diff --git a/.npmignore b/.npmignore
new file mode 100644
index 000000000..f8465d198
--- /dev/null
+++ b/.npmignore
@@ -0,0 +1 @@
+examples/large.jpg
diff --git a/Gruntfile.js b/Gruntfile.js
index 2a82cb0b2..141c3d876 100644
--- a/Gruntfile.js
+++ b/Gruntfile.js
@@ -27,6 +27,7 @@ module.exports = function(grunt) {
L: false,
$: false,
LeafletToolbar: false,
+ warpWebGl: false,
// Mocha
diff --git a/README.md b/README.md
index c4e3121d3..dbfc6b329 100644
--- a/README.md
+++ b/README.md
@@ -23,17 +23,56 @@ Here's a screenshot:

+## Demo
+
Check out this [simple demo](https://publiclab.github.io/Leaflet.DistortableImage/examples/index.html).
And watch this GIF demo:
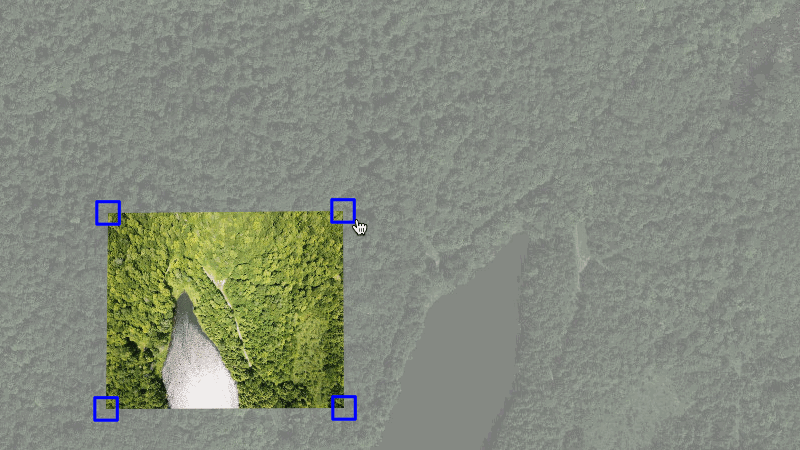
-
-Download as zip or clone to get a copy of the Repo.
-
To test the code, open `index.html` in your browser and click and drag the markers on the edges of the image. The image will show perspectival distortions.
+## Usage
+
+```js
+// basic Leaflet map setup
+map = new L.map('map').setView([51.505, -0.09], 13);
+L.tileLayer('https://{s}.tiles.mapbox.com/v3/anishshah101.ipm9j6em/{z}/{x}/{y}.png', {
+ maxZoom: 18,
+ attribution: 'Map data © OpenStreetMap contributors, ' +
+ 'CC-BY-SA, ' +
+ 'Imagery © Mapbox',
+ id: 'examples.map-i86knfo3'
+}).addTo(map);
+
+// create an image
+img = new L.DistortableImageOverlay(
+ 'example.png', {
+ corners: [
+ new L.latLng(51.52,-0.10),
+ new L.latLng(51.52,-0.14),
+ new L.latLng(51.50,-0.10),
+ new L.latLng(51.50,-0.14)
+ ],
+ fullResolutionSrc: 'large.jpg' // optionally pass in a higher resolution image to use in full-res exporting
+ }
+).addTo(map);
+
+L.DomEvent.on(img._image, 'load', img.editing.enable, img.editing); // enable editing
+
+```
+
+## Full-resolution download
+
+We've added a GPU-accelerated means to generate a full resolution version of the distorted image; it requires two additional dependencies to enable; see how we've included them in the demo:
+
+
+```
+
+
+```
+
****
## Setup
diff --git a/dist/leaflet.distortableimage.js b/dist/leaflet.distortableimage.js
index 6ba20a511..5ba11eed3 100644
--- a/dist/leaflet.distortableimage.js
+++ b/dist/leaflet.distortableimage.js
@@ -636,6 +636,25 @@ var EditOverlayAction = LeafletToolbar.ToolbarAction.extend({
editing._toggleRotateDistort();
this.disable();
}
+ }),
+
+
+ ToggleExport = EditOverlayAction.extend({
+ options: {
+ toolbarIcon: {
+ html: '',
+ tooltip: 'Export Image',
+ title: 'Export Image'
+ }
+ },
+
+ addHooks: function ()
+ {
+ var editing = this._overlay.editing;
+
+ editing._toggleExport();
+ this.disable();
+ }
});
L.DistortableImage.EditToolbar = LeafletToolbar.Popup.extend({
@@ -645,7 +664,8 @@ L.DistortableImage.EditToolbar = LeafletToolbar.Popup.extend({
RemoveOverlay,
ToggleOutline,
ToggleEditable,
- ToggleRotateDistort
+ ToggleRotateDistort,
+ ToggleExport
]
}
});
@@ -658,6 +678,7 @@ L.DistortableImage.Edit = L.Handler.extend({
outline: '1px solid red',
keymap: {
68: '_toggleRotateDistort', // d
+ 69: '_toggleIsolate', // e
73: '_toggleIsolate', // i
76: '_toggleLock', // l
79: '_toggleOutline', // o
@@ -901,6 +922,65 @@ L.DistortableImage.Edit = L.Handler.extend({
L.DomEvent.stopPropagation(event);
},
+
+ // Based on https://github.com/publiclab/mapknitter/blob/8d94132c81b3040ae0d0b4627e685ff75275b416/app/assets/javascripts/mapknitter/Map.js#L47-L82
+ _toggleExport: function (){
+ var map = this._overlay._map;
+ var overlay = this._overlay;
+
+ // make a new image
+ var downloadable = new Image();
+
+ downloadable.id = downloadable.id || "tempId12345";
+ $('body').append(downloadable);
+
+ downloadable.onload = function onLoadDownloadableImage() {
+
+ var height = downloadable.height,
+ width = downloadable.width,
+ nw = map.latLngToLayerPoint(overlay._corners[0]),
+ ne = map.latLngToLayerPoint(overlay._corners[1]),
+ sw = map.latLngToLayerPoint(overlay._corners[2]),
+ se = map.latLngToLayerPoint(overlay._corners[3]);
+
+ // I think this is to move the image to the upper left corner,
+ // jywarren: i think we may need these or the image goes off the edge of the canvas
+ // jywarren: but these seem to break the distortion math...
+
+ // jywarren: i think it should be rejiggered so it
+ // finds the most negative values of x and y and then
+ // adds those to all coordinates
+
+ //nw.x -= nw.x;
+ //ne.x -= nw.x;
+ //se.x -= nw.x;
+ //sw.x -= nw.x;
+
+ //nw.y -= nw.y;
+ //ne.y -= nw.y;
+ //se.y -= nw.y;
+ //sw.y -= nw.y;
+
+ // run once warping is complete
+ downloadable.onload = function() {
+ $(downloadable).remove();
+ };
+
+ if (window && window.hasOwnProperty('warpWebGl')) {
+ warpWebGl(
+ downloadable.id,
+ [0, 0, width, 0, width, height, 0, height],
+ [nw.x, nw.y, ne.x, ne.y, se.x, se.y, sw.x, sw.y],
+ true // trigger download
+ );
+ }
+
+ };
+
+ downloadable.src = overlay.options.fullResolutionSrc || overlay._image.src;
+
+ },
+
toggleIsolate: function() {
// this.isolated = !this.isolated;
// if (this.isolated) {
diff --git a/examples/example.jpg b/examples/example.jpg
deleted file mode 100644
index 8e824c949..000000000
Binary files a/examples/example.jpg and /dev/null differ
diff --git a/examples/example.png b/examples/example.png
new file mode 100644
index 000000000..fb5b7994a
Binary files /dev/null and b/examples/example.png differ
diff --git a/examples/index.html b/examples/index.html
index a38383d0d..2dbea03a4 100644
--- a/examples/index.html
+++ b/examples/index.html
@@ -12,6 +12,11 @@
+
+
+
+
+
@@ -41,18 +46,19 @@
// create an image
img = new L.DistortableImageOverlay(
- 'example.jpg', {
+ 'example.png', {
corners: [
new L.latLng(51.52,-0.10),
new L.latLng(51.52,-0.14),
new L.latLng(51.50,-0.10),
new L.latLng(51.50,-0.14)
- ]
+ ],
+ fullResolutionSrc: 'large.jpg'
}
).addTo(map);
L.DomEvent.on(img._image, 'load', img.editing.enable, img.editing);
})();
-
+