% endhighlight %}
{% highlight bash %}
-$ pip install mxnet==1.3.1
+pip install mxnet==1.3.1
{% endhighlight %}
MKL-DNN enabled pip packages are optimized for Intel hardware. You can find
@@ -41,14 +64,14 @@ performance numbers
in the
MXNet tuning guide.
{% highlight bash %}
-$ pip install mxnet-mkl==1.3.1
+pip install mxnet-mkl==1.3.1
{% endhighlight %}
{% highlight bash %}
-$ pip install mxnet==1.2.1
+pip install mxnet==1.2.1
{% endhighlight %}
MKL-DNN enabled pip packages are optimized for Intel hardware. You can find
@@ -56,7 +79,7 @@ performance numbers
in the
MXNet tuning guide.
{% highlight bash %}
-$ pip install mxnet-mkl==1.2.1
+pip install mxnet-mkl==1.2.1
{% endhighlight %}
@@ -64,7 +87,7 @@ $ pip install mxnet-mkl==1.2.1
{% highlight bash %}
-$ pip install mxnet==1.1.0
+pip install mxnet==1.1.0
{% endhighlight %}
@@ -72,7 +95,7 @@ $ pip install mxnet==1.1.0
{% highlight bash %}
-$ pip install mxnet==1.0.0
+pip install mxnet==1.0.0
{% endhighlight %}
@@ -81,13 +104,13 @@ $ pip install mxnet==1.0.0
{% highlight bash %}
-$ pip install mxnet==0.12.1
+pip install mxnet==0.12.1
{% endhighlight %}
For MXNet 0.12.0:
{% highlight bash %}
-$ pip install mxnet==0.12.0
+pip install mxnet==0.12.0
{% endhighlight %}
@@ -95,27 +118,12 @@ $ pip install mxnet==0.12.0
{% highlight bash %}
-$ pip install mxnet==0.11.0
+pip install mxnet==0.11.0
{% endhighlight %}
-
-
-{% highlight bash %}
-$ pip install mxnet --pre
-{% endhighlight %}
-
-MKL-DNN enabled pip packages are optimized for Intel hardware. You can find
-performance numbers
-in the
MXNet tuning guide.
-
-{% highlight bash %}
-$ pip install mxnet-mkl --pre
-{% endhighlight %}
-
-
-{% include /get_started/pip_snippet.md %}
\ No newline at end of file
+{% include /get_started/pip_snippet.md %}
diff --git a/docs/static_site/src/_includes/get_started/linux/python/gpu/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/python/gpu/build-from-source.md
index 4adf039739d5..cef507527bfe 100644
--- a/docs/static_site/src/_includes/get_started/linux/python/gpu/build-from-source.md
+++ b/docs/static_site/src/_includes/get_started/linux/python/gpu/build-from-source.md
@@ -1 +1 @@
-To build from source, refer to the [MXNet Ubuntu installation guide]({{'/get_started/ubuntu_setup' | relative_url}}).
+Please follow the build from source instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/python/gpu/docker.md b/docs/static_site/src/_includes/get_started/linux/python/gpu/docker.md
index dc21cf10b4ef..f963bc9c58de 100644
--- a/docs/static_site/src/_includes/get_started/linux/python/gpu/docker.md
+++ b/docs/static_site/src/_includes/get_started/linux/python/gpu/docker.md
@@ -1,24 +1,19 @@
-Docker images with *MXNet* are available at [DockerHub](https://hub.docker.com/r/mxnet/).
-
-**Step 1** Install Docker on your machine by following the [docker installation
-instructions](https://docs.docker.com/engine/installation/linux/ubuntu/#install-using-the-repository).
-
-*Note* - You can install Community Edition (CE) to get started with *MXNet*.
-
-**Step 2** [Optional] Post installation steps to manage Docker as a non-root user.
+WARNING: the following links and names of binary distributions are provided for
+your convenience but they point to packages that are *not* provided nor endorsed
+by the Apache Software Foundation. As such, they might contain software
+components with more restrictive licenses than the Apache License and you'll
+need to decide whether they are appropriate for your usage. Like all Apache
+Releases, the official Apache MXNet (incubating) releases consist of source code
+only and are found at
+the [Download page](https://mxnet.apache.org/get_started/download).
-Follow the four steps in this [docker
-documentation](https://docs.docker.com/engine/installation/linux/linux-postinstall/#manage-docker-as-a-non-root-user)
-to allow managing docker containers without *sudo*.
-
-If you skip this step, you need to use *sudo* each time you invoke Docker.
+Docker images with *MXNet* are available at [DockerHub](https://hub.docker.com/r/mxnet/).
-**Step 3** Install *nvidia-docker-plugin* following the [installation
-instructions](https://github.com/NVIDIA/nvidia-docker/wiki). *nvidia-docker-plugin*
-is required to
-enable the usage of GPUs from the docker containers.
+Please follow the [NVidia Docker installation
+instructions](https://github.com/NVIDIA/nvidia-docker/wiki) to enable the usage
+of GPUs from the docker containers.
-**Step 4** Pull the MXNet docker image.
+After you installed Docker on your machine, you can use them via:
{% highlight bash %}
$ docker pull mxnet/python:gpu # Use sudo if you skip Step 2
@@ -33,16 +28,4 @@ REPOSITORY TAG IMAGE ID CREATED SIZE
mxnet/python gpu 493b2683c269 3 weeks ago 4.77 GB
{% endhighlight %}
-Using the latest MXNet with [Intel MKL-DNN](https://github.com/intel/mkl-dnn) is
-recommended for the
-fastest inference speeds with MXNet.
-
-{% highlight bash %}
-$ docker pull mxnet/python:1.3.0_cpu_mkl # Use sudo if you skip Step 2
-$ docker images # Use sudo if you skip Step 2
-
-REPOSITORY TAG IMAGE ID CREATED SIZE
-mxnet/python 1.3.0_gpu_cu92_mkl adcb3ab19f50 4 days ago 4.23 GB
-{% endhighlight %}
-
-**Step 5** Validate the installation.
\ No newline at end of file
+You can then validate the installation.
diff --git a/docs/static_site/src/_includes/get_started/linux/python/gpu/pip.md b/docs/static_site/src/_includes/get_started/linux/python/gpu/pip.md
index 249cd5b54052..b47226c73977 100644
--- a/docs/static_site/src/_includes/get_started/linux/python/gpu/pip.md
+++ b/docs/static_site/src/_includes/get_started/linux/python/gpu/pip.md
@@ -1,11 +1,26 @@
+WARNING: the following PyPI package names are provided for your convenience but
+they point to packages that are *not* provided nor endorsed by the Apache
+Software Foundation. As such, they might contain software components with more
+restrictive licenses than the Apache License and you'll need to decide whether
+they are appropriate for your usage. Like all Apache Releases, the official
+Apache MXNet (incubating) releases consist of source code only and are found at
+the [Download page](https://mxnet.apache.org/get_started/download).
+
Run the following command:
-
+
+{% highlight bash %}
+$ pip install mxnet-cu102
+{% endhighlight %}
+
+
+
+
{% highlight bash %}
-$ pip install mxnet-cu101
+$ pip install mxnet-cu101==1.5.1
{% endhighlight %}
-
+
{% highlight bash %}
@@ -60,15 +75,7 @@ $ pip install mxnet-cu80==0.11.0
-
-
-{% highlight bash %}
-$ pip install mxnet-cu101 --pre
-{% endhighlight %}
-
-
-
{% include /get_started/pip_snippet.md %}
-{% include /get_started/gpu_snippet.md %}
\ No newline at end of file
+{% include /get_started/gpu_snippet.md %}
diff --git a/docs/static_site/src/_includes/get_started/linux/r/build-from-source.md b/docs/static_site/src/_includes/get_started/linux/r/build-from-source.md
new file mode 100644
index 000000000000..1150a309223d
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/linux/r/build-from-source.md
@@ -0,0 +1,2 @@
+You will need to R v3.4.4+ and build MXNet from source. Please follow the
+instructions linked above.
diff --git a/docs/static_site/src/_includes/get_started/linux/r/cpu.md b/docs/static_site/src/_includes/get_started/linux/r/cpu.md
deleted file mode 100644
index 1077362c9a65..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/r/cpu.md
+++ /dev/null
@@ -1,10 +0,0 @@
-The default version of R that is installed with `apt-get` is insufficient. You will need
-to first [install R v3.4.4+ and build MXNet from source](/get_started/ubuntu_setup.html#install-the-mxnet-package-for-r).
-
-After you have setup R v3.4.4+ and MXNet, you can build and install the MXNet R bindings with the following, assuming that `incubator-mxnet` is the source directory you used to build MXNet as follows:
-
-{% highlight bash %}
-$ cd incubator-mxnet
-$ mkdir build; cd build; cmake -DUSE_CUDA=OFF ..; make -j $(nproc); cd ..
-$ make -f R-package/Makefile rpkg
-{% endhighlight %}
diff --git a/docs/static_site/src/_includes/get_started/linux/r/gpu.md b/docs/static_site/src/_includes/get_started/linux/r/gpu.md
deleted file mode 100644
index 1ef16df25da5..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/r/gpu.md
+++ /dev/null
@@ -1,17 +0,0 @@
-The default version of R that is installed with `apt-get` is insufficient. You will need
-to first
-[install R v3.4.4+ and build MXNet from
-source](/get_started/ubuntu_setup.html#install-the-mxnet-package-for-r).
-
-After you have setup R v3.4.4+ and MXNet, you can build and install the MXNet R bindings
-with the
-following, assuming that `incubator-mxnet` is the source directory you used to build
-MXNet as follows:
-
-{% highlight bash %}
-$ cd incubator-mxnet
-$ mkdir build; cd build; cmake ..; make -j $(nproc); cd ..
-$ make -f R-package/Makefile rpkg
-{% endhighlight %}
-
-{% include /get_started/gpu_snippet.md %}
diff --git a/docs/static_site/src/_includes/get_started/linux/java/cpu.md b/docs/static_site/src/_includes/get_started/linux/scala/build-from-source.md
similarity index 100%
rename from docs/static_site/src/_includes/get_started/linux/java/cpu.md
rename to docs/static_site/src/_includes/get_started/linux/scala/build-from-source.md
diff --git a/docs/static_site/src/_includes/get_started/linux/scala/cpu.md b/docs/static_site/src/_includes/get_started/linux/scala/cpu.md
deleted file mode 100644
index 3cc96bade7df..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/scala/cpu.md
+++ /dev/null
@@ -1,14 +0,0 @@
-You can use the Maven packages defined in the following dependency to include MXNet in your Scala
-project. Please refer to the [MXNet-Scala setup guide]({{'/get_started/scala_setup'|relative_url}}) for
-a detailed set of instructions to help you with the setup process.
-
-
-
-{% highlight html %}
-
-org.apache.mxnet
-mxnet-full_2.11-linux-x86_64-cpu
-
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/linux/scala/gpu.md b/docs/static_site/src/_includes/get_started/linux/scala/gpu.md
deleted file mode 100644
index 5e2f9b179205..000000000000
--- a/docs/static_site/src/_includes/get_started/linux/scala/gpu.md
+++ /dev/null
@@ -1,16 +0,0 @@
-You can use the Maven packages defined in the following dependency to include MXNet in
-your Scala
-project. Please refer to the MXNet-Scala setup guide for
-a detailed set
-of instructions to help you with the setup process.
-
-
-
-{% highlight html %}
-
-org.apache.mxnet
-mxnet-full_2.11-linux-x86_64-gpu
-
-{% endhighlight %}
diff --git a/docs/static_site/src/_includes/get_started/macos b/docs/static_site/src/_includes/get_started/macos
new file mode 120000
index 000000000000..9c52cb36f47e
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/macos
@@ -0,0 +1 @@
+linux
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/clojure/cpu.md b/docs/static_site/src/_includes/get_started/macos/clojure/cpu.md
deleted file mode 100644
index 1e27af5e5dd7..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/clojure/cpu.md
+++ /dev/null
@@ -1,17 +0,0 @@
-
-You can use the Maven packages defined in the following dependency to include MXNet in
-your Clojure project. To maximize leverage, the Clojure package has been built on the existing Scala
-package. Please refer to the [MXNet-Scala setup guide](scala_setup.html) for a detailed set
-of instructions to help you with the setup process that is required to use the Clojure dependency.
-
-
-
-{% highlight html %}
-
- org.apache.mxnet.contrib.clojure
- clojure-mxnet-osx-cpu
-
-{% endhighlight %}
-
diff --git a/docs/static_site/src/_includes/get_started/macos/clojure/gpu.md b/docs/static_site/src/_includes/get_started/macos/clojure/gpu.md
deleted file mode 100644
index ccbc24db96e7..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/clojure/gpu.md
+++ /dev/null
@@ -1 +0,0 @@
-Not available at this time.
diff --git a/docs/static_site/src/_includes/get_started/macos/cpp/cpp.md b/docs/static_site/src/_includes/get_started/macos/cpp/cpp.md
deleted file mode 100644
index cb9e613928d9..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/cpp/cpp.md
+++ /dev/null
@@ -1,3 +0,0 @@
-To enable the C++ package, build from source using `make USE_CPP_PACKAGE=1`.
-Refer to the [MXNet C++ setup guide](/get_started/cpp_setup.html) for full instructions.
-
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/java/cpu.md b/docs/static_site/src/_includes/get_started/macos/java/cpu.md
deleted file mode 100644
index 002037a15771..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/java/cpu.md
+++ /dev/null
@@ -1,16 +0,0 @@
-You can use the Maven packages defined in the following dependency to include MXNet in
-your Java project. The Java API is provided as a subset of the Scala API and is intended for
-inference only.
-Please refer to the [MXNet-Java setup guide](/get_started/java_setup.html) for a detailed set of instructions to help you with the setup process.
-
-
-
-{% highlight html %}
-
- org.apache.mxnet
- mxnet-full_2.11-linux-x86_64-cpu
- [1.5.0, )
-
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/java/gpu.md b/docs/static_site/src/_includes/get_started/macos/java/gpu.md
deleted file mode 100644
index b17ef33478ea..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/java/gpu.md
+++ /dev/null
@@ -1 +0,0 @@
-Not available at this time.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/julia/build-from-source.md b/docs/static_site/src/_includes/get_started/macos/julia/build-from-source.md
deleted file mode 100644
index b864d8a49086..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/julia/build-from-source.md
+++ /dev/null
@@ -1 +0,0 @@
-Refer to the [Julia section of the MXNet macOS installation guide](get_started/osx_setup.html#install-the-mxnet-package-for-julia).
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/julia/pkg.md b/docs/static_site/src/_includes/get_started/macos/julia/pkg.md
deleted file mode 100644
index 35971305ee2d..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/julia/pkg.md
+++ /dev/null
@@ -1,10 +0,0 @@
-Install a pinned version of MXNet like this:
-
-{% highlight julia %}
-]add MXNet#v1.5.0
-{% endhighlight %}
-
-Or directly install the latest release:
-{% highlight julia %}
-]add MXNet
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/perl/perl.md b/docs/static_site/src/_includes/get_started/macos/perl/perl.md
deleted file mode 100644
index 45d59ddf78a6..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/perl/perl.md
+++ /dev/null
@@ -1 +0,0 @@
-Refer to the [Perl section of installation guide](/get_started/osx_setup.html#install-the-mxnet-package-for-perl).
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/python/cpu/build-from-source.md b/docs/static_site/src/_includes/get_started/macos/python/cpu/build-from-source.md
deleted file mode 100644
index ee8e378ec20e..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/python/cpu/build-from-source.md
+++ /dev/null
@@ -1,2 +0,0 @@
-To build from source, refer to the [MXNet macOS installation guide](/get_started/osx_setup.html).
-MXNet developers should refer to the MXNet wiki's [Developer Setup on Mac](https://cwiki.apache.org/confluence/display/MXNET/MXNet+Developer+Setup+on+Mac).
diff --git a/docs/static_site/src/_includes/get_started/macos/python/cpu/docker.md b/docs/static_site/src/_includes/get_started/macos/python/cpu/docker.md
deleted file mode 100644
index c8631ec26ab3..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/python/cpu/docker.md
+++ /dev/null
@@ -1,35 +0,0 @@
-Docker images with *MXNet* are available at [DockerHub](https://hub.docker.com/r/mxnet/).
-
-**Step 1** Install Docker on your machine by following the [docker installation
-instructions](https://docs.docker.com/docker-for-mac/install/#install-and-run-docker-for-mac).
-
-*Note* - You can install Community Edition (CE) to get started with *MXNet*.
-
-**Step 2** Pull the MXNet docker image.
-
-{% highlight bash %}
-$ docker pull mxnet/python
-{% endhighlight %}
-
-You can list docker images to see if mxnet/python docker image pull was successful.
-
-{% highlight bash %}
-$ docker images
-
-REPOSITORY TAG IMAGE ID CREATED SIZE
-mxnet/python latest 00d026968b3c 3 weeks ago 1.41 GB
-{% endhighlight %}
-
-Using the latest MXNet with [Intel MKL-DNN](https://github.com/intel/mkl-dnn) is
-recommended for the
-fastest inference speeds with MXNet.
-
-{% highlight bash %}
-$ docker pull mxnet/python:1.3.0_cpu_mkl # Use sudo if you skip Step 2
-$ docker images # Use sudo if you skip Step 2
-
-REPOSITORY TAG IMAGE ID CREATED SIZE
-mxnet/python 1.3.0_cpu_mkl deaf9bf61d29 4 days ago 678 MB
-{% endhighlight %}
-
-**Step 4** Validate the installation.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/python/cpu/pip.md b/docs/static_site/src/_includes/get_started/macos/python/cpu/pip.md
deleted file mode 100644
index beb5eb4fb797..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/python/cpu/pip.md
+++ /dev/null
@@ -1,73 +0,0 @@
-Run the following command:
-
-
-
-{% highlight bash %}
-$ pip install mxnet
-{% endhighlight %}
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.4.1
-{% endhighlight %}
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.3.1
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.2.1
-{% endhighlight %}
-
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.1.0
-{% endhighlight %}
-
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.0.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet=0.12.1
-{% endhighlight %}
-
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==0.11.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet --pre
-{% endhighlight %}
-
-
-
-{% include /get_started/pip_snippet.md %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/python/gpu/build-from-source.md b/docs/static_site/src/_includes/get_started/macos/python/gpu/build-from-source.md
deleted file mode 100644
index ffcf88ecdd25..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/python/gpu/build-from-source.md
+++ /dev/null
@@ -1,2 +0,0 @@
-Refer to the [MXNet macOS installation guide](get_started/osx_setup.html).
-MXNet developers should refer to the MXNet wiki's [Developer Setup on Mac](https://cwiki.apache.org/confluence/display/MXNET/MXNet+Developer+Setup+on+Mac).
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/python/gpu/pip_docker.md b/docs/static_site/src/_includes/get_started/macos/python/gpu/pip_docker.md
deleted file mode 100644
index 87281fe85e38..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/python/gpu/pip_docker.md
+++ /dev/null
@@ -1 +0,0 @@
-This option is only available by building from source. Refer to the [MXNet macOS installation guide](get_started/osx_setup.html).
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/r/cpu.md b/docs/static_site/src/_includes/get_started/macos/r/cpu.md
deleted file mode 100644
index 1e0d96b91ed7..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/r/cpu.md
+++ /dev/null
@@ -1,28 +0,0 @@
-To run MXNet you also should have OpenCV and OpenBLAS installed. You may install them with `brew` as follows:
-
-{% highlight bash %}
-brew install opencv
-brew install openblas
-{% endhighlight %}
-
-To ensure MXNet R package runs with the version of OpenBLAS installed, create a symbolic link as follows:
-
-{% highlight bash %}
-ln -sf /usr/local/opt/openblas/lib/libopenblas.dylib
-/usr/local/opt/openblas/lib/libopenblasp-r0.3.1.dylib
-{% endhighlight %}
-
-Note: packages for 3.6.x are not yet available.
-
-Install 3.5.x of R from [CRAN](https://cran.r-project.org/bin/macosx/). The latest is
-[v3.5.3](https://cran.r-project.org/bin/macosx/R-3.5.3.pkg).
-
-You can [build MXNet-R from source](get_started/osx_setup.html#install-the-mxnet-package-for-r), or
-you can use a pre-built binary:
-
-{% highlight r %}
-cran <- getOption("repos")
-cran["dmlc"] <- "https://apache-mxnet.s3-accelerate.dualstack.amazonaws.com/R/CRAN/"
-options(repos = cran)
-install.packages("mxnet")
-{% endhighlight %}
diff --git a/docs/static_site/src/_includes/get_started/macos/r/gpu.md b/docs/static_site/src/_includes/get_started/macos/r/gpu.md
deleted file mode 100644
index 3fc556a8dd91..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/r/gpu.md
+++ /dev/null
@@ -1 +0,0 @@
-Be the first one to contribute this guide!
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/scala/cpu.md b/docs/static_site/src/_includes/get_started/macos/scala/cpu.md
deleted file mode 100644
index 0bc69eb2e63e..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/scala/cpu.md
+++ /dev/null
@@ -1,14 +0,0 @@
-You can use the Maven packages defined in the following dependency to include MXNet in your Scala
-project. Please refer to the [MXNet-Scala setup guide](/get_started/scala_setup.html) for a detailed set
-of instructions to help you with the setup process.
-
-
-
-{% highlight html %}
-
- org.apache.mxnet
- mxnet-full_2.11-osx-x86_64-cpu
-
-{% endhighlight %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos/scala/gpu.md b/docs/static_site/src/_includes/get_started/macos/scala/gpu.md
deleted file mode 100644
index b17ef33478ea..000000000000
--- a/docs/static_site/src/_includes/get_started/macos/scala/gpu.md
+++ /dev/null
@@ -1 +0,0 @@
-Not available at this time.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/macos~b6b40878f... Consolidate installation instructions on website and add disclaimer for non-ASF ressources (#18487) b/docs/static_site/src/_includes/get_started/macos~b6b40878f... Consolidate installation instructions on website and add disclaimer for non-ASF ressources (#18487)
new file mode 120000
index 000000000000..9c52cb36f47e
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/macos~b6b40878f... Consolidate installation instructions on website and add disclaimer for non-ASF ressources (#18487)
@@ -0,0 +1 @@
+linux
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/pip_snippet.md b/docs/static_site/src/_includes/get_started/pip_snippet.md
index 2c4d932fc816..96ef52250a03 100644
--- a/docs/static_site/src/_includes/get_started/pip_snippet.md
+++ b/docs/static_site/src/_includes/get_started/pip_snippet.md
@@ -1,6 +1,4 @@
-MXNet offers MKL pip packages that will be much faster when running on Intel hardware.
-Check the chart below for other options, refer to PyPI for
-other MXNet pip packages, or validate your MXNet installation.
+You can then validate your MXNet installation.
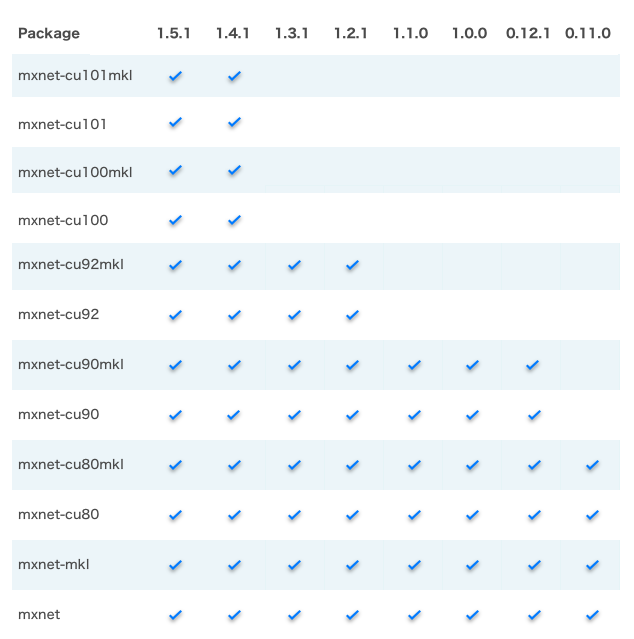
, or
vali
**NOTES:**
-*mxnet-cu101mkl* means the package is built with CUDA/cuDNN and MKL-DNN enabled and the CUDA version is 10.1.
+*mxnet-cu101* means the package is built with CUDA/cuDNN and the CUDA version is
+10.1.
All MKL pip packages are experimental prior to version 1.3.0.
diff --git a/docs/static_site/src/_includes/get_started/windows b/docs/static_site/src/_includes/get_started/windows
new file mode 120000
index 000000000000..9c52cb36f47e
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/windows
@@ -0,0 +1 @@
+linux
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/clojure/clojure.md b/docs/static_site/src/_includes/get_started/windows/clojure/clojure.md
deleted file mode 100644
index 0b25ab9018d3..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/clojure/clojure.md
+++ /dev/null
@@ -1 +0,0 @@
-MXNet-Clojure for Windows is not yet available.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/cpp/cpp.md b/docs/static_site/src/_includes/get_started/windows/cpp/cpp.md
deleted file mode 100644
index 023735b6c3b1..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/cpp/cpp.md
+++ /dev/null
@@ -1,3 +0,0 @@
-To enable the C++ package, build from source using `make USE_CPP_PACKAGE=1`.
-Refer to the [MXNet C++ setup guide](/get_started/cpp_setup.html) for full instructions.
-
diff --git a/docs/static_site/src/_includes/get_started/windows/java/java.md b/docs/static_site/src/_includes/get_started/windows/java/java.md
deleted file mode 100644
index 0db1f50590a2..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/java/java.md
+++ /dev/null
@@ -1 +0,0 @@
-MXNet-Java for Windows is not yet available.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/julia/build-from-source.md b/docs/static_site/src/_includes/get_started/windows/julia/build-from-source.md
deleted file mode 100644
index 4fc600468ad1..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/julia/build-from-source.md
+++ /dev/null
@@ -1 +0,0 @@
-Refer to the [Julia section of the MXNet Windows installation guide](/get_started/windows_setup.html#install-the-mxnet-package-for-julia).
diff --git a/docs/static_site/src/_includes/get_started/windows/julia/pkg.md b/docs/static_site/src/_includes/get_started/windows/julia/pkg.md
deleted file mode 100644
index cb79177e5bbe..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/julia/pkg.md
+++ /dev/null
@@ -1,10 +0,0 @@
-Install a pinned version of MXNet like this:
-
-{% highlight julia %}
-]add MXNet#v1.5.0
-{% endhighlight %}
-
-Or directly install the latest release:
-{% highlight julia %}
-]add MXNet
-{% endhighlight %}
diff --git a/docs/static_site/src/_includes/get_started/windows/perl/perl.md b/docs/static_site/src/_includes/get_started/windows/perl/perl.md
deleted file mode 100644
index 1a8eea5261ba..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/perl/perl.md
+++ /dev/null
@@ -1 +0,0 @@
-Refer to the [Perl section of the MXNet Windows installation guide](/get_started/windows_setup.html#install-the-mxnet-package-for-perl).
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/python/cpu/build-from-source.md b/docs/static_site/src/_includes/get_started/windows/python/cpu/build-from-source.md
deleted file mode 100644
index af36205337d2..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/python/cpu/build-from-source.md
+++ /dev/null
@@ -1 +0,0 @@
-Refer to the [MXNet Windows installation guide](/get_started/windows_setup.html)
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/python/cpu/docker.md b/docs/static_site/src/_includes/get_started/windows/python/cpu/docker.md
deleted file mode 100644
index a061b8bbab65..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/python/cpu/docker.md
+++ /dev/null
@@ -1,34 +0,0 @@
-Docker images with *MXNet* are available at [Docker Hub](https://hub.docker.com/r/mxnet/).
-
-**Step 1** Install Docker on your machine by following the docker installation instructions
-
-*Note* - You can install Community Edition (CE) to get started with *MXNet*.
-
-**Step 2** Pull the MXNet docker image.
-
-{% highlight bash %}
-$ docker pull mxnet/python # Use sudo if you skip Step 2
-{% endhighlight %}
-
-You can list docker images to see if mxnet/python docker image pull was successful.
-
-{% highlight bash %}
-$ docker images # Use sudo if you skip Step 2
-
-REPOSITORY TAG IMAGE ID CREATED SIZE
-mxnet/python latest 00d026968b3c 3 weeks ago 1.41 GB
-{% endhighlight %}
-
-Using the latest MXNet with [Intel MKL-DNN](https://github.com/intel/mkl-dnn) is
-recommended for the
-fastest inference speeds with MXNet.
-
-{% highlight bash %}
-$ docker pull mxnet/python:1.3.0_cpu_mkl # Use sudo if you skip Step 2
-$ docker images # Use sudo if you skip Step 2
-
-REPOSITORY TAG IMAGE ID CREATED SIZE
-mxnet/python 1.3.0_cpu_mkl deaf9bf61d29 4 days ago 678 MB
-{% endhighlight %}
-
-**Step 4** Validate the installation.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/python/cpu/pip.md b/docs/static_site/src/_includes/get_started/windows/python/cpu/pip.md
deleted file mode 100644
index d5c7f1fd08f0..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/python/cpu/pip.md
+++ /dev/null
@@ -1,73 +0,0 @@
-Run the following command:
-
-
-
-{% highlight bash %}
-$ pip install mxnet
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.4.1
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.3.1
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.2.1
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.1.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==1.0.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==0.12.1
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet==0.11.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet --pre
-{% endhighlight %}
-
-
-
-{% include /get_started/pip_snippet.md %}
-{% include /get_started/gpu_snippet.md %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/python/gpu/build-from-source.md b/docs/static_site/src/_includes/get_started/windows/python/gpu/build-from-source.md
deleted file mode 100644
index 55bca3a129d8..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/python/gpu/build-from-source.md
+++ /dev/null
@@ -1 +0,0 @@
-To build from source, refer to the [MXNet Windows installation guide](/get_started/windows_setup.html).
diff --git a/docs/static_site/src/_includes/get_started/windows/python/gpu/pip.md b/docs/static_site/src/_includes/get_started/windows/python/gpu/pip.md
deleted file mode 100644
index cbcd9d44d6af..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/python/gpu/pip.md
+++ /dev/null
@@ -1,74 +0,0 @@
-Run the following command:
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu101
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu101==1.4.1
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu92==1.3.1
-{% endhighlight %}
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu92==1.2.1
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu91==1.1.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu90==1.0.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu90==0.12.1
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu80==0.11.0
-{% endhighlight %}
-
-
-
-
-
-{% highlight bash %}
-$ pip install mxnet-cu101 --pre
-{% endhighlight %}
-
-
-
-
-{% include /get_started/pip_snippet.md %}
-{% include /get_started/gpu_snippet.md %}
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/r/cpu.md b/docs/static_site/src/_includes/get_started/windows/r/cpu.md
deleted file mode 100644
index 926b8355c984..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/r/cpu.md
+++ /dev/null
@@ -1,15 +0,0 @@
-Note: packages for 3.6.x are not yet available.
-Install 3.5.x of R from [CRAN](https://cran.r-project.org/bin/windows/base/old/).
-
-You can [build MXNet-R from source](/get_started/windows_setup.html#install-mxnet-package-for-r), or
-you can use a
-pre-built binary:
-
-{% highlight r %}
-cran <- getOption("repos")
-cran["dmlc"] <- "https://apache-mxnet.s3-accelerate.dualstack.amazonaws.com/R/CRAN/"
-options(repos = cran)
-install.packages("mxnet")
-{% endhighlight %}
-
-To run MXNet you also should have OpenCV and OpenBLAS installed.
diff --git a/docs/static_site/src/_includes/get_started/windows/r/gpu.md b/docs/static_site/src/_includes/get_started/windows/r/gpu.md
deleted file mode 100644
index 084f1a5a4012..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/r/gpu.md
+++ /dev/null
@@ -1,16 +0,0 @@
-You can [build MXNet-R from source](/get_started/windows_setup.html#install-mxnet-package-for-r), or
-you can use a
-pre-built binary:
-
-{% highlight r %}
-cran <- getOption("repos")
-cran["dmlc"] <-
-"https://apache-mxnet.s3-accelerate.dualstack.amazonaws.com/R/CRAN/GPU/cu92"
-options(repos = cran)
-install.packages("mxnet")
-{% endhighlight %}
-
-Change cu92 to cu90, cu91 or cuda100 based on your CUDA toolkit version. Currently, MXNet supports these versions of CUDA.
-Note : You also need to have cuDNN installed on Windows. Check out this
-[guide](https://docs.nvidia.com/deeplearning/sdk/cudnn-install/index.html#installwindows)
-on the steps for installation.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows/scala/scala.md b/docs/static_site/src/_includes/get_started/windows/scala/scala.md
deleted file mode 100644
index 74b7d45c6d79..000000000000
--- a/docs/static_site/src/_includes/get_started/windows/scala/scala.md
+++ /dev/null
@@ -1 +0,0 @@
-MXNet-Scala for Windows is not yet available.
\ No newline at end of file
diff --git a/docs/static_site/src/_includes/get_started/windows~b6b40878f... Consolidate installation instructions on website and add disclaimer for non-ASF ressources (#18487) b/docs/static_site/src/_includes/get_started/windows~b6b40878f... Consolidate installation instructions on website and add disclaimer for non-ASF ressources (#18487)
new file mode 120000
index 000000000000..9c52cb36f47e
--- /dev/null
+++ b/docs/static_site/src/_includes/get_started/windows~b6b40878f... Consolidate installation instructions on website and add disclaimer for non-ASF ressources (#18487)
@@ -0,0 +1 @@
+linux
\ No newline at end of file
diff --git a/docs/static_site/src/pages/get_started/build_from_source.md b/docs/static_site/src/pages/get_started/build_from_source.md
index 1dfa95a82ade..19f2f1a313ca 100644
--- a/docs/static_site/src/pages/get_started/build_from_source.md
+++ b/docs/static_site/src/pages/get_started/build_from_source.md
@@ -25,307 +25,381 @@ permalink: /get_started/build_from_source
# Build MXNet from Source
-This document explains how to build MXNet from source code.
-
-**For Java/Scala/Clojure, please follow [this guide instead](scala_setup)**
-
-## Overview
-
-Building from source follows this general two-step flow of building the shared library, then installing your preferred language binding. Use the following links to jump to the different sections of this guide.
-
-1. Build the MXNet shared library, `libmxnet.so`.
- * [Clone the repository](#clone-the-mxnet-project)
- * [Prerequisites](#prerequisites)
- * [Math library selection](#math-library-selection)
- * [Install GPU software](#install-gpu-software)
- * [Install optional software](#install-optional-software)
- * [Adjust your build configuration](#build-configurations)
- * [Build MXNet](#build-mxnet)
- * [with NCCL](#build-mxnet-with-nccl) (optional)
- * [for C++](#build-mxnet-with-c++) (optional)
- * [Usage Examples](#usage-examples)
- * [systems with GPUs and Intel CPUs](#recommended-for-Systems-with-NVIDIA-GPUs-and-Intel-CPUs)
- * [GPUs with non-Intel CPUs](#recommended-for-Systems-with-Intel-CPUs)
- * [Intel CPUs](#recommended-for-Systems-with-Intel-CPUs)
- * [non-Intel CPUs](#recommended-for-Systems-with-non-Intel-CPUs)
-2. [Install the language API binding(s)](#installing-mxnet-language-bindings) you would like to use for MXNet.
-MXNet's newest and most popular API is Gluon. Gluon is built into the Python binding. If Python isn't your preference, you still have more options. MXNet supports several other language APIs:
- - [Python (includes Gluon)]({{'/api/python/docs/api/index.html'|relative_url}})
- - [C++]({{'/api/cpp'|relative_url}})
- - [Clojure]({{'/api/clojure'|relative_url}})
- - [Java]({{'/api/java'|relative_url}})
- - [Julia]({{'/api/julia'|relative_url}})
- - [Perl]({{'/api/perl'|relative_url}})
- - [R]({{'/api/r'|relative_url}})
- - [Scala]({{'/api/scala'|relative_url}})
+Building and installing MXNet from source is a three-step process. First, build
+the shared `libmxnet` which provides the MXNet backend, then install your
+preferred language binding and finally validate that MXNet was installed
+correctly by running a small example.
-
+1. [Obtaining the source](#obtaining-the-source-code)
+2. [Installing MXNet's recommended dependencies](#installing-mxnet's-recommended-dependencies)
+3. [Overview of optional dependencies and optional features](#overview-of-optional-dependencies-and-optional-features)
+4. [Building MXNet](#building-mxnet)
+5. [Install the language API binding(s)](#installing-mxnet-language-bindings) you would like to use for MXNet.
-## Build Instructions by Operating System
+MXNet's newest and most popular API is Gluon. Gluon is built into the Python
+binding. If Python isn't your preference, you still have more options. MXNet
+supports several other language bindings. Please see the [API Documentation
+page](/api) for an overview of all supported languages and their APIs.
-Detailed instructions are provided per operating system. Each of these guides also covers how to install the specific [Language Bindings](#installing-mxnet-language-bindings) you require.
-You may jump to those, but it is recommended that you continue reading to understand more general "build from source" options.
-* [Amazon Linux / CentOS / RHEL](centos_setup)
-* [macOS](osx_setup)
-* [Devices](index.html?&platform=devices&language=python&environ=pip&processor=cpu)
-* [Ubuntu](ubuntu_setup)
-* [Windows](windows_setup)
+## Obtaining the source code
+To obtain the source code of the latest Apache MXNet (incubating) release,
+please access the [Download page](/get_started/download) and download the
+`.tar.gz` source archive corresponding to the release you wish to build.
-
+Developers can also obtain the unreleased development code from the git
+repository via `git clone --recursive https://github.com/apache/incubator-mxnet mxnet`
+
+Building a MXNet 1.x release from source requires a C++11 compliant compiler.
+
+Building the development version of MXNet or any 2.x release from source
+requires a C++17 compliant compiler. The oldest compiler versions tested during
+MXNet 2 development are GCC 7, Clang 6 and MSVC 2019.
-## Clone the MXNet Project
+## Installing MXNet's recommended dependencies
+To install the build tools and recommended dependencies, please run the
+following commands respectively based on your Operating System. Please see the
+next section for further explanations on the set of required and optional
+dependencies of MXNet.
-1. Clone or fork the MXNet project.
+### Debian Linux derivatives (Debian, Ubuntu, ...)
```bash
git clone --recursive https://github.com/apache/incubator-mxnet mxnet
cd mxnet
+sudo apt-get update
+sudo apt-get install -y build-essential git ninja-build ccache libopenblas-dev libopencv-dev cmake
```
+### Red Hat Enterprise Linux derivatives (RHEL, CentOS, Fedora, ...)
+```bash
+sudo yum install epel-release centos-release-scl
+sudo yum install git make ninja-build automake autoconf libtool protobuf-compiler protobuf-devel \
+ atlas-devel openblas-devel lapack-devel opencv-devel openssl-devel zeromq-devel python3 \
+ devtoolset-7
+source /opt/rh/devtoolset-7/enable
+```
+Here `devtoolset-7` refers to the [Developer Toolset
+7](https://www.softwarecollections.org/en/scls/rhscl/devtoolset-7/) created by
+Red Hat for developers working on CentOS or Red Hat Enterprise Linux platform
+and providing the GNU Compiler Collection 7.
+
+### macOS
+```bash
+# Install OS X Developer Tools
+xcode-select --install
-## Prerequisites
+# Install Homebrew
+/usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
+
+# Install dependencies
+brew install cmake ninja ccache opencv
+```
+
+Note: the compiler provided by Apple on macOS does not support OpenMP. To use
+OpenMP on macOS you need to install for example the Clang compiler via `brew`:
+
+```bash
+brew install llvm
+```
-The following sections will help you decide which specific prerequisites you need to install.
+### Windows
+You can use Chocolatey software management solution to install some dependencies
+on Windows.
-#### Math Library Selection
-It is useful to consider your math library selection prior to your other prerequisites.
+```bash
+choco install python git 7zip cmake ninja opencv
+```
+
+Currently OpenBLAS is not available from Chocolatey. You may download it from
+from [the OpenBLAS release page](https://github.com/xianyi/OpenBLAS/releases)
+and compile from source. Set the `OpenBLAS_HOME` environment variable to point
+to the OpenBLAS directory that contains the `include` and `lib` directories for
+example by typing `set OpenBLAS_HOME=C:\utils\OpenBLAS`.
+
+If you like to compile MXNet with Visual Studio compiler, please install at
+least [VS2019](https://www.visualstudio.com/downloads/).
+
+## Overview of optional dependencies and optional features
+
+### Math Library Selection
MXNet relies on the
[BLAS](https://en.wikipedia.org/wiki/Basic_Linear_Algebra_Subprograms) (Basic
-Linear Algebra Subprograms) library for numerical computations.
-Those can be extended with [LAPACK (Linear Algebra Package)](https://github.com/Reference-LAPACK/lapack), an additional set of mathematical functions.
+Linear Algebra Subprograms) library for numerical computations. In addition to
+BLAS, some operators in MXNet rely on the [LAPACK (Linear Algebra
+Package)](https://github.com/Reference-LAPACK/lapack), an additional set of
+mathematical functions.
+
+Several BLAS and LAPACK implementations exist. Among them, MXNet is tested with:
-MXNet supports multiple mathematical backends for computations on the CPU:
* [Apple Accelerate](https://developer.apple.com/documentation/accelerate)
* [ATLAS](http://math-atlas.sourceforge.net/)
-* [MKL](https://software.intel.com/en-us/intel-mkl) (MKL, MKLML)
-* [MKL-DNN](https://github.com/intel/mkl-dnn)
+* [Intel MKL](https://software.intel.com/en-us/intel-mkl)
* [OpenBLAS](https://www.openblas.net/)
-The default order of choice for the libraries if found follows the path from the most
-(recommended) to less performant backends.
-The following lists show this order by library and `cmake` switch.
+Apple Accelerate and MKL are proprietary. ATLAS and OpenBLAS are Open Source. If
+you don't have any specific requirements, MXNet recommends OpenBLAS as it
+typically outperforms ATLAS, is portable across many platforms, provides a
+LAPACK implementation and has a permissive license.
-For desktop platforms (x86_64):
+### Optional GPU support
-1. MKL-DNN (submodule) | `USE_MKLDNN`
-2. MKL | `USE_MKL_IF_AVAILABLE`
-3. MKLML (downloaded) | `USE_MKLML`
-4. Apple Accelerate | `USE_APPLE_ACCELERATE_IF_AVAILABLE` | Mac only
-5. OpenBLAS | `BLAS` | Options: Atlas, Open, MKL, Apple
+MXNet optionally supports [NVDIA CUDA and
+cuDNN](https://developer.nvidia.com/cuda-downloads) for better performance on
+NVidia devices. MXNet releases in general are tested with the last two major
+CUDA versions available at the time of the release. For example, CUDA 9.2 and
+10.2.
-Note: If `USE_MKL_IF_AVAILABLE` is set to False then MKLML and MKL-DNN will be disabled as well for configuration
-backwards compatibility.
+To compile MXNet with CUDA support, define the `USE_CUDA` option. If you compile
+MXNet on a system with NVidia GPUs, the build system will automatically detect
+the CUDA Architecture. If you are compiling on a system without NVidia GPUs,
+please specify the `MXNET_CUDA_ARCH` option to select the CUDA Architecture and
+avoid a lengthy build targeting all common CUDA Architectures. Please see the
+MXNet build configuration instructions in the next step.
-For embedded platforms (all other and if cross compiled):
+MXNet also supports [NCCL](https://developer.nvidia.com/nccl) - NVIDIA's
+Collective Communications Library. NCCL is useful when using MXNet on multiple
+GPUs that require communication. Instructions for installing NCCL are found in
+the following [Build MXNet with NCCL](#build-mxnet-with-nccl) section.
-1. OpenBLAS | `BLAS` | Options: Atlas, Open, MKL, Apple
+To enable building MXNet with NCCL, install NCCL and define the `USE_NCCL`
+option in the MXNet build configuration in the next step.
-You can set the BLAS library explicitly by setting the BLAS variable to:
+After building with NCCL, you may optionally use the tests in
+`tests/python/gpu/test_nccl.py` to ensure NCCL is enabled correctly. Please
+first delete the line containing `skip(reason="Test requires NCCL library
+installed and enabled during build")` before running the test. In MXNet 2.x
+versions, the test can be run via `pytest --verbose
+tests/python/gpu/test_nccl.py`. In MXNet 1.x it is run via `python
+tests/python/gpu/test_nccl.py`.
-* Atlas
-* Open
-* MKL
-* Apple
+To get the best performance out of NCCL it is recommended to set environment
+variable `NCCL_LAUNCH_MODE=PARALLEL` when using NCCL version 2.1 or newer.
-See the [cmake/ChooseBLAS.cmake](https://github.com/apache/incubator-mxnet/blob/master/cmake/ChooseBlas.cmake) file for the options.
+### Optional OpenCV support
-[Intel's MKL (Math Kernel Library)](https://software.intel.com/en-us/mkl) is one of the most powerful math libraries
+MXNet's Image Loading and Augmentation features rely on
+[OpenCV](http://opencv.org/). Image Loading and Augmentation
-It has following flavors:
+## Building MXNet
-* MKL is a complete math library, containing all the functionality found in ATLAS, OpenBlas and LAPACK. It is free under
-community support licensing (https://software.intel.com/en-us/articles/free-mkl),
-but needs to be downloaded and installed manually.
+MXNet 1.x can be built either with a classic Makefile setup or with the `cmake`
+cross platform build system. Starting with MXNet 1.7, MXNet recommends using the
+`cmake` cross platform build tool.
-* MKLML is a subset of MKL. It contains a smaller number of functions to reduce the
-size of the download and reduce the number of dynamic libraries user needs.
+Note: The `cmake` build requires CMake 3.13 or higher. If you are running an
+older version of CMake, you will see an error message like `CMake 3.13 or higher
+is required. You are running version 3.10.2`. Please update CMake on your
+system. You can download and install latest CMake from https://cmake.org or via
+the Python package manager `pip` with `python3 -m pip install --user --upgrade
+"cmake>=3.13.2"`. After installing cmake with `pip3`, it is usually available at
+`~/.local/bin/cmake` or directly as `cmake`.
-
+Please see the [`cmake configuration
+files`](https://github.com/apache/incubator-mxnet/tree/v1.x/config) files for
+instructions on how to configure and build MXNet with cmake.
-* MKL-DNN is a separate open-source library, it can be used separately from MKL or MKLML. It is
-shipped as a subrepo with MXNet source code (see 3rdparty/mkldnn or the [MKL-DNN project](https://github.com/intel/mkl-dnn))
+Up to the MXNet 1.6 release, please follow the instructions in the
+[`make/config.mk`](https://github.com/apache/incubator-mxnet/blob/v1.x/make/config.mk)
+file on how to configure and compile MXNet. This method is supported on all 1.x
+releases.
-Since the full MKL library is almost always faster than any other BLAS library it's turned on by default,
-however it needs to be downloaded and installed manually before doing `cmake` configuration.
-Register and download on the [Intel performance libraries website](https://software.intel.com/en-us/performance-libraries).
-You can also install MKL through [YUM](https://software.intel.com/en-us/articles/installing-intel-free-libs-and-python-yum-repo)
-or [APT](https://software.intel.com/en-us/articles/installing-intel-free-libs-and-python-apt-repo) Repository.
+To enable the optional MXNet C++ package, please set the `USE_CPP_PACKAGE=1`
+option prior to compiling. See the [C++ guide](cpp_setup) for more information.
-Note: MKL is supported only for desktop builds and the framework itself supports the following
-hardware:
-* Intel® Xeon Phi™ processor
-* Intel® Xeon® processor
-* Intel® Core™ processor family
-* Intel Atom® processor
+## Installing MXNet Language Bindings
+After building MXNet's shared library, you can install other language bindings.
-If you have a different processor you can still try to use MKL, but performance results are
-unpredictable.
+**NOTE:** The C++ API binding must be built when you build MXNet from source. See [Build MXNet with C++]({{'/api/cpp.html'|relative_url}}).
+## Installing Language Packages for MXNet
-#### Install GPU Software
+After you have installed the MXNet core library. You may install MXNet interface
+packages for the programming language of your choice:
+- [Python](#install-mxnet-for-python)
+- [C++](#install-the-mxnet-package-for-c++)
+- [Clojure](#install-the-mxnet-package-for-clojure)
+- [Julia](#install-the-mxnet-package-for-julia)
+- [Perl](#install-the-mxnet-package-for-perl)
+- [R](#install-the-mxnet-package-for-r)
+- [Scala](#install-the-mxnet-package-for-scala)
+- [Java](#install-the-mxnet-package-for-java)
-If you want to run MXNet with GPUs, you must install [NVDIA CUDA and cuDNN](https://developer.nvidia.com/cuda-downloads).
+### Install MXNet for Python
-#### Install Optional Software
+To install the MXNet Python binding navigate to the root of the MXNet folder then run the following:
-These might be optional, but they're typically desirable as the extend or enhance MXNet's functionality.
+```bash
+python3 -m pip install --user -e ./python
+```
-* [OpenCV](http://opencv.org/) - Image Loading and Augmentation. Each operating system has different packages and build from source options for OpenCV. Refer to your OS's link in the [Build Instructions by Operating System](#build-instructions-by-operating-system) section for further instructions.
-* [NCCL](https://developer.nvidia.com/nccl) - NVIDIA's Collective Communications Library. Instructions for installing NCCL are found in the following [Build MXNet with NCCL](#build-mxnet-with-nccl) section.
+Note that the `-e` flag is optional. It is equivalent to `--editable` and means
+that if you edit the source files, these changes will be reflected in the
+package installed.
-More information on turning these features on or off are found in the following [build configurations](#build-configurations) section.
+You may optionally install ```graphviz``` library that is used for visualizing
+network graphs you build on MXNet. You may also install [Jupyter
+Notebook](http://jupyter.readthedocs.io/) which is used for running MXNet
+tutorials and examples.
+```bash
+python3 -m pip install --user graphviz==0.8.4 jupyter
+```
-
+Please also see the [MXNet Python API](/api/python) page.
-## Build Configurations
+### Install the MXNet Package for C++
-There is a configuration file for make,
-[`make/config.mk`](https://github.com/apache/incubator-mxnet/blob/master/make/config.mk), that contains all the compilation options. You can edit it and then run `make` or `cmake`. `cmake` is recommended for building MXNet (and is required to build with MKLDNN), however you may use `make` instead. For building with Java/Scala/Clojure, only `make` is supported.
+To enable C++ package, just add `USE_CPP_PACKAGE=1` as build option when
+building the MXNet shared library following the instructions from the previous
+section.
-**NOTE:** When certain set of build flags are set, MXNet archive increases to more than 4 GB. Since MXNet uses archive internally archive runs into a bug ("File Truncated": [bugreport](https://sourceware.org/bugzilla/show_bug.cgi?id=14625)) for archives greater than 4 GB. Please use ar version 2.27 or greater to overcome this bug. Please see https://github.com/apache/incubator-mxnet/issues/15084 for more details.
+You can find C++ code examples in the `cpp-package/example` folder of the MXNet
+project. The folder contains a README explaining how to build the examples. The
+`predict-cpp` explains Image Classification using MXNet's C Predict API.
-
+Please also see the [MXNet C++ API](/api/cpp) page.
-## Build MXNet
-
-### Build MXNet with NCCL
-- Download and install the latest NCCL library from NVIDIA.
-- Note the directory path in which NCCL libraries and header files are installed.
-- Ensure that the installation directory contains ```lib``` and ```include``` folders.
-- Ensure that the prerequisites for using NCCL such as Cuda libraries are met.
-- Append the ```config.mk``` file with following, in addition to the CUDA related options.
-- USE_NCCL=1
-- USE_NCCL_PATH=path-to-nccl-installation-folder
-
-``` bash
-echo "USE_NCCL=1" >> make/config.mk
-echo "USE_NCCL_PATH=path-to-nccl-installation-folder" >> make/config.mk
-cp make/config.mk .
-```
-- Run make command
-``` bash
-make -j"$(nproc)"
-```
-#### Validating NCCL
-- Follow the steps to install MXNet Python binding.
-- Comment the following line in ```test_nccl.py``` file at ```incubator-mxnet/tests/python/gpu/test_nccl.py```
-``` bash
-@unittest.skip("Test requires NCCL library installed and enabled during build")
-```
-- Run test_nccl.py script as follows. The test should complete. It does not produce any output.
-``` bash
-nosetests --verbose tests/python/gpu/test_nccl.py
-```
+### Install the MXNet Package for Clojure
-**Recommendation to get the best performance out of NCCL:**
-It is recommended to set environment variable NCCL_LAUNCH_MODE to PARALLEL when using NCCL version 2.1 or newer.
+Refer to the [Clojure setup
+guide](https://github.com/apache/incubator-mxnet/tree/master/contrib/clojure-package).
-
+Please also see the [MXNet Clojure API](/api/clojure) page.
-### Build MXNet with C++
+### Install the MXNet Package for Julia
-* To enable C++ package, just add `USE_CPP_PACKAGE=1` when you run `make` or `cmake` (see examples).
+Make sure to install at least Julia 1.0.3.
-
+To use the Julia binding you need to set the `MXNET_HOME` and `LD_LIBRARY_PATH`
+environment variables. For example,
-### Usage Examples
+```bash
+export MXNET_HOME=$HOME/incubator-mxnet
+export LD_LIBRARY_PATH=$HOME/incubator-mxnet/build:$LD_LIBRARY_PATH
+```
-For example, you can specify using all cores on Linux as follows:
+Then install MXNet with Julia:
```bash
-mkdir build && cd build
-cmake -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+julia --color=yes --project=./ -e \
+ 'using Pkg; \
+ Pkg.develop(PackageSpec(name="MXNet", path = joinpath(ENV["MXNET_HOME"], "julia")))'
```
+Please also see the [MXNet Julia API](/api/julia) page.
+
-#### Recommended for Systems with NVIDIA GPUs and Intel CPUs
-* Build MXNet with `cmake` and install with MKL DNN, GPU, and OpenCV support:
+### Install the MXNet Package for Perl
+#### Installing perl package dependencies on Debian Linux derivatives (Debian, Ubuntu, ...)
+
+```
+sudo apt-get install libmouse-perl pdl cpanminus swig libgraphviz-perl
+cpanm -q -L "${HOME}/perl5" Function::Parameters Hash::Ordered PDL::CCS
+```
+
+#### Installing perl package dependencies on macOS
```bash
-mkdir build && cd build
-cmake -DUSE_CUDA=1 -DUSE_CUDA_PATH=/usr/local/cuda -DUSE_CUDNN=1 -DUSE_MKLDNN=1 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+brew install swig
+sudo sh -c 'curl -L https://cpanmin.us | perl - App::cpanminus'
+sudo cpanm -q -n PDL Mouse Function::Parameters Hash::Ordered PDL::CCS
```
-#### Recommended for Systems with NVIDIA GPUs
-* Build with both OpenBLAS, GPU, and OpenCV support:
+#### Install the MXNet Package for Perl
+After you build the shared library, run the following command from the MXNet
+source root directory to build the MXNet Perl package:
```bash
-mkdir build && cd build
-cmake -DBLAS=open -DUSE_CUDA=1 -DUSE_CUDA_PATH=/usr/local/cuda -DUSE_CUDNN=1 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+MXNET_HOME=${PWD}
+export LD_LIBRARY_PATH=${MXNET_HOME}/lib
+export PERL5LIB=${HOME}/perl5/lib/perl5
+
+cd ${MXNET_HOME}/perl-package/AI-MXNetCAPI/
+perl Makefile.PL INSTALL_BASE=${HOME}/perl5
+make install
+
+cd ${MXNET_HOME}/perl-package/AI-NNVMCAPI/
+perl Makefile.PL INSTALL_BASE=${HOME}/perl5
+make install
+
+cd ${MXNET_HOME}/perl-package/AI-MXNet/
+perl Makefile.PL INSTALL_BASE=${HOME}/perl5
+make install
```
-#### Recommended for Systems with Intel CPUs
-* Build MXNet with `cmake` and install with MKL DNN, and OpenCV support:
+Please also see the [MXNet Perl API](/api/perl) page.
+
+### Install the MXNet Package for R
+
+To install R and the devtools, run
```bash
-mkdir build && cd build
-cmake -DUSE_CUDA=0 -DUSE_MKLDNN=1 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+sudo apt-get update
+sudo apt-get install -y r-base-core r-cran-devtools libcairo2-dev libxml2-dev
```
-#### Recommended for Systems with non-Intel CPUs
-* Build MXNet with `cmake` and install with OpenBLAS and OpenCV support:
+`libxml2-dev` is required for the `roxygen2` dependency and `libcairo2-dev` is
+required for the suggested `imager` dependency.
+
+To generate documentation, it is also required to install `roxygen2`.
```bash
-mkdir build && cd build
-cmake -DUSE_CUDA=0 -DBLAS=open -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+R
+> install.packages("roxygen2")
+> Would you like to use a personal library instead? (y/n) y
+> Would you like to create a personal library ... to install packages into? (y/n) y
```
-#### Other Examples
+Note: To successfully complete the next step, you need a personal R library. If
+you were able to run `install.packages("roxygen2")` above, you either had
+already, or you have successfully created a personal library just now.
-* Build without using OpenCV:
+To build and install the MXNet-R bindings, run:
```bash
-mkdir build && cd build
-cmake -DUSE_OPENCV=0 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+make -f R-package/Makefile rpkg
```
-* Build on **macOS** with the default BLAS library (Apple Accelerate) and Clang installed with `xcode` (OPENMP is disabled because it is not supported by the Apple version of Clang):
+Please also see the [MXNet R API](/api/r) page.
+
+### Install the MXNet Package for Scala
+
+After building the MXNet shared library, you may simply run the following from
+the MXNet scala-package folder:
```bash
-mkdir build && cd build
-cmake -DBLAS=apple -DUSE_OPENCV=0 -DUSE_OPENMP=0 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+mvn install
```
-* To use OpenMP on **macOS** you need to install the Clang compiler, `llvm` (the one provided by Apple does not support OpenMP):
+This will install both the Java Inference API and the required MXNet-Scala package.
+
+Please also see the [MXNet Scala API](/api/scala) page.
+
+### Install the MXNet Package for Java
+
+After building the MXNet shared library, you may simply run the following from
+the MXNet scala-package folder:
```bash
-brew install llvm
-mkdir build && cd build
-cmake -DBLAS=apple -DUSE_OPENMP=1 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
+mvn install
```
-
+This will install both the Java Inference API and the required MXNet-Scala package.
-## Installing MXNet Language Bindings
-After building MXNet's shared library, you can install other language bindings.
+Please also see the [MXNet Java API](/api/java) page.
-**NOTE:** The C++ API binding must be built when you build MXNet from source. See [Build MXNet with C++]({{'/api/cpp'|relative_url}}).
+## Contributions
-The following table provides links to each language binding by operating system:
+You are more than welcome to contribute easy installation scripts for other operating systems and programming languages.
+See the [community contributions page]({{'/community/contribute'|relative_url}}) for further information.
-| Language | [Ubuntu](ubuntu_setup) | [macOS](osx_setup) | [Windows](windows_setup) |
-| --- | ---- | --- | ------- |
-| Python | [Ubuntu guide](ubuntu_setup.html#install-mxnet-for-python) | [OSX guide](osx_setup) | [Windows guide](windows_setup.html#install-mxnet-for-python) |
-| C++ | [C++ guide](cpp_setup) | [C++ guide](cpp_setup) | [C++ guide](cpp_setup) |
-| Clojure | [Clojure guide](https://github.com/apache/incubator-mxnet/tree/master/contrib/clojure-package) | [Clojure guide](https://github.com/apache/incubator-mxnet/tree/master/contrib/clojure-package) | n/a |
-| Julia | [Ubuntu guide](ubuntu_setup.html#install-the-mxnet-package-for-julia) | [OSX guide](osx_setup.html#install-the-mxnet-package-for-julia) | [Windows guide](windows_setup.html#install-the-mxnet-package-for-julia) |
-| Perl | [Ubuntu guide](ubuntu_setup.html#install-the-mxnet-package-for-perl) | [OSX guide](osx_setup.html#install-the-mxnet-package-for-perl) | n/a |
-| R | [Ubuntu guide](ubuntu_setup.html#install-the-mxnet-package-for-r) | [OSX guide](osx_setup.html#install-the-mxnet-package-for-r) | [Windows guide](windows_setup.html#install-the-mxnet-package-for-r) |
-| Scala | [Scala guide](scala_setup.html) | [Scala guide](scala_setup.html) | n/a |
-| Java | [Java guide](java_setup.html) | [Java Guide](java_setup.html) | n/a |
+## Next Steps
+* [Tutorials]({{'/api'|relative_url}})
+* [How To]({{'/api/faq/add_op_in_backend'|relative_url}})
+* [Architecture]({{'/api/architecture/overview'|relative_url}})
diff --git a/docs/static_site/src/pages/get_started/c_plus_plus.md b/docs/static_site/src/pages/get_started/c_plus_plus.md
deleted file mode 100644
index 9f4800cd99bf..000000000000
--- a/docs/static_site/src/pages/get_started/c_plus_plus.md
+++ /dev/null
@@ -1,55 +0,0 @@
----
-layout: page
-title: C++ Setup
-action: Get Started
-action_url: /get_started
-permalink: /get_started/cpp_setup
----
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-## Build the C++ package
-The C++ package has the same prerequisites as the MXNet library.
-
-To enable C++ package, just add `USE_CPP_PACKAGE=1` in the [build from source](build_from_source) options when building the MXNet shared library.
-
-For example to build MXNet with GPU support and the C++ package, OpenCV, and OpenBLAS, from the project root you would run:
-
-```bash
-cmake -DUSE_CUDA=1 -DUSE_CUDA_PATH=/usr/local/cuda -DUSE_CUDNN=1 -DUSE_MKLDNN=1 -DUSE_CPP_PACKAGE=1 -DCMAKE_BUILD_TYPE=Release -GNinja ..
-ninja -v
-```
-
-You may also want to add the MXNet shared library to your `LD_LIBRARY_PATH`:
-
-```bash
-export LD_LIBRARY_PATH=~/incubator-mxnet/lib
-```
-
-Setting the `LD_LIBRARY_PATH` is required to run the examples mentioned in the following section.
-
-## C++ Example Code
-You can find C++ code examples in the `cpp-package/example` folder of the MXNet project. Refer to the [cpp-package's README](https://github.com/apache/incubator-mxnet/tree/master/cpp-package) for instructions on building the examples.
-
-## Tutorials
-
-* [MXNet C++ API Basics]({{'/api/cpp/docs/tutorials/basics'|relative_url}})
-
-## Related Topics
-
-* [Image Classification using MXNet's C Predict API](https://github.com/apache/incubator-mxnet/tree/master/example/image-classification/predict-cpp)
diff --git a/docs/static_site/src/pages/get_started/centos_setup.md b/docs/static_site/src/pages/get_started/centos_setup.md
deleted file mode 100644
index 315787d21f28..000000000000
--- a/docs/static_site/src/pages/get_started/centos_setup.md
+++ /dev/null
@@ -1,115 +0,0 @@
----
-layout: page
-title: CentOS setup
-action: Get Started
-action_url: /get_started
-permalink: /get_started/centos_setup
----
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-# Installing MXNet on CentOS and other non-Ubuntu Linux systems
-
-Step 1. Install build tools and git on `CentOS >= 7` and `Fedora >= 19`:
-
-```bash
-sudo yum groupinstall -y "Development Tools" && sudo yum install -y git
-```
-
-Step 2. Install Atlas:
-
-```bash
-sudo yum install atlas-devel
-```
-
-Installing both `git` and `cmake` or `make` by following instructions on the websites is
-straightforward. Here we provide the instructions to build `gcc-4.8` from source codes.
-
-Step 3. Install the 32-bit `libc` with one of the following system-specific commands:
-
-```bash
-sudo apt-get install libc6-dev-i386 # In Ubuntu
-sudo yum install glibc-devel.i686 # In RHEL (Red Hat Linux)
-sudo yum install glibc-devel.i386 # In CentOS 5.8
-sudo yum install glibc-devel.i686 # In CentOS 6/7
-```
-
-Step 4. Download and extract the `gcc` source code with the prerequisites:
-
-```bash
-wget http://mirrors.concertpass.com/gcc/releases/gcc-4.8.5/gcc-4.8.5.tar.gz
-tar -zxf gcc-4.8.5.tar.gz
-cd gcc-4.8.5
-./contrib/download_prerequisites
-```
-
-Step 5. Build `gcc` by using 10 threads and then install to `/usr/local`
-
-```bash
-mkdir release && cd release
-../configure --prefix=/usr/local --enable-languages=c,c++
-make -j10
-sudo make install
-```
-
-Step 6. Add the lib path to your configure file such as `~/.bashrc`:
-
-```bash
-export LD_LIBRARY_PATH=${LD_LIBRARY_PATH}:/usr/local/lib64
-```
-
-Step 7. Build [OpenBLAS from source](https://github.com/xianyi/OpenBLAS#installation-from-source).
-
-Step 8. Build OpenCV
-
-To build OpenCV from source code, you need the [cmake](https://cmake.org) library.
-
-* If you don't have cmake or if your version of cmake is earlier than 3.6.1, run the following commands to install a newer version of cmake:
-
-```bash
-wget https://cmake.org/files/v3.6/cmake-3.6.1-Linux-x86_64.tar.gz
-tar -zxvf cmake-3.6.1-Linux-x86_64.tar.gz
-alias cmake="cmake-3.6.1-Linux-x86_64/bin/cmake"
-```
-
-* To download and extract the OpenCV source code, run the following commands:
-
-```bash
-wget https://codeload.github.com/opencv/opencv/zip/2.4.13
-unzip 2.4.13
-cd opencv-2.4.13
-mkdir release
-cd release/
-```
-
-* Build OpenCV. The following commands build OpenCV with 10 threads. We
-disabled GPU support, which might significantly slow down an MXNet program
-running on a GPU processor. It also disables 1394 which might generate a
-warning. Then install it on `/usr/local`.
-
-```bash
-cmake -D BUILD_opencv_gpu=OFF -D WITH_CUDA=OFF -D WITH_1394=OFF -D CMAKE_BUILD_TYPE=RELEASE -D CMAKE_INSTALL_PREFIX=/usr/local ..
-make -j10
-sudo make install
-```
-
-* Add the lib path to your configuration such as `~/.bashrc`.
-
-```bash
-export PKG_CONFIG_PATH=$PKG_CONFIG_PATH:/usr/local/lib/pkgconfig/
-```
diff --git a/docs/static_site/src/pages/get_started/download.md b/docs/static_site/src/pages/get_started/download.md
index cf3f8c1ddf48..eb07b3b7dceb 100644
--- a/docs/static_site/src/pages/get_started/download.md
+++ b/docs/static_site/src/pages/get_started/download.md
@@ -25,16 +25,23 @@ permalink: /get_started/download
# Source Download
-These source archives are generated from tagged releases. Updates and patches will not have been applied. For any updates refer to the corresponding branches in the [GitHub repository](https://github.com/apache/incubator-mxnet). Choose your flavor of download from the following links:
+The source archives listed on this page are official MXNet releases following
+the [Apache Software Foundation Release
+Policy](http://www.apache.org/legal/release-policy.html).
+
+If you would like to actively participate in the MXNet development, you are
+encouraged to contribute to our development version on
+[GitHub](https://github.com/apache/incubator-mxnet).
| Version | Source | PGP | SHA |
|---------|-------------------------------------------------------------------------------------------------------------|-----------------------------------------------------------------------------------------------------------------|---------------------------------------------------------------------------------------------------------------------|
-| 1.5.1 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.5.1/apache-mxnet-src-1.5.1-incubating.tar.gz) | [Download](https://apache.org/dist/incubator/mxnet/1.5.1/apache-mxnet-src-1.5.1-incubating.tar.gz.asc) | [Download](https://apache.org/dist/incubator/mxnet/1.5.1/apache-mxnet-src-1.5.1-incubating.tar.gz.sha512) |
-| 1.5.0 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.5.0/apache-mxnet-src-1.5.0-incubating.tar.gz) | [Download](https://apache.org/dist/incubator/mxnet/1.5.0/apache-mxnet-src-1.5.0-incubating.tar.gz.asc) | [Download](https://apache.org/dist/incubator/mxnet/1.5.0/apache-mxnet-src-1.5.0-incubating.tar.gz.sha512) |
-| 1.4.1 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.4.1/apache-mxnet-src-1.4.1-incubating.tar.gz) | [Download](https://apache.org/dist/incubator/mxnet/1.4.1/apache-mxnet-src-1.4.1-incubating.tar.gz.asc) | [Download](https://apache.org/dist/incubator/mxnet/1.4.1/apache-mxnet-src-1.4.1-incubating.tar.gz.sha512) |
-| 1.4.0 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.4.0/apache-mxnet-src-1.4.0-incubating.tar.gz) | [Download](https://apache.org/dist/incubator/mxnet/1.4.0/apache-mxnet-src-1.4.0-incubating.tar.gz.asc) | [Download](https://apache.org/dist/incubator/mxnet/1.4.0/apache-mxnet-src-1.4.0-incubating.tar.gz.sha512) |
-| 1.3.1 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.3.1/apache-mxnet-src-1.3.1-incubating.tar.gz) | [Download](https://apache.org/dist/incubator/mxnet/1.3.1/apache-mxnet-src-1.3.1-incubating.tar.gz.asc) | [Download](https://apache.org/dist/incubator/mxnet/1.3.1/apache-mxnet-src-1.3.1-incubating.tar.gz.sha512) |
-| 1.3.0 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.3.0/apache-mxnet-src-1.3.0-incubating.tar.gz) | [Download](https://apache.org/dist/incubator/mxnet/1.3.0/apache-mxnet-src-1.3.0-incubating.tar.gz.asc) | [Download](https://apache.org/dist/incubator/mxnet/1.3.0/apache-mxnet-src-1.3.0-incubating.tar.gz.sha512) |
+| 1.6.0 | [Download](https://www.apache.org/dyn/closer.cgi/incubator/mxnet/1.6.0/apache-mxnet-src-1.6.0-incubating.tar.gz) | [Download](https://downloads.apache.org/incubator/mxnet/1.6.0/apache-mxnet-src-1.6.0-incubating.tar.gz.asc) | [Download](https://downloads.apache.org/incubator/mxnet/1.6.0/apache-mxnet-src-1.6.0-incubating.tar.gz.sha512) |
+| 1.5.1 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.5.1/apache-mxnet-src-1.5.1-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.5.1/apache-mxnet-src-1.5.1-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.5.1/apache-mxnet-src-1.5.1-incubating.tar.gz.sha512) |
+| 1.5.0 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.5.0/apache-mxnet-src-1.5.0-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.5.0/apache-mxnet-src-1.5.0-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.5.0/apache-mxnet-src-1.5.0-incubating.tar.gz.sha512) |
+| 1.4.1 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.4.1/apache-mxnet-src-1.4.1-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.4.1/apache-mxnet-src-1.4.1-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.4.1/apache-mxnet-src-1.4.1-incubating.tar.gz.sha512) |
+| 1.4.0 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.4.0/apache-mxnet-src-1.4.0-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.4.0/apache-mxnet-src-1.4.0-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.4.0/apache-mxnet-src-1.4.0-incubating.tar.gz.sha512) |
+| 1.3.1 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.3.1/apache-mxnet-src-1.3.1-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.3.1/apache-mxnet-src-1.3.1-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.3.1/apache-mxnet-src-1.3.1-incubating.tar.gz.sha512) |
+| 1.3.0 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.3.0/apache-mxnet-src-1.3.0-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.3.0/apache-mxnet-src-1.3.0-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.3.0/apache-mxnet-src-1.3.0-incubating.tar.gz.sha512) |
| 1.2.1 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.2.1/apache-mxnet-src-1.2.1-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.2.1/apache-mxnet-src-1.2.1-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.2.1/apache-mxnet-src-1.2.1-incubating.tar.gz.sha512) |
| 1.2.0 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.2.0/apache-mxnet-src-1.2.0-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.2.0/apache-mxnet-src-1.2.0-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.2.0/apache-mxnet-src-1.2.0-incubating.tar.gz.sha512) |
| 1.1.0 | [Download](https://archive.apache.org/dist/incubator/mxnet/1.1.0/apache-mxnet-src-1.1.0-incubating.tar.gz) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.1.0/apache-mxnet-src-1.1.0-incubating.tar.gz.asc) | [Download](https://archive.apache.org/dist/incubator/mxnet/1.1.0/apache-mxnet-src-1.1.0-incubating.tar.gz.sha512) |
diff --git a/docs/static_site/src/pages/get_started/index.html b/docs/static_site/src/pages/get_started/index.html
index 02e7cf1b8641..f83396e28e10 100644
--- a/docs/static_site/src/pages/get_started/index.html
+++ b/docs/static_site/src/pages/get_started/index.html
@@ -22,12 +22,18 @@
-{% include /get_started/get_started.html %}
-
-
Download from source
-
The signed source code for Apache MXNet (incubating) is available for download here
+
Build and install Apache MXNet (incubating) from source
+
+ To build and install MXNet from the official Apache Software Foundation
+ signed source code please follow our Building From Source guide.
+
+
+ The signed source releases are available here
+
+
+{% include /get_started/get_started.html %}
diff --git a/docs/static_site/src/pages/get_started/validate_mxnet.md b/docs/static_site/src/pages/get_started/validate_mxnet.md
index d613c3bf0508..392682acc6e7 100644
--- a/docs/static_site/src/pages/get_started/validate_mxnet.md
+++ b/docs/static_site/src/pages/get_started/validate_mxnet.md
@@ -73,75 +73,6 @@ array([[ 3., 3., 3.],
```
-## Verify GPU Training
-
-From the MXNet root directory run: `python example/image-classification/train_mnist.py --network lenet --gpus 0` to test GPU training.
-
-
-## Virtualenv
-
-Activate the virtualenv environment created for *MXNet*.
-
-```bash
-$ source ~/mxnet/bin/activate
-```
-
-After activating the environment, you should see the prompt as below.
-
-```bash
-(mxnet)$
-```
-
-Start the python terminal.
-
-```bash
-$ python
-```
-
-Run the previous Python example.
-
-
-## Docker with CPU
-
-Launch a Docker container with `mxnet/python` image and run example *MXNet* python program on the terminal.
-
-```bash
-$ docker run -it mxnet/python bash # Use sudo if you skip Step 2 in the installation instruction
-
-# Start a python terminal
-root@4919c4f58cac:/# python
-```
-
-Run the previous Python example.
-
-
-## Docker with GPU
-
-Launch a NVIDIA Docker container with `mxnet/python:gpu` image and run example *MXNet* python program on the terminal.
-
-```bash
-$ nvidia-docker run -it mxnet/python:gpu bash # Use sudo if you skip Step 2 in the installation instruction
-
-# Start a python terminal
-root@4919c4f58cac:/# python
-```
-
-Run the previous Python example and run the previous GPU examples.
-
-
-## Cloud
-
-Login to the cloud instance you launched, with pre-installed *MXNet*, following the guide by corresponding cloud provider.
-
-Start the python terminal.
-
-```bash
-$ python
-```
-
-Run the previous Python example, and for GPU instances run the previous GPU example.
-
-
## Alternative Language Bindings
### C++