-
Notifications
You must be signed in to change notification settings - Fork 1
/
Copy pathindex.js
35 lines (33 loc) · 1.47 KB
/
index.js
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
const settings = require('./config.json')
const getConfig = require('probot-config')
module.exports = app => {
app.on('pull_request.opened', async context => {
const title = context.payload.pull_request.title
const code = title.split(':')[0]
const config = await getConfig(context, 'jira-linker.yml')
let JiraApi = require('jira-client')
let jira = new JiraApi({
protocol: 'https',
host: config.host,
username: config.username,
password: config.password,
apiVersion: '2',
strictSSL: true
})
const url = 'https://' + config.host + '/browse/' + code
const markdownLink = '[' + code + '](' + url + ')'
jira.findIssue(code)
.then(function (issue) {
if (title.split(':').length != 2 || title.split(':')[1][0] != ' ') {
return context.github.issues.createComment({ ...context.issue(), body: '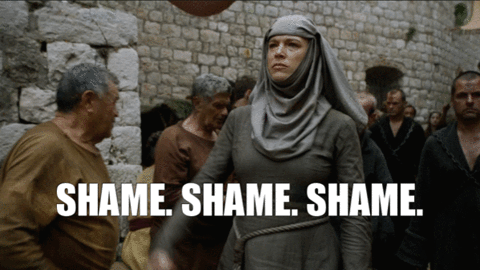' })
} else if (issue == null || issue.key == null || issue.key != code) {
return context.github.issues.createComment({ ...context.issue(), body: 'Issue not found.' })
}
return context.github.issues.createComment({ ...context.issue(), body: '### ' + markdownLink + ': ' + issue.fields.summary + '\n\n ```\n' + issue.fields.description + '\n```' })
})
.catch(function (err) {
app.log(err)
return context.github.issues.createComment({ ...context.issue(), body: 'Issue not found.' })
})
})
}