diff --git a/.github/workflows/black.yml b/.github/workflows/black.yml
new file mode 100644
index 0000000000..053967a9ff
--- /dev/null
+++ b/.github/workflows/black.yml
@@ -0,0 +1,27 @@
+name: Black
+
+env:
+ PYTHONUNBUFFERED: 1
+
+on:
+ push:
+ branches: [ master ]
+ pull_request:
+ branches: [ master ]
+
+jobs:
+ black:
+ runs-on: ubuntu-latest
+ steps:
+ - uses: actions/checkout@v4
+
+ - name: Set up Python
+ uses: actions/setup-python@v4
+ with:
+ python-version: '3.11'
+
+ - name: Install black
+ run: pip install black==23.7.0
+
+ - name: Check code with black
+ run: black --check --diff --color .
diff --git a/README.md b/README.md
index a7a5389a7c..6297b9a96c 100644
--- a/README.md
+++ b/README.md
@@ -1,6 +1,7 @@
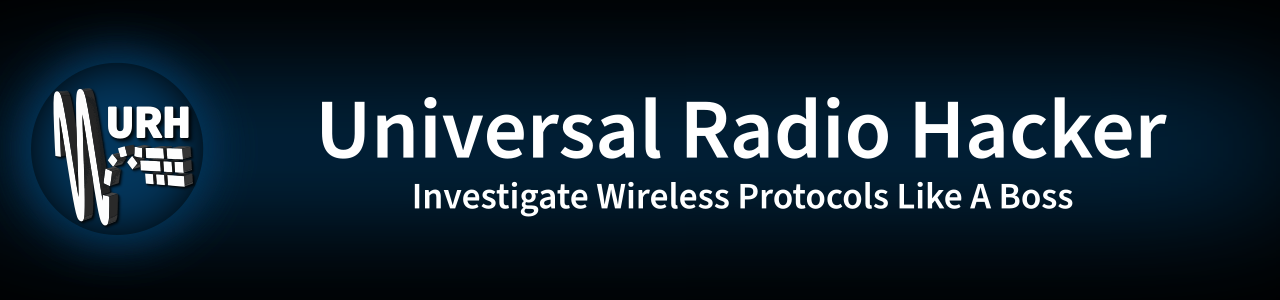
[](https://github.com/jopohl/urh/actions/workflows/ci.yml)
+[](https://github.com/psf/black)
[](https://badge.fury.io/py/urh)
[](https://repology.org/project/urh/versions)
[](http://www.toolswatch.org/2017/06/the-black-hat-arsenal-usa-2017-phenomenal-line-up-announced/)
diff --git a/data/build_icons.py b/data/build_icons.py
index 65e9314ae2..dc8a4c9323 100644
--- a/data/build_icons.py
+++ b/data/build_icons.py
@@ -25,7 +25,7 @@ def get_used_icon_names():
with open(sourcefile, "r") as f:
for line in f:
if "QIcon.fromTheme" in line:
- icon = line[line.find("QIcon.fromTheme"):]
+ icon = line[line.find("QIcon.fromTheme") :]
icon = icon.replace('"', "'")
start = icon.find("'") + 1
end = icon.find("'", start)
@@ -35,7 +35,11 @@ def get_used_icon_names():
def copy_icons(icon_names: set):
target_dir = "/tmp/oxy"
- sizes = [s for s in os.listdir(OXYGEN_PATH) if os.path.isdir(os.path.join(OXYGEN_PATH, s))] # 8x8, 22x22 ...
+ sizes = [
+ s
+ for s in os.listdir(OXYGEN_PATH)
+ if os.path.isdir(os.path.join(OXYGEN_PATH, s))
+ ] # 8x8, 22x22 ...
for size in sizes:
target_size_dir = os.path.join(target_dir, size)
os.makedirs(target_size_dir, exist_ok=True)
@@ -59,7 +63,7 @@ def copy_icons(icon_names: set):
for size in sizes:
f.write("\n")
f.write("[" + size + "]\n")
- f.write("Size=" + size[:size.index("x")] + "\n")
+ f.write("Size=" + size[: size.index("x")] + "\n")
f.write("\n")
root = ET.Element("RCC")
diff --git a/data/check_native_backends.py b/data/check_native_backends.py
index 5c1503b774..9fea18cafc 100755
--- a/data/check_native_backends.py
+++ b/data/check_native_backends.py
@@ -3,21 +3,33 @@
import importlib
import os
import sys
+
rc = 0
if sys.platform == "win32":
- shared_lib_dir = os.path.realpath(os.path.join(os.path.dirname(__file__), "..", "src/urh/dev/native/lib/shared"))
+ shared_lib_dir = os.path.realpath(
+ os.path.join(os.path.dirname(__file__), "..", "src/urh/dev/native/lib/shared")
+ )
print("Attempting to read", shared_lib_dir)
if os.path.isdir(shared_lib_dir):
os.environ["PATH"] = os.environ.get("PATH", "") + os.pathsep + shared_lib_dir
print("PATH updated")
-for sdr in ("AirSpy", "BladeRF", "HackRF", "RTLSDR", "LimeSDR", "PlutoSDR", "SDRPlay", "USRP"):
+for sdr in (
+ "AirSpy",
+ "BladeRF",
+ "HackRF",
+ "RTLSDR",
+ "LimeSDR",
+ "PlutoSDR",
+ "SDRPlay",
+ "USRP",
+):
try:
- importlib.import_module('.{}'.format(sdr.lower()), 'urh.dev.native.lib')
- print("{:<10} \033[92mSUCCESS\033[0m".format(sdr+":"))
+ importlib.import_module(".{}".format(sdr.lower()), "urh.dev.native.lib")
+ print("{:<10} \033[92mSUCCESS\033[0m".format(sdr + ":"))
except ImportError as e:
- print("{:<10} \033[91mFAILURE\033[0m ({})".format(sdr+":", e))
+ print("{:<10} \033[91mFAILURE\033[0m ({})".format(sdr + ":", e))
rc = 1
sys.exit(rc)
diff --git a/data/generate_ui.py b/data/generate_ui.py
index e4fa353c34..5a400681a8 100644
--- a/data/generate_ui.py
+++ b/data/generate_ui.py
@@ -44,13 +44,14 @@ def gen(force=False):
# Remove Line: # Form implementation generated from reading ui file '/home/joe/GIT/urh/ui/fuzzing.ui'
# to avoid useless git updates when working on another computer
for line in fileinput.input(out_file_path, inplace=True):
- if line.startswith("# Form implementation generated from reading ui file") or line.startswith(
- "# Created by: "):
+ if line.startswith(
+ "# Form implementation generated from reading ui file"
+ ) or line.startswith("# Created by: "):
continue
if line.strip().startswith("QtCore.QMetaObject.connectSlotsByName("):
# disable auto slot connection, as we do not use it, and it causes crash on python 3.7
continue
- print(line, end='')
+ print(line, end="")
for f in rc_files:
file_path = os.path.join(rc_path, f)
diff --git a/data/pyinstaller_helper.py b/data/pyinstaller_helper.py
index 7869148c62..1f65d91838 100644
--- a/data/pyinstaller_helper.py
+++ b/data/pyinstaller_helper.py
@@ -2,14 +2,24 @@
import shutil
import sys
-HIDDEN_IMPORTS = ["packaging.specifiers", "packaging.requirements", "pkg_resources.py2_warn",
- "numpy.core._methods", "numpy.core._dtype_ctypes",
- "numpy.random.common", "numpy.random.entropy", "numpy.random.bounded_integers"]
-DATA = [("src/urh/dev/native/lib/shared", "."), ("src/urh/plugins", "urh/plugins"), ]
+HIDDEN_IMPORTS = [
+ "packaging.specifiers",
+ "packaging.requirements",
+ "pkg_resources.py2_warn",
+ "numpy.core._methods",
+ "numpy.core._dtype_ctypes",
+ "numpy.random.common",
+ "numpy.random.entropy",
+ "numpy.random.bounded_integers",
+]
+DATA = [
+ ("src/urh/dev/native/lib/shared", "."),
+ ("src/urh/plugins", "urh/plugins"),
+]
EXCLUDE = ["matplotlib"]
-def run_pyinstaller(cmd_list: list, env: list=None):
+def run_pyinstaller(cmd_list: list, env: list = None):
cmd = " ".join(cmd_list)
print(cmd, flush=True)
@@ -20,7 +30,7 @@ def run_pyinstaller(cmd_list: list, env: list=None):
os.system(cmd)
-if __name__ == '__main__':
+if __name__ == "__main__":
cmd = ["pyinstaller", "--clean"]
if sys.platform == "darwin":
cmd.append("--onefile")
@@ -38,31 +48,52 @@ def run_pyinstaller(cmd_list: list, env: list=None):
urh_path = os.path.realpath(os.path.join(os.path.dirname(__file__), ".."))
if sys.platform == "darwin":
- cmd.append('--icon="{}"'.format(os.path.join(urh_path, "data/icons/appicon.icns")))
+ cmd.append(
+ '--icon="{}"'.format(os.path.join(urh_path, "data/icons/appicon.icns"))
+ )
else:
- cmd.append('--icon="{}"'.format(os.path.join(urh_path, "data/icons/appicon.ico")))
+ cmd.append(
+ '--icon="{}"'.format(os.path.join(urh_path, "data/icons/appicon.ico"))
+ )
cmd.extend(["--distpath", "./pyinstaller"])
- urh_cmd = cmd + ["--name=urh", "--windowed", "--workpath", "./urh_build",
- os.path.join(urh_path, "src/urh/main.py")]
-
- urh_debug_cmd = cmd + ["--name=urh_debug", "--workpath", "./urh_debug_build",
- os.path.join(urh_path, "src/urh/main.py")]
-
- cli_cmd = cmd + ["--workpath", "./urh_cli_build",
- os.path.join(urh_path, "src/urh/cli/urh_cli.py")]
+ urh_cmd = cmd + [
+ "--name=urh",
+ "--windowed",
+ "--workpath",
+ "./urh_build",
+ os.path.join(urh_path, "src/urh/main.py"),
+ ]
+
+ urh_debug_cmd = cmd + [
+ "--name=urh_debug",
+ "--workpath",
+ "./urh_debug_build",
+ os.path.join(urh_path, "src/urh/main.py"),
+ ]
+
+ cli_cmd = cmd + [
+ "--workpath",
+ "./urh_cli_build",
+ os.path.join(urh_path, "src/urh/cli/urh_cli.py"),
+ ]
os.makedirs("./pyinstaller")
if sys.platform == "darwin":
- run_pyinstaller(urh_cmd, env=["DYLD_LIBRARY_PATH=src/urh/dev/native/lib/shared"])
+ run_pyinstaller(
+ urh_cmd, env=["DYLD_LIBRARY_PATH=src/urh/dev/native/lib/shared"]
+ )
import plistlib
+
with open("pyinstaller/urh.app/Contents/Info.plist", "rb") as f:
p = plistlib.load(f)
p["NSHighResolutionCapable"] = True
p["NSRequiresAquaSystemAppearance"] = True
- p["NSMicrophoneUsageDescription"] = "URH needs access to your microphone to capture signals via Soundcard."
+ p[
+ "NSMicrophoneUsageDescription"
+ ] = "URH needs access to your microphone to capture signals via Soundcard."
with open("pyinstaller/urh.app/Contents/Info.plist", "wb") as f:
plistlib.dump(p, f)
@@ -70,5 +101,9 @@ def run_pyinstaller(cmd_list: list, env: list=None):
for cmd in [urh_cmd, cli_cmd, urh_debug_cmd]:
run_pyinstaller(cmd)
- shutil.copy("./pyinstaller/urh_cli/urh_cli.exe", "./pyinstaller/urh/urh_cli.exe")
- shutil.copy("./pyinstaller/urh_debug/urh_debug.exe", "./pyinstaller/urh/urh_debug.exe")
+ shutil.copy(
+ "./pyinstaller/urh_cli/urh_cli.exe", "./pyinstaller/urh/urh_cli.exe"
+ )
+ shutil.copy(
+ "./pyinstaller/urh_debug/urh_debug.exe", "./pyinstaller/urh/urh_debug.exe"
+ )
diff --git a/data/release.py b/data/release.py
index 4c42f4914b..aee41c6fe6 100644
--- a/data/release.py
+++ b/data/release.py
@@ -11,7 +11,11 @@ def cleanup():
Remove all cache directories
:return:
"""
- script_dir = os.path.dirname(__file__) if not os.path.islink(__file__) else os.path.dirname(os.readlink(__file__))
+ script_dir = (
+ os.path.dirname(__file__)
+ if not os.path.islink(__file__)
+ else os.path.dirname(os.readlink(__file__))
+ )
script_dir = os.path.realpath(os.path.join(script_dir, ".."))
shutil.rmtree(os.path.join(script_dir, "dist"), ignore_errors=True)
shutil.rmtree(os.path.join(script_dir, "tmp"), ignore_errors=True)
@@ -21,11 +25,19 @@ def cleanup():
def release():
- script_dir = os.path.dirname(__file__) if not os.path.islink(__file__) else os.path.dirname(os.readlink(__file__))
+ script_dir = (
+ os.path.dirname(__file__)
+ if not os.path.islink(__file__)
+ else os.path.dirname(os.readlink(__file__))
+ )
script_dir = os.path.realpath(os.path.join(script_dir, ".."))
os.chdir(script_dir)
- current_branch = check_output(["git", "rev-parse", "--abbrev-ref", "HEAD"]).decode("UTF-8").strip()
+ current_branch = (
+ check_output(["git", "rev-parse", "--abbrev-ref", "HEAD"])
+ .decode("UTF-8")
+ .strip()
+ )
if current_branch != "master":
print("You can only release from master!")
@@ -34,7 +46,10 @@ def release():
open(os.path.join(tempfile.gettempdir(), "urh_releasing"), "w").close()
from src.urh import version
- version_file = os.path.realpath(os.path.join(script_dir, "src", "urh", "version.py"))
+
+ version_file = os.path.realpath(
+ os.path.join(script_dir, "src", "urh", "version.py")
+ )
cur_version = version.VERSION
numbers = cur_version.split(".")
@@ -44,7 +59,7 @@ def release():
for line in fileinput.input(version_file, inplace=True):
if line.startswith("VERSION"):
line = 'VERSION = "{0}" \n'.format(cur_version)
- print(line, end='')
+ print(line, end="")
# Publish new version number
call(["git", "add", version_file])
@@ -61,16 +76,27 @@ def release():
# Push new tag
call(["git", "tag", "v" + cur_version, "-m", "version " + cur_version])
- call(["git", "push", "origin", "--tags"]) # Creates tar package on https://github.com/jopohl/urh/tarball/va.b.c.d
+ call(
+ ["git", "push", "origin", "--tags"]
+ ) # Creates tar package on https://github.com/jopohl/urh/tarball/va.b.c.d
os.remove(os.path.join(tempfile.gettempdir(), "urh_releasing"))
# region Build docker image and push to DockerHub
os.chdir(os.path.dirname(__file__))
call(["docker", "login"])
- call(["docker", "build", "--no-cache",
- "--tag", "jopohl/urh:latest",
- "--tag", "jopohl/urh:{}".format(cur_version), "."])
+ call(
+ [
+ "docker",
+ "build",
+ "--no-cache",
+ "--tag",
+ "jopohl/urh:latest",
+ "--tag",
+ "jopohl/urh:{}".format(cur_version),
+ ".",
+ ]
+ )
call(["docker", "push", "jopohl/urh:latest"])
call(["docker", "push", "jopohl/urh:{}".format(cur_version)])
# endregion
diff --git a/setup.py b/setup.py
index 8b51ec6f57..ac76efcbad 100644
--- a/setup.py
+++ b/setup.py
@@ -31,7 +31,11 @@
NO_NUMPY_WARNINGS_FLAG = "-Wno-cpp"
UI_SUBDIRS = ("actions", "delegates", "views")
-PLUGINS = [path for path in os.listdir("src/urh/plugins") if os.path.isdir(os.path.join("src/urh/plugins", path))]
+PLUGINS = [
+ path
+ for path in os.listdir("src/urh/plugins")
+ if os.path.isdir(os.path.join("src/urh/plugins", path))
+]
URH_DIR = "urh"
IS_RELEASE = os.path.isfile(os.path.join(tempfile.gettempdir(), "urh_releasing"))
@@ -39,9 +43,11 @@
try:
from Cython.Build import cythonize
except ImportError:
- print("You need Cython to build URH's extensions!\n"
- "You can get it e.g. with python3 -m pip install cython.",
- file=sys.stderr)
+ print(
+ "You need Cython to build URH's extensions!\n"
+ "You can get it e.g. with python3 -m pip install cython.",
+ file=sys.stderr,
+ )
sys.exit(1)
@@ -52,6 +58,7 @@ def finalize_options(self):
# Prevent numpy from thinking it is still in its setup process:
__builtins__.__NUMPY_SETUP__ = False
import numpy
+
self.include_dirs.append(numpy.get_include())
@@ -59,7 +66,9 @@ def get_packages():
packages = [URH_DIR]
separator = os.path.normpath("/")
for dirpath, dirnames, filenames in os.walk(os.path.join("./src/", URH_DIR)):
- package_path = os.path.relpath(dirpath, os.path.join("./src/", URH_DIR)).replace(separator, ".")
+ package_path = os.path.relpath(
+ dirpath, os.path.join("./src/", URH_DIR)
+ ).replace(separator, ".")
if len(package_path) > 1:
packages.append(URH_DIR + "." + package_path)
@@ -69,7 +78,7 @@ def get_packages():
def get_package_data():
package_data = {"urh.cythonext": ["*.pyx", "*.pxd"]}
for plugin in PLUGINS:
- package_data["urh.plugins." + plugin] = ['*.ui', "*.txt"]
+ package_data["urh.plugins." + plugin] = ["*.ui", "*.txt"]
package_data["urh.dev.native.lib"] = ["*.pyx", "*.pxd"]
@@ -80,21 +89,38 @@ def get_package_data():
def get_extensions():
- filenames = [os.path.splitext(f)[0] for f in os.listdir("src/urh/cythonext") if f.endswith(".pyx")]
- extensions = [Extension("urh.cythonext." + f, ["src/urh/cythonext/" + f + ".pyx"],
- extra_compile_args=[OPEN_MP_FLAG],
- extra_link_args=[OPEN_MP_FLAG],
- language="c++") for f in filenames]
+ filenames = [
+ os.path.splitext(f)[0]
+ for f in os.listdir("src/urh/cythonext")
+ if f.endswith(".pyx")
+ ]
+ extensions = [
+ Extension(
+ "urh.cythonext." + f,
+ ["src/urh/cythonext/" + f + ".pyx"],
+ extra_compile_args=[OPEN_MP_FLAG],
+ extra_link_args=[OPEN_MP_FLAG],
+ language="c++",
+ )
+ for f in filenames
+ ]
ExtensionHelper.USE_RELATIVE_PATHS = True
- device_extensions, device_extras = ExtensionHelper.get_device_extensions_and_extras()
+ (
+ device_extensions,
+ device_extras,
+ ) = ExtensionHelper.get_device_extensions_and_extras()
extensions += device_extensions
if NO_NUMPY_WARNINGS_FLAG:
for extension in extensions:
extension.extra_compile_args.append(NO_NUMPY_WARNINGS_FLAG)
- extensions = cythonize(extensions, compiler_directives=COMPILER_DIRECTIVES, compile_time_env=device_extras)
+ extensions = cythonize(
+ extensions,
+ compiler_directives=COMPILER_DIRECTIVES,
+ compile_time_env=device_extras,
+ )
return extensions
@@ -117,7 +143,7 @@ def read_long_description():
install_requires.append("pyqt5")
if sys.version_info < (3, 4):
- install_requires.append('enum34')
+ install_requires.append("enum34")
setup(
name="urh",
@@ -133,14 +159,15 @@ def read_long_description():
license="GNU General Public License (GPL)",
download_url="https://github.com/jopohl/urh/tarball/v" + str(version.VERSION),
install_requires=install_requires,
- setup_requires=['numpy'],
+ setup_requires=["numpy"],
packages=get_packages(),
ext_modules=get_extensions(),
- cmdclass={'build_ext': build_ext},
+ cmdclass={"build_ext": build_ext},
zip_safe=False,
entry_points={
- 'console_scripts': [
- 'urh = urh.main:main',
- 'urh_cli = urh.cli.urh_cli:main',
- ]}
+ "console_scripts": [
+ "urh = urh.main:main",
+ "urh_cli = urh.cli.urh_cli:main",
+ ]
+ },
)
diff --git a/src/urh/ainterpretation/AutoInterpretation.py b/src/urh/ainterpretation/AutoInterpretation.py
index aaede6cd48..8f3fc13acf 100644
--- a/src/urh/ainterpretation/AutoInterpretation.py
+++ b/src/urh/ainterpretation/AutoInterpretation.py
@@ -26,6 +26,7 @@ def min_without_outliers(data: np.ndarray, z=2):
return np.min(data[abs(data - np.mean(data)) <= z * np.std(data)])
+
def get_most_frequent_value(values: list):
"""
Return the most frequent value in list.
@@ -66,9 +67,15 @@ def detect_noise_level(magnitudes):
chunksize_percent = 1
chunksize = max(1, int(len(magnitudes) * chunksize_percent / 100))
- chunks = [magnitudes[i - chunksize:i] for i in range(len(magnitudes), 0, -chunksize) if i - chunksize >= 0]
+ chunks = [
+ magnitudes[i - chunksize : i]
+ for i in range(len(magnitudes), 0, -chunksize)
+ if i - chunksize >= 0
+ ]
- mean_values = np.fromiter((np.mean(chunk) for chunk in chunks), dtype=np.float32, count=len(chunks))
+ mean_values = np.fromiter(
+ (np.mean(chunk) for chunk in chunks), dtype=np.float32, count=len(chunks)
+ )
minimum, maximum = util.minmax(mean_values)
if maximum == 0 or minimum / maximum > 0.9:
# Mean values are very close to each other, so there is probably no noise in the signal
@@ -94,7 +101,9 @@ def segment_messages_from_magnitudes(magnitudes: np.ndarray, noise_threshold: fl
:param noise_threshold: Threshold for noise
:return:
"""
- return c_auto_interpretation.segment_messages_from_magnitudes(magnitudes, noise_threshold)
+ return c_auto_interpretation.segment_messages_from_magnitudes(
+ magnitudes, noise_threshold
+ )
def merge_message_segments_for_ook(segments: list):
@@ -106,13 +115,13 @@ def merge_message_segments_for_ook(segments: list):
pauses = np.fromiter(
(segments[i + 1][0] - segments[i][1] for i in range(len(segments) - 1)),
count=len(segments) - 1,
- dtype=np.uint64
+ dtype=np.uint64,
)
pulses = np.fromiter(
(segments[i][1] - segments[i][0] for i in range(len(segments))),
count=len(segments),
- dtype=np.uint64
+ dtype=np.uint64,
)
# Find relatively large pauses, these mark new messages
@@ -122,7 +131,9 @@ def merge_message_segments_for_ook(segments: list):
# Merge Pulse Lengths between long pauses
for i in range(0, len(large_pause_indices) + 1):
if i == 0:
- start, end = 0, large_pause_indices[i] + 1 if len(large_pause_indices) >= 1 else len(segments)
+ start, end = 0, large_pause_indices[i] + 1 if len(
+ large_pause_indices
+ ) >= 1 else len(segments)
elif i == len(large_pause_indices):
start, end = large_pause_indices[i - 1] + 1, len(segments)
else:
@@ -130,7 +141,9 @@ def merge_message_segments_for_ook(segments: list):
msg_begin = segments[start][0]
msg_length = sum(segments[j][1] - segments[j][0] for j in range(start, end))
- msg_length += sum(segments[j][0] - segments[j - 1][1] for j in range(start + 1, end))
+ msg_length += sum(
+ segments[j][0] - segments[j - 1][1] for j in range(start + 1, end)
+ )
result.append((msg_begin, msg_begin + msg_length))
@@ -156,10 +169,17 @@ def detect_modulation(data: np.ndarray, wavelet_scale=4, median_filter_order=11)
var_mag = np.var(mag_wavlt)
var_norm_mag = np.var(norm_mag_wavlt)
- var_filtered_mag = np.var(c_auto_interpretation.median_filter(mag_wavlt, k=median_filter_order))
- var_filtered_norm_mag = np.var(c_auto_interpretation.median_filter(norm_mag_wavlt, k=median_filter_order))
+ var_filtered_mag = np.var(
+ c_auto_interpretation.median_filter(mag_wavlt, k=median_filter_order)
+ )
+ var_filtered_norm_mag = np.var(
+ c_auto_interpretation.median_filter(norm_mag_wavlt, k=median_filter_order)
+ )
- if all(v < 0.15 for v in (var_mag, var_norm_mag, var_filtered_mag, var_filtered_norm_mag)):
+ if all(
+ v < 0.15
+ for v in (var_mag, var_norm_mag, var_filtered_mag, var_filtered_norm_mag)
+ ):
return "OOK"
if var_mag > 1.5 * var_norm_mag:
@@ -173,14 +193,17 @@ def detect_modulation(data: np.ndarray, wavelet_scale=4, median_filter_order=11)
else:
# Now we either have a FSK signal or we a have OOK single pulse
# If we have an FSK, there should be at least two peaks in FFT
- fft = np.fft.fft(data[0:2 ** int(np.log2(len(data)))])
+ fft = np.fft.fft(data[0 : 2 ** int(np.log2(len(data)))])
fft = np.abs(np.fft.fftshift(fft))
ten_greatest_indices = np.argsort(fft)[::-1][0:10]
greatest_index = ten_greatest_indices[0]
min_distance = 10
min_freq = 100 # 100 seems to be magnitude of noise frequency
- if any(abs(i - greatest_index) >= min_distance and fft[i] >= min_freq for i in ten_greatest_indices):
+ if any(
+ abs(i - greatest_index) >= min_distance and fft[i] >= min_freq
+ for i in ten_greatest_indices
+ ):
return "FSK"
else:
return "OOK"
@@ -207,7 +230,7 @@ def detect_center(rectangular_signal: np.ndarray, max_size=None):
# Ignore the first and last 5% of samples,
# because there tends to be an overshoot at start/end of rectangular signal
- rect = rect[int(0.05*len(rect)):int(0.95*len(rect))]
+ rect = rect[int(0.05 * len(rect)) : int(0.95 * len(rect))]
if max_size is not None and len(rect) > max_size:
rect = rect[0:max_size]
@@ -219,7 +242,9 @@ def detect_center(rectangular_signal: np.ndarray, max_size=None):
hist_step = float(np.var(rect))
try:
- y, x = np.histogram(rect, bins=np.arange(hist_min, hist_max + hist_step, hist_step))
+ y, x = np.histogram(
+ rect, bins=np.arange(hist_min, hist_max + hist_step, hist_step)
+ )
except (ZeroDivisionError, ValueError):
# For a segment with zero variance (constant line) it is not possible to find a center
return None
@@ -227,7 +252,7 @@ def detect_center(rectangular_signal: np.ndarray, max_size=None):
num_values = 2
most_common_levels = []
- window_size = max(2, int(0.05*len(y)) + 1)
+ window_size = max(2, int(0.05 * len(y)) + 1)
def get_elem(arr, index: int, default):
if 0 <= index < len(arr):
@@ -237,9 +262,11 @@ def get_elem(arr, index: int, default):
for index in np.argsort(y)[::-1]:
# check if we have a local maximum in histogram, if yes, append the value
- if all(y[index] > get_elem(y, index+i, 0) and
- y[index] > get_elem(y, index-i, 0)
- for i in range(1, window_size)):
+ if all(
+ y[index] > get_elem(y, index + i, 0)
+ and y[index] > get_elem(y, index - i, 0)
+ for i in range(1, window_size)
+ ):
most_common_levels.append(x[index])
if len(most_common_levels) == num_values:
@@ -280,7 +307,9 @@ def merge_plateau_lengths(plateau_lengths, tolerance=None) -> list:
if tolerance == 0 or tolerance is None:
return plateau_lengths
- return c_auto_interpretation.merge_plateaus(plateau_lengths, tolerance, max_count=10000)
+ return c_auto_interpretation.merge_plateaus(
+ plateau_lengths, tolerance, max_count=10000
+ )
def round_plateau_lengths(plateau_lengths: list):
@@ -322,7 +351,9 @@ def get_bit_length_from_plateau_lengths(merged_plateau_lengths) -> int:
return int(merged_plateau_lengths[0])
round_plateau_lengths(merged_plateau_lengths)
- histogram = c_auto_interpretation.get_threshold_divisor_histogram(merged_plateau_lengths)
+ histogram = c_auto_interpretation.get_threshold_divisor_histogram(
+ merged_plateau_lengths
+ )
if len(histogram) == 0:
return 0
@@ -350,10 +381,16 @@ def estimate(iq_array: IQArray, noise: float = None, modulation: str = None) ->
noise = detect_noise_level(magnitudes) if noise is None else noise
# segment messages
- message_indices = segment_messages_from_magnitudes(magnitudes, noise_threshold=noise)
+ message_indices = segment_messages_from_magnitudes(
+ magnitudes, noise_threshold=noise
+ )
# detect modulation
- modulation = detect_modulation_for_messages(iq_array, message_indices) if modulation is None else modulation
+ modulation = (
+ detect_modulation_for_messages(iq_array, message_indices)
+ if modulation is None
+ else modulation
+ )
if modulation is None:
return None
@@ -379,7 +416,9 @@ def estimate(iq_array: IQArray, noise: float = None, modulation: str = None) ->
if center is None:
continue
- plateau_lengths = c_auto_interpretation.get_plateau_lengths(msg_rect_data, center, percentage=25)
+ plateau_lengths = c_auto_interpretation.get_plateau_lengths(
+ msg_rect_data, center, percentage=25
+ )
tolerance = estimate_tolerance_from_plateau_lengths(plateau_lengths)
if tolerance is None:
tolerance = 0
@@ -428,7 +467,7 @@ def estimate(iq_array: IQArray, noise: float = None, modulation: str = None) ->
"bit_length": bit_length,
"center": center,
"tolerance": int(tolerance),
- "noise": noise
+ "noise": noise,
}
return result
diff --git a/src/urh/ainterpretation/Wavelet.py b/src/urh/ainterpretation/Wavelet.py
index 37ca23c8e8..74af0af9f5 100644
--- a/src/urh/ainterpretation/Wavelet.py
+++ b/src/urh/ainterpretation/Wavelet.py
@@ -1,6 +1,6 @@
import numpy as np
-from urh.cythonext import auto_interpretation as cy_auto_interpretation
+from urh.cythonext import auto_interpretation as cy_auto_interpretation
from urh.signalprocessing.Modulator import Modulator
@@ -27,16 +27,20 @@ def cwt_haar(x: np.ndarray, scale=10):
x_hat = np.fft.fft(x)
# Get omega (eq. (5) in paper)
- f = (2.0 * np.pi / num_data)
- omega = f * np.concatenate((np.arange(0, num_data // 2), np.arange(num_data // 2, num_data) * -1))
+ f = 2.0 * np.pi / num_data
+ omega = f * np.concatenate(
+ (np.arange(0, num_data // 2), np.arange(num_data // 2, num_data) * -1)
+ )
# get psi hat (eq. (6) in paper)
- psi_hat = np.sqrt(2.0 * np.pi * scale) * normalized_haar_wavelet(scale * omega, scale)
+ psi_hat = np.sqrt(2.0 * np.pi * scale) * normalized_haar_wavelet(
+ scale * omega, scale
+ )
# get W (eq. (4) in paper)
W = np.fft.ifft(x_hat * psi_hat)
- return W[2 * scale:-2 * scale]
+ return W[2 * scale : -2 * scale]
if __name__ == "__main__":
@@ -45,8 +49,10 @@ def cwt_haar(x: np.ndarray, scale=10):
# data = np.fromfile("/home/joe/GIT/urh/tests/data/fsk.complex", dtype=np.complex64)[5:15000]
# data = np.fromfile("/home/joe/GIT/urh/tests/data/ask.complex", dtype=np.complex64)[462:754]
# data = np.fromfile("/home/joe/GIT/urh/tests/data/enocean.complex", dtype=np.complex64)[9724:10228]
- data = np.fromfile("/home/joe/GIT/publications/ainterpretation/experiments/signals/esaver_test4on.complex",
- dtype=np.complex64)[86452:115541]
+ data = np.fromfile(
+ "/home/joe/GIT/publications/ainterpretation/experiments/signals/esaver_test4on.complex",
+ dtype=np.complex64,
+ )[86452:115541]
# data = np.fromfile("/home/joe/GIT/urh/tests/data/action_ook.complex", dtype=np.complex64)[3780:4300]
diff --git a/src/urh/awre/AutoAssigner.py b/src/urh/awre/AutoAssigner.py
index 6b37d9a1a3..8333b3cfae 100644
--- a/src/urh/awre/AutoAssigner.py
+++ b/src/urh/awre/AutoAssigner.py
@@ -24,7 +24,9 @@ def auto_assign_participants(messages, participants):
src_address = msg.get_src_address_from_data()
if src_address:
try:
- msg.participant = next(p for p in participants if p.address_hex == src_address)
+ msg.participant = next(
+ p for p in participants if p.address_hex == src_address
+ )
except StopIteration:
pass
diff --git a/src/urh/awre/CommonRange.py b/src/urh/awre/CommonRange.py
index a7f1c50aba..f43729bb2b 100644
--- a/src/urh/awre/CommonRange.py
+++ b/src/urh/awre/CommonRange.py
@@ -8,8 +8,17 @@
class CommonRange(object):
- def __init__(self, start, length, value: np.ndarray = None, score=0, field_type="Generic", message_indices=None,
- range_type="bit", byte_order="big"):
+ def __init__(
+ self,
+ start,
+ length,
+ value: np.ndarray = None,
+ score=0,
+ field_type="Generic",
+ message_indices=None,
+ range_type="bit",
+ byte_order="big",
+ ):
"""
:param start:
@@ -31,7 +40,9 @@ def __init__(self, start, length, value: np.ndarray = None, score=0, field_type=
self.range_type = range_type.lower() # one of bit/hex/byte
- self.message_indices = set() if message_indices is None else set(message_indices)
+ self.message_indices = (
+ set() if message_indices is None else set(message_indices)
+ )
"""
Set of message indices, this range applies to
"""
@@ -46,7 +57,12 @@ def bit_start(self):
@property
def bit_end(self):
- return self.__convert_number(self.start) + self.__convert_number(self.length) - 1 + self.sync_end
+ return (
+ self.__convert_number(self.start)
+ + self.__convert_number(self.length)
+ - 1
+ + self.sync_end
+ )
@property
def length_in_bits(self):
@@ -85,9 +101,11 @@ def byte_order_is_unknown(self) -> bool:
return self.__byte_order is None
def matches(self, start: int, value: np.ndarray):
- return self.start == start and \
- self.length == len(value) and \
- self.value.tobytes() == value.tobytes()
+ return (
+ self.start == start
+ and self.length == len(value)
+ and self.value.tobytes() == value.tobytes()
+ )
def __convert_number(self, n):
if self.range_type == "bit":
@@ -100,22 +118,31 @@ def __convert_number(self, n):
raise ValueError("Unknown range type {}".format(self.range_type))
def __repr__(self):
- result = "{} {}-{} ({} {})".format(self.field_type, self.bit_start,
- self.bit_end, self.length, self.range_type)
+ result = "{} {}-{} ({} {})".format(
+ self.field_type, self.bit_start, self.bit_end, self.length, self.range_type
+ )
- result += " Values: " + " ".join(map(util.convert_numbers_to_hex_string, self.values))
+ result += " Values: " + " ".join(
+ map(util.convert_numbers_to_hex_string, self.values)
+ )
if self.score is not None:
result += " Score: " + str(self.score)
- result += " Message indices: {" + ",".join(map(str, sorted(self.message_indices))) + "}"
+ result += (
+ " Message indices: {"
+ + ",".join(map(str, sorted(self.message_indices)))
+ + "}"
+ )
return result
def __eq__(self, other):
if not isinstance(other, CommonRange):
return False
- return self.bit_start == other.bit_start and \
- self.bit_end == other.bit_end and \
- self.field_type == other.field_type
+ return (
+ self.bit_start == other.bit_start
+ and self.bit_end == other.bit_end
+ and self.field_type == other.field_type
+ )
def __hash__(self):
return hash((self.start, self.length, self.field_type))
@@ -126,8 +153,10 @@ def __lt__(self, other):
def overlaps_with(self, other) -> bool:
if not isinstance(other, CommonRange):
raise ValueError("Need another bit range to compare")
- return any(i in range(self.bit_start, self.bit_end)
- for i in range(other.bit_start, other.bit_end))
+ return any(
+ i in range(self.bit_start, self.bit_end)
+ for i in range(other.bit_start, other.bit_end)
+ )
def ensure_not_overlaps(self, start: int, end: int):
"""
@@ -145,14 +174,16 @@ def ensure_not_overlaps(self, start: int, end: int):
result = copy.deepcopy(self)
result.length -= end - result.start
result.start = end
- result.value = result.value[result.start-self.start:(result.start-self.start)+result.length]
+ result.value = result.value[
+ result.start - self.start : (result.start - self.start) + result.length
+ ]
return [result]
if self.start < start <= self.end <= end:
# overlaps on the right
result = copy.deepcopy(self)
result.length -= self.end + 1 - start
- result.value = result.value[:result.length]
+ result.value = result.value[: result.length]
return [result]
if self.start < start and self.end > end:
@@ -160,21 +191,36 @@ def ensure_not_overlaps(self, start: int, end: int):
left = copy.deepcopy(self)
right = copy.deepcopy(self)
- left.length -= (left.end + 1 - start)
- left.value = self.value[:left.length]
+ left.length -= left.end + 1 - start
+ left.value = self.value[: left.length]
right.start = end + 1
right.length = self.end - end
- right.value = self.value[right.start-self.start:(right.start-self.start)+right.length]
+ right.value = self.value[
+ right.start - self.start : (right.start - self.start) + right.length
+ ]
return [left, right]
return []
class ChecksumRange(CommonRange):
- def __init__(self, start, length, crc: GenericCRC, data_range_start, data_range_end, value: np.ndarray = None,
- score=0, field_type="Generic", message_indices=None, range_type="bit"):
- super().__init__(start, length, value, score, field_type, message_indices, range_type)
+ def __init__(
+ self,
+ start,
+ length,
+ crc: GenericCRC,
+ data_range_start,
+ data_range_end,
+ value: np.ndarray = None,
+ score=0,
+ field_type="Generic",
+ message_indices=None,
+ range_type="bit",
+ ):
+ super().__init__(
+ start, length, value, score, field_type, message_indices, range_type
+ )
self.data_range_start = data_range_start
self.data_range_end = data_range_end
self.crc = crc
@@ -188,18 +234,31 @@ def data_range_bit_end(self):
return self.data_range_end + self.sync_end
def __eq__(self, other):
- return super().__eq__(other) \
- and self.data_range_start == other.data_range_start \
- and self.data_range_end == other.data_range_end \
- and self.crc == other.crc
+ return (
+ super().__eq__(other)
+ and self.data_range_start == other.data_range_start
+ and self.data_range_end == other.data_range_end
+ and self.crc == other.crc
+ )
def __hash__(self):
- return hash((self.start, self.length, self.data_range_start, self.data_range_end, self.crc))
+ return hash(
+ (
+ self.start,
+ self.length,
+ self.data_range_start,
+ self.data_range_end,
+ self.crc,
+ )
+ )
def __repr__(self):
- return super().__repr__() + " \t" + \
- "{}".format(self.crc.caption) + \
- " Datarange: {}-{} ".format(self.data_range_start, self.data_range_end)
+ return (
+ super().__repr__()
+ + " \t"
+ + "{}".format(self.crc.caption)
+ + " Datarange: {}-{} ".format(self.data_range_start, self.data_range_end)
+ )
class EmptyCommonRange(CommonRange):
@@ -212,8 +271,9 @@ def __init__(self, field_type="Generic"):
self.field_type = field_type
def __eq__(self, other):
- return isinstance(other, EmptyCommonRange) \
- and other.field_type == self.field_type
+ return (
+ isinstance(other, EmptyCommonRange) and other.field_type == self.field_type
+ )
def __repr__(self):
return "No " + self.field_type
@@ -229,7 +289,6 @@ class CommonRangeContainer(object):
"""
def __init__(self, ranges: list, message_indices: set = None):
-
assert isinstance(ranges, list)
self.__ranges = ranges # type: list[CommonRange]
@@ -287,10 +346,14 @@ def __getitem__(self, item):
def __repr__(self):
from pprint import pformat
+
return pformat(self.__ranges)
def __eq__(self, other):
if not isinstance(other, CommonRangeContainer):
return False
- return self.__ranges == other.__ranges and self.message_indices == other.message_indices
+ return (
+ self.__ranges == other.__ranges
+ and self.message_indices == other.message_indices
+ )
diff --git a/src/urh/awre/FormatFinder.py b/src/urh/awre/FormatFinder.py
index ae2be2d8f2..79c39fef26 100644
--- a/src/urh/awre/FormatFinder.py
+++ b/src/urh/awre/FormatFinder.py
@@ -5,7 +5,12 @@
import numpy as np
from urh.awre import AutoAssigner
-from urh.awre.CommonRange import CommonRange, EmptyCommonRange, CommonRangeContainer, ChecksumRange
+from urh.awre.CommonRange import (
+ CommonRange,
+ EmptyCommonRange,
+ CommonRangeContainer,
+ ChecksumRange,
+)
from urh.awre.Preprocessor import Preprocessor
from urh.awre.engines.AddressEngine import AddressEngine
from urh.awre.engines.ChecksumEngine import ChecksumEngine
@@ -32,25 +37,43 @@ def __init__(self, messages, participants=None, shortest_field_length=None):
if participants is not None:
AutoAssigner.auto_assign_participants(messages, participants)
- existing_message_types_by_msg = {i: msg.message_type for i, msg in enumerate(messages)}
+ existing_message_types_by_msg = {
+ i: msg.message_type for i, msg in enumerate(messages)
+ }
self.existing_message_types = defaultdict(list)
for i, message_type in existing_message_types_by_msg.items():
self.existing_message_types[message_type].append(i)
- preprocessor = Preprocessor(self.get_bitvectors_from_messages(messages), existing_message_types_by_msg)
- self.preamble_starts, self.preamble_lengths, sync_len = preprocessor.preprocess()
+ preprocessor = Preprocessor(
+ self.get_bitvectors_from_messages(messages), existing_message_types_by_msg
+ )
+ (
+ self.preamble_starts,
+ self.preamble_lengths,
+ sync_len,
+ ) = preprocessor.preprocess()
self.sync_ends = self.preamble_starts + self.preamble_lengths + sync_len
n = shortest_field_length
if n is None:
# 0 = no sync found
- n = 8 if sync_len >= 8 else 4 if sync_len >= 4 else 1 if sync_len >= 1 else 0
+ n = (
+ 8
+ if sync_len >= 8
+ else 4
+ if sync_len >= 4
+ else 1
+ if sync_len >= 1
+ else 0
+ )
for i, value in enumerate(self.sync_ends):
# In doubt it is better to under estimate the sync end
if n > 0:
- self.sync_ends[i] = n * max(int(math.floor((value - self.preamble_starts[i]) / n)), 1) + \
- self.preamble_starts[i]
+ self.sync_ends[i] = (
+ n * max(int(math.floor((value - self.preamble_starts[i]) / n)), 1)
+ + self.preamble_starts[i]
+ )
else:
self.sync_ends[i] = self.preamble_starts[i]
@@ -61,12 +84,21 @@ def __init__(self, messages, participants=None, shortest_field_length=None):
self.hexvectors = self.get_hexvectors(self.bitvectors)
self.current_iteration = 0
- participants = list(sorted(set(msg.participant for msg in messages if msg.participant is not None)))
- self.participant_indices = [participants.index(msg.participant) if msg.participant is not None else -1
- for msg in messages]
+ participants = list(
+ sorted(
+ set(msg.participant for msg in messages if msg.participant is not None)
+ )
+ )
+ self.participant_indices = [
+ participants.index(msg.participant) if msg.participant is not None else -1
+ for msg in messages
+ ]
self.known_participant_addresses = {
- participants.index(p): np.array([int(h, 16) for h in p.address_hex], dtype=np.uint8)
- for p in participants if p and p.address_hex
+ participants.index(p): np.array(
+ [int(h, 16) for h in p.address_hex], dtype=np.uint8
+ )
+ for p in participants
+ if p and p.address_hex
}
@property
@@ -92,34 +124,65 @@ def perform_iteration_for_message_type(self, message_type: MessageType):
# because if the existing labels would have different sync positions,
# they would not belong to the same message type in the first place
sync_end = self.sync_ends[indices[0]] if indices else 0
- already_labeled = [(lbl.start - sync_end, lbl.end - sync_end) for lbl in message_type if lbl.start >= sync_end]
+ already_labeled = [
+ (lbl.start - sync_end, lbl.end - sync_end)
+ for lbl in message_type
+ if lbl.start >= sync_end
+ ]
if not message_type.get_first_label_with_type(FieldType.Function.LENGTH):
- engines.append(LengthEngine([self.bitvectors[i] for i in indices], already_labeled=already_labeled))
+ engines.append(
+ LengthEngine(
+ [self.bitvectors[i] for i in indices],
+ already_labeled=already_labeled,
+ )
+ )
if not message_type.get_first_label_with_type(FieldType.Function.SRC_ADDRESS):
- engines.append(AddressEngine([self.hexvectors[i] for i in indices],
- [self.participant_indices[i] for i in indices],
- self.known_participant_addresses,
- already_labeled=already_labeled))
+ engines.append(
+ AddressEngine(
+ [self.hexvectors[i] for i in indices],
+ [self.participant_indices[i] for i in indices],
+ self.known_participant_addresses,
+ already_labeled=already_labeled,
+ )
+ )
elif not message_type.get_first_label_with_type(FieldType.Function.DST_ADDRESS):
- engines.append(AddressEngine([self.hexvectors[i] for i in indices],
- [self.participant_indices[i] for i in indices],
- self.known_participant_addresses,
- already_labeled=already_labeled,
- src_field_present=True))
-
- if not message_type.get_first_label_with_type(FieldType.Function.SEQUENCE_NUMBER):
- engines.append(SequenceNumberEngine([self.bitvectors[i] for i in indices], already_labeled=already_labeled))
+ engines.append(
+ AddressEngine(
+ [self.hexvectors[i] for i in indices],
+ [self.participant_indices[i] for i in indices],
+ self.known_participant_addresses,
+ already_labeled=already_labeled,
+ src_field_present=True,
+ )
+ )
+
+ if not message_type.get_first_label_with_type(
+ FieldType.Function.SEQUENCE_NUMBER
+ ):
+ engines.append(
+ SequenceNumberEngine(
+ [self.bitvectors[i] for i in indices],
+ already_labeled=already_labeled,
+ )
+ )
if not message_type.get_first_label_with_type(FieldType.Function.CHECKSUM):
# If checksum was not found in first iteration, it will also not be found in next one
if self.current_iteration == 0:
- engines.append(ChecksumEngine([self.bitvectors[i] for i in indices], already_labeled=already_labeled))
+ engines.append(
+ ChecksumEngine(
+ [self.bitvectors[i] for i in indices],
+ already_labeled=already_labeled,
+ )
+ )
result = set()
for engine in engines:
high_scored_ranges = engine.find() # type: list[CommonRange]
- high_scored_ranges = self.retransform_message_indices(high_scored_ranges, indices, self.sync_ends)
+ high_scored_ranges = self.retransform_message_indices(
+ high_scored_ranges, indices, self.sync_ends
+ )
merged_ranges = self.merge_common_ranges(high_scored_ranges)
result.update(merged_ranges)
return result
@@ -128,22 +191,39 @@ def perform_iteration(self) -> bool:
new_field_found = False
for message_type in self.existing_message_types.copy():
- new_fields_for_message_type = self.perform_iteration_for_message_type(message_type)
+ new_fields_for_message_type = self.perform_iteration_for_message_type(
+ message_type
+ )
new_fields_for_message_type.update(
- self.get_preamble_and_sync(self.preamble_starts, self.preamble_lengths, self.sync_ends,
- message_type_indices=self.existing_message_types[message_type])
+ self.get_preamble_and_sync(
+ self.preamble_starts,
+ self.preamble_lengths,
+ self.sync_ends,
+ message_type_indices=self.existing_message_types[message_type],
+ )
)
self.remove_overlapping_fields(new_fields_for_message_type, message_type)
- containers = self.create_common_range_containers(new_fields_for_message_type)
+ containers = self.create_common_range_containers(
+ new_fields_for_message_type
+ )
# Store addresses of participants if we found a SRC address field
- participants_with_unknown_address = set(self.participant_indices) - set(self.known_participant_addresses)
+ participants_with_unknown_address = set(self.participant_indices) - set(
+ self.known_participant_addresses
+ )
participants_with_unknown_address.discard(-1)
if participants_with_unknown_address:
for container in containers:
- src_range = next((rng for rng in container if rng.field_type == "source address"), None)
+ src_range = next(
+ (
+ rng
+ for rng in container
+ if rng.field_type == "source address"
+ ),
+ None,
+ )
if src_range is None:
continue
for msg_index in src_range.message_indices:
@@ -152,7 +232,9 @@ def perform_iteration(self) -> bool:
p = self.participant_indices[msg_index]
if p not in self.known_participant_addresses:
hex_vector = self.hexvectors[msg_index]
- self.known_participant_addresses[p] = hex_vector[src_range.start:src_range.end + 1]
+ self.known_participant_addresses[p] = hex_vector[
+ src_range.start : src_range.end + 1
+ ]
participants_with_unknown_address.discard(p)
new_field_found |= len(containers) > 0
@@ -167,13 +249,17 @@ def perform_iteration(self) -> bool:
new_message_type = copy.deepcopy(message_type) # type: MessageType
if i > 0:
- new_message_type.name = "Message Type {}.{}".format(self.current_iteration+1, i)
+ new_message_type.name = "Message Type {}.{}".format(
+ self.current_iteration + 1, i
+ )
new_message_type.give_new_id()
for rng in container:
self.add_range_to_message_type(rng, new_message_type)
- self.existing_message_types[new_message_type].extend(sorted(container.message_indices))
+ self.existing_message_types[new_message_type].extend(
+ sorted(container.message_indices)
+ )
return new_field_found
@@ -184,11 +270,13 @@ def run(self, max_iterations=10):
if len(self.message_types) > 0:
messages_without_message_type = set(range(len(self.bitvectors))) - set(
- i for l in self.existing_message_types.values() for i in l)
+ i for l in self.existing_message_types.values() for i in l
+ )
# add to default message type
- self.existing_message_types[self.message_types[0]].extend(list(messages_without_message_type))
-
+ self.existing_message_types[self.message_types[0]].extend(
+ list(messages_without_message_type)
+ )
@staticmethod
def remove_overlapping_fields(common_ranges, message_type: MessageType):
@@ -204,7 +292,10 @@ def remove_overlapping_fields(common_ranges, message_type: MessageType):
for rng in common_ranges.copy():
for lbl in message_type: # type: ProtocolLabel
- if any(i in range(rng.bit_start, rng.bit_end) for i in range(lbl.start, lbl.end)):
+ if any(
+ i in range(rng.bit_start, rng.bit_end)
+ for i in range(lbl.start, lbl.end)
+ ):
common_ranges.discard(rng)
break
@@ -220,10 +311,13 @@ def merge_common_ranges(common_ranges):
for common_range in common_ranges:
assert isinstance(common_range, CommonRange)
try:
- same_range = next(rng for rng in merged_ranges
- if rng.bit_start == common_range.bit_start
- and rng.bit_end == common_range.bit_end
- and rng.field_type == common_range.field_type)
+ same_range = next(
+ rng
+ for rng in merged_ranges
+ if rng.bit_start == common_range.bit_start
+ and rng.bit_end == common_range.bit_end
+ and rng.field_type == common_range.field_type
+ )
same_range.values.extend(common_range.values)
same_range.message_indices.update(common_range.message_indices)
except StopIteration:
@@ -234,17 +328,21 @@ def merge_common_ranges(common_ranges):
@staticmethod
def add_range_to_message_type(common_range: CommonRange, message_type: MessageType):
field_type = FieldType.from_caption(common_range.field_type)
- label = message_type.add_protocol_label(name=common_range.field_type,
- start=common_range.bit_start, end=common_range.bit_end,
- auto_created=True,
- type=field_type
- )
+ label = message_type.add_protocol_label(
+ name=common_range.field_type,
+ start=common_range.bit_start,
+ end=common_range.bit_end,
+ auto_created=True,
+ type=field_type,
+ )
label.display_endianness = common_range.byte_order
if field_type.function == FieldType.Function.CHECKSUM:
assert isinstance(label, ChecksumLabel)
assert isinstance(common_range, ChecksumRange)
- label.data_ranges = [[common_range.data_range_bit_start, common_range.data_range_bit_end]]
+ label.data_ranges = [
+ [common_range.data_range_bit_start, common_range.data_range_bit_end]
+ ]
if isinstance(common_range.crc, WSPChecksum):
label.category = ChecksumLabel.Category.wsp
@@ -261,7 +359,10 @@ def get_bitvectors_from_messages(messages: list, sync_ends: np.ndarray = None):
if sync_ends is None:
sync_ends = defaultdict(lambda: None)
- return [np.array(msg.decoded_bits[sync_ends[i]:], dtype=np.uint8, order="C") for i, msg in enumerate(messages)]
+ return [
+ np.array(msg.decoded_bits[sync_ends[i] :], dtype=np.uint8, order="C")
+ for i, msg in enumerate(messages)
+ ]
@staticmethod
def create_common_range_containers(label_set: set, num_messages: int = None):
@@ -275,16 +376,31 @@ def create_common_range_containers(label_set: set, num_messages: int = None):
:rtype: list of CommonRangeContainer
"""
if num_messages is None:
- message_indices = sorted(set(i for rng in label_set for i in rng.message_indices))
+ message_indices = sorted(
+ set(i for rng in label_set for i in rng.message_indices)
+ )
else:
message_indices = range(num_messages)
result = []
for i in message_indices:
- labels = sorted(set(rng for rng in label_set if i in rng.message_indices
- and not isinstance(rng, EmptyCommonRange)))
+ labels = sorted(
+ set(
+ rng
+ for rng in label_set
+ if i in rng.message_indices
+ and not isinstance(rng, EmptyCommonRange)
+ )
+ )
- container = next((container for container in result if container.has_same_ranges(labels)), None)
+ container = next(
+ (
+ container
+ for container in result
+ if container.has_same_ranges(labels)
+ ),
+ None,
+ )
if container is None:
result.append(CommonRangeContainer(labels, message_indices={i}))
else:
@@ -305,13 +421,21 @@ def handle_overlapping_conflict(containers):
result = []
for container in containers:
if container.ranges_overlap:
- conflicted_handled = FormatFinder.__handle_container_overlapping_conflict(container)
+ conflicted_handled = (
+ FormatFinder.__handle_container_overlapping_conflict(container)
+ )
else:
conflicted_handled = container
try:
- same_rng_container = next(c for c in result if c.has_same_ranges_as_container(conflicted_handled))
- same_rng_container.message_indices.update(conflicted_handled.message_indices)
+ same_rng_container = next(
+ c
+ for c in result
+ if c.has_same_ranges_as_container(conflicted_handled)
+ )
+ same_rng_container.message_indices.update(
+ conflicted_handled.message_indices
+ )
except StopIteration:
result.append(conflicted_handled)
@@ -356,23 +480,31 @@ def __handle_container_overlapping_conflict(container: CommonRangeContainer):
possible_solutions = []
for i, rng in enumerate(partition):
# Append every range to this solution that does not overlap with current rng
- solution = [rng] + [r for r in partition[i + 1:] if not rng.overlaps_with(r)]
+ solution = [rng] + [
+ r for r in partition[i + 1 :] if not rng.overlaps_with(r)
+ ]
possible_solutions.append(solution)
# Take solution that maximizes score. In case of tie, choose solution with shorter total length.
# if there is still a tie prefer solution that contains a length field as is is very likely to be correct
# if nothing else helps break tie by names of field types to prevent randomness
- best_solution = max(possible_solutions,
- key=lambda sol: (sum(r.score for r in sol),
- -sum(r.length_in_bits for r in sol),
- "length" in {r.field_type for r in sol},
- "".join(r.field_type[0] for r in sol)))
+ best_solution = max(
+ possible_solutions,
+ key=lambda sol: (
+ sum(r.score for r in sol),
+ -sum(r.length_in_bits for r in sol),
+ "length" in {r.field_type for r in sol},
+ "".join(r.field_type[0] for r in sol),
+ ),
+ )
result.extend(best_solution)
return CommonRangeContainer(result, message_indices=container.message_indices)
@staticmethod
- def retransform_message_indices(common_ranges, message_type_indices: list, sync_ends) -> list:
+ def retransform_message_indices(
+ common_ranges, message_type_indices: list, sync_ends
+ ) -> list:
"""
Retransform the found message indices of an engine to the original index space
based on the message indices of the message type.
@@ -388,21 +520,28 @@ def retransform_message_indices(common_ranges, message_type_indices: list, sync_
result = []
for common_range in common_ranges:
# Retransform message indices into original space
- message_indices = np.fromiter((message_type_indices[i] for i in common_range.message_indices),
- dtype=int, count=len(common_range.message_indices))
+ message_indices = np.fromiter(
+ (message_type_indices[i] for i in common_range.message_indices),
+ dtype=int,
+ count=len(common_range.message_indices),
+ )
# If we have different sync_ends we need to create a new common range for each different sync_length
matching_sync_ends = sync_ends[message_indices]
for sync_end in np.unique(matching_sync_ends):
rng = copy.deepcopy(common_range)
rng.sync_end = sync_end
- rng.message_indices = set(message_indices[np.nonzero(matching_sync_ends == sync_end)])
+ rng.message_indices = set(
+ message_indices[np.nonzero(matching_sync_ends == sync_end)]
+ )
result.append(rng)
return result
@staticmethod
- def get_preamble_and_sync(preamble_starts, preamble_lengths, sync_ends, message_type_indices):
+ def get_preamble_and_sync(
+ preamble_starts, preamble_lengths, sync_ends, message_type_indices
+ ):
"""
Get preamble and sync common ranges based on the data
@@ -416,7 +555,12 @@ def get_preamble_and_sync(preamble_starts, preamble_lengths, sync_ends, message_
result = set() # type: set[CommonRange]
for i in message_type_indices:
- preamble = CommonRange(preamble_starts[i], preamble_lengths[i], field_type="preamble", message_indices={i})
+ preamble = CommonRange(
+ preamble_starts[i],
+ preamble_lengths[i],
+ field_type="preamble",
+ message_indices={i},
+ )
existing_preamble = next((rng for rng in result if preamble == rng), None)
if existing_preamble is not None:
existing_preamble.message_indices.add(i)
@@ -425,7 +569,12 @@ def get_preamble_and_sync(preamble_starts, preamble_lengths, sync_ends, message_
preamble_end = preamble_starts[i] + preamble_lengths[i]
sync_end = sync_ends[i]
- sync = CommonRange(preamble_end, sync_end - preamble_end, field_type="synchronization", message_indices={i})
+ sync = CommonRange(
+ preamble_end,
+ sync_end - preamble_end,
+ field_type="synchronization",
+ message_indices={i},
+ )
existing_sync = next((rng for rng in result if sync == rng), None)
if existing_sync is not None:
existing_sync.message_indices.add(i)
diff --git a/src/urh/awre/Histogram.py b/src/urh/awre/Histogram.py
index b863de6cea..8bf6661778 100644
--- a/src/urh/awre/Histogram.py
+++ b/src/urh/awre/Histogram.py
@@ -22,13 +22,17 @@ def __init__(self, vectors, indices=None, normalize=True, debug=False):
:param normalize:
"""
self.__vectors = vectors # type: list[np.ndarray]
- self.__active_indices = list(range(len(vectors))) if indices is None else indices
+ self.__active_indices = (
+ list(range(len(vectors))) if indices is None else indices
+ )
self.normalize = normalize
self.data = self.__create_histogram()
def __create_histogram(self):
- return awre_util.create_difference_histogram(self.__vectors, self.__active_indices)
+ return awre_util.create_difference_histogram(
+ self.__vectors, self.__active_indices
+ )
def __repr__(self):
return str(self.data.tolist())
@@ -58,15 +62,29 @@ def find_common_ranges(self, alpha=0.95, range_type="bit"):
else:
if length >= 2:
value = self.__get_value_for_common_range(start, length)
- result.append(CommonRange(start, length, value, message_indices=set(self.__active_indices),
- range_type=range_type))
+ result.append(
+ CommonRange(
+ start,
+ length,
+ value,
+ message_indices=set(self.__active_indices),
+ range_type=range_type,
+ )
+ )
start, length = None, 0
if i == len(data_indices) - 1 and length >= 2:
value = self.__get_value_for_common_range(start, length)
- result.append(CommonRange(start, length, value, message_indices=set(self.__active_indices),
- range_type=range_type))
+ result.append(
+ CommonRange(
+ start,
+ length,
+ value,
+ message_indices=set(self.__active_indices),
+ range_type=range_type,
+ )
+ )
return result
@@ -81,10 +99,10 @@ def __get_value_for_common_range(self, start: int, length: int):
values = defaultdict(list)
for i in self.__active_indices:
vector = self.__vectors[i]
- values[vector[start:start + length].tostring()].append(i)
+ values[vector[start : start + length].tostring()].append(i)
value = max(values, key=lambda x: len(x))
indices = values[value]
- return self.__vectors[indices[0]][start:start + length]
+ return self.__vectors[indices[0]][start : start + length]
def __vector_to_string(self, data_vector) -> str:
lut = {i: "{0:x}".format(i) for i in range(16)}
@@ -92,6 +110,7 @@ def __vector_to_string(self, data_vector) -> str:
def plot(self):
import matplotlib.pyplot as plt
+
self.subplot_on(plt)
plt.show()
diff --git a/src/urh/awre/MessageTypeBuilder.py b/src/urh/awre/MessageTypeBuilder.py
index a35a02a577..5190887e0e 100644
--- a/src/urh/awre/MessageTypeBuilder.py
+++ b/src/urh/awre/MessageTypeBuilder.py
@@ -10,7 +10,7 @@ def __init__(self, name: str):
self.name = name
self.message_type = MessageType(name)
- def add_label(self, label_type: FieldType.Function, length: int, name: str=None):
+ def add_label(self, label_type: FieldType.Function, length: int, name: str = None):
try:
start = self.message_type[-1].end
color_index = self.message_type[-1].color_index + 1
@@ -20,10 +20,18 @@ def add_label(self, label_type: FieldType.Function, length: int, name: str=None)
if name is None:
name = label_type.value
- lbl = ProtocolLabel(name, start, start+length-1, color_index, field_type=FieldType(label_type.name, label_type))
+ lbl = ProtocolLabel(
+ name,
+ start,
+ start + length - 1,
+ color_index,
+ field_type=FieldType(label_type.name, label_type),
+ )
self.message_type.append(lbl)
- def add_checksum_label(self, length, checksum, data_start=None, data_end=None, name: str=None):
+ def add_checksum_label(
+ self, length, checksum, data_start=None, data_end=None, name: str = None
+ ):
label_type = FieldType.Function.CHECKSUM
try:
start = self.message_type[-1].end
@@ -36,11 +44,15 @@ def add_checksum_label(self, length, checksum, data_start=None, data_end=None, n
if data_start is None:
# End of sync or preamble
- sync_label = self.message_type.get_first_label_with_type(FieldType.Function.SYNC)
+ sync_label = self.message_type.get_first_label_with_type(
+ FieldType.Function.SYNC
+ )
if sync_label:
data_start = sync_label.end
else:
- preamble_label = self.message_type.get_first_label_with_type(FieldType.Function.PREAMBLE)
+ preamble_label = self.message_type.get_first_label_with_type(
+ FieldType.Function.PREAMBLE
+ )
if preamble_label:
data_start = preamble_label.end
else:
@@ -49,7 +61,13 @@ def add_checksum_label(self, length, checksum, data_start=None, data_end=None, n
if data_end is None:
data_end = start
- lbl = ChecksumLabel(name, start, start+length-1, color_index, field_type=FieldType(label_type.name, label_type))
+ lbl = ChecksumLabel(
+ name,
+ start,
+ start + length - 1,
+ color_index,
+ field_type=FieldType(label_type.name, label_type),
+ )
lbl.data_ranges = [(data_start, data_end)]
lbl.checksum = checksum
self.message_type.append(lbl)
diff --git a/src/urh/awre/Preprocessor.py b/src/urh/awre/Preprocessor.py
index 0747899827..9c65ef0aa6 100644
--- a/src/urh/awre/Preprocessor.py
+++ b/src/urh/awre/Preprocessor.py
@@ -22,7 +22,9 @@ class Preprocessor(object):
def __init__(self, bitvectors: list, existing_message_types: dict = None):
self.bitvectors = bitvectors # type: list[np.ndarray]
- self.existing_message_types = existing_message_types if existing_message_types is not None else dict()
+ self.existing_message_types = (
+ existing_message_types if existing_message_types is not None else dict()
+ )
def preprocess(self) -> (np.ndarray, int):
raw_preamble_positions = self.get_raw_preamble_positions()
@@ -34,11 +36,15 @@ def preprocess(self) -> (np.ndarray, int):
sync_words = existing_sync_words
preamble_starts = raw_preamble_positions[:, 0]
- preamble_lengths = self.get_preamble_lengths_from_sync_words(sync_words, preamble_starts=preamble_starts)
+ preamble_lengths = self.get_preamble_lengths_from_sync_words(
+ sync_words, preamble_starts=preamble_starts
+ )
sync_len = len(sync_words[0]) if len(sync_words) > 0 else 0
return preamble_starts, preamble_lengths, sync_len
- def get_preamble_lengths_from_sync_words(self, sync_words: list, preamble_starts: np.ndarray):
+ def get_preamble_lengths_from_sync_words(
+ self, sync_words: list, preamble_starts: np.ndarray
+ ):
"""
Get the preamble lengths based on the found sync words for all messages.
If there should be more than one sync word in a message, use the first one.
@@ -65,7 +71,9 @@ def get_preamble_lengths_from_sync_words(self, sync_words: list, preamble_starts
preamble_lengths.append(sync_start - preamble_starts[i])
# Consider case where sync word starts with preamble pattern
- sync_start = bits.find(sync_word, sync_start + 1, sync_start + 2 * len(sync_word))
+ sync_start = bits.find(
+ sync_word, sync_start + 1, sync_start + 2 * len(sync_word)
+ )
if sync_start != -1:
if sync_start - preamble_starts[i] >= 2:
@@ -79,12 +87,18 @@ def get_preamble_lengths_from_sync_words(self, sync_words: list, preamble_starts
result[i] = preamble_lengths[0]
else:
# consider all indices not more than one byte before first one
- preamble_lengths = list(filter(lambda x: x < preamble_lengths[0] + 7, preamble_lengths))
+ preamble_lengths = list(
+ filter(lambda x: x < preamble_lengths[0] + 7, preamble_lengths)
+ )
# take the smallest preamble_length, but prefer a greater one if it is divisible by 8 (or 4)
- preamble_length = next((pl for pl in preamble_lengths if pl % 8 == 0), None)
+ preamble_length = next(
+ (pl for pl in preamble_lengths if pl % 8 == 0), None
+ )
if preamble_length is None:
- preamble_length = next((pl for pl in preamble_lengths if pl % 4 == 0), None)
+ preamble_length = next(
+ (pl for pl in preamble_lengths if pl % 4 == 0), None
+ )
if preamble_length is None:
preamble_length = preamble_lengths[0]
result[i] = preamble_length
@@ -95,7 +109,9 @@ def find_possible_syncs(self, raw_preamble_positions=None):
difference_matrix = self.get_difference_matrix()
if raw_preamble_positions is None:
raw_preamble_positions = self.get_raw_preamble_positions()
- return self.determine_sync_candidates(raw_preamble_positions, difference_matrix, n_gram_length=4)
+ return self.determine_sync_candidates(
+ raw_preamble_positions, difference_matrix, n_gram_length=4
+ )
@staticmethod
def merge_possible_sync_words(possible_sync_words: dict, n_gram_length: int):
@@ -112,25 +128,31 @@ def merge_possible_sync_words(possible_sync_words: dict, n_gram_length: int):
for sync1, sync2 in itertools.combinations(possible_sync_words, 2):
common_prefix = os.path.commonprefix([sync1, sync2])
if len(common_prefix) > n_gram_length:
- result[common_prefix] += possible_sync_words[sync1] + possible_sync_words[sync2]
+ result[common_prefix] += (
+ possible_sync_words[sync1] + possible_sync_words[sync2]
+ )
else:
result[sync1] += possible_sync_words[sync1]
result[sync2] += possible_sync_words[sync2]
return result
- def determine_sync_candidates(self,
- raw_preamble_positions: np.ndarray,
- difference_matrix: np.ndarray,
- n_gram_length=4) -> list:
-
- possible_sync_words = awre_util.find_possible_sync_words(difference_matrix, raw_preamble_positions,
- self.bitvectors, n_gram_length)
+ def determine_sync_candidates(
+ self,
+ raw_preamble_positions: np.ndarray,
+ difference_matrix: np.ndarray,
+ n_gram_length=4,
+ ) -> list:
+ possible_sync_words = awre_util.find_possible_sync_words(
+ difference_matrix, raw_preamble_positions, self.bitvectors, n_gram_length
+ )
self.__debug("Possible sync words", possible_sync_words)
if len(possible_sync_words) == 0:
return []
- possible_sync_words = self.merge_possible_sync_words(possible_sync_words, n_gram_length)
+ possible_sync_words = self.merge_possible_sync_words(
+ possible_sync_words, n_gram_length
+ )
self.__debug("Merged sync words", possible_sync_words)
scores = self.__score_sync_lengths(possible_sync_words)
@@ -138,17 +160,24 @@ def determine_sync_candidates(self,
sorted_scores = sorted(scores, reverse=True, key=scores.get)
estimated_sync_length = sorted_scores[0]
if estimated_sync_length % 8 != 0:
- for other in filter(lambda x: 0 < estimated_sync_length-x < 7, sorted_scores):
+ for other in filter(
+ lambda x: 0 < estimated_sync_length - x < 7, sorted_scores
+ ):
if other % 8 == 0:
estimated_sync_length = other
break
# Now we look at all possible sync words with this length
- sync_words = {word: frequency for word, frequency in possible_sync_words.items()
- if len(word) == estimated_sync_length}
+ sync_words = {
+ word: frequency
+ for word, frequency in possible_sync_words.items()
+ if len(word) == estimated_sync_length
+ }
self.__debug("Sync words", sync_words)
- additional_syncs = self.__find_additional_sync_words(estimated_sync_length, sync_words, possible_sync_words)
+ additional_syncs = self.__find_additional_sync_words(
+ estimated_sync_length, sync_words, possible_sync_words
+ )
if additional_syncs:
self.__debug("Found additional sync words", additional_syncs)
@@ -161,7 +190,9 @@ def determine_sync_candidates(self,
return result
- def __find_additional_sync_words(self, sync_length: int, present_sync_words, possible_sync_words) -> dict:
+ def __find_additional_sync_words(
+ self, sync_length: int, present_sync_words, possible_sync_words
+ ) -> dict:
"""
Look for additional sync words, in case we had varying preamble lengths and multiple sync words
(see test_with_three_syncs_different_preamble_lengths for an example)
@@ -171,29 +202,48 @@ def __find_additional_sync_words(self, sync_length: int, present_sync_words, pos
:type possible_sync_words: dict
:return:
"""
- np_syn = [np.fromiter(map(int, sync_word), dtype=np.uint8, count=len(sync_word))
- for sync_word in present_sync_words]
-
- messages_without_sync = [i for i, bv in enumerate(self.bitvectors)
- if not any(awre_util.find_occurrences(bv, s, return_after_first=True) for s in np_syn)]
+ np_syn = [
+ np.fromiter(map(int, sync_word), dtype=np.uint8, count=len(sync_word))
+ for sync_word in present_sync_words
+ ]
+
+ messages_without_sync = [
+ i
+ for i, bv in enumerate(self.bitvectors)
+ if not any(
+ awre_util.find_occurrences(bv, s, return_after_first=True)
+ for s in np_syn
+ )
+ ]
result = dict()
if len(messages_without_sync) == 0:
return result
# Is there another sync word that applies to all messages without sync?
- additional_candidates = {word: score for word, score in possible_sync_words.items()
- if len(word) > sync_length and not any(s in word for s in present_sync_words)}
-
- for sync in sorted(additional_candidates, key=additional_candidates.get, reverse=True):
+ additional_candidates = {
+ word: score
+ for word, score in possible_sync_words.items()
+ if len(word) > sync_length
+ and not any(s in word for s in present_sync_words)
+ }
+
+ for sync in sorted(
+ additional_candidates, key=additional_candidates.get, reverse=True
+ ):
if len(messages_without_sync) == 0:
break
score = additional_candidates[sync]
s = sync[:sync_length]
np_s = np.fromiter(s, dtype=np.uint8, count=len(s))
- matching = [i for i in messages_without_sync
- if awre_util.find_occurrences(self.bitvectors[i], np_s, return_after_first=True)]
+ matching = [
+ i
+ for i in messages_without_sync
+ if awre_util.find_occurrences(
+ self.bitvectors[i], np_s, return_after_first=True
+ )
+ ]
if matching:
result[s] = score
for m in matching:
@@ -210,7 +260,9 @@ def get_raw_preamble_positions(self) -> np.ndarray:
for i, bitvector in enumerate(self.bitvectors):
if i in self.existing_message_types:
- preamble_label = self.existing_message_types[i].get_first_label_with_type(FieldType.Function.PREAMBLE)
+ preamble_label = self.existing_message_types[
+ i
+ ].get_first_label_with_type(FieldType.Function.PREAMBLE)
else:
preamble_label = None
@@ -218,7 +270,11 @@ def get_raw_preamble_positions(self) -> np.ndarray:
start, lower, upper = awre_util.get_raw_preamble_position(bitvector)
else:
# If this message is already labeled with a preamble we just use it's values
- start, lower, upper = preamble_label.start, preamble_label.end, preamble_label.end
+ start, lower, upper = (
+ preamble_label.start,
+ preamble_label.end,
+ preamble_label.end,
+ )
result[i, 0] = start
result[i, 1] = lower - start
@@ -246,12 +302,16 @@ def __get_existing_sync_words(self) -> list:
result = []
for i, bitvector in enumerate(self.bitvectors):
if i in self.existing_message_types:
- sync_label = self.existing_message_types[i].get_first_label_with_type(FieldType.Function.SYNC)
+ sync_label = self.existing_message_types[i].get_first_label_with_type(
+ FieldType.Function.SYNC
+ )
else:
sync_label = None
if sync_label is not None:
- result.append("".join(map(str, bitvector[sync_label.start:sync_label.end])))
+ result.append(
+ "".join(map(str, bitvector[sync_label.start : sync_label.end]))
+ )
return result
def __debug(self, *args):
diff --git a/src/urh/awre/ProtocolGenerator.py b/src/urh/awre/ProtocolGenerator.py
index b17003daa2..6643775828 100644
--- a/src/urh/awre/ProtocolGenerator.py
+++ b/src/urh/awre/ProtocolGenerator.py
@@ -20,9 +20,18 @@ class ProtocolGenerator(object):
DEFAULT_SYNC = "1001"
BROADCAST_ADDRESS = "0xffff"
- def __init__(self, message_types: list, participants: list = None, preambles_by_mt=None,
- syncs_by_mt=None, little_endian=False, length_in_bytes=True, sequence_numbers=None,
- sequence_number_increment=1, message_type_codes=None):
+ def __init__(
+ self,
+ message_types: list,
+ participants: list = None,
+ preambles_by_mt=None,
+ syncs_by_mt=None,
+ little_endian=False,
+ length_in_bytes=True,
+ sequence_numbers=None,
+ sequence_number_increment=1,
+ message_type_codes=None,
+ ):
"""
:param message_types:
@@ -65,7 +74,6 @@ def __init__(self, message_types: list, participants: list = None, preambles_by_
message_type_codes[mt] = i
self.message_type_codes = message_type_codes
-
@property
def messages(self):
return self.protocol.messages
@@ -78,8 +86,11 @@ def __get_address_for_participant(self, participant: Participant):
if participant is None:
return self.to_bits(self.BROADCAST_ADDRESS)
- address = "0x" + participant.address_hex if not participant.address_hex.startswith(
- "0x") else participant.address_hex
+ address = (
+ "0x" + participant.address_hex
+ if not participant.address_hex.startswith("0x")
+ else participant.address_hex
+ )
return self.to_bits(address)
@staticmethod
@@ -93,7 +104,9 @@ def to_bits(bit_or_hex_str: str):
def decimal_to_bits(self, number: int, num_bits: int) -> str:
len_formats = {8: "B", 16: "H", 32: "I", 64: "Q"}
if num_bits not in len_formats:
- raise ValueError("Invalid length for length field: {} bits".format(num_bits))
+ raise ValueError(
+ "Invalid length for length field: {} bits".format(num_bits)
+ )
struct_format = "<" if self.little_endian else ">"
struct_format += len_formats[num_bits]
@@ -101,10 +114,18 @@ def decimal_to_bits(self, number: int, num_bits: int) -> str:
byte_length = struct.pack(struct_format, number)
return "".join("{0:08b}".format(byte) for byte in byte_length)
- def generate_message(self, message_type=None, data="0x00", source: Participant = None,
- destination: Participant = None):
+ def generate_message(
+ self,
+ message_type=None,
+ data="0x00",
+ source: Participant = None,
+ destination: Participant = None,
+ ):
for participant in (source, destination):
- if isinstance(participant, Participant) and participant not in self.participants:
+ if (
+ isinstance(participant, Participant)
+ and participant not in self.participants
+ ):
self.participants.append(participant)
if isinstance(message_type, MessageType):
@@ -123,7 +144,9 @@ def generate_message(self, message_type=None, data="0x00", source: Participant =
start = 0
- data_label_present = mt.get_first_label_with_type(FieldType.Function.DATA) is not None
+ data_label_present = (
+ mt.get_first_label_with_type(FieldType.Function.DATA) is not None
+ )
if data_label_present:
message_length = mt[-1].end - 1
@@ -158,15 +181,26 @@ def generate_message(self, message_type=None, data="0x00", source: Participant =
bits.append(self.decimal_to_bits(value, len_field))
elif lbl.field_type.function == FieldType.Function.TYPE:
- bits.append(self.decimal_to_bits(self.message_type_codes[mt] % (2 ** len_field), len_field))
+ bits.append(
+ self.decimal_to_bits(
+ self.message_type_codes[mt] % (2**len_field), len_field
+ )
+ )
elif lbl.field_type.function == FieldType.Function.SEQUENCE_NUMBER:
- bits.append(self.decimal_to_bits(self.sequence_numbers[mt] % (2 ** len_field), len_field))
+ bits.append(
+ self.decimal_to_bits(
+ self.sequence_numbers[mt] % (2**len_field), len_field
+ )
+ )
elif lbl.field_type.function == FieldType.Function.DST_ADDRESS:
dst_bits = self.__get_address_for_participant(destination)
if len(dst_bits) != len_field:
raise ValueError(
- "Length of dst ({0} bits) != length dst field ({1} bits)".format(len(dst_bits), len_field))
+ "Length of dst ({0} bits) != length dst field ({1} bits)".format(
+ len(dst_bits), len_field
+ )
+ )
bits.append(dst_bits)
elif lbl.field_type.function == FieldType.Function.SRC_ADDRESS:
@@ -174,13 +208,19 @@ def generate_message(self, message_type=None, data="0x00", source: Participant =
if len(src_bits) != len_field:
raise ValueError(
- "Length of src ({0} bits) != length src field ({1} bits)".format(len(src_bits), len_field))
+ "Length of src ({0} bits) != length src field ({1} bits)".format(
+ len(src_bits), len_field
+ )
+ )
bits.append(src_bits)
elif lbl.field_type.function == FieldType.Function.DATA:
if len(data) != len_field:
raise ValueError(
- "Length of data ({} bits) != length data field ({} bits)".format(len(data), len_field))
+ "Length of data ({} bits) != length data field ({} bits)".format(
+ len(data), len_field
+ )
+ )
bits.append(data)
start = lbl.end
@@ -194,7 +234,9 @@ def generate_message(self, message_type=None, data="0x00", source: Participant =
self.sequence_numbers[mt] += self.sequence_number_increment
for checksum_label in checksum_labels:
- msg[checksum_label.start:checksum_label.end] = checksum_label.calculate_checksum_for_message(msg, False)
+ msg[
+ checksum_label.start : checksum_label.end
+ ] = checksum_label.calculate_checksum_for_message(msg, False)
self.protocol.messages.append(msg)
@@ -206,15 +248,39 @@ def export_message_type_to_latex(message_type, f):
f.write(" \\begin{itemize}\n")
for lbl in message_type: # type: ProtocolLabel
if lbl.field_type.function == FieldType.Function.SYNC:
- sync = array("B", map(int, self.syncs_by_message_type[message_type]))
- f.write(" \\item {}: \\texttt{{0x{}}}\n".format(lbl.name, util.bit2hex(sync)))
+ sync = array(
+ "B", map(int, self.syncs_by_message_type[message_type])
+ )
+ f.write(
+ " \\item {}: \\texttt{{0x{}}}\n".format(
+ lbl.name, util.bit2hex(sync)
+ )
+ )
elif lbl.field_type.function == FieldType.Function.PREAMBLE:
- preamble = array("B", map(int, self.preambles_by_message_type[message_type]))
- f.write(" \\item {}: \\texttt{{0x{}}}\n".format(lbl.name, util.bit2hex(preamble)))
+ preamble = array(
+ "B", map(int, self.preambles_by_message_type[message_type])
+ )
+ f.write(
+ " \\item {}: \\texttt{{0x{}}}\n".format(
+ lbl.name, util.bit2hex(preamble)
+ )
+ )
elif lbl.field_type.function == FieldType.Function.CHECKSUM:
- f.write(" \\item {}: {}\n".format(lbl.name, lbl.checksum.caption))
- elif lbl.field_type.function in (FieldType.Function.LENGTH, FieldType.Function.SEQUENCE_NUMBER) and lbl.length > 8:
- f.write(" \\item {}: {} bit (\\textbf{{{} endian}})\n".format(lbl.name, lbl.length, "little" if self.little_endian else "big"))
+ f.write(
+ " \\item {}: {}\n".format(lbl.name, lbl.checksum.caption)
+ )
+ elif (
+ lbl.field_type.function
+ in (FieldType.Function.LENGTH, FieldType.Function.SEQUENCE_NUMBER)
+ and lbl.length > 8
+ ):
+ f.write(
+ " \\item {}: {} bit (\\textbf{{{} endian}})\n".format(
+ lbl.name,
+ lbl.length,
+ "little" if self.little_endian else "big",
+ )
+ )
elif lbl.field_type.function == FieldType.Function.DATA:
f.write(" \\item payload: {} byte\n".format(lbl.length // 8))
else:
@@ -225,16 +291,35 @@ def export_message_type_to_latex(message_type, f):
f.write("\\subsection{{Protocol {}}}\n".format(number))
if len(self.participants) > 1:
- f.write("There were {} participants involved in communication: ".format(len(self.participants)))
- f.write(", ".join("{} (\\texttt{{0x{}}})".format(p.name, p.address_hex) for p in self.participants[:-1]))
- f.write(" and {} (\\texttt{{0x{}}})".format(self.participants[-1].name, self.participants[-1].address_hex))
+ f.write(
+ "There were {} participants involved in communication: ".format(
+ len(self.participants)
+ )
+ )
+ f.write(
+ ", ".join(
+ "{} (\\texttt{{0x{}}})".format(p.name, p.address_hex)
+ for p in self.participants[:-1]
+ )
+ )
+ f.write(
+ " and {} (\\texttt{{0x{}}})".format(
+ self.participants[-1].name, self.participants[-1].address_hex
+ )
+ )
f.write(".\n")
if len(self.message_types) == 1:
- f.write("The protocol has one message type with the following fields:\n")
+ f.write(
+ "The protocol has one message type with the following fields:\n"
+ )
export_message_type_to_latex(self.message_types[0], f)
else:
- f.write("The protocol has {} message types with the following fields:\n".format(len(self.message_types)))
+ f.write(
+ "The protocol has {} message types with the following fields:\n".format(
+ len(self.message_types)
+ )
+ )
f.write("\\begin{itemize}\n")
for mt in self.message_types:
f.write(" \\item \\textbf{{{}}}\n".format(mt.name))
@@ -244,7 +329,7 @@ def export_message_type_to_latex(message_type, f):
f.write("\n")
-if __name__ == '__main__':
+if __name__ == "__main__":
mb = MessageTypeBuilder("test")
mb.add_label(FieldType.Function.PREAMBLE, 8)
mb.add_label(FieldType.Function.SYNC, 4)
@@ -255,6 +340,9 @@ def export_message_type_to_latex(message_type, f):
pg = ProtocolGenerator([mb.message_type], [], little_endian=False)
pg.generate_message(data="1" * 8)
pg.generate_message(data="1" * 16)
- pg.generate_message(data="0xab", source=Participant("Alice", "A", "1234"),
- destination=Participant("Bob", "B", "4567"))
+ pg.generate_message(
+ data="0xab",
+ source=Participant("Alice", "A", "1234"),
+ destination=Participant("Bob", "B", "4567"),
+ )
pg.to_file("/tmp/test.proto")
diff --git a/src/urh/awre/engines/AddressEngine.py b/src/urh/awre/engines/AddressEngine.py
index 7aa50f1248..e382bed8bb 100644
--- a/src/urh/awre/engines/AddressEngine.py
+++ b/src/urh/awre/engines/AddressEngine.py
@@ -12,8 +12,14 @@
class AddressEngine(Engine):
- def __init__(self, msg_vectors, participant_indices, known_participant_addresses: dict = None,
- already_labeled: list = None, src_field_present=False):
+ def __init__(
+ self,
+ msg_vectors,
+ participant_indices,
+ known_participant_addresses: dict = None,
+ already_labeled: list = None,
+ src_field_present=False,
+ ):
"""
:param msg_vectors: Message data behind synchronization
@@ -35,7 +41,9 @@ def __init__(self, msg_vectors, participant_indices, known_participant_addresses
if already_labeled is not None:
for start, end in already_labeled:
# convert it to hex
- self.already_labeled.append((int(math.ceil(start / 4)), int(math.ceil(end / 4))))
+ self.already_labeled.append(
+ (int(math.ceil(start / 4)), int(math.ceil(end / 4)))
+ )
self.message_indices_by_participant = defaultdict(list)
for i, participant_index in enumerate(self.participant_indices):
@@ -44,44 +52,69 @@ def __init__(self, msg_vectors, participant_indices, known_participant_addresses
if known_participant_addresses is None:
self.known_addresses_by_participant = dict() # type: dict[int, np.ndarray]
else:
- self.known_addresses_by_participant = known_participant_addresses # type: dict[int, np.ndarray]
+ self.known_addresses_by_participant = (
+ known_participant_addresses
+ ) # type: dict[int, np.ndarray]
@staticmethod
def cross_swap_check(rng1: CommonRange, rng2: CommonRange):
- return (rng1.start == rng2.start + rng1.length or rng1.start == rng2.start - rng1.length) \
- and rng1.value.tobytes() == rng2.value.tobytes()
+ return (
+ rng1.start == rng2.start + rng1.length
+ or rng1.start == rng2.start - rng1.length
+ ) and rng1.value.tobytes() == rng2.value.tobytes()
@staticmethod
def ack_check(rng1: CommonRange, rng2: CommonRange):
- return rng1.start == rng2.start and rng1.length == rng2.length and rng1.value.tobytes() != rng2.value.tobytes()
+ return (
+ rng1.start == rng2.start
+ and rng1.length == rng2.length
+ and rng1.value.tobytes() != rng2.value.tobytes()
+ )
def find(self):
- addresses_by_participant = {p: [addr.tostring()] for p, addr in self.known_addresses_by_participant.items()}
+ addresses_by_participant = {
+ p: [addr.tostring()]
+ for p, addr in self.known_addresses_by_participant.items()
+ }
addresses_by_participant.update(self.find_addresses())
self._debug("Addresses by participant", addresses_by_participant)
# Find the address candidates by participant in messages
ranges_by_participant = defaultdict(list) # type: dict[int, list[CommonRange]]
- addresses = [np.array(np.frombuffer(a, dtype=np.uint8))
- for address_list in addresses_by_participant.values()
- for a in address_list]
+ addresses = [
+ np.array(np.frombuffer(a, dtype=np.uint8))
+ for address_list in addresses_by_participant.values()
+ for a in address_list
+ ]
- already_labeled_cols = array("L", [e for rng in self.already_labeled for e in range(*rng)])
+ already_labeled_cols = array(
+ "L", [e for rng in self.already_labeled for e in range(*rng)]
+ )
# Find occurrences of address candidates in messages and create common ranges over matching positions
for i, msg_vector in enumerate(self.msg_vectors):
participant = self.participant_indices[i]
for address in addresses:
- for index in awre_util.find_occurrences(msg_vector, address, already_labeled_cols):
+ for index in awre_util.find_occurrences(
+ msg_vector, address, already_labeled_cols
+ ):
common_ranges = ranges_by_participant[participant]
- rng = next((cr for cr in common_ranges if cr.matches(index, address)), None) # type: CommonRange
+ rng = next(
+ (cr for cr in common_ranges if cr.matches(index, address)), None
+ ) # type: CommonRange
if rng is not None:
rng.message_indices.add(i)
else:
- common_ranges.append(CommonRange(index, len(address), address,
- message_indices={i},
- range_type="hex"))
+ common_ranges.append(
+ CommonRange(
+ index,
+ len(address),
+ address,
+ message_indices={i},
+ range_type="hex",
+ )
+ )
num_messages_by_participant = defaultdict(int)
for participant in self.participant_indices:
@@ -89,23 +122,37 @@ def find(self):
# Look for cross swapped values between participant clusters
for p1, p2 in itertools.combinations(ranges_by_participant, 2):
- ranges1_set, ranges2_set = set(ranges_by_participant[p1]), set(ranges_by_participant[p2])
+ ranges1_set, ranges2_set = set(ranges_by_participant[p1]), set(
+ ranges_by_participant[p2]
+ )
- for rng1, rng2 in itertools.product(ranges_by_participant[p1], ranges_by_participant[p2]):
+ for rng1, rng2 in itertools.product(
+ ranges_by_participant[p1], ranges_by_participant[p2]
+ ):
if rng1 in ranges2_set and rng2 in ranges1_set:
if self.cross_swap_check(rng1, rng2):
- rng1.score += len(rng2.message_indices) / num_messages_by_participant[p2]
- rng2.score += len(rng1.message_indices) / num_messages_by_participant[p1]
+ rng1.score += (
+ len(rng2.message_indices) / num_messages_by_participant[p2]
+ )
+ rng2.score += (
+ len(rng1.message_indices) / num_messages_by_participant[p1]
+ )
elif self.ack_check(rng1, rng2):
# Add previous score in divisor to add bonus to ranges that apply to all messages
- rng1.score += len(rng2.message_indices) / (num_messages_by_participant[p2] + rng1.score)
- rng2.score += len(rng1.message_indices) / (num_messages_by_participant[p1] + rng2.score)
+ rng1.score += len(rng2.message_indices) / (
+ num_messages_by_participant[p2] + rng1.score
+ )
+ rng2.score += len(rng1.message_indices) / (
+ num_messages_by_participant[p1] + rng2.score
+ )
if len(ranges_by_participant) == 1 and not self.src_field_present:
for p, ranges in ranges_by_participant.items():
for rng in sorted(ranges):
try:
- if np.array_equal(rng.value, self.known_addresses_by_participant[p]):
+ if np.array_equal(
+ rng.value, self.known_addresses_by_participant[p]
+ ):
# Only one participant in this iteration and address already known -> Highscore
rng.score = 1
break # Take only the first (leftmost) range
@@ -120,21 +167,28 @@ def find(self):
for participant, common_ranges in ranges_by_participant.items():
# Sort by negative score so ranges with highest score appear first
# Secondary sort by tuple to ensure order when ranges have same score
- sorted_ranges = sorted(filter(lambda cr: cr.score > self.minimum_score, common_ranges),
- key=lambda cr: (-cr.score, cr))
+ sorted_ranges = sorted(
+ filter(lambda cr: cr.score > self.minimum_score, common_ranges),
+ key=lambda cr: (-cr.score, cr),
+ )
if len(sorted_ranges) == 0:
addresses_by_participant[participant] = dict()
continue
- addresses_by_participant[participant] = {a for a in addresses_by_participant.get(participant, [])
- if len(a) == address_length}
+ addresses_by_participant[participant] = {
+ a
+ for a in addresses_by_participant.get(participant, [])
+ if len(a) == address_length
+ }
for rng in filter(lambda r: r.length == address_length, sorted_ranges):
rng.score = min(rng.score, 1.0)
high_scored_ranges_by_participant[participant].append(rng)
# Now we find the most probable address for all participants
- self.__assign_participant_addresses(addresses_by_participant, high_scored_ranges_by_participant)
+ self.__assign_participant_addresses(
+ addresses_by_participant, high_scored_ranges_by_participant
+ )
# Eliminate participants for which we could not assign an address
for participant, address in addresses_by_participant.copy().items():
@@ -152,17 +206,30 @@ def find(self):
result = []
for rng in sorted(ranges, key=lambda r: r.score, reverse=True):
- rng.field_type = "source address" if rng.value.tostring() == address else "destination address"
+ rng.field_type = (
+ "source address"
+ if rng.value.tostring() == address
+ else "destination address"
+ )
if len(result) == 0:
result.append(rng)
else:
- subset = next((r for r in result if rng.message_indices.issubset(r.message_indices)), None)
+ subset = next(
+ (
+ r
+ for r in result
+ if rng.message_indices.issubset(r.message_indices)
+ ),
+ None,
+ )
if subset is not None:
if rng.field_type == subset.field_type:
# Avoid adding same address type twice
continue
- if rng.length != subset.length or (rng.start != subset.end + 1 and rng.end + 1 != subset.start):
+ if rng.length != subset.length or (
+ rng.start != subset.end + 1 and rng.end + 1 != subset.start
+ ):
# Ensure addresses are next to each other
continue
@@ -170,9 +237,15 @@ def find(self):
high_scored_ranges_by_participant[participant] = result
- self.__find_broadcast_fields(high_scored_ranges_by_participant, addresses_by_participant)
+ self.__find_broadcast_fields(
+ high_scored_ranges_by_participant, addresses_by_participant
+ )
- result = [rng for ranges in high_scored_ranges_by_participant.values() for rng in ranges]
+ result = [
+ rng
+ for ranges in high_scored_ranges_by_participant.values()
+ for rng in ranges
+ ]
# If we did not find a SRC address, lower the score a bit,
# so DST fields do not win later e.g. again length fields in case of tie
if not any(rng.field_type == "source address" for rng in result):
@@ -190,18 +263,26 @@ def __estimate_address_length(self, ranges_by_participant: dict):
"""
address_lengths = []
for participant, common_ranges in ranges_by_participant.items():
- sorted_ranges = sorted(filter(lambda cr: cr.score > self.minimum_score, common_ranges),
- key=lambda cr: (-cr.score, cr))
+ sorted_ranges = sorted(
+ filter(lambda cr: cr.score > self.minimum_score, common_ranges),
+ key=lambda cr: (-cr.score, cr),
+ )
max_scored = [r for r in sorted_ranges if r.score == sorted_ranges[0].score]
# Prevent overestimation of address length by looking for substrings
for rng in max_scored[:]:
- same_message_rng = [r for r in sorted_ranges
- if r not in max_scored and r.score > 0 and r.message_indices == rng.message_indices]
+ same_message_rng = [
+ r
+ for r in sorted_ranges
+ if r not in max_scored
+ and r.score > 0
+ and r.message_indices == rng.message_indices
+ ]
if len(same_message_rng) > 1 and all(
- r.value.tobytes() in rng.value.tobytes() for r in same_message_rng):
+ r.value.tobytes() in rng.value.tobytes() for r in same_message_rng
+ ):
# remove the longer range and add the smaller ones
max_scored.remove(rng)
max_scored.extend(same_message_rng)
@@ -225,7 +306,9 @@ def __estimate_address_length(self, ranges_by_participant: dict):
except ValueError: # max() arg is an empty sequence
return 0
- def __assign_participant_addresses(self, addresses_by_participant, high_scored_ranges_by_participant):
+ def __assign_participant_addresses(
+ self, addresses_by_participant, high_scored_ranges_by_participant
+ ):
scored_participants_addresses = dict()
for participant in addresses_by_participant:
scored_participants_addresses[participant] = defaultdict(int)
@@ -237,8 +320,11 @@ def __assign_participant_addresses(self, addresses_by_participant, high_scored_r
continue
for i in self.message_indices_by_participant[participant]:
- matching = [rng for rng in high_scored_ranges_by_participant[participant]
- if i in rng.message_indices and rng.value.tostring() in addresses]
+ matching = [
+ rng
+ for rng in high_scored_ranges_by_participant[participant]
+ if i in rng.message_indices and rng.value.tostring() in addresses
+ ]
if len(matching) == 1:
address = matching[0].value.tostring()
@@ -248,25 +334,44 @@ def __assign_participant_addresses(self, addresses_by_participant, high_scored_r
# Since this is probably an ACK, the address is probably SRC of participant of previous message
if i > 0 and self.participant_indices[i - 1] != participant:
prev_participant = self.participant_indices[i - 1]
- prev_matching = [rng for rng in high_scored_ranges_by_participant[prev_participant]
- if i - 1 in rng.message_indices and rng.value.tostring() in addresses]
+ prev_matching = [
+ rng
+ for rng in high_scored_ranges_by_participant[
+ prev_participant
+ ]
+ if i - 1 in rng.message_indices
+ and rng.value.tostring() in addresses
+ ]
if len(prev_matching) > 1:
- for prev_rng in filter(lambda r: r.value.tostring() == address, prev_matching):
- scored_participants_addresses[prev_participant][address] += prev_rng.score
+ for prev_rng in filter(
+ lambda r: r.value.tostring() == address, prev_matching
+ ):
+ scored_participants_addresses[prev_participant][
+ address
+ ] += prev_rng.score
elif len(matching) > 1:
# more than one address, so there must be a source address included
for rng in matching:
- scored_participants_addresses[participant][rng.value.tostring()] += rng.score
+ scored_participants_addresses[participant][
+ rng.value.tostring()
+ ] += rng.score
minimum_score = 0.5
taken_addresses = set()
self._debug("Scored addresses", scored_participants_addresses)
# If all participants have exactly one possible address and they all differ, we can assign them right away
- if all(len(addresses) == 1 for addresses in scored_participants_addresses.values()):
- all_addresses = [list(addresses)[0] for addresses in scored_participants_addresses.values()]
- if len(all_addresses) == len(set(all_addresses)): # ensure all addresses are different
+ if all(
+ len(addresses) == 1 for addresses in scored_participants_addresses.values()
+ ):
+ all_addresses = [
+ list(addresses)[0]
+ for addresses in scored_participants_addresses.values()
+ ]
+ if len(all_addresses) == len(
+ set(all_addresses)
+ ): # ensure all addresses are different
for p, addresses in scored_participants_addresses.items():
addresses_by_participant[p] = list(addresses)[0]
return
@@ -274,10 +379,17 @@ def __assign_participant_addresses(self, addresses_by_participant, high_scored_r
for participant, addresses in sorted(scored_participants_addresses.items()):
try:
# sort filtered results to prevent randomness for equal scores
- found_address = max(sorted(
- filter(lambda a: a not in taken_addresses and addresses[a] >= minimum_score, addresses),
- reverse=True
- ), key=addresses.get)
+ found_address = max(
+ sorted(
+ filter(
+ lambda a: a not in taken_addresses
+ and addresses[a] >= minimum_score,
+ addresses,
+ ),
+ reverse=True,
+ ),
+ key=addresses.get,
+ )
except ValueError:
# Could not assign address for this participant
addresses_by_participant[participant] = None
@@ -286,7 +398,9 @@ def __assign_participant_addresses(self, addresses_by_participant, high_scored_r
addresses_by_participant[participant] = found_address
taken_addresses.add(found_address)
- def __find_broadcast_fields(self, high_scored_ranges_by_participant, addresses_by_participant: dict):
+ def __find_broadcast_fields(
+ self, high_scored_ranges_by_participant, addresses_by_participant: dict
+ ):
"""
Last we check for messages that were sent to broadcast
1. we search for messages that have a SRC address but no DST address
@@ -302,18 +416,35 @@ def __find_broadcast_fields(self, high_scored_ranges_by_participant, addresses_b
broadcast_bag = defaultdict(list) # type: dict[CommonRange, list[int]]
for common_ranges in high_scored_ranges_by_participant.values():
- src_address_fields = sorted(filter(lambda r: r.field_type == "source address", common_ranges))
- dst_address_fields = sorted(filter(lambda r: r.field_type == "destination address", common_ranges))
- msg_with_dst = {i for dst_address_field in dst_address_fields for i in dst_address_field.message_indices}
+ src_address_fields = sorted(
+ filter(lambda r: r.field_type == "source address", common_ranges)
+ )
+ dst_address_fields = sorted(
+ filter(lambda r: r.field_type == "destination address", common_ranges)
+ )
+ msg_with_dst = {
+ i
+ for dst_address_field in dst_address_fields
+ for i in dst_address_field.message_indices
+ }
for src_address_field in src_address_fields: # type: CommonRange
- msg_without_dst = {i for i in src_address_field.message_indices if i not in msg_with_dst}
+ msg_without_dst = {
+ i
+ for i in src_address_field.message_indices
+ if i not in msg_with_dst
+ }
if len(msg_without_dst) == 0:
continue
try:
- matching_dst = next(dst for dst in dst_address_fields
- if all(i in dst.message_indices
- for i in src_address_field.message_indices - msg_without_dst))
+ matching_dst = next(
+ dst
+ for dst in dst_address_fields
+ if all(
+ i in dst.message_indices
+ for i in src_address_field.message_indices - msg_without_dst
+ )
+ )
except StopIteration:
continue
for msg in msg_without_dst:
@@ -325,7 +456,7 @@ def __find_broadcast_fields(self, high_scored_ranges_by_participant, addresses_b
broadcast_address = None
for dst, messages in broadcast_bag.items():
for msg_index in messages:
- value = self.msg_vectors[msg_index][dst.start:dst.end + 1]
+ value = self.msg_vectors[msg_index][dst.start : dst.end + 1]
if broadcast_address is None:
broadcast_address = value
elif value.tobytes() != broadcast_address.tobytes():
@@ -350,11 +481,14 @@ def find_addresses(self) -> dict:
for i in message_indices:
length_clusters[len(self.msg_vectors[i])].append(i)
- common_ranges_by_length = self.find_common_ranges_by_cluster(self.msg_vectors, length_clusters, range_type="hex")
+ common_ranges_by_length = self.find_common_ranges_by_cluster(
+ self.msg_vectors, length_clusters, range_type="hex"
+ )
common_ranges_by_participant[participant] = []
for ranges in common_ranges_by_length.values():
- common_ranges_by_participant[participant].extend(self.ignore_already_labeled(ranges,
- self.already_labeled))
+ common_ranges_by_participant[participant].extend(
+ self.ignore_already_labeled(ranges, self.already_labeled)
+ )
self._debug("Common ranges by participant:", common_ranges_by_participant)
@@ -367,8 +501,15 @@ def find_addresses(self) -> dict:
# If we already know the address length we do not need to bother with other candidates
if len(already_assigned) > 0:
addr_len = len(self.known_addresses_by_participant[already_assigned[0]])
- if any(len(self.known_addresses_by_participant[i]) != addr_len for i in already_assigned):
- logger.warning("Addresses do not have a common length. Assuming length of {}".format(addr_len))
+ if any(
+ len(self.known_addresses_by_participant[i]) != addr_len
+ for i in already_assigned
+ ):
+ logger.warning(
+ "Addresses do not have a common length. Assuming length of {}".format(
+ addr_len
+ )
+ )
else:
addr_len = None
@@ -392,8 +533,16 @@ def find_addresses(self) -> dict:
if not p1_already_assigned and not p2_already_assigned:
result[p1].add(val.tostring())
result[p2].add(val.tostring())
- elif p1_already_assigned and val.tostring() != self.known_addresses_by_participant[p1].tostring():
+ elif (
+ p1_already_assigned
+ and val.tostring()
+ != self.known_addresses_by_participant[p1].tostring()
+ ):
result[p2].add(val.tostring())
- elif p2_already_assigned and val.tostring() != self.known_addresses_by_participant[p2].tostring():
+ elif (
+ p2_already_assigned
+ and val.tostring()
+ != self.known_addresses_by_participant[p2].tostring()
+ ):
result[p1].add(val.tostring())
return result
diff --git a/src/urh/awre/engines/ChecksumEngine.py b/src/urh/awre/engines/ChecksumEngine.py
index 9ad5d7a3a7..ccc80944a0 100644
--- a/src/urh/awre/engines/ChecksumEngine.py
+++ b/src/urh/awre/engines/ChecksumEngine.py
@@ -12,7 +12,13 @@
class ChecksumEngine(Engine):
- def __init__(self, bitvectors, n_gram_length=8, minimum_score=0.9, already_labeled: list = None):
+ def __init__(
+ self,
+ bitvectors,
+ n_gram_length=8,
+ minimum_score=0.9,
+ already_labeled: list = None,
+ ):
"""
:type bitvectors: list of np.ndarray
:param bitvectors: bitvectors behind the synchronization
@@ -23,7 +29,9 @@ def __init__(self, bitvectors, n_gram_length=8, minimum_score=0.9, already_label
if already_labeled is None:
self.already_labeled_cols = set()
else:
- self.already_labeled_cols = {e for rng in already_labeled for e in range(*rng)}
+ self.already_labeled_cols = {
+ e for rng in already_labeled for e in range(*rng)
+ }
def find(self):
result = list()
@@ -37,31 +45,58 @@ def find(self):
checksums_for_length = []
for index in message_indices:
bits = self.bitvectors[index]
- data_start, data_stop, crc_start, crc_stop = WSPChecksum.search_for_wsp_checksum(bits)
+ (
+ data_start,
+ data_stop,
+ crc_start,
+ crc_stop,
+ ) = WSPChecksum.search_for_wsp_checksum(bits)
if (data_start, data_stop, crc_start, crc_stop) != (0, 0, 0, 0):
- checksum_range = ChecksumRange(start=crc_start, length=crc_stop-crc_start,
- data_range_start=data_start, data_range_end=data_stop,
- crc=WSPChecksum(), score=1/len(message_indices),
- field_type="checksum", message_indices={index})
+ checksum_range = ChecksumRange(
+ start=crc_start,
+ length=crc_stop - crc_start,
+ data_range_start=data_start,
+ data_range_end=data_stop,
+ crc=WSPChecksum(),
+ score=1 / len(message_indices),
+ field_type="checksum",
+ message_indices={index},
+ )
try:
- present = next(c for c in checksums_for_length if c == checksum_range)
+ present = next(
+ c for c in checksums_for_length if c == checksum_range
+ )
present.message_indices.add(index)
except StopIteration:
checksums_for_length.append(checksum_range)
continue
- crc_object, data_start, data_stop, crc_start, crc_stop = crc.guess_all(bits,
- ignore_positions=self.already_labeled_cols)
-
- if (crc_object, data_start, data_stop, crc_start, crc_stop) != (0, 0, 0, 0, 0):
- checksum_range = ChecksumRange(start=crc_start, length=crc_stop - crc_start,
- data_range_start=data_start, data_range_end=data_stop,
- crc=copy.copy(crc_object), score=1 / len(message_indices),
- field_type="checksum", message_indices={index}
- )
+ crc_object, data_start, data_stop, crc_start, crc_stop = crc.guess_all(
+ bits, ignore_positions=self.already_labeled_cols
+ )
+
+ if (crc_object, data_start, data_stop, crc_start, crc_stop) != (
+ 0,
+ 0,
+ 0,
+ 0,
+ 0,
+ ):
+ checksum_range = ChecksumRange(
+ start=crc_start,
+ length=crc_stop - crc_start,
+ data_range_start=data_start,
+ data_range_end=data_stop,
+ crc=copy.copy(crc_object),
+ score=1 / len(message_indices),
+ field_type="checksum",
+ message_indices={index},
+ )
try:
- present = next(rng for rng in checksums_for_length if rng == checksum_range)
+ present = next(
+ rng for rng in checksums_for_length if rng == checksum_range
+ )
present.message_indices.add(index)
continue
except StopIteration:
@@ -69,10 +104,15 @@ def find(self):
checksums_for_length.append(checksum_range)
- matching = awre_util.check_crc_for_messages(message_indices, self.bitvectors,
- data_start, data_stop,
- crc_start, crc_stop,
- *crc_object.get_parameters())
+ matching = awre_util.check_crc_for_messages(
+ message_indices,
+ self.bitvectors,
+ data_start,
+ data_stop,
+ crc_start,
+ crc_stop,
+ *crc_object.get_parameters()
+ )
checksum_range.message_indices.update(matching)
@@ -87,8 +127,14 @@ def find(self):
self._debug("Found Checksums", result)
try:
- max_scored = max(filter(lambda x: len(x.message_indices) >= 2 and x.score >= self.minimum_score, result),
- key=lambda x: x.score)
+ max_scored = max(
+ filter(
+ lambda x: len(x.message_indices) >= 2
+ and x.score >= self.minimum_score,
+ result,
+ ),
+ key=lambda x: x.score,
+ )
except ValueError:
return []
diff --git a/src/urh/awre/engines/Engine.py b/src/urh/awre/engines/Engine.py
index 3198797d5c..d50f655010 100644
--- a/src/urh/awre/engines/Engine.py
+++ b/src/urh/awre/engines/Engine.py
@@ -13,7 +13,9 @@ def _debug(self, *args):
print("[{}]".format(self.__class__.__name__), *args)
@staticmethod
- def find_common_ranges_by_cluster(msg_vectors, clustered_bitvectors, alpha=0.95, range_type="bit"):
+ def find_common_ranges_by_cluster(
+ msg_vectors, clustered_bitvectors, alpha=0.95, range_type="bit"
+ ):
"""
:param alpha: How many percent of values must be equal per range?
@@ -36,13 +38,22 @@ def find_common_ranges_by_cluster(msg_vectors, clustered_bitvectors, alpha=0.95,
return common_ranges_by_cluster
@staticmethod
- def find_common_ranges_exhaustive(msg_vectors, msg_indices, range_type="bit") -> list:
+ def find_common_ranges_exhaustive(
+ msg_vectors, msg_indices, range_type="bit"
+ ) -> list:
result = []
for i, j in itertools.combinations(msg_indices, 2):
- for rng in Histogram(msg_vectors, indices=[i, j]).find_common_ranges(alpha=1, range_type=range_type):
+ for rng in Histogram(msg_vectors, indices=[i, j]).find_common_ranges(
+ alpha=1, range_type=range_type
+ ):
try:
- common_range = next(cr for cr in result if cr.start == rng.start and cr.value.tobytes() == rng.value.tobytes())
+ common_range = next(
+ cr
+ for cr in result
+ if cr.start == rng.start
+ and cr.value.tobytes() == rng.value.tobytes()
+ )
common_range.message_indices.update({i, j})
except StopIteration:
result.append(rng)
diff --git a/src/urh/awre/engines/LengthEngine.py b/src/urh/awre/engines/LengthEngine.py
index 9dc2a697fb..f9fcdefd3c 100644
--- a/src/urh/awre/engines/LengthEngine.py
+++ b/src/urh/awre/engines/LengthEngine.py
@@ -25,12 +25,14 @@ def find(self, n_gram_length=8, minimum_score=0.1):
bin_num = int(math.ceil(len(bitvector) / n_gram_length))
bitvectors_by_n_gram_length[bin_num].append(i)
- common_ranges_by_length = self.find_common_ranges_by_cluster(self.bitvectors,
- bitvectors_by_n_gram_length,
- alpha=0.7)
+ common_ranges_by_length = self.find_common_ranges_by_cluster(
+ self.bitvectors, bitvectors_by_n_gram_length, alpha=0.7
+ )
for length, ranges in common_ranges_by_length.items():
- common_ranges_by_length[length] = self.ignore_already_labeled(ranges, self.already_labeled)
+ common_ranges_by_length[length] = self.ignore_already_labeled(
+ ranges, self.already_labeled
+ )
self.filter_common_ranges(common_ranges_by_length)
self._debug("Common Ranges:", common_ranges_by_length)
@@ -39,8 +41,9 @@ def find(self, n_gram_length=8, minimum_score=0.1):
self._debug("Scored Ranges", scored_ranges)
# Take the ranges with highest score per cluster if it's score surpasses the minimum score
- high_scores_by_length = self.choose_high_scored_ranges(scored_ranges, bitvectors_by_n_gram_length,
- minimum_score)
+ high_scores_by_length = self.choose_high_scored_ranges(
+ scored_ranges, bitvectors_by_n_gram_length, minimum_score
+ )
self._debug("Highscored Ranges", high_scores_by_length)
return high_scores_by_length.values()
@@ -57,10 +60,15 @@ def filter_common_ranges(common_ranges_by_length: dict):
ranges = [r for rng in common_ranges_by_length.values() for r in rng]
for rng in ranges:
- count = len([r for r in ranges if rng.start == r.start
- and rng.length == r.length
- and rng.value.tobytes() == r.value.tobytes()]
- )
+ count = len(
+ [
+ r
+ for r in ranges
+ if rng.start == r.start
+ and rng.length == r.length
+ and rng.value.tobytes() == r.value.tobytes()
+ ]
+ )
if count < 2:
continue
@@ -97,47 +105,65 @@ def score_ranges(common_ranges_by_length: dict, n_gram_length: int):
byteorders = ["big", "little"] if n_gram_length == 8 else ["big"]
for window_length in window_lengths:
for length, common_ranges in common_ranges_by_length.items():
- for common_range in filter(lambda cr: cr.length >= window_length, common_ranges):
+ for common_range in filter(
+ lambda cr: cr.length >= window_length, common_ranges
+ ):
bits = common_range.value
rng_byte_order = "big"
max_score = max_start = -1
for start in range(0, len(bits) + 1 - window_length, n_gram_length):
for byteorder in byteorders:
- score = LengthEngine.score_bits(bits[start:start + window_length],
- length, position=start, byteorder=byteorder)
+ score = LengthEngine.score_bits(
+ bits[start : start + window_length],
+ length,
+ position=start,
+ byteorder=byteorder,
+ )
if score > max_score:
max_score = score
max_start = start
rng_byte_order = byteorder
- rng = CommonRange(common_range.start + max_start, window_length,
- common_range.value[max_start:max_start + window_length],
- score=max_score, field_type="length",
- message_indices=common_range.message_indices,
- range_type=common_range.range_type,
- byte_order=rng_byte_order)
+ rng = CommonRange(
+ common_range.start + max_start,
+ window_length,
+ common_range.value[max_start : max_start + window_length],
+ score=max_score,
+ field_type="length",
+ message_indices=common_range.message_indices,
+ range_type=common_range.range_type,
+ byte_order=rng_byte_order,
+ )
scored_ranges[length][window_length].append(rng)
return scored_ranges
- def choose_high_scored_ranges(self, scored_ranges: dict, bitvectors_by_n_gram_length: dict, minimum_score: float):
-
+ def choose_high_scored_ranges(
+ self,
+ scored_ranges: dict,
+ bitvectors_by_n_gram_length: dict,
+ minimum_score: float,
+ ):
# Set for every window length the highest scored range as candidate
possible_window_lengths = defaultdict(int)
for length, ranges_by_window_length in scored_ranges.items():
for window_length, ranges in ranges_by_window_length.items():
try:
- ranges_by_window_length[window_length] = max(filter(lambda x: x.score >= minimum_score, ranges),
- key=lambda x: x.score)
+ ranges_by_window_length[window_length] = max(
+ filter(lambda x: x.score >= minimum_score, ranges),
+ key=lambda x: x.score,
+ )
possible_window_lengths[window_length] += 1
except ValueError:
ranges_by_window_length[window_length] = None
try:
# Choose window length -> window length that has a result most often and choose greater on tie
- chosen_window_length = max(possible_window_lengths, key=lambda x: (possible_window_lengths[x], x))
+ chosen_window_length = max(
+ possible_window_lengths, key=lambda x: (possible_window_lengths[x], x)
+ )
except ValueError:
return dict()
@@ -147,7 +173,9 @@ def choose_high_scored_ranges(self, scored_ranges: dict, bitvectors_by_n_gram_le
for length, ranges_by_window_length in scored_ranges.items():
try:
if ranges_by_window_length[chosen_window_length]:
- high_scores_by_length[length] = ranges_by_window_length[chosen_window_length]
+ high_scores_by_length[length] = ranges_by_window_length[
+ chosen_window_length
+ ]
except KeyError:
continue
@@ -161,30 +189,39 @@ def choose_high_scored_ranges(self, scored_ranges: dict, bitvectors_by_n_gram_le
max_score, best_match = 0, None
for rng in high_scores_by_length.values():
- bits = bitvector[rng.start:rng.end + 1]
+ bits = bitvector[rng.start : rng.end + 1]
if len(bits) > 0:
score = self.score_bits(bits, length, rng.start)
if score > max_score:
best_match, max_score = rng, score
if best_match is not None:
- high_scores_by_length[length] = CommonRange(best_match.start, best_match.length,
- value=bitvector[best_match.start:best_match.end + 1],
- score=max_score, field_type="length",
- message_indices={msg_index}, range_type="bit")
+ high_scores_by_length[length] = CommonRange(
+ best_match.start,
+ best_match.length,
+ value=bitvector[best_match.start : best_match.end + 1],
+ score=max_score,
+ field_type="length",
+ message_indices={msg_index},
+ range_type="bit",
+ )
return high_scores_by_length
@staticmethod
- def score_bits(bits: np.ndarray, target_length: int, position: int, byteorder="big"):
+ def score_bits(
+ bits: np.ndarray, target_length: int, position: int, byteorder="big"
+ ):
value = util.bit_array_to_number(bits, len(bits))
if byteorder == "little":
if len(bits) > 8 and len(bits) % 8 == 0:
n = len(bits) // 8
- value = int.from_bytes(value.to_bytes(n, byteorder="big"), byteorder="little", signed=False)
+ value = int.from_bytes(
+ value.to_bytes(n, byteorder="big"), byteorder="little", signed=False
+ )
# Length field should be at front, so we give lower scores for large starts
- f = (1 / (1 + 0.25 * position))
+ f = 1 / (1 + 0.25 * position)
return f * LengthEngine.gauss(value, target_length)
diff --git a/src/urh/awre/engines/SequenceNumberEngine.py b/src/urh/awre/engines/SequenceNumberEngine.py
index aa64406963..ced8206aa4 100644
--- a/src/urh/awre/engines/SequenceNumberEngine.py
+++ b/src/urh/awre/engines/SequenceNumberEngine.py
@@ -6,7 +6,13 @@
class SequenceNumberEngine(Engine):
- def __init__(self, bitvectors, n_gram_length=8, minimum_score=0.75, already_labeled: list = None):
+ def __init__(
+ self,
+ bitvectors,
+ n_gram_length=8,
+ minimum_score=0.75,
+ already_labeled: list = None,
+ ):
"""
:type bitvectors: list of np.ndarray
@@ -18,7 +24,9 @@ def __init__(self, bitvectors, n_gram_length=8, minimum_score=0.75, already_labe
if already_labeled is None:
self.already_labeled_cols = set()
else:
- self.already_labeled_cols = {e // n_gram_length for rng in already_labeled for e in range(*rng)}
+ self.already_labeled_cols = {
+ e // n_gram_length for rng in already_labeled for e in range(*rng)
+ }
def find(self):
n = self.n_gram_length
@@ -44,28 +52,43 @@ def find(self):
self._debug("Scores by column", scores_by_column)
result = []
- for candidate_column in sorted(scores_by_column, key=scores_by_column.get, reverse=True):
+ for candidate_column in sorted(
+ scores_by_column, key=scores_by_column.get, reverse=True
+ ):
score = scores_by_column[candidate_column]
if score < self.minimum_score:
continue
- most_common_diff = self.get_most_frequent(diff_frequencies_by_column[candidate_column])
+ most_common_diff = self.get_most_frequent(
+ diff_frequencies_by_column[candidate_column]
+ )
message_indices = np.flatnonzero(
# get all rows that have the most common difference or zero
- (diff_matrix[:, candidate_column] == most_common_diff) | (diff_matrix[:, candidate_column] == 0)
+ (diff_matrix[:, candidate_column] == most_common_diff)
+ | (diff_matrix[:, candidate_column] == 0)
)
# For example, index 1 in diff matrix corresponds to index 1 and 2 of messages
message_indices = set(message_indices) | set(message_indices + 1)
values = set()
for i in message_indices:
- values.add(self.bitvectors[i][candidate_column * n:(candidate_column + 1) * n].tobytes())
+ values.add(
+ self.bitvectors[i][
+ candidate_column * n : (candidate_column + 1) * n
+ ].tobytes()
+ )
- matching_ranges = [r for r in result if r.message_indices == message_indices]
+ matching_ranges = [
+ r for r in result if r.message_indices == message_indices
+ ]
try:
- matching_range = next(r for r in matching_ranges if r.start == (candidate_column - 1) * n
- and (r.byte_order_is_unknown or r.byte_order == "big"))
+ matching_range = next(
+ r
+ for r in matching_ranges
+ if r.start == (candidate_column - 1) * n
+ and (r.byte_order_is_unknown or r.byte_order == "big")
+ )
matching_range.length += n
matching_range.byte_order = "big"
matching_range.values.extend(list(values))
@@ -74,8 +97,12 @@ def find(self):
pass
try:
- matching_range = next(r for r in matching_ranges if r.start == (candidate_column + 1) * n
- and (r.byte_order_is_unknown or r.byte_order == "little"))
+ matching_range = next(
+ r
+ for r in matching_ranges
+ if r.start == (candidate_column + 1) * n
+ and (r.byte_order_is_unknown or r.byte_order == "little")
+ )
matching_range.start -= n
matching_range.length += n
matching_range.byte_order = "little"
@@ -84,9 +111,14 @@ def find(self):
except StopIteration:
pass
- new_range = CommonRange(start=candidate_column * n, length=n, score=score,
- field_type="sequence number", message_indices=message_indices,
- byte_order=None)
+ new_range = CommonRange(
+ start=candidate_column * n,
+ length=n,
+ score=score,
+ field_type="sequence number",
+ message_indices=message_indices,
+ byte_order=None,
+ )
new_range.values.extend(list(values))
result.append(new_range)
@@ -95,7 +127,10 @@ def find(self):
@staticmethod
def get_most_frequent(diff_frequencies: dict):
- return max(filter(lambda x: x not in (0, -1), diff_frequencies), key=diff_frequencies.get)
+ return max(
+ filter(lambda x: x not in (0, -1), diff_frequencies),
+ key=diff_frequencies.get,
+ )
@staticmethod
def calc_score(diff_frequencies: dict) -> float:
diff --git a/src/urh/cli/urh_cli.py b/src/urh/cli/urh_cli.py
index 5765437ed0..025b945810 100755
--- a/src/urh/cli/urh_cli.py
+++ b/src/urh/cli/urh_cli.py
@@ -18,7 +18,7 @@
DEFAULT_CENTER_SPACING = 0.1
DEFAULT_TOLERANCE = 5
-cli_exe = sys.executable if hasattr(sys, 'frozen') else sys.argv[0]
+cli_exe = sys.executable if hasattr(sys, "frozen") else sys.argv[0]
cur_dir = os.path.realpath(os.path.dirname(os.path.realpath(cli_exe)))
SRC_DIR = os.path.realpath(os.path.join(cur_dir, "..", ".."))
@@ -64,9 +64,11 @@
def cli_progress_bar(value, end_value, bar_length=20, title="Percent"):
percent = value / end_value
- hashes = '#' * int(round(percent * bar_length))
- spaces = ' ' * (bar_length - len(hashes))
- sys.stdout.write("\r{0}:\t[{1}] {2}%".format(title, hashes + spaces, int(round(percent * 100))))
+ hashes = "#" * int(round(percent * bar_length))
+ spaces = " " * (bar_length - len(hashes))
+ sys.stdout.write(
+ "\r{0}:\t[{1}] {2}%".format(title, hashes + spaces, int(round(percent * 100)))
+ )
sys.stdout.flush()
@@ -83,7 +85,11 @@ def build_modulator_from_args(arguments: argparse.Namespace):
n = 2 ** int(arguments.bits_per_symbol)
if arguments.parameters is None or len(arguments.parameters) != n:
- raise ValueError("You need to give {} parameters for {} bits per symbol".format(n, int(arguments.bits_per_symbol)))
+ raise ValueError(
+ "You need to give {} parameters for {} bits per symbol".format(
+ n, int(arguments.bits_per_symbol)
+ )
+ )
result = Modulator("CLI Modulator")
result.carrier_freq_hz = float(arguments.carrier_frequency)
@@ -107,6 +113,7 @@ def build_modulator_from_args(arguments: argparse.Namespace):
def build_backend_handler_from_args(arguments: argparse.Namespace):
from urh.dev.BackendHandler import Backends
+
bh = BackendHandler()
if arguments.device_backend == "native":
bh.device_backends[arguments.device.lower()].selected_backend = Backends.native
@@ -119,13 +126,23 @@ def build_backend_handler_from_args(arguments: argparse.Namespace):
def build_device_from_args(arguments: argparse.Namespace):
from urh.dev.VirtualDevice import Mode
+
bh = build_backend_handler_from_args(arguments)
- bandwidth = arguments.sample_rate if arguments.bandwidth is None else arguments.bandwidth
- result = VirtualDevice(bh, name=arguments.device, mode=Mode.receive if arguments.receive else Mode.send,
- freq=arguments.frequency, sample_rate=arguments.sample_rate,
- bandwidth=bandwidth,
- gain=arguments.gain, if_gain=arguments.if_gain, baseband_gain=arguments.baseband_gain)
+ bandwidth = (
+ arguments.sample_rate if arguments.bandwidth is None else arguments.bandwidth
+ )
+ result = VirtualDevice(
+ bh,
+ name=arguments.device,
+ mode=Mode.receive if arguments.receive else Mode.send,
+ freq=arguments.frequency,
+ sample_rate=arguments.sample_rate,
+ bandwidth=bandwidth,
+ gain=arguments.gain,
+ if_gain=arguments.if_gain,
+ baseband_gain=arguments.baseband_gain,
+ )
result.freq_correction = arguments.frequency_correction
if arguments.device_identifier is not None:
@@ -142,13 +159,22 @@ def build_device_from_args(arguments: argparse.Namespace):
def build_protocol_sniffer_from_args(arguments: argparse.Namespace):
bh = build_backend_handler_from_args(arguments)
- result = ProtocolSniffer(arguments.samples_per_symbol, arguments.center, arguments.center_spacing,
- arguments.noise, arguments.tolerance,
- arguments.modulation_type, arguments.bits_per_symbol,
- arguments.device.lower(), bh)
+ result = ProtocolSniffer(
+ arguments.samples_per_symbol,
+ arguments.center,
+ arguments.center_spacing,
+ arguments.noise,
+ arguments.tolerance,
+ arguments.modulation_type,
+ arguments.bits_per_symbol,
+ arguments.device.lower(),
+ bh,
+ )
result.rcv_device.frequency = arguments.frequency
result.rcv_device.sample_rate = arguments.sample_rate
- result.rcv_device.bandwidth = arguments.sample_rate if arguments.bandwidth is None else arguments.bandwidth
+ result.rcv_device.bandwidth = (
+ arguments.sample_rate if arguments.bandwidth is None else arguments.bandwidth
+ )
result.rcv_device.freq_correction = arguments.frequency_correction
if arguments.gain is not None:
result.rcv_device.gain = arguments.gain
@@ -187,23 +213,28 @@ def read_messages_to_send(arguments: argparse.Namespace):
print("Either give messages (-m) or a file to read from (-file) not both.")
sys.exit(1)
elif arguments.messages is not None:
- #support for calls from external tools e.g. metasploit
+ # support for calls from external tools e.g. metasploit
if len(arguments.messages) == 1:
- message_strings = arguments.messages[0].split(' ')
+ message_strings = arguments.messages[0].split(" ")
else:
message_strings = arguments.messages
elif arguments.filename is not None:
with open(arguments.filename) as f:
message_strings = list(map(str.strip, f.readlines()))
else:
- print("You need to give messages to send either with (-m) or a file (-file) to read them from.")
+ print(
+ "You need to give messages to send either with (-m) or a file (-file) to read them from."
+ )
sys.exit(1)
encoding = build_encoding_from_args(arguments)
- result = ProtocolAnalyzer.get_protocol_from_string(message_strings, is_hex=arguments.hex,
- default_pause=arguments.pause,
- sample_rate=arguments.sample_rate).messages
+ result = ProtocolAnalyzer.get_protocol_from_string(
+ message_strings,
+ is_hex=arguments.hex,
+ default_pause=arguments.pause,
+ sample_rate=arguments.sample_rate,
+ ).messages
if encoding:
for msg in result:
msg.decoder = encoding
@@ -216,13 +247,16 @@ def modulate_messages(messages, modulator):
return None
cli_progress_bar(0, len(messages), title="Modulating")
- nsamples = sum(int(len(msg.encoded_bits) * modulator.samples_per_symbol + msg.pause) for msg in messages)
+ nsamples = sum(
+ int(len(msg.encoded_bits) * modulator.samples_per_symbol + msg.pause)
+ for msg in messages
+ )
buffer = IQArray(None, dtype=np.float32, n=nsamples)
pos = 0
for i, msg in enumerate(messages):
# We do not need to modulate the pause extra, as result is already initialized with zeros
modulated = modulator.modulate(start=0, data=msg.encoded_bits, pause=0)
- buffer[pos:pos + len(modulated)] = modulated
+ buffer[pos : pos + len(modulated)] = modulated
pos += len(modulated) + msg.pause
cli_progress_bar(i + 1, len(messages), title="Modulating")
print("\nSuccessfully modulated {} messages".format(len(messages)))
@@ -260,90 +294,220 @@ def parse_project_file(file_path: str):
def create_parser():
- parser = argparse.ArgumentParser(description='This is the Command Line Interface for the Universal Radio Hacker.',
- add_help=False)
- parser.add_argument('project_file', nargs='?', default=None)
-
- group1 = parser.add_argument_group('Software Defined Radio Settings', "Configure Software Defined Radio options")
- group1.add_argument("-d", "--device", choices=DEVICES, metavar="DEVICE",
- help="Choose a Software Defined Radio. Allowed values are " + ", ".join(DEVICES))
+ parser = argparse.ArgumentParser(
+ description="This is the Command Line Interface for the Universal Radio Hacker.",
+ add_help=False,
+ )
+ parser.add_argument("project_file", nargs="?", default=None)
+
+ group1 = parser.add_argument_group(
+ "Software Defined Radio Settings", "Configure Software Defined Radio options"
+ )
+ group1.add_argument(
+ "-d",
+ "--device",
+ choices=DEVICES,
+ metavar="DEVICE",
+ help="Choose a Software Defined Radio. Allowed values are "
+ + ", ".join(DEVICES),
+ )
group1.add_argument("-di", "--device-identifier")
- group1.add_argument("-db", "--device-backend", choices=["native", "gnuradio"], default="native")
- group1.add_argument("-f", "--frequency", type=float,
- help="Center frequency the SDR shall be tuned to")
+ group1.add_argument(
+ "-db", "--device-backend", choices=["native", "gnuradio"], default="native"
+ )
+ group1.add_argument(
+ "-f",
+ "--frequency",
+ type=float,
+ help="Center frequency the SDR shall be tuned to",
+ )
group1.add_argument("-s", "--sample-rate", type=float, help="Sample rate to use")
- group1.add_argument("-b", "--bandwidth", type=float, help="Bandwidth to use (defaults to sample rate)")
+ group1.add_argument(
+ "-b",
+ "--bandwidth",
+ type=float,
+ help="Bandwidth to use (defaults to sample rate)",
+ )
group1.add_argument("-g", "--gain", type=int, help="RF gain the SDR shall use")
- group1.add_argument("-if", "--if-gain", type=int, help="IF gain to use (only supported for some SDRs)")
- group1.add_argument("-bb", "--baseband-gain", type=int, help="Baseband gain to use (only supported for some SDRs)")
- group1.add_argument("-a", "--adaptive-noise", action="store_true", help="Use adaptive noise when receiving.")
- group1.add_argument("-fcorr", "--frequency-correction", default=1, type=int,
- help="Set the frequency correction for SDR (if supported)")
-
- group2 = parser.add_argument_group('Modulation/Demodulation settings',
- "Configure the Modulator/Demodulator. Not required in raw mode."
- "In case of RX there are additional demodulation options.")
- group2.add_argument("-cf", "--carrier-frequency", type=float,
- help="Carrier frequency in Hertz (default: {})".format(DEFAULT_CARRIER_FREQUENCY))
- group2.add_argument("-ca", "--carrier-amplitude", type=float,
- help="Carrier amplitude (default: {})".format(DEFAULT_CARRIER_AMPLITUDE))
- group2.add_argument("-cp", "--carrier-phase", type=float,
- help="Carrier phase in degree (default: {})".format(DEFAULT_CARRIER_PHASE))
- group2.add_argument("-mo", "--modulation-type", choices=MODULATIONS, metavar="MOD_TYPE", default="FSK",
- help="Modulation type must be one of " + ", ".join(MODULATIONS) + " (default: %(default)s)")
- group2.add_argument("-bps", "--bits-per-symbol", type=int,
- help="Bits per symbol e.g. 1 means binary modulation (default: 1).")
- group2.add_argument("-pm", "--parameters", nargs='+', help="Parameters for modulation. Separate with spaces")
+ group1.add_argument(
+ "-if",
+ "--if-gain",
+ type=int,
+ help="IF gain to use (only supported for some SDRs)",
+ )
+ group1.add_argument(
+ "-bb",
+ "--baseband-gain",
+ type=int,
+ help="Baseband gain to use (only supported for some SDRs)",
+ )
+ group1.add_argument(
+ "-a",
+ "--adaptive-noise",
+ action="store_true",
+ help="Use adaptive noise when receiving.",
+ )
+ group1.add_argument(
+ "-fcorr",
+ "--frequency-correction",
+ default=1,
+ type=int,
+ help="Set the frequency correction for SDR (if supported)",
+ )
+
+ group2 = parser.add_argument_group(
+ "Modulation/Demodulation settings",
+ "Configure the Modulator/Demodulator. Not required in raw mode."
+ "In case of RX there are additional demodulation options.",
+ )
+ group2.add_argument(
+ "-cf",
+ "--carrier-frequency",
+ type=float,
+ help="Carrier frequency in Hertz (default: {})".format(
+ DEFAULT_CARRIER_FREQUENCY
+ ),
+ )
+ group2.add_argument(
+ "-ca",
+ "--carrier-amplitude",
+ type=float,
+ help="Carrier amplitude (default: {})".format(DEFAULT_CARRIER_AMPLITUDE),
+ )
+ group2.add_argument(
+ "-cp",
+ "--carrier-phase",
+ type=float,
+ help="Carrier phase in degree (default: {})".format(DEFAULT_CARRIER_PHASE),
+ )
+ group2.add_argument(
+ "-mo",
+ "--modulation-type",
+ choices=MODULATIONS,
+ metavar="MOD_TYPE",
+ default="FSK",
+ help="Modulation type must be one of "
+ + ", ".join(MODULATIONS)
+ + " (default: %(default)s)",
+ )
+ group2.add_argument(
+ "-bps",
+ "--bits-per-symbol",
+ type=int,
+ help="Bits per symbol e.g. 1 means binary modulation (default: 1).",
+ )
+ group2.add_argument(
+ "-pm",
+ "--parameters",
+ nargs="+",
+ help="Parameters for modulation. Separate with spaces",
+ )
# Legacy
group2.add_argument("-p0", "--parameter-zero", help=argparse.SUPPRESS)
group2.add_argument("-p1", "--parameter-one", help=argparse.SUPPRESS)
- group2.add_argument("-sps", "--samples-per-symbol", type=int,
- help="Length of a symbol in samples (default: {}).".format(DEFAULT_SAMPLES_PER_SYMBOL))
- group2.add_argument("-bl", "--bit-length", type=int,
- help="Same as samples per symbol, just there for legacy support (default: {}).".format(DEFAULT_SAMPLES_PER_SYMBOL))
-
- group2.add_argument("-n", "--noise", type=float,
- help="Noise threshold (default: {}). Used for RX only.".format(DEFAULT_NOISE))
- group2.add_argument("-c", "--center", type=float,
- help="Center between symbols for demodulation (default: {}). "
- "Used for RX only.".format(DEFAULT_CENTER))
- group2.add_argument("-cs", "--center-spacing", type=float,
- help="Center spacing between symbols for demodulation (default: {}). "
- "Value has only effect for modulations with more than 1 bit per symbol. "
- "Used only for RX.".format(DEFAULT_CENTER_SPACING))
- group2.add_argument("-t", "--tolerance", type=float,
- help="Tolerance for demodulation in samples (default: {}). "
- "Used for RX only.".format(DEFAULT_TOLERANCE))
-
- group3 = parser.add_argument_group('Data configuration', "Configure which data to send or where to receive it.")
- group3.add_argument("--hex", action='store_true', help="Give messages as hex instead of bits")
+ group2.add_argument(
+ "-sps",
+ "--samples-per-symbol",
+ type=int,
+ help="Length of a symbol in samples (default: {}).".format(
+ DEFAULT_SAMPLES_PER_SYMBOL
+ ),
+ )
+ group2.add_argument(
+ "-bl",
+ "--bit-length",
+ type=int,
+ help="Same as samples per symbol, just there for legacy support (default: {}).".format(
+ DEFAULT_SAMPLES_PER_SYMBOL
+ ),
+ )
+
+ group2.add_argument(
+ "-n",
+ "--noise",
+ type=float,
+ help="Noise threshold (default: {}). Used for RX only.".format(DEFAULT_NOISE),
+ )
+ group2.add_argument(
+ "-c",
+ "--center",
+ type=float,
+ help="Center between symbols for demodulation (default: {}). "
+ "Used for RX only.".format(DEFAULT_CENTER),
+ )
+ group2.add_argument(
+ "-cs",
+ "--center-spacing",
+ type=float,
+ help="Center spacing between symbols for demodulation (default: {}). "
+ "Value has only effect for modulations with more than 1 bit per symbol. "
+ "Used only for RX.".format(DEFAULT_CENTER_SPACING),
+ )
+ group2.add_argument(
+ "-t",
+ "--tolerance",
+ type=float,
+ help="Tolerance for demodulation in samples (default: {}). "
+ "Used for RX only.".format(DEFAULT_TOLERANCE),
+ )
+
+ group3 = parser.add_argument_group(
+ "Data configuration", "Configure which data to send or where to receive it."
+ )
+ group3.add_argument(
+ "--hex", action="store_true", help="Give messages as hex instead of bits"
+ )
group3.add_argument("-e", "--encoding", help="Specify encoding")
- group3.add_argument("-m", "--messages", nargs='+', help="Messages to send. Give pauses after with a {0}. "
- "Separate with spaces e.g. "
- "1001{0}42ms 1100{0}3ns 0001 1111{0}200. "
- "If you give no time suffix after a pause "
- "it is assumed to be in samples. ".format(PAUSE_SEP))
-
- group3.add_argument("-file", "--filename", help="Filename to read messages from in send mode. "
- "In receive mode messages will be written to this file "
- "instead to STDOUT.")
- group3.add_argument("-p", "--pause", default="250ms",
- help="The default pause which is inserted after a every message "
- "which does not have a pause configured. (default: %(default)s) "
- "Supported time units: s (second), ms (millisecond), µs (microsecond), ns (nanosecond) "
- "If you do not give a time suffix the pause is assumed to be in samples.")
+ group3.add_argument(
+ "-m",
+ "--messages",
+ nargs="+",
+ help="Messages to send. Give pauses after with a {0}. "
+ "Separate with spaces e.g. "
+ "1001{0}42ms 1100{0}3ns 0001 1111{0}200. "
+ "If you give no time suffix after a pause "
+ "it is assumed to be in samples. ".format(PAUSE_SEP),
+ )
+
+ group3.add_argument(
+ "-file",
+ "--filename",
+ help="Filename to read messages from in send mode. "
+ "In receive mode messages will be written to this file "
+ "instead to STDOUT.",
+ )
+ group3.add_argument(
+ "-p",
+ "--pause",
+ default="250ms",
+ help="The default pause which is inserted after a every message "
+ "which does not have a pause configured. (default: %(default)s) "
+ "Supported time units: s (second), ms (millisecond), µs (microsecond), ns (nanosecond) "
+ "If you do not give a time suffix the pause is assumed to be in samples.",
+ )
group3.add_argument("-rx", "--receive", action="store_true", help="Enter RX mode")
group3.add_argument("-tx", "--transmit", action="store_true", help="Enter TX mode")
- group3.add_argument("-rt", "--receive-time", default="3.0", type=float,
- help="How long to receive messages. (default: %(default)s) "
- "Any negative value means infinite.")
- group3.add_argument("-r", "--raw", action="store_true",
- help="Use raw mode i.e. send/receive IQ data instead of bits.")
+ group3.add_argument(
+ "-rt",
+ "--receive-time",
+ default="3.0",
+ type=float,
+ help="How long to receive messages. (default: %(default)s) "
+ "Any negative value means infinite.",
+ )
+ group3.add_argument(
+ "-r",
+ "--raw",
+ action="store_true",
+ help="Use raw mode i.e. send/receive IQ data instead of bits.",
+ )
group4 = parser.add_argument_group("Miscellaneous options")
- group4.add_argument("-h", "--help", action="help", help="show this help message and exit")
+ group4.add_argument(
+ "-h", "--help", action="help", help="show this help message and exit"
+ )
group4.add_argument("-v", "--verbose", action="count")
return parser
@@ -359,14 +523,17 @@ def get_val(value, project_params: dict, project_param_key: str, default):
return default
import multiprocessing as mp
+
mp.set_start_method("spawn") # allow usage of prange (OpenMP) in Processes
mp.freeze_support()
parser = create_parser()
args = parser.parse_args()
if args.parameter_zero is not None or args.parameter_one is not None:
- print("Options -p0 (--parameter-zero) and -p1 (--parameter-one) are not supported anymore.\n"
- "Use --parameters instead e.g. --parameters 20K 40K for a binary FSK.")
+ print(
+ "Options -p0 (--parameter-zero) and -p1 (--parameter-one) are not supported anymore.\n"
+ "Use --parameters instead e.g. --parameters 20K 40K for a binary FSK."
+ )
sys.exit(1)
project_params = parse_project_file(args.project_file)
@@ -384,19 +551,25 @@ def get_val(value, project_params: dict, project_param_key: str, default):
print("You cannot use receive and transmit mode at the same time.")
sys.exit(1)
if not args.receive and not args.transmit:
- print("You must choose a mode either RX (-rx, --receive) or TX (-tx, --transmit)")
+ print(
+ "You must choose a mode either RX (-rx, --receive) or TX (-tx, --transmit)"
+ )
sys.exit(1)
args.bandwidth = get_val(args.bandwidth, project_params, "bandwidth", None)
rx_tx_prefix = "rx_" if args.receive else "tx_"
args.gain = get_val(args.gain, project_params, rx_tx_prefix + "gain", None)
args.if_gain = get_val(args.if_gain, project_params, rx_tx_prefix + "if_gain", None)
- args.baseband_gain = get_val(args.baseband_gain, project_params, rx_tx_prefix + "baseband_gain", None)
+ args.baseband_gain = get_val(
+ args.baseband_gain, project_params, rx_tx_prefix + "baseband_gain", None
+ )
if args.modulation_type is None:
try:
if project_params["modulation_type"] is None:
- args.modulation_type = MODULATIONS[int(project_params["modulation_index"])]
+ args.modulation_type = MODULATIONS[
+ int(project_params["modulation_index"])
+ ]
else:
args.modulation_type = project_params["modulation_type"]
except:
@@ -405,20 +578,40 @@ def get_val(value, project_params: dict, project_param_key: str, default):
if args.bit_length is not None and args.samples_per_symbol is None:
args.samples_per_symbol = args.bit_length # legacy
else:
- args.samples_per_symbol = get_val(args.samples_per_symbol, project_params,
- "samples_per_symbol", DEFAULT_SAMPLES_PER_SYMBOL)
+ args.samples_per_symbol = get_val(
+ args.samples_per_symbol,
+ project_params,
+ "samples_per_symbol",
+ DEFAULT_SAMPLES_PER_SYMBOL,
+ )
args.center = get_val(args.center, project_params, "center", DEFAULT_CENTER)
- args.center_spacing = get_val(args.center_spacing, project_params, "center_spacing", DEFAULT_CENTER_SPACING)
+ args.center_spacing = get_val(
+ args.center_spacing, project_params, "center_spacing", DEFAULT_CENTER_SPACING
+ )
args.noise = get_val(args.noise, project_params, "noise", DEFAULT_NOISE)
- args.tolerance = get_val(args.tolerance, project_params, "tolerance", DEFAULT_TOLERANCE)
- args.bits_per_symbol = get_val(args.bits_per_symbol, project_params, "bits_per_symbol", 1)
-
- args.carrier_frequency = get_val(args.carrier_frequency, project_params, "carrier_frequency",
- DEFAULT_CARRIER_FREQUENCY)
- args.carrier_amplitude = get_val(args.carrier_amplitude, project_params, "carrier_amplitude",
- DEFAULT_CARRIER_AMPLITUDE)
- args.carrier_phase = get_val(args.carrier_phase, project_params, "carrier_phase", DEFAULT_CARRIER_PHASE)
+ args.tolerance = get_val(
+ args.tolerance, project_params, "tolerance", DEFAULT_TOLERANCE
+ )
+ args.bits_per_symbol = get_val(
+ args.bits_per_symbol, project_params, "bits_per_symbol", 1
+ )
+
+ args.carrier_frequency = get_val(
+ args.carrier_frequency,
+ project_params,
+ "carrier_frequency",
+ DEFAULT_CARRIER_FREQUENCY,
+ )
+ args.carrier_amplitude = get_val(
+ args.carrier_amplitude,
+ project_params,
+ "carrier_amplitude",
+ DEFAULT_CARRIER_AMPLITUDE,
+ )
+ args.carrier_phase = get_val(
+ args.carrier_phase, project_params, "carrier_phase", DEFAULT_CARRIER_PHASE
+ )
args.parameters = get_val(args.parameters, project_params, "parameters", None)
if args.parameters is None:
print("You must give modulation parameters (--parameters)")
@@ -432,14 +625,18 @@ def get_val(value, project_params: dict, project_param_key: str, default):
logger.setLevel(logging.DEBUG)
Logger.save_log_level()
- argument_string = "\n".join("{} {}".format(arg, getattr(args, arg)) for arg in vars(args))
+ argument_string = "\n".join(
+ "{} {}".format(arg, getattr(args, arg)) for arg in vars(args)
+ )
logger.debug("Using these parameters\n" + argument_string)
if args.transmit:
device = build_device_from_args(args)
if args.raw:
if args.filename is None:
- print("You need to give a file (-file, --filename) where to read samples from.")
+ print(
+ "You need to give a file (-file, --filename) where to read samples from."
+ )
sys.exit(1)
samples_to_send = np.fromfile(args.filename, dtype=np.complex64)
else:
@@ -454,7 +651,11 @@ def get_val(value, project_params: dict, project_param_key: str, default):
time.sleep(0.1)
device.read_messages()
if device.current_index > 0:
- cli_progress_bar(device.current_index, len(device.samples_to_send), title="Sending")
+ cli_progress_bar(
+ device.current_index,
+ len(device.samples_to_send),
+ title="Sending",
+ )
except KeyboardInterrupt:
break
@@ -463,7 +664,9 @@ def get_val(value, project_params: dict, project_param_key: str, default):
elif args.receive:
if args.raw:
if args.filename is None:
- print("You need to give a file (-file, --filename) to receive into when using raw RX mode.")
+ print(
+ "You need to give a file (-file, --filename) to receive into when using raw RX mode."
+ )
sys.exit(1)
receiver = build_device_from_args(args)
@@ -495,7 +698,10 @@ def get_val(value, project_params: dict, project_param_key: str, default):
if not args.raw:
num_messages = len(receiver.messages)
for msg in receiver.messages[:num_messages]:
- print(msg.decoded_hex_str if args.hex else msg.decoded_bits_str, **kwargs)
+ print(
+ msg.decoded_hex_str if args.hex else msg.decoded_bits_str,
+ **kwargs
+ )
del receiver.messages[:num_messages]
except KeyboardInterrupt:
break
@@ -503,7 +709,7 @@ def get_val(value, project_params: dict, project_param_key: str, default):
print("\nStopping receiving...")
if args.raw:
receiver.stop("Receiving finished")
- receiver.data[:receiver.current_index].tofile(f)
+ receiver.data[: receiver.current_index].tofile(f)
else:
receiver.stop()
@@ -512,5 +718,5 @@ def get_val(value, project_params: dict, project_param_key: str, default):
print("Received data written to {}".format(args.filename))
-if __name__ == '__main__':
+if __name__ == "__main__":
main()
diff --git a/src/urh/colormaps.py b/src/urh/colormaps.py
index 21cb6c4caf..aa12a3752f 100644
--- a/src/urh/colormaps.py
+++ b/src/urh/colormaps.py
@@ -2,1033 +2,1041 @@
from urh import settings
-magma = [[0.001462, 0.000466, 0.013866],
- [0.002258, 0.001295, 0.018331],
- [0.003279, 0.002305, 0.023708],
- [0.004512, 0.003490, 0.029965],
- [0.005950, 0.004843, 0.037130],
- [0.007588, 0.006356, 0.044973],
- [0.009426, 0.008022, 0.052844],
- [0.011465, 0.009828, 0.060750],
- [0.013708, 0.011771, 0.068667],
- [0.016156, 0.013840, 0.076603],
- [0.018815, 0.016026, 0.084584],
- [0.021692, 0.018320, 0.092610],
- [0.024792, 0.020715, 0.100676],
- [0.028123, 0.023201, 0.108787],
- [0.031696, 0.025765, 0.116965],
- [0.035520, 0.028397, 0.125209],
- [0.039608, 0.031090, 0.133515],
- [0.043830, 0.033830, 0.141886],
- [0.048062, 0.036607, 0.150327],
- [0.052320, 0.039407, 0.158841],
- [0.056615, 0.042160, 0.167446],
- [0.060949, 0.044794, 0.176129],
- [0.065330, 0.047318, 0.184892],
- [0.069764, 0.049726, 0.193735],
- [0.074257, 0.052017, 0.202660],
- [0.078815, 0.054184, 0.211667],
- [0.083446, 0.056225, 0.220755],
- [0.088155, 0.058133, 0.229922],
- [0.092949, 0.059904, 0.239164],
- [0.097833, 0.061531, 0.248477],
- [0.102815, 0.063010, 0.257854],
- [0.107899, 0.064335, 0.267289],
- [0.113094, 0.065492, 0.276784],
- [0.118405, 0.066479, 0.286321],
- [0.123833, 0.067295, 0.295879],
- [0.129380, 0.067935, 0.305443],
- [0.135053, 0.068391, 0.315000],
- [0.140858, 0.068654, 0.324538],
- [0.146785, 0.068738, 0.334011],
- [0.152839, 0.068637, 0.343404],
- [0.159018, 0.068354, 0.352688],
- [0.165308, 0.067911, 0.361816],
- [0.171713, 0.067305, 0.370771],
- [0.178212, 0.066576, 0.379497],
- [0.184801, 0.065732, 0.387973],
- [0.191460, 0.064818, 0.396152],
- [0.198177, 0.063862, 0.404009],
- [0.204935, 0.062907, 0.411514],
- [0.211718, 0.061992, 0.418647],
- [0.218512, 0.061158, 0.425392],
- [0.225302, 0.060445, 0.431742],
- [0.232077, 0.059889, 0.437695],
- [0.238826, 0.059517, 0.443256],
- [0.245543, 0.059352, 0.448436],
- [0.252220, 0.059415, 0.453248],
- [0.258857, 0.059706, 0.457710],
- [0.265447, 0.060237, 0.461840],
- [0.271994, 0.060994, 0.465660],
- [0.278493, 0.061978, 0.469190],
- [0.284951, 0.063168, 0.472451],
- [0.291366, 0.064553, 0.475462],
- [0.297740, 0.066117, 0.478243],
- [0.304081, 0.067835, 0.480812],
- [0.310382, 0.069702, 0.483186],
- [0.316654, 0.071690, 0.485380],
- [0.322899, 0.073782, 0.487408],
- [0.329114, 0.075972, 0.489287],
- [0.335308, 0.078236, 0.491024],
- [0.341482, 0.080564, 0.492631],
- [0.347636, 0.082946, 0.494121],
- [0.353773, 0.085373, 0.495501],
- [0.359898, 0.087831, 0.496778],
- [0.366012, 0.090314, 0.497960],
- [0.372116, 0.092816, 0.499053],
- [0.378211, 0.095332, 0.500067],
- [0.384299, 0.097855, 0.501002],
- [0.390384, 0.100379, 0.501864],
- [0.396467, 0.102902, 0.502658],
- [0.402548, 0.105420, 0.503386],
- [0.408629, 0.107930, 0.504052],
- [0.414709, 0.110431, 0.504662],
- [0.420791, 0.112920, 0.505215],
- [0.426877, 0.115395, 0.505714],
- [0.432967, 0.117855, 0.506160],
- [0.439062, 0.120298, 0.506555],
- [0.445163, 0.122724, 0.506901],
- [0.451271, 0.125132, 0.507198],
- [0.457386, 0.127522, 0.507448],
- [0.463508, 0.129893, 0.507652],
- [0.469640, 0.132245, 0.507809],
- [0.475780, 0.134577, 0.507921],
- [0.481929, 0.136891, 0.507989],
- [0.488088, 0.139186, 0.508011],
- [0.494258, 0.141462, 0.507988],
- [0.500438, 0.143719, 0.507920],
- [0.506629, 0.145958, 0.507806],
- [0.512831, 0.148179, 0.507648],
- [0.519045, 0.150383, 0.507443],
- [0.525270, 0.152569, 0.507192],
- [0.531507, 0.154739, 0.506895],
- [0.537755, 0.156894, 0.506551],
- [0.544015, 0.159033, 0.506159],
- [0.550287, 0.161158, 0.505719],
- [0.556571, 0.163269, 0.505230],
- [0.562866, 0.165368, 0.504692],
- [0.569172, 0.167454, 0.504105],
- [0.575490, 0.169530, 0.503466],
- [0.581819, 0.171596, 0.502777],
- [0.588158, 0.173652, 0.502035],
- [0.594508, 0.175701, 0.501241],
- [0.600868, 0.177743, 0.500394],
- [0.607238, 0.179779, 0.499492],
- [0.613617, 0.181811, 0.498536],
- [0.620005, 0.183840, 0.497524],
- [0.626401, 0.185867, 0.496456],
- [0.632805, 0.187893, 0.495332],
- [0.639216, 0.189921, 0.494150],
- [0.645633, 0.191952, 0.492910],
- [0.652056, 0.193986, 0.491611],
- [0.658483, 0.196027, 0.490253],
- [0.664915, 0.198075, 0.488836],
- [0.671349, 0.200133, 0.487358],
- [0.677786, 0.202203, 0.485819],
- [0.684224, 0.204286, 0.484219],
- [0.690661, 0.206384, 0.482558],
- [0.697098, 0.208501, 0.480835],
- [0.703532, 0.210638, 0.479049],
- [0.709962, 0.212797, 0.477201],
- [0.716387, 0.214982, 0.475290],
- [0.722805, 0.217194, 0.473316],
- [0.729216, 0.219437, 0.471279],
- [0.735616, 0.221713, 0.469180],
- [0.742004, 0.224025, 0.467018],
- [0.748378, 0.226377, 0.464794],
- [0.754737, 0.228772, 0.462509],
- [0.761077, 0.231214, 0.460162],
- [0.767398, 0.233705, 0.457755],
- [0.773695, 0.236249, 0.455289],
- [0.779968, 0.238851, 0.452765],
- [0.786212, 0.241514, 0.450184],
- [0.792427, 0.244242, 0.447543],
- [0.798608, 0.247040, 0.444848],
- [0.804752, 0.249911, 0.442102],
- [0.810855, 0.252861, 0.439305],
- [0.816914, 0.255895, 0.436461],
- [0.822926, 0.259016, 0.433573],
- [0.828886, 0.262229, 0.430644],
- [0.834791, 0.265540, 0.427671],
- [0.840636, 0.268953, 0.424666],
- [0.846416, 0.272473, 0.421631],
- [0.852126, 0.276106, 0.418573],
- [0.857763, 0.279857, 0.415496],
- [0.863320, 0.283729, 0.412403],
- [0.868793, 0.287728, 0.409303],
- [0.874176, 0.291859, 0.406205],
- [0.879464, 0.296125, 0.403118],
- [0.884651, 0.300530, 0.400047],
- [0.889731, 0.305079, 0.397002],
- [0.894700, 0.309773, 0.393995],
- [0.899552, 0.314616, 0.391037],
- [0.904281, 0.319610, 0.388137],
- [0.908884, 0.324755, 0.385308],
- [0.913354, 0.330052, 0.382563],
- [0.917689, 0.335500, 0.379915],
- [0.921884, 0.341098, 0.377376],
- [0.925937, 0.346844, 0.374959],
- [0.929845, 0.352734, 0.372677],
- [0.933606, 0.358764, 0.370541],
- [0.937221, 0.364929, 0.368567],
- [0.940687, 0.371224, 0.366762],
- [0.944006, 0.377643, 0.365136],
- [0.947180, 0.384178, 0.363701],
- [0.950210, 0.390820, 0.362468],
- [0.953099, 0.397563, 0.361438],
- [0.955849, 0.404400, 0.360619],
- [0.958464, 0.411324, 0.360014],
- [0.960949, 0.418323, 0.359630],
- [0.963310, 0.425390, 0.359469],
- [0.965549, 0.432519, 0.359529],
- [0.967671, 0.439703, 0.359810],
- [0.969680, 0.446936, 0.360311],
- [0.971582, 0.454210, 0.361030],
- [0.973381, 0.461520, 0.361965],
- [0.975082, 0.468861, 0.363111],
- [0.976690, 0.476226, 0.364466],
- [0.978210, 0.483612, 0.366025],
- [0.979645, 0.491014, 0.367783],
- [0.981000, 0.498428, 0.369734],
- [0.982279, 0.505851, 0.371874],
- [0.983485, 0.513280, 0.374198],
- [0.984622, 0.520713, 0.376698],
- [0.985693, 0.528148, 0.379371],
- [0.986700, 0.535582, 0.382210],
- [0.987646, 0.543015, 0.385210],
- [0.988533, 0.550446, 0.388365],
- [0.989363, 0.557873, 0.391671],
- [0.990138, 0.565296, 0.395122],
- [0.990871, 0.572706, 0.398714],
- [0.991558, 0.580107, 0.402441],
- [0.992196, 0.587502, 0.406299],
- [0.992785, 0.594891, 0.410283],
- [0.993326, 0.602275, 0.414390],
- [0.993834, 0.609644, 0.418613],
- [0.994309, 0.616999, 0.422950],
- [0.994738, 0.624350, 0.427397],
- [0.995122, 0.631696, 0.431951],
- [0.995480, 0.639027, 0.436607],
- [0.995810, 0.646344, 0.441361],
- [0.996096, 0.653659, 0.446213],
- [0.996341, 0.660969, 0.451160],
- [0.996580, 0.668256, 0.456192],
- [0.996775, 0.675541, 0.461314],
- [0.996925, 0.682828, 0.466526],
- [0.997077, 0.690088, 0.471811],
- [0.997186, 0.697349, 0.477182],
- [0.997254, 0.704611, 0.482635],
- [0.997325, 0.711848, 0.488154],
- [0.997351, 0.719089, 0.493755],
- [0.997351, 0.726324, 0.499428],
- [0.997341, 0.733545, 0.505167],
- [0.997285, 0.740772, 0.510983],
- [0.997228, 0.747981, 0.516859],
- [0.997138, 0.755190, 0.522806],
- [0.997019, 0.762398, 0.528821],
- [0.996898, 0.769591, 0.534892],
- [0.996727, 0.776795, 0.541039],
- [0.996571, 0.783977, 0.547233],
- [0.996369, 0.791167, 0.553499],
- [0.996162, 0.798348, 0.559820],
- [0.995932, 0.805527, 0.566202],
- [0.995680, 0.812706, 0.572645],
- [0.995424, 0.819875, 0.579140],
- [0.995131, 0.827052, 0.585701],
- [0.994851, 0.834213, 0.592307],
- [0.994524, 0.841387, 0.598983],
- [0.994222, 0.848540, 0.605696],
- [0.993866, 0.855711, 0.612482],
- [0.993545, 0.862859, 0.619299],
- [0.993170, 0.870024, 0.626189],
- [0.992831, 0.877168, 0.633109],
- [0.992440, 0.884330, 0.640099],
- [0.992089, 0.891470, 0.647116],
- [0.991688, 0.898627, 0.654202],
- [0.991332, 0.905763, 0.661309],
- [0.990930, 0.912915, 0.668481],
- [0.990570, 0.920049, 0.675675],
- [0.990175, 0.927196, 0.682926],
- [0.989815, 0.934329, 0.690198],
- [0.989434, 0.941470, 0.697519],
- [0.989077, 0.948604, 0.704863],
- [0.988717, 0.955742, 0.712242],
- [0.988367, 0.962878, 0.719649],
- [0.988033, 0.970012, 0.727077],
- [0.987691, 0.977154, 0.734536],
- [0.987387, 0.984288, 0.742002],
- [0.987053, 0.991438, 0.749504]]
+magma = [
+ [0.001462, 0.000466, 0.013866],
+ [0.002258, 0.001295, 0.018331],
+ [0.003279, 0.002305, 0.023708],
+ [0.004512, 0.003490, 0.029965],
+ [0.005950, 0.004843, 0.037130],
+ [0.007588, 0.006356, 0.044973],
+ [0.009426, 0.008022, 0.052844],
+ [0.011465, 0.009828, 0.060750],
+ [0.013708, 0.011771, 0.068667],
+ [0.016156, 0.013840, 0.076603],
+ [0.018815, 0.016026, 0.084584],
+ [0.021692, 0.018320, 0.092610],
+ [0.024792, 0.020715, 0.100676],
+ [0.028123, 0.023201, 0.108787],
+ [0.031696, 0.025765, 0.116965],
+ [0.035520, 0.028397, 0.125209],
+ [0.039608, 0.031090, 0.133515],
+ [0.043830, 0.033830, 0.141886],
+ [0.048062, 0.036607, 0.150327],
+ [0.052320, 0.039407, 0.158841],
+ [0.056615, 0.042160, 0.167446],
+ [0.060949, 0.044794, 0.176129],
+ [0.065330, 0.047318, 0.184892],
+ [0.069764, 0.049726, 0.193735],
+ [0.074257, 0.052017, 0.202660],
+ [0.078815, 0.054184, 0.211667],
+ [0.083446, 0.056225, 0.220755],
+ [0.088155, 0.058133, 0.229922],
+ [0.092949, 0.059904, 0.239164],
+ [0.097833, 0.061531, 0.248477],
+ [0.102815, 0.063010, 0.257854],
+ [0.107899, 0.064335, 0.267289],
+ [0.113094, 0.065492, 0.276784],
+ [0.118405, 0.066479, 0.286321],
+ [0.123833, 0.067295, 0.295879],
+ [0.129380, 0.067935, 0.305443],
+ [0.135053, 0.068391, 0.315000],
+ [0.140858, 0.068654, 0.324538],
+ [0.146785, 0.068738, 0.334011],
+ [0.152839, 0.068637, 0.343404],
+ [0.159018, 0.068354, 0.352688],
+ [0.165308, 0.067911, 0.361816],
+ [0.171713, 0.067305, 0.370771],
+ [0.178212, 0.066576, 0.379497],
+ [0.184801, 0.065732, 0.387973],
+ [0.191460, 0.064818, 0.396152],
+ [0.198177, 0.063862, 0.404009],
+ [0.204935, 0.062907, 0.411514],
+ [0.211718, 0.061992, 0.418647],
+ [0.218512, 0.061158, 0.425392],
+ [0.225302, 0.060445, 0.431742],
+ [0.232077, 0.059889, 0.437695],
+ [0.238826, 0.059517, 0.443256],
+ [0.245543, 0.059352, 0.448436],
+ [0.252220, 0.059415, 0.453248],
+ [0.258857, 0.059706, 0.457710],
+ [0.265447, 0.060237, 0.461840],
+ [0.271994, 0.060994, 0.465660],
+ [0.278493, 0.061978, 0.469190],
+ [0.284951, 0.063168, 0.472451],
+ [0.291366, 0.064553, 0.475462],
+ [0.297740, 0.066117, 0.478243],
+ [0.304081, 0.067835, 0.480812],
+ [0.310382, 0.069702, 0.483186],
+ [0.316654, 0.071690, 0.485380],
+ [0.322899, 0.073782, 0.487408],
+ [0.329114, 0.075972, 0.489287],
+ [0.335308, 0.078236, 0.491024],
+ [0.341482, 0.080564, 0.492631],
+ [0.347636, 0.082946, 0.494121],
+ [0.353773, 0.085373, 0.495501],
+ [0.359898, 0.087831, 0.496778],
+ [0.366012, 0.090314, 0.497960],
+ [0.372116, 0.092816, 0.499053],
+ [0.378211, 0.095332, 0.500067],
+ [0.384299, 0.097855, 0.501002],
+ [0.390384, 0.100379, 0.501864],
+ [0.396467, 0.102902, 0.502658],
+ [0.402548, 0.105420, 0.503386],
+ [0.408629, 0.107930, 0.504052],
+ [0.414709, 0.110431, 0.504662],
+ [0.420791, 0.112920, 0.505215],
+ [0.426877, 0.115395, 0.505714],
+ [0.432967, 0.117855, 0.506160],
+ [0.439062, 0.120298, 0.506555],
+ [0.445163, 0.122724, 0.506901],
+ [0.451271, 0.125132, 0.507198],
+ [0.457386, 0.127522, 0.507448],
+ [0.463508, 0.129893, 0.507652],
+ [0.469640, 0.132245, 0.507809],
+ [0.475780, 0.134577, 0.507921],
+ [0.481929, 0.136891, 0.507989],
+ [0.488088, 0.139186, 0.508011],
+ [0.494258, 0.141462, 0.507988],
+ [0.500438, 0.143719, 0.507920],
+ [0.506629, 0.145958, 0.507806],
+ [0.512831, 0.148179, 0.507648],
+ [0.519045, 0.150383, 0.507443],
+ [0.525270, 0.152569, 0.507192],
+ [0.531507, 0.154739, 0.506895],
+ [0.537755, 0.156894, 0.506551],
+ [0.544015, 0.159033, 0.506159],
+ [0.550287, 0.161158, 0.505719],
+ [0.556571, 0.163269, 0.505230],
+ [0.562866, 0.165368, 0.504692],
+ [0.569172, 0.167454, 0.504105],
+ [0.575490, 0.169530, 0.503466],
+ [0.581819, 0.171596, 0.502777],
+ [0.588158, 0.173652, 0.502035],
+ [0.594508, 0.175701, 0.501241],
+ [0.600868, 0.177743, 0.500394],
+ [0.607238, 0.179779, 0.499492],
+ [0.613617, 0.181811, 0.498536],
+ [0.620005, 0.183840, 0.497524],
+ [0.626401, 0.185867, 0.496456],
+ [0.632805, 0.187893, 0.495332],
+ [0.639216, 0.189921, 0.494150],
+ [0.645633, 0.191952, 0.492910],
+ [0.652056, 0.193986, 0.491611],
+ [0.658483, 0.196027, 0.490253],
+ [0.664915, 0.198075, 0.488836],
+ [0.671349, 0.200133, 0.487358],
+ [0.677786, 0.202203, 0.485819],
+ [0.684224, 0.204286, 0.484219],
+ [0.690661, 0.206384, 0.482558],
+ [0.697098, 0.208501, 0.480835],
+ [0.703532, 0.210638, 0.479049],
+ [0.709962, 0.212797, 0.477201],
+ [0.716387, 0.214982, 0.475290],
+ [0.722805, 0.217194, 0.473316],
+ [0.729216, 0.219437, 0.471279],
+ [0.735616, 0.221713, 0.469180],
+ [0.742004, 0.224025, 0.467018],
+ [0.748378, 0.226377, 0.464794],
+ [0.754737, 0.228772, 0.462509],
+ [0.761077, 0.231214, 0.460162],
+ [0.767398, 0.233705, 0.457755],
+ [0.773695, 0.236249, 0.455289],
+ [0.779968, 0.238851, 0.452765],
+ [0.786212, 0.241514, 0.450184],
+ [0.792427, 0.244242, 0.447543],
+ [0.798608, 0.247040, 0.444848],
+ [0.804752, 0.249911, 0.442102],
+ [0.810855, 0.252861, 0.439305],
+ [0.816914, 0.255895, 0.436461],
+ [0.822926, 0.259016, 0.433573],
+ [0.828886, 0.262229, 0.430644],
+ [0.834791, 0.265540, 0.427671],
+ [0.840636, 0.268953, 0.424666],
+ [0.846416, 0.272473, 0.421631],
+ [0.852126, 0.276106, 0.418573],
+ [0.857763, 0.279857, 0.415496],
+ [0.863320, 0.283729, 0.412403],
+ [0.868793, 0.287728, 0.409303],
+ [0.874176, 0.291859, 0.406205],
+ [0.879464, 0.296125, 0.403118],
+ [0.884651, 0.300530, 0.400047],
+ [0.889731, 0.305079, 0.397002],
+ [0.894700, 0.309773, 0.393995],
+ [0.899552, 0.314616, 0.391037],
+ [0.904281, 0.319610, 0.388137],
+ [0.908884, 0.324755, 0.385308],
+ [0.913354, 0.330052, 0.382563],
+ [0.917689, 0.335500, 0.379915],
+ [0.921884, 0.341098, 0.377376],
+ [0.925937, 0.346844, 0.374959],
+ [0.929845, 0.352734, 0.372677],
+ [0.933606, 0.358764, 0.370541],
+ [0.937221, 0.364929, 0.368567],
+ [0.940687, 0.371224, 0.366762],
+ [0.944006, 0.377643, 0.365136],
+ [0.947180, 0.384178, 0.363701],
+ [0.950210, 0.390820, 0.362468],
+ [0.953099, 0.397563, 0.361438],
+ [0.955849, 0.404400, 0.360619],
+ [0.958464, 0.411324, 0.360014],
+ [0.960949, 0.418323, 0.359630],
+ [0.963310, 0.425390, 0.359469],
+ [0.965549, 0.432519, 0.359529],
+ [0.967671, 0.439703, 0.359810],
+ [0.969680, 0.446936, 0.360311],
+ [0.971582, 0.454210, 0.361030],
+ [0.973381, 0.461520, 0.361965],
+ [0.975082, 0.468861, 0.363111],
+ [0.976690, 0.476226, 0.364466],
+ [0.978210, 0.483612, 0.366025],
+ [0.979645, 0.491014, 0.367783],
+ [0.981000, 0.498428, 0.369734],
+ [0.982279, 0.505851, 0.371874],
+ [0.983485, 0.513280, 0.374198],
+ [0.984622, 0.520713, 0.376698],
+ [0.985693, 0.528148, 0.379371],
+ [0.986700, 0.535582, 0.382210],
+ [0.987646, 0.543015, 0.385210],
+ [0.988533, 0.550446, 0.388365],
+ [0.989363, 0.557873, 0.391671],
+ [0.990138, 0.565296, 0.395122],
+ [0.990871, 0.572706, 0.398714],
+ [0.991558, 0.580107, 0.402441],
+ [0.992196, 0.587502, 0.406299],
+ [0.992785, 0.594891, 0.410283],
+ [0.993326, 0.602275, 0.414390],
+ [0.993834, 0.609644, 0.418613],
+ [0.994309, 0.616999, 0.422950],
+ [0.994738, 0.624350, 0.427397],
+ [0.995122, 0.631696, 0.431951],
+ [0.995480, 0.639027, 0.436607],
+ [0.995810, 0.646344, 0.441361],
+ [0.996096, 0.653659, 0.446213],
+ [0.996341, 0.660969, 0.451160],
+ [0.996580, 0.668256, 0.456192],
+ [0.996775, 0.675541, 0.461314],
+ [0.996925, 0.682828, 0.466526],
+ [0.997077, 0.690088, 0.471811],
+ [0.997186, 0.697349, 0.477182],
+ [0.997254, 0.704611, 0.482635],
+ [0.997325, 0.711848, 0.488154],
+ [0.997351, 0.719089, 0.493755],
+ [0.997351, 0.726324, 0.499428],
+ [0.997341, 0.733545, 0.505167],
+ [0.997285, 0.740772, 0.510983],
+ [0.997228, 0.747981, 0.516859],
+ [0.997138, 0.755190, 0.522806],
+ [0.997019, 0.762398, 0.528821],
+ [0.996898, 0.769591, 0.534892],
+ [0.996727, 0.776795, 0.541039],
+ [0.996571, 0.783977, 0.547233],
+ [0.996369, 0.791167, 0.553499],
+ [0.996162, 0.798348, 0.559820],
+ [0.995932, 0.805527, 0.566202],
+ [0.995680, 0.812706, 0.572645],
+ [0.995424, 0.819875, 0.579140],
+ [0.995131, 0.827052, 0.585701],
+ [0.994851, 0.834213, 0.592307],
+ [0.994524, 0.841387, 0.598983],
+ [0.994222, 0.848540, 0.605696],
+ [0.993866, 0.855711, 0.612482],
+ [0.993545, 0.862859, 0.619299],
+ [0.993170, 0.870024, 0.626189],
+ [0.992831, 0.877168, 0.633109],
+ [0.992440, 0.884330, 0.640099],
+ [0.992089, 0.891470, 0.647116],
+ [0.991688, 0.898627, 0.654202],
+ [0.991332, 0.905763, 0.661309],
+ [0.990930, 0.912915, 0.668481],
+ [0.990570, 0.920049, 0.675675],
+ [0.990175, 0.927196, 0.682926],
+ [0.989815, 0.934329, 0.690198],
+ [0.989434, 0.941470, 0.697519],
+ [0.989077, 0.948604, 0.704863],
+ [0.988717, 0.955742, 0.712242],
+ [0.988367, 0.962878, 0.719649],
+ [0.988033, 0.970012, 0.727077],
+ [0.987691, 0.977154, 0.734536],
+ [0.987387, 0.984288, 0.742002],
+ [0.987053, 0.991438, 0.749504],
+]
-inferno = [[0.001462, 0.000466, 0.013866],
- [0.002267, 0.001270, 0.018570],
- [0.003299, 0.002249, 0.024239],
- [0.004547, 0.003392, 0.030909],
- [0.006006, 0.004692, 0.038558],
- [0.007676, 0.006136, 0.046836],
- [0.009561, 0.007713, 0.055143],
- [0.011663, 0.009417, 0.063460],
- [0.013995, 0.011225, 0.071862],
- [0.016561, 0.013136, 0.080282],
- [0.019373, 0.015133, 0.088767],
- [0.022447, 0.017199, 0.097327],
- [0.025793, 0.019331, 0.105930],
- [0.029432, 0.021503, 0.114621],
- [0.033385, 0.023702, 0.123397],
- [0.037668, 0.025921, 0.132232],
- [0.042253, 0.028139, 0.141141],
- [0.046915, 0.030324, 0.150164],
- [0.051644, 0.032474, 0.159254],
- [0.056449, 0.034569, 0.168414],
- [0.061340, 0.036590, 0.177642],
- [0.066331, 0.038504, 0.186962],
- [0.071429, 0.040294, 0.196354],
- [0.076637, 0.041905, 0.205799],
- [0.081962, 0.043328, 0.215289],
- [0.087411, 0.044556, 0.224813],
- [0.092990, 0.045583, 0.234358],
- [0.098702, 0.046402, 0.243904],
- [0.104551, 0.047008, 0.253430],
- [0.110536, 0.047399, 0.262912],
- [0.116656, 0.047574, 0.272321],
- [0.122908, 0.047536, 0.281624],
- [0.129285, 0.047293, 0.290788],
- [0.135778, 0.046856, 0.299776],
- [0.142378, 0.046242, 0.308553],
- [0.149073, 0.045468, 0.317085],
- [0.155850, 0.044559, 0.325338],
- [0.162689, 0.043554, 0.333277],
- [0.169575, 0.042489, 0.340874],
- [0.176493, 0.041402, 0.348111],
- [0.183429, 0.040329, 0.354971],
- [0.190367, 0.039309, 0.361447],
- [0.197297, 0.038400, 0.367535],
- [0.204209, 0.037632, 0.373238],
- [0.211095, 0.037030, 0.378563],
- [0.217949, 0.036615, 0.383522],
- [0.224763, 0.036405, 0.388129],
- [0.231538, 0.036405, 0.392400],
- [0.238273, 0.036621, 0.396353],
- [0.244967, 0.037055, 0.400007],
- [0.251620, 0.037705, 0.403378],
- [0.258234, 0.038571, 0.406485],
- [0.264810, 0.039647, 0.409345],
- [0.271347, 0.040922, 0.411976],
- [0.277850, 0.042353, 0.414392],
- [0.284321, 0.043933, 0.416608],
- [0.290763, 0.045644, 0.418637],
- [0.297178, 0.047470, 0.420491],
- [0.303568, 0.049396, 0.422182],
- [0.309935, 0.051407, 0.423721],
- [0.316282, 0.053490, 0.425116],
- [0.322610, 0.055634, 0.426377],
- [0.328921, 0.057827, 0.427511],
- [0.335217, 0.060060, 0.428524],
- [0.341500, 0.062325, 0.429425],
- [0.347771, 0.064616, 0.430217],
- [0.354032, 0.066925, 0.430906],
- [0.360284, 0.069247, 0.431497],
- [0.366529, 0.071579, 0.431994],
- [0.372768, 0.073915, 0.432400],
- [0.379001, 0.076253, 0.432719],
- [0.385228, 0.078591, 0.432955],
- [0.391453, 0.080927, 0.433109],
- [0.397674, 0.083257, 0.433183],
- [0.403894, 0.085580, 0.433179],
- [0.410113, 0.087896, 0.433098],
- [0.416331, 0.090203, 0.432943],
- [0.422549, 0.092501, 0.432714],
- [0.428768, 0.094790, 0.432412],
- [0.434987, 0.097069, 0.432039],
- [0.441207, 0.099338, 0.431594],
- [0.447428, 0.101597, 0.431080],
- [0.453651, 0.103848, 0.430498],
- [0.459875, 0.106089, 0.429846],
- [0.466100, 0.108322, 0.429125],
- [0.472328, 0.110547, 0.428334],
- [0.478558, 0.112764, 0.427475],
- [0.484789, 0.114974, 0.426548],
- [0.491022, 0.117179, 0.425552],
- [0.497257, 0.119379, 0.424488],
- [0.503493, 0.121575, 0.423356],
- [0.509730, 0.123769, 0.422156],
- [0.515967, 0.125960, 0.420887],
- [0.522206, 0.128150, 0.419549],
- [0.528444, 0.130341, 0.418142],
- [0.534683, 0.132534, 0.416667],
- [0.540920, 0.134729, 0.415123],
- [0.547157, 0.136929, 0.413511],
- [0.553392, 0.139134, 0.411829],
- [0.559624, 0.141346, 0.410078],
- [0.565854, 0.143567, 0.408258],
- [0.572081, 0.145797, 0.406369],
- [0.578304, 0.148039, 0.404411],
- [0.584521, 0.150294, 0.402385],
- [0.590734, 0.152563, 0.400290],
- [0.596940, 0.154848, 0.398125],
- [0.603139, 0.157151, 0.395891],
- [0.609330, 0.159474, 0.393589],
- [0.615513, 0.161817, 0.391219],
- [0.621685, 0.164184, 0.388781],
- [0.627847, 0.166575, 0.386276],
- [0.633998, 0.168992, 0.383704],
- [0.640135, 0.171438, 0.381065],
- [0.646260, 0.173914, 0.378359],
- [0.652369, 0.176421, 0.375586],
- [0.658463, 0.178962, 0.372748],
- [0.664540, 0.181539, 0.369846],
- [0.670599, 0.184153, 0.366879],
- [0.676638, 0.186807, 0.363849],
- [0.682656, 0.189501, 0.360757],
- [0.688653, 0.192239, 0.357603],
- [0.694627, 0.195021, 0.354388],
- [0.700576, 0.197851, 0.351113],
- [0.706500, 0.200728, 0.347777],
- [0.712396, 0.203656, 0.344383],
- [0.718264, 0.206636, 0.340931],
- [0.724103, 0.209670, 0.337424],
- [0.729909, 0.212759, 0.333861],
- [0.735683, 0.215906, 0.330245],
- [0.741423, 0.219112, 0.326576],
- [0.747127, 0.222378, 0.322856],
- [0.752794, 0.225706, 0.319085],
- [0.758422, 0.229097, 0.315266],
- [0.764010, 0.232554, 0.311399],
- [0.769556, 0.236077, 0.307485],
- [0.775059, 0.239667, 0.303526],
- [0.780517, 0.243327, 0.299523],
- [0.785929, 0.247056, 0.295477],
- [0.791293, 0.250856, 0.291390],
- [0.796607, 0.254728, 0.287264],
- [0.801871, 0.258674, 0.283099],
- [0.807082, 0.262692, 0.278898],
- [0.812239, 0.266786, 0.274661],
- [0.817341, 0.270954, 0.270390],
- [0.822386, 0.275197, 0.266085],
- [0.827372, 0.279517, 0.261750],
- [0.832299, 0.283913, 0.257383],
- [0.837165, 0.288385, 0.252988],
- [0.841969, 0.292933, 0.248564],
- [0.846709, 0.297559, 0.244113],
- [0.851384, 0.302260, 0.239636],
- [0.855992, 0.307038, 0.235133],
- [0.860533, 0.311892, 0.230606],
- [0.865006, 0.316822, 0.226055],
- [0.869409, 0.321827, 0.221482],
- [0.873741, 0.326906, 0.216886],
- [0.878001, 0.332060, 0.212268],
- [0.882188, 0.337287, 0.207628],
- [0.886302, 0.342586, 0.202968],
- [0.890341, 0.347957, 0.198286],
- [0.894305, 0.353399, 0.193584],
- [0.898192, 0.358911, 0.188860],
- [0.902003, 0.364492, 0.184116],
- [0.905735, 0.370140, 0.179350],
- [0.909390, 0.375856, 0.174563],
- [0.912966, 0.381636, 0.169755],
- [0.916462, 0.387481, 0.164924],
- [0.919879, 0.393389, 0.160070],
- [0.923215, 0.399359, 0.155193],
- [0.926470, 0.405389, 0.150292],
- [0.929644, 0.411479, 0.145367],
- [0.932737, 0.417627, 0.140417],
- [0.935747, 0.423831, 0.135440],
- [0.938675, 0.430091, 0.130438],
- [0.941521, 0.436405, 0.125409],
- [0.944285, 0.442772, 0.120354],
- [0.946965, 0.449191, 0.115272],
- [0.949562, 0.455660, 0.110164],
- [0.952075, 0.462178, 0.105031],
- [0.954506, 0.468744, 0.099874],
- [0.956852, 0.475356, 0.094695],
- [0.959114, 0.482014, 0.089499],
- [0.961293, 0.488716, 0.084289],
- [0.963387, 0.495462, 0.079073],
- [0.965397, 0.502249, 0.073859],
- [0.967322, 0.509078, 0.068659],
- [0.969163, 0.515946, 0.063488],
- [0.970919, 0.522853, 0.058367],
- [0.972590, 0.529798, 0.053324],
- [0.974176, 0.536780, 0.048392],
- [0.975677, 0.543798, 0.043618],
- [0.977092, 0.550850, 0.039050],
- [0.978422, 0.557937, 0.034931],
- [0.979666, 0.565057, 0.031409],
- [0.980824, 0.572209, 0.028508],
- [0.981895, 0.579392, 0.026250],
- [0.982881, 0.586606, 0.024661],
- [0.983779, 0.593849, 0.023770],
- [0.984591, 0.601122, 0.023606],
- [0.985315, 0.608422, 0.024202],
- [0.985952, 0.615750, 0.025592],
- [0.986502, 0.623105, 0.027814],
- [0.986964, 0.630485, 0.030908],
- [0.987337, 0.637890, 0.034916],
- [0.987622, 0.645320, 0.039886],
- [0.987819, 0.652773, 0.045581],
- [0.987926, 0.660250, 0.051750],
- [0.987945, 0.667748, 0.058329],
- [0.987874, 0.675267, 0.065257],
- [0.987714, 0.682807, 0.072489],
- [0.987464, 0.690366, 0.079990],
- [0.987124, 0.697944, 0.087731],
- [0.986694, 0.705540, 0.095694],
- [0.986175, 0.713153, 0.103863],
- [0.985566, 0.720782, 0.112229],
- [0.984865, 0.728427, 0.120785],
- [0.984075, 0.736087, 0.129527],
- [0.983196, 0.743758, 0.138453],
- [0.982228, 0.751442, 0.147565],
- [0.981173, 0.759135, 0.156863],
- [0.980032, 0.766837, 0.166353],
- [0.978806, 0.774545, 0.176037],
- [0.977497, 0.782258, 0.185923],
- [0.976108, 0.789974, 0.196018],
- [0.974638, 0.797692, 0.206332],
- [0.973088, 0.805409, 0.216877],
- [0.971468, 0.813122, 0.227658],
- [0.969783, 0.820825, 0.238686],
- [0.968041, 0.828515, 0.249972],
- [0.966243, 0.836191, 0.261534],
- [0.964394, 0.843848, 0.273391],
- [0.962517, 0.851476, 0.285546],
- [0.960626, 0.859069, 0.298010],
- [0.958720, 0.866624, 0.310820],
- [0.956834, 0.874129, 0.323974],
- [0.954997, 0.881569, 0.337475],
- [0.953215, 0.888942, 0.351369],
- [0.951546, 0.896226, 0.365627],
- [0.950018, 0.903409, 0.380271],
- [0.948683, 0.910473, 0.395289],
- [0.947594, 0.917399, 0.410665],
- [0.946809, 0.924168, 0.426373],
- [0.946392, 0.930761, 0.442367],
- [0.946403, 0.937159, 0.458592],
- [0.946903, 0.943348, 0.474970],
- [0.947937, 0.949318, 0.491426],
- [0.949545, 0.955063, 0.507860],
- [0.951740, 0.960587, 0.524203],
- [0.954529, 0.965896, 0.540361],
- [0.957896, 0.971003, 0.556275],
- [0.961812, 0.975924, 0.571925],
- [0.966249, 0.980678, 0.587206],
- [0.971162, 0.985282, 0.602154],
- [0.976511, 0.989753, 0.616760],
- [0.982257, 0.994109, 0.631017],
- [0.988362, 0.998364, 0.644924]]
+inferno = [
+ [0.001462, 0.000466, 0.013866],
+ [0.002267, 0.001270, 0.018570],
+ [0.003299, 0.002249, 0.024239],
+ [0.004547, 0.003392, 0.030909],
+ [0.006006, 0.004692, 0.038558],
+ [0.007676, 0.006136, 0.046836],
+ [0.009561, 0.007713, 0.055143],
+ [0.011663, 0.009417, 0.063460],
+ [0.013995, 0.011225, 0.071862],
+ [0.016561, 0.013136, 0.080282],
+ [0.019373, 0.015133, 0.088767],
+ [0.022447, 0.017199, 0.097327],
+ [0.025793, 0.019331, 0.105930],
+ [0.029432, 0.021503, 0.114621],
+ [0.033385, 0.023702, 0.123397],
+ [0.037668, 0.025921, 0.132232],
+ [0.042253, 0.028139, 0.141141],
+ [0.046915, 0.030324, 0.150164],
+ [0.051644, 0.032474, 0.159254],
+ [0.056449, 0.034569, 0.168414],
+ [0.061340, 0.036590, 0.177642],
+ [0.066331, 0.038504, 0.186962],
+ [0.071429, 0.040294, 0.196354],
+ [0.076637, 0.041905, 0.205799],
+ [0.081962, 0.043328, 0.215289],
+ [0.087411, 0.044556, 0.224813],
+ [0.092990, 0.045583, 0.234358],
+ [0.098702, 0.046402, 0.243904],
+ [0.104551, 0.047008, 0.253430],
+ [0.110536, 0.047399, 0.262912],
+ [0.116656, 0.047574, 0.272321],
+ [0.122908, 0.047536, 0.281624],
+ [0.129285, 0.047293, 0.290788],
+ [0.135778, 0.046856, 0.299776],
+ [0.142378, 0.046242, 0.308553],
+ [0.149073, 0.045468, 0.317085],
+ [0.155850, 0.044559, 0.325338],
+ [0.162689, 0.043554, 0.333277],
+ [0.169575, 0.042489, 0.340874],
+ [0.176493, 0.041402, 0.348111],
+ [0.183429, 0.040329, 0.354971],
+ [0.190367, 0.039309, 0.361447],
+ [0.197297, 0.038400, 0.367535],
+ [0.204209, 0.037632, 0.373238],
+ [0.211095, 0.037030, 0.378563],
+ [0.217949, 0.036615, 0.383522],
+ [0.224763, 0.036405, 0.388129],
+ [0.231538, 0.036405, 0.392400],
+ [0.238273, 0.036621, 0.396353],
+ [0.244967, 0.037055, 0.400007],
+ [0.251620, 0.037705, 0.403378],
+ [0.258234, 0.038571, 0.406485],
+ [0.264810, 0.039647, 0.409345],
+ [0.271347, 0.040922, 0.411976],
+ [0.277850, 0.042353, 0.414392],
+ [0.284321, 0.043933, 0.416608],
+ [0.290763, 0.045644, 0.418637],
+ [0.297178, 0.047470, 0.420491],
+ [0.303568, 0.049396, 0.422182],
+ [0.309935, 0.051407, 0.423721],
+ [0.316282, 0.053490, 0.425116],
+ [0.322610, 0.055634, 0.426377],
+ [0.328921, 0.057827, 0.427511],
+ [0.335217, 0.060060, 0.428524],
+ [0.341500, 0.062325, 0.429425],
+ [0.347771, 0.064616, 0.430217],
+ [0.354032, 0.066925, 0.430906],
+ [0.360284, 0.069247, 0.431497],
+ [0.366529, 0.071579, 0.431994],
+ [0.372768, 0.073915, 0.432400],
+ [0.379001, 0.076253, 0.432719],
+ [0.385228, 0.078591, 0.432955],
+ [0.391453, 0.080927, 0.433109],
+ [0.397674, 0.083257, 0.433183],
+ [0.403894, 0.085580, 0.433179],
+ [0.410113, 0.087896, 0.433098],
+ [0.416331, 0.090203, 0.432943],
+ [0.422549, 0.092501, 0.432714],
+ [0.428768, 0.094790, 0.432412],
+ [0.434987, 0.097069, 0.432039],
+ [0.441207, 0.099338, 0.431594],
+ [0.447428, 0.101597, 0.431080],
+ [0.453651, 0.103848, 0.430498],
+ [0.459875, 0.106089, 0.429846],
+ [0.466100, 0.108322, 0.429125],
+ [0.472328, 0.110547, 0.428334],
+ [0.478558, 0.112764, 0.427475],
+ [0.484789, 0.114974, 0.426548],
+ [0.491022, 0.117179, 0.425552],
+ [0.497257, 0.119379, 0.424488],
+ [0.503493, 0.121575, 0.423356],
+ [0.509730, 0.123769, 0.422156],
+ [0.515967, 0.125960, 0.420887],
+ [0.522206, 0.128150, 0.419549],
+ [0.528444, 0.130341, 0.418142],
+ [0.534683, 0.132534, 0.416667],
+ [0.540920, 0.134729, 0.415123],
+ [0.547157, 0.136929, 0.413511],
+ [0.553392, 0.139134, 0.411829],
+ [0.559624, 0.141346, 0.410078],
+ [0.565854, 0.143567, 0.408258],
+ [0.572081, 0.145797, 0.406369],
+ [0.578304, 0.148039, 0.404411],
+ [0.584521, 0.150294, 0.402385],
+ [0.590734, 0.152563, 0.400290],
+ [0.596940, 0.154848, 0.398125],
+ [0.603139, 0.157151, 0.395891],
+ [0.609330, 0.159474, 0.393589],
+ [0.615513, 0.161817, 0.391219],
+ [0.621685, 0.164184, 0.388781],
+ [0.627847, 0.166575, 0.386276],
+ [0.633998, 0.168992, 0.383704],
+ [0.640135, 0.171438, 0.381065],
+ [0.646260, 0.173914, 0.378359],
+ [0.652369, 0.176421, 0.375586],
+ [0.658463, 0.178962, 0.372748],
+ [0.664540, 0.181539, 0.369846],
+ [0.670599, 0.184153, 0.366879],
+ [0.676638, 0.186807, 0.363849],
+ [0.682656, 0.189501, 0.360757],
+ [0.688653, 0.192239, 0.357603],
+ [0.694627, 0.195021, 0.354388],
+ [0.700576, 0.197851, 0.351113],
+ [0.706500, 0.200728, 0.347777],
+ [0.712396, 0.203656, 0.344383],
+ [0.718264, 0.206636, 0.340931],
+ [0.724103, 0.209670, 0.337424],
+ [0.729909, 0.212759, 0.333861],
+ [0.735683, 0.215906, 0.330245],
+ [0.741423, 0.219112, 0.326576],
+ [0.747127, 0.222378, 0.322856],
+ [0.752794, 0.225706, 0.319085],
+ [0.758422, 0.229097, 0.315266],
+ [0.764010, 0.232554, 0.311399],
+ [0.769556, 0.236077, 0.307485],
+ [0.775059, 0.239667, 0.303526],
+ [0.780517, 0.243327, 0.299523],
+ [0.785929, 0.247056, 0.295477],
+ [0.791293, 0.250856, 0.291390],
+ [0.796607, 0.254728, 0.287264],
+ [0.801871, 0.258674, 0.283099],
+ [0.807082, 0.262692, 0.278898],
+ [0.812239, 0.266786, 0.274661],
+ [0.817341, 0.270954, 0.270390],
+ [0.822386, 0.275197, 0.266085],
+ [0.827372, 0.279517, 0.261750],
+ [0.832299, 0.283913, 0.257383],
+ [0.837165, 0.288385, 0.252988],
+ [0.841969, 0.292933, 0.248564],
+ [0.846709, 0.297559, 0.244113],
+ [0.851384, 0.302260, 0.239636],
+ [0.855992, 0.307038, 0.235133],
+ [0.860533, 0.311892, 0.230606],
+ [0.865006, 0.316822, 0.226055],
+ [0.869409, 0.321827, 0.221482],
+ [0.873741, 0.326906, 0.216886],
+ [0.878001, 0.332060, 0.212268],
+ [0.882188, 0.337287, 0.207628],
+ [0.886302, 0.342586, 0.202968],
+ [0.890341, 0.347957, 0.198286],
+ [0.894305, 0.353399, 0.193584],
+ [0.898192, 0.358911, 0.188860],
+ [0.902003, 0.364492, 0.184116],
+ [0.905735, 0.370140, 0.179350],
+ [0.909390, 0.375856, 0.174563],
+ [0.912966, 0.381636, 0.169755],
+ [0.916462, 0.387481, 0.164924],
+ [0.919879, 0.393389, 0.160070],
+ [0.923215, 0.399359, 0.155193],
+ [0.926470, 0.405389, 0.150292],
+ [0.929644, 0.411479, 0.145367],
+ [0.932737, 0.417627, 0.140417],
+ [0.935747, 0.423831, 0.135440],
+ [0.938675, 0.430091, 0.130438],
+ [0.941521, 0.436405, 0.125409],
+ [0.944285, 0.442772, 0.120354],
+ [0.946965, 0.449191, 0.115272],
+ [0.949562, 0.455660, 0.110164],
+ [0.952075, 0.462178, 0.105031],
+ [0.954506, 0.468744, 0.099874],
+ [0.956852, 0.475356, 0.094695],
+ [0.959114, 0.482014, 0.089499],
+ [0.961293, 0.488716, 0.084289],
+ [0.963387, 0.495462, 0.079073],
+ [0.965397, 0.502249, 0.073859],
+ [0.967322, 0.509078, 0.068659],
+ [0.969163, 0.515946, 0.063488],
+ [0.970919, 0.522853, 0.058367],
+ [0.972590, 0.529798, 0.053324],
+ [0.974176, 0.536780, 0.048392],
+ [0.975677, 0.543798, 0.043618],
+ [0.977092, 0.550850, 0.039050],
+ [0.978422, 0.557937, 0.034931],
+ [0.979666, 0.565057, 0.031409],
+ [0.980824, 0.572209, 0.028508],
+ [0.981895, 0.579392, 0.026250],
+ [0.982881, 0.586606, 0.024661],
+ [0.983779, 0.593849, 0.023770],
+ [0.984591, 0.601122, 0.023606],
+ [0.985315, 0.608422, 0.024202],
+ [0.985952, 0.615750, 0.025592],
+ [0.986502, 0.623105, 0.027814],
+ [0.986964, 0.630485, 0.030908],
+ [0.987337, 0.637890, 0.034916],
+ [0.987622, 0.645320, 0.039886],
+ [0.987819, 0.652773, 0.045581],
+ [0.987926, 0.660250, 0.051750],
+ [0.987945, 0.667748, 0.058329],
+ [0.987874, 0.675267, 0.065257],
+ [0.987714, 0.682807, 0.072489],
+ [0.987464, 0.690366, 0.079990],
+ [0.987124, 0.697944, 0.087731],
+ [0.986694, 0.705540, 0.095694],
+ [0.986175, 0.713153, 0.103863],
+ [0.985566, 0.720782, 0.112229],
+ [0.984865, 0.728427, 0.120785],
+ [0.984075, 0.736087, 0.129527],
+ [0.983196, 0.743758, 0.138453],
+ [0.982228, 0.751442, 0.147565],
+ [0.981173, 0.759135, 0.156863],
+ [0.980032, 0.766837, 0.166353],
+ [0.978806, 0.774545, 0.176037],
+ [0.977497, 0.782258, 0.185923],
+ [0.976108, 0.789974, 0.196018],
+ [0.974638, 0.797692, 0.206332],
+ [0.973088, 0.805409, 0.216877],
+ [0.971468, 0.813122, 0.227658],
+ [0.969783, 0.820825, 0.238686],
+ [0.968041, 0.828515, 0.249972],
+ [0.966243, 0.836191, 0.261534],
+ [0.964394, 0.843848, 0.273391],
+ [0.962517, 0.851476, 0.285546],
+ [0.960626, 0.859069, 0.298010],
+ [0.958720, 0.866624, 0.310820],
+ [0.956834, 0.874129, 0.323974],
+ [0.954997, 0.881569, 0.337475],
+ [0.953215, 0.888942, 0.351369],
+ [0.951546, 0.896226, 0.365627],
+ [0.950018, 0.903409, 0.380271],
+ [0.948683, 0.910473, 0.395289],
+ [0.947594, 0.917399, 0.410665],
+ [0.946809, 0.924168, 0.426373],
+ [0.946392, 0.930761, 0.442367],
+ [0.946403, 0.937159, 0.458592],
+ [0.946903, 0.943348, 0.474970],
+ [0.947937, 0.949318, 0.491426],
+ [0.949545, 0.955063, 0.507860],
+ [0.951740, 0.960587, 0.524203],
+ [0.954529, 0.965896, 0.540361],
+ [0.957896, 0.971003, 0.556275],
+ [0.961812, 0.975924, 0.571925],
+ [0.966249, 0.980678, 0.587206],
+ [0.971162, 0.985282, 0.602154],
+ [0.976511, 0.989753, 0.616760],
+ [0.982257, 0.994109, 0.631017],
+ [0.988362, 0.998364, 0.644924],
+]
-plasma = [[0.050383, 0.029803, 0.527975],
- [0.063536, 0.028426, 0.533124],
- [0.075353, 0.027206, 0.538007],
- [0.086222, 0.026125, 0.542658],
- [0.096379, 0.025165, 0.547103],
- [0.105980, 0.024309, 0.551368],
- [0.115124, 0.023556, 0.555468],
- [0.123903, 0.022878, 0.559423],
- [0.132381, 0.022258, 0.563250],
- [0.140603, 0.021687, 0.566959],
- [0.148607, 0.021154, 0.570562],
- [0.156421, 0.020651, 0.574065],
- [0.164070, 0.020171, 0.577478],
- [0.171574, 0.019706, 0.580806],
- [0.178950, 0.019252, 0.584054],
- [0.186213, 0.018803, 0.587228],
- [0.193374, 0.018354, 0.590330],
- [0.200445, 0.017902, 0.593364],
- [0.207435, 0.017442, 0.596333],
- [0.214350, 0.016973, 0.599239],
- [0.221197, 0.016497, 0.602083],
- [0.227983, 0.016007, 0.604867],
- [0.234715, 0.015502, 0.607592],
- [0.241396, 0.014979, 0.610259],
- [0.248032, 0.014439, 0.612868],
- [0.254627, 0.013882, 0.615419],
- [0.261183, 0.013308, 0.617911],
- [0.267703, 0.012716, 0.620346],
- [0.274191, 0.012109, 0.622722],
- [0.280648, 0.011488, 0.625038],
- [0.287076, 0.010855, 0.627295],
- [0.293478, 0.010213, 0.629490],
- [0.299855, 0.009561, 0.631624],
- [0.306210, 0.008902, 0.633694],
- [0.312543, 0.008239, 0.635700],
- [0.318856, 0.007576, 0.637640],
- [0.325150, 0.006915, 0.639512],
- [0.331426, 0.006261, 0.641316],
- [0.337683, 0.005618, 0.643049],
- [0.343925, 0.004991, 0.644710],
- [0.350150, 0.004382, 0.646298],
- [0.356359, 0.003798, 0.647810],
- [0.362553, 0.003243, 0.649245],
- [0.368733, 0.002724, 0.650601],
- [0.374897, 0.002245, 0.651876],
- [0.381047, 0.001814, 0.653068],
- [0.387183, 0.001434, 0.654177],
- [0.393304, 0.001114, 0.655199],
- [0.399411, 0.000859, 0.656133],
- [0.405503, 0.000678, 0.656977],
- [0.411580, 0.000577, 0.657730],
- [0.417642, 0.000564, 0.658390],
- [0.423689, 0.000646, 0.658956],
- [0.429719, 0.000831, 0.659425],
- [0.435734, 0.001127, 0.659797],
- [0.441732, 0.001540, 0.660069],
- [0.447714, 0.002080, 0.660240],
- [0.453677, 0.002755, 0.660310],
- [0.459623, 0.003574, 0.660277],
- [0.465550, 0.004545, 0.660139],
- [0.471457, 0.005678, 0.659897],
- [0.477344, 0.006980, 0.659549],
- [0.483210, 0.008460, 0.659095],
- [0.489055, 0.010127, 0.658534],
- [0.494877, 0.011990, 0.657865],
- [0.500678, 0.014055, 0.657088],
- [0.506454, 0.016333, 0.656202],
- [0.512206, 0.018833, 0.655209],
- [0.517933, 0.021563, 0.654109],
- [0.523633, 0.024532, 0.652901],
- [0.529306, 0.027747, 0.651586],
- [0.534952, 0.031217, 0.650165],
- [0.540570, 0.034950, 0.648640],
- [0.546157, 0.038954, 0.647010],
- [0.551715, 0.043136, 0.645277],
- [0.557243, 0.047331, 0.643443],
- [0.562738, 0.051545, 0.641509],
- [0.568201, 0.055778, 0.639477],
- [0.573632, 0.060028, 0.637349],
- [0.579029, 0.064296, 0.635126],
- [0.584391, 0.068579, 0.632812],
- [0.589719, 0.072878, 0.630408],
- [0.595011, 0.077190, 0.627917],
- [0.600266, 0.081516, 0.625342],
- [0.605485, 0.085854, 0.622686],
- [0.610667, 0.090204, 0.619951],
- [0.615812, 0.094564, 0.617140],
- [0.620919, 0.098934, 0.614257],
- [0.625987, 0.103312, 0.611305],
- [0.631017, 0.107699, 0.608287],
- [0.636008, 0.112092, 0.605205],
- [0.640959, 0.116492, 0.602065],
- [0.645872, 0.120898, 0.598867],
- [0.650746, 0.125309, 0.595617],
- [0.655580, 0.129725, 0.592317],
- [0.660374, 0.134144, 0.588971],
- [0.665129, 0.138566, 0.585582],
- [0.669845, 0.142992, 0.582154],
- [0.674522, 0.147419, 0.578688],
- [0.679160, 0.151848, 0.575189],
- [0.683758, 0.156278, 0.571660],
- [0.688318, 0.160709, 0.568103],
- [0.692840, 0.165141, 0.564522],
- [0.697324, 0.169573, 0.560919],
- [0.701769, 0.174005, 0.557296],
- [0.706178, 0.178437, 0.553657],
- [0.710549, 0.182868, 0.550004],
- [0.714883, 0.187299, 0.546338],
- [0.719181, 0.191729, 0.542663],
- [0.723444, 0.196158, 0.538981],
- [0.727670, 0.200586, 0.535293],
- [0.731862, 0.205013, 0.531601],
- [0.736019, 0.209439, 0.527908],
- [0.740143, 0.213864, 0.524216],
- [0.744232, 0.218288, 0.520524],
- [0.748289, 0.222711, 0.516834],
- [0.752312, 0.227133, 0.513149],
- [0.756304, 0.231555, 0.509468],
- [0.760264, 0.235976, 0.505794],
- [0.764193, 0.240396, 0.502126],
- [0.768090, 0.244817, 0.498465],
- [0.771958, 0.249237, 0.494813],
- [0.775796, 0.253658, 0.491171],
- [0.779604, 0.258078, 0.487539],
- [0.783383, 0.262500, 0.483918],
- [0.787133, 0.266922, 0.480307],
- [0.790855, 0.271345, 0.476706],
- [0.794549, 0.275770, 0.473117],
- [0.798216, 0.280197, 0.469538],
- [0.801855, 0.284626, 0.465971],
- [0.805467, 0.289057, 0.462415],
- [0.809052, 0.293491, 0.458870],
- [0.812612, 0.297928, 0.455338],
- [0.816144, 0.302368, 0.451816],
- [0.819651, 0.306812, 0.448306],
- [0.823132, 0.311261, 0.444806],
- [0.826588, 0.315714, 0.441316],
- [0.830018, 0.320172, 0.437836],
- [0.833422, 0.324635, 0.434366],
- [0.836801, 0.329105, 0.430905],
- [0.840155, 0.333580, 0.427455],
- [0.843484, 0.338062, 0.424013],
- [0.846788, 0.342551, 0.420579],
- [0.850066, 0.347048, 0.417153],
- [0.853319, 0.351553, 0.413734],
- [0.856547, 0.356066, 0.410322],
- [0.859750, 0.360588, 0.406917],
- [0.862927, 0.365119, 0.403519],
- [0.866078, 0.369660, 0.400126],
- [0.869203, 0.374212, 0.396738],
- [0.872303, 0.378774, 0.393355],
- [0.875376, 0.383347, 0.389976],
- [0.878423, 0.387932, 0.386600],
- [0.881443, 0.392529, 0.383229],
- [0.884436, 0.397139, 0.379860],
- [0.887402, 0.401762, 0.376494],
- [0.890340, 0.406398, 0.373130],
- [0.893250, 0.411048, 0.369768],
- [0.896131, 0.415712, 0.366407],
- [0.898984, 0.420392, 0.363047],
- [0.901807, 0.425087, 0.359688],
- [0.904601, 0.429797, 0.356329],
- [0.907365, 0.434524, 0.352970],
- [0.910098, 0.439268, 0.349610],
- [0.912800, 0.444029, 0.346251],
- [0.915471, 0.448807, 0.342890],
- [0.918109, 0.453603, 0.339529],
- [0.920714, 0.458417, 0.336166],
- [0.923287, 0.463251, 0.332801],
- [0.925825, 0.468103, 0.329435],
- [0.928329, 0.472975, 0.326067],
- [0.930798, 0.477867, 0.322697],
- [0.933232, 0.482780, 0.319325],
- [0.935630, 0.487712, 0.315952],
- [0.937990, 0.492667, 0.312575],
- [0.940313, 0.497642, 0.309197],
- [0.942598, 0.502639, 0.305816],
- [0.944844, 0.507658, 0.302433],
- [0.947051, 0.512699, 0.299049],
- [0.949217, 0.517763, 0.295662],
- [0.951344, 0.522850, 0.292275],
- [0.953428, 0.527960, 0.288883],
- [0.955470, 0.533093, 0.285490],
- [0.957469, 0.538250, 0.282096],
- [0.959424, 0.543431, 0.278701],
- [0.961336, 0.548636, 0.275305],
- [0.963203, 0.553865, 0.271909],
- [0.965024, 0.559118, 0.268513],
- [0.966798, 0.564396, 0.265118],
- [0.968526, 0.569700, 0.261721],
- [0.970205, 0.575028, 0.258325],
- [0.971835, 0.580382, 0.254931],
- [0.973416, 0.585761, 0.251540],
- [0.974947, 0.591165, 0.248151],
- [0.976428, 0.596595, 0.244767],
- [0.977856, 0.602051, 0.241387],
- [0.979233, 0.607532, 0.238013],
- [0.980556, 0.613039, 0.234646],
- [0.981826, 0.618572, 0.231287],
- [0.983041, 0.624131, 0.227937],
- [0.984199, 0.629718, 0.224595],
- [0.985301, 0.635330, 0.221265],
- [0.986345, 0.640969, 0.217948],
- [0.987332, 0.646633, 0.214648],
- [0.988260, 0.652325, 0.211364],
- [0.989128, 0.658043, 0.208100],
- [0.989935, 0.663787, 0.204859],
- [0.990681, 0.669558, 0.201642],
- [0.991365, 0.675355, 0.198453],
- [0.991985, 0.681179, 0.195295],
- [0.992541, 0.687030, 0.192170],
- [0.993032, 0.692907, 0.189084],
- [0.993456, 0.698810, 0.186041],
- [0.993814, 0.704741, 0.183043],
- [0.994103, 0.710698, 0.180097],
- [0.994324, 0.716681, 0.177208],
- [0.994474, 0.722691, 0.174381],
- [0.994553, 0.728728, 0.171622],
- [0.994561, 0.734791, 0.168938],
- [0.994495, 0.740880, 0.166335],
- [0.994355, 0.746995, 0.163821],
- [0.994141, 0.753137, 0.161404],
- [0.993851, 0.759304, 0.159092],
- [0.993482, 0.765499, 0.156891],
- [0.993033, 0.771720, 0.154808],
- [0.992505, 0.777967, 0.152855],
- [0.991897, 0.784239, 0.151042],
- [0.991209, 0.790537, 0.149377],
- [0.990439, 0.796859, 0.147870],
- [0.989587, 0.803205, 0.146529],
- [0.988648, 0.809579, 0.145357],
- [0.987621, 0.815978, 0.144363],
- [0.986509, 0.822401, 0.143557],
- [0.985314, 0.828846, 0.142945],
- [0.984031, 0.835315, 0.142528],
- [0.982653, 0.841812, 0.142303],
- [0.981190, 0.848329, 0.142279],
- [0.979644, 0.854866, 0.142453],
- [0.977995, 0.861432, 0.142808],
- [0.976265, 0.868016, 0.143351],
- [0.974443, 0.874622, 0.144061],
- [0.972530, 0.881250, 0.144923],
- [0.970533, 0.887896, 0.145919],
- [0.968443, 0.894564, 0.147014],
- [0.966271, 0.901249, 0.148180],
- [0.964021, 0.907950, 0.149370],
- [0.961681, 0.914672, 0.150520],
- [0.959276, 0.921407, 0.151566],
- [0.956808, 0.928152, 0.152409],
- [0.954287, 0.934908, 0.152921],
- [0.951726, 0.941671, 0.152925],
- [0.949151, 0.948435, 0.152178],
- [0.946602, 0.955190, 0.150328],
- [0.944152, 0.961916, 0.146861],
- [0.941896, 0.968590, 0.140956],
- [0.940015, 0.975158, 0.131326]]
+plasma = [
+ [0.050383, 0.029803, 0.527975],
+ [0.063536, 0.028426, 0.533124],
+ [0.075353, 0.027206, 0.538007],
+ [0.086222, 0.026125, 0.542658],
+ [0.096379, 0.025165, 0.547103],
+ [0.105980, 0.024309, 0.551368],
+ [0.115124, 0.023556, 0.555468],
+ [0.123903, 0.022878, 0.559423],
+ [0.132381, 0.022258, 0.563250],
+ [0.140603, 0.021687, 0.566959],
+ [0.148607, 0.021154, 0.570562],
+ [0.156421, 0.020651, 0.574065],
+ [0.164070, 0.020171, 0.577478],
+ [0.171574, 0.019706, 0.580806],
+ [0.178950, 0.019252, 0.584054],
+ [0.186213, 0.018803, 0.587228],
+ [0.193374, 0.018354, 0.590330],
+ [0.200445, 0.017902, 0.593364],
+ [0.207435, 0.017442, 0.596333],
+ [0.214350, 0.016973, 0.599239],
+ [0.221197, 0.016497, 0.602083],
+ [0.227983, 0.016007, 0.604867],
+ [0.234715, 0.015502, 0.607592],
+ [0.241396, 0.014979, 0.610259],
+ [0.248032, 0.014439, 0.612868],
+ [0.254627, 0.013882, 0.615419],
+ [0.261183, 0.013308, 0.617911],
+ [0.267703, 0.012716, 0.620346],
+ [0.274191, 0.012109, 0.622722],
+ [0.280648, 0.011488, 0.625038],
+ [0.287076, 0.010855, 0.627295],
+ [0.293478, 0.010213, 0.629490],
+ [0.299855, 0.009561, 0.631624],
+ [0.306210, 0.008902, 0.633694],
+ [0.312543, 0.008239, 0.635700],
+ [0.318856, 0.007576, 0.637640],
+ [0.325150, 0.006915, 0.639512],
+ [0.331426, 0.006261, 0.641316],
+ [0.337683, 0.005618, 0.643049],
+ [0.343925, 0.004991, 0.644710],
+ [0.350150, 0.004382, 0.646298],
+ [0.356359, 0.003798, 0.647810],
+ [0.362553, 0.003243, 0.649245],
+ [0.368733, 0.002724, 0.650601],
+ [0.374897, 0.002245, 0.651876],
+ [0.381047, 0.001814, 0.653068],
+ [0.387183, 0.001434, 0.654177],
+ [0.393304, 0.001114, 0.655199],
+ [0.399411, 0.000859, 0.656133],
+ [0.405503, 0.000678, 0.656977],
+ [0.411580, 0.000577, 0.657730],
+ [0.417642, 0.000564, 0.658390],
+ [0.423689, 0.000646, 0.658956],
+ [0.429719, 0.000831, 0.659425],
+ [0.435734, 0.001127, 0.659797],
+ [0.441732, 0.001540, 0.660069],
+ [0.447714, 0.002080, 0.660240],
+ [0.453677, 0.002755, 0.660310],
+ [0.459623, 0.003574, 0.660277],
+ [0.465550, 0.004545, 0.660139],
+ [0.471457, 0.005678, 0.659897],
+ [0.477344, 0.006980, 0.659549],
+ [0.483210, 0.008460, 0.659095],
+ [0.489055, 0.010127, 0.658534],
+ [0.494877, 0.011990, 0.657865],
+ [0.500678, 0.014055, 0.657088],
+ [0.506454, 0.016333, 0.656202],
+ [0.512206, 0.018833, 0.655209],
+ [0.517933, 0.021563, 0.654109],
+ [0.523633, 0.024532, 0.652901],
+ [0.529306, 0.027747, 0.651586],
+ [0.534952, 0.031217, 0.650165],
+ [0.540570, 0.034950, 0.648640],
+ [0.546157, 0.038954, 0.647010],
+ [0.551715, 0.043136, 0.645277],
+ [0.557243, 0.047331, 0.643443],
+ [0.562738, 0.051545, 0.641509],
+ [0.568201, 0.055778, 0.639477],
+ [0.573632, 0.060028, 0.637349],
+ [0.579029, 0.064296, 0.635126],
+ [0.584391, 0.068579, 0.632812],
+ [0.589719, 0.072878, 0.630408],
+ [0.595011, 0.077190, 0.627917],
+ [0.600266, 0.081516, 0.625342],
+ [0.605485, 0.085854, 0.622686],
+ [0.610667, 0.090204, 0.619951],
+ [0.615812, 0.094564, 0.617140],
+ [0.620919, 0.098934, 0.614257],
+ [0.625987, 0.103312, 0.611305],
+ [0.631017, 0.107699, 0.608287],
+ [0.636008, 0.112092, 0.605205],
+ [0.640959, 0.116492, 0.602065],
+ [0.645872, 0.120898, 0.598867],
+ [0.650746, 0.125309, 0.595617],
+ [0.655580, 0.129725, 0.592317],
+ [0.660374, 0.134144, 0.588971],
+ [0.665129, 0.138566, 0.585582],
+ [0.669845, 0.142992, 0.582154],
+ [0.674522, 0.147419, 0.578688],
+ [0.679160, 0.151848, 0.575189],
+ [0.683758, 0.156278, 0.571660],
+ [0.688318, 0.160709, 0.568103],
+ [0.692840, 0.165141, 0.564522],
+ [0.697324, 0.169573, 0.560919],
+ [0.701769, 0.174005, 0.557296],
+ [0.706178, 0.178437, 0.553657],
+ [0.710549, 0.182868, 0.550004],
+ [0.714883, 0.187299, 0.546338],
+ [0.719181, 0.191729, 0.542663],
+ [0.723444, 0.196158, 0.538981],
+ [0.727670, 0.200586, 0.535293],
+ [0.731862, 0.205013, 0.531601],
+ [0.736019, 0.209439, 0.527908],
+ [0.740143, 0.213864, 0.524216],
+ [0.744232, 0.218288, 0.520524],
+ [0.748289, 0.222711, 0.516834],
+ [0.752312, 0.227133, 0.513149],
+ [0.756304, 0.231555, 0.509468],
+ [0.760264, 0.235976, 0.505794],
+ [0.764193, 0.240396, 0.502126],
+ [0.768090, 0.244817, 0.498465],
+ [0.771958, 0.249237, 0.494813],
+ [0.775796, 0.253658, 0.491171],
+ [0.779604, 0.258078, 0.487539],
+ [0.783383, 0.262500, 0.483918],
+ [0.787133, 0.266922, 0.480307],
+ [0.790855, 0.271345, 0.476706],
+ [0.794549, 0.275770, 0.473117],
+ [0.798216, 0.280197, 0.469538],
+ [0.801855, 0.284626, 0.465971],
+ [0.805467, 0.289057, 0.462415],
+ [0.809052, 0.293491, 0.458870],
+ [0.812612, 0.297928, 0.455338],
+ [0.816144, 0.302368, 0.451816],
+ [0.819651, 0.306812, 0.448306],
+ [0.823132, 0.311261, 0.444806],
+ [0.826588, 0.315714, 0.441316],
+ [0.830018, 0.320172, 0.437836],
+ [0.833422, 0.324635, 0.434366],
+ [0.836801, 0.329105, 0.430905],
+ [0.840155, 0.333580, 0.427455],
+ [0.843484, 0.338062, 0.424013],
+ [0.846788, 0.342551, 0.420579],
+ [0.850066, 0.347048, 0.417153],
+ [0.853319, 0.351553, 0.413734],
+ [0.856547, 0.356066, 0.410322],
+ [0.859750, 0.360588, 0.406917],
+ [0.862927, 0.365119, 0.403519],
+ [0.866078, 0.369660, 0.400126],
+ [0.869203, 0.374212, 0.396738],
+ [0.872303, 0.378774, 0.393355],
+ [0.875376, 0.383347, 0.389976],
+ [0.878423, 0.387932, 0.386600],
+ [0.881443, 0.392529, 0.383229],
+ [0.884436, 0.397139, 0.379860],
+ [0.887402, 0.401762, 0.376494],
+ [0.890340, 0.406398, 0.373130],
+ [0.893250, 0.411048, 0.369768],
+ [0.896131, 0.415712, 0.366407],
+ [0.898984, 0.420392, 0.363047],
+ [0.901807, 0.425087, 0.359688],
+ [0.904601, 0.429797, 0.356329],
+ [0.907365, 0.434524, 0.352970],
+ [0.910098, 0.439268, 0.349610],
+ [0.912800, 0.444029, 0.346251],
+ [0.915471, 0.448807, 0.342890],
+ [0.918109, 0.453603, 0.339529],
+ [0.920714, 0.458417, 0.336166],
+ [0.923287, 0.463251, 0.332801],
+ [0.925825, 0.468103, 0.329435],
+ [0.928329, 0.472975, 0.326067],
+ [0.930798, 0.477867, 0.322697],
+ [0.933232, 0.482780, 0.319325],
+ [0.935630, 0.487712, 0.315952],
+ [0.937990, 0.492667, 0.312575],
+ [0.940313, 0.497642, 0.309197],
+ [0.942598, 0.502639, 0.305816],
+ [0.944844, 0.507658, 0.302433],
+ [0.947051, 0.512699, 0.299049],
+ [0.949217, 0.517763, 0.295662],
+ [0.951344, 0.522850, 0.292275],
+ [0.953428, 0.527960, 0.288883],
+ [0.955470, 0.533093, 0.285490],
+ [0.957469, 0.538250, 0.282096],
+ [0.959424, 0.543431, 0.278701],
+ [0.961336, 0.548636, 0.275305],
+ [0.963203, 0.553865, 0.271909],
+ [0.965024, 0.559118, 0.268513],
+ [0.966798, 0.564396, 0.265118],
+ [0.968526, 0.569700, 0.261721],
+ [0.970205, 0.575028, 0.258325],
+ [0.971835, 0.580382, 0.254931],
+ [0.973416, 0.585761, 0.251540],
+ [0.974947, 0.591165, 0.248151],
+ [0.976428, 0.596595, 0.244767],
+ [0.977856, 0.602051, 0.241387],
+ [0.979233, 0.607532, 0.238013],
+ [0.980556, 0.613039, 0.234646],
+ [0.981826, 0.618572, 0.231287],
+ [0.983041, 0.624131, 0.227937],
+ [0.984199, 0.629718, 0.224595],
+ [0.985301, 0.635330, 0.221265],
+ [0.986345, 0.640969, 0.217948],
+ [0.987332, 0.646633, 0.214648],
+ [0.988260, 0.652325, 0.211364],
+ [0.989128, 0.658043, 0.208100],
+ [0.989935, 0.663787, 0.204859],
+ [0.990681, 0.669558, 0.201642],
+ [0.991365, 0.675355, 0.198453],
+ [0.991985, 0.681179, 0.195295],
+ [0.992541, 0.687030, 0.192170],
+ [0.993032, 0.692907, 0.189084],
+ [0.993456, 0.698810, 0.186041],
+ [0.993814, 0.704741, 0.183043],
+ [0.994103, 0.710698, 0.180097],
+ [0.994324, 0.716681, 0.177208],
+ [0.994474, 0.722691, 0.174381],
+ [0.994553, 0.728728, 0.171622],
+ [0.994561, 0.734791, 0.168938],
+ [0.994495, 0.740880, 0.166335],
+ [0.994355, 0.746995, 0.163821],
+ [0.994141, 0.753137, 0.161404],
+ [0.993851, 0.759304, 0.159092],
+ [0.993482, 0.765499, 0.156891],
+ [0.993033, 0.771720, 0.154808],
+ [0.992505, 0.777967, 0.152855],
+ [0.991897, 0.784239, 0.151042],
+ [0.991209, 0.790537, 0.149377],
+ [0.990439, 0.796859, 0.147870],
+ [0.989587, 0.803205, 0.146529],
+ [0.988648, 0.809579, 0.145357],
+ [0.987621, 0.815978, 0.144363],
+ [0.986509, 0.822401, 0.143557],
+ [0.985314, 0.828846, 0.142945],
+ [0.984031, 0.835315, 0.142528],
+ [0.982653, 0.841812, 0.142303],
+ [0.981190, 0.848329, 0.142279],
+ [0.979644, 0.854866, 0.142453],
+ [0.977995, 0.861432, 0.142808],
+ [0.976265, 0.868016, 0.143351],
+ [0.974443, 0.874622, 0.144061],
+ [0.972530, 0.881250, 0.144923],
+ [0.970533, 0.887896, 0.145919],
+ [0.968443, 0.894564, 0.147014],
+ [0.966271, 0.901249, 0.148180],
+ [0.964021, 0.907950, 0.149370],
+ [0.961681, 0.914672, 0.150520],
+ [0.959276, 0.921407, 0.151566],
+ [0.956808, 0.928152, 0.152409],
+ [0.954287, 0.934908, 0.152921],
+ [0.951726, 0.941671, 0.152925],
+ [0.949151, 0.948435, 0.152178],
+ [0.946602, 0.955190, 0.150328],
+ [0.944152, 0.961916, 0.146861],
+ [0.941896, 0.968590, 0.140956],
+ [0.940015, 0.975158, 0.131326],
+]
-viridis = [[0.267004, 0.004874, 0.329415],
- [0.268510, 0.009605, 0.335427],
- [0.269944, 0.014625, 0.341379],
- [0.271305, 0.019942, 0.347269],
- [0.272594, 0.025563, 0.353093],
- [0.273809, 0.031497, 0.358853],
- [0.274952, 0.037752, 0.364543],
- [0.276022, 0.044167, 0.370164],
- [0.277018, 0.050344, 0.375715],
- [0.277941, 0.056324, 0.381191],
- [0.278791, 0.062145, 0.386592],
- [0.279566, 0.067836, 0.391917],
- [0.280267, 0.073417, 0.397163],
- [0.280894, 0.078907, 0.402329],
- [0.281446, 0.084320, 0.407414],
- [0.281924, 0.089666, 0.412415],
- [0.282327, 0.094955, 0.417331],
- [0.282656, 0.100196, 0.422160],
- [0.282910, 0.105393, 0.426902],
- [0.283091, 0.110553, 0.431554],
- [0.283197, 0.115680, 0.436115],
- [0.283229, 0.120777, 0.440584],
- [0.283187, 0.125848, 0.444960],
- [0.283072, 0.130895, 0.449241],
- [0.282884, 0.135920, 0.453427],
- [0.282623, 0.140926, 0.457517],
- [0.282290, 0.145912, 0.461510],
- [0.281887, 0.150881, 0.465405],
- [0.281412, 0.155834, 0.469201],
- [0.280868, 0.160771, 0.472899],
- [0.280255, 0.165693, 0.476498],
- [0.279574, 0.170599, 0.479997],
- [0.278826, 0.175490, 0.483397],
- [0.278012, 0.180367, 0.486697],
- [0.277134, 0.185228, 0.489898],
- [0.276194, 0.190074, 0.493001],
- [0.275191, 0.194905, 0.496005],
- [0.274128, 0.199721, 0.498911],
- [0.273006, 0.204520, 0.501721],
- [0.271828, 0.209303, 0.504434],
- [0.270595, 0.214069, 0.507052],
- [0.269308, 0.218818, 0.509577],
- [0.267968, 0.223549, 0.512008],
- [0.266580, 0.228262, 0.514349],
- [0.265145, 0.232956, 0.516599],
- [0.263663, 0.237631, 0.518762],
- [0.262138, 0.242286, 0.520837],
- [0.260571, 0.246922, 0.522828],
- [0.258965, 0.251537, 0.524736],
- [0.257322, 0.256130, 0.526563],
- [0.255645, 0.260703, 0.528312],
- [0.253935, 0.265254, 0.529983],
- [0.252194, 0.269783, 0.531579],
- [0.250425, 0.274290, 0.533103],
- [0.248629, 0.278775, 0.534556],
- [0.246811, 0.283237, 0.535941],
- [0.244972, 0.287675, 0.537260],
- [0.243113, 0.292092, 0.538516],
- [0.241237, 0.296485, 0.539709],
- [0.239346, 0.300855, 0.540844],
- [0.237441, 0.305202, 0.541921],
- [0.235526, 0.309527, 0.542944],
- [0.233603, 0.313828, 0.543914],
- [0.231674, 0.318106, 0.544834],
- [0.229739, 0.322361, 0.545706],
- [0.227802, 0.326594, 0.546532],
- [0.225863, 0.330805, 0.547314],
- [0.223925, 0.334994, 0.548053],
- [0.221989, 0.339161, 0.548752],
- [0.220057, 0.343307, 0.549413],
- [0.218130, 0.347432, 0.550038],
- [0.216210, 0.351535, 0.550627],
- [0.214298, 0.355619, 0.551184],
- [0.212395, 0.359683, 0.551710],
- [0.210503, 0.363727, 0.552206],
- [0.208623, 0.367752, 0.552675],
- [0.206756, 0.371758, 0.553117],
- [0.204903, 0.375746, 0.553533],
- [0.203063, 0.379716, 0.553925],
- [0.201239, 0.383670, 0.554294],
- [0.199430, 0.387607, 0.554642],
- [0.197636, 0.391528, 0.554969],
- [0.195860, 0.395433, 0.555276],
- [0.194100, 0.399323, 0.555565],
- [0.192357, 0.403199, 0.555836],
- [0.190631, 0.407061, 0.556089],
- [0.188923, 0.410910, 0.556326],
- [0.187231, 0.414746, 0.556547],
- [0.185556, 0.418570, 0.556753],
- [0.183898, 0.422383, 0.556944],
- [0.182256, 0.426184, 0.557120],
- [0.180629, 0.429975, 0.557282],
- [0.179019, 0.433756, 0.557430],
- [0.177423, 0.437527, 0.557565],
- [0.175841, 0.441290, 0.557685],
- [0.174274, 0.445044, 0.557792],
- [0.172719, 0.448791, 0.557885],
- [0.171176, 0.452530, 0.557965],
- [0.169646, 0.456262, 0.558030],
- [0.168126, 0.459988, 0.558082],
- [0.166617, 0.463708, 0.558119],
- [0.165117, 0.467423, 0.558141],
- [0.163625, 0.471133, 0.558148],
- [0.162142, 0.474838, 0.558140],
- [0.160665, 0.478540, 0.558115],
- [0.159194, 0.482237, 0.558073],
- [0.157729, 0.485932, 0.558013],
- [0.156270, 0.489624, 0.557936],
- [0.154815, 0.493313, 0.557840],
- [0.153364, 0.497000, 0.557724],
- [0.151918, 0.500685, 0.557587],
- [0.150476, 0.504369, 0.557430],
- [0.149039, 0.508051, 0.557250],
- [0.147607, 0.511733, 0.557049],
- [0.146180, 0.515413, 0.556823],
- [0.144759, 0.519093, 0.556572],
- [0.143343, 0.522773, 0.556295],
- [0.141935, 0.526453, 0.555991],
- [0.140536, 0.530132, 0.555659],
- [0.139147, 0.533812, 0.555298],
- [0.137770, 0.537492, 0.554906],
- [0.136408, 0.541173, 0.554483],
- [0.135066, 0.544853, 0.554029],
- [0.133743, 0.548535, 0.553541],
- [0.132444, 0.552216, 0.553018],
- [0.131172, 0.555899, 0.552459],
- [0.129933, 0.559582, 0.551864],
- [0.128729, 0.563265, 0.551229],
- [0.127568, 0.566949, 0.550556],
- [0.126453, 0.570633, 0.549841],
- [0.125394, 0.574318, 0.549086],
- [0.124395, 0.578002, 0.548287],
- [0.123463, 0.581687, 0.547445],
- [0.122606, 0.585371, 0.546557],
- [0.121831, 0.589055, 0.545623],
- [0.121148, 0.592739, 0.544641],
- [0.120565, 0.596422, 0.543611],
- [0.120092, 0.600104, 0.542530],
- [0.119738, 0.603785, 0.541400],
- [0.119512, 0.607464, 0.540218],
- [0.119423, 0.611141, 0.538982],
- [0.119483, 0.614817, 0.537692],
- [0.119699, 0.618490, 0.536347],
- [0.120081, 0.622161, 0.534946],
- [0.120638, 0.625828, 0.533488],
- [0.121380, 0.629492, 0.531973],
- [0.122312, 0.633153, 0.530398],
- [0.123444, 0.636809, 0.528763],
- [0.124780, 0.640461, 0.527068],
- [0.126326, 0.644107, 0.525311],
- [0.128087, 0.647749, 0.523491],
- [0.130067, 0.651384, 0.521608],
- [0.132268, 0.655014, 0.519661],
- [0.134692, 0.658636, 0.517649],
- [0.137339, 0.662252, 0.515571],
- [0.140210, 0.665859, 0.513427],
- [0.143303, 0.669459, 0.511215],
- [0.146616, 0.673050, 0.508936],
- [0.150148, 0.676631, 0.506589],
- [0.153894, 0.680203, 0.504172],
- [0.157851, 0.683765, 0.501686],
- [0.162016, 0.687316, 0.499129],
- [0.166383, 0.690856, 0.496502],
- [0.170948, 0.694384, 0.493803],
- [0.175707, 0.697900, 0.491033],
- [0.180653, 0.701402, 0.488189],
- [0.185783, 0.704891, 0.485273],
- [0.191090, 0.708366, 0.482284],
- [0.196571, 0.711827, 0.479221],
- [0.202219, 0.715272, 0.476084],
- [0.208030, 0.718701, 0.472873],
- [0.214000, 0.722114, 0.469588],
- [0.220124, 0.725509, 0.466226],
- [0.226397, 0.728888, 0.462789],
- [0.232815, 0.732247, 0.459277],
- [0.239374, 0.735588, 0.455688],
- [0.246070, 0.738910, 0.452024],
- [0.252899, 0.742211, 0.448284],
- [0.259857, 0.745492, 0.444467],
- [0.266941, 0.748751, 0.440573],
- [0.274149, 0.751988, 0.436601],
- [0.281477, 0.755203, 0.432552],
- [0.288921, 0.758394, 0.428426],
- [0.296479, 0.761561, 0.424223],
- [0.304148, 0.764704, 0.419943],
- [0.311925, 0.767822, 0.415586],
- [0.319809, 0.770914, 0.411152],
- [0.327796, 0.773980, 0.406640],
- [0.335885, 0.777018, 0.402049],
- [0.344074, 0.780029, 0.397381],
- [0.352360, 0.783011, 0.392636],
- [0.360741, 0.785964, 0.387814],
- [0.369214, 0.788888, 0.382914],
- [0.377779, 0.791781, 0.377939],
- [0.386433, 0.794644, 0.372886],
- [0.395174, 0.797475, 0.367757],
- [0.404001, 0.800275, 0.362552],
- [0.412913, 0.803041, 0.357269],
- [0.421908, 0.805774, 0.351910],
- [0.430983, 0.808473, 0.346476],
- [0.440137, 0.811138, 0.340967],
- [0.449368, 0.813768, 0.335384],
- [0.458674, 0.816363, 0.329727],
- [0.468053, 0.818921, 0.323998],
- [0.477504, 0.821444, 0.318195],
- [0.487026, 0.823929, 0.312321],
- [0.496615, 0.826376, 0.306377],
- [0.506271, 0.828786, 0.300362],
- [0.515992, 0.831158, 0.294279],
- [0.525776, 0.833491, 0.288127],
- [0.535621, 0.835785, 0.281908],
- [0.545524, 0.838039, 0.275626],
- [0.555484, 0.840254, 0.269281],
- [0.565498, 0.842430, 0.262877],
- [0.575563, 0.844566, 0.256415],
- [0.585678, 0.846661, 0.249897],
- [0.595839, 0.848717, 0.243329],
- [0.606045, 0.850733, 0.236712],
- [0.616293, 0.852709, 0.230052],
- [0.626579, 0.854645, 0.223353],
- [0.636902, 0.856542, 0.216620],
- [0.647257, 0.858400, 0.209861],
- [0.657642, 0.860219, 0.203082],
- [0.668054, 0.861999, 0.196293],
- [0.678489, 0.863742, 0.189503],
- [0.688944, 0.865448, 0.182725],
- [0.699415, 0.867117, 0.175971],
- [0.709898, 0.868751, 0.169257],
- [0.720391, 0.870350, 0.162603],
- [0.730889, 0.871916, 0.156029],
- [0.741388, 0.873449, 0.149561],
- [0.751884, 0.874951, 0.143228],
- [0.762373, 0.876424, 0.137064],
- [0.772852, 0.877868, 0.131109],
- [0.783315, 0.879285, 0.125405],
- [0.793760, 0.880678, 0.120005],
- [0.804182, 0.882046, 0.114965],
- [0.814576, 0.883393, 0.110347],
- [0.824940, 0.884720, 0.106217],
- [0.835270, 0.886029, 0.102646],
- [0.845561, 0.887322, 0.099702],
- [0.855810, 0.888601, 0.097452],
- [0.866013, 0.889868, 0.095953],
- [0.876168, 0.891125, 0.095250],
- [0.886271, 0.892374, 0.095374],
- [0.896320, 0.893616, 0.096335],
- [0.906311, 0.894855, 0.098125],
- [0.916242, 0.896091, 0.100717],
- [0.926106, 0.897330, 0.104071],
- [0.935904, 0.898570, 0.108131],
- [0.945636, 0.899815, 0.112838],
- [0.955300, 0.901065, 0.118128],
- [0.964894, 0.902323, 0.123941],
- [0.974417, 0.903590, 0.130215],
- [0.983868, 0.904867, 0.136897],
- [0.993248, 0.906157, 0.143936]]
+viridis = [
+ [0.267004, 0.004874, 0.329415],
+ [0.268510, 0.009605, 0.335427],
+ [0.269944, 0.014625, 0.341379],
+ [0.271305, 0.019942, 0.347269],
+ [0.272594, 0.025563, 0.353093],
+ [0.273809, 0.031497, 0.358853],
+ [0.274952, 0.037752, 0.364543],
+ [0.276022, 0.044167, 0.370164],
+ [0.277018, 0.050344, 0.375715],
+ [0.277941, 0.056324, 0.381191],
+ [0.278791, 0.062145, 0.386592],
+ [0.279566, 0.067836, 0.391917],
+ [0.280267, 0.073417, 0.397163],
+ [0.280894, 0.078907, 0.402329],
+ [0.281446, 0.084320, 0.407414],
+ [0.281924, 0.089666, 0.412415],
+ [0.282327, 0.094955, 0.417331],
+ [0.282656, 0.100196, 0.422160],
+ [0.282910, 0.105393, 0.426902],
+ [0.283091, 0.110553, 0.431554],
+ [0.283197, 0.115680, 0.436115],
+ [0.283229, 0.120777, 0.440584],
+ [0.283187, 0.125848, 0.444960],
+ [0.283072, 0.130895, 0.449241],
+ [0.282884, 0.135920, 0.453427],
+ [0.282623, 0.140926, 0.457517],
+ [0.282290, 0.145912, 0.461510],
+ [0.281887, 0.150881, 0.465405],
+ [0.281412, 0.155834, 0.469201],
+ [0.280868, 0.160771, 0.472899],
+ [0.280255, 0.165693, 0.476498],
+ [0.279574, 0.170599, 0.479997],
+ [0.278826, 0.175490, 0.483397],
+ [0.278012, 0.180367, 0.486697],
+ [0.277134, 0.185228, 0.489898],
+ [0.276194, 0.190074, 0.493001],
+ [0.275191, 0.194905, 0.496005],
+ [0.274128, 0.199721, 0.498911],
+ [0.273006, 0.204520, 0.501721],
+ [0.271828, 0.209303, 0.504434],
+ [0.270595, 0.214069, 0.507052],
+ [0.269308, 0.218818, 0.509577],
+ [0.267968, 0.223549, 0.512008],
+ [0.266580, 0.228262, 0.514349],
+ [0.265145, 0.232956, 0.516599],
+ [0.263663, 0.237631, 0.518762],
+ [0.262138, 0.242286, 0.520837],
+ [0.260571, 0.246922, 0.522828],
+ [0.258965, 0.251537, 0.524736],
+ [0.257322, 0.256130, 0.526563],
+ [0.255645, 0.260703, 0.528312],
+ [0.253935, 0.265254, 0.529983],
+ [0.252194, 0.269783, 0.531579],
+ [0.250425, 0.274290, 0.533103],
+ [0.248629, 0.278775, 0.534556],
+ [0.246811, 0.283237, 0.535941],
+ [0.244972, 0.287675, 0.537260],
+ [0.243113, 0.292092, 0.538516],
+ [0.241237, 0.296485, 0.539709],
+ [0.239346, 0.300855, 0.540844],
+ [0.237441, 0.305202, 0.541921],
+ [0.235526, 0.309527, 0.542944],
+ [0.233603, 0.313828, 0.543914],
+ [0.231674, 0.318106, 0.544834],
+ [0.229739, 0.322361, 0.545706],
+ [0.227802, 0.326594, 0.546532],
+ [0.225863, 0.330805, 0.547314],
+ [0.223925, 0.334994, 0.548053],
+ [0.221989, 0.339161, 0.548752],
+ [0.220057, 0.343307, 0.549413],
+ [0.218130, 0.347432, 0.550038],
+ [0.216210, 0.351535, 0.550627],
+ [0.214298, 0.355619, 0.551184],
+ [0.212395, 0.359683, 0.551710],
+ [0.210503, 0.363727, 0.552206],
+ [0.208623, 0.367752, 0.552675],
+ [0.206756, 0.371758, 0.553117],
+ [0.204903, 0.375746, 0.553533],
+ [0.203063, 0.379716, 0.553925],
+ [0.201239, 0.383670, 0.554294],
+ [0.199430, 0.387607, 0.554642],
+ [0.197636, 0.391528, 0.554969],
+ [0.195860, 0.395433, 0.555276],
+ [0.194100, 0.399323, 0.555565],
+ [0.192357, 0.403199, 0.555836],
+ [0.190631, 0.407061, 0.556089],
+ [0.188923, 0.410910, 0.556326],
+ [0.187231, 0.414746, 0.556547],
+ [0.185556, 0.418570, 0.556753],
+ [0.183898, 0.422383, 0.556944],
+ [0.182256, 0.426184, 0.557120],
+ [0.180629, 0.429975, 0.557282],
+ [0.179019, 0.433756, 0.557430],
+ [0.177423, 0.437527, 0.557565],
+ [0.175841, 0.441290, 0.557685],
+ [0.174274, 0.445044, 0.557792],
+ [0.172719, 0.448791, 0.557885],
+ [0.171176, 0.452530, 0.557965],
+ [0.169646, 0.456262, 0.558030],
+ [0.168126, 0.459988, 0.558082],
+ [0.166617, 0.463708, 0.558119],
+ [0.165117, 0.467423, 0.558141],
+ [0.163625, 0.471133, 0.558148],
+ [0.162142, 0.474838, 0.558140],
+ [0.160665, 0.478540, 0.558115],
+ [0.159194, 0.482237, 0.558073],
+ [0.157729, 0.485932, 0.558013],
+ [0.156270, 0.489624, 0.557936],
+ [0.154815, 0.493313, 0.557840],
+ [0.153364, 0.497000, 0.557724],
+ [0.151918, 0.500685, 0.557587],
+ [0.150476, 0.504369, 0.557430],
+ [0.149039, 0.508051, 0.557250],
+ [0.147607, 0.511733, 0.557049],
+ [0.146180, 0.515413, 0.556823],
+ [0.144759, 0.519093, 0.556572],
+ [0.143343, 0.522773, 0.556295],
+ [0.141935, 0.526453, 0.555991],
+ [0.140536, 0.530132, 0.555659],
+ [0.139147, 0.533812, 0.555298],
+ [0.137770, 0.537492, 0.554906],
+ [0.136408, 0.541173, 0.554483],
+ [0.135066, 0.544853, 0.554029],
+ [0.133743, 0.548535, 0.553541],
+ [0.132444, 0.552216, 0.553018],
+ [0.131172, 0.555899, 0.552459],
+ [0.129933, 0.559582, 0.551864],
+ [0.128729, 0.563265, 0.551229],
+ [0.127568, 0.566949, 0.550556],
+ [0.126453, 0.570633, 0.549841],
+ [0.125394, 0.574318, 0.549086],
+ [0.124395, 0.578002, 0.548287],
+ [0.123463, 0.581687, 0.547445],
+ [0.122606, 0.585371, 0.546557],
+ [0.121831, 0.589055, 0.545623],
+ [0.121148, 0.592739, 0.544641],
+ [0.120565, 0.596422, 0.543611],
+ [0.120092, 0.600104, 0.542530],
+ [0.119738, 0.603785, 0.541400],
+ [0.119512, 0.607464, 0.540218],
+ [0.119423, 0.611141, 0.538982],
+ [0.119483, 0.614817, 0.537692],
+ [0.119699, 0.618490, 0.536347],
+ [0.120081, 0.622161, 0.534946],
+ [0.120638, 0.625828, 0.533488],
+ [0.121380, 0.629492, 0.531973],
+ [0.122312, 0.633153, 0.530398],
+ [0.123444, 0.636809, 0.528763],
+ [0.124780, 0.640461, 0.527068],
+ [0.126326, 0.644107, 0.525311],
+ [0.128087, 0.647749, 0.523491],
+ [0.130067, 0.651384, 0.521608],
+ [0.132268, 0.655014, 0.519661],
+ [0.134692, 0.658636, 0.517649],
+ [0.137339, 0.662252, 0.515571],
+ [0.140210, 0.665859, 0.513427],
+ [0.143303, 0.669459, 0.511215],
+ [0.146616, 0.673050, 0.508936],
+ [0.150148, 0.676631, 0.506589],
+ [0.153894, 0.680203, 0.504172],
+ [0.157851, 0.683765, 0.501686],
+ [0.162016, 0.687316, 0.499129],
+ [0.166383, 0.690856, 0.496502],
+ [0.170948, 0.694384, 0.493803],
+ [0.175707, 0.697900, 0.491033],
+ [0.180653, 0.701402, 0.488189],
+ [0.185783, 0.704891, 0.485273],
+ [0.191090, 0.708366, 0.482284],
+ [0.196571, 0.711827, 0.479221],
+ [0.202219, 0.715272, 0.476084],
+ [0.208030, 0.718701, 0.472873],
+ [0.214000, 0.722114, 0.469588],
+ [0.220124, 0.725509, 0.466226],
+ [0.226397, 0.728888, 0.462789],
+ [0.232815, 0.732247, 0.459277],
+ [0.239374, 0.735588, 0.455688],
+ [0.246070, 0.738910, 0.452024],
+ [0.252899, 0.742211, 0.448284],
+ [0.259857, 0.745492, 0.444467],
+ [0.266941, 0.748751, 0.440573],
+ [0.274149, 0.751988, 0.436601],
+ [0.281477, 0.755203, 0.432552],
+ [0.288921, 0.758394, 0.428426],
+ [0.296479, 0.761561, 0.424223],
+ [0.304148, 0.764704, 0.419943],
+ [0.311925, 0.767822, 0.415586],
+ [0.319809, 0.770914, 0.411152],
+ [0.327796, 0.773980, 0.406640],
+ [0.335885, 0.777018, 0.402049],
+ [0.344074, 0.780029, 0.397381],
+ [0.352360, 0.783011, 0.392636],
+ [0.360741, 0.785964, 0.387814],
+ [0.369214, 0.788888, 0.382914],
+ [0.377779, 0.791781, 0.377939],
+ [0.386433, 0.794644, 0.372886],
+ [0.395174, 0.797475, 0.367757],
+ [0.404001, 0.800275, 0.362552],
+ [0.412913, 0.803041, 0.357269],
+ [0.421908, 0.805774, 0.351910],
+ [0.430983, 0.808473, 0.346476],
+ [0.440137, 0.811138, 0.340967],
+ [0.449368, 0.813768, 0.335384],
+ [0.458674, 0.816363, 0.329727],
+ [0.468053, 0.818921, 0.323998],
+ [0.477504, 0.821444, 0.318195],
+ [0.487026, 0.823929, 0.312321],
+ [0.496615, 0.826376, 0.306377],
+ [0.506271, 0.828786, 0.300362],
+ [0.515992, 0.831158, 0.294279],
+ [0.525776, 0.833491, 0.288127],
+ [0.535621, 0.835785, 0.281908],
+ [0.545524, 0.838039, 0.275626],
+ [0.555484, 0.840254, 0.269281],
+ [0.565498, 0.842430, 0.262877],
+ [0.575563, 0.844566, 0.256415],
+ [0.585678, 0.846661, 0.249897],
+ [0.595839, 0.848717, 0.243329],
+ [0.606045, 0.850733, 0.236712],
+ [0.616293, 0.852709, 0.230052],
+ [0.626579, 0.854645, 0.223353],
+ [0.636902, 0.856542, 0.216620],
+ [0.647257, 0.858400, 0.209861],
+ [0.657642, 0.860219, 0.203082],
+ [0.668054, 0.861999, 0.196293],
+ [0.678489, 0.863742, 0.189503],
+ [0.688944, 0.865448, 0.182725],
+ [0.699415, 0.867117, 0.175971],
+ [0.709898, 0.868751, 0.169257],
+ [0.720391, 0.870350, 0.162603],
+ [0.730889, 0.871916, 0.156029],
+ [0.741388, 0.873449, 0.149561],
+ [0.751884, 0.874951, 0.143228],
+ [0.762373, 0.876424, 0.137064],
+ [0.772852, 0.877868, 0.131109],
+ [0.783315, 0.879285, 0.125405],
+ [0.793760, 0.880678, 0.120005],
+ [0.804182, 0.882046, 0.114965],
+ [0.814576, 0.883393, 0.110347],
+ [0.824940, 0.884720, 0.106217],
+ [0.835270, 0.886029, 0.102646],
+ [0.845561, 0.887322, 0.099702],
+ [0.855810, 0.888601, 0.097452],
+ [0.866013, 0.889868, 0.095953],
+ [0.876168, 0.891125, 0.095250],
+ [0.886271, 0.892374, 0.095374],
+ [0.896320, 0.893616, 0.096335],
+ [0.906311, 0.894855, 0.098125],
+ [0.916242, 0.896091, 0.100717],
+ [0.926106, 0.897330, 0.104071],
+ [0.935904, 0.898570, 0.108131],
+ [0.945636, 0.899815, 0.112838],
+ [0.955300, 0.901065, 0.118128],
+ [0.964894, 0.902323, 0.123941],
+ [0.974417, 0.903590, 0.130215],
+ [0.983868, 0.904867, 0.136897],
+ [0.993248, 0.906157, 0.143936],
+]
default_colormap = "plasma"
@@ -1039,7 +1047,9 @@
def read_selected_colormap_name_from_settings() -> str:
- selected = settings.read("spectrogram_colormap", default_value=default_colormap, type=str)
+ selected = settings.read(
+ "spectrogram_colormap", default_value=default_colormap, type=str
+ )
if selected not in maps.keys():
selected = default_colormap
return selected
diff --git a/src/urh/controller/CompareFrameController.py b/src/urh/controller/CompareFrameController.py
index 54c85acb5c..a5f4563749 100644
--- a/src/urh/controller/CompareFrameController.py
+++ b/src/urh/controller/CompareFrameController.py
@@ -5,10 +5,26 @@
from datetime import datetime
import numpy
-from PyQt5.QtCore import pyqtSlot, QTimer, Qt, pyqtSignal, QItemSelection, QItemSelectionModel, QLocale, \
- QModelIndex
+from PyQt5.QtCore import (
+ pyqtSlot,
+ QTimer,
+ Qt,
+ pyqtSignal,
+ QItemSelection,
+ QItemSelectionModel,
+ QLocale,
+ QModelIndex,
+)
from PyQt5.QtGui import QContextMenuEvent, QIcon
-from PyQt5.QtWidgets import QMessageBox, QAbstractItemView, QUndoStack, QMenu, QWidget, QHeaderView, QInputDialog
+from PyQt5.QtWidgets import (
+ QMessageBox,
+ QAbstractItemView,
+ QUndoStack,
+ QMenu,
+ QWidget,
+ QHeaderView,
+ QInputDialog,
+)
from urh import settings
from urh.awre import AutoAssigner
@@ -44,8 +60,9 @@ class CompareFrameController(QWidget):
show_config_field_types_triggered = pyqtSignal()
load_protocol_clicked = pyqtSignal()
- def __init__(self, plugin_manager: PluginManager, project_manager: ProjectManager, parent):
-
+ def __init__(
+ self, plugin_manager: PluginManager, project_manager: ProjectManager, parent
+ ):
super().__init__(parent)
self.proto_analyzer = ProtocolAnalyzer(None)
@@ -71,23 +88,39 @@ def __init__(self, plugin_manager: PluginManager, project_manager: ProjectManage
self.selected_protocols = set()
self.search_select_filter_align_menu = QMenu()
- self.search_action = self.search_select_filter_align_menu.addAction(self.tr("Search"))
- self.select_action = self.search_select_filter_align_menu.addAction(self.tr("Select all"))
- self.filter_action = self.search_select_filter_align_menu.addAction(self.tr("Filter"))
- self.align_action = self.search_select_filter_align_menu.addAction(self.tr("Align"))
+ self.search_action = self.search_select_filter_align_menu.addAction(
+ self.tr("Search")
+ )
+ self.select_action = self.search_select_filter_align_menu.addAction(
+ self.tr("Select all")
+ )
+ self.filter_action = self.search_select_filter_align_menu.addAction(
+ self.tr("Filter")
+ )
+ self.align_action = self.search_select_filter_align_menu.addAction(
+ self.tr("Align")
+ )
self.ui.btnSearchSelectFilter.setMenu(self.search_select_filter_align_menu)
self.analyze_menu = QMenu()
- self.assign_participants_action = self.analyze_menu.addAction(self.tr("Assign participants"))
+ self.assign_participants_action = self.analyze_menu.addAction(
+ self.tr("Assign participants")
+ )
self.assign_participants_action.setCheckable(True)
self.assign_participants_action.setChecked(True)
- self.assign_message_type_action = self.analyze_menu.addAction(self.tr("Assign message type"))
+ self.assign_message_type_action = self.analyze_menu.addAction(
+ self.tr("Assign message type")
+ )
self.assign_message_type_action.setCheckable(True)
self.assign_message_type_action.setChecked(True)
- self.assign_labels_action = self.analyze_menu.addAction(self.tr("Assign labels"))
+ self.assign_labels_action = self.analyze_menu.addAction(
+ self.tr("Assign labels")
+ )
self.assign_labels_action.setCheckable(True)
self.assign_labels_action.setChecked(True)
- self.assign_participant_address_action = self.analyze_menu.addAction(self.tr("Assign participant addresses"))
+ self.assign_participant_address_action = self.analyze_menu.addAction(
+ self.tr("Assign participant addresses")
+ )
self.assign_participant_address_action.setCheckable(True)
self.assign_participant_address_action.setChecked(True)
self.ui.btnAnalyze.setMenu(self.analyze_menu)
@@ -95,32 +128,49 @@ def __init__(self, plugin_manager: PluginManager, project_manager: ProjectManage
self.ui.lblShownRows.hide()
self.ui.lblClearAlignment.hide()
- self.protocol_model = ProtocolTableModel(self.proto_analyzer, project_manager.participants,
- self) # type: ProtocolTableModel
- self.message_type_table_model = MessageTypeTableModel(self.proto_analyzer.message_types)
+ self.protocol_model = ProtocolTableModel(
+ self.proto_analyzer, project_manager.participants, self
+ ) # type: ProtocolTableModel
+ self.message_type_table_model = MessageTypeTableModel(
+ self.proto_analyzer.message_types
+ )
- self.label_value_model = LabelValueTableModel(self.proto_analyzer,
- controller=self) # type: LabelValueTableModel
+ self.label_value_model = LabelValueTableModel(
+ self.proto_analyzer, controller=self
+ ) # type: LabelValueTableModel
self.ui.tblViewProtocol.setModel(self.protocol_model)
self.ui.tblViewProtocol.controller = self
self.ui.tblLabelValues.setModel(self.label_value_model)
self.ui.tblViewMessageTypes.setModel(self.message_type_table_model)
- self.ui.tblViewMessageTypes.setItemDelegateForColumn(1, MessageTypeButtonDelegate(
- parent=self.ui.tblViewMessageTypes))
- self.ui.tblViewMessageTypes.horizontalHeader().setSectionResizeMode(0, QHeaderView.Stretch)
- self.ui.tblViewMessageTypes.horizontalHeader().setSectionResizeMode(1, QHeaderView.ResizeToContents)
-
- self.ui.tblLabelValues.horizontalHeader().setSectionResizeMode(1, QHeaderView.ResizeToContents)
- self.ui.tblLabelValues.horizontalHeader().setSectionResizeMode(2, QHeaderView.ResizeToContents)
- self.ui.tblLabelValues.horizontalHeader().setSectionResizeMode(3, QHeaderView.ResizeToContents)
+ self.ui.tblViewMessageTypes.setItemDelegateForColumn(
+ 1, MessageTypeButtonDelegate(parent=self.ui.tblViewMessageTypes)
+ )
+ self.ui.tblViewMessageTypes.horizontalHeader().setSectionResizeMode(
+ 0, QHeaderView.Stretch
+ )
+ self.ui.tblViewMessageTypes.horizontalHeader().setSectionResizeMode(
+ 1, QHeaderView.ResizeToContents
+ )
+
+ self.ui.tblLabelValues.horizontalHeader().setSectionResizeMode(
+ 1, QHeaderView.ResizeToContents
+ )
+ self.ui.tblLabelValues.horizontalHeader().setSectionResizeMode(
+ 2, QHeaderView.ResizeToContents
+ )
+ self.ui.tblLabelValues.horizontalHeader().setSectionResizeMode(
+ 3, QHeaderView.ResizeToContents
+ )
self.selection_timer = QTimer(self)
self.selection_timer.setSingleShot(True)
self.setAcceptDrops(False)
- self.proto_tree_model = ProtocolTreeModel(controller=self) # type: ProtocolTreeModel
+ self.proto_tree_model = ProtocolTreeModel(
+ controller=self
+ ) # type: ProtocolTreeModel
self.ui.treeViewProtocols.setModel(self.proto_tree_model)
self.create_connects()
@@ -238,68 +288,119 @@ def __set_decoding_error_label(self, message: Message):
if message:
errors = message.decoding_errors
percent = 100 * (errors / len(message))
- state = message.decoding_state if message.decoding_state != message.decoder.ErrorState.SUCCESS else ""
+ state = (
+ message.decoding_state
+ if message.decoding_state != message.decoder.ErrorState.SUCCESS
+ else ""
+ )
color = "green" if errors == 0 and state == "" else "red"
self.ui.lDecodingErrorsValue.setStyleSheet("color: " + color)
- self.ui.lDecodingErrorsValue.setText(locale.format_string("%d (%.02f%%) %s", (errors, percent, state)))
+ self.ui.lDecodingErrorsValue.setText(
+ locale.format_string("%d (%.02f%%) %s", (errors, percent, state))
+ )
else:
self.ui.lDecodingErrorsValue.setText("No message selected")
def create_connects(self):
self.protocol_undo_stack.indexChanged.connect(self.on_undo_stack_index_changed)
self.ui.cbProtoView.currentIndexChanged.connect(self.on_protocol_view_changed)
- self.ui.cbDecoding.currentIndexChanged.connect(self.on_combobox_decoding_current_index_changed)
+ self.ui.cbDecoding.currentIndexChanged.connect(
+ self.on_combobox_decoding_current_index_changed
+ )
self.ui.cbShowDiffs.clicked.connect(self.on_chkbox_show_differences_clicked)
- self.ui.chkBoxOnlyShowLabelsInProtocol.stateChanged.connect(self.on_check_box_show_only_labels_state_changed)
- self.ui.chkBoxShowOnlyDiffs.stateChanged.connect(self.on_check_box_show_only_diffs_state_changed)
+ self.ui.chkBoxOnlyShowLabelsInProtocol.stateChanged.connect(
+ self.on_check_box_show_only_labels_state_changed
+ )
+ self.ui.chkBoxShowOnlyDiffs.stateChanged.connect(
+ self.on_check_box_show_only_diffs_state_changed
+ )
self.protocol_model.vertical_header_color_status_changed.connect(
- self.ui.tblViewProtocol.on_vertical_header_color_status_changed)
-
- self.ui.tblViewProtocol.show_interpretation_clicked.connect(self.show_interpretation_clicked.emit)
- self.ui.tblViewProtocol.protocol_view_change_clicked.connect(self.ui.cbProtoView.setCurrentIndex)
- self.ui.tblViewProtocol.selection_changed.connect(self.on_table_selection_changed)
+ self.ui.tblViewProtocol.on_vertical_header_color_status_changed
+ )
+
+ self.ui.tblViewProtocol.show_interpretation_clicked.connect(
+ self.show_interpretation_clicked.emit
+ )
+ self.ui.tblViewProtocol.protocol_view_change_clicked.connect(
+ self.ui.cbProtoView.setCurrentIndex
+ )
+ self.ui.tblViewProtocol.selection_changed.connect(
+ self.on_table_selection_changed
+ )
self.ui.tblViewProtocol.writeable_changed.connect(self.on_writeable_changed)
- self.ui.tblViewProtocol.row_visibility_changed.connect(self.on_tbl_view_protocol_row_visibility_changed)
- self.ui.tblViewProtocol.edit_label_triggered.connect(self.on_edit_label_clicked_in_table)
- self.ui.tblViewProtocol.create_label_triggered.connect(self.on_create_label_triggered)
+ self.ui.tblViewProtocol.row_visibility_changed.connect(
+ self.on_tbl_view_protocol_row_visibility_changed
+ )
+ self.ui.tblViewProtocol.edit_label_triggered.connect(
+ self.on_edit_label_clicked_in_table
+ )
+ self.ui.tblViewProtocol.create_label_triggered.connect(
+ self.on_create_label_triggered
+ )
self.ui.tblViewProtocol.participant_changed.connect(self.on_participant_edited)
- self.ui.tblViewProtocol.messagetype_selected.connect(self.on_message_type_selected)
- self.ui.tblViewProtocol.new_messagetype_clicked.connect(self.on_table_new_message_type_clicked)
+ self.ui.tblViewProtocol.messagetype_selected.connect(
+ self.on_message_type_selected
+ )
+ self.ui.tblViewProtocol.new_messagetype_clicked.connect(
+ self.on_table_new_message_type_clicked
+ )
self.ui.tblViewProtocol.files_dropped.connect(self.on_files_dropped)
- self.ui.tblLabelValues.edit_label_action_triggered.connect(self.on_edit_label_action_triggered)
+ self.ui.tblLabelValues.edit_label_action_triggered.connect(
+ self.on_edit_label_action_triggered
+ )
self.ui.tblLabelValues.configure_field_types_action_triggered.connect(
- self.show_config_field_types_triggered.emit)
-
- self.label_value_model.protolabel_visibility_changed.connect(self.on_protolabel_visibility_changed)
- self.label_value_model.protocol_label_name_edited.connect(self.label_value_model.update)
+ self.show_config_field_types_triggered.emit
+ )
+
+ self.label_value_model.protolabel_visibility_changed.connect(
+ self.on_protolabel_visibility_changed
+ )
+ self.label_value_model.protocol_label_name_edited.connect(
+ self.label_value_model.update
+ )
self.label_value_model.label_removed.connect(self.on_label_removed)
self.label_value_model.label_color_changed.connect(self.on_label_color_changed)
self.ui.tblViewMessageTypes.selectionModel().currentRowChanged.connect(
- self.on_tbl_view_message_current_row_changed)
+ self.on_tbl_view_message_current_row_changed
+ )
self.ui.tblViewMessageTypes.configure_message_type_rules_triggered.connect(
- self.on_configure_message_type_rules_triggered)
+ self.on_configure_message_type_rules_triggered
+ )
self.ui.tblViewMessageTypes.auto_message_type_update_triggered.connect(
- self.update_automatic_assigned_message_types)
-
- self.message_type_table_model.modelReset.connect(self.on_message_type_table_model_updated)
- self.message_type_table_model.message_type_removed.connect(self.on_message_type_removed)
- self.message_type_table_model.message_type_visibility_changed.connect(self.on_message_type_visibility_changed)
- self.message_type_table_model.message_type_name_edited.connect(self.on_message_type_name_edited)
+ self.update_automatic_assigned_message_types
+ )
+
+ self.message_type_table_model.modelReset.connect(
+ self.on_message_type_table_model_updated
+ )
+ self.message_type_table_model.message_type_removed.connect(
+ self.on_message_type_removed
+ )
+ self.message_type_table_model.message_type_visibility_changed.connect(
+ self.on_message_type_visibility_changed
+ )
+ self.message_type_table_model.message_type_name_edited.connect(
+ self.on_message_type_name_edited
+ )
self.ui.btnSearchSelectFilter.clicked.connect(self.on_btn_search_clicked)
self.ui.btnNextSearch.clicked.connect(self.on_btn_next_search_clicked)
self.ui.btnPrevSearch.clicked.connect(self.on_btn_prev_search_clicked)
- self.ui.lineEditSearch.returnPressed.connect(self.ui.btnSearchSelectFilter.click)
+ self.ui.lineEditSearch.returnPressed.connect(
+ self.ui.btnSearchSelectFilter.click
+ )
self.search_action.triggered.connect(self.on_search_action_triggered)
self.select_action.triggered.connect(self.on_select_action_triggered)
self.filter_action.triggered.connect(self.on_filter_action_triggered)
self.align_action.triggered.connect(self.on_align_action_triggered)
self.ui.lblShownRows.linkActivated.connect(self.on_label_shown_link_activated)
- self.ui.lblClearAlignment.linkActivated.connect(self.on_label_clear_alignment_link_activated)
+ self.ui.lblClearAlignment.linkActivated.connect(
+ self.on_label_clear_alignment_link_activated
+ )
self.ui.btnSaveProto.clicked.connect(self.on_btn_save_protocol_clicked)
self.ui.btnLoadProto.clicked.connect(self.on_btn_load_proto_clicked)
@@ -309,12 +410,16 @@ def create_connects(self):
self.protocol_model.ref_index_changed.connect(self.on_ref_index_changed)
self.project_manager.project_updated.connect(self.on_project_updated)
- self.participant_list_model.show_state_changed.connect(self.on_participant_show_state_changed)
+ self.participant_list_model.show_state_changed.connect(
+ self.on_participant_show_state_changed
+ )
self.ui.btnAddMessagetype.clicked.connect(self.on_btn_new_message_type_clicked)
self.selection_timer.timeout.connect(self.on_table_selection_timer_timeout)
- self.ui.treeViewProtocols.selection_changed.connect(self.on_tree_view_selection_changed)
+ self.ui.treeViewProtocols.selection_changed.connect(
+ self.on_tree_view_selection_changed
+ )
self.proto_tree_model.item_dropped.connect(self.on_item_in_proto_tree_dropped)
self.proto_tree_model.group_deleted.connect(self.on_group_deleted)
@@ -323,7 +428,14 @@ def create_connects(self):
self.ui.tabWidget.tabBarDoubleClicked.connect(self.on_tab_bar_double_clicked)
def get_message_type_for_label(self, lbl: ProtocolLabel) -> MessageType:
- return next((msg_type for msg_type in self.proto_analyzer.message_types if lbl in msg_type), None)
+ return next(
+ (
+ msg_type
+ for msg_type in self.proto_analyzer.message_types
+ if lbl in msg_type
+ ),
+ None,
+ )
def update_field_type_combobox(self):
field_types = [ft.caption for ft in self.field_types]
@@ -343,9 +455,14 @@ def set_decoding(self, decoding: Encoding, messages=None):
if messages is None:
messages = self.proto_analyzer.messages
if len(messages) > 10:
- reply = QMessageBox.question(self, "Set decoding",
- "Do you want to apply the selected decoding to {} messages?".format(
- len(messages)), QMessageBox.Yes | QMessageBox.No)
+ reply = QMessageBox.question(
+ self,
+ "Set decoding",
+ "Do you want to apply the selected decoding to {} messages?".format(
+ len(messages)
+ ),
+ QMessageBox.Yes | QMessageBox.No,
+ )
if reply != QMessageBox.Yes:
self.ui.cbDecoding.blockSignals(True)
self.ui.cbDecoding.setCurrentText("...")
@@ -363,7 +480,11 @@ def set_decoding(self, decoding: Encoding, messages=None):
selected = self.ui.tblViewProtocol.selectionModel().selection()
- if not selected.isEmpty() and self.isVisible() and self.proto_analyzer.num_messages > 0:
+ if (
+ not selected.isEmpty()
+ and self.isVisible()
+ and self.proto_analyzer.num_messages > 0
+ ):
min_row = min(rng.top() for rng in selected)
min_row = min_row if min_row < len(self.proto_analyzer.messages) else -1
try:
@@ -396,7 +517,9 @@ def refresh_existing_encodings(self):
update = False
for msg in self.proto_analyzer.messages:
- decoder = next((d for d in self.decodings if d.name == msg.decoder.name), None)
+ decoder = next(
+ (d for d in self.decodings if d.name == msg.decoder.name), None
+ )
if decoder is None:
continue
@@ -411,7 +534,9 @@ def refresh_existing_encodings(self):
self.label_value_model.update()
def fill_decoding_combobox(self):
- cur_item = self.ui.cbDecoding.currentText() if self.ui.cbDecoding.count() > 0 else None
+ cur_item = (
+ self.ui.cbDecoding.currentText() if self.ui.cbDecoding.count() > 0 else None
+ )
self.ui.cbDecoding.blockSignals(True)
self.ui.cbDecoding.clear()
prev_index = 0
@@ -425,12 +550,16 @@ def fill_decoding_combobox(self):
self.ui.cbDecoding.setToolTip(self.ui.cbDecoding.currentText())
self.ui.cbDecoding.blockSignals(False)
- def add_protocol(self, protocol: ProtocolAnalyzer, group_id: int = 0) -> ProtocolAnalyzer:
+ def add_protocol(
+ self, protocol: ProtocolAnalyzer, group_id: int = 0
+ ) -> ProtocolAnalyzer:
self.__protocols = None
self.proto_tree_model.add_protocol(protocol, group_id)
protocol.qt_signals.protocol_updated.connect(self.on_protocol_updated)
if protocol.signal:
- protocol.signal.sample_rate_changed.connect(self.set_shown_protocols) # Refresh times
+ protocol.signal.sample_rate_changed.connect(
+ self.set_shown_protocols
+ ) # Refresh times
protocol.qt_signals.show_state_changed.connect(self.set_shown_protocols)
protocol.qt_signals.show_state_changed.connect(self.filter_search_results)
for i in range(self.proto_tree_model.ngroups):
@@ -447,13 +576,22 @@ def add_protocol_from_file(self, filename: str) -> ProtocolAnalyzer:
pa.from_xml_file(filename=filename, read_bits=True)
for messsage_type in pa.message_types:
if messsage_type not in self.proto_analyzer.message_types:
- if messsage_type.name in (mt.name for mt in self.proto_analyzer.message_types):
- messsage_type.name += " (" + os.path.split(filename)[1].rstrip(".xml").rstrip(".proto") + ")"
+ if messsage_type.name in (
+ mt.name for mt in self.proto_analyzer.message_types
+ ):
+ messsage_type.name += (
+ " ("
+ + os.path.split(filename)[1].rstrip(".xml").rstrip(".proto")
+ + ")"
+ )
self.proto_analyzer.message_types.append(messsage_type)
update_project = False
for msg in pa.messages:
- if msg.participant is not None and msg.participant not in self.project_manager.participants:
+ if (
+ msg.participant is not None
+ and msg.participant not in self.project_manager.participants
+ ):
self.project_manager.participants.append(msg.participant)
update_project = True
@@ -469,23 +607,40 @@ def add_protocol_from_file(self, filename: str) -> ProtocolAnalyzer:
def add_sniffed_protocol_messages(self, messages: list):
if len(messages) > 0:
proto_analyzer = ProtocolAnalyzer(None)
- proto_analyzer.name = datetime.fromtimestamp(messages[0].timestamp).strftime("%Y-%m-%d %H:%M:%S")
+ proto_analyzer.name = datetime.fromtimestamp(
+ messages[0].timestamp
+ ).strftime("%Y-%m-%d %H:%M:%S")
proto_analyzer.messages = messages
- self.add_protocol(proto_analyzer, group_id=self.proto_tree_model.ngroups - 1)
+ self.add_protocol(
+ proto_analyzer, group_id=self.proto_tree_model.ngroups - 1
+ )
self.refresh()
- def add_protocol_label(self, start: int, end: int, messagenr: int, proto_view: int, edit_label_name=True):
+ def add_protocol_label(
+ self,
+ start: int,
+ end: int,
+ messagenr: int,
+ proto_view: int,
+ edit_label_name=True,
+ ):
# Ensure at least one Group is active
- start, end = self.proto_analyzer.convert_range(start, end, proto_view, 0, decoded=True, message_indx=messagenr)
+ start, end = self.proto_analyzer.convert_range(
+ start, end, proto_view, 0, decoded=True, message_indx=messagenr
+ )
message_type = self.proto_analyzer.messages[messagenr].message_type
try:
used_field_types = [lbl.field_type for lbl in message_type]
- first_unused_type = next(ft for ft in self.field_types if ft not in used_field_types)
+ first_unused_type = next(
+ ft for ft in self.field_types if ft not in used_field_types
+ )
name = first_unused_type.caption
except (StopIteration, AttributeError):
first_unused_type, name = None, None
- proto_label = message_type.add_protocol_label(start=start, end=end, name=name, type=first_unused_type)
+ proto_label = message_type.add_protocol_label(
+ start=start, end=end, name=name, type=first_unused_type
+ )
self.message_type_table_model.update()
self.protocol_model.update()
@@ -493,7 +648,9 @@ def add_protocol_label(self, start: int, end: int, messagenr: int, proto_view: i
if edit_label_name:
try:
- index = self.ui.tblLabelValues.model().index(message_type.index(proto_label), 0)
+ index = self.ui.tblLabelValues.model().index(
+ message_type.index(proto_label), 0
+ )
self.ui.tblLabelValues.setCurrentIndex(index)
self.ui.tblLabelValues.edit(index)
except ValueError:
@@ -502,8 +659,12 @@ def add_protocol_label(self, start: int, end: int, messagenr: int, proto_view: i
return True
def add_message_type(self, selected_messages: list = None):
- selected_messages = selected_messages if isinstance(selected_messages, list) else []
- self.proto_analyzer.add_new_message_type(labels=self.proto_analyzer.default_message_type)
+ selected_messages = (
+ selected_messages if isinstance(selected_messages, list) else []
+ )
+ self.proto_analyzer.add_new_message_type(
+ labels=self.proto_analyzer.default_message_type
+ )
self.message_type_table_model.update()
self.active_message_type = self.proto_analyzer.message_types[-1]
for msg in selected_messages:
@@ -521,13 +682,18 @@ def remove_protocol(self, protocol: ProtocolAnalyzer):
self.set_shown_protocols()
def set_shown_protocols(self):
- hidden_rows = {i for i in range(self.protocol_model.row_count) if self.ui.tblViewProtocol.isRowHidden(i)}
+ hidden_rows = {
+ i
+ for i in range(self.protocol_model.row_count)
+ if self.ui.tblViewProtocol.isRowHidden(i)
+ }
relative_hidden_row_positions = {}
for proto in self.rows_for_protocols.keys():
if any(i in hidden_rows for i in self.rows_for_protocols[proto]):
m = min(self.rows_for_protocols[proto])
- relative_hidden_row_positions[proto] = [i - m for i in hidden_rows if
- i in self.rows_for_protocols[proto]]
+ relative_hidden_row_positions[proto] = [
+ i - m for i in hidden_rows if i in self.rows_for_protocols[proto]
+ ]
# self.protocol_undo_stack.clear()
self.proto_analyzer.messages[:] = []
@@ -549,13 +715,19 @@ def set_shown_protocols(self):
try:
if hasattr(proto.signal, "sample_rate"):
if i > 0:
- rel_time = proto.messages[i - 1].get_duration(proto.signal.sample_rate)
+ rel_time = proto.messages[i - 1].get_duration(
+ proto.signal.sample_rate
+ )
abs_time += rel_time
else:
# No signal, loaded from protocol file
- abs_time = datetime.fromtimestamp(message.timestamp).strftime("%Y-%m-%d %H:%M:%S.%f")
+ abs_time = datetime.fromtimestamp(
+ message.timestamp
+ ).strftime("%Y-%m-%d %H:%M:%S.%f")
if i > 0:
- rel_time = message.timestamp - proto.messages[i - 1].timestamp
+ rel_time = (
+ message.timestamp - proto.messages[i - 1].timestamp
+ )
except IndexError:
pass
@@ -622,16 +794,22 @@ def restore_selection(self, old_view: int, sel_cols, sel_rows):
start_index = self.protocol_model.index(start_row, start_col)
end_index = self.protocol_model.index(end_row, end_col)
- mid_index = self.protocol_model.index(int((start_row + end_row) / 2), int((start_col + end_col) / 2))
+ mid_index = self.protocol_model.index(
+ int((start_row + end_row) / 2), int((start_col + end_col) / 2)
+ )
sel = QItemSelection()
sel.select(start_index, end_index)
- self.ui.tblViewProtocol.selectionModel().select(sel, QItemSelectionModel.ClearAndSelect)
+ self.ui.tblViewProtocol.selectionModel().select(
+ sel, QItemSelectionModel.ClearAndSelect
+ )
self.ui.tblViewProtocol.scrollTo(mid_index)
def expand_group_node(self, group_id):
- index = self.proto_tree_model.createIndex(group_id, 0, self.proto_tree_model.rootItem.child(group_id))
+ index = self.proto_tree_model.createIndex(
+ group_id, 0, self.proto_tree_model.rootItem.child(group_id)
+ )
self.ui.treeViewProtocols.expand(index)
def updateUI(self, ignore_table_model=False, resize_table=True):
@@ -658,13 +836,25 @@ def create_protocol_label_dialog(self, selected_index=None):
view_type = self.ui.cbProtoView.currentIndex()
try:
longest_message = max(
- (msg for msg in self.proto_analyzer.messages if msg.message_type == self.active_message_type), key=len)
+ (
+ msg
+ for msg in self.proto_analyzer.messages
+ if msg.message_type == self.active_message_type
+ ),
+ key=len,
+ )
except ValueError:
logger.warning("Configuring message type with empty message set.")
longest_message = Message([True] * 1000, 1000, self.active_message_type)
- protocol_label_dialog = ProtocolLabelDialog(message=longest_message, viewtype=view_type,
- selected_index=selected_index, parent=self)
- protocol_label_dialog.apply_decoding_changed.connect(self.on_apply_decoding_changed)
+ protocol_label_dialog = ProtocolLabelDialog(
+ message=longest_message,
+ viewtype=view_type,
+ selected_index=selected_index,
+ parent=self,
+ )
+ protocol_label_dialog.apply_decoding_changed.connect(
+ self.on_apply_decoding_changed
+ )
protocol_label_dialog.finished.connect(self.on_protocol_label_dialog_finished)
return protocol_label_dialog
@@ -692,14 +882,20 @@ def select_all_search_results(self):
for search_result in self.protocol_model.search_results:
startindex = self.protocol_model.index(search_result[0], search_result[1])
- endindex = self.protocol_model.index(search_result[0],
- search_result[1] + len(self.protocol_model.search_value) - 1)
+ endindex = self.protocol_model.index(
+ search_result[0],
+ search_result[1] + len(self.protocol_model.search_value) - 1,
+ )
sel = QItemSelection()
sel.select(startindex, endindex)
- self.ui.tblViewProtocol.selectionModel().select(sel, QItemSelectionModel.Select)
- self.ui.tblViewProtocol.scrollTo(startindex, QAbstractItemView.PositionAtCenter)
+ self.ui.tblViewProtocol.selectionModel().select(
+ sel, QItemSelectionModel.Select
+ )
+ self.ui.tblViewProtocol.scrollTo(
+ startindex, QAbstractItemView.PositionAtCenter
+ )
self.ui.tblViewProtocol.setFocus()
@@ -714,7 +910,9 @@ def filter_search_results(self):
self.search()
self.ui.tblLabelValues.clearSelection()
- matching_rows = set(search_result[0] for search_result in self.protocol_model.search_results)
+ matching_rows = set(
+ search_result[0] for search_result in self.protocol_model.search_results
+ )
rows_to_hide = set(range(0, self.protocol_model.row_count)) - matching_rows
self.ui.tblViewProtocol.hide_rows(rows_to_hide)
else:
@@ -727,15 +925,21 @@ def __set_shown_rows_status_label(self):
if len(self.protocol_model.hidden_rows) > 0:
rc = self.protocol_model.row_count
text = self.tr("shown: {}/{} (reset)")
- self.ui.lblShownRows.setText(text.format(rc - len(self.protocol_model.hidden_rows), rc))
+ self.ui.lblShownRows.setText(
+ text.format(rc - len(self.protocol_model.hidden_rows), rc)
+ )
self.ui.lblShownRows.show()
else:
self.ui.lblShownRows.hide()
def align_messages(self, pattern=None):
pattern = self.ui.lineEditSearch.text() if pattern is None else pattern
- self.proto_analyzer.align_messages(pattern, view_type=self.ui.cbProtoView.currentIndex())
- self.ui.lblClearAlignment.setVisible(any(msg.alignment_offset != 0 for msg in self.proto_analyzer.messages))
+ self.proto_analyzer.align_messages(
+ pattern, view_type=self.ui.cbProtoView.currentIndex()
+ )
+ self.ui.lblClearAlignment.setVisible(
+ any(msg.alignment_offset != 0 for msg in self.proto_analyzer.messages)
+ )
self.protocol_model.update()
row = column = 0
@@ -743,10 +947,17 @@ def align_messages(self, pattern=None):
if self.ui.tblViewProtocol.isRowHidden(i):
continue
- data = message.view_to_string(self.ui.cbProtoView.currentIndex(), decoded=True)
+ data = message.view_to_string(
+ self.ui.cbProtoView.currentIndex(), decoded=True
+ )
try:
row = i
- column = data.index(pattern) + len(pattern) + self.protocol_model.get_alignment_offset_at(i) - 1
+ column = (
+ data.index(pattern)
+ + len(pattern)
+ + self.protocol_model.get_alignment_offset_at(i)
+ - 1
+ )
break
except ValueError:
pass
@@ -763,14 +974,20 @@ def next_search_result(self):
try:
search_result = self.protocol_model.search_results[index]
startindex = self.protocol_model.index(search_result[0], search_result[1])
- endindex = self.protocol_model.index(search_result[0],
- search_result[1] + len(self.protocol_model.search_value) - 1)
+ endindex = self.protocol_model.index(
+ search_result[0],
+ search_result[1] + len(self.protocol_model.search_value) - 1,
+ )
sel = QItemSelection()
sel.select(startindex, endindex)
- self.ui.tblViewProtocol.selectionModel().select(sel, QItemSelectionModel.ClearAndSelect)
- self.ui.tblViewProtocol.scrollTo(startindex, QAbstractItemView.PositionAtCenter)
+ self.ui.tblViewProtocol.selectionModel().select(
+ sel, QItemSelectionModel.ClearAndSelect
+ )
+ self.ui.tblViewProtocol.scrollTo(
+ startindex, QAbstractItemView.PositionAtCenter
+ )
self.ui.lSearchCurrent.setText(str(index + 1))
except IndexError:
@@ -788,14 +1005,20 @@ def prev_search_result(self):
try:
search_result = self.protocol_model.search_results[index]
startindex = self.protocol_model.index(search_result[0], search_result[1])
- endindex = self.protocol_model.index(search_result[0],
- search_result[1] + len(self.protocol_model.search_value) - 1)
+ endindex = self.protocol_model.index(
+ search_result[0],
+ search_result[1] + len(self.protocol_model.search_value) - 1,
+ )
sel = QItemSelection()
sel.select(startindex, endindex)
- self.ui.tblViewProtocol.selectionModel().select(sel, QItemSelectionModel.ClearAndSelect)
- self.ui.tblViewProtocol.scrollTo(startindex, QAbstractItemView.PositionAtCenter)
+ self.ui.tblViewProtocol.selectionModel().select(
+ sel, QItemSelectionModel.ClearAndSelect
+ )
+ self.ui.tblViewProtocol.scrollTo(
+ startindex, QAbstractItemView.PositionAtCenter
+ )
self.ui.lSearchCurrent.setText(str(index + 1))
except IndexError:
@@ -815,10 +1038,20 @@ def clear_search(self):
self.protocol_model.search_results[:] = []
self.protocol_model.search_value = ""
- def set_protocol_label_visibility(self, lbl: ProtocolLabel, message: Message = None):
+ def set_protocol_label_visibility(
+ self, lbl: ProtocolLabel, message: Message = None
+ ):
try:
- message = message if message else next(m for m in self.proto_analyzer.messages if lbl in m.message_type)
- start, end = message.get_label_range(lbl, self.ui.cbProtoView.currentIndex(), True, consider_alignment=True)
+ message = (
+ message
+ if message
+ else next(
+ m for m in self.proto_analyzer.messages if lbl in m.message_type
+ )
+ )
+ start, end = message.get_label_range(
+ lbl, self.ui.cbProtoView.currentIndex(), True, consider_alignment=True
+ )
for i in range(start, end):
self.ui.tblViewProtocol.setColumnHidden(i, not lbl.show)
@@ -827,7 +1060,11 @@ def set_protocol_label_visibility(self, lbl: ProtocolLabel, message: Message = N
def set_message_type_visibility(self, message_type: MessageType):
try:
- rows = {i for i, msg in enumerate(self.proto_analyzer.messages) if msg.message_type == message_type}
+ rows = {
+ i
+ for i, msg in enumerate(self.proto_analyzer.messages)
+ if msg.message_type == message_type
+ }
if message_type.show:
self.ui.tblViewProtocol.show_rows(rows)
else:
@@ -847,17 +1084,23 @@ def show_all_cols(self):
def save_protocol(self):
for msg in self.proto_analyzer.messages:
if not msg.decoder.is_nrz:
- reply = QMessageBox.question(self, "Saving of protocol",
- "You want to save this protocol with an encoding different from NRZ.\n"
- "This may cause loss of information if you load it again.\n\n"
- "Save anyway?", QMessageBox.Yes | QMessageBox.No)
+ reply = QMessageBox.question(
+ self,
+ "Saving of protocol",
+ "You want to save this protocol with an encoding different from NRZ.\n"
+ "This may cause loss of information if you load it again.\n\n"
+ "Save anyway?",
+ QMessageBox.Yes | QMessageBox.No,
+ )
if reply != QMessageBox.Yes:
return
else:
break
text = "protocol"
- filename = FileOperator.ask_save_file_name("{0}.proto.xml".format(text), caption="Save protocol")
+ filename = FileOperator.ask_save_file_name(
+ "{0}.proto.xml".format(text), caption="Save protocol"
+ )
if not filename:
return
@@ -865,13 +1108,25 @@ def save_protocol(self):
if filename.endswith(".bin"):
self.proto_analyzer.to_binary(filename, use_decoded=True)
elif filename.endswith(".pcapng"):
- data_link_type, ok = QInputDialog.getInt(self, "Link type",
- "Interface Link Type to use (probably one between DLT_USER0-DLT_USER15 (147-162)):", 147, 0, 65535)
+ data_link_type, ok = QInputDialog.getInt(
+ self,
+ "Link type",
+ "Interface Link Type to use (probably one between DLT_USER0-DLT_USER15 (147-162)):",
+ 147,
+ 0,
+ 65535,
+ )
if ok:
- self.proto_analyzer.to_pcapng(filename=filename, link_type=data_link_type)
+ self.proto_analyzer.to_pcapng(
+ filename=filename, link_type=data_link_type
+ )
else:
- self.proto_analyzer.to_xml_file(filename=filename, decoders=self.decodings,
- participants=self.project_manager.participants, write_bits=True)
+ self.proto_analyzer.to_xml_file(
+ filename=filename,
+ decoders=self.decodings,
+ participants=self.project_manager.participants,
+ write_bits=True,
+ )
def show_differences(self, show_differences: bool):
if show_differences:
@@ -890,15 +1145,27 @@ def set_show_only_status(self):
- Show only labels
- Show only Diffs
"""
- if self.ui.chkBoxShowOnlyDiffs.isChecked() and not self.ui.cbShowDiffs.isChecked():
+ if (
+ self.ui.chkBoxShowOnlyDiffs.isChecked()
+ and not self.ui.cbShowDiffs.isChecked()
+ ):
self.ui.cbShowDiffs.setChecked(True)
self.show_differences(True)
- if self.ui.chkBoxOnlyShowLabelsInProtocol.isChecked() and self.ui.chkBoxShowOnlyDiffs.isChecked():
+ if (
+ self.ui.chkBoxOnlyShowLabelsInProtocol.isChecked()
+ and self.ui.chkBoxShowOnlyDiffs.isChecked()
+ ):
self.show_only_diffs_and_labels()
- elif self.ui.chkBoxOnlyShowLabelsInProtocol.isChecked() and not self.ui.chkBoxShowOnlyDiffs.isChecked():
+ elif (
+ self.ui.chkBoxOnlyShowLabelsInProtocol.isChecked()
+ and not self.ui.chkBoxShowOnlyDiffs.isChecked()
+ ):
self.show_only_labels()
- elif not self.ui.chkBoxOnlyShowLabelsInProtocol.isChecked() and self.ui.chkBoxShowOnlyDiffs.isChecked():
+ elif (
+ not self.ui.chkBoxOnlyShowLabelsInProtocol.isChecked()
+ and self.ui.chkBoxShowOnlyDiffs.isChecked()
+ ):
self.show_only_diffs()
else:
self.restore_visibility()
@@ -909,17 +1176,27 @@ def show_only_labels(self):
visible_columns = set()
for msg in self.proto_analyzer.messages:
for lbl in filter(lambda lbl: lbl.show, msg.message_type):
- start, end = msg.get_label_range(lbl=lbl, view=self.ui.cbProtoView.currentIndex(), decode=True)
+ start, end = msg.get_label_range(
+ lbl=lbl, view=self.ui.cbProtoView.currentIndex(), decode=True
+ )
visible_columns |= set(range(start, end))
for i in range(self.protocol_model.col_count):
self.ui.tblViewProtocol.setColumnHidden(i, i not in visible_columns)
def show_only_diffs(self):
- visible_rows = [i for i in range(self.protocol_model.row_count) if not self.ui.tblViewProtocol.isRowHidden(i)
- and i != self.protocol_model.refindex]
-
- visible_diff_columns = [diff_col for i in visible_rows for diff_col in self.protocol_model.diff_columns[i]]
+ visible_rows = [
+ i
+ for i in range(self.protocol_model.row_count)
+ if not self.ui.tblViewProtocol.isRowHidden(i)
+ and i != self.protocol_model.refindex
+ ]
+
+ visible_diff_columns = [
+ diff_col
+ for i in visible_rows
+ for diff_col in self.protocol_model.diff_columns[i]
+ ]
for j in range(self.protocol_model.col_count):
if j in visible_diff_columns:
@@ -931,14 +1208,25 @@ def show_only_diffs_and_labels(self):
visible_label_columns = set()
for lbl in self.proto_analyzer.protocol_labels:
if lbl.show:
- start, end = self.proto_analyzer.messages[0].get_label_range(lbl, self.ui.cbProtoView.currentIndex(),
- True)
- visible_label_columns |= (set(range(start, end)))
-
- visible_rows = [i for i in range(self.protocol_model.row_count) if not self.ui.tblViewProtocol.isRowHidden(i)
- and i != self.protocol_model.refindex]
-
- visible_diff_columns = set([diff_col for i in visible_rows for diff_col in self.protocol_model.diff_columns[i]])
+ start, end = self.proto_analyzer.messages[0].get_label_range(
+ lbl, self.ui.cbProtoView.currentIndex(), True
+ )
+ visible_label_columns |= set(range(start, end))
+
+ visible_rows = [
+ i
+ for i in range(self.protocol_model.row_count)
+ if not self.ui.tblViewProtocol.isRowHidden(i)
+ and i != self.protocol_model.refindex
+ ]
+
+ visible_diff_columns = set(
+ [
+ diff_col
+ for i in visible_rows
+ for diff_col in self.protocol_model.diff_columns[i]
+ ]
+ )
visible_cols = visible_label_columns & visible_diff_columns
for j in range(self.protocol_model.col_count):
@@ -948,12 +1236,16 @@ def show_only_diffs_and_labels(self):
self.ui.tblViewProtocol.hideColumn(j)
def restore_visibility(self):
- selected = self.ui.tblViewProtocol.selectionModel().selection() # type: QItemSelection
+ selected = (
+ self.ui.tblViewProtocol.selectionModel().selection()
+ ) # type: QItemSelection
for i in range(self.protocol_model.col_count):
self.ui.tblViewProtocol.showColumn(i)
- for lbl in filter(lambda lbl: not lbl.show, self.proto_analyzer.protocol_labels):
+ for lbl in filter(
+ lambda lbl: not lbl.show, self.proto_analyzer.protocol_labels
+ ):
self.set_protocol_label_visibility(lbl)
if not selected.isEmpty():
@@ -961,7 +1253,9 @@ def restore_visibility(self):
start = numpy.min([rng.left() for rng in selected])
self.ui.tblViewProtocol.scrollTo(self.protocol_model.index(min_row, start))
- def get_labels_from_selection(self, row_start: int, row_end: int, col_start: int, col_end: int):
+ def get_labels_from_selection(
+ self, row_start: int, row_end: int, col_start: int, col_end: int
+ ):
"""
:rtype: list of ProtocolLabel
@@ -971,15 +1265,26 @@ def get_labels_from_selection(self, row_start: int, row_end: int, col_start: int
view = self.ui.cbProtoView.currentIndex()
result = []
- f = 1 if self.ui.cbProtoView.currentIndex() == 0 else 4 if self.ui.cbProtoView.currentIndex() == 1 else 8
+ f = (
+ 1
+ if self.ui.cbProtoView.currentIndex() == 0
+ else 4
+ if self.ui.cbProtoView.currentIndex() == 1
+ else 8
+ )
for i in range(row_start, row_end):
message = self.proto_analyzer.messages[i]
for label in message.message_type:
if label in result:
continue
- lbl_start, lbl_end = message.get_label_range(lbl=label, view=view, decode=True)
+ lbl_start, lbl_end = message.get_label_range(
+ lbl=label, view=view, decode=True
+ )
a = message.alignment_offset
- if any(j in range(lbl_start, lbl_end) for j in range(col_start - a//f, col_end - a//f)):
+ if any(
+ j in range(lbl_start, lbl_end)
+ for j in range(col_start - a // f, col_end - a // f)
+ ):
result.append(label)
return result
@@ -1003,8 +1308,12 @@ def refresh_assigned_participants_ui(self):
def refresh_field_types_for_labels(self):
for mt in self.proto_analyzer.message_types:
- for lbl in (lbl for lbl in mt if lbl.field_type is not None): # type: ProtocolLabel
- mt.change_field_type_of_label(lbl, self.field_types_by_caption.get(lbl.field_type.caption, None))
+ for lbl in (
+ lbl for lbl in mt if lbl.field_type is not None
+ ): # type: ProtocolLabel
+ mt.change_field_type_of_label(
+ lbl, self.field_types_by_caption.get(lbl.field_type.caption, None)
+ )
self.update_field_type_combobox()
@@ -1036,7 +1345,9 @@ def on_btn_analyze_clicked(self):
if self.assign_participants_action.isChecked():
for protocol in self.protocol_list:
- AutoAssigner.auto_assign_participants(protocol.messages, self.protocol_model.participants)
+ AutoAssigner.auto_assign_participants(
+ protocol.messages, self.protocol_model.participants
+ )
self.refresh_assigned_participants_ui()
self.ui.progressBarLogicAnalyzer.setFormat("%p% (Assign message type by rules)")
@@ -1045,7 +1356,9 @@ def on_btn_analyze_clicked(self):
if self.assign_message_type_action.isChecked():
self.update_automatic_assigned_message_types()
- self.ui.progressBarLogicAnalyzer.setFormat("%p% (Find new labels/message types)")
+ self.ui.progressBarLogicAnalyzer.setFormat(
+ "%p% (Find new labels/message types)"
+ )
self.ui.progressBarLogicAnalyzer.setValue(75)
if self.assign_labels_action.isChecked():
@@ -1061,8 +1374,9 @@ def on_btn_analyze_clicked(self):
self.ui.progressBarLogicAnalyzer.setValue(90)
if self.assign_participant_address_action.isChecked():
- AutoAssigner.auto_assign_participant_addresses(self.proto_analyzer.messages,
- self.protocol_model.participants)
+ AutoAssigner.auto_assign_participant_addresses(
+ self.proto_analyzer.messages, self.protocol_model.participants
+ )
self.ui.progressBarLogicAnalyzer.setValue(100)
self.unsetCursor()
@@ -1092,7 +1406,9 @@ def on_btn_search_clicked(self):
@pyqtSlot(int)
def on_configure_message_type_rules_triggered(self, message_type_index: int):
- dialog = MessageTypeDialog(self.proto_analyzer.message_types[message_type_index], parent=self)
+ dialog = MessageTypeDialog(
+ self.proto_analyzer.message_types[message_type_index], parent=self
+ )
dialog.show()
dialog.accepted.connect(self.on_message_type_dialog_accepted)
@@ -1118,13 +1434,19 @@ def on_combobox_decoding_current_index_changed(self):
if new_index == self.ui.cbDecoding.count() - 1:
self.set_decoding(None)
else:
- self.set_decoding(self.decodings[new_index],
- messages=self.selected_messages if self.selected_messages else None)
+ self.set_decoding(
+ self.decodings[new_index],
+ messages=self.selected_messages if self.selected_messages else None,
+ )
@pyqtSlot()
def on_participant_show_state_changed(self):
for i, msg in enumerate(self.proto_analyzer.messages):
- hide = not msg.participant.show if msg.participant is not None else not self.participant_list_model.show_unassigned
+ hide = (
+ not msg.participant.show
+ if msg.participant is not None
+ else not self.participant_list_model.show_unassigned
+ )
self.ui.tblViewProtocol.setRowHidden(i, hide)
self.set_shown_protocols()
@@ -1212,11 +1534,17 @@ def on_btn_search_select_filter_clicked():
self.ui.btnSearchSelectFilter.setIcon(QIcon.fromTheme("align-horizontal-left"))
self.set_search_ui_visibility(False)
self.ui.btnSearchSelectFilter.clicked.disconnect()
- self.ui.btnSearchSelectFilter.clicked.connect(on_btn_search_select_filter_clicked)
+ self.ui.btnSearchSelectFilter.clicked.connect(
+ on_btn_search_select_filter_clicked
+ )
@pyqtSlot(bool)
def on_writeable_changed(self, writeable_status: bool):
- hidden_rows = {i for i in range(self.protocol_model.row_count) if self.ui.tblViewProtocol.isRowHidden(i)}
+ hidden_rows = {
+ i
+ for i in range(self.protocol_model.row_count)
+ if self.ui.tblViewProtocol.isRowHidden(i)
+ }
self.protocol_model.is_writeable = writeable_status
self.proto_tree_model.set_copy_mode(writeable_status)
self.ui.cbDecoding.setDisabled(writeable_status)
@@ -1264,8 +1592,12 @@ def on_edit_label_clicked_in_table(self, proto_label_index: int):
@pyqtSlot(int, int, int)
def on_create_label_triggered(self, msg_index, start, end):
a = self.protocol_model.get_alignment_offset_at(msg_index)
- self.add_protocol_label(start=start - a, end=end - a - 1,
- messagenr=msg_index, proto_view=self.ui.cbProtoView.currentIndex())
+ self.add_protocol_label(
+ start=start - a,
+ end=end - a - 1,
+ messagenr=msg_index,
+ proto_view=self.ui.cbProtoView.currentIndex(),
+ )
@pyqtSlot()
def on_edit_label_action_triggered(self):
@@ -1309,7 +1641,9 @@ def on_tree_view_selection_changed(self):
if item.is_group:
active_group_ids.add(self.proto_tree_model.rootItem.index_of(item))
elif item.show:
- active_group_ids.add(self.proto_tree_model.rootItem.index_of(item.parent()))
+ active_group_ids.add(
+ self.proto_tree_model.rootItem.index_of(item.parent())
+ )
if len(active_group_ids) == 0:
active_group_ids.add(0)
@@ -1321,7 +1655,9 @@ def on_tree_view_selection_changed(self):
self.active_group_ids = list(active_group_ids)
self.active_group_ids.sort()
- self.ui.tblViewProtocol.selectionModel().select(sel, QItemSelectionModel.ClearAndSelect)
+ self.ui.tblViewProtocol.selectionModel().select(
+ sel, QItemSelectionModel.ClearAndSelect
+ )
self.ui.tblViewProtocol.blockSignals(False)
self.updateUI(ignore_table_model=ignore_table_model_on_update)
@@ -1342,14 +1678,17 @@ def on_table_selection_timer_timeout(self):
self.ui.lDecimalSelection.setText("")
self.ui.lHexSelection.setText("")
self.ui.lNumSelectedColumns.setText("0")
- self.ui.lblLabelValues.setText(self.tr("Labels of {}".format(self.active_message_type.name)))
+ self.ui.lblLabelValues.setText(
+ self.tr("Labels of {}".format(self.active_message_type.name))
+ )
self.__set_decoding_error_label(message=None)
self.updateUI(ignore_table_model=True, resize_table=False)
return -1, -1
selected_messages = self.selected_messages
self.message_type_table_model.selected_message_type_indices = {
- self.proto_analyzer.message_types.index(msg.message_type) for msg in selected_messages
+ self.proto_analyzer.message_types.index(msg.message_type)
+ for msg in selected_messages
}
cur_view = self.ui.cbProtoView.currentIndex()
@@ -1358,7 +1697,9 @@ def on_table_selection_timer_timeout(self):
message = self.proto_analyzer.messages[min_row]
self.active_message_type = message.message_type
- selected_labels = self.get_labels_from_selection(min_row, min_row, start, end - 1)
+ selected_labels = self.get_labels_from_selection(
+ min_row, min_row, start, end - 1
+ )
self.label_value_model.selected_label_indices = {
self.active_message_type.index(lbl) for lbl in selected_labels
}
@@ -1366,8 +1707,12 @@ def on_table_selection_timer_timeout(self):
f = 4 if cur_view == 1 else 8 if cur_view == 2 else 1
start, end = start * f, end * f
- bits = message.decoded_bits_str[start-message.alignment_offset:end-message.alignment_offset]
- hexs = "".join(("{0:x}".format(int(bits[i:i + 4], 2)) for i in range(0, len(bits), 4)))
+ bits = message.decoded_bits_str[
+ start - message.alignment_offset : end - message.alignment_offset
+ ]
+ hexs = "".join(
+ ("{0:x}".format(int(bits[i : i + 4], 2)) for i in range(0, len(bits), 4))
+ )
decimals = str(int(bits, 2)) if len(bits) > 0 else ""
self.ui.lBitsSelection.setText(bits)
@@ -1375,7 +1720,9 @@ def on_table_selection_timer_timeout(self):
self.ui.lDecimalSelection.setText(decimals)
self.__set_decoding_error_label(message)
- self.ui.lblLabelValues.setText(self.tr("Labels for message #") + str(min_row + 1))
+ self.ui.lblLabelValues.setText(
+ self.tr("Labels for message #") + str(min_row + 1)
+ )
if min_row != self.label_value_model.message_index:
self.label_value_model.message_index = min_row
@@ -1385,7 +1732,10 @@ def on_table_selection_timer_timeout(self):
for i, tree_item in enumerate(tree_items):
proto = tree_item.protocol
if proto.show and proto in self.rows_for_protocols:
- if any(i in self.rows_for_protocols[proto] for i in range(min_row, max_row + 1)):
+ if any(
+ i in self.rows_for_protocols[proto]
+ for i in range(min_row, max_row + 1)
+ ):
active_group_ids.add(group)
self.selected_protocols.add(proto)
@@ -1394,7 +1744,9 @@ def on_table_selection_timer_timeout(self):
self.active_group_ids.sort()
if message.rssi > 0:
- self.ui.lblRSSI.setText(locale.format_string("%.2f dBm", 10*math.log10(message.rssi)))
+ self.ui.lblRSSI.setText(
+ locale.format_string("%.2f dBm", 10 * math.log10(message.rssi))
+ )
else:
self.ui.lblRSSI.setText("-\u221e dBm")
if isinstance(message.absolute_time, str):
@@ -1408,8 +1760,12 @@ def on_table_selection_timer_timeout(self):
# Set Decoding Combobox
self.ui.cbDecoding.blockSignals(True)
- different_encodings = any(msg.decoder != message.decoder for msg in selected_messages)
- self.ui.cbDecoding.setCurrentText("..." if different_encodings else message.decoder.name)
+ different_encodings = any(
+ msg.decoder != message.decoder for msg in selected_messages
+ )
+ self.ui.cbDecoding.setCurrentText(
+ "..." if different_encodings else message.decoder.name
+ )
self.ui.cbDecoding.blockSignals(False)
self.updateUI(ignore_table_model=True, resize_table=False)
@@ -1419,29 +1775,39 @@ def on_ref_index_changed(self, new_ref_index: int):
if new_ref_index != -1 and self.protocol_model.row_count:
hide_correction = 0
for i in range(0, self.protocol_model.row_count):
- if self.ui.tblViewProtocol.isRowHidden((new_ref_index + i) % self.protocol_model.row_count):
+ if self.ui.tblViewProtocol.isRowHidden(
+ (new_ref_index + i) % self.protocol_model.row_count
+ ):
hide_correction = 0
else:
hide_correction = i
break
- self.protocol_model.refindex = (new_ref_index + hide_correction) % self.protocol_model.row_count
+ self.protocol_model.refindex = (
+ new_ref_index + hide_correction
+ ) % self.protocol_model.row_count
self.set_show_only_status()
@pyqtSlot(QModelIndex, QModelIndex)
- def on_tbl_view_message_current_row_changed(self, current: QModelIndex, previous: QModelIndex):
+ def on_tbl_view_message_current_row_changed(
+ self, current: QModelIndex, previous: QModelIndex
+ ):
row = current.row()
if row == -1:
return
self.active_message_type = self.proto_analyzer.message_types[row]
- self.ui.lblLabelValues.setText("Labels of {}".format(self.active_message_type.name))
+ self.ui.lblLabelValues.setText(
+ "Labels of {}".format(self.active_message_type.name)
+ )
self.label_value_model.show_label_values = False
self.label_value_model.update()
@pyqtSlot(MessageType, list)
- def on_message_type_selected(self, message_type: MessageType, selected_messages: list):
+ def on_message_type_selected(
+ self, message_type: MessageType, selected_messages: list
+ ):
for msg in selected_messages:
msg.message_type = message_type
self.active_message_type = message_type
diff --git a/src/urh/controller/GeneratorTabController.py b/src/urh/controller/GeneratorTabController.py
index e9900d38e2..934f39edd6 100644
--- a/src/urh/controller/GeneratorTabController.py
+++ b/src/urh/controller/GeneratorTabController.py
@@ -15,7 +15,9 @@
from urh.models.GeneratorListModel import GeneratorListModel
from urh.models.GeneratorTableModel import GeneratorTableModel
from urh.models.GeneratorTreeModel import GeneratorTreeModel
-from urh.plugins.NetworkSDRInterface.NetworkSDRInterfacePlugin import NetworkSDRInterfacePlugin
+from urh.plugins.NetworkSDRInterface.NetworkSDRInterfacePlugin import (
+ NetworkSDRInterfacePlugin,
+)
from urh.plugins.PluginManager import PluginManager
from urh.plugins.RfCat.RfCatPlugin import RfCatPlugin
from urh.plugins.FlipperZeroSub.FlipperZeroSubPlugin import FlipperZeroSubPlugin
@@ -35,7 +37,12 @@
class GeneratorTabController(QWidget):
- def __init__(self, compare_frame_controller: CompareFrameController, project_manager: ProjectManager, parent=None):
+ def __init__(
+ self,
+ compare_frame_controller: CompareFrameController,
+ project_manager: ProjectManager,
+ parent=None,
+ ):
super().__init__(parent)
self.ui = Ui_GeneratorTab()
self.ui.setupUi(self)
@@ -45,12 +52,16 @@ def __init__(self, compare_frame_controller: CompareFrameController, project_man
self.ui.treeProtocols.setHeaderHidden(True)
self.tree_model = GeneratorTreeModel(compare_frame_controller)
- self.tree_model.set_root_item(compare_frame_controller.proto_tree_model.rootItem)
+ self.tree_model.set_root_item(
+ compare_frame_controller.proto_tree_model.rootItem
+ )
self.tree_model.controller = self
self.ui.treeProtocols.setModel(self.tree_model)
- self.table_model = GeneratorTableModel(compare_frame_controller.proto_tree_model.rootItem,
- compare_frame_controller.decodings)
+ self.table_model = GeneratorTableModel(
+ compare_frame_controller.proto_tree_model.rootItem,
+ compare_frame_controller.decodings,
+ )
self.table_model.controller = self
self.ui.tableMessages.setModel(self.table_model)
@@ -91,7 +102,9 @@ def selected_message_index(self) -> int:
@property
def selected_message(self) -> Message:
selected_msg_index = self.selected_message_index
- if selected_msg_index == -1 or selected_msg_index >= len(self.table_model.protocol.messages):
+ if selected_msg_index == -1 or selected_msg_index >= len(
+ self.table_model.protocol.messages
+ ):
return None
return self.table_model.protocol.messages[selected_msg_index]
@@ -106,8 +119,14 @@ def modulators(self):
@property
def total_modulated_samples(self) -> int:
- return sum(int(len(msg.encoded_bits) * self.__get_modulator_of_message(msg).samples_per_symbol + msg.pause)
- for msg in self.table_model.protocol.messages)
+ return sum(
+ int(
+ len(msg.encoded_bits)
+ * self.__get_modulator_of_message(msg).samples_per_symbol
+ + msg.pause
+ )
+ for msg in self.table_model.protocol.messages
+ )
@modulators.setter
def modulators(self, value):
@@ -116,19 +135,36 @@ def modulators(self, value):
def create_connects(self, compare_frame_controller):
compare_frame_controller.proto_tree_model.modelReset.connect(self.refresh_tree)
- compare_frame_controller.participant_changed.connect(self.table_model.refresh_vertical_header)
+ compare_frame_controller.participant_changed.connect(
+ self.table_model.refresh_vertical_header
+ )
self.ui.btnEditModulation.clicked.connect(self.show_modulation_dialog)
- self.ui.cBoxModulations.currentIndexChanged.connect(self.on_selected_modulation_changed)
- self.ui.tableMessages.selectionModel().selectionChanged.connect(self.on_table_selection_changed)
+ self.ui.cBoxModulations.currentIndexChanged.connect(
+ self.on_selected_modulation_changed
+ )
+ self.ui.tableMessages.selectionModel().selectionChanged.connect(
+ self.on_table_selection_changed
+ )
self.ui.tableMessages.encodings_updated.connect(self.on_table_selection_changed)
- self.table_model.undo_stack.indexChanged.connect(self.on_undo_stack_index_changed)
- self.table_model.protocol.qt_signals.line_duplicated.connect(self.refresh_pause_list)
- self.table_model.protocol.qt_signals.fuzzing_started.connect(self.on_fuzzing_started)
+ self.table_model.undo_stack.indexChanged.connect(
+ self.on_undo_stack_index_changed
+ )
+ self.table_model.protocol.qt_signals.line_duplicated.connect(
+ self.refresh_pause_list
+ )
+ self.table_model.protocol.qt_signals.fuzzing_started.connect(
+ self.on_fuzzing_started
+ )
self.table_model.protocol.qt_signals.current_fuzzing_message_changed.connect(
- self.on_current_fuzzing_message_changed)
- self.table_model.protocol.qt_signals.fuzzing_finished.connect(self.on_fuzzing_finished)
+ self.on_current_fuzzing_message_changed
+ )
+ self.table_model.protocol.qt_signals.fuzzing_finished.connect(
+ self.on_fuzzing_finished
+ )
self.table_model.first_protocol_added.connect(self.on_first_protocol_added)
- self.label_list_model.protolabel_fuzzing_status_changed.connect(self.set_fuzzing_ui_status)
+ self.label_list_model.protolabel_fuzzing_status_changed.connect(
+ self.set_fuzzing_ui_status
+ )
self.ui.cbViewType.currentIndexChanged.connect(self.on_view_type_changed)
self.ui.btnSend.clicked.connect(self.on_btn_send_clicked)
self.ui.btnSave.clicked.connect(self.on_btn_save_clicked)
@@ -137,29 +173,46 @@ def create_connects(self, compare_frame_controller):
self.project_manager.project_updated.connect(self.on_project_updated)
self.table_model.vertical_header_color_status_changed.connect(
- self.ui.tableMessages.on_vertical_header_color_status_changed)
+ self.ui.tableMessages.on_vertical_header_color_status_changed
+ )
- self.label_list_model.protolabel_removed.connect(self.handle_proto_label_removed)
+ self.label_list_model.protolabel_removed.connect(
+ self.handle_proto_label_removed
+ )
self.ui.lWPauses.item_edit_clicked.connect(self.edit_pause_item)
self.ui.lWPauses.edit_all_items_clicked.connect(self.edit_all_pause_items)
- self.ui.lWPauses.itemSelectionChanged.connect(self.on_lWpauses_selection_changed)
+ self.ui.lWPauses.itemSelectionChanged.connect(
+ self.on_lWpauses_selection_changed
+ )
self.ui.lWPauses.lost_focus.connect(self.on_lWPauses_lost_focus)
self.ui.lWPauses.doubleClicked.connect(self.on_lWPauses_double_clicked)
self.ui.btnGenerate.clicked.connect(self.generate_file)
- self.label_list_model.protolabel_fuzzing_status_changed.connect(self.handle_plabel_fuzzing_state_changed)
+ self.label_list_model.protolabel_fuzzing_status_changed.connect(
+ self.handle_plabel_fuzzing_state_changed
+ )
self.ui.btnFuzz.clicked.connect(self.on_btn_fuzzing_clicked)
self.ui.tableMessages.create_label_triggered.connect(self.create_fuzzing_label)
self.ui.tableMessages.edit_label_triggered.connect(self.show_fuzzing_dialog)
- self.ui.listViewProtoLabels.selection_changed.connect(self.handle_label_selection_changed)
- self.ui.listViewProtoLabels.edit_on_item_triggered.connect(self.show_fuzzing_dialog)
+ self.ui.listViewProtoLabels.selection_changed.connect(
+ self.handle_label_selection_changed
+ )
+ self.ui.listViewProtoLabels.edit_on_item_triggered.connect(
+ self.show_fuzzing_dialog
+ )
self.ui.btnNetworkSDRSend.clicked.connect(self.on_btn_network_sdr_clicked)
self.ui.btnRfCatSend.clicked.connect(self.on_btn_rfcat_clicked)
- self.network_sdr_plugin.sending_status_changed.connect(self.on_network_sdr_sending_status_changed)
- self.network_sdr_plugin.sending_stop_requested.connect(self.on_network_sdr_sending_stop_requested)
- self.network_sdr_plugin.current_send_message_changed.connect(self.on_send_message_changed)
+ self.network_sdr_plugin.sending_status_changed.connect(
+ self.on_network_sdr_sending_status_changed
+ )
+ self.network_sdr_plugin.sending_stop_requested.connect(
+ self.on_network_sdr_sending_stop_requested
+ )
+ self.network_sdr_plugin.current_send_message_changed.connect(
+ self.on_send_message_changed
+ )
# Flipper Zero
self.ui.btnFZSave.clicked.connect(self.on_btn_FZSave_clicked)
@@ -174,7 +227,10 @@ def refresh_tree(self):
def refresh_table(self):
self.table_model.update()
self.ui.tableMessages.resize_columns()
- is_data_there = self.table_model.display_data is not None and len(self.table_model.display_data) > 0
+ is_data_there = (
+ self.table_model.display_data is not None
+ and len(self.table_model.display_data) > 0
+ )
self.ui.btnSend.setEnabled(is_data_there)
self.ui.btnGenerate.setEnabled(is_data_there)
@@ -256,16 +312,27 @@ def prepare_modulation_dialog(self) -> (ModulatorDialog, Message):
selected_message = self.table_model.protocol.messages[min_row]
preselected_index = selected_message.modulator_index
except IndexError:
- selected_message = Message([1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0], 0, [], MessageType("empty"))
+ selected_message = Message(
+ [1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0],
+ 0,
+ [],
+ MessageType("empty"),
+ )
else:
- selected_message = Message([1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0], 0, [], MessageType("empty"))
+ selected_message = Message(
+ [1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0], 0, [], MessageType("empty")
+ )
if len(self.table_model.protocol.messages) > 0:
- selected_message.samples_per_symbol = self.table_model.protocol.messages[0].samples_per_symbol
+ selected_message.samples_per_symbol = (
+ self.table_model.protocol.messages[0].samples_per_symbol
+ )
for m in self.modulators:
m.default_sample_rate = self.project_manager.device_conf["sample_rate"]
- modulator_dialog = ModulatorDialog(self.modulators, tree_model=self.tree_model, parent=self.parent())
+ modulator_dialog = ModulatorDialog(
+ self.modulators, tree_model=self.tree_model, parent=self.parent()
+ )
modulator_dialog.ui.comboBoxCustomModulations.setCurrentIndex(preselected_index)
modulator_dialog.finished.connect(self.refresh_modulators)
@@ -280,7 +347,9 @@ def set_modulation_profile_status(self):
def init_rfcat_plugin(self):
self.set_rfcat_button_visibility()
self.rfcat_plugin = RfCatPlugin()
- self.rfcat_plugin.current_send_message_changed.connect(self.on_send_message_changed)
+ self.rfcat_plugin.current_send_message_changed.connect(
+ self.on_send_message_changed
+ )
self.ui.btnRfCatSend.setEnabled(self.rfcat_plugin.rfcat_is_found)
@pyqtSlot()
@@ -314,7 +383,9 @@ def on_table_selection_changed(self):
self.label_list_model.message = message
decoder_name = message.decoder.name
metrics = QFontMetrics(self.ui.lEncodingValue.font())
- elidedName = metrics.elidedText(decoder_name, Qt.ElideRight, self.ui.lEncodingValue.width())
+ elidedName = metrics.elidedText(
+ decoder_name, Qt.ElideRight, self.ui.lEncodingValue.width()
+ )
self.ui.lEncodingValue.setText(elidedName)
self.ui.lEncodingValue.setToolTip(decoder_name)
self.ui.cBoxModulations.blockSignals(True)
@@ -326,8 +397,13 @@ def on_table_selection_changed(self):
def edit_pause_item(self, index: int):
message = self.table_model.protocol.messages[index]
cur_len = message.pause
- new_len, ok = QInputDialog.getInt(self, self.tr("Enter new Pause Length"),
- self.tr("Pause Length:"), cur_len, 0)
+ new_len, ok = QInputDialog.getInt(
+ self,
+ self.tr("Enter new Pause Length"),
+ self.tr("Pause Length:"),
+ cur_len,
+ 0,
+ )
if ok:
message.pause = new_len
self.refresh_pause_list()
@@ -336,8 +412,13 @@ def edit_pause_item(self, index: int):
def edit_all_pause_items(self):
message = self.table_model.protocol.messages[0]
cur_len = message.pause
- new_len, ok = QInputDialog.getInt(self, self.tr("Enter new Pause Length"),
- self.tr("Pause Length:"), cur_len, 0)
+ new_len, ok = QInputDialog.getInt(
+ self,
+ self.tr("Enter new Pause Length"),
+ self.tr("Pause Length:"),
+ cur_len,
+ 0,
+ )
if ok:
for message in self.table_model.protocol.messages:
message.pause = new_len
@@ -356,8 +437,12 @@ def refresh_pause_list(self):
fmt_str = "Pause ({1:d}-{2:d}) <{0:d} samples ({3})>"
for i, pause in enumerate(self.table_model.protocol.pauses):
- sr = self.__get_modulator_of_message(self.table_model.protocol.messages[i]).sample_rate
- item = fmt_str.format(pause, i + 1, i + 2, Formatter.science_time(pause / sr))
+ sr = self.__get_modulator_of_message(
+ self.table_model.protocol.messages[i]
+ ).sample_rate
+ item = fmt_str.format(
+ pause, i + 1, i + 2, Formatter.science_time(pause / sr)
+ )
self.ui.lWPauses.addItem(item)
self.refresh_estimated_time()
@@ -383,7 +468,10 @@ def generate_file(self):
total_samples = self.total_modulated_samples
buffer = self.prepare_modulation_buffer(total_samples, show_error=False)
if buffer is None:
- Errors.generic_error(self.tr("File too big"), self.tr("This file would get too big to save."))
+ Errors.generic_error(
+ self.tr("File too big"),
+ self.tr("This file would get too big to save."),
+ )
self.unsetCursor()
return
modulated_samples = self.modulate_data(buffer)
@@ -392,7 +480,9 @@ def generate_file(self):
except Exception as e:
logger.exception(e)
sample_rate = 1e6
- FileOperator.ask_signal_file_name_and_save("generated", modulated_samples, sample_rate=sample_rate, parent=self)
+ FileOperator.ask_signal_file_name_and_save(
+ "generated", modulated_samples, sample_rate=sample_rate, parent=self
+ )
except Exception as e:
Errors.exception(e)
self.unsetCursor()
@@ -402,23 +492,27 @@ def prepare_modulation_buffer(self, total_samples: int, show_error=True) -> IQAr
n = 2 if dtype == np.int8 else 4 if dtype == np.int16 else 8
memory_size_for_buffer = total_samples * n
- logger.debug("Allocating {0:.2f}MB for modulated samples".format(memory_size_for_buffer / (1024 ** 2)))
+ logger.debug(
+ "Allocating {0:.2f}MB for modulated samples".format(
+ memory_size_for_buffer / (1024**2)
+ )
+ )
try:
# allocate it three times as we need the same amount for the sending process
- IQArray(None, dtype=dtype, n=3*total_samples)
+ IQArray(None, dtype=dtype, n=3 * total_samples)
except MemoryError:
# will go into continuous mode in this case
if show_error:
- Errors.not_enough_ram_for_sending_precache(3*memory_size_for_buffer)
+ Errors.not_enough_ram_for_sending_precache(3 * memory_size_for_buffer)
return None
return IQArray(None, dtype=dtype, n=total_samples)
def modulate_data(self, buffer: IQArray) -> IQArray:
"""
-
+
:param buffer: Buffer in which the modulated data shall be written, initialized with zeros
- :return:
+ :return:
"""
self.ui.prBarGeneration.show()
self.ui.prBarGeneration.setValue(0)
@@ -431,7 +525,7 @@ def modulate_data(self, buffer: IQArray) -> IQArray:
modulator = self.__get_modulator_of_message(message)
# We do not need to modulate the pause extra, as result is already initialized with zeros
modulated = modulator.modulate(start=0, data=message.encoded_bits, pause=0)
- buffer[pos:pos + len(modulated)] = modulated
+ buffer[pos : pos + len(modulated)] = modulated
pos += len(modulated) + message.pause
self.modulation_msg_indices.append(pos)
self.ui.prBarGeneration.setValue(i + 1)
@@ -445,9 +539,16 @@ def show_fuzzing_dialog(self, label_index: int):
view = self.ui.cbViewType.currentIndex()
if self.label_list_model.message is not None:
- msg_index = self.table_model.protocol.messages.index(self.label_list_model.message)
- fdc = FuzzingDialog(protocol=self.table_model.protocol, label_index=label_index,
- msg_index=msg_index, proto_view=view, parent=self)
+ msg_index = self.table_model.protocol.messages.index(
+ self.label_list_model.message
+ )
+ fdc = FuzzingDialog(
+ protocol=self.table_model.protocol,
+ label_index=label_index,
+ msg_index=msg_index,
+ proto_view=view,
+ parent=self,
+ )
fdc.show()
fdc.finished.connect(self.on_fuzzing_dialog_finished)
@@ -488,7 +589,11 @@ def on_btn_fuzzing_clicked(self):
@pyqtSlot()
def set_fuzzing_ui_status(self):
btn_was_enabled = self.ui.btnFuzz.isEnabled()
- fuzz_active = any(lbl.active_fuzzing for msg in self.table_model.protocol.messages for lbl in msg.message_type)
+ fuzz_active = any(
+ lbl.active_fuzzing
+ for msg in self.table_model.protocol.messages
+ for lbl in msg.message_type
+ )
self.ui.btnFuzz.setEnabled(fuzz_active)
if self.ui.btnFuzz.isEnabled() and not btn_was_enabled:
font = self.ui.btnFuzz.font()
@@ -514,7 +619,14 @@ def refresh_existing_encodings(self, encodings_from_file):
update = False
for msg in self.table_model.protocol.messages:
- i = next((i for i, d in enumerate(encodings_from_file) if d.name == msg.decoder.name), 0)
+ i = next(
+ (
+ i
+ for i, d in enumerate(encodings_from_file)
+ if d.name == msg.decoder.name
+ ),
+ 0,
+ )
if msg.decoder != encodings_from_file[i]:
update = True
msg.decoder = encodings_from_file[i]
@@ -533,18 +645,25 @@ def refresh_estimated_time(self):
return
avg_msg_len = numpy.mean([len(msg.encoded_bits) for msg in c.messages])
- avg_samples_per_symbol = numpy.mean([m.samples_per_symbol for m in self.modulators])
+ avg_samples_per_symbol = numpy.mean(
+ [m.samples_per_symbol for m in self.modulators]
+ )
avg_sample_rate = numpy.mean([m.sample_rate for m in self.modulators])
pause_samples = sum(c.pauses)
nsamples = c.num_messages * avg_msg_len * avg_samples_per_symbol + pause_samples
self.ui.lEstimatedTime.setText(
- locale.format_string("Estimated Time: %.04f seconds", nsamples / avg_sample_rate))
+ locale.format_string(
+ "Estimated Time: %.04f seconds", nsamples / avg_sample_rate
+ )
+ )
@pyqtSlot(int, int, int)
def create_fuzzing_label(self, msg_index: int, start: int, end: int):
con = self.table_model.protocol
- start, end = con.convert_range(start, end - 1, self.ui.cbViewType.currentIndex(), 0, False, msg_index)
+ start, end = con.convert_range(
+ start, end - 1, self.ui.cbViewType.currentIndex(), 0, False, msg_index
+ )
lbl = con.create_fuzzing_label(start, end, msg_index)
self.show_fuzzing_dialog(con.protocol_labels.index(lbl))
@@ -561,8 +680,9 @@ def handle_label_selection_changed(self):
except IndexError:
return
if label.show and self.selected_message:
- start, end = self.selected_message.get_label_range(lbl=label, view=self.table_model.proto_view,
- decode=False)
+ start, end = self.selected_message.get_label_range(
+ lbl=label, view=self.table_model.proto_view, decode=False
+ )
indx = self.table_model.index(0, int((start + end) / 2))
self.ui.tableMessages.scrollTo(indx)
@@ -587,18 +707,31 @@ def on_btn_send_clicked(self):
try:
if modulated_data is not None:
try:
- dialog = SendDialog(self.project_manager, modulated_data=modulated_data,
- modulation_msg_indices=self.modulation_msg_indices, parent=self)
+ dialog = SendDialog(
+ self.project_manager,
+ modulated_data=modulated_data,
+ modulation_msg_indices=self.modulation_msg_indices,
+ parent=self,
+ )
except MemoryError:
# Not enough memory for device buffer so we need to create a continuous send dialog
del modulated_data
Errors.not_enough_ram_for_sending_precache(None)
- dialog = ContinuousSendDialog(self.project_manager,
- self.table_model.protocol.messages,
- self.modulators, total_samples, parent=self)
+ dialog = ContinuousSendDialog(
+ self.project_manager,
+ self.table_model.protocol.messages,
+ self.modulators,
+ total_samples,
+ parent=self,
+ )
else:
- dialog = ContinuousSendDialog(self.project_manager, self.table_model.protocol.messages,
- self.modulators, total_samples, parent=self)
+ dialog = ContinuousSendDialog(
+ self.project_manager,
+ self.table_model.protocol.messages,
+ self.modulators,
+ total_samples,
+ parent=self,
+ )
except OSError as e:
logger.exception(e)
return
@@ -607,7 +740,9 @@ def on_btn_send_clicked(self):
dialog.close()
return
- dialog.device_parameters_changed.connect(self.project_manager.set_device_parameters)
+ dialog.device_parameters_changed.connect(
+ self.project_manager.set_device_parameters
+ )
dialog.show()
dialog.graphics_view.show_full_scene(reinitialize=True)
except Exception as e:
@@ -616,16 +751,22 @@ def on_btn_send_clicked(self):
@pyqtSlot()
def on_btn_save_clicked(self):
- filename = FileOperator.ask_save_file_name("profile.fuzz.xml", caption="Save fuzzing profile")
+ filename = FileOperator.ask_save_file_name(
+ "profile.fuzz.xml", caption="Save fuzzing profile"
+ )
if filename:
- self.table_model.protocol.to_xml_file(filename,
- decoders=self.project_manager.decodings,
- participants=self.project_manager.participants,
- modulators=self.modulators)
+ self.table_model.protocol.to_xml_file(
+ filename,
+ decoders=self.project_manager.decodings,
+ participants=self.project_manager.participants,
+ modulators=self.modulators,
+ )
@pyqtSlot()
def on_btn_open_clicked(self):
- dialog = FileOperator.get_open_dialog(directory_mode=False, parent=self, name_filter="fuzz")
+ dialog = FileOperator.get_open_dialog(
+ directory_mode=False, parent=self, name_filter="fuzz"
+ )
if dialog.exec_():
for filename in dialog.selectedFiles():
self.load_from_file(filename)
@@ -661,15 +802,23 @@ def set_FZSave_button_visibility(self):
@pyqtSlot()
def on_btn_FZSave_clicked(self):
- filename, _ = QFileDialog.getSaveFileName(self, self.tr("Choose file"), directory=FileOperator.RECENT_PATH,
- filter="SUB files (*.sub);;All files (*.*)")
+ filename, _ = QFileDialog.getSaveFileName(
+ self,
+ self.tr("Choose file"),
+ directory=FileOperator.RECENT_PATH,
+ filter="SUB files (*.sub);;All files (*.*)",
+ )
if len(filename) > 0:
if not filename.endswith(".sub"):
- filename = filename+".sub"
+ filename = filename + ".sub"
messages = self.table_model.protocol.messages
- sample_rates = [self.__get_modulator_of_message(msg).sample_rate for msg in messages]
- self.flipperzerosub_plugin.write_sub_file(filename, messages, sample_rates, self.modulators, self.project_manager)
+ sample_rates = [
+ self.__get_modulator_of_message(msg).sample_rate for msg in messages
+ ]
+ self.flipperzerosub_plugin.write_sub_file(
+ filename, messages, sample_rates, self.modulators, self.project_manager
+ )
else:
logger.debug("Filename was empty!")
@@ -677,7 +826,9 @@ def on_btn_FZSave_clicked(self):
def on_btn_network_sdr_clicked(self):
if not self.network_sdr_plugin.is_sending:
messages = self.table_model.protocol.messages
- sample_rates = [self.__get_modulator_of_message(msg).sample_rate for msg in messages]
+ sample_rates = [
+ self.__get_modulator_of_message(msg).sample_rate for msg in messages
+ ]
self.network_sdr_plugin.start_message_sending_thread(messages, sample_rates)
else:
self.network_sdr_plugin.stop_sending_thread()
@@ -687,7 +838,10 @@ def on_network_sdr_sending_status_changed(self, is_sending: bool):
self.ui.btnNetworkSDRSend.setChecked(is_sending)
self.ui.btnNetworkSDRSend.setEnabled(True)
self.ui.btnNetworkSDRSend.setToolTip(
- "Sending in progress" if is_sending else self.network_sdr_button_orig_tooltip)
+ "Sending in progress"
+ if is_sending
+ else self.network_sdr_button_orig_tooltip
+ )
if not is_sending:
self.ui.tableMessages.clearSelection()
@@ -704,9 +858,12 @@ def on_send_message_changed(self, message_index: int):
def on_btn_rfcat_clicked(self):
if not self.rfcat_plugin.is_sending:
messages = self.table_model.protocol.messages
- sample_rates = [self.__get_modulator_of_message(msg).sample_rate for msg in messages]
- self.rfcat_plugin.start_message_sending_thread(messages, sample_rates, self.modulators,
- self.project_manager)
+ sample_rates = [
+ self.__get_modulator_of_message(msg).sample_rate for msg in messages
+ ]
+ self.rfcat_plugin.start_message_sending_thread(
+ messages, sample_rates, self.modulators, self.project_manager
+ )
else:
self.rfcat_plugin.stop_sending_thread()
diff --git a/src/urh/controller/MainController.py b/src/urh/controller/MainController.py
index e1ff624ba4..01b302bbdf 100644
--- a/src/urh/controller/MainController.py
+++ b/src/urh/controller/MainController.py
@@ -3,7 +3,16 @@
from PyQt5.QtCore import QDir, Qt, pyqtSlot, QTimer
from PyQt5.QtGui import QIcon, QCloseEvent, QKeySequence
-from PyQt5.QtWidgets import QMainWindow, QUndoGroup, QActionGroup, QHeaderView, QAction, QMessageBox, QApplication, qApp
+from PyQt5.QtWidgets import (
+ QMainWindow,
+ QUndoGroup,
+ QActionGroup,
+ QHeaderView,
+ QAction,
+ QMessageBox,
+ QApplication,
+ qApp,
+)
from urh import settings, version
from urh.controller.CompareFrameController import CompareFrameController
@@ -45,24 +54,31 @@ def __init__(self, *args):
self.project_save_timer = QTimer()
self.project_manager = ProjectManager(self)
self.plugin_manager = PluginManager()
- self.signal_tab_controller = SignalTabController(self.project_manager,
- parent=self.ui.tab_interpretation)
+ self.signal_tab_controller = SignalTabController(
+ self.project_manager, parent=self.ui.tab_interpretation
+ )
self.ui.tab_interpretation.layout().addWidget(self.signal_tab_controller)
- self.compare_frame_controller = CompareFrameController(parent=self.ui.tab_protocol,
- plugin_manager=self.plugin_manager,
- project_manager=self.project_manager)
+ self.compare_frame_controller = CompareFrameController(
+ parent=self.ui.tab_protocol,
+ plugin_manager=self.plugin_manager,
+ project_manager=self.project_manager,
+ )
self.compare_frame_controller.ui.splitter.setSizes([1, 1000000])
self.ui.tab_protocol.layout().addWidget(self.compare_frame_controller)
- self.generator_tab_controller = GeneratorTabController(self.compare_frame_controller,
- self.project_manager,
- parent=self.ui.tab_generator)
+ self.generator_tab_controller = GeneratorTabController(
+ self.compare_frame_controller,
+ self.project_manager,
+ parent=self.ui.tab_generator,
+ )
- self.simulator_tab_controller = SimulatorTabController(parent=self.ui.tab_simulator,
- compare_frame_controller=self.compare_frame_controller,
- generator_tab_controller=self.generator_tab_controller,
- project_manager=self.project_manager)
+ self.simulator_tab_controller = SimulatorTabController(
+ parent=self.ui.tab_simulator,
+ compare_frame_controller=self.compare_frame_controller,
+ generator_tab_controller=self.generator_tab_controller,
+ project_manager=self.project_manager,
+ )
self.ui.tab_simulator.layout().addWidget(self.simulator_tab_controller)
@@ -73,15 +89,21 @@ def __init__(self, *args):
self.undo_group.setActiveStack(self.signal_tab_controller.signal_undo_stack)
self.cancel_action = QAction(self.tr("Cancel"), self)
- self.cancel_action.setShortcut(QKeySequence.Cancel if hasattr(QKeySequence, "Cancel") else "Esc")
+ self.cancel_action.setShortcut(
+ QKeySequence.Cancel if hasattr(QKeySequence, "Cancel") else "Esc"
+ )
self.cancel_action.triggered.connect(self.on_cancel_triggered)
self.cancel_action.setShortcutContext(Qt.WidgetWithChildrenShortcut)
self.cancel_action.setIcon(QIcon.fromTheme("dialog-cancel"))
self.addAction(self.cancel_action)
- self.ui.actionAuto_detect_new_signals.setChecked(settings.read("auto_detect_new_signals", True, bool))
+ self.ui.actionAuto_detect_new_signals.setChecked(
+ settings.read("auto_detect_new_signals", True, bool)
+ )
- self.participant_legend_model = ParticipantLegendListModel(self.project_manager.participants)
+ self.participant_legend_model = ParticipantLegendListModel(
+ self.project_manager.participants
+ )
self.ui.listViewParticipants.setModel(self.participant_legend_model)
gtc = self.generator_tab_controller
@@ -102,7 +124,9 @@ def __init__(self, *args):
noise_threshold_setting = settings.read("default_noise_threshold", "automatic")
noise_threshold_group = QActionGroup(self)
self.ui.actionAutomaticNoiseThreshold.setActionGroup(noise_threshold_group)
- self.ui.actionAutomaticNoiseThreshold.setChecked(noise_threshold_setting == "automatic")
+ self.ui.actionAutomaticNoiseThreshold.setChecked(
+ noise_threshold_setting == "automatic"
+ )
self.ui.action1NoiseThreshold.setActionGroup(noise_threshold_group)
self.ui.action1NoiseThreshold.setChecked(noise_threshold_setting == "1")
self.ui.action5NoiseThreshold.setActionGroup(noise_threshold_group)
@@ -125,7 +149,9 @@ def __init__(self, *args):
self.file_proxy_model.setSourceModel(self.filemodel)
self.ui.fileTree.setModel(self.file_proxy_model)
- self.ui.fileTree.setRootIndex(self.file_proxy_model.mapFromSource(self.filemodel.index(path)))
+ self.ui.fileTree.setRootIndex(
+ self.file_proxy_model.mapFromSource(self.filemodel.index(path))
+ )
self.ui.fileTree.setToolTip(path)
self.ui.fileTree.header().setSectionResizeMode(0, QHeaderView.ResizeToContents)
self.ui.fileTree.header().setSectionResizeMode(1, QHeaderView.Stretch)
@@ -146,24 +172,33 @@ def __init__(self, *args):
self.ui.menuEdit.insertAction(self.ui.actionDecoding, redo_action)
self.ui.menuEdit.insertSeparator(self.ui.actionDecoding)
- self.ui.actionAbout_Qt.setIcon(QIcon(":/qt-project.org/qmessagebox/images/qtlogo-64.png"))
+ self.ui.actionAbout_Qt.setIcon(
+ QIcon(":/qt-project.org/qmessagebox/images/qtlogo-64.png")
+ )
self.__set_non_project_warning_visibility()
self.ui.splitter.setSizes([0, 1])
self.refresh_main_menu()
- self.apply_default_view(settings.read('default_view', type=int))
- self.project_save_timer.start(ProjectManager.AUTOSAVE_INTERVAL_MINUTES * 60 * 1000)
+ self.apply_default_view(settings.read("default_view", type=int))
+ self.project_save_timer.start(
+ ProjectManager.AUTOSAVE_INTERVAL_MINUTES * 60 * 1000
+ )
self.ui.actionProject_settings.setVisible(False)
self.ui.actionSave_project.setVisible(False)
self.ui.actionClose_project.setVisible(False)
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
def __set_non_project_warning_visibility(self):
- show = settings.read("show_non_project_warning", True, bool) and not self.project_manager.project_loaded
+ show = (
+ settings.read("show_non_project_warning", True, bool)
+ and not self.project_manager.project_loaded
+ )
self.ui.labelNonProjectMode.setVisible(show)
def create_connects(self):
@@ -173,78 +208,149 @@ def create_connects(self):
self.ui.menuEdit.aboutToShow.connect(self.on_edit_menu_about_to_show)
- self.ui.actionNew_Project.triggered.connect(self.on_new_project_action_triggered)
+ self.ui.actionNew_Project.triggered.connect(
+ self.on_new_project_action_triggered
+ )
self.ui.actionNew_Project.setShortcut(QKeySequence.New)
- self.ui.actionProject_settings.triggered.connect(self.on_project_settings_action_triggered)
+ self.ui.actionProject_settings.triggered.connect(
+ self.on_project_settings_action_triggered
+ )
self.ui.actionSave_project.triggered.connect(self.save_project)
self.ui.actionClose_project.triggered.connect(self.close_project)
- self.ui.actionAbout_AutomaticHacker.triggered.connect(self.on_show_about_clicked)
- self.ui.actionRecord.triggered.connect(self.on_show_record_dialog_action_triggered)
-
- self.ui.actionFullscreen_mode.triggered.connect(self.on_fullscreen_action_triggered)
- self.ui.actionSaveAllSignals.triggered.connect(self.signal_tab_controller.save_all)
- self.ui.actionCloseAllFiles.triggered.connect(self.on_close_all_files_action_triggered)
+ self.ui.actionAbout_AutomaticHacker.triggered.connect(
+ self.on_show_about_clicked
+ )
+ self.ui.actionRecord.triggered.connect(
+ self.on_show_record_dialog_action_triggered
+ )
+
+ self.ui.actionFullscreen_mode.triggered.connect(
+ self.on_fullscreen_action_triggered
+ )
+ self.ui.actionSaveAllSignals.triggered.connect(
+ self.signal_tab_controller.save_all
+ )
+ self.ui.actionCloseAllFiles.triggered.connect(
+ self.on_close_all_files_action_triggered
+ )
self.ui.actionOpen.triggered.connect(self.on_open_file_action_triggered)
- self.ui.actionOpen_directory.triggered.connect(self.on_open_directory_action_triggered)
+ self.ui.actionOpen_directory.triggered.connect(
+ self.on_open_directory_action_triggered
+ )
self.ui.actionDecoding.triggered.connect(self.on_show_decoding_dialog_triggered)
- self.ui.actionSpectrum_Analyzer.triggered.connect(self.on_show_spectrum_dialog_action_triggered)
- self.ui.actionOptions.triggered.connect(self.show_options_dialog_action_triggered)
+ self.ui.actionSpectrum_Analyzer.triggered.connect(
+ self.on_show_spectrum_dialog_action_triggered
+ )
+ self.ui.actionOptions.triggered.connect(
+ self.show_options_dialog_action_triggered
+ )
self.ui.actionSniff_protocol.triggered.connect(self.show_proto_sniff_dialog)
self.ui.actionAbout_Qt.triggered.connect(QApplication.instance().aboutQt)
- self.ui.actionSamples_from_csv.triggered.connect(self.on_import_samples_from_csv_action_triggered)
- self.ui.actionAuto_detect_new_signals.triggered.connect(self.on_auto_detect_new_signals_action_triggered)
-
- self.ui.actionAutomaticNoiseThreshold.triggered.connect(self.on_action_automatic_noise_threshold_triggered)
- self.ui.action1NoiseThreshold.triggered.connect(self.on_action_1_noise_threshold_triggered)
- self.ui.action5NoiseThreshold.triggered.connect(self.on_action_5_noise_threshold_triggered)
- self.ui.action10NoiseThreshold.triggered.connect(self.on_action_10_noise_threshold_triggered)
- self.ui.action100NoiseThreshold.triggered.connect(self.on_action_100_noise_threshold_triggered)
+ self.ui.actionSamples_from_csv.triggered.connect(
+ self.on_import_samples_from_csv_action_triggered
+ )
+ self.ui.actionAuto_detect_new_signals.triggered.connect(
+ self.on_auto_detect_new_signals_action_triggered
+ )
+
+ self.ui.actionAutomaticNoiseThreshold.triggered.connect(
+ self.on_action_automatic_noise_threshold_triggered
+ )
+ self.ui.action1NoiseThreshold.triggered.connect(
+ self.on_action_1_noise_threshold_triggered
+ )
+ self.ui.action5NoiseThreshold.triggered.connect(
+ self.on_action_5_noise_threshold_triggered
+ )
+ self.ui.action10NoiseThreshold.triggered.connect(
+ self.on_action_10_noise_threshold_triggered
+ )
+ self.ui.action100NoiseThreshold.triggered.connect(
+ self.on_action_100_noise_threshold_triggered
+ )
self.ui.btnFileTreeGoUp.clicked.connect(self.on_btn_file_tree_go_up_clicked)
- self.ui.fileTree.directory_open_wanted.connect(self.project_manager.set_project_folder)
+ self.ui.fileTree.directory_open_wanted.connect(
+ self.project_manager.set_project_folder
+ )
self.signal_tab_controller.frame_closed.connect(self.close_signal_frame)
self.signal_tab_controller.signal_created.connect(self.on_signal_created)
- self.signal_tab_controller.ui.scrollArea.files_dropped.connect(self.on_files_dropped)
+ self.signal_tab_controller.ui.scrollArea.files_dropped.connect(
+ self.on_files_dropped
+ )
self.signal_tab_controller.files_dropped.connect(self.on_files_dropped)
self.signal_tab_controller.frame_was_dropped.connect(self.set_frame_numbers)
- self.simulator_tab_controller.open_in_analysis_requested.connect(self.on_simulator_open_in_analysis_requested)
- self.simulator_tab_controller.rx_file_saved.connect(self.adjust_for_current_file)
+ self.simulator_tab_controller.open_in_analysis_requested.connect(
+ self.on_simulator_open_in_analysis_requested
+ )
+ self.simulator_tab_controller.rx_file_saved.connect(
+ self.adjust_for_current_file
+ )
self.compare_frame_controller.show_interpretation_clicked.connect(
- self.show_protocol_selection_in_interpretation)
+ self.show_protocol_selection_in_interpretation
+ )
self.compare_frame_controller.files_dropped.connect(self.on_files_dropped)
- self.compare_frame_controller.show_decoding_clicked.connect(self.on_show_decoding_dialog_triggered)
+ self.compare_frame_controller.show_decoding_clicked.connect(
+ self.on_show_decoding_dialog_triggered
+ )
self.compare_frame_controller.ui.treeViewProtocols.files_dropped_on_group.connect(
- self.on_files_dropped_on_group)
- self.compare_frame_controller.participant_changed.connect(self.signal_tab_controller.on_participant_changed)
- self.compare_frame_controller.ui.treeViewProtocols.close_wanted.connect(self.on_cfc_close_wanted)
+ self.on_files_dropped_on_group
+ )
+ self.compare_frame_controller.participant_changed.connect(
+ self.signal_tab_controller.on_participant_changed
+ )
+ self.compare_frame_controller.ui.treeViewProtocols.close_wanted.connect(
+ self.on_cfc_close_wanted
+ )
self.compare_frame_controller.show_config_field_types_triggered.connect(
- self.on_show_field_types_config_action_triggered)
+ self.on_show_field_types_config_action_triggered
+ )
- self.compare_frame_controller.load_protocol_clicked.connect(self.on_compare_frame_controller_load_protocol_clicked)
- self.compare_frame_controller.ui.listViewParticipants.doubleClicked.connect(self.on_project_settings_action_triggered)
+ self.compare_frame_controller.load_protocol_clicked.connect(
+ self.on_compare_frame_controller_load_protocol_clicked
+ )
+ self.compare_frame_controller.ui.listViewParticipants.doubleClicked.connect(
+ self.on_project_settings_action_triggered
+ )
- self.ui.lnEdtTreeFilter.textChanged.connect(self.on_file_tree_filter_text_changed)
+ self.ui.lnEdtTreeFilter.textChanged.connect(
+ self.on_file_tree_filter_text_changed
+ )
self.ui.tabWidget.currentChanged.connect(self.on_selected_tab_changed)
self.project_save_timer.timeout.connect(self.save_project)
- self.ui.actionConvert_Folder_to_Project.triggered.connect(self.project_manager.convert_folder_to_project)
- self.project_manager.project_loaded_status_changed.connect(self.on_project_loaded_status_changed)
+ self.ui.actionConvert_Folder_to_Project.triggered.connect(
+ self.project_manager.convert_folder_to_project
+ )
+ self.project_manager.project_loaded_status_changed.connect(
+ self.on_project_loaded_status_changed
+ )
self.project_manager.project_updated.connect(self.on_project_updated)
- self.ui.textEditProjectDescription.textChanged.connect(self.on_text_edit_project_description_text_changed)
- self.ui.tabWidget_Project.tabBarDoubleClicked.connect(self.on_project_tab_bar_double_clicked)
+ self.ui.textEditProjectDescription.textChanged.connect(
+ self.on_text_edit_project_description_text_changed
+ )
+ self.ui.tabWidget_Project.tabBarDoubleClicked.connect(
+ self.on_project_tab_bar_double_clicked
+ )
- self.ui.listViewParticipants.doubleClicked.connect(self.on_project_settings_action_triggered)
+ self.ui.listViewParticipants.doubleClicked.connect(
+ self.on_project_settings_action_triggered
+ )
- self.ui.actionShowFileTree.triggered.connect(self.on_action_show_filetree_triggered)
+ self.ui.actionShowFileTree.triggered.connect(
+ self.on_action_show_filetree_triggered
+ )
self.ui.actionShowFileTree.setShortcut(QKeySequence("F10"))
- self.ui.labelNonProjectMode.linkActivated.connect(self.on_label_non_project_mode_link_activated)
+ self.ui.labelNonProjectMode.linkActivated.connect(
+ self.on_label_non_project_mode_link_activated
+ )
self.ui.menuFile.addSeparator()
for i in range(settings.MAX_RECENT_FILE_NR):
@@ -287,9 +393,13 @@ def add_simulator_profile(self, filename):
def add_signalfile(self, filename: str, group_id=0, enforce_sample_rate=None):
if not os.path.exists(filename):
- QMessageBox.critical(self, self.tr("File not Found"),
- self.tr("The file {0} could not be found. Was it moved or renamed?").format(
- filename))
+ QMessageBox.critical(
+ self,
+ self.tr("File not Found"),
+ self.tr(
+ "The file {0} could not be found. Was it moved or renamed?"
+ ).format(filename),
+ )
return
sig_name = os.path.splitext(os.path.basename(filename))[0]
@@ -315,7 +425,11 @@ def add_signal(self, signal, group_id=0, index=-1):
signal.blockSignals(True)
has_entry = self.project_manager.read_project_file_for_signal(signal)
- if self.ui.actionAuto_detect_new_signals.isChecked() and not has_entry and not signal.changed:
+ if (
+ self.ui.actionAuto_detect_new_signals.isChecked()
+ and not has_entry
+ and not signal.changed
+ ):
sig_frame.ui.stackedWidget.setCurrentWidget(sig_frame.ui.pageLoading)
qApp.processEvents()
if not signal.already_demodulated:
@@ -351,7 +465,9 @@ def close_protocol(self, protocol):
def close_signal_frame(self, signal_frame: SignalFrame):
try:
- self.project_manager.write_signal_information_to_project_file(signal_frame.signal)
+ self.project_manager.write_signal_information_to_project_file(
+ signal_frame.signal
+ )
try:
proto = self.signal_protocol_dict[signal_frame]
except KeyError:
@@ -361,9 +477,14 @@ def close_signal_frame(self, signal_frame: SignalFrame):
self.close_protocol(proto)
del self.signal_protocol_dict[signal_frame]
- if self.signal_tab_controller.ui.scrlAreaSignals.minimumHeight() > signal_frame.height():
+ if (
+ self.signal_tab_controller.ui.scrlAreaSignals.minimumHeight()
+ > signal_frame.height()
+ ):
self.signal_tab_controller.ui.scrlAreaSignals.setMinimumHeight(
- self.signal_tab_controller.ui.scrlAreaSignals.minimumHeight() - signal_frame.height())
+ self.signal_tab_controller.ui.scrlAreaSignals.minimumHeight()
+ - signal_frame.height()
+ )
if signal_frame.signal is not None:
# Non-Empty Frame (when a signal and not a protocol is opened)
@@ -398,20 +519,35 @@ def add_files(self, filepaths, group_id=0, enforce_sample_rate=None):
FileOperator.RECENT_PATH = os.path.split(filename)[0]
if filename.endswith(".complex"):
- self.add_signalfile(filename, group_id, enforce_sample_rate=enforce_sample_rate)
+ self.add_signalfile(
+ filename, group_id, enforce_sample_rate=enforce_sample_rate
+ )
elif filename.endswith(".coco"):
- self.add_signalfile(filename, group_id, enforce_sample_rate=enforce_sample_rate)
- elif filename.endswith(".proto") or filename.endswith(".proto.xml") or filename.endswith(".bin"):
+ self.add_signalfile(
+ filename, group_id, enforce_sample_rate=enforce_sample_rate
+ )
+ elif (
+ filename.endswith(".proto")
+ or filename.endswith(".proto.xml")
+ or filename.endswith(".bin")
+ ):
self.add_protocol_file(filename)
elif filename.endswith(".wav"):
try:
import wave
+
w = wave.open(filename)
w.close()
except wave.Error as e:
- Errors.generic_error("Unsupported WAV type", "Only uncompressed WAVs (PCM) are supported.", str(e))
+ Errors.generic_error(
+ "Unsupported WAV type",
+ "Only uncompressed WAVs (PCM) are supported.",
+ str(e),
+ )
continue
- self.add_signalfile(filename, group_id, enforce_sample_rate=enforce_sample_rate)
+ self.add_signalfile(
+ filename, group_id, enforce_sample_rate=enforce_sample_rate
+ )
elif filename.endswith(".fuzz") or filename.endswith(".fuzz.xml"):
self.add_fuzz_profile(filename)
elif filename.endswith(".sim") or filename.endswith(".sim.xml"):
@@ -424,7 +560,9 @@ def add_files(self, filepaths, group_id=0, enforce_sample_rate=None):
elif os.path.basename(filename) == settings.PROJECT_FILE:
self.project_manager.set_project_folder(os.path.split(filename)[0])
else:
- self.add_signalfile(filename, group_id, enforce_sample_rate=enforce_sample_rate)
+ self.add_signalfile(
+ filename, group_id, enforce_sample_rate=enforce_sample_rate
+ )
if self.project_manager.project_file is None:
self.adjust_for_current_file(filename)
@@ -436,7 +574,9 @@ def set_frame_numbers(self):
def closeEvent(self, event: QCloseEvent):
self.save_project()
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
def close_all_files(self):
@@ -472,14 +612,18 @@ def apply_default_view(self, view_index: int):
sig_frame.ui.cbProtoView.setCurrentIndex(view_index)
def show_project_settings(self):
- pdc = ProjectDialog(new_project=False, project_manager=self.project_manager, parent=self)
+ pdc = ProjectDialog(
+ new_project=False, project_manager=self.project_manager, parent=self
+ )
pdc.finished.connect(self.on_project_dialog_finished)
pdc.show()
def collapse_project_tab_bar(self):
self.ui.tabParticipants.hide()
self.ui.tabDescription.hide()
- self.ui.tabWidget_Project.setMaximumHeight(self.ui.tabWidget_Project.tabBar().height())
+ self.ui.tabWidget_Project.setMaximumHeight(
+ self.ui.tabWidget_Project.tabBar().height()
+ )
def expand_project_tab_bar(self):
self.ui.tabDescription.show()
@@ -487,7 +631,9 @@ def expand_project_tab_bar(self):
self.ui.tabWidget_Project.setMaximumHeight(9000)
def save_project(self):
- self.project_manager.save_project(simulator_config=self.simulator_tab_controller.simulator_config)
+ self.project_manager.save_project(
+ simulator_config=self.simulator_tab_controller.simulator_config
+ )
def close_project(self):
self.save_project()
@@ -499,7 +645,9 @@ def close_project(self):
self.participant_legend_model.update()
self.filemodel.setRootPath(QDir.homePath())
- self.ui.fileTree.setRootIndex(self.file_proxy_model.mapFromSource(self.filemodel.index(QDir.homePath())))
+ self.ui.fileTree.setRootIndex(
+ self.file_proxy_model.mapFromSource(self.filemodel.index(QDir.homePath()))
+ )
self.hide_file_tree()
self.project_manager.project_path = ""
@@ -533,10 +681,16 @@ def adjust_for_current_file(self, file_path):
file_path = copy.copy(FileOperator.archives[file_path])
recent_file_paths = settings.read("recentFiles", [], list)
- recent_file_paths = [] if recent_file_paths is None else recent_file_paths # check None for OSX
- recent_file_paths = [p for p in recent_file_paths if p != file_path and p is not None and os.path.exists(p)]
+ recent_file_paths = (
+ [] if recent_file_paths is None else recent_file_paths
+ ) # check None for OSX
+ recent_file_paths = [
+ p
+ for p in recent_file_paths
+ if p != file_path and p is not None and os.path.exists(p)
+ ]
recent_file_paths.insert(0, file_path)
- recent_file_paths = recent_file_paths[:settings.MAX_RECENT_FILE_NR]
+ recent_file_paths = recent_file_paths[: settings.MAX_RECENT_FILE_NR]
self.init_recent_file_action_list(recent_file_paths)
@@ -580,7 +734,9 @@ def on_open_recent_action_triggered(self):
self.project_manager.set_project_folder(action.data())
elif os.path.isfile(action.data()):
self.setCursor(Qt.WaitCursor)
- self.add_files(FileOperator.uncompress_archives([action.data()], QDir.tempPath()))
+ self.add_files(
+ FileOperator.uncompress_archives([action.data()], QDir.tempPath())
+ )
self.unsetCursor()
except Exception as e:
Errors.exception(e)
@@ -588,17 +744,21 @@ def on_open_recent_action_triggered(self):
@pyqtSlot()
def on_show_about_clicked(self):
- descr = "Universal Radio Hacker
Version: {0}
" \
- "GitHub: https://github.com/jopohl/urh
" \
- "Creators:
".format(version.VERSION)
+ descr = (
+ "Universal Radio Hacker
Version: {0}
"
+ "GitHub: https://github.com/jopohl/urh
"
+ "Creators:
".format(version.VERSION)
+ )
QMessageBox.about(self, self.tr("About"), self.tr(descr))
@pyqtSlot(int, int, int, int)
- def show_protocol_selection_in_interpretation(self, start_message, start, end_message, end):
+ def show_protocol_selection_in_interpretation(
+ self, start_message, start, end_message, end
+ ):
try:
cfc = self.compare_frame_controller
msg_total = 0
@@ -608,20 +768,37 @@ def show_protocol_selection_in_interpretation(self, start_message, start, end_me
continue
n = protocol.num_messages
view_type = cfc.ui.cbProtoView.currentIndex()
- messages = [i - msg_total for i in range(msg_total, msg_total + n) if start_message <= i <= end_message]
+ messages = [
+ i - msg_total
+ for i in range(msg_total, msg_total + n)
+ if start_message <= i <= end_message
+ ]
if len(messages) > 0:
try:
- signal_frame = next((sf for sf, pf in self.signal_protocol_dict.items() if pf == protocol))
+ signal_frame = next(
+ (
+ sf
+ for sf, pf in self.signal_protocol_dict.items()
+ if pf == protocol
+ )
+ )
except StopIteration:
- QMessageBox.critical(self, self.tr("Error"),
- self.tr("Could not find corresponding signal frame."))
+ QMessageBox.critical(
+ self,
+ self.tr("Error"),
+ self.tr("Could not find corresponding signal frame."),
+ )
return
- signal_frame.set_roi_from_protocol_analysis(min(messages), start, max(messages), end + 1, view_type)
+ signal_frame.set_roi_from_protocol_analysis(
+ min(messages), start, max(messages), end + 1, view_type
+ )
last_sig_frame = signal_frame
msg_total += n
focus_frame = last_sig_frame
if last_sig_frame is not None:
- self.signal_tab_controller.ui.scrollArea.ensureWidgetVisible(last_sig_frame, 0, 0)
+ self.signal_tab_controller.ui.scrollArea.ensureWidgetVisible(
+ last_sig_frame, 0, 0
+ )
QApplication.instance().processEvents()
self.ui.tabWidget.setCurrentIndex(0)
@@ -641,8 +818,11 @@ def on_file_tree_filter_text_changed(self, text: str):
def on_show_decoding_dialog_triggered(self):
signals = [sf.signal for sf in self.signal_tab_controller.signal_frames]
decoding_controller = DecoderDialog(
- self.compare_frame_controller.decodings, signals,
- self.project_manager, parent=self)
+ self.compare_frame_controller.decodings,
+ signals,
+ self.project_manager,
+ parent=self,
+ )
decoding_controller.finished.connect(self.update_decodings)
decoding_controller.show()
decoding_controller.decoder_update()
@@ -653,32 +833,46 @@ def update_decodings(self):
self.compare_frame_controller.fill_decoding_combobox()
self.compare_frame_controller.refresh_existing_encodings()
- self.generator_tab_controller.refresh_existing_encodings(self.compare_frame_controller.decodings)
+ self.generator_tab_controller.refresh_existing_encodings(
+ self.compare_frame_controller.decodings
+ )
@pyqtSlot(int)
def on_selected_tab_changed(self, index: int):
if index == 0:
self.undo_group.setActiveStack(self.signal_tab_controller.signal_undo_stack)
elif index == 1:
- self.undo_group.setActiveStack(self.compare_frame_controller.protocol_undo_stack)
+ self.undo_group.setActiveStack(
+ self.compare_frame_controller.protocol_undo_stack
+ )
self.compare_frame_controller.ui.tblViewProtocol.resize_columns()
self.compare_frame_controller.ui.tblViewProtocol.resize_vertical_header()
- h = max(self.compare_frame_controller.ui.btnSaveProto.height(),
- self.generator_tab_controller.ui.btnSave.height())
+ h = max(
+ self.compare_frame_controller.ui.btnSaveProto.height(),
+ self.generator_tab_controller.ui.btnSave.height(),
+ )
self.compare_frame_controller.ui.btnSaveProto.setMinimumHeight(h)
th = self.compare_frame_controller.ui.tabWidget.tabBar().height()
for i in range(self.compare_frame_controller.ui.tabWidget.count()):
- self.compare_frame_controller.ui.tabWidget.widget(i).layout().setContentsMargins(0, 7 + h - th, 0, 0)
+ self.compare_frame_controller.ui.tabWidget.widget(
+ i
+ ).layout().setContentsMargins(0, 7 + h - th, 0, 0)
elif index == 2:
- self.undo_group.setActiveStack(self.generator_tab_controller.generator_undo_stack)
- h = max(self.compare_frame_controller.ui.btnSaveProto.height(),
- self.generator_tab_controller.ui.btnSave.height())
+ self.undo_group.setActiveStack(
+ self.generator_tab_controller.generator_undo_stack
+ )
+ h = max(
+ self.compare_frame_controller.ui.btnSaveProto.height(),
+ self.generator_tab_controller.ui.btnSave.height(),
+ )
self.generator_tab_controller.ui.btnSave.setMinimumHeight(h)
th = self.generator_tab_controller.ui.tabWidget.tabBar().height()
for i in range(self.generator_tab_controller.ui.tabWidget.count()):
- self.generator_tab_controller.ui.tabWidget.widget(i).layout().setContentsMargins(0, 7 + h - th, 0, 0)
+ self.generator_tab_controller.ui.tabWidget.widget(
+ i
+ ).layout().setContentsMargins(0, 7 + h - th, 0, 0)
# Modulators may got changed from Simulator Dialog
self.generator_tab_controller.refresh_modulators()
# Signals may got reordered in analysis
@@ -705,11 +899,21 @@ def on_show_record_dialog_action_triggered(self):
def create_protocol_sniff_dialog(self, testing_mode=False):
pm = self.project_manager
- signal = next((proto.signal for proto in self.compare_frame_controller.protocol_list), None)
- signals = [f.signal for f in self.signal_tab_controller.signal_frames if f.signal]
-
- psd = ProtocolSniffDialog(project_manager=pm, signal=signal, signals=signals,
- testing_mode=testing_mode, parent=self)
+ signal = next(
+ (proto.signal for proto in self.compare_frame_controller.protocol_list),
+ None,
+ )
+ signals = [
+ f.signal for f in self.signal_tab_controller.signal_frames if f.signal
+ ]
+
+ psd = ProtocolSniffDialog(
+ project_manager=pm,
+ signal=signal,
+ signals=signals,
+ testing_mode=testing_mode,
+ parent=self,
+ )
if psd.has_empty_device_list:
Errors.no_device()
@@ -717,7 +921,9 @@ def create_protocol_sniff_dialog(self, testing_mode=False):
return None
else:
psd.device_parameters_changed.connect(pm.set_device_parameters)
- psd.protocol_accepted.connect(self.compare_frame_controller.add_sniffed_protocol_messages)
+ psd.protocol_accepted.connect(
+ self.compare_frame_controller.add_sniffed_protocol_messages
+ )
return psd
@pyqtSlot()
@@ -791,7 +997,9 @@ def on_open_directory_action_triggered(self):
self.show_open_dialog(directory=True)
def show_open_dialog(self, directory=False):
- dialog = FileOperator.get_open_dialog(directory_mode=directory, parent=self, name_filter="full")
+ dialog = FileOperator.get_open_dialog(
+ directory_mode=directory, parent=self, name_filter="full"
+ )
if dialog.exec_():
try:
file_names = dialog.selectedFiles()
@@ -805,7 +1013,9 @@ def show_open_dialog(self, directory=False):
self.project_manager.set_project_folder(folder)
else:
self.setCursor(Qt.WaitCursor)
- file_names = FileOperator.uncompress_archives(file_names, QDir.tempPath())
+ file_names = FileOperator.uncompress_archives(
+ file_names, QDir.tempPath()
+ )
self.add_files(file_names)
self.unsetCursor()
except Exception as e:
@@ -832,21 +1042,31 @@ def on_files_dropped_on_group(self, files, group_id: int):
self.__add_urls_to_group(files, group_id=group_id)
def __add_urls_to_group(self, file_urls, group_id=0):
- local_files = [file_url.toLocalFile() for file_url in file_urls if file_url.isLocalFile()]
+ local_files = [
+ file_url.toLocalFile() for file_url in file_urls if file_url.isLocalFile()
+ ]
if len(local_files) > 0:
self.setCursor(Qt.WaitCursor)
- self.add_files(FileOperator.uncompress_archives(local_files, QDir.tempPath()), group_id=group_id)
+ self.add_files(
+ FileOperator.uncompress_archives(local_files, QDir.tempPath()),
+ group_id=group_id,
+ )
self.unsetCursor()
@pyqtSlot(list)
def on_cfc_close_wanted(self, protocols: list):
- frame_protos = {sframe: protocol for sframe, protocol in self.signal_protocol_dict.items() if
- protocol in protocols}
+ frame_protos = {
+ sframe: protocol
+ for sframe, protocol in self.signal_protocol_dict.items()
+ if protocol in protocols
+ }
for frame in frame_protos:
self.close_signal_frame(frame)
- for proto in (proto for proto in protocols if proto not in frame_protos.values()):
+ for proto in (
+ proto for proto in protocols if proto not in frame_protos.values()
+ ):
# close protocols without associated signal frame
self.close_protocol(proto)
@@ -870,7 +1090,9 @@ def on_options_changed(self, changed_options: dict):
self.generator_tab_controller.set_FZSave_button_visibility()
if "num_sending_repeats" in changed_options:
- self.project_manager.device_conf["num_sending_repeats"] = changed_options["num_sending_repeats"]
+ self.project_manager.device_conf["num_sending_repeats"] = changed_options[
+ "num_sending_repeats"
+ ]
if "default_view" in changed_options:
self.apply_default_view(int(changed_options["default_view"]))
@@ -880,7 +1102,9 @@ def on_options_changed(self, changed_options: dict):
@pyqtSlot()
def on_text_edit_project_description_text_changed(self):
- self.project_manager.description = self.ui.textEditProjectDescription.toPlainText()
+ self.project_manager.description = (
+ self.ui.textEditProjectDescription.toPlainText()
+ )
@pyqtSlot()
def on_btn_file_tree_go_up_clicked(self):
@@ -888,7 +1112,9 @@ def on_btn_file_tree_go_up_clicked(self):
if cur_dir.cdUp():
path = cur_dir.path()
self.filemodel.setRootPath(path)
- self.ui.fileTree.setRootIndex(self.file_proxy_model.mapFromSource(self.filemodel.index(path)))
+ self.ui.fileTree.setRootIndex(
+ self.file_proxy_model.mapFromSource(self.filemodel.index(path))
+ )
@pyqtSlot(int, Signal)
def on_signal_created(self, index: int, signal: Signal):
@@ -910,7 +1136,9 @@ def on_auto_detect_new_signals_action_triggered(self, checked: bool):
def __import_csv(self, file_name, group_id=0):
def on_data_imported(complex_file, sample_rate):
sample_rate = None if sample_rate == 0 else sample_rate
- self.add_files([complex_file], group_id=group_id, enforce_sample_rate=sample_rate)
+ self.add_files(
+ [complex_file], group_id=group_id, enforce_sample_rate=sample_rate
+ )
dialog = CSVImportDialog(file_name, parent=self)
dialog.data_imported.connect(on_data_imported)
@@ -934,7 +1162,9 @@ def on_project_loaded_status_changed(self, project_loaded: bool):
@pyqtSlot()
def on_compare_frame_controller_load_protocol_clicked(self):
- dialog = FileOperator.get_open_dialog(directory_mode=False, parent=self, name_filter="proto")
+ dialog = FileOperator.get_open_dialog(
+ directory_mode=False, parent=self, name_filter="proto"
+ )
if dialog.exec_():
for filename in dialog.selectedFiles():
self.add_protocol_file(filename)
diff --git a/src/urh/controller/SignalTabController.py b/src/urh/controller/SignalTabController.py
index 44b8cf9222..f0aefdce94 100644
--- a/src/urh/controller/SignalTabController.py
+++ b/src/urh/controller/SignalTabController.py
@@ -26,8 +26,11 @@ def signal_frames(self):
:rtype: list of SignalFrame
"""
splitter = self.ui.splitter
- return [splitter.widget(i) for i in range(splitter.count())
- if isinstance(splitter.widget(i), SignalFrame)]
+ return [
+ splitter.widget(i)
+ for i in range(splitter.count())
+ if isinstance(splitter.widget(i), SignalFrame)
+ ]
@property
def signal_undo_stack(self):
@@ -52,12 +55,14 @@ def __init__(self, project_manager, parent=None):
def on_files_dropped(self, files):
self.files_dropped.emit(files)
- def close_frame(self, frame:SignalFrame):
+ def close_frame(self, frame: SignalFrame):
self.frame_closed.emit(frame)
def add_signal_frame(self, proto_analyzer, index=-1):
self.__set_getting_started_status(False)
- sig_frame = SignalFrame(proto_analyzer, self.undo_stack, self.project_manager, parent=self)
+ sig_frame = SignalFrame(
+ proto_analyzer, self.undo_stack, self.project_manager, parent=self
+ )
sframes = self.signal_frames
if len(proto_analyzer.signal.filename) == 0:
@@ -67,12 +72,16 @@ def add_signal_frame(self, proto_analyzer, index=-1):
self.__create_connects_for_signal_frame(signal_frame=sig_frame)
sig_frame.signal_created.connect(self.emit_signal_created)
sig_frame.not_show_again_changed.connect(self.not_show_again_changed.emit)
- sig_frame.ui.lineEditSignalName.setToolTip(self.tr("Sourcefile: ") + proto_analyzer.signal.filename)
+ sig_frame.ui.lineEditSignalName.setToolTip(
+ self.tr("Sourcefile: ") + proto_analyzer.signal.filename
+ )
sig_frame.apply_to_all_clicked.connect(self.on_apply_to_all_clicked)
prev_signal_frame = sframes[-1] if len(sframes) > 0 else None
if prev_signal_frame is not None and hasattr(prev_signal_frame, "ui"):
- sig_frame.ui.cbProtoView.setCurrentIndex(prev_signal_frame.ui.cbProtoView.currentIndex())
+ sig_frame.ui.cbProtoView.setCurrentIndex(
+ prev_signal_frame.ui.cbProtoView.currentIndex()
+ )
sig_frame.blockSignals(True)
@@ -80,15 +89,19 @@ def add_signal_frame(self, proto_analyzer, index=-1):
self.ui.splitter.insertWidget(index, sig_frame)
sig_frame.blockSignals(False)
- default_view = settings.read('default_view', 0, int)
+ default_view = settings.read("default_view", 0, int)
sig_frame.ui.cbProtoView.setCurrentIndex(default_view)
return sig_frame
def add_empty_frame(self, filename: str, proto):
self.__set_getting_started_status(False)
- sig_frame = SignalFrame(proto_analyzer=proto, undo_stack=self.undo_stack, project_manager=self.project_manager,
- parent=self)
+ sig_frame = SignalFrame(
+ proto_analyzer=proto,
+ undo_stack=self.undo_stack,
+ project_manager=self.project_manager,
+ parent=self,
+ )
sig_frame.ui.lineEditSignalName.setText(filename)
self.__create_connects_for_signal_frame(signal_frame=sig_frame)
@@ -110,7 +123,7 @@ def __set_getting_started_status(self, getting_started: bool):
self.ui.splitter.addWidget(w)
def __create_connects_for_signal_frame(self, signal_frame: SignalFrame):
- signal_frame.hold_shift = settings.read('hold_shift_to_drag', True, type=bool)
+ signal_frame.hold_shift = settings.read("hold_shift_to_drag", True, type=bool)
signal_frame.drag_started.connect(self.frame_dragged)
signal_frame.frame_dropped.connect(self.frame_dropped)
signal_frame.files_dropped.connect(self.on_files_dropped)
@@ -126,14 +139,17 @@ def save_all(self):
return
try:
- not_show = settings.read('not_show_save_dialog', False, type=bool)
+ not_show = settings.read("not_show_save_dialog", False, type=bool)
except TypeError:
not_show = False
if not not_show:
cb = QCheckBox("Don't ask me again.")
- msg_box = QMessageBox(QMessageBox.Question, self.tr("Confirm saving all signals"),
- self.tr("All changed signal files will be overwritten. OK?"))
+ msg_box = QMessageBox(
+ QMessageBox.Question,
+ self.tr("Confirm saving all signals"),
+ self.tr("All changed signal files will be overwritten. OK?"),
+ )
msg_box.addButton(QMessageBox.Yes)
msg_box.addButton(QMessageBox.No)
msg_box.setCheckBox(cb)
@@ -179,7 +195,9 @@ def on_apply_to_all_clicked(self, signal: Signal):
proto_needs_update = True
if frame.signal.noise_threshold != signal.noise_threshold:
- frame.signal.noise_threshold_relative = signal.noise_threshold_relative
+ frame.signal.noise_threshold_relative = (
+ signal.noise_threshold_relative
+ )
proto_needs_update = True
if frame.signal.samples_per_symbol != signal.samples_per_symbol:
diff --git a/src/urh/controller/SimulatorTabController.py b/src/urh/controller/SimulatorTabController.py
index 1ec7f07ca7..758ecbda5c 100644
--- a/src/urh/controller/SimulatorTabController.py
+++ b/src/urh/controller/SimulatorTabController.py
@@ -3,8 +3,16 @@
import numpy
from PyQt5.QtCore import pyqtSlot, Qt, QDir, QStringListModel, pyqtSignal
from PyQt5.QtGui import QIcon
-from PyQt5.QtWidgets import QWidget, QFileDialog, QCompleter, QMessageBox, QFrame, \
- QHBoxLayout, QToolButton, QDialog
+from PyQt5.QtWidgets import (
+ QWidget,
+ QFileDialog,
+ QCompleter,
+ QMessageBox,
+ QFrame,
+ QHBoxLayout,
+ QToolButton,
+ QDialog,
+)
from urh.controller.CompareFrameController import CompareFrameController
from urh.controller.GeneratorTabController import GeneratorTabController
@@ -43,9 +51,13 @@ class SimulatorTabController(QWidget):
open_in_analysis_requested = pyqtSignal(str)
rx_file_saved = pyqtSignal(str)
- def __init__(self, compare_frame_controller: CompareFrameController,
- generator_tab_controller: GeneratorTabController,
- project_manager: ProjectManager, parent):
+ def __init__(
+ self,
+ compare_frame_controller: CompareFrameController,
+ generator_tab_controller: GeneratorTabController,
+ project_manager: ProjectManager,
+ parent,
+ ):
super().__init__(parent)
self.project_manager = project_manager
@@ -69,42 +81,69 @@ def __init__(self, compare_frame_controller: CompareFrameController,
self.tree_model = self.generator_tab_controller.tree_model
self.ui.treeProtocols.setModel(self.tree_model)
- self.participant_table_model = ParticipantTableModel(project_manager.participants)
+ self.participant_table_model = ParticipantTableModel(
+ project_manager.participants
+ )
self.ui.tableViewParticipants.setModel(self.participant_table_model)
self.participant_table_model.update()
self.simulator_message_field_model = SimulatorMessageFieldModel(self)
self.ui.tblViewFieldValues.setModel(self.simulator_message_field_model)
- self.ui.tblViewFieldValues.setItemDelegateForColumn(1, ComboBoxDelegate(ProtocolLabel.DISPLAY_FORMATS,
- parent=self.ui.tblViewFieldValues))
- self.ui.tblViewFieldValues.setItemDelegateForColumn(2, ComboBoxDelegate(SimulatorProtocolLabel.VALUE_TYPES,
- parent=self.ui.tblViewFieldValues))
- self.ui.tblViewFieldValues.setItemDelegateForColumn(3, ProtocolValueDelegate(controller=self,
- parent=self.ui.tblViewFieldValues))
+ self.ui.tblViewFieldValues.setItemDelegateForColumn(
+ 1,
+ ComboBoxDelegate(
+ ProtocolLabel.DISPLAY_FORMATS, parent=self.ui.tblViewFieldValues
+ ),
+ )
+ self.ui.tblViewFieldValues.setItemDelegateForColumn(
+ 2,
+ ComboBoxDelegate(
+ SimulatorProtocolLabel.VALUE_TYPES, parent=self.ui.tblViewFieldValues
+ ),
+ )
+ self.ui.tblViewFieldValues.setItemDelegateForColumn(
+ 3, ProtocolValueDelegate(controller=self, parent=self.ui.tblViewFieldValues)
+ )
self.project_manager.reload_field_types()
self.update_field_name_column()
- self.simulator_message_table_model = SimulatorMessageTableModel(self.project_manager, self)
+ self.simulator_message_table_model = SimulatorMessageTableModel(
+ self.project_manager, self
+ )
self.ui.tblViewMessage.setModel(self.simulator_message_table_model)
- self.ui.ruleCondLineEdit.setValidator(RuleExpressionValidator(self.sim_expression_parser, is_formula=False))
+ self.ui.ruleCondLineEdit.setValidator(
+ RuleExpressionValidator(self.sim_expression_parser, is_formula=False)
+ )
self.completer_model = QStringListModel([])
- self.ui.ruleCondLineEdit.setCompleter(QCompleter(self.completer_model, self.ui.ruleCondLineEdit))
- self.ui.ruleCondLineEdit.setToolTip(self.sim_expression_parser.rule_condition_help)
-
- self.simulator_scene = SimulatorScene(mode=0, simulator_config=self.simulator_config)
- self.simulator_scene.tree_root_item = compare_frame_controller.proto_tree_model.rootItem
+ self.ui.ruleCondLineEdit.setCompleter(
+ QCompleter(self.completer_model, self.ui.ruleCondLineEdit)
+ )
+ self.ui.ruleCondLineEdit.setToolTip(
+ self.sim_expression_parser.rule_condition_help
+ )
+
+ self.simulator_scene = SimulatorScene(
+ mode=0, simulator_config=self.simulator_config
+ )
+ self.simulator_scene.tree_root_item = (
+ compare_frame_controller.proto_tree_model.rootItem
+ )
self.ui.gvSimulator.setScene(self.simulator_scene)
self.ui.gvSimulator.setAlignment(Qt.AlignLeft | Qt.AlignTop)
self.ui.gvSimulator.proto_analyzer = compare_frame_controller.proto_analyzer
self.__active_item = None
- self.ui.listViewSimulate.setModel(SimulatorParticipantListModel(self.simulator_config))
+ self.ui.listViewSimulate.setModel(
+ SimulatorParticipantListModel(self.simulator_config)
+ )
self.ui.spinBoxNRepeat.setValue(self.project_manager.simulator_num_repeat)
self.ui.spinBoxTimeout.setValue(self.project_manager.simulator_timeout_ms)
self.ui.spinBoxRetries.setValue(self.project_manager.simulator_retries)
- self.ui.comboBoxError.setCurrentIndex(self.project_manager.simulator_error_handling_index)
+ self.ui.comboBoxError.setCurrentIndex(
+ self.project_manager.simulator_error_handling_index
+ )
# place save/load button at corner of tab widget
frame = QFrame(parent=self)
@@ -120,15 +159,18 @@ def __init__(self, compare_frame_controller: CompareFrameController,
frame.layout().setContentsMargins(0, 0, 0, 0)
self.ui.tabWidget.setCornerWidget(frame)
- self.ui.splitterLeftRight.setSizes([int(0.2 * self.width()), int(0.8 * self.width())])
+ self.ui.splitterLeftRight.setSizes(
+ [int(0.2 * self.width()), int(0.8 * self.width())]
+ )
self.create_connects()
def refresh_field_types_for_labels(self):
for msg in self.simulator_config.get_all_messages():
for lbl in (lbl for lbl in msg.message_type if lbl.field_type is not None):
- msg.message_type.change_field_type_of_label(lbl, self.field_types_by_caption.get(lbl.field_type.caption,
- None))
+ msg.message_type.change_field_type_of_label(
+ lbl, self.field_types_by_caption.get(lbl.field_type.caption, None)
+ )
self.update_field_name_column()
self.update_ui()
@@ -143,43 +185,83 @@ def field_types_by_caption(self):
def update_field_name_column(self):
field_types = [ft.caption for ft in self.field_types]
- self.ui.tblViewFieldValues.setItemDelegateForColumn(0, ComboBoxDelegate(field_types, is_editable=True,
- return_index=False,
- parent=self.ui.tblViewFieldValues))
+ self.ui.tblViewFieldValues.setItemDelegateForColumn(
+ 0,
+ ComboBoxDelegate(
+ field_types,
+ is_editable=True,
+ return_index=False,
+ parent=self.ui.tblViewFieldValues,
+ ),
+ )
def create_connects(self):
self.ui.btnChooseCommand.clicked.connect(self.on_btn_choose_command_clicked)
- self.ui.lineEditTriggerCommand.textChanged.connect(self.on_line_edit_trigger_command_text_changed)
- self.ui.checkBoxPassTranscriptSTDIN.clicked.connect(self.on_check_box_pass_transcript_STDIN_clicked)
- self.ui.doubleSpinBoxSleep.editingFinished.connect(self.on_spinbox_sleep_editing_finished)
- self.ui.ruleCondLineEdit.textChanged.connect(self.on_rule_cond_line_edit_text_changed)
+ self.ui.lineEditTriggerCommand.textChanged.connect(
+ self.on_line_edit_trigger_command_text_changed
+ )
+ self.ui.checkBoxPassTranscriptSTDIN.clicked.connect(
+ self.on_check_box_pass_transcript_STDIN_clicked
+ )
+ self.ui.doubleSpinBoxSleep.editingFinished.connect(
+ self.on_spinbox_sleep_editing_finished
+ )
+ self.ui.ruleCondLineEdit.textChanged.connect(
+ self.on_rule_cond_line_edit_text_changed
+ )
self.ui.btnStartSim.clicked.connect(self.on_btn_simulate_clicked)
- self.ui.goto_combobox.currentIndexChanged.connect(self.on_goto_combobox_index_changed)
+ self.ui.goto_combobox.currentIndexChanged.connect(
+ self.on_goto_combobox_index_changed
+ )
self.ui.spinBoxRepeat.valueChanged.connect(self.on_repeat_value_changed)
self.ui.cbViewType.currentIndexChanged.connect(self.on_view_type_changed)
- self.ui.tblViewMessage.create_label_triggered.connect(self.create_simulator_label)
- self.ui.tblViewMessage.open_modulator_dialog_clicked.connect(self.open_modulator_dialog)
- self.ui.tblViewMessage.selectionModel().selectionChanged.connect(self.on_table_selection_changed)
+ self.ui.tblViewMessage.create_label_triggered.connect(
+ self.create_simulator_label
+ )
+ self.ui.tblViewMessage.open_modulator_dialog_clicked.connect(
+ self.open_modulator_dialog
+ )
+ self.ui.tblViewMessage.selectionModel().selectionChanged.connect(
+ self.on_table_selection_changed
+ )
self.ui.tabWidget.currentChanged.connect(self.on_selected_tab_changed)
self.ui.btnSave.clicked.connect(self.on_btn_save_clicked)
self.ui.btnLoad.clicked.connect(self.on_btn_load_clicked)
- self.ui.listViewSimulate.model().participant_simulate_changed.connect(self.on_participant_simulate_changed)
-
- self.ui.btnAddParticipant.clicked.connect(self.ui.tableViewParticipants.on_add_action_triggered)
- self.ui.btnRemoveParticipant.clicked.connect(self.ui.tableViewParticipants.on_remove_action_triggered)
- self.ui.btnUp.clicked.connect(self.ui.tableViewParticipants.on_move_up_action_triggered)
- self.ui.btnDown.clicked.connect(self.ui.tableViewParticipants.on_move_down_action_triggered)
- self.participant_table_model.participant_edited.connect(self.on_participant_edited)
+ self.ui.listViewSimulate.model().participant_simulate_changed.connect(
+ self.on_participant_simulate_changed
+ )
+
+ self.ui.btnAddParticipant.clicked.connect(
+ self.ui.tableViewParticipants.on_add_action_triggered
+ )
+ self.ui.btnRemoveParticipant.clicked.connect(
+ self.ui.tableViewParticipants.on_remove_action_triggered
+ )
+ self.ui.btnUp.clicked.connect(
+ self.ui.tableViewParticipants.on_move_up_action_triggered
+ )
+ self.ui.btnDown.clicked.connect(
+ self.ui.tableViewParticipants.on_move_down_action_triggered
+ )
+ self.participant_table_model.participant_edited.connect(
+ self.on_participant_edited
+ )
self.tree_model.modelReset.connect(self.refresh_tree)
- self.simulator_scene.selectionChanged.connect(self.on_simulator_scene_selection_changed)
+ self.simulator_scene.selectionChanged.connect(
+ self.on_simulator_scene_selection_changed
+ )
self.simulator_scene.files_dropped.connect(self.on_files_dropped)
- self.simulator_message_field_model.protocol_label_updated.connect(self.item_updated)
+ self.simulator_message_field_model.protocol_label_updated.connect(
+ self.item_updated
+ )
self.ui.gvSimulator.message_updated.connect(self.item_updated)
- self.ui.gvSimulator.consolidate_messages_clicked.connect(self.consolidate_messages)
+ self.ui.gvSimulator.consolidate_messages_clicked.connect(
+ self.consolidate_messages
+ )
self.simulator_config.items_added.connect(self.refresh_message_table)
self.simulator_config.items_updated.connect(self.refresh_message_table)
@@ -187,20 +269,40 @@ def create_connects(self):
self.simulator_config.items_deleted.connect(self.refresh_message_table)
self.simulator_config.participants_changed.connect(self.on_participants_changed)
self.simulator_config.item_dict_updated.connect(self.on_item_dict_updated)
- self.simulator_config.active_participants_updated.connect(self.on_active_participants_updated)
-
- self.ui.gvSimulator.message_updated.connect(self.on_message_source_or_destination_updated)
-
- self.ui.spinBoxNRepeat.valueChanged.connect(self.on_spinbox_num_repeat_value_changed)
- self.ui.spinBoxTimeout.valueChanged.connect(self.on_spinbox_timeout_value_changed)
- self.ui.comboBoxError.currentIndexChanged.connect(self.on_combobox_error_handling_index_changed)
- self.ui.spinBoxRetries.valueChanged.connect(self.on_spinbox_retries_value_changed)
-
- self.ui.tblViewFieldValues.item_link_clicked.connect(self.on_table_item_link_clicked)
- self.ui.tblViewMessage.edit_label_triggered.connect(self.on_edit_label_triggered)
-
- self.ui.spinBoxCounterStart.editingFinished.connect(self.on_spinbox_counter_start_editing_finished)
- self.ui.spinBoxCounterStep.editingFinished.connect(self.on_spinbox_counter_step_editing_finished)
+ self.simulator_config.active_participants_updated.connect(
+ self.on_active_participants_updated
+ )
+
+ self.ui.gvSimulator.message_updated.connect(
+ self.on_message_source_or_destination_updated
+ )
+
+ self.ui.spinBoxNRepeat.valueChanged.connect(
+ self.on_spinbox_num_repeat_value_changed
+ )
+ self.ui.spinBoxTimeout.valueChanged.connect(
+ self.on_spinbox_timeout_value_changed
+ )
+ self.ui.comboBoxError.currentIndexChanged.connect(
+ self.on_combobox_error_handling_index_changed
+ )
+ self.ui.spinBoxRetries.valueChanged.connect(
+ self.on_spinbox_retries_value_changed
+ )
+
+ self.ui.tblViewFieldValues.item_link_clicked.connect(
+ self.on_table_item_link_clicked
+ )
+ self.ui.tblViewMessage.edit_label_triggered.connect(
+ self.on_edit_label_triggered
+ )
+
+ self.ui.spinBoxCounterStart.editingFinished.connect(
+ self.on_spinbox_counter_start_editing_finished
+ )
+ self.ui.spinBoxCounterStep.editingFinished.connect(
+ self.on_spinbox_counter_step_editing_finished
+ )
def consolidate_messages(self):
self.simulator_config.consolidate_messages()
@@ -223,7 +325,9 @@ def on_selected_tab_changed(self, index: int):
self.update_vertical_table_header()
def refresh_message_table(self):
- self.simulator_message_table_model.protocol.messages[:] = self.simulator_config.get_all_messages()
+ self.simulator_message_table_model.protocol.messages[
+ :
+ ] = self.simulator_config.get_all_messages()
self.simulator_message_table_model.update()
if isinstance(self.active_item, SimulatorMessage):
@@ -259,27 +363,39 @@ def close_all(self):
@pyqtSlot(int, int, int)
def create_simulator_label(self, msg_index: int, start: int, end: int):
con = self.simulator_message_table_model.protocol
- start, end = con.convert_range(start, end - 1, self.ui.cbViewType.currentIndex(), 0, False, msg_index)
- lbl = self.simulator_config.add_label(start=start, end=end, parent_item=con.messages[msg_index])
+ start, end = con.convert_range(
+ start, end - 1, self.ui.cbViewType.currentIndex(), 0, False, msg_index
+ )
+ lbl = self.simulator_config.add_label(
+ start=start, end=end, parent_item=con.messages[msg_index]
+ )
try:
index = self.simulator_message_field_model.message_type.index(lbl)
- self.ui.tblViewFieldValues.edit(self.simulator_message_field_model.createIndex(index, 0))
+ self.ui.tblViewFieldValues.edit(
+ self.simulator_message_field_model.createIndex(index, 0)
+ )
except ValueError:
pass
@pyqtSlot()
def open_modulator_dialog(self):
- selected_message = self.simulator_message_table_model.protocol.messages[self.ui.tblViewMessage.selected_rows[0]]
+ selected_message = self.simulator_message_table_model.protocol.messages[
+ self.ui.tblViewMessage.selected_rows[0]
+ ]
preselected_index = selected_message.modulator_index
- modulator_dialog = ModulatorDialog(self.project_manager.modulators, tree_model=self.tree_model, parent=self)
+ modulator_dialog = ModulatorDialog(
+ self.project_manager.modulators, tree_model=self.tree_model, parent=self
+ )
modulator_dialog.ui.comboBoxCustomModulations.setCurrentIndex(preselected_index)
modulator_dialog.showMaximized()
modulator_dialog.initialize(selected_message.encoded_bits_str[0:16])
modulator_dialog.finished.connect(self.refresh_modulators)
- modulator_dialog.finished.connect(self.generator_tab_controller.refresh_pause_list)
+ modulator_dialog.finished.connect(
+ self.generator_tab_controller.refresh_pause_list
+ )
@pyqtSlot()
def refresh_modulators(self):
@@ -297,7 +413,9 @@ def refresh_modulators(self):
index = self.sender().ui.comboBoxCustomModulations.currentIndex()
for row in self.ui.tblViewMessage.selected_rows:
- self.simulator_message_table_model.protocol.messages[row].modulator_index = index
+ self.simulator_message_table_model.protocol.messages[
+ row
+ ].modulator_index = index
def update_goto_combobox(self, active_item: SimulatorGotoAction):
assert isinstance(active_item, SimulatorGotoAction)
@@ -341,7 +459,9 @@ def on_rule_cond_line_edit_text_changed(self):
@pyqtSlot()
def on_view_type_changed(self):
- self.simulator_message_table_model.proto_view = self.ui.cbViewType.currentIndex()
+ self.simulator_message_table_model.proto_view = (
+ self.ui.cbViewType.currentIndex()
+ )
self.simulator_message_table_model.update()
self.ui.tblViewMessage.resize_columns()
@@ -350,7 +470,11 @@ def on_goto_combobox_index_changed(self):
if not isinstance(self.active_item, SimulatorGotoAction):
return
- self.active_item.goto_target = None if self.ui.goto_combobox.currentIndex() == 0 else self.ui.goto_combobox.currentText()
+ self.active_item.goto_target = (
+ None
+ if self.ui.goto_combobox.currentIndex() == 0
+ else self.ui.goto_combobox.currentText()
+ )
self.item_updated(self.active_item)
@pyqtSlot()
@@ -369,7 +493,9 @@ def on_table_selection_changed(self):
self.ui.lNumSelectedColumns.setText("0")
else:
max_row = numpy.max([rng.bottom() for rng in selection])
- self.active_item = self.simulator_message_table_model.protocol.messages[max_row]
+ self.active_item = self.simulator_message_table_model.protocol.messages[
+ max_row
+ ]
_, _, start, end = self.ui.tblViewMessage.selection_range()
self.ui.lNumSelectedColumns.setText(str(end - start))
@@ -393,13 +519,17 @@ def active_item(self, value):
self.ui.lblEncodingDecoding.setText(self.active_item.decoder.name)
self.ui.detail_view_widget.setCurrentIndex(2)
- elif (isinstance(self.active_item, SimulatorRuleCondition) and
- self.active_item.type != ConditionType.ELSE):
+ elif (
+ isinstance(self.active_item, SimulatorRuleCondition)
+ and self.active_item.type != ConditionType.ELSE
+ ):
self.ui.ruleCondLineEdit.setText(self.active_item.condition)
self.ui.detail_view_widget.setCurrentIndex(3)
elif isinstance(self.active_item, SimulatorTriggerCommandAction):
self.ui.lineEditTriggerCommand.setText(self.active_item.command)
- self.ui.checkBoxPassTranscriptSTDIN.setChecked(self.active_item.pass_transcript)
+ self.ui.checkBoxPassTranscriptSTDIN.setChecked(
+ self.active_item.pass_transcript
+ )
self.ui.detail_view_widget.setCurrentIndex(4)
elif isinstance(self.active_item, SimulatorSleepAction):
self.ui.doubleSpinBoxSleep.setValue(self.active_item.sleep_time)
@@ -416,27 +546,47 @@ def active_item(self, value):
@pyqtSlot()
def on_btn_simulate_clicked(self):
if not self.simulator_config.protocol_valid():
- QMessageBox.critical(self, self.tr("Invalid protocol configuration"),
- self.tr(
- "There are some problems with your protocol configuration. Please fix them first."))
+ QMessageBox.critical(
+ self,
+ self.tr("Invalid protocol configuration"),
+ self.tr(
+ "There are some problems with your protocol configuration. Please fix them first."
+ ),
+ )
return
if not len(self.simulator_config.get_all_messages()):
- QMessageBox.critical(self, self.tr("No messages found"), self.tr("Please add at least one message."))
+ QMessageBox.critical(
+ self,
+ self.tr("No messages found"),
+ self.tr("Please add at least one message."),
+ )
return
- num_simulated = len([p for p in self.project_manager.participants if p.simulate])
+ num_simulated = len(
+ [p for p in self.project_manager.participants if p.simulate]
+ )
if num_simulated == 0:
if self.ui.listViewSimulate.model().rowCount() == 0:
- QMessageBox.critical(self, self.tr("No active participants"),
- self.tr("You have no active participants.
"
- "Please add a participant in the Participants tab and "
- "assign it to at least one message as source or destination."))
+ QMessageBox.critical(
+ self,
+ self.tr("No active participants"),
+ self.tr(
+ "You have no active participants.
"
+ "Please add a participant in the Participants tab and "
+ "assign it to at least one message as source or destination."
+ ),
+ )
return
else:
- QMessageBox.critical(self, self.tr("No participant for simulation selected"),
- self.tr("Please check at least one participant from the "
- "Simulate these participants list."))
+ QMessageBox.critical(
+ self,
+ self.tr("No participant for simulation selected"),
+ self.tr(
+ "Please check at least one participant from the "
+ "Simulate these participants list."
+ ),
+ )
return
try:
@@ -445,16 +595,30 @@ def on_btn_simulate_clicked(self):
Errors.exception(e)
def get_simulator_dialog(self) -> SimulatorDialog:
- protos = [p for proto_list in self.tree_model.protocols.values() for p in proto_list]
+ protos = [
+ p for proto_list in self.tree_model.protocols.values() for p in proto_list
+ ]
signals = [p.signal for p in protos if p.signal is not None]
- s = SimulatorDialog(self.simulator_config, self.project_manager.modulators,
- self.sim_expression_parser, self.project_manager, signals=signals,
- signal_tree_model=self.tree_model, parent=self)
-
- s.rx_parameters_changed.connect(self.project_manager.on_simulator_rx_parameters_changed)
- s.sniff_parameters_changed.connect(self.project_manager.on_simulator_sniff_parameters_changed)
- s.tx_parameters_changed.connect(self.project_manager.on_simulator_tx_parameters_changed)
+ s = SimulatorDialog(
+ self.simulator_config,
+ self.project_manager.modulators,
+ self.sim_expression_parser,
+ self.project_manager,
+ signals=signals,
+ signal_tree_model=self.tree_model,
+ parent=self,
+ )
+
+ s.rx_parameters_changed.connect(
+ self.project_manager.on_simulator_rx_parameters_changed
+ )
+ s.sniff_parameters_changed.connect(
+ self.project_manager.on_simulator_sniff_parameters_changed
+ )
+ s.tx_parameters_changed.connect(
+ self.project_manager.on_simulator_tx_parameters_changed
+ )
s.open_in_analysis_requested.connect(self.open_in_analysis_requested.emit)
s.rx_file_saved.connect(self.rx_file_saved.emit)
@@ -462,7 +626,9 @@ def get_simulator_dialog(self) -> SimulatorDialog:
@pyqtSlot()
def on_btn_choose_command_clicked(self):
- file_name, ok = QFileDialog.getOpenFileName(self, self.tr("Choose program"), QDir.homePath())
+ file_name, ok = QFileDialog.getOpenFileName(
+ self, self.tr("Choose program"), QDir.homePath()
+ )
if file_name is not None and ok:
self.ui.lineEditTriggerCommand.setText(file_name)
@@ -474,7 +640,9 @@ def on_line_edit_trigger_command_text_changed(self):
@pyqtSlot()
def on_check_box_pass_transcript_STDIN_clicked(self):
- self.active_item.pass_transcript = self.ui.checkBoxPassTranscriptSTDIN.isChecked()
+ self.active_item.pass_transcript = (
+ self.ui.checkBoxPassTranscriptSTDIN.isChecked()
+ )
self.item_updated(self.active_item)
@pyqtSlot()
@@ -507,13 +675,17 @@ def refresh_tree(self):
@pyqtSlot()
def on_btn_save_clicked(self):
- filename = FileOperator.ask_save_file_name(initial_name="myprofile.sim.xml", caption="Save simulator profile")
+ filename = FileOperator.ask_save_file_name(
+ initial_name="myprofile.sim.xml", caption="Save simulator profile"
+ )
if filename:
self.save_simulator_file(filename)
@pyqtSlot()
def on_btn_load_clicked(self):
- dialog = FileOperator.get_open_dialog(False, parent=self, name_filter="simulator")
+ dialog = FileOperator.get_open_dialog(
+ False, parent=self, name_filter="simulator"
+ )
if dialog.exec_():
self.load_simulator_file(dialog.selectedFiles()[0])
@@ -544,7 +716,9 @@ def on_message_source_or_destination_updated(self):
@pyqtSlot(int, int)
def on_table_item_link_clicked(self, row: int, column: int):
try:
- lbl = self.simulator_message_field_model.message_type[row] # type: SimulatorProtocolLabel
+ lbl = self.simulator_message_field_model.message_type[
+ row
+ ] # type: SimulatorProtocolLabel
assert lbl.is_checksum_label
assert isinstance(self.active_item, SimulatorMessage)
except (IndexError, AssertionError):
@@ -552,7 +726,11 @@ def on_table_item_link_clicked(self, row: int, column: int):
d = QDialog(parent=self)
layout = QHBoxLayout()
- layout.addWidget(ChecksumWidget(lbl.label, self.active_item, self.ui.cbViewType.currentIndex()))
+ layout.addWidget(
+ ChecksumWidget(
+ lbl.label, self.active_item, self.ui.cbViewType.currentIndex()
+ )
+ )
d.setLayout(layout)
d.show()
@@ -567,8 +745,12 @@ def on_active_participants_updated(self):
@pyqtSlot(int)
def on_edit_label_triggered(self, label_index: int):
view_type = self.ui.cbViewType.currentIndex()
- protocol_label_dialog = ProtocolLabelDialog(message=self.ui.tblViewMessage.selected_message,
- viewtype=view_type, selected_index=label_index, parent=self)
+ protocol_label_dialog = ProtocolLabelDialog(
+ message=self.ui.tblViewMessage.selected_message,
+ viewtype=view_type,
+ selected_index=label_index,
+ parent=self,
+ )
protocol_label_dialog.finished.connect(self.on_protocol_label_dialog_finished)
protocol_label_dialog.showMaximized()
@@ -580,5 +762,7 @@ def on_protocol_label_dialog_finished(self):
@pyqtSlot(list)
def on_files_dropped(self, file_urls: list):
- for filename in (file_url.toLocalFile() for file_url in file_urls if file_url.isLocalFile()):
- self.load_simulator_file(filename)
\ No newline at end of file
+ for filename in (
+ file_url.toLocalFile() for file_url in file_urls if file_url.isLocalFile()
+ ):
+ self.load_simulator_file(filename)
diff --git a/src/urh/controller/dialogs/AdvancedModulationOptionsDialog.py b/src/urh/controller/dialogs/AdvancedModulationOptionsDialog.py
index 9864ea2678..dcac5a95f9 100644
--- a/src/urh/controller/dialogs/AdvancedModulationOptionsDialog.py
+++ b/src/urh/controller/dialogs/AdvancedModulationOptionsDialog.py
@@ -33,6 +33,8 @@ def on_accept_clicked(self):
self.pause_threshold_edited.emit(self.ui.spinBoxPauseThreshold.value())
if self.message_length_divisor != self.ui.spinBoxMessageLengthDivisor.value():
- self.message_length_divisor_edited.emit(self.ui.spinBoxMessageLengthDivisor.value())
+ self.message_length_divisor_edited.emit(
+ self.ui.spinBoxMessageLengthDivisor.value()
+ )
self.accept()
diff --git a/src/urh/controller/dialogs/CSVImportDialog.py b/src/urh/controller/dialogs/CSVImportDialog.py
index b412a92bc1..84c556eb1a 100644
--- a/src/urh/controller/dialogs/CSVImportDialog.py
+++ b/src/urh/controller/dialogs/CSVImportDialog.py
@@ -3,7 +3,14 @@
import os
import numpy as np
from PyQt5.QtCore import Qt, pyqtSlot, pyqtSignal
-from PyQt5.QtWidgets import QDialog, QInputDialog, QApplication, QCompleter, QDirModel, QFileDialog
+from PyQt5.QtWidgets import (
+ QDialog,
+ QInputDialog,
+ QApplication,
+ QCompleter,
+ QDirModel,
+ QFileDialog,
+)
from urh.ui.ui_csv_wizard import Ui_DialogCSVImport
from urh.util import FileOperator, util
@@ -13,7 +20,6 @@
class CSVImportDialog(QDialog):
data_imported = pyqtSignal(str, float) # Complex Filename + Sample Rate
-
PREVIEW_ROWS = 100
COLUMNS = {"T": 0, "I": 1, "Q": 2}
@@ -41,13 +47,23 @@ def __init__(self, filename="", parent=None):
def create_connects(self):
self.accepted.connect(self.on_accepted)
- self.ui.lineEditFilename.editingFinished.connect(self.on_line_edit_filename_editing_finished)
+ self.ui.lineEditFilename.editingFinished.connect(
+ self.on_line_edit_filename_editing_finished
+ )
self.ui.btnChooseFile.clicked.connect(self.on_btn_choose_file_clicked)
self.ui.btnAddSeparator.clicked.connect(self.on_btn_add_separator_clicked)
- self.ui.comboBoxCSVSeparator.currentIndexChanged.connect(self.on_combobox_csv_separator_current_index_changed)
- self.ui.spinBoxIDataColumn.valueChanged.connect(self.on_spinbox_i_data_column_value_changed)
- self.ui.spinBoxQDataColumn.valueChanged.connect(self.on_spinbox_q_data_column_value_changed)
- self.ui.spinBoxTimestampColumn.valueChanged.connect(self.on_spinbox_timestamp_value_changed)
+ self.ui.comboBoxCSVSeparator.currentIndexChanged.connect(
+ self.on_combobox_csv_separator_current_index_changed
+ )
+ self.ui.spinBoxIDataColumn.valueChanged.connect(
+ self.on_spinbox_i_data_column_value_changed
+ )
+ self.ui.spinBoxQDataColumn.valueChanged.connect(
+ self.on_spinbox_q_data_column_value_changed
+ )
+ self.ui.spinBoxTimestampColumn.valueChanged.connect(
+ self.on_spinbox_timestamp_value_changed
+ )
def update_file(self):
filename = self.ui.lineEditFilename.text()
@@ -85,18 +101,26 @@ def update_preview(self):
self.ui.tableWidgetPreview.setRowCount(self.PREVIEW_ROWS)
with open(self.filename, encoding="utf-8-sig") as f:
- csv_reader = csv.reader(f, delimiter=self.ui.comboBoxCSVSeparator.currentText())
+ csv_reader = csv.reader(
+ f, delimiter=self.ui.comboBoxCSVSeparator.currentText()
+ )
row = -1
for line in csv_reader:
row += 1
- result = self.parse_csv_line(line, i_data_col, q_data_col, timestamp_col)
+ result = self.parse_csv_line(
+ line, i_data_col, q_data_col, timestamp_col
+ )
if result is not None:
for key, value in result.items():
- self.ui.tableWidgetPreview.setItem(row, self.COLUMNS[key], util.create_table_item(value))
+ self.ui.tableWidgetPreview.setItem(
+ row, self.COLUMNS[key], util.create_table_item(value)
+ )
else:
for col in self.COLUMNS.values():
- self.ui.tableWidgetPreview.setItem(row, col, util.create_table_item("Invalid"))
+ self.ui.tableWidgetPreview.setItem(
+ row, col, util.create_table_item("Invalid")
+ )
if row >= self.PREVIEW_ROWS - 1:
break
@@ -104,7 +128,9 @@ def update_preview(self):
self.ui.tableWidgetPreview.setRowCount(row + 1)
@staticmethod
- def parse_csv_line(csv_line: str, i_data_col: int, q_data_col: int, timestamp_col: int):
+ def parse_csv_line(
+ csv_line: str, i_data_col: int, q_data_col: int, timestamp_col: int
+ ):
result = dict()
if i_data_col >= 0:
@@ -132,13 +158,17 @@ def parse_csv_line(csv_line: str, i_data_col: int, q_data_col: int, timestamp_co
return result
@staticmethod
- def parse_csv_file(filename: str, separator: str, i_data_col: int, q_data_col=-1, t_data_col=-1):
+ def parse_csv_file(
+ filename: str, separator: str, i_data_col: int, q_data_col=-1, t_data_col=-1
+ ):
iq_data = []
timestamps = [] if t_data_col > -1 else None
with open(filename, encoding="utf-8-sig") as f:
csv_reader = csv.reader(f, delimiter=separator)
for line in csv_reader:
- parsed = CSVImportDialog.parse_csv_line(line, i_data_col, q_data_col, t_data_col)
+ parsed = CSVImportDialog.parse_csv_line(
+ line, i_data_col, q_data_col, t_data_col
+ )
if parsed is None:
continue
@@ -158,8 +188,8 @@ def estimate_sample_rate(timestamps):
previous_timestamp = timestamps[0]
durations = []
- for timestamp in timestamps[1:CSVImportDialog.PREVIEW_ROWS]:
- durations.append(abs(timestamp-previous_timestamp))
+ for timestamp in timestamps[1 : CSVImportDialog.PREVIEW_ROWS]:
+ durations.append(abs(timestamp - previous_timestamp))
previous_timestamp = timestamp
return 1 / (sum(durations) / len(durations))
@@ -171,8 +201,12 @@ def on_line_edit_filename_editing_finished(self):
@pyqtSlot()
def on_btn_choose_file_clicked(self):
- filename, _ = QFileDialog.getOpenFileName(self, self.tr("Choose file"), directory=FileOperator.RECENT_PATH,
- filter="CSV files (*.csv);;All files (*.*)")
+ filename, _ = QFileDialog.getOpenFileName(
+ self,
+ self.tr("Choose file"),
+ directory=FileOperator.RECENT_PATH,
+ filter="CSV files (*.csv);;All files (*.*)",
+ )
if filename:
self.ui.lineEditFilename.setText(filename)
@@ -181,12 +215,16 @@ def on_btn_choose_file_clicked(self):
@pyqtSlot()
def on_btn_add_separator_clicked(self):
sep, ok = QInputDialog.getText(self, "Enter Separator", "Separator:", text=",")
- if ok and sep not in (self.ui.comboBoxCSVSeparator.itemText(i) for i in
- range(self.ui.comboBoxCSVSeparator.count())):
+ if ok and sep not in (
+ self.ui.comboBoxCSVSeparator.itemText(i)
+ for i in range(self.ui.comboBoxCSVSeparator.count())
+ ):
if len(sep) == 1:
self.ui.comboBoxCSVSeparator.addItem(sep)
else:
- Errors.generic_error("Invalid Separator", "Separator must be exactly one character.")
+ Errors.generic_error(
+ "Invalid Separator", "Separator must be exactly one character."
+ )
@pyqtSlot(int)
def on_combobox_csv_separator_current_index_changed(self, index: int):
@@ -209,10 +247,13 @@ def on_spinbox_timestamp_value_changed(self, value: int):
def on_accepted(self):
QApplication.setOverrideCursor(Qt.WaitCursor)
- iq_data, sample_rate = self.parse_csv_file(self.filename, self.ui.comboBoxCSVSeparator.currentText(),
- self.ui.spinBoxIDataColumn.value()-1,
- self.ui.spinBoxQDataColumn.value()-1,
- self.ui.spinBoxTimestampColumn.value()-1)
+ iq_data, sample_rate = self.parse_csv_file(
+ self.filename,
+ self.ui.comboBoxCSVSeparator.currentText(),
+ self.ui.spinBoxIDataColumn.value() - 1,
+ self.ui.spinBoxQDataColumn.value() - 1,
+ self.ui.spinBoxTimestampColumn.value() - 1,
+ )
target_filename = self.filename.rstrip(".csv")
if os.path.exists(target_filename + ".complex"):
@@ -227,10 +268,13 @@ def on_accepted(self):
iq_data.tofile(target_filename)
- self.data_imported.emit(target_filename, sample_rate if sample_rate is not None else 0)
+ self.data_imported.emit(
+ target_filename, sample_rate if sample_rate is not None else 0
+ )
QApplication.restoreOverrideCursor()
-if __name__ == '__main__':
+
+if __name__ == "__main__":
app = QApplication(["urh"])
csv_dia = CSVImportDialog()
csv_dia.exec_()
diff --git a/src/urh/controller/dialogs/ContinuousSendDialog.py b/src/urh/controller/dialogs/ContinuousSendDialog.py
index 5865d0f5ef..b24a533e73 100644
--- a/src/urh/controller/dialogs/ContinuousSendDialog.py
+++ b/src/urh/controller/dialogs/ContinuousSendDialog.py
@@ -9,9 +9,23 @@
class ContinuousSendDialog(SendDialog):
- def __init__(self, project_manager, messages, modulators, total_samples: int, parent, testing_mode=False):
- super().__init__(project_manager, modulated_data=None, modulation_msg_indices=None,
- continuous_send_mode=True, parent=parent, testing_mode=testing_mode)
+ def __init__(
+ self,
+ project_manager,
+ messages,
+ modulators,
+ total_samples: int,
+ parent,
+ testing_mode=False,
+ ):
+ super().__init__(
+ project_manager,
+ modulated_data=None,
+ modulation_msg_indices=None,
+ continuous_send_mode=True,
+ parent=parent,
+ testing_mode=testing_mode,
+ )
self.messages = messages
self.modulators = modulators
@@ -24,8 +38,12 @@ def __init__(self, project_manager, messages, modulators, total_samples: int, pa
self.ui.progressBarMessage.setMaximum(len(messages))
num_repeats = self.device_settings_widget.ui.spinBoxNRepeat.value()
- self.continuous_modulator = ContinuousModulator(messages, modulators, num_repeats=num_repeats)
- self.scene_manager = ContinuousSceneManager(ring_buffer=self.continuous_modulator.ring_buffer, parent=self)
+ self.continuous_modulator = ContinuousModulator(
+ messages, modulators, num_repeats=num_repeats
+ )
+ self.scene_manager = ContinuousSceneManager(
+ ring_buffer=self.continuous_modulator.ring_buffer, parent=self
+ )
self.scene_manager.init_scene()
self.graphics_view.setScene(self.scene_manager.scene)
self.graphics_view.scene_manager = self.scene_manager
@@ -44,7 +62,9 @@ def _update_send_indicator(self, width: int):
def update_view(self):
super().update_view()
- self.ui.progressBarMessage.setValue(self.continuous_modulator.current_message_index.value + 1)
+ self.ui.progressBarMessage.setValue(
+ self.continuous_modulator.current_message_index.value + 1
+ )
self.scene_manager.init_scene()
self.scene_manager.show_full_scene()
self.graphics_view.update()
@@ -86,7 +106,9 @@ def on_clear_clicked(self):
@pyqtSlot()
def on_num_repeats_changed(self):
super().on_num_repeats_changed()
- self.continuous_modulator.num_repeats = self.device_settings_widget.ui.spinBoxNRepeat.value()
+ self.continuous_modulator.num_repeats = (
+ self.device_settings_widget.ui.spinBoxNRepeat.value()
+ )
def on_selected_device_changed(self):
self.ui.txtEditErrors.clear()
@@ -96,13 +118,21 @@ def init_device(self):
device_name = self.selected_device_name
num_repeats = self.device_settings_widget.ui.spinBoxNRepeat.value()
- self.device = VirtualDevice(self.backend_handler, device_name, Mode.send,
- device_ip="192.168.10.2", sending_repeats=num_repeats, parent=self)
+ self.device = VirtualDevice(
+ self.backend_handler,
+ device_name,
+ Mode.send,
+ device_ip="192.168.10.2",
+ sending_repeats=num_repeats,
+ parent=self,
+ )
self.ui.btnStart.setEnabled(True)
try:
self.device.is_send_continuous = True
- self.device.continuous_send_ring_buffer = self.continuous_modulator.ring_buffer
+ self.device.continuous_send_ring_buffer = (
+ self.continuous_modulator.ring_buffer
+ )
self.device.num_samples_to_send = self.total_samples
self._create_device_connects()
diff --git a/src/urh/controller/dialogs/CostaOptionsDialog.py b/src/urh/controller/dialogs/CostaOptionsDialog.py
index 39c9709a54..626bf58cdb 100644
--- a/src/urh/controller/dialogs/CostaOptionsDialog.py
+++ b/src/urh/controller/dialogs/CostaOptionsDialog.py
@@ -20,7 +20,9 @@ def __init__(self, loop_bandwidth, parent=None):
def create_connects(self):
self.ui.buttonBox.accepted.connect(self.accept)
self.ui.buttonBox.rejected.connect(self.reject)
- self.ui.doubleSpinBoxLoopBandwidth.valueChanged.connect(self.on_spinbox_loop_bandwidth_value_changed)
+ self.ui.doubleSpinBoxLoopBandwidth.valueChanged.connect(
+ self.on_spinbox_loop_bandwidth_value_changed
+ )
@pyqtSlot(float)
def on_spinbox_loop_bandwidth_value_changed(self, value):
diff --git a/src/urh/controller/dialogs/DecoderDialog.py b/src/urh/controller/dialogs/DecoderDialog.py
index bdf976527a..ed22be29ca 100644
--- a/src/urh/controller/dialogs/DecoderDialog.py
+++ b/src/urh/controller/dialogs/DecoderDialog.py
@@ -3,8 +3,14 @@
from PyQt5.QtCore import QDir, Qt, pyqtSlot
from PyQt5.QtGui import QCloseEvent, QDropEvent, QDragEnterEvent, QIcon
-from PyQt5.QtWidgets import QDialog, QTableWidgetItem, QFileDialog, QInputDialog, \
- QLineEdit, QMessageBox
+from PyQt5.QtWidgets import (
+ QDialog,
+ QTableWidgetItem,
+ QFileDialog,
+ QInputDialog,
+ QLineEdit,
+ QMessageBox,
+)
from urh import settings
from urh.signalprocessing.Encoding import Encoding
@@ -16,8 +22,9 @@
class DecoderDialog(QDialog):
- def __init__(self, decodings, signals, project_manager: ProjectManager,
- parent=None):
+ def __init__(
+ self, decodings, signals, project_manager: ProjectManager, parent=None
+ ):
"""
:type decodings: list of Encoding
:type signals: list of Signal
@@ -81,7 +88,7 @@ def __init__(self, decodings, signals, project_manager: ProjectManager,
self.ui.substitution.setColumnCount(2)
self.ui.substitution.setRowCount(self.ui.substitution_rows.value())
- self.ui.substitution.setHorizontalHeaderLabels(['From', 'To'])
+ self.ui.substitution.setHorizontalHeaderLabels(["From", "To"])
self.ui.substitution.setColumnWidth(0, 190)
self.ui.substitution.setColumnWidth(1, 190)
@@ -90,13 +97,17 @@ def __init__(self, decodings, signals, project_manager: ProjectManager,
# Connects
self.create_connects()
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
def create_connects(self):
self.ui.inpt.textChanged.connect(self.decoder_update)
self.ui.multiple.valueChanged.connect(self.handle_multiple_changed)
self.ui.carrier.textChanged.connect(self.handle_carrier_changed)
- self.ui.substitution_rows.valueChanged.connect(self.handle_substitution_rows_changed)
+ self.ui.substitution_rows.valueChanged.connect(
+ self.handle_substitution_rows_changed
+ )
self.ui.substitution.itemChanged.connect(self.handle_substitution_changed)
self.ui.btnChooseDecoder.clicked.connect(self.choose_decoder)
@@ -111,10 +122,18 @@ def create_connects(self):
self.ui.decoderchain.itemChanged.connect(self.decoderchainUpdate)
self.ui.decoderchain.internalMove.connect(self.decoderchainUpdate)
self.ui.decoderchain.deleteElement.connect(self.deleteElement)
- self.ui.decoderchain.currentRowChanged.connect(self.on_decoder_chain_current_row_changed)
- self.ui.basefunctions.currentRowChanged.connect(self.on_base_functions_current_row_changed)
- self.ui.additionalfunctions.currentRowChanged.connect(self.on_additional_functions_current_row_changed)
- self.ui.btnAddtoYourDecoding.clicked.connect(self.on_btn_add_to_your_decoding_clicked)
+ self.ui.decoderchain.currentRowChanged.connect(
+ self.on_decoder_chain_current_row_changed
+ )
+ self.ui.basefunctions.currentRowChanged.connect(
+ self.on_base_functions_current_row_changed
+ )
+ self.ui.additionalfunctions.currentRowChanged.connect(
+ self.on_additional_functions_current_row_changed
+ )
+ self.ui.btnAddtoYourDecoding.clicked.connect(
+ self.on_btn_add_to_your_decoding_clicked
+ )
self.ui.combobox_decodings.currentIndexChanged.connect(self.set_e)
self.ui.combobox_signals.currentIndexChanged.connect(self.set_signal)
@@ -133,17 +152,23 @@ def create_connects(self):
self.ui.morse_wait.valueChanged.connect(self.handle_morse_changed)
def closeEvent(self, event: QCloseEvent):
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
def choose_decoder(self):
- f, ok = QFileDialog.getOpenFileName(self, self.tr("Choose decoder program"), QDir.homePath())
+ f, ok = QFileDialog.getOpenFileName(
+ self, self.tr("Choose decoder program"), QDir.homePath()
+ )
if f and ok:
self.ui.external_decoder.setText(f)
self.handle_external()
def choose_encoder(self):
- f, ok = QFileDialog.getOpenFileName(self, self.tr("Choose encoder program"), QDir.homePath())
+ f, ok = QFileDialog.getOpenFileName(
+ self, self.tr("Choose encoder program"), QDir.homePath()
+ )
if f and ok:
self.ui.external_encoder.setText(f)
self.handle_external()
@@ -152,7 +177,9 @@ def save_to_file(self):
if self.project_manager.project_file:
self.project_manager.decodings = self.decodings
else:
- prefix = os.path.realpath(os.path.join(settings.get_qt_settings_filename(), ".."))
+ prefix = os.path.realpath(
+ os.path.join(settings.get_qt_settings_filename(), "..")
+ )
with open(os.path.join(prefix, settings.DECODINGS_FILE), "w") as f:
for i in range(0, self.ui.combobox_decodings.count()):
str = ""
@@ -163,8 +190,13 @@ def save_to_file(self):
def saveas(self):
# Ask for a name
- name, ok = QInputDialog.getText(self, self.tr("Save decoding"),
- self.tr("Please enter a name:"), QLineEdit.Normal, self.e.chain[0])
+ name, ok = QInputDialog.getText(
+ self,
+ self.tr("Save decoding"),
+ self.tr("Please enter a name:"),
+ QLineEdit.Normal,
+ self.e.chain[0],
+ )
if ok and name != "":
self.e.chain[0] = name
@@ -182,17 +214,24 @@ def saveas(self):
self.decodings.append(Encoding(self.chainstr))
self.ui.combobox_decodings.addItem(self.chainstr[0])
- self.ui.combobox_decodings.setCurrentIndex(self.ui.combobox_decodings.count() - 1)
+ self.ui.combobox_decodings.setCurrentIndex(
+ self.ui.combobox_decodings.count() - 1
+ )
self.set_e()
self.save_to_file()
def delete_decoding(self):
num = self.ui.combobox_decodings.currentIndex()
if num >= 0:
- reply = QMessageBox.question(self, self.tr("Delete Decoding?"),
- self.tr("Do you really want to delete " + "'{}'?".format(
- self.decodings[num].name)),
- QMessageBox.Yes | QMessageBox.No)
+ reply = QMessageBox.question(
+ self,
+ self.tr("Delete Decoding?"),
+ self.tr(
+ "Do you really want to delete "
+ + "'{}'?".format(self.decodings[num].name)
+ ),
+ QMessageBox.Yes | QMessageBox.No,
+ )
if reply == QMessageBox.Yes:
self.decodings.pop(num)
@@ -203,26 +242,48 @@ def set_e(self):
if self.ui.combobox_decodings.count() < 1: # Empty list
return
- self.e = copy.deepcopy(self.decodings[self.ui.combobox_decodings.currentIndex()])
+ self.e = copy.deepcopy(
+ self.decodings[self.ui.combobox_decodings.currentIndex()]
+ )
""":type: encoding """
chain = self.e.get_chain()
self.ui.decoderchain.clear()
self.chainoptions.clear()
last_i = ""
for i in chain:
- if i in [settings.DECODING_INVERT, settings.DECODING_ENOCEAN, settings.DECODING_DIFFERENTIAL,
- settings.DECODING_REDUNDANCY, settings.DECODING_CARRIER, settings.DECODING_BITORDER,
- settings.DECODING_EDGE, settings.DECODING_DATAWHITENING, settings.DECODING_SUBSTITUTION,
- settings.DECODING_EXTERNAL, settings.DECODING_CUT, settings.DECODING_MORSE,
- settings.DECODING_DISABLED_PREFIX]:
+ if i in [
+ settings.DECODING_INVERT,
+ settings.DECODING_ENOCEAN,
+ settings.DECODING_DIFFERENTIAL,
+ settings.DECODING_REDUNDANCY,
+ settings.DECODING_CARRIER,
+ settings.DECODING_BITORDER,
+ settings.DECODING_EDGE,
+ settings.DECODING_DATAWHITENING,
+ settings.DECODING_SUBSTITUTION,
+ settings.DECODING_EXTERNAL,
+ settings.DECODING_CUT,
+ settings.DECODING_MORSE,
+ settings.DECODING_DISABLED_PREFIX,
+ ]:
self.ui.decoderchain.addItem(i)
self.decoderchainUpdate()
- last_i = self.ui.decoderchain.item(self.ui.decoderchain.count() - 1).text()
+ last_i = self.ui.decoderchain.item(
+ self.ui.decoderchain.count() - 1
+ ).text()
else:
- if any(x in last_i for x in [settings.DECODING_REDUNDANCY, settings.DECODING_CARRIER,
- settings.DECODING_SUBSTITUTION, settings.DECODING_EXTERNAL,
- settings.DECODING_DATAWHITENING, settings.DECODING_CUT,
- settings.DECODING_MORSE]):
+ if any(
+ x in last_i
+ for x in [
+ settings.DECODING_REDUNDANCY,
+ settings.DECODING_CARRIER,
+ settings.DECODING_SUBSTITUTION,
+ settings.DECODING_EXTERNAL,
+ settings.DECODING_DATAWHITENING,
+ settings.DECODING_CUT,
+ settings.DECODING_MORSE,
+ ]
+ ):
self.chainoptions[last_i] = i
self.decoderchainUpdate()
@@ -321,7 +382,10 @@ def eliminateDuplicates(self):
if decoderchain_count > 1 and decoderchain_count > olddecoderchain_count:
elem = 0
while elem < olddecoderchain_count:
- if self.ui.decoderchain.item(elem).text() == self.old_decoderchain[elem]:
+ if (
+ self.ui.decoderchain.item(elem).text()
+ == self.old_decoderchain[elem]
+ ):
elem += 1
else:
break
@@ -454,17 +518,21 @@ def set_information(self, mode: int):
# Remove "[Disabled] " for further tasks
if settings.DECODING_DISABLED_PREFIX in element:
- element = element[len(settings.DECODING_DISABLED_PREFIX):]
+ element = element[len(settings.DECODING_DISABLED_PREFIX) :]
# Write info text and show options
if settings.DECODING_EDGE in element:
- txt += "Trigger on signal edge, i.e. the transition between low and high.\n" \
- "- Low to High (01) is 1\n" \
- "- High to Low (10) is 0"
+ txt += (
+ "Trigger on signal edge, i.e. the transition between low and high.\n"
+ "- Low to High (01) is 1\n"
+ "- High to Low (10) is 0"
+ )
elif settings.DECODING_SUBSTITUTION in element:
- txt += "A set of manual defined signal sequences FROM (e.g. 110, 100) is replaced by another set of " \
- "sequences TO (e.g. 01, 10). Note that all FROM entries must have the same length, otherwise " \
- "the result is unpredictable! (For TX: all TO entries must have the same length)"
+ txt += (
+ "A set of manual defined signal sequences FROM (e.g. 110, 100) is replaced by another set of "
+ "sequences TO (e.g. 01, 10). Note that all FROM entries must have the same length, otherwise "
+ "the result is unpredictable! (For TX: all TO entries must have the same length)"
+ )
self.ui.optionWidget.setCurrentIndex(3)
# Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -484,8 +552,12 @@ def set_information(self, mode: int):
self.ui.substitution_rows.setValue(len(arrs[0]))
self.ui.substitution.setRowCount(len(arrs[0]))
for i in range(0, len(arrs[0])):
- self.ui.substitution.setItem(i, 0, QTableWidgetItem(self.e.bit2str(arrs[0][i])))
- self.ui.substitution.setItem(i, 1, QTableWidgetItem(self.e.bit2str(arrs[1][i])))
+ self.ui.substitution.setItem(
+ i, 0, QTableWidgetItem(self.e.bit2str(arrs[0][i]))
+ )
+ self.ui.substitution.setItem(
+ i, 1, QTableWidgetItem(self.e.bit2str(arrs[1][i]))
+ )
else:
self.ui.substitution_rows.setValue(4)
self.ui.substitution.setRowCount(0)
@@ -494,9 +566,11 @@ def set_information(self, mode: int):
self.ui.substitution_rows.setEnabled(decoderEdit)
elif settings.DECODING_EXTERNAL in element:
- txt += "The decoding (and encoding) process is delegated to external programs or scripts via parameter.\n" \
- "Example: Given the signal 10010110, your program is called as './decoder 10010110'. Your program " \
- "computes and prints a corresponding set of 0s and 1s which is fed back into the decoding process. "
+ txt += (
+ "The decoding (and encoding) process is delegated to external programs or scripts via parameter.\n"
+ "Example: Given the signal 10010110, your program is called as './decoder 10010110'. Your program "
+ "computes and prints a corresponding set of 0s and 1s which is fed back into the decoding process. "
+ )
self.ui.optionWidget.setCurrentIndex(4)
# Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -525,15 +599,21 @@ def set_information(self, mode: int):
elif settings.DECODING_ENOCEAN in element:
txt += "Remove Wireless Short-Packet (WSP) encoding that is used by EnOcean standard."
elif settings.DECODING_DIFFERENTIAL in element:
- txt += "Every transition between low and high (0->1 or 1->0) becomes 1, no transition (0->0 or 1->1) remains 0.\n" \
- "The first signal bit is regarded as start value and directly copied.\n" \
- "Example: 0011 becomes 0010 [0|(0->0)|(0->1)|(1->1)]."
+ txt += (
+ "Every transition between low and high (0->1 or 1->0) becomes 1, no transition (0->0 or 1->1) remains 0.\n"
+ "The first signal bit is regarded as start value and directly copied.\n"
+ "Example: 0011 becomes 0010 [0|(0->0)|(0->1)|(1->1)]."
+ )
elif settings.DECODING_BITORDER in element:
- txt += "Every byte (8 bit) is reversed, i.e. the order of the bits 01234567 (e.g. least significant bit first) " \
- "is changed to 76543210 (e.g. most significant bit first)."
+ txt += (
+ "Every byte (8 bit) is reversed, i.e. the order of the bits 01234567 (e.g. least significant bit first) "
+ "is changed to 76543210 (e.g. most significant bit first)."
+ )
elif settings.DECODING_REDUNDANCY in element:
- txt += "If the source signal always has multiple redundant bits for one bit (e.g. 1111=1, 0000=0), the " \
- "redundancy is removed here. You have to define the number of redundant bits."
+ txt += (
+ "If the source signal always has multiple redundant bits for one bit (e.g. 1111=1, 0000=0), the "
+ "redundancy is removed here. You have to define the number of redundant bits."
+ )
self.ui.optionWidget.setCurrentIndex(1)
# Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -549,9 +629,11 @@ def set_information(self, mode: int):
self.ui.multiple.setValue(2)
self.ui.multiple.setEnabled(decoderEdit)
elif settings.DECODING_MORSE in element:
- txt += "If the signal is a morse code, e.g. 00111001001110011100, where information are " \
- "transported with long and short sequences of 1 (0 just for padding), then this " \
- "decoding evaluates those sequences (Example output: 1011)."
+ txt += (
+ "If the signal is a morse code, e.g. 00111001001110011100, where information are "
+ "transported with long and short sequences of 1 (0 just for padding), then this "
+ "decoding evaluates those sequences (Example output: 1011)."
+ )
self.ui.optionWidget.setCurrentIndex(7)
# # Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -583,11 +665,13 @@ def set_information(self, mode: int):
self.ui.morse_high.setEnabled(decoderEdit)
self.ui.morse_wait.setEnabled(decoderEdit)
elif settings.DECODING_CARRIER in element:
- txt += "A carrier is a fixed pattern like 1_1_1_1 where the actual data lies in between, e.g. 1a1a1b1. This " \
- "function extracts the actual bit information (here: aab) from the signal at '_'/'.' positions.\n" \
- "Examples:\n" \
- "- Carrier = '1_' means 1_1_1_...\n" \
- "- Carrier = '01_' means 01_01_01_01..."
+ txt += (
+ "A carrier is a fixed pattern like 1_1_1_1 where the actual data lies in between, e.g. 1a1a1b1. This "
+ "function extracts the actual bit information (here: aab) from the signal at '_'/'.' positions.\n"
+ "Examples:\n"
+ "- Carrier = '1_' means 1_1_1_...\n"
+ "- Carrier = '01_' means 01_01_01_01..."
+ )
self.ui.optionWidget.setCurrentIndex(2)
# Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -603,10 +687,12 @@ def set_information(self, mode: int):
self.ui.carrier.setText("1_")
self.ui.carrier.setEnabled(decoderEdit)
elif settings.DECODING_DATAWHITENING in element:
- txt += "Texas Instruments CC110x chips allow a data whitening that is applied before sending the signals to HF. " \
- "After a preamble (1010...) there is a fixed 16/32 bit sync word. The following data (incl. 16 bit CRC) " \
- "is masked (XOR) with the output of a LFSR.\n" \
- "This unmasks the data."
+ txt += (
+ "Texas Instruments CC110x chips allow a data whitening that is applied before sending the signals to HF. "
+ "After a preamble (1010...) there is a fixed 16/32 bit sync word. The following data (incl. 16 bit CRC) "
+ "is masked (XOR) with the output of a LFSR.\n"
+ "This unmasks the data."
+ )
self.ui.optionWidget.setCurrentIndex(5)
# Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -622,10 +708,18 @@ def set_information(self, mode: int):
self.ui.datawhitening_overwrite_crc.setChecked(False)
else:
try:
- whitening_sync, whitening_polynomial, whitening_overwrite_crc = value.split(";")
+ (
+ whitening_sync,
+ whitening_polynomial,
+ whitening_overwrite_crc,
+ ) = value.split(";")
self.ui.datawhitening_sync.setText(whitening_sync)
- self.ui.datawhitening_polynomial.setText(whitening_polynomial)
- self.ui.datawhitening_overwrite_crc.setChecked(True if whitening_overwrite_crc == "1" else False)
+ self.ui.datawhitening_polynomial.setText(
+ whitening_polynomial
+ )
+ self.ui.datawhitening_overwrite_crc.setChecked(
+ True if whitening_overwrite_crc == "1" else False
+ )
except ValueError:
self.ui.datawhitening_sync.setText("0xe9cae9ca")
@@ -637,11 +731,13 @@ def set_information(self, mode: int):
self.ui.datawhitening_overwrite_crc.setEnabled(decoderEdit)
elif settings.DECODING_CUT in element:
- txt += "This function enables you to cut data from your messages, in order to shorten or align them for a " \
- "better view. Note that this decoding does NOT support encoding, because cut data is gone!\n" \
- "Example:\n" \
- "- Cut before '1010' would delete everything before first '1010' bits.\n" \
- "- Cut before Position = 3 (in bit) would delete the first three bits.\n"
+ txt += (
+ "This function enables you to cut data from your messages, in order to shorten or align them for a "
+ "better view. Note that this decoding does NOT support encoding, because cut data is gone!\n"
+ "Example:\n"
+ "- Cut before '1010' would delete everything before first '1010' bits.\n"
+ "- Cut before Position = 3 (in bit) would delete the first three bits.\n"
+ )
self.ui.optionWidget.setCurrentIndex(6)
# Values can only be changed when editing decoder, otherwise default value
if not decoderEdit:
@@ -721,15 +817,22 @@ def set_information(self, mode: int):
@pyqtSlot()
def handle_datawhitening(self):
- datawhiteningstr = self.ui.datawhitening_sync.text() + ";" + self.ui.datawhitening_polynomial.text() + ";" + \
- ("1" if self.ui.datawhitening_overwrite_crc.isChecked() else "0")
+ datawhiteningstr = (
+ self.ui.datawhitening_sync.text()
+ + ";"
+ + self.ui.datawhitening_polynomial.text()
+ + ";"
+ + ("1" if self.ui.datawhitening_overwrite_crc.isChecked() else "0")
+ )
if settings.DECODING_DATAWHITENING in self.active_message:
self.chainoptions[self.active_message] = datawhiteningstr
self.decoderchainUpdate()
@pyqtSlot()
def handle_external(self):
- externalstr = self.ui.external_decoder.text() + ";" + self.ui.external_encoder.text()
+ externalstr = (
+ self.ui.external_decoder.text() + ";" + self.ui.external_encoder.text()
+ )
if settings.DECODING_EXTERNAL in self.active_message:
self.chainoptions[self.active_message] = externalstr
self.decoderchainUpdate()
@@ -739,7 +842,12 @@ def handle_substitution_changed(self):
subststr = ""
for i in range(0, self.ui.substitution_rows.value()):
if self.ui.substitution.item(i, 0) and self.ui.substitution.item(i, 1):
- subststr += self.ui.substitution.item(i, 0).text() + ":" + self.ui.substitution.item(i, 1).text() + ";"
+ subststr += (
+ self.ui.substitution.item(i, 0).text()
+ + ":"
+ + self.ui.substitution.item(i, 1).text()
+ + ";"
+ )
if settings.DECODING_SUBSTITUTION in self.active_message:
self.chainoptions[self.active_message] = subststr
self.decoderchainUpdate()
@@ -773,15 +881,18 @@ def handle_morse_changed(self):
self.old_morse = (val_low, val_high)
if settings.DECODING_MORSE in self.active_message:
- self.chainoptions[self.active_message] = "{};{};{}".format(val_low, val_high, val_wait)
+ self.chainoptions[self.active_message] = "{};{};{}".format(
+ val_low, val_high, val_wait
+ )
self.decoderchainUpdate()
@pyqtSlot()
def handle_carrier_changed(self):
# Only allow {0, 1}
carrier_txt = self.ui.carrier.text()
- if carrier_txt.count("0") + carrier_txt.count("1") + carrier_txt.count("_") + carrier_txt.count(
- ".") + carrier_txt.count("*") < len(carrier_txt):
+ if carrier_txt.count("0") + carrier_txt.count("1") + carrier_txt.count(
+ "_"
+ ) + carrier_txt.count(".") + carrier_txt.count("*") < len(carrier_txt):
self.ui.carrier.setText(self.old_carrier_txt)
else:
self.old_carrier_txt = carrier_txt
@@ -830,7 +941,7 @@ def on_btn_add_to_your_decoding_clicked(self):
if self.last_selected_item != "":
self.ui.decoderchain.addItem(self.last_selected_item)
self.decoderchainUpdate()
- self.ui.decoderchain.setCurrentRow(self.ui.decoderchain.count()-1)
+ self.ui.decoderchain.setCurrentRow(self.ui.decoderchain.count() - 1)
def dragEnterEvent(self, event: QDragEnterEvent):
event.accept()
@@ -864,9 +975,10 @@ def set_signal(self):
last_message = pa.messages[-1]
lookup = {i: msg.bit_sample_pos for i, msg in enumerate(pa.messages)}
- plot_data = signal.qad[lookup[0][0]:lookup[pa.num_messages - 1][len(last_message) - 1]]
+ plot_data = signal.qad[
+ lookup[0][0] : lookup[pa.num_messages - 1][len(last_message) - 1]
+ ]
self.ui.graphicsView_signal.plot_data(plot_data)
self.ui.graphicsView_signal.centerOn(0, 0)
self.unsetCursor()
-
diff --git a/src/urh/controller/dialogs/FilterBandwidthDialog.py b/src/urh/controller/dialogs/FilterBandwidthDialog.py
index 5ccae1b6e3..191a5ad6f9 100644
--- a/src/urh/controller/dialogs/FilterBandwidthDialog.py
+++ b/src/urh/controller/dialogs/FilterBandwidthDialog.py
@@ -20,22 +20,44 @@ def __init__(self, parent=None):
item = getattr(self.ui, item)
if isinstance(item, QLabel):
name = item.objectName().replace("label", "")
- key = next((key for key in Filter.BANDWIDTHS.keys() if name.startswith(key.replace(" ", ""))), None)
+ key = next(
+ (
+ key
+ for key in Filter.BANDWIDTHS.keys()
+ if name.startswith(key.replace(" ", ""))
+ ),
+ None,
+ )
if key is not None and name.endswith("Bandwidth"):
item.setText("{0:n}".format(Filter.BANDWIDTHS[key]))
elif key is not None and name.endswith("KernelLength"):
- item.setText(str(Filter.get_filter_length_from_bandwidth(Filter.BANDWIDTHS[key])))
+ item.setText(
+ str(
+ Filter.get_filter_length_from_bandwidth(
+ Filter.BANDWIDTHS[key]
+ )
+ )
+ )
elif isinstance(item, QRadioButton):
- item.setChecked(bw_type.replace(" ", "_") == item.objectName().replace("radioButton", ""))
+ item.setChecked(
+ bw_type.replace(" ", "_")
+ == item.objectName().replace("radioButton", "")
+ )
self.ui.doubleSpinBoxCustomBandwidth.setValue(custom_bw)
- self.ui.spinBoxCustomKernelLength.setValue(Filter.get_filter_length_from_bandwidth(custom_bw))
+ self.ui.spinBoxCustomKernelLength.setValue(
+ Filter.get_filter_length_from_bandwidth(custom_bw)
+ )
self.create_connects()
def create_connects(self):
- self.ui.doubleSpinBoxCustomBandwidth.valueChanged.connect(self.on_spin_box_custom_bandwidth_value_changed)
- self.ui.spinBoxCustomKernelLength.valueChanged.connect(self.on_spin_box_custom_kernel_length_value_changed)
+ self.ui.doubleSpinBoxCustomBandwidth.valueChanged.connect(
+ self.on_spin_box_custom_bandwidth_value_changed
+ )
+ self.ui.spinBoxCustomKernelLength.valueChanged.connect(
+ self.on_spin_box_custom_kernel_length_value_changed
+ )
self.ui.buttonBox.accepted.connect(self.on_accepted)
@property
@@ -49,19 +71,29 @@ def checked_radiobutton(self):
@pyqtSlot(float)
def on_spin_box_custom_bandwidth_value_changed(self, bw: float):
self.ui.spinBoxCustomKernelLength.blockSignals(True)
- self.ui.spinBoxCustomKernelLength.setValue(Filter.get_filter_length_from_bandwidth(bw))
+ self.ui.spinBoxCustomKernelLength.setValue(
+ Filter.get_filter_length_from_bandwidth(bw)
+ )
self.ui.spinBoxCustomKernelLength.blockSignals(False)
@pyqtSlot(int)
def on_spin_box_custom_kernel_length_value_changed(self, filter_len: int):
self.ui.doubleSpinBoxCustomBandwidth.blockSignals(True)
- self.ui.doubleSpinBoxCustomBandwidth.setValue(Filter.get_bandwidth_from_filter_length(filter_len))
+ self.ui.doubleSpinBoxCustomBandwidth.setValue(
+ Filter.get_bandwidth_from_filter_length(filter_len)
+ )
self.ui.doubleSpinBoxCustomBandwidth.blockSignals(False)
@pyqtSlot()
def on_accepted(self):
if self.checked_radiobutton is not None:
- bw_type = self.checked_radiobutton.objectName().replace("radioButton", "").replace("_", " ")
+ bw_type = (
+ self.checked_radiobutton.objectName()
+ .replace("radioButton", "")
+ .replace("_", " ")
+ )
settings.write("bandpass_filter_bw_type", bw_type)
- settings.write("bandpass_filter_custom_bw", self.ui.doubleSpinBoxCustomBandwidth.value())
+ settings.write(
+ "bandpass_filter_custom_bw", self.ui.doubleSpinBoxCustomBandwidth.value()
+ )
diff --git a/src/urh/controller/dialogs/FilterDialog.py b/src/urh/controller/dialogs/FilterDialog.py
index bf60d68cbb..f2ad87367c 100644
--- a/src/urh/controller/dialogs/FilterDialog.py
+++ b/src/urh/controller/dialogs/FilterDialog.py
@@ -34,9 +34,15 @@ def set_dsp_filter_status(self, dsp_filter_type: FilterType):
self.ui.lineEditCustomTaps.setEnabled(True)
def create_connects(self):
- self.ui.radioButtonMovingAverage.clicked.connect(self.on_radio_button_moving_average_clicked)
- self.ui.radioButtonCustomTaps.clicked.connect(self.on_radio_button_custom_taps_clicked)
- self.ui.radioButtonDCcorrection.clicked.connect(self.on_radio_button_dc_correction_clicked)
+ self.ui.radioButtonMovingAverage.clicked.connect(
+ self.on_radio_button_moving_average_clicked
+ )
+ self.ui.radioButtonCustomTaps.clicked.connect(
+ self.on_radio_button_custom_taps_clicked
+ )
+ self.ui.radioButtonDCcorrection.clicked.connect(
+ self.on_radio_button_dc_correction_clicked
+ )
self.ui.spinBoxNumTaps.valueChanged.connect(self.set_error_status)
self.ui.lineEditCustomTaps.textEdited.connect(self.set_error_status)
@@ -46,7 +52,9 @@ def create_connects(self):
def build_filter(self) -> Filter:
if self.ui.radioButtonMovingAverage.isChecked():
n = self.ui.spinBoxNumTaps.value()
- return Filter([1/n for _ in range(n)], filter_type=FilterType.moving_average)
+ return Filter(
+ [1 / n for _ in range(n)], filter_type=FilterType.moving_average
+ )
elif self.ui.radioButtonDCcorrection.isChecked():
return Filter([], filter_type=FilterType.dc_correction)
else:
@@ -72,7 +80,9 @@ def set_error_status(self):
self.ui.lineEditCustomTaps.setToolTip(self.error_message)
elif len(dsp_filter.taps) != self.ui.spinBoxNumTaps.value():
self.ui.lineEditCustomTaps.setStyleSheet("background: yellow")
- self.ui.lineEditCustomTaps.setToolTip("The number of the filter taps does not match the configured number of taps. I will use your configured filter taps.")
+ self.ui.lineEditCustomTaps.setToolTip(
+ "The number of the filter taps does not match the configured number of taps. I will use your configured filter taps."
+ )
else:
self.ui.lineEditCustomTaps.setStyleSheet("")
self.ui.lineEditCustomTaps.setToolTip("")
diff --git a/src/urh/controller/dialogs/FuzzingDialog.py b/src/urh/controller/dialogs/FuzzingDialog.py
index f06543589d..63df280b60 100644
--- a/src/urh/controller/dialogs/FuzzingDialog.py
+++ b/src/urh/controller/dialogs/FuzzingDialog.py
@@ -12,8 +12,14 @@
class FuzzingDialog(QDialog):
- def __init__(self, protocol: ProtocolAnalyzerContainer, label_index: int, msg_index: int, proto_view: int,
- parent=None):
+ def __init__(
+ self,
+ protocol: ProtocolAnalyzerContainer,
+ label_index: int,
+ msg_index: int,
+ proto_view: int,
+ parent=None,
+ ):
super().__init__(parent)
self.ui = Ui_FuzzingDialog()
self.ui.setupUi(self)
@@ -26,12 +32,16 @@ def __init__(self, protocol: ProtocolAnalyzerContainer, label_index: int, msg_in
self.ui.spinBoxFuzzMessage.setMinimum(1)
self.ui.spinBoxFuzzMessage.setMaximum(self.protocol.num_messages)
- self.ui.comboBoxFuzzingLabel.addItems([l.name for l in self.message.message_type])
+ self.ui.comboBoxFuzzingLabel.addItems(
+ [l.name for l in self.message.message_type]
+ )
self.ui.comboBoxFuzzingLabel.setCurrentIndex(label_index)
self.proto_view = proto_view
self.fuzz_table_model = FuzzingTableModel(self.current_label, proto_view)
- self.fuzz_table_model.remove_duplicates = self.ui.chkBRemoveDuplicates.isChecked()
+ self.fuzz_table_model.remove_duplicates = (
+ self.ui.chkBRemoveDuplicates.isChecked()
+ )
self.ui.tblFuzzingValues.setModel(self.fuzz_table_model)
self.fuzz_table_model.update()
@@ -44,7 +54,9 @@ def __init__(self, protocol: ProtocolAnalyzerContainer, label_index: int, msg_in
self.ui.tblFuzzingValues.resize_me()
self.create_connects()
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
@property
def message(self):
@@ -61,23 +73,31 @@ def current_label(self) -> ProtocolLabel:
cur_label = self.message.message_type[self.current_label_index].get_copy()
self.message.message_type[self.current_label_index] = cur_label
- cur_label.fuzz_values = [fv for fv in cur_label.fuzz_values if fv] # Remove empty strings
+ cur_label.fuzz_values = [
+ fv for fv in cur_label.fuzz_values if fv
+ ] # Remove empty strings
if len(cur_label.fuzz_values) == 0:
- cur_label.fuzz_values.append(self.message.plain_bits_str[cur_label.start:cur_label.end])
+ cur_label.fuzz_values.append(
+ self.message.plain_bits_str[cur_label.start : cur_label.end]
+ )
return cur_label
@property
def current_label_start(self):
if self.current_label and self.message:
- return self.message.get_label_range(self.current_label, self.proto_view, False)[0]
+ return self.message.get_label_range(
+ self.current_label, self.proto_view, False
+ )[0]
else:
return -1
@property
def current_label_end(self):
if self.current_label and self.message:
- return self.message.get_label_range(self.current_label, self.proto_view, False)[1]
+ return self.message.get_label_range(
+ self.current_label, self.proto_view, False
+ )[1]
else:
return -1
@@ -95,23 +115,41 @@ def message_data(self):
def create_connects(self):
self.ui.spinBoxFuzzingStart.valueChanged.connect(self.on_fuzzing_start_changed)
self.ui.spinBoxFuzzingEnd.valueChanged.connect(self.on_fuzzing_end_changed)
- self.ui.comboBoxFuzzingLabel.currentIndexChanged.connect(self.on_combo_box_fuzzing_label_current_index_changed)
+ self.ui.comboBoxFuzzingLabel.currentIndexChanged.connect(
+ self.on_combo_box_fuzzing_label_current_index_changed
+ )
self.ui.btnRepeatValues.clicked.connect(self.on_btn_repeat_values_clicked)
self.ui.btnAddRow.clicked.connect(self.on_btn_add_row_clicked)
self.ui.btnDelRow.clicked.connect(self.on_btn_del_row_clicked)
self.ui.tblFuzzingValues.deletion_wanted.connect(self.delete_lines)
- self.ui.chkBRemoveDuplicates.stateChanged.connect(self.on_remove_duplicates_state_changed)
- self.ui.sBAddRangeStart.valueChanged.connect(self.on_fuzzing_range_start_changed)
+ self.ui.chkBRemoveDuplicates.stateChanged.connect(
+ self.on_remove_duplicates_state_changed
+ )
+ self.ui.sBAddRangeStart.valueChanged.connect(
+ self.on_fuzzing_range_start_changed
+ )
self.ui.sBAddRangeEnd.valueChanged.connect(self.on_fuzzing_range_end_changed)
- self.ui.checkBoxLowerBound.stateChanged.connect(self.on_lower_bound_checked_changed)
- self.ui.checkBoxUpperBound.stateChanged.connect(self.on_upper_bound_checked_changed)
+ self.ui.checkBoxLowerBound.stateChanged.connect(
+ self.on_lower_bound_checked_changed
+ )
+ self.ui.checkBoxUpperBound.stateChanged.connect(
+ self.on_upper_bound_checked_changed
+ )
self.ui.spinBoxLowerBound.valueChanged.connect(self.on_lower_bound_changed)
self.ui.spinBoxUpperBound.valueChanged.connect(self.on_upper_bound_changed)
- self.ui.spinBoxRandomMinimum.valueChanged.connect(self.on_random_range_min_changed)
- self.ui.spinBoxRandomMaximum.valueChanged.connect(self.on_random_range_max_changed)
+ self.ui.spinBoxRandomMinimum.valueChanged.connect(
+ self.on_random_range_min_changed
+ )
+ self.ui.spinBoxRandomMaximum.valueChanged.connect(
+ self.on_random_range_max_changed
+ )
self.ui.spinBoxFuzzMessage.valueChanged.connect(self.on_fuzz_msg_changed)
- self.ui.btnAddFuzzingValues.clicked.connect(self.on_btn_add_fuzzing_values_clicked)
- self.ui.comboBoxFuzzingLabel.editTextChanged.connect(self.set_current_label_name)
+ self.ui.btnAddFuzzingValues.clicked.connect(
+ self.on_btn_add_fuzzing_values_clicked
+ )
+ self.ui.comboBoxFuzzingLabel.editTextChanged.connect(
+ self.set_current_label_name
+ )
def update_message_data_string(self):
fuz_start = self.current_label_start
@@ -136,13 +174,19 @@ def update_message_data_string(self):
fuz_end = fuz_start + num_fuz_bits
fuzamble = "..."
- self.ui.lPreBits.setText(preambel + self.message_data[proto_start:self.current_label_start])
+ self.ui.lPreBits.setText(
+ preambel + self.message_data[proto_start : self.current_label_start]
+ )
self.ui.lFuzzedBits.setText(self.message_data[fuz_start:fuz_end] + fuzamble)
- self.ui.lPostBits.setText(self.message_data[self.current_label_end:proto_end] + postambel)
+ self.ui.lPostBits.setText(
+ self.message_data[self.current_label_end : proto_end] + postambel
+ )
self.set_add_spinboxes_maximum_on_label_change()
def closeEvent(self, event: QCloseEvent):
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
@pyqtSlot(int)
@@ -158,7 +202,9 @@ def on_fuzzing_start_changed(self, value: int):
@pyqtSlot(int)
def on_fuzzing_end_changed(self, value: int):
self.ui.spinBoxFuzzingStart.setMaximum(self.ui.spinBoxFuzzingEnd.value())
- new_end = self.message.convert_index(value - 1, self.proto_view, 0, False)[1] + 1
+ new_end = (
+ self.message.convert_index(value - 1, self.proto_view, 0, False)[1] + 1
+ )
self.current_label.end = new_end
self.current_label.fuzz_values[:] = []
self.update_message_data_string()
@@ -195,8 +241,10 @@ def delete_lines(self, min_row, max_row):
if min_row == -1:
self.current_label.fuzz_values = self.current_label.fuzz_values[:-1]
else:
- self.current_label.fuzz_values = self.current_label.fuzz_values[:min_row] + self.current_label.fuzz_values[
- max_row + 1:]
+ self.current_label.fuzz_values = (
+ self.current_label.fuzz_values[:min_row]
+ + self.current_label.fuzz_values[max_row + 1 :]
+ )
_ = self.current_label # if user deleted all, this will restore a fuzz value
@@ -204,16 +252,20 @@ def delete_lines(self, min_row, max_row):
@pyqtSlot()
def on_remove_duplicates_state_changed(self):
- self.fuzz_table_model.remove_duplicates = self.ui.chkBRemoveDuplicates.isChecked()
+ self.fuzz_table_model.remove_duplicates = (
+ self.ui.chkBRemoveDuplicates.isChecked()
+ )
self.fuzz_table_model.update()
self.remove_duplicates()
@pyqtSlot()
def set_add_spinboxes_maximum_on_label_change(self):
- nbits = self.current_label.end - self.current_label.start # Use Bit Start/End for maximum calc.
+ nbits = (
+ self.current_label.end - self.current_label.start
+ ) # Use Bit Start/End for maximum calc.
if nbits >= 32:
nbits = 31
- max_val = 2 ** nbits - 1
+ max_val = 2**nbits - 1
self.ui.sBAddRangeStart.setMaximum(max_val - 1)
self.ui.sBAddRangeEnd.setMaximum(max_val)
self.ui.sBAddRangeEnd.setValue(max_val)
@@ -261,14 +313,22 @@ def on_upper_bound_checked_changed(self):
@pyqtSlot()
def on_lower_bound_changed(self):
self.ui.spinBoxUpperBound.setMinimum(self.ui.spinBoxLowerBound.value())
- self.ui.spinBoxBoundaryNumber.setMaximum(math.ceil((self.ui.spinBoxUpperBound.value()
- - self.ui.spinBoxLowerBound.value()) / 2))
+ self.ui.spinBoxBoundaryNumber.setMaximum(
+ math.ceil(
+ (self.ui.spinBoxUpperBound.value() - self.ui.spinBoxLowerBound.value())
+ / 2
+ )
+ )
@pyqtSlot()
def on_upper_bound_changed(self):
self.ui.spinBoxLowerBound.setMaximum(self.ui.spinBoxUpperBound.value() - 1)
- self.ui.spinBoxBoundaryNumber.setMaximum(math.ceil((self.ui.spinBoxUpperBound.value()
- - self.ui.spinBoxLowerBound.value()) / 2))
+ self.ui.spinBoxBoundaryNumber.setMaximum(
+ math.ceil(
+ (self.ui.spinBoxUpperBound.value() - self.ui.spinBoxLowerBound.value())
+ / 2
+ )
+ )
@pyqtSlot()
def on_random_range_min_changed(self):
@@ -276,7 +336,9 @@ def on_random_range_min_changed(self):
@pyqtSlot()
def on_random_range_max_changed(self):
- self.ui.spinBoxRandomMinimum.setMaximum(self.ui.spinBoxRandomMaximum.value() - 1)
+ self.ui.spinBoxRandomMinimum.setMaximum(
+ self.ui.spinBoxRandomMaximum.value() - 1
+ )
@pyqtSlot()
def on_btn_add_fuzzing_values_clicked(self):
@@ -322,7 +384,9 @@ def remove_duplicates(self):
@pyqtSlot()
def set_current_label_name(self):
self.current_label.name = self.ui.comboBoxFuzzingLabel.currentText()
- self.ui.comboBoxFuzzingLabel.setItemText(self.ui.comboBoxFuzzingLabel.currentIndex(), self.current_label.name)
+ self.ui.comboBoxFuzzingLabel.setItemText(
+ self.ui.comboBoxFuzzingLabel.currentIndex(), self.current_label.name
+ )
@pyqtSlot(int)
def on_fuzz_msg_changed(self, index: int):
@@ -336,7 +400,9 @@ def on_fuzz_msg_changed(self, index: int):
self.ui.comboBoxFuzzingLabel.setDisabled(True)
return
- self.ui.comboBoxFuzzingLabel.addItems([lbl.name for lbl in self.message.message_type])
+ self.ui.comboBoxFuzzingLabel.addItems(
+ [lbl.name for lbl in self.message.message_type]
+ )
self.ui.comboBoxFuzzingLabel.blockSignals(False)
if sel_label_ind < self.ui.comboBoxFuzzingLabel.count():
@@ -350,8 +416,13 @@ def on_fuzz_msg_changed(self, index: int):
@pyqtSlot()
def on_btn_repeat_values_clicked(self):
- num_repeats, ok = QInputDialog.getInt(self, self.tr("How many times shall values be repeated?"),
- self.tr("Number of repeats:"), 1, 1)
+ num_repeats, ok = QInputDialog.getInt(
+ self,
+ self.tr("How many times shall values be repeated?"),
+ self.tr("Number of repeats:"),
+ 1,
+ 1,
+ )
if ok:
self.ui.chkBRemoveDuplicates.setChecked(False)
min_row, max_row, _, _ = self.ui.tblFuzzingValues.selection_range()
diff --git a/src/urh/controller/dialogs/MessageTypeDialog.py b/src/urh/controller/dialogs/MessageTypeDialog.py
index f1431329e9..b95b0b1e5d 100644
--- a/src/urh/controller/dialogs/MessageTypeDialog.py
+++ b/src/urh/controller/dialogs/MessageTypeDialog.py
@@ -14,7 +14,6 @@
class MessageTypeDialog(QDialog):
-
def __init__(self, message_type: MessageType, parent=None):
super().__init__(parent)
self.ui = Ui_DialogMessageType()
@@ -29,7 +28,9 @@ def __init__(self, message_type: MessageType, parent=None):
self.message_type = message_type
self.original_ruleset = copy.deepcopy(message_type.ruleset)
self.original_assigned_status = message_type.assigned_by_ruleset
- self.ruleset_table_model = RulesetTableModel(message_type.ruleset, operator_descriptions, parent=self)
+ self.ruleset_table_model = RulesetTableModel(
+ message_type.ruleset, operator_descriptions, parent=self
+ )
self.ui.tblViewRuleset.setModel(self.ruleset_table_model)
self.ui.btnRemoveRule.setEnabled(len(message_type.ruleset) > 0)
@@ -38,8 +39,12 @@ def __init__(self, message_type: MessageType, parent=None):
self.ui.rbAssignAutomatically.setChecked(self.message_type.assigned_by_ruleset)
self.ui.rbAssignManually.setChecked(self.message_type.assign_manually)
- self.ui.tblViewRuleset.setItemDelegateForColumn(2, ComboBoxDelegate(["Bit", "Hex", "ASCII"], parent=self))
- self.ui.tblViewRuleset.setItemDelegateForColumn(3, ComboBoxDelegate(operator_descriptions, parent=self))
+ self.ui.tblViewRuleset.setItemDelegateForColumn(
+ 2, ComboBoxDelegate(["Bit", "Hex", "ASCII"], parent=self)
+ )
+ self.ui.tblViewRuleset.setItemDelegateForColumn(
+ 3, ComboBoxDelegate(operator_descriptions, parent=self)
+ )
for i in range(len(message_type.ruleset)):
self.open_editors(i)
@@ -47,32 +52,46 @@ def __init__(self, message_type: MessageType, parent=None):
self.ui.cbRulesetMode.setCurrentIndex(self.message_type.ruleset.mode.value)
self.create_connects()
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
def create_connects(self):
self.ui.btnAddRule.clicked.connect(self.on_btn_add_rule_clicked)
self.ui.btnRemoveRule.clicked.connect(self.on_btn_remove_rule_clicked)
- self.ui.rbAssignAutomatically.clicked.connect(self.on_rb_assign_automatically_clicked)
+ self.ui.rbAssignAutomatically.clicked.connect(
+ self.on_rb_assign_automatically_clicked
+ )
self.ui.rbAssignManually.clicked.connect(self.on_rb_assign_manually_clicked)
- self.ui.cbRulesetMode.currentIndexChanged.connect(self.on_cb_rulesetmode_current_index_changed)
+ self.ui.cbRulesetMode.currentIndexChanged.connect(
+ self.on_cb_rulesetmode_current_index_changed
+ )
self.ui.buttonBox.accepted.connect(self.accept)
self.ui.buttonBox.rejected.connect(self.on_rejected)
def set_ruleset_ui_status(self):
self.ui.tblViewRuleset.setEnabled(self.message_type.assigned_by_ruleset)
- self.ui.btnRemoveRule.setEnabled(self.message_type.assigned_by_ruleset and len(self.message_type.ruleset) > 0)
+ self.ui.btnRemoveRule.setEnabled(
+ self.message_type.assigned_by_ruleset and len(self.message_type.ruleset) > 0
+ )
self.ui.btnAddRule.setEnabled(self.message_type.assigned_by_ruleset)
self.ui.cbRulesetMode.setEnabled(self.message_type.assigned_by_ruleset)
def open_editors(self, row):
- self.ui.tblViewRuleset.openPersistentEditor(self.ruleset_table_model.index(row, 2))
- self.ui.tblViewRuleset.openPersistentEditor(self.ruleset_table_model.index(row, 3))
+ self.ui.tblViewRuleset.openPersistentEditor(
+ self.ruleset_table_model.index(row, 2)
+ )
+ self.ui.tblViewRuleset.openPersistentEditor(
+ self.ruleset_table_model.index(row, 3)
+ )
def closeEvent(self, event: QCloseEvent):
self.ui.tblViewRuleset.setItemDelegateForColumn(2, None)
self.ui.tblViewRuleset.setItemDelegateForColumn(3, None)
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
@pyqtSlot()
@@ -84,7 +103,9 @@ def on_rejected(self):
@pyqtSlot()
def on_btn_add_rule_clicked(self):
self.ui.btnRemoveRule.setEnabled(True)
- self.message_type.ruleset.append(Rule(start=0, end=0, operator="=", target_value="1", value_type=0))
+ self.message_type.ruleset.append(
+ Rule(start=0, end=0, operator="=", target_value="1", value_type=0)
+ )
self.ruleset_table_model.update()
for i in range(len(self.message_type.ruleset)):
diff --git a/src/urh/controller/dialogs/ModulationParametersDialog.py b/src/urh/controller/dialogs/ModulationParametersDialog.py
index 468a3a2a69..042c55374d 100644
--- a/src/urh/controller/dialogs/ModulationParametersDialog.py
+++ b/src/urh/controller/dialogs/ModulationParametersDialog.py
@@ -20,13 +20,21 @@ def __init__(self, parameters: list, modulation_type: str, parent=None):
self.num_bits = int(math.log2(len(parameters)))
if "FSK" in modulation_type:
- self.ui.tblSymbolParameters.setItemDelegateForColumn(1, KillerSpinBoxDelegate(-1e12, 1e12, self))
- self.ui.tblSymbolParameters.horizontalHeaderItem(1).setText("Frequency in Hz")
+ self.ui.tblSymbolParameters.setItemDelegateForColumn(
+ 1, KillerSpinBoxDelegate(-1e12, 1e12, self)
+ )
+ self.ui.tblSymbolParameters.horizontalHeaderItem(1).setText(
+ "Frequency in Hz"
+ )
elif "ASK" in modulation_type:
self.ui.tblSymbolParameters.horizontalHeaderItem(1).setText("Amplitude")
- self.ui.tblSymbolParameters.setItemDelegateForColumn(1, SpinBoxDelegate(0, 100, self, "%"))
+ self.ui.tblSymbolParameters.setItemDelegateForColumn(
+ 1, SpinBoxDelegate(0, 100, self, "%")
+ )
elif "PSK" in modulation_type:
- self.ui.tblSymbolParameters.setItemDelegateForColumn(1, SpinBoxDelegate(-360, 360, self, "°"))
+ self.ui.tblSymbolParameters.setItemDelegateForColumn(
+ 1, SpinBoxDelegate(-360, 360, self, "°")
+ )
self.ui.tblSymbolParameters.horizontalHeaderItem(1).setText("Phase")
fmt = "{0:0" + str(self.num_bits) + "b}"
@@ -42,7 +50,9 @@ def __init__(self, parameters: list, modulation_type: str, parent=None):
item = QTableWidgetItem()
item.setData(Qt.DisplayRole, self.parameters[i])
self.ui.tblSymbolParameters.setItem(i, 1, item)
- self.ui.tblSymbolParameters.openPersistentEditor(self.ui.tblSymbolParameters.item(i, 1))
+ self.ui.tblSymbolParameters.openPersistentEditor(
+ self.ui.tblSymbolParameters.item(i, 1)
+ )
self.create_connects()
@@ -58,8 +68,9 @@ def on_accepted(self):
self.accept()
-if __name__ == '__main__':
+if __name__ == "__main__":
from PyQt5.QtWidgets import QApplication
+
app = QApplication(["urh"])
dialog = ModulationParametersDialog([0, 100.0], "ASK")
diff --git a/src/urh/controller/dialogs/ModulatorDialog.py b/src/urh/controller/dialogs/ModulatorDialog.py
index 318b7a0883..306704b7c7 100644
--- a/src/urh/controller/dialogs/ModulatorDialog.py
+++ b/src/urh/controller/dialogs/ModulatorDialog.py
@@ -55,15 +55,19 @@ def __init__(self, modulators, tree_model=None, parent=None):
self.original_bits = ""
- self.restore_bits_action = self.ui.linEdDataBits.addAction(QIcon.fromTheme("edit-undo"),
- QLineEdit.TrailingPosition)
+ self.restore_bits_action = self.ui.linEdDataBits.addAction(
+ QIcon.fromTheme("edit-undo"), QLineEdit.TrailingPosition
+ )
self.restore_bits_action.setEnabled(False)
- self.configure_parameters_action = self.ui.lineEditParameters.addAction(QIcon.fromTheme("configure"),
- QLineEdit.TrailingPosition)
+ self.configure_parameters_action = self.ui.lineEditParameters.addAction(
+ QIcon.fromTheme("configure"), QLineEdit.TrailingPosition
+ )
self.create_connects()
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
self.set_bits_per_symbol_enabled_status()
self.set_modulation_profile_status()
@@ -73,7 +77,7 @@ def __init__(self, modulators, tree_model=None, parent=None):
def __cur_selected_mod_type(self):
s = self.ui.comboBoxModulationType.currentText()
- return s[s.rindex("(") + 1:s.rindex(")")]
+ return s[s.rindex("(") + 1 : s.rindex(")")]
@staticmethod
def __trim_number(number):
@@ -103,14 +107,23 @@ def __set_gauss_ui_visibility(self, show: bool):
self.ui.spinBoxGaussBT.setVisible(show)
self.ui.spinBoxGaussFilterWidth.setVisible(show)
- self.ui.spinBoxGaussFilterWidth.setValue(self.current_modulator.gauss_filter_width)
+ self.ui.spinBoxGaussFilterWidth.setValue(
+ self.current_modulator.gauss_filter_width
+ )
self.ui.spinBoxGaussBT.setValue(self.current_modulator.gauss_bt)
def closeEvent(self, event: QCloseEvent):
self.ui.lineEditParameters.editingFinished.emit()
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
-
- for gv in (self.ui.gVCarrier, self.ui.gVData, self.ui.gVModulated, self.ui.gVOriginalSignal):
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
+
+ for gv in (
+ self.ui.gVCarrier,
+ self.ui.gVData,
+ self.ui.gVModulated,
+ self.ui.gVOriginalSignal,
+ ):
# Eliminate graphic views to prevent segfaults
gv.eliminate()
@@ -121,49 +134,84 @@ def current_modulator(self):
return self.modulators[self.ui.comboBoxCustomModulations.currentIndex()]
def set_ui_for_current_modulator(self):
- index = self.ui.comboBoxModulationType.findText("*(" + self.current_modulator.modulation_type + ")",
- Qt.MatchWildcard)
+ index = self.ui.comboBoxModulationType.findText(
+ "*(" + self.current_modulator.modulation_type + ")", Qt.MatchWildcard
+ )
self.ui.comboBoxModulationType.setCurrentIndex(index)
- self.ui.doubleSpinBoxCarrierFreq.setValue(self.current_modulator.carrier_freq_hz)
- self.ui.doubleSpinBoxCarrierPhase.setValue(self.current_modulator.carrier_phase_deg)
- self.ui.spinBoxSamplesPerSymbol.setValue(self.current_modulator.samples_per_symbol)
+ self.ui.doubleSpinBoxCarrierFreq.setValue(
+ self.current_modulator.carrier_freq_hz
+ )
+ self.ui.doubleSpinBoxCarrierPhase.setValue(
+ self.current_modulator.carrier_phase_deg
+ )
+ self.ui.spinBoxSamplesPerSymbol.setValue(
+ self.current_modulator.samples_per_symbol
+ )
self.ui.spinBoxSampleRate.setValue(self.current_modulator.sample_rate)
self.ui.spinBoxBitsPerSymbol.setValue(self.current_modulator.bits_per_symbol)
self.update_modulation_parameters()
def create_connects(self):
- self.ui.doubleSpinBoxCarrierFreq.valueChanged.connect(self.on_carrier_freq_changed)
- self.ui.doubleSpinBoxCarrierPhase.valueChanged.connect(self.on_carrier_phase_changed)
- self.ui.spinBoxSamplesPerSymbol.valueChanged.connect(self.on_samples_per_symbol_changed)
+ self.ui.doubleSpinBoxCarrierFreq.valueChanged.connect(
+ self.on_carrier_freq_changed
+ )
+ self.ui.doubleSpinBoxCarrierPhase.valueChanged.connect(
+ self.on_carrier_phase_changed
+ )
+ self.ui.spinBoxSamplesPerSymbol.valueChanged.connect(
+ self.on_samples_per_symbol_changed
+ )
self.ui.spinBoxSampleRate.valueChanged.connect(self.on_sample_rate_changed)
self.ui.linEdDataBits.textChanged.connect(self.on_data_bits_changed)
- self.ui.spinBoxBitsPerSymbol.valueChanged.connect(self.on_bits_per_symbol_changed)
- self.ui.comboBoxModulationType.currentIndexChanged.connect(self.on_modulation_type_changed)
+ self.ui.spinBoxBitsPerSymbol.valueChanged.connect(
+ self.on_bits_per_symbol_changed
+ )
+ self.ui.comboBoxModulationType.currentIndexChanged.connect(
+ self.on_modulation_type_changed
+ )
self.ui.gVOriginalSignal.zoomed.connect(self.on_orig_signal_zoomed)
- self.ui.cbShowDataBitsOnly.stateChanged.connect(self.on_show_data_bits_only_changed)
+ self.ui.cbShowDataBitsOnly.stateChanged.connect(
+ self.on_show_data_bits_only_changed
+ )
self.ui.btnSearchNext.clicked.connect(self.on_btn_next_search_result_clicked)
self.ui.btnSearchPrev.clicked.connect(self.on_btn_prev_search_result_clicked)
- self.ui.comboBoxCustomModulations.editTextChanged.connect(self.on_custom_modulation_name_edited)
- self.ui.comboBoxCustomModulations.currentIndexChanged.connect(self.on_custom_modulation_index_changed)
+ self.ui.comboBoxCustomModulations.editTextChanged.connect(
+ self.on_custom_modulation_name_edited
+ )
+ self.ui.comboBoxCustomModulations.currentIndexChanged.connect(
+ self.on_custom_modulation_index_changed
+ )
self.ui.btnAddModulation.clicked.connect(self.add_modulator)
self.ui.btnRemoveModulation.clicked.connect(self.on_remove_modulator_clicked)
self.ui.gVModulated.zoomed.connect(self.on_carrier_data_modulated_zoomed)
self.ui.gVCarrier.zoomed.connect(self.on_carrier_data_modulated_zoomed)
self.ui.gVData.zoomed.connect(self.on_carrier_data_modulated_zoomed)
- self.ui.gVModulated.selection_width_changed.connect(self.on_modulated_selection_changed)
- self.ui.gVOriginalSignal.selection_width_changed.connect(self.on_original_selection_changed)
+ self.ui.gVModulated.selection_width_changed.connect(
+ self.on_modulated_selection_changed
+ )
+ self.ui.gVOriginalSignal.selection_width_changed.connect(
+ self.on_original_selection_changed
+ )
self.ui.spinBoxGaussBT.valueChanged.connect(self.on_gauss_bt_changed)
- self.ui.spinBoxGaussFilterWidth.valueChanged.connect(self.on_gauss_filter_width_changed)
+ self.ui.spinBoxGaussFilterWidth.valueChanged.connect(
+ self.on_gauss_filter_width_changed
+ )
self.ui.chkBoxLockSIV.stateChanged.connect(self.on_lock_siv_changed)
self.ui.gVOriginalSignal.signal_loaded.connect(self.handle_signal_loaded)
self.ui.btnAutoDetect.clicked.connect(self.on_btn_autodetect_clicked)
- self.restore_bits_action.triggered.connect(self.on_restore_bits_action_triggered)
- self.configure_parameters_action.triggered.connect(self.on_configure_parameters_action_triggered)
- self.ui.lineEditParameters.editingFinished.connect(self.on_line_edit_parameters_editing_finished)
+ self.restore_bits_action.triggered.connect(
+ self.on_restore_bits_action_triggered
+ )
+ self.configure_parameters_action.triggered.connect(
+ self.on_configure_parameters_action_triggered
+ )
+ self.ui.lineEditParameters.editingFinished.connect(
+ self.on_line_edit_parameters_editing_finished
+ )
def draw_carrier(self):
self.ui.gVCarrier.plot_data(self.current_modulator.carrier_data)
@@ -207,7 +255,10 @@ def update_views(self):
self.ui.gVOriginalSignal.update()
def search_data_sequence(self):
- if not self.ui.cbShowDataBitsOnly.isEnabled() or not self.ui.cbShowDataBitsOnly.isChecked():
+ if (
+ not self.ui.cbShowDataBitsOnly.isEnabled()
+ or not self.ui.cbShowDataBitsOnly.isChecked()
+ ):
return
search_seq = self.ui.linEdDataBits.text()
@@ -235,7 +286,9 @@ def show_search_result(self, i: int):
message, start_index, end_index = self.search_results[i]
- start, nsamples = self.protocol.get_samplepos_of_bitseq(message, start_index, message, end_index, False)
+ start, nsamples = self.protocol.get_samplepos_of_bitseq(
+ message, start_index, message, end_index, False
+ )
self.draw_original_signal(start=start, end=start + nsamples)
self.ui.lCurrentSearchResult.setText(str(i + 1))
@@ -255,7 +308,9 @@ def add_modulator(self):
self.ui.btnRemoveModulation.setEnabled(True)
def adjust_samples_in_view(self, target_siv: float):
- self.ui.gVOriginalSignal.scale(self.ui.gVOriginalSignal.view_rect().width() / target_siv, 1)
+ self.ui.gVOriginalSignal.scale(
+ self.ui.gVOriginalSignal.view_rect().width() / target_siv, 1
+ )
mod_zoom_factor = self.ui.gVModulated.view_rect().width() / target_siv
self.ui.gVModulated.scale(mod_zoom_factor, 1)
self.ui.gVCarrier.scale(mod_zoom_factor, 1)
@@ -271,8 +326,12 @@ def detect_fsk_frequencies(self):
if not self.current_modulator.is_binary_modulation:
raise NotImplementedError()
- zero_freq = self.protocol.estimate_frequency_for_zero(self.current_modulator.sample_rate)
- one_freq = self.protocol.estimate_frequency_for_one(self.current_modulator.sample_rate)
+ zero_freq = self.protocol.estimate_frequency_for_zero(
+ self.current_modulator.sample_rate
+ )
+ one_freq = self.protocol.estimate_frequency_for_one(
+ self.current_modulator.sample_rate
+ )
zero_freq = self.__trim_number(zero_freq)
one_freq = self.__trim_number(one_freq)
zero_freq, one_freq = self.__ensure_multitude(zero_freq, one_freq)
@@ -297,7 +356,9 @@ def handle_signal_loaded(self, protocol):
self.protocol = protocol
# Apply bit length of original signal to current modulator
- self.ui.spinBoxSamplesPerSymbol.setValue(self.ui.gVOriginalSignal.signal.samples_per_symbol)
+ self.ui.spinBoxSamplesPerSymbol.setValue(
+ self.ui.gVOriginalSignal.signal.samples_per_symbol
+ )
# https://github.com/jopohl/urh/issues/130
self.ui.gVModulated.show_full_scene(reinitialize=True)
@@ -307,15 +368,21 @@ def handle_signal_loaded(self, protocol):
self.unsetCursor()
def mark_samples_in_view(self):
- self.ui.lSamplesInViewModulated.setText(str(int(self.ui.gVModulated.view_rect().width())))
+ self.ui.lSamplesInViewModulated.setText(
+ str(int(self.ui.gVModulated.view_rect().width()))
+ )
if self.ui.gVOriginalSignal.scene_manager is not None:
- self.ui.lSamplesInViewOrigSignal.setText(str(int(self.ui.gVOriginalSignal.view_rect().width())))
+ self.ui.lSamplesInViewOrigSignal.setText(
+ str(int(self.ui.gVOriginalSignal.view_rect().width()))
+ )
else:
self.ui.lSamplesInViewOrigSignal.setText("-")
return
- if int(self.ui.gVOriginalSignal.view_rect().width()) != int(self.ui.gVModulated.view_rect().width()):
+ if int(self.ui.gVOriginalSignal.view_rect().width()) != int(
+ self.ui.gVModulated.view_rect().width()
+ ):
font = self.ui.lSamplesInViewModulated.font()
font.setBold(False)
self.ui.lSamplesInViewModulated.setFont(font)
@@ -333,7 +400,9 @@ def mark_samples_in_view(self):
self.ui.lSamplesInViewModulated.setStyleSheet("")
def set_default_modulation_parameters(self):
- self.current_modulator.parameters = self.current_modulator.get_default_parameters()
+ self.current_modulator.parameters = (
+ self.current_modulator.get_default_parameters()
+ )
self.update_modulation_parameters()
def set_modulation_profile_status(self):
@@ -393,19 +462,25 @@ def show_full_scene(self):
@pyqtSlot()
def on_carrier_freq_changed(self):
- self.current_modulator.carrier_freq_hz = self.ui.doubleSpinBoxCarrierFreq.value()
+ self.current_modulator.carrier_freq_hz = (
+ self.ui.doubleSpinBoxCarrierFreq.value()
+ )
self.draw_carrier()
self.draw_modulated()
@pyqtSlot()
def on_carrier_phase_changed(self):
- self.current_modulator.carrier_phase_deg = self.ui.doubleSpinBoxCarrierPhase.value()
+ self.current_modulator.carrier_phase_deg = (
+ self.ui.doubleSpinBoxCarrierPhase.value()
+ )
self.draw_carrier()
self.draw_modulated()
@pyqtSlot()
def on_samples_per_symbol_changed(self):
- self.current_modulator.samples_per_symbol = self.ui.spinBoxSamplesPerSymbol.value()
+ self.current_modulator.samples_per_symbol = (
+ self.ui.spinBoxSamplesPerSymbol.value()
+ )
self.draw_carrier()
self.draw_data_bits()
self.draw_modulated()
@@ -414,7 +489,7 @@ def on_samples_per_symbol_changed(self):
@pyqtSlot()
def on_data_bits_changed(self):
text = self.ui.linEdDataBits.text()
- text = ''.join(c for c in text if c == "1" or c == "0")
+ text = "".join(c for c in text if c == "1" or c == "0")
self.ui.linEdDataBits.blockSignals(True)
self.ui.linEdDataBits.setText(text)
self.ui.linEdDataBits.blockSignals(False)
@@ -428,7 +503,9 @@ def on_data_bits_changed(self):
else:
display_text = text
self.ui.cbShowDataBitsOnly.setToolTip(text)
- self.ui.cbShowDataBitsOnly.setText(self.tr("Show Only Data Sequence\n") + "(" + display_text + ")")
+ self.ui.cbShowDataBitsOnly.setText(
+ self.tr("Show Only Data Sequence\n") + "(" + display_text + ")"
+ )
else:
self.ui.cbShowDataBitsOnly.setToolTip("")
self.ui.cbShowDataBitsOnly.setText(self.tr("Show Only Data Sequence\n"))
@@ -451,22 +528,28 @@ def on_gauss_bt_changed(self):
@pyqtSlot()
def on_gauss_filter_width_changed(self):
- self.current_modulator.gauss_filter_width = self.ui.spinBoxGaussFilterWidth.value()
+ self.current_modulator.gauss_filter_width = (
+ self.ui.spinBoxGaussFilterWidth.value()
+ )
self.draw_modulated()
@pyqtSlot()
def on_bits_per_symbol_changed(self):
- if self.current_modulator.bits_per_symbol == self.ui.spinBoxBitsPerSymbol.value():
+ if (
+ self.current_modulator.bits_per_symbol
+ == self.ui.spinBoxBitsPerSymbol.value()
+ ):
return
self.current_modulator.bits_per_symbol = self.ui.spinBoxBitsPerSymbol.value()
self.set_default_modulation_parameters()
self.draw_modulated()
self.show_full_scene()
-
@pyqtSlot()
def on_modulation_type_changed(self):
- write_default_parameters = self.current_modulator.modulation_type != self.__cur_selected_mod_type()
+ write_default_parameters = (
+ self.current_modulator.modulation_type != self.__cur_selected_mod_type()
+ )
self.current_modulator.modulation_type = self.__cur_selected_mod_type()
self.__set_gauss_ui_visibility(self.__cur_selected_mod_type() == "GFSK")
@@ -490,7 +573,10 @@ def on_orig_signal_zoomed(self):
if self.lock_samples_in_view:
self.adjust_samples_in_view(self.ui.gVOriginalSignal.view_rect().width())
- x = self.ui.gVOriginalSignal.view_rect().x() + self.ui.gVOriginalSignal.view_rect().width() / 2
+ x = (
+ self.ui.gVOriginalSignal.view_rect().x()
+ + self.ui.gVOriginalSignal.view_rect().width() / 2
+ )
y = 0
self.ui.gVModulated.centerOn(x, y)
@@ -501,7 +587,6 @@ def on_orig_signal_zoomed(self):
@pyqtSlot(float)
def on_carrier_data_modulated_zoomed(self, factor: float):
-
x = self.sender().view_rect().x() + self.sender().view_rect().width() / 2
y = 0
for gv in (self.ui.gVCarrier, self.ui.gVData, self.ui.gVModulated):
@@ -582,8 +667,11 @@ def on_btn_autodetect_clicked(self):
freq = self.current_modulator.estimate_carrier_frequency(signal, self.protocol)
if freq is None or freq == 0:
- QMessageBox.information(self, self.tr("No results"),
- self.tr("Unable to detect parameters from current signal"))
+ QMessageBox.information(
+ self,
+ self.tr("No results"),
+ self.tr("Unable to detect parameters from current signal"),
+ )
return
self.ui.doubleSpinBoxCarrierFreq.setValue(freq)
@@ -600,8 +688,11 @@ def on_original_selection_changed(self, new_width: int):
@pyqtSlot()
def on_configure_parameters_action_triggered(self):
self.ui.lineEditParameters.editingFinished.emit()
- dialog = ModulationParametersDialog(self.current_modulator.parameters, self.current_modulator.modulation_type,
- self)
+ dialog = ModulationParametersDialog(
+ self.current_modulator.parameters,
+ self.current_modulator.modulation_type,
+ self,
+ )
dialog.accepted.connect(self.update_modulation_parameters)
dialog.show()
@@ -616,13 +707,13 @@ def on_line_edit_parameters_editing_finished(self):
param = param.upper().replace(",", ".")
factor = 1
if param.endswith("G"):
- factor = 10 ** 9
+ factor = 10**9
param = param[:-1]
elif param.endswith("M"):
- factor = 10 ** 6
+ factor = 10**6
param = param[:-1]
elif param.endswith("K"):
- factor = 10 ** 3
+ factor = 10**3
param = param[:-1]
try:
diff --git a/src/urh/controller/dialogs/OptionsDialog.py b/src/urh/controller/dialogs/OptionsDialog.py
index 8a0d543869..450df8c2e1 100644
--- a/src/urh/controller/dialogs/OptionsDialog.py
+++ b/src/urh/controller/dialogs/OptionsDialog.py
@@ -5,10 +5,26 @@
import time
import numpy as np
-from PyQt5.QtCore import Qt, pyqtSlot, pyqtSignal, QSize, QAbstractTableModel, QModelIndex
+from PyQt5.QtCore import (
+ Qt,
+ pyqtSlot,
+ pyqtSignal,
+ QSize,
+ QAbstractTableModel,
+ QModelIndex,
+)
from PyQt5.QtGui import QCloseEvent, QIcon, QPixmap
-from PyQt5.QtWidgets import QDialog, QHBoxLayout, QCompleter, QDirModel, QApplication, QHeaderView, QRadioButton, \
- QFileDialog, qApp
+from PyQt5.QtWidgets import (
+ QDialog,
+ QHBoxLayout,
+ QCompleter,
+ QDirModel,
+ QApplication,
+ QHeaderView,
+ QRadioButton,
+ QFileDialog,
+ qApp,
+)
from urh import settings, colormaps
from urh.controller.widgets.PluginFrame import PluginFrame
@@ -25,7 +41,12 @@
class DeviceOptionsTableModel(QAbstractTableModel):
- header_labels = ["Software Defined Radio", "Info", "Native backend (recommended)", "GNU Radio backend"]
+ header_labels = [
+ "Software Defined Radio",
+ "Info",
+ "Native backend (recommended)",
+ "GNU Radio backend",
+ ]
def __init__(self, backend_handler: BackendHandler, parent=None):
self.backend_handler = backend_handler
@@ -48,7 +69,9 @@ def headerData(self, section, orientation, role=Qt.DisplayRole):
return super().headerData(section, orientation, role)
def get_device_at(self, index: int):
- dev_key = self.backend_handler.get_key_from_device_display_text(self.backend_handler.DEVICE_NAMES[index])
+ dev_key = self.backend_handler.get_key_from_device_display_text(
+ self.backend_handler.DEVICE_NAMES[index]
+ )
return self.backend_handler.device_backends[dev_key]
def data(self, index: QModelIndex, role=Qt.DisplayRole):
@@ -83,9 +106,17 @@ def data(self, index: QModelIndex, role=Qt.DisplayRole):
if j == 0 and (device.has_native_backend or device.has_gnuradio_backend):
return Qt.Checked if device.is_enabled else Qt.Unchecked
elif j == 2 and device.has_native_backend:
- return Qt.Checked if device.selected_backend == Backends.native else Qt.Unchecked
+ return (
+ Qt.Checked
+ if device.selected_backend == Backends.native
+ else Qt.Unchecked
+ )
elif j == 3 and device.has_gnuradio_backend:
- return Qt.Checked if device.selected_backend == Backends.grc else Qt.Unchecked
+ return (
+ Qt.Checked
+ if device.selected_backend == Backends.grc
+ else Qt.Unchecked
+ )
def setData(self, index: QModelIndex, value, role=None):
if not index.isValid():
@@ -155,11 +186,15 @@ def __init__(self, installed_plugins, highlighted_plugins=None, parent=None):
self.ui.tblDevices.setModel(self.device_options_model)
self.ui.tblDevices.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
- self.ui.tblDevices.setItemDelegateForColumn(1, ComboBoxDelegate(["native", "GNU Radio"]))
+ self.ui.tblDevices.setItemDelegateForColumn(
+ 1, ComboBoxDelegate(["native", "GNU Radio"])
+ )
self.setAttribute(Qt.WA_DeleteOnClose)
layout = QHBoxLayout(self.ui.tab_plugins)
- self.plugin_controller = PluginFrame(installed_plugins, highlighted_plugins, parent=self)
+ self.plugin_controller = PluginFrame(
+ installed_plugins, highlighted_plugins, parent=self
+ )
layout.addWidget(self.plugin_controller)
self.ui.tab_plugins.setLayout(layout)
@@ -172,26 +207,52 @@ def __init__(self, installed_plugins, highlighted_plugins=None, parent=None):
self.ui.comboBoxIconTheme.setVisible(sys.platform == "linux")
self.ui.comboBoxTheme.setCurrentIndex(settings.read("theme_index", 0, int))
- self.ui.comboBoxIconTheme.setCurrentIndex(settings.read("icon_theme_index", 0, int))
- self.ui.checkBoxShowConfirmCloseDialog.setChecked(not settings.read('not_show_close_dialog', False, bool))
- self.ui.checkBoxHoldShiftToDrag.setChecked(settings.read('hold_shift_to_drag', True, bool))
- self.ui.checkBoxDefaultFuzzingPause.setChecked(settings.read('use_default_fuzzing_pause', True, bool))
-
- self.ui.checkBoxAlignLabels.setChecked(settings.read('align_labels', True, bool))
-
- self.ui.doubleSpinBoxRAMThreshold.setValue(100 * settings.read('ram_threshold', 0.6, float))
+ self.ui.comboBoxIconTheme.setCurrentIndex(
+ settings.read("icon_theme_index", 0, int)
+ )
+ self.ui.checkBoxShowConfirmCloseDialog.setChecked(
+ not settings.read("not_show_close_dialog", False, bool)
+ )
+ self.ui.checkBoxHoldShiftToDrag.setChecked(
+ settings.read("hold_shift_to_drag", True, bool)
+ )
+ self.ui.checkBoxDefaultFuzzingPause.setChecked(
+ settings.read("use_default_fuzzing_pause", True, bool)
+ )
+
+ self.ui.checkBoxAlignLabels.setChecked(
+ settings.read("align_labels", True, bool)
+ )
+
+ self.ui.doubleSpinBoxRAMThreshold.setValue(
+ 100 * settings.read("ram_threshold", 0.6, float)
+ )
if self.backend_handler.gr_python_interpreter:
- self.ui.lineEditGRPythonInterpreter.setText(self.backend_handler.gr_python_interpreter)
-
- self.ui.doubleSpinBoxFuzzingPause.setValue(settings.read("default_fuzzing_pause", 10 ** 6, int))
- self.ui.doubleSpinBoxFuzzingPause.setEnabled(settings.read('use_default_fuzzing_pause', True, bool))
-
- self.ui.checkBoxMultipleModulations.setChecked(settings.read("multiple_modulations", False, bool))
-
- self.ui.radioButtonLowModulationAccuracy.setChecked(Modulator.get_dtype() == np.int8)
- self.ui.radioButtonMediumModulationAccuracy.setChecked(Modulator.get_dtype() == np.int16)
- self.ui.radioButtonHighModulationAccuracy.setChecked(Modulator.get_dtype() == np.float32)
+ self.ui.lineEditGRPythonInterpreter.setText(
+ self.backend_handler.gr_python_interpreter
+ )
+
+ self.ui.doubleSpinBoxFuzzingPause.setValue(
+ settings.read("default_fuzzing_pause", 10**6, int)
+ )
+ self.ui.doubleSpinBoxFuzzingPause.setEnabled(
+ settings.read("use_default_fuzzing_pause", True, bool)
+ )
+
+ self.ui.checkBoxMultipleModulations.setChecked(
+ settings.read("multiple_modulations", False, bool)
+ )
+
+ self.ui.radioButtonLowModulationAccuracy.setChecked(
+ Modulator.get_dtype() == np.int8
+ )
+ self.ui.radioButtonMediumModulationAccuracy.setChecked(
+ Modulator.get_dtype() == np.int16
+ )
+ self.ui.radioButtonHighModulationAccuracy.setChecked(
+ Modulator.get_dtype() == np.float32
+ )
completer = QCompleter()
completer.setModel(QDirModel(completer))
@@ -206,11 +267,19 @@ def __init__(self, installed_plugins, highlighted_plugins=None, parent=None):
self.field_type_table_model = FieldTypeTableModel([], parent=self)
self.ui.tblLabeltypes.setModel(self.field_type_table_model)
- self.ui.tblLabeltypes.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
-
- self.ui.tblLabeltypes.setItemDelegateForColumn(1, ComboBoxDelegate([f.name for f in FieldType.Function],
- return_index=False, parent=self))
- self.ui.tblLabeltypes.setItemDelegateForColumn(2, ComboBoxDelegate(ProtocolLabel.DISPLAY_FORMATS, parent=self))
+ self.ui.tblLabeltypes.horizontalHeader().setSectionResizeMode(
+ QHeaderView.Stretch
+ )
+
+ self.ui.tblLabeltypes.setItemDelegateForColumn(
+ 1,
+ ComboBoxDelegate(
+ [f.name for f in FieldType.Function], return_index=False, parent=self
+ ),
+ )
+ self.ui.tblLabeltypes.setItemDelegateForColumn(
+ 2, ComboBoxDelegate(ProtocolLabel.DISPLAY_FORMATS, parent=self)
+ )
self.read_options()
@@ -219,46 +288,98 @@ def __init__(self, installed_plugins, highlighted_plugins=None, parent=None):
self.ui.labelRebuildNativeStatus.setText("")
self.show_available_colormaps()
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
def create_connects(self):
- self.ui.doubleSpinBoxFuzzingPause.valueChanged.connect(self.on_spinbox_fuzzing_pause_value_changed)
- self.ui.lineEditGRPythonInterpreter.editingFinished.connect(self.on_gr_python_interpreter_path_edited)
- self.ui.btnChooseGRPythonInterpreter.clicked.connect(self.on_btn_choose_gr_python_interpreter_clicked)
- self.ui.comboBoxTheme.currentIndexChanged.connect(self.on_combo_box_theme_index_changed)
- self.ui.checkBoxShowConfirmCloseDialog.clicked.connect(self.on_checkbox_confirm_close_dialog_clicked)
- self.ui.checkBoxHoldShiftToDrag.clicked.connect(self.on_checkbox_hold_shift_to_drag_clicked)
- self.ui.checkBoxAlignLabels.clicked.connect(self.on_checkbox_align_labels_clicked)
- self.ui.checkBoxDefaultFuzzingPause.clicked.connect(self.on_checkbox_default_fuzzing_pause_clicked)
+ self.ui.doubleSpinBoxFuzzingPause.valueChanged.connect(
+ self.on_spinbox_fuzzing_pause_value_changed
+ )
+ self.ui.lineEditGRPythonInterpreter.editingFinished.connect(
+ self.on_gr_python_interpreter_path_edited
+ )
+ self.ui.btnChooseGRPythonInterpreter.clicked.connect(
+ self.on_btn_choose_gr_python_interpreter_clicked
+ )
+ self.ui.comboBoxTheme.currentIndexChanged.connect(
+ self.on_combo_box_theme_index_changed
+ )
+ self.ui.checkBoxShowConfirmCloseDialog.clicked.connect(
+ self.on_checkbox_confirm_close_dialog_clicked
+ )
+ self.ui.checkBoxHoldShiftToDrag.clicked.connect(
+ self.on_checkbox_hold_shift_to_drag_clicked
+ )
+ self.ui.checkBoxAlignLabels.clicked.connect(
+ self.on_checkbox_align_labels_clicked
+ )
+ self.ui.checkBoxDefaultFuzzingPause.clicked.connect(
+ self.on_checkbox_default_fuzzing_pause_clicked
+ )
self.ui.btnAddLabelType.clicked.connect(self.on_btn_add_label_type_clicked)
- self.ui.btnRemoveLabeltype.clicked.connect(self.on_btn_remove_label_type_clicked)
- self.ui.radioButtonLowModulationAccuracy.clicked.connect(self.on_radio_button_low_modulation_accuracy_clicked)
- self.ui.radioButtonMediumModulationAccuracy.clicked.connect(self.on_radio_button_medium_modulation_accuracy_clicked)
- self.ui.radioButtonHighModulationAccuracy.clicked.connect(self.on_radio_button_high_modulation_accuracy_clicked)
-
- self.ui.doubleSpinBoxRAMThreshold.valueChanged.connect(self.on_double_spinbox_ram_threshold_value_changed)
+ self.ui.btnRemoveLabeltype.clicked.connect(
+ self.on_btn_remove_label_type_clicked
+ )
+ self.ui.radioButtonLowModulationAccuracy.clicked.connect(
+ self.on_radio_button_low_modulation_accuracy_clicked
+ )
+ self.ui.radioButtonMediumModulationAccuracy.clicked.connect(
+ self.on_radio_button_medium_modulation_accuracy_clicked
+ )
+ self.ui.radioButtonHighModulationAccuracy.clicked.connect(
+ self.on_radio_button_high_modulation_accuracy_clicked
+ )
+
+ self.ui.doubleSpinBoxRAMThreshold.valueChanged.connect(
+ self.on_double_spinbox_ram_threshold_value_changed
+ )
self.ui.btnRebuildNative.clicked.connect(self.on_btn_rebuild_native_clicked)
- self.ui.comboBoxIconTheme.currentIndexChanged.connect(self.on_combobox_icon_theme_index_changed)
- self.ui.checkBoxMultipleModulations.clicked.connect(self.on_checkbox_multiple_modulations_clicked)
+ self.ui.comboBoxIconTheme.currentIndexChanged.connect(
+ self.on_combobox_icon_theme_index_changed
+ )
+ self.ui.checkBoxMultipleModulations.clicked.connect(
+ self.on_checkbox_multiple_modulations_clicked
+ )
self.ui.btnViewBuildLog.clicked.connect(self.on_btn_view_build_log_clicked)
- self.ui.labelDeviceMissingInfo.linkActivated.connect(self.on_label_device_missing_info_link_activated)
- self.ui.doubleSpinBoxFontSize.editingFinished.connect(self.on_spin_box_font_size_editing_finished)
+ self.ui.labelDeviceMissingInfo.linkActivated.connect(
+ self.on_label_device_missing_info_link_activated
+ )
+ self.ui.doubleSpinBoxFontSize.editingFinished.connect(
+ self.on_spin_box_font_size_editing_finished
+ )
def show_gnuradio_infos(self):
- self.ui.lineEditGRPythonInterpreter.setText(self.backend_handler.gr_python_interpreter)
+ self.ui.lineEditGRPythonInterpreter.setText(
+ self.backend_handler.gr_python_interpreter
+ )
if self.backend_handler.gnuradio_is_installed:
- self.ui.lineEditGRPythonInterpreter.setStyleSheet("background-color: lightgreen")
- self.ui.lineEditGRPythonInterpreter.setToolTip("GNU Radio interface is working.")
+ self.ui.lineEditGRPythonInterpreter.setStyleSheet(
+ "background-color: lightgreen"
+ )
+ self.ui.lineEditGRPythonInterpreter.setToolTip(
+ "GNU Radio interface is working."
+ )
else:
- self.ui.lineEditGRPythonInterpreter.setStyleSheet("background-color: orange")
- self.ui.lineEditGRPythonInterpreter.setToolTip("GNU Radio is not installed or incompatible with "
- "the configured python interpreter.")
+ self.ui.lineEditGRPythonInterpreter.setStyleSheet(
+ "background-color: orange"
+ )
+ self.ui.lineEditGRPythonInterpreter.setToolTip(
+ "GNU Radio is not installed or incompatible with "
+ "the configured python interpreter."
+ )
def read_options(self):
- self.ui.comboBoxDefaultView.setCurrentIndex(settings.read('default_view', 0, type=int))
- self.ui.spinBoxNumSendingRepeats.setValue(settings.read('num_sending_repeats', 0, type=int))
- self.ui.checkBoxPauseTime.setChecked(settings.read('show_pause_as_time', False, type=bool))
+ self.ui.comboBoxDefaultView.setCurrentIndex(
+ settings.read("default_view", 0, type=int)
+ )
+ self.ui.spinBoxNumSendingRepeats.setValue(
+ settings.read("num_sending_repeats", 0, type=int)
+ )
+ self.ui.checkBoxPauseTime.setChecked(
+ settings.read("show_pause_as_time", False, type=bool)
+ )
self.old_show_pause_as_time = bool(self.ui.checkBoxPauseTime.isChecked())
@@ -286,55 +407,80 @@ def show_available_colormaps(self):
def closeEvent(self, event: QCloseEvent):
changed_values = {}
if bool(self.ui.checkBoxPauseTime.isChecked()) != self.old_show_pause_as_time:
- changed_values['show_pause_as_time'] = bool(self.ui.checkBoxPauseTime.isChecked())
+ changed_values["show_pause_as_time"] = bool(
+ self.ui.checkBoxPauseTime.isChecked()
+ )
if self.old_default_view != self.ui.comboBoxDefaultView.currentIndex():
- changed_values['default_view'] = self.ui.comboBoxDefaultView.currentIndex()
+ changed_values["default_view"] = self.ui.comboBoxDefaultView.currentIndex()
if self.old_num_sending_repeats != self.ui.spinBoxNumSendingRepeats.value():
- changed_values["num_sending_repeats"] = self.ui.spinBoxNumSendingRepeats.value()
+ changed_values[
+ "num_sending_repeats"
+ ] = self.ui.spinBoxNumSendingRepeats.value()
- settings.write('default_view', self.ui.comboBoxDefaultView.currentIndex())
- settings.write('num_sending_repeats', self.ui.spinBoxNumSendingRepeats.value())
- settings.write('show_pause_as_time', self.ui.checkBoxPauseTime.isChecked())
+ settings.write("default_view", self.ui.comboBoxDefaultView.currentIndex())
+ settings.write("num_sending_repeats", self.ui.spinBoxNumSendingRepeats.value())
+ settings.write("show_pause_as_time", self.ui.checkBoxPauseTime.isChecked())
FieldType.save_to_xml(self.field_type_table_model.field_types)
self.plugin_controller.save_enabled_states()
for plugin in self.plugin_controller.model.plugins:
plugin.destroy_settings_frame()
- for i in range(self.ui.scrollAreaWidgetSpectrogramColormapContents.layout().count()):
- widget = self.ui.scrollAreaWidgetSpectrogramColormapContents.layout().itemAt(i).widget()
+ for i in range(
+ self.ui.scrollAreaWidgetSpectrogramColormapContents.layout().count()
+ ):
+ widget = (
+ self.ui.scrollAreaWidgetSpectrogramColormapContents.layout()
+ .itemAt(i)
+ .widget()
+ )
if isinstance(widget, QRadioButton) and widget.isChecked():
selected_colormap_name = widget.objectName()
- if selected_colormap_name != colormaps.read_selected_colormap_name_from_settings():
+ if (
+ selected_colormap_name
+ != colormaps.read_selected_colormap_name_from_settings()
+ ):
colormaps.choose_colormap(selected_colormap_name)
- colormaps.write_selected_colormap_to_settings(selected_colormap_name)
+ colormaps.write_selected_colormap_to_settings(
+ selected_colormap_name
+ )
changed_values["spectrogram_colormap"] = selected_colormap_name
break
self.values_changed.emit(changed_values)
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
def set_gnuradio_status(self):
- self.backend_handler.gr_python_interpreter = self.ui.lineEditGRPythonInterpreter.text()
+ self.backend_handler.gr_python_interpreter = (
+ self.ui.lineEditGRPythonInterpreter.text()
+ )
self.refresh_device_tab()
@pyqtSlot()
def on_btn_add_label_type_clicked(self):
suffix = 1
- field_type_names = {ft.caption for ft in self.field_type_table_model.field_types}
+ field_type_names = {
+ ft.caption for ft in self.field_type_table_model.field_types
+ }
while "New Fieldtype #" + str(suffix) in field_type_names:
suffix += 1
caption = "New Fieldtype #" + str(suffix)
- self.field_type_table_model.field_types.append(FieldType(caption, FieldType.Function.CUSTOM))
+ self.field_type_table_model.field_types.append(
+ FieldType(caption, FieldType.Function.CUSTOM)
+ )
self.field_type_table_model.update()
@pyqtSlot()
def on_btn_remove_label_type_clicked(self):
if self.field_type_table_model.field_types:
- selected_indices = {i.row() for i in self.ui.tblLabeltypes.selectedIndexes()}
+ selected_indices = {
+ i.row() for i in self.ui.tblLabeltypes.selectedIndexes()
+ }
if selected_indices:
for i in reversed(sorted(selected_indices)):
@@ -355,11 +501,11 @@ def on_checkbox_confirm_close_dialog_clicked(self, checked: bool):
@pyqtSlot(int)
def on_combo_box_theme_index_changed(self, index: int):
- settings.write('theme_index', index)
+ settings.write("theme_index", index)
@pyqtSlot(int)
def on_combobox_icon_theme_index_changed(self, index: int):
- settings.write('icon_theme_index', index)
+ settings.write("icon_theme_index", index)
util.set_icon_theme()
@pyqtSlot(bool)
@@ -368,7 +514,7 @@ def on_checkbox_hold_shift_to_drag_clicked(self, checked: bool):
@pyqtSlot(bool)
def on_checkbox_default_fuzzing_pause_clicked(self, checked: bool):
- settings.write('use_default_fuzzing_pause', checked)
+ settings.write("use_default_fuzzing_pause", checked)
self.ui.doubleSpinBoxFuzzingPause.setEnabled(checked)
@pyqtSlot(float)
@@ -385,7 +531,9 @@ def on_btn_choose_gr_python_interpreter_clicked(self):
dialog_filter = "Executable (*.exe);;All files (*.*)"
else:
dialog_filter = ""
- filename, _ = QFileDialog.getOpenFileName(self, self.tr("Choose python interpreter"), filter=dialog_filter)
+ filename, _ = QFileDialog.getOpenFileName(
+ self, self.tr("Choose python interpreter"), filter=dialog_filter
+ )
if filename:
self.ui.lineEditGRPythonInterpreter.setText(filename)
self.set_gnuradio_status()
@@ -396,28 +544,55 @@ def on_checkbox_align_labels_clicked(self, checked: bool):
@pyqtSlot()
def on_btn_rebuild_native_clicked(self):
- library_dirs = None if not self.ui.lineEditLibDirs.text() \
+ library_dirs = (
+ None
+ if not self.ui.lineEditLibDirs.text()
else list(map(str.strip, self.ui.lineEditLibDirs.text().split(",")))
- include_dirs = None if not self.ui.lineEditIncludeDirs.text() \
+ )
+ include_dirs = (
+ None
+ if not self.ui.lineEditIncludeDirs.text()
else list(map(str.strip, self.ui.lineEditIncludeDirs.text().split(",")))
+ )
- extensions, _ = ExtensionHelper.get_device_extensions_and_extras(library_dirs=library_dirs, include_dirs=include_dirs)
+ extensions, _ = ExtensionHelper.get_device_extensions_and_extras(
+ library_dirs=library_dirs, include_dirs=include_dirs
+ )
- self.ui.labelRebuildNativeStatus.setText(self.tr("Rebuilding device extensions..."))
+ self.ui.labelRebuildNativeStatus.setText(
+ self.tr("Rebuilding device extensions...")
+ )
QApplication.instance().processEvents()
- build_cmd = [sys.executable, os.path.realpath(ExtensionHelper.__file__),
- "build_ext", "--inplace", "-t", tempfile.gettempdir()]
+ build_cmd = [
+ sys.executable,
+ os.path.realpath(ExtensionHelper.__file__),
+ "build_ext",
+ "--inplace",
+ "-t",
+ tempfile.gettempdir(),
+ ]
if library_dirs:
build_cmd.extend(["-L", ":".join(library_dirs)])
if include_dirs:
build_cmd.extend(["-I", ":".join(include_dirs)])
- subprocess.call([sys.executable, os.path.realpath(ExtensionHelper.__file__), "clean", "--all"])
- p = subprocess.Popen(build_cmd, stdout=subprocess.PIPE, stderr=subprocess.STDOUT)
+ subprocess.call(
+ [
+ sys.executable,
+ os.path.realpath(ExtensionHelper.__file__),
+ "clean",
+ "--all",
+ ]
+ )
+ p = subprocess.Popen(
+ build_cmd, stdout=subprocess.PIPE, stderr=subprocess.STDOUT
+ )
num_dots = 1
while p.poll() is None:
- self.ui.labelRebuildNativeStatus.setText(self.tr("Rebuilding device extensions" + ". " * num_dots))
+ self.ui.labelRebuildNativeStatus.setText(
+ self.tr("Rebuilding device extensions" + ". " * num_dots)
+ )
QApplication.instance().processEvents()
time.sleep(0.1)
num_dots %= 10
@@ -425,23 +600,33 @@ def on_btn_rebuild_native_clicked(self):
rc = p.returncode
if rc == 0:
- self.ui.labelRebuildNativeStatus.setText(self.tr(""
- "Rebuilt {0} device extensions. "
- ""
- "Please restart URH.".format(len(extensions))))
+ self.ui.labelRebuildNativeStatus.setText(
+ self.tr(
+ ""
+ "Rebuilt {0} device extensions. "
+ ""
+ "Please restart URH.".format(len(extensions))
+ )
+ )
else:
- self.ui.labelRebuildNativeStatus.setText(self.tr(""
- "Failed to rebuild {0} device extensions. "
- ""
- "Run URH as root (sudo urh) "
- "and try again.".format(len(extensions))))
+ self.ui.labelRebuildNativeStatus.setText(
+ self.tr(
+ ""
+ "Failed to rebuild {0} device extensions. "
+ ""
+ "Run URH as root (sudo urh) "
+ "and try again.".format(len(extensions))
+ )
+ )
self.build_log = p.stdout.read().decode()
self.ui.btnViewBuildLog.show()
@pyqtSlot()
def on_checkbox_multiple_modulations_clicked(self):
- settings.write("multiple_modulations", self.ui.checkBoxMultipleModulations.isChecked())
+ settings.write(
+ "multiple_modulations", self.ui.checkBoxMultipleModulations.isChecked()
+ )
@pyqtSlot()
def on_btn_view_build_log_clicked(self):
@@ -459,11 +644,18 @@ def on_label_device_missing_info_link_activated(self, link: str):
if util.get_shared_library_path():
if sys.platform == "win32":
- info += "\n\n[INFO] Used DLLs from " + util.get_shared_library_path()
+ info += (
+ "\n\n[INFO] Used DLLs from " + util.get_shared_library_path()
+ )
else:
- info += "\n\n[INFO] Used shared libraries from " + util.get_shared_library_path()
-
- d = util.create_textbox_dialog(info, "Health check for native extensions", self)
+ info += (
+ "\n\n[INFO] Used shared libraries from "
+ + util.get_shared_library_path()
+ )
+
+ d = util.create_textbox_dialog(
+ info, "Health check for native extensions", self
+ )
d.show()
@pyqtSlot()
@@ -492,14 +684,14 @@ def on_radio_button_low_modulation_accuracy_clicked(self, checked):
def write_default_options():
keys = settings.all_keys()
- if 'default_view' not in keys:
- settings.write('default_view', 0)
+ if "default_view" not in keys:
+ settings.write("default_view", 0)
- if 'num_sending_repeats' not in keys:
- settings.write('num_sending_repeats', 0)
+ if "num_sending_repeats" not in keys:
+ settings.write("num_sending_repeats", 0)
- if 'show_pause_as_time' not in keys:
- settings.write('show_pause_as_time', False)
+ if "show_pause_as_time" not in keys:
+ settings.write("show_pause_as_time", False)
settings.sync() # Ensure conf dir is created to have field types in place
diff --git a/src/urh/controller/dialogs/ProjectDialog.py b/src/urh/controller/dialogs/ProjectDialog.py
index b78c29693b..7431dd125b 100644
--- a/src/urh/controller/dialogs/ProjectDialog.py
+++ b/src/urh/controller/dialogs/ProjectDialog.py
@@ -17,7 +17,9 @@
class ProjectDialog(QDialog):
- def __init__(self, new_project=True, project_manager: ProjectManager = None, parent=None):
+ def __init__(
+ self, new_project=True, project_manager: ProjectManager = None, parent=None
+ ):
super().__init__(parent)
if not new_project:
assert project_manager is not None
@@ -29,15 +31,23 @@ def __init__(self, new_project=True, project_manager: ProjectManager = None, par
if new_project:
self.participant_table_model = ParticipantTableModel([])
else:
- self.participant_table_model = ParticipantTableModel(project_manager.participants)
+ self.participant_table_model = ParticipantTableModel(
+ project_manager.participants
+ )
- self.ui.spinBoxSampleRate.setValue(project_manager.device_conf["sample_rate"])
+ self.ui.spinBoxSampleRate.setValue(
+ project_manager.device_conf["sample_rate"]
+ )
self.ui.spinBoxFreq.setValue(project_manager.device_conf["frequency"])
self.ui.spinBoxBandwidth.setValue(project_manager.device_conf["bandwidth"])
- self.ui.spinBoxGain.setValue(project_manager.device_conf.get("gain", config.DEFAULT_GAIN))
+ self.ui.spinBoxGain.setValue(
+ project_manager.device_conf.get("gain", config.DEFAULT_GAIN)
+ )
self.ui.txtEdDescription.setPlainText(project_manager.description)
self.ui.lineEdit_Path.setText(project_manager.project_path)
- self.ui.lineEditBroadcastAddress.setText(project_manager.broadcast_address_hex)
+ self.ui.lineEditBroadcastAddress.setText(
+ project_manager.broadcast_address_hex
+ )
self.ui.btnSelectPath.hide()
self.ui.lineEdit_Path.setDisabled(True)
@@ -47,7 +57,9 @@ def __init__(self, new_project=True, project_manager: ProjectManager = None, par
self.ui.tblParticipants.setModel(self.participant_table_model)
self.participant_table_model.update()
- self.ui.lineEditBroadcastAddress.setValidator(QRegExpValidator(QRegExp("([a-fA-F ]|[0-9]){,}")))
+ self.ui.lineEditBroadcastAddress.setValidator(
+ QRegExpValidator(QRegExp("([a-fA-F ]|[0-9]){,}"))
+ )
self.sample_rate = self.ui.spinBoxSampleRate.value()
self.freq = self.ui.spinBoxFreq.value()
@@ -72,10 +84,14 @@ def __init__(self, new_project=True, project_manager: ProjectManager = None, par
self.ui.btnAddParticipant.click()
if new_project:
- self.ui.lineEdit_Path.setText(os.path.realpath(os.path.join(os.curdir, "new")))
+ self.ui.lineEdit_Path.setText(
+ os.path.realpath(os.path.join(os.curdir, "new"))
+ )
self.on_line_edit_path_text_edited()
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
@property
def participants(self):
@@ -86,23 +102,43 @@ def participants(self):
return self.participant_table_model.participants
def create_connects(self):
- self.ui.spinBoxFreq.valueChanged.connect(self.on_spin_box_frequency_value_changed)
- self.ui.spinBoxSampleRate.valueChanged.connect(self.on_spin_box_sample_rate_value_changed)
- self.ui.spinBoxBandwidth.valueChanged.connect(self.on_spin_box_bandwidth_value_changed)
+ self.ui.spinBoxFreq.valueChanged.connect(
+ self.on_spin_box_frequency_value_changed
+ )
+ self.ui.spinBoxSampleRate.valueChanged.connect(
+ self.on_spin_box_sample_rate_value_changed
+ )
+ self.ui.spinBoxBandwidth.valueChanged.connect(
+ self.on_spin_box_bandwidth_value_changed
+ )
self.ui.spinBoxGain.valueChanged.connect(self.on_spin_box_gain_value_changed)
- self.ui.txtEdDescription.textChanged.connect(self.on_txt_edit_description_text_changed)
- self.ui.lineEditBroadcastAddress.textEdited.connect(self.on_line_edit_broadcast_address_text_edited)
-
- self.ui.btnAddParticipant.clicked.connect(self.ui.tblParticipants.on_add_action_triggered)
- self.ui.btnRemoveParticipant.clicked.connect(self.ui.tblParticipants.on_remove_action_triggered)
- self.ui.btnUp.clicked.connect(self.ui.tblParticipants.on_move_up_action_triggered)
- self.ui.btnDown.clicked.connect(self.ui.tblParticipants.on_move_down_action_triggered)
+ self.ui.txtEdDescription.textChanged.connect(
+ self.on_txt_edit_description_text_changed
+ )
+ self.ui.lineEditBroadcastAddress.textEdited.connect(
+ self.on_line_edit_broadcast_address_text_edited
+ )
+
+ self.ui.btnAddParticipant.clicked.connect(
+ self.ui.tblParticipants.on_add_action_triggered
+ )
+ self.ui.btnRemoveParticipant.clicked.connect(
+ self.ui.tblParticipants.on_remove_action_triggered
+ )
+ self.ui.btnUp.clicked.connect(
+ self.ui.tblParticipants.on_move_up_action_triggered
+ )
+ self.ui.btnDown.clicked.connect(
+ self.ui.tblParticipants.on_move_down_action_triggered
+ )
self.ui.lineEdit_Path.textEdited.connect(self.on_line_edit_path_text_edited)
self.ui.buttonBox.accepted.connect(self.on_button_box_accepted)
self.ui.buttonBox.rejected.connect(self.reject)
self.ui.btnSelectPath.clicked.connect(self.on_btn_select_path_clicked)
- self.ui.lOpenSpectrumAnalyzer.linkActivated.connect(self.on_spectrum_analyzer_link_activated)
+ self.ui.lOpenSpectrumAnalyzer.linkActivated.connect(
+ self.on_spectrum_analyzer_link_activated
+ )
def set_path(self, path):
self.path = path
@@ -113,7 +149,9 @@ def set_path(self, path):
self.ui.lblNewPath.setVisible(not os.path.isdir(self.path))
def closeEvent(self, event: QCloseEvent):
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
@pyqtSlot(float)
@@ -183,5 +221,7 @@ def on_spectrum_analyzer_link_activated(self, link: str):
r.close()
return
- r.device_parameters_changed.connect(self.set_recording_params_from_spectrum_analyzer_link)
+ r.device_parameters_changed.connect(
+ self.set_recording_params_from_spectrum_analyzer_link
+ )
r.show()
diff --git a/src/urh/controller/dialogs/ProtocolLabelDialog.py b/src/urh/controller/dialogs/ProtocolLabelDialog.py
index e6db628917..ea86795c2d 100644
--- a/src/urh/controller/dialogs/ProtocolLabelDialog.py
+++ b/src/urh/controller/dialogs/ProtocolLabelDialog.py
@@ -24,7 +24,9 @@ class ProtocolLabelDialog(QDialog):
SPECIAL_CONFIG_TYPES = [FieldType.Function.CHECKSUM]
- def __init__(self, message: Message, viewtype: int, selected_index=None, parent=None):
+ def __init__(
+ self, message: Message, viewtype: int, selected_index=None, parent=None
+ ):
super().__init__(parent)
self.ui = Ui_DialogLabels()
self.ui.setupUi(self)
@@ -33,24 +35,43 @@ def __init__(self, message: Message, viewtype: int, selected_index=None, parent=
field_types = FieldType.load_from_xml()
self.model = PLabelTableModel(message, field_types)
- self.ui.tblViewProtoLabels.setItemDelegateForColumn(0, ComboBoxDelegate([ft.caption for ft in field_types],
- is_editable=True,
- return_index=False, parent=self))
- self.ui.tblViewProtoLabels.setItemDelegateForColumn(1, SpinBoxDelegate(1, len(message), self))
- self.ui.tblViewProtoLabels.setItemDelegateForColumn(2, SpinBoxDelegate(1, len(message), self))
- self.ui.tblViewProtoLabels.setItemDelegateForColumn(3,
- ComboBoxDelegate([""] * len(settings.LABEL_COLORS),
- colors=settings.LABEL_COLORS,
- parent=self))
+ self.ui.tblViewProtoLabels.setItemDelegateForColumn(
+ 0,
+ ComboBoxDelegate(
+ [ft.caption for ft in field_types],
+ is_editable=True,
+ return_index=False,
+ parent=self,
+ ),
+ )
+ self.ui.tblViewProtoLabels.setItemDelegateForColumn(
+ 1, SpinBoxDelegate(1, len(message), self)
+ )
+ self.ui.tblViewProtoLabels.setItemDelegateForColumn(
+ 2, SpinBoxDelegate(1, len(message), self)
+ )
+ self.ui.tblViewProtoLabels.setItemDelegateForColumn(
+ 3,
+ ComboBoxDelegate(
+ [""] * len(settings.LABEL_COLORS),
+ colors=settings.LABEL_COLORS,
+ parent=self,
+ ),
+ )
self.ui.tblViewProtoLabels.setItemDelegateForColumn(4, CheckBoxDelegate(self))
self.ui.tblViewProtoLabels.setModel(self.model)
self.ui.tblViewProtoLabels.setEditTriggers(QAbstractItemView.AllEditTriggers)
- self.ui.tblViewProtoLabels.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
+ self.ui.tblViewProtoLabels.horizontalHeader().setSectionResizeMode(
+ QHeaderView.Stretch
+ )
self.ui.tblViewProtoLabels.resizeColumnsToContents()
self.setWindowFlags(Qt.Window)
- self.setWindowTitle(self.tr("Edit Protocol Labels From Message Type %s") % message.message_type.name)
+ self.setWindowTitle(
+ self.tr("Edit Protocol Labels From Message Type %s")
+ % message.message_type.name
+ )
self.configure_special_config_tabs()
self.ui.splitter.setSizes([int(self.height() / 2), int(self.height() / 2)])
@@ -58,13 +79,17 @@ def __init__(self, message: Message, viewtype: int, selected_index=None, parent=
self.create_connects()
if selected_index is not None:
- self.ui.tblViewProtoLabels.setCurrentIndex(self.model.index(selected_index, 0))
+ self.ui.tblViewProtoLabels.setCurrentIndex(
+ self.model.index(selected_index, 0)
+ )
self.ui.cbProtoView.setCurrentIndex(viewtype)
self.setAttribute(Qt.WA_DeleteOnClose)
self.setWindowFlags(Qt.Window)
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
for i in range(self.model.rowCount()):
self.open_editors(i)
@@ -75,24 +100,34 @@ def configure_special_config_tabs(self):
if isinstance(lbl, SimulatorProtocolLabel):
lbl = lbl.label
- if lbl.field_type is not None and lbl.field_type.function in self.SPECIAL_CONFIG_TYPES:
+ if (
+ lbl.field_type is not None
+ and lbl.field_type.function in self.SPECIAL_CONFIG_TYPES
+ ):
if isinstance(lbl, ChecksumLabel):
w = ChecksumWidget(lbl, self.model.message, self.model.proto_view)
self.ui.tabWidgetAdvancedSettings.addTab(w, lbl.name)
else:
- logger.error("No Special Config Dialog for field type " + lbl.field_type.caption)
+ logger.error(
+ "No Special Config Dialog for field type "
+ + lbl.field_type.caption
+ )
if self.ui.tabWidgetAdvancedSettings.count() > 0:
self.ui.tabWidgetAdvancedSettings.setCurrentIndex(0)
self.ui.tabWidgetAdvancedSettings.setFocus()
- self.ui.groupBoxAdvancedSettings.setVisible(self.ui.tabWidgetAdvancedSettings.count() > 0)
+ self.ui.groupBoxAdvancedSettings.setVisible(
+ self.ui.tabWidgetAdvancedSettings.count() > 0
+ )
def create_connects(self):
self.ui.btnConfirm.clicked.connect(self.confirm)
self.ui.cbProtoView.currentIndexChanged.connect(self.set_view_index)
self.model.apply_decoding_changed.connect(self.on_apply_decoding_changed)
- self.model.special_status_label_changed.connect(self.on_label_special_status_changed)
+ self.model.special_status_label_changed.connect(
+ self.on_label_special_status_changed
+ )
def open_editors(self, row):
self.ui.tblViewProtoLabels.openPersistentEditor(self.model.index(row, 4))
@@ -104,7 +139,9 @@ def keyPressEvent(self, event: QKeyEvent):
event.accept()
def closeEvent(self, event: QCloseEvent):
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
@pyqtSlot()
diff --git a/src/urh/controller/dialogs/ProtocolSniffDialog.py b/src/urh/controller/dialogs/ProtocolSniffDialog.py
index f0e6ca8942..8801fd9161 100644
--- a/src/urh/controller/dialogs/ProtocolSniffDialog.py
+++ b/src/urh/controller/dialogs/ProtocolSniffDialog.py
@@ -12,8 +12,17 @@
class ProtocolSniffDialog(SendRecvDialog):
protocol_accepted = pyqtSignal(list)
- def __init__(self, project_manager, signal=None, signals=None, parent=None, testing_mode=False):
- super().__init__(project_manager, is_tx=False, parent=parent, testing_mode=testing_mode)
+ def __init__(
+ self,
+ project_manager,
+ signal=None,
+ signals=None,
+ parent=None,
+ testing_mode=False,
+ ):
+ super().__init__(
+ project_manager, is_tx=False, parent=parent, testing_mode=testing_mode
+ )
self.graphics_view = self.ui.graphicsView_sniff_Preview
self.ui.stackedWidget.setCurrentWidget(self.ui.page_sniff)
@@ -24,11 +33,16 @@ def __init__(self, project_manager, signal=None, signals=None, parent=None, test
signals = [] if signals is None else signals
- self.sniff_settings_widget = SniffSettingsWidget(project_manager=project_manager,
- device_name=self.selected_device_name,
- signal=signal, signals=signals,
- backend_handler=self.backend_handler)
- self.ui.scrollAreaWidgetContents_2.layout().insertWidget(1, self.sniff_settings_widget)
+ self.sniff_settings_widget = SniffSettingsWidget(
+ project_manager=project_manager,
+ device_name=self.selected_device_name,
+ signal=signal,
+ signals=signals,
+ backend_handler=self.backend_handler,
+ )
+ self.ui.scrollAreaWidgetContents_2.layout().insertWidget(
+ 1, self.sniff_settings_widget
+ )
self.sniff_settings_widget.ui.btn_sniff_use_signal.setAutoDefault(False)
self.sniffer = self.sniff_settings_widget.sniffer
@@ -57,19 +71,27 @@ def closeEvent(self, event: QCloseEvent):
def create_connects(self):
super().create_connects()
self.ui.btnAccept.clicked.connect(self.on_btn_accept_clicked)
- self.sniff_settings_widget.sniff_parameters_changed.connect(self.device_parameters_changed.emit)
+ self.sniff_settings_widget.sniff_parameters_changed.connect(
+ self.device_parameters_changed.emit
+ )
- self.sniff_settings_widget.sniff_setting_edited.connect(self.on_sniff_setting_edited)
+ self.sniff_settings_widget.sniff_setting_edited.connect(
+ self.on_sniff_setting_edited
+ )
self.sniff_settings_widget.sniff_file_edited.connect(self.on_sniff_file_edited)
self.sniffer.message_sniffed.connect(self.on_message_sniffed)
- self.sniffer.qt_signals.sniff_device_errors_changed.connect(self.on_device_errors_changed)
+ self.sniffer.qt_signals.sniff_device_errors_changed.connect(
+ self.on_device_errors_changed
+ )
def init_device(self):
self.sniffer.device_name = self.selected_device_name
self.device = self.sniffer.rcv_device
self._create_device_connects()
- self.scene_manager = SniffSceneManager(np.array([], dtype=self.device.data_type), parent=self)
+ self.scene_manager = SniffSceneManager(
+ np.array([], dtype=self.device.data_type), parent=self
+ )
def emit_editing_finished_signals(self):
super().emit_editing_finished_signals()
@@ -84,7 +106,9 @@ def update_view(self):
@pyqtSlot()
def on_device_started(self):
- self.scene_manager.data_array = self.device.data.real if hasattr(self.device.data, "real") else None
+ self.scene_manager.data_array = (
+ self.device.data.real if hasattr(self.device.data, "real") else None
+ )
super().on_device_started()
@@ -93,8 +117,11 @@ def on_device_started(self):
@pyqtSlot()
def on_sniff_setting_edited(self):
- self.ui.txtEd_sniff_Preview.setPlainText(self.sniffer.decoded_to_string(self.view_type,
- include_timestamps=self.show_timestamp))
+ self.ui.txtEd_sniff_Preview.setPlainText(
+ self.sniffer.decoded_to_string(
+ self.view_type, include_timestamps=self.show_timestamp
+ )
+ )
@pyqtSlot()
def on_start_clicked(self):
@@ -118,11 +145,14 @@ def on_message_sniffed(self, index: int):
msg = self.sniffer.messages[index]
except IndexError:
return
- new_data = self.sniffer.message_to_string(msg, self.view_type, include_timestamps=self.show_timestamp)
+ new_data = self.sniffer.message_to_string(
+ msg, self.view_type, include_timestamps=self.show_timestamp
+ )
if new_data.strip():
self.ui.txtEd_sniff_Preview.appendPlainText(new_data)
self.ui.txtEd_sniff_Preview.verticalScrollBar().setValue(
- self.ui.txtEd_sniff_Preview.verticalScrollBar().maximum())
+ self.ui.txtEd_sniff_Preview.verticalScrollBar().maximum()
+ )
@pyqtSlot()
def on_btn_accept_clicked(self):
diff --git a/src/urh/controller/dialogs/ReceiveDialog.py b/src/urh/controller/dialogs/ReceiveDialog.py
index 4338b3aa5c..d319fbe1eb 100644
--- a/src/urh/controller/dialogs/ReceiveDialog.py
+++ b/src/urh/controller/dialogs/ReceiveDialog.py
@@ -10,12 +10,15 @@
from urh.util.Formatter import Formatter
from datetime import datetime
+
class ReceiveDialog(SendRecvDialog):
files_recorded = pyqtSignal(list, float)
def __init__(self, project_manager, parent=None, testing_mode=False):
try:
- super().__init__(project_manager, is_tx=False, parent=parent, testing_mode=testing_mode)
+ super().__init__(
+ project_manager, is_tx=False, parent=parent, testing_mode=testing_mode
+ )
except ValueError:
return
@@ -39,9 +42,12 @@ def create_connects(self):
def save_before_close(self):
if not self.already_saved and self.device.current_index > 0:
- reply = QMessageBox.question(self, self.tr("Save data?"),
- self.tr("Do you want to save the data you have captured so far?"),
- QMessageBox.Yes | QMessageBox.No | QMessageBox.Abort)
+ reply = QMessageBox.question(
+ self,
+ self.tr("Save data?"),
+ self.tr("Do you want to save the data you have captured so far?"),
+ QMessageBox.Yes | QMessageBox.No | QMessageBox.Abort,
+ )
if reply == QMessageBox.Yes:
self.on_save_clicked()
elif reply == QMessageBox.Abort:
@@ -63,10 +69,17 @@ def update_view(self):
self.graphics_view.update()
def init_device(self):
- self.device = VirtualDevice(self.backend_handler, self.selected_device_name, Mode.receive,
- device_ip="192.168.10.2", parent=self)
+ self.device = VirtualDevice(
+ self.backend_handler,
+ self.selected_device_name,
+ Mode.receive,
+ device_ip="192.168.10.2",
+ parent=self,
+ )
self._create_device_connects()
- self.scene_manager = LiveSceneManager(np.array([], dtype=self.device.data_type), parent=self)
+ self.scene_manager = LiveSceneManager(
+ np.array([], dtype=self.device.data_type), parent=self
+ )
@pyqtSlot()
def on_start_clicked(self):
@@ -75,7 +88,9 @@ def on_start_clicked(self):
@pyqtSlot()
def on_device_started(self):
- self.scene_manager.plot_data = self.device.data.real if self.device.data is not None else None
+ self.scene_manager.plot_data = (
+ self.device.data.real if self.device.data is not None else None
+ )
super().on_device_started()
@@ -90,21 +105,25 @@ def on_clear_clicked(self):
@pyqtSlot()
def on_save_clicked(self):
- data = self.device.data[:self.device.current_index]
+ data = self.device.data[: self.device.current_index]
dev = self.device
big_val = Formatter.big_value_with_suffix
timestamp = datetime.now().strftime("%Y%m%d_%H%M%S")
- initial_name = "{0}-{1}-{2}Hz-{3}Sps".format(dev.name, timestamp,
- big_val(dev.frequency), big_val(dev.sample_rate))
+ initial_name = "{0}-{1}-{2}Hz-{3}Sps".format(
+ dev.name, timestamp, big_val(dev.frequency), big_val(dev.sample_rate)
+ )
if dev.bandwidth_is_adjustable:
initial_name += "-{}Hz".format(big_val(dev.bandwidth))
- initial_name = initial_name.replace(Formatter.local_decimal_seperator(), "_").replace("_000", "")
+ initial_name = initial_name.replace(
+ Formatter.local_decimal_seperator(), "_"
+ ).replace("_000", "")
- filename = FileOperator.ask_signal_file_name_and_save(initial_name, data,
- sample_rate=dev.sample_rate, parent=self)
+ filename = FileOperator.ask_signal_file_name_and_save(
+ initial_name, data, sample_rate=dev.sample_rate, parent=self
+ )
self.already_saved = True
if filename is not None and filename not in self.recorded_files:
self.recorded_files.append(filename)
diff --git a/src/urh/controller/dialogs/SendDialog.py b/src/urh/controller/dialogs/SendDialog.py
index 550b59fc76..13df0fd465 100644
--- a/src/urh/controller/dialogs/SendDialog.py
+++ b/src/urh/controller/dialogs/SendDialog.py
@@ -13,10 +13,22 @@
class SendDialog(SendRecvDialog):
- def __init__(self, project_manager, modulated_data, modulation_msg_indices=None, continuous_send_mode=False,
- parent=None, testing_mode=False):
- super().__init__(project_manager, is_tx=True, continuous_send_mode=continuous_send_mode,
- parent=parent, testing_mode=testing_mode)
+ def __init__(
+ self,
+ project_manager,
+ modulated_data,
+ modulation_msg_indices=None,
+ continuous_send_mode=False,
+ parent=None,
+ testing_mode=False,
+ ):
+ super().__init__(
+ project_manager,
+ is_tx=True,
+ continuous_send_mode=continuous_send_mode,
+ parent=parent,
+ testing_mode=testing_mode,
+ )
self.graphics_view = self.ui.graphicsViewSend
self.ui.stackedWidget.setCurrentWidget(self.ui.page_send)
@@ -44,9 +56,14 @@ def __init__(self, project_manager, modulated_data, modulation_msg_indices=None,
signal = Signal("", "Modulated Preview", sample_rate=samp_rate)
signal.iq_array = modulated_data
self.scene_manager = SignalSceneManager(signal, parent=self)
- self.send_indicator = self.scene_manager.scene.addRect(0, -2, 0, 4,
- QPen(QColor(Qt.transparent), 0),
- QBrush(settings.SEND_INDICATOR_COLOR))
+ self.send_indicator = self.scene_manager.scene.addRect(
+ 0,
+ -2,
+ 0,
+ 4,
+ QPen(QColor(Qt.transparent), 0),
+ QBrush(settings.SEND_INDICATOR_COLOR),
+ )
self.send_indicator.stackBefore(self.scene_manager.scene.selection_area)
self.scene_manager.init_scene()
self.graphics_view.set_signal(signal)
@@ -58,17 +75,28 @@ def __init__(self, project_manager, modulated_data, modulation_msg_indices=None,
def create_connects(self):
super().create_connects()
- self.graphics_view.save_as_clicked.connect(self.on_graphics_view_save_as_clicked)
+ self.graphics_view.save_as_clicked.connect(
+ self.on_graphics_view_save_as_clicked
+ )
self.scene_manager.signal.data_edited.connect(self.on_signal_data_edited)
def _update_send_indicator(self, width: int):
- y, h = self.ui.graphicsViewSend.view_rect().y(), self.ui.graphicsViewSend.view_rect().height()
+ y, h = (
+ self.ui.graphicsViewSend.view_rect().y(),
+ self.ui.graphicsViewSend.view_rect().height(),
+ )
self.send_indicator.setRect(0, y - h, width, 2 * h + abs(y))
def set_current_message_progress_bar_value(self, current_sample: int):
if self.modulation_msg_indices is not None:
- msg_index = next((i for i, sample in enumerate(self.modulation_msg_indices) if sample >= current_sample),
- len(self.modulation_msg_indices))
+ msg_index = next(
+ (
+ i
+ for i, sample in enumerate(self.modulation_msg_indices)
+ if sample >= current_sample
+ ),
+ len(self.modulation_msg_indices),
+ )
self.ui.progressBarMessage.setValue(msg_index + 1)
def update_view(self):
@@ -78,7 +106,9 @@ def update_view(self):
self.set_current_message_progress_bar_value(self.device.current_index)
if not self.device.sending_finished:
- self.ui.lblCurrentRepeatValue.setText(str(self.device.current_iteration + 1))
+ self.ui.lblCurrentRepeatValue.setText(
+ str(self.device.current_iteration + 1)
+ )
else:
self.ui.btnStop.click()
self.ui.lblCurrentRepeatValue.setText("Sending finished")
@@ -88,8 +118,15 @@ def init_device(self):
num_repeats = self.device_settings_widget.ui.spinBoxNRepeat.value()
sts = self.scene_manager.signal.iq_array
- self.device = VirtualDevice(self.backend_handler, device_name, Mode.send, samples_to_send=sts,
- device_ip="192.168.10.2", sending_repeats=num_repeats, parent=self)
+ self.device = VirtualDevice(
+ self.backend_handler,
+ device_name,
+ Mode.send,
+ samples_to_send=sts,
+ device_ip="192.168.10.2",
+ sending_repeats=num_repeats,
+ parent=self,
+ )
self._create_device_connects()
@pyqtSlot()
diff --git a/src/urh/controller/dialogs/SendRecvDialog.py b/src/urh/controller/dialogs/SendRecvDialog.py
index fe66a13123..c97c29f410 100644
--- a/src/urh/controller/dialogs/SendRecvDialog.py
+++ b/src/urh/controller/dialogs/SendRecvDialog.py
@@ -9,7 +9,9 @@
from urh.controller.widgets.DeviceSettingsWidget import DeviceSettingsWidget
from urh.dev.BackendHandler import BackendHandler, Backends
from urh.dev.VirtualDevice import VirtualDevice
-from urh.plugins.NetworkSDRInterface.NetworkSDRInterfacePlugin import NetworkSDRInterfacePlugin
+from urh.plugins.NetworkSDRInterface.NetworkSDRInterfacePlugin import (
+ NetworkSDRInterfacePlugin,
+)
from urh.ui.ui_send_recv import Ui_SendRecvDialog
from urh.util import util
from urh.util.Errors import Errors
@@ -21,7 +23,14 @@
class SendRecvDialog(QDialog):
device_parameters_changed = pyqtSignal(dict)
- def __init__(self, project_manager: ProjectManager, is_tx: bool, continuous_send_mode=False, parent=None, testing_mode=False):
+ def __init__(
+ self,
+ project_manager: ProjectManager,
+ is_tx: bool,
+ continuous_send_mode=False,
+ parent=None,
+ testing_mode=False,
+ ):
super().__init__(parent)
self.is_tx = is_tx
self.update_interval = 25
@@ -47,16 +56,25 @@ def __init__(self, project_manager: ProjectManager, is_tx: bool, continuous_send
self.start = 0
- self.device_settings_widget = DeviceSettingsWidget(project_manager, is_tx,
- backend_handler=self.backend_handler,
- continuous_send_mode=continuous_send_mode)
- self.ui.scrollAreaWidgetContents_2.layout().insertWidget(0, self.device_settings_widget)
+ self.device_settings_widget = DeviceSettingsWidget(
+ project_manager,
+ is_tx,
+ backend_handler=self.backend_handler,
+ continuous_send_mode=continuous_send_mode,
+ )
+ self.ui.scrollAreaWidgetContents_2.layout().insertWidget(
+ 0, self.device_settings_widget
+ )
if testing_mode:
- self.device_settings_widget.ui.cbDevice.setCurrentText(NetworkSDRInterfacePlugin.NETWORK_SDR_NAME)
+ self.device_settings_widget.ui.cbDevice.setCurrentText(
+ NetworkSDRInterfacePlugin.NETWORK_SDR_NAME
+ )
self.timer = QTimer(self)
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
self.ui.splitter.setSizes([int(0.4 * self.width()), int(0.6 * self.width())])
@@ -89,13 +107,28 @@ def _eliminate_graphic_view(self):
self.graphics_view = None
def hide_send_ui_items(self):
- for item in ("lblCurrentRepeatValue", "progressBarMessage",
- "lblRepeatText", "lSamplesSentText", "progressBarSample", "labelCurrentMessage"):
+ for item in (
+ "lblCurrentRepeatValue",
+ "progressBarMessage",
+ "lblRepeatText",
+ "lSamplesSentText",
+ "progressBarSample",
+ "labelCurrentMessage",
+ ):
getattr(self.ui, item).hide()
def hide_receive_ui_items(self):
- for item in ("lSamplesCaptured", "lSamplesCapturedText", "lSignalSize", "lSignalSizeText",
- "lTime", "lTimeText", "btnSave", "labelReceiveBufferFull", "lReceiveBufferFullText"):
+ for item in (
+ "lSamplesCaptured",
+ "lSamplesCapturedText",
+ "lSignalSize",
+ "lSignalSizeText",
+ "lTime",
+ "lTimeText",
+ "btnSave",
+ "labelReceiveBufferFull",
+ "lReceiveBufferFullText",
+ ):
getattr(self.ui, item).hide()
def set_device_ui_items_enabled(self, enabled: bool):
@@ -109,8 +142,12 @@ def create_connects(self):
self.timer.timeout.connect(self.update_view)
self.ui.sliderYscale.valueChanged.connect(self.on_slider_y_scale_value_changed)
- self.device_settings_widget.selected_device_changed.connect(self.on_selected_device_changed)
- self.device_settings_widget.device_parameters_changed.connect(self.device_parameters_changed.emit)
+ self.device_settings_widget.selected_device_changed.connect(
+ self.on_selected_device_changed
+ )
+ self.device_settings_widget.device_parameters_changed.connect(
+ self.device_parameters_changed.emit
+ )
def _create_device_connects(self):
self.device.stopped.connect(self.on_device_stopped)
@@ -191,7 +228,9 @@ def __parse_error_messages(self, messages):
Errors.usrp_found()
self.on_clear_clicked()
- elif any(e in messages for e in ("hackrf_error_not_found", "hackrf_error_libusb")):
+ elif any(
+ e in messages for e in ("hackrf_error_not_found", "hackrf_error_libusb")
+ ):
self.device.stop_on_error("Could not establish connection to HackRF")
Errors.hackrf_not_found()
self.on_clear_clicked()
@@ -222,13 +261,24 @@ def update_view(self):
if len(new_messages) > 1:
self.ui.txtEditErrors.setPlainText(txt + new_messages)
- self.ui.lSamplesCaptured.setText(Formatter.big_value_with_suffix(self.device.current_index, decimals=1))
- self.ui.lSignalSize.setText(locale.format_string("%.2f", (8 * self.device.current_index) / (1024 ** 2)))
- self.ui.lTime.setText(locale.format_string("%.2f", self.device.current_index / self.device.sample_rate))
+ self.ui.lSamplesCaptured.setText(
+ Formatter.big_value_with_suffix(self.device.current_index, decimals=1)
+ )
+ self.ui.lSignalSize.setText(
+ locale.format_string("%.2f", (8 * self.device.current_index) / (1024**2))
+ )
+ self.ui.lTime.setText(
+ locale.format_string(
+ "%.2f", self.device.current_index / self.device.sample_rate
+ )
+ )
if self.is_rx and self.device.data is not None and len(self.device.data) > 0:
- self.ui.labelReceiveBufferFull.setText("{0}%".format(int(100 * self.device.current_index /
- len(self.device.data))))
+ self.ui.labelReceiveBufferFull.setText(
+ "{0}%".format(
+ int(100 * self.device.current_index / len(self.device.data))
+ )
+ )
if self.device.current_index == 0:
return False
@@ -269,7 +319,9 @@ def closeEvent(self, event: QCloseEvent):
logger.debug("Successfully cleaned up device")
self.device_settings_widget.emit_device_parameters_changed()
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
if self.device is not None:
self.device.free_data()
diff --git a/src/urh/controller/dialogs/SignalDetailsDialog.py b/src/urh/controller/dialogs/SignalDetailsDialog.py
index 894fdf6904..67c81fc8c3 100644
--- a/src/urh/controller/dialogs/SignalDetailsDialog.py
+++ b/src/urh/controller/dialogs/SignalDetailsDialog.py
@@ -26,22 +26,32 @@ def __init__(self, signal, parent=None):
if os.path.isfile(file):
self.ui.lblFile.setText(file)
- self.ui.lblFileSize.setText(locale.format_string("%.2fMB", os.path.getsize(file) / (1024 ** 2)))
+ self.ui.lblFileSize.setText(
+ locale.format_string("%.2fMB", os.path.getsize(file) / (1024**2))
+ )
self.ui.lFileCreated.setText(time.ctime(os.path.getctime(file)))
else:
self.ui.lblFile.setText(self.tr("signal file not found"))
self.ui.lblFileSize.setText("-")
self.ui.lFileCreated.setText("-")
- self.ui.lblSamplesTotal.setText("{0:n}".format(self.signal.num_samples).replace(",", " "))
+ self.ui.lblSamplesTotal.setText(
+ "{0:n}".format(self.signal.num_samples).replace(",", " ")
+ )
self.ui.dsb_sample_rate.setValue(self.signal.sample_rate)
self.set_duration()
- self.ui.dsb_sample_rate.valueChanged.connect(self.on_dsb_sample_rate_value_changed)
- self.restoreGeometry(settings.read("{}/geometry".format(self.__class__.__name__), type=bytes))
+ self.ui.dsb_sample_rate.valueChanged.connect(
+ self.on_dsb_sample_rate_value_changed
+ )
+ self.restoreGeometry(
+ settings.read("{}/geometry".format(self.__class__.__name__), type=bytes)
+ )
def closeEvent(self, event: QCloseEvent):
- settings.write("{}/geometry".format(self.__class__.__name__), self.saveGeometry())
+ settings.write(
+ "{}/geometry".format(self.__class__.__name__), self.saveGeometry()
+ )
super().closeEvent(event)
@pyqtSlot(float)
diff --git a/src/urh/controller/dialogs/SimulatorDialog.py b/src/urh/controller/dialogs/SimulatorDialog.py
index 24bf478642..e5a4ecdb7f 100644
--- a/src/urh/controller/dialogs/SimulatorDialog.py
+++ b/src/urh/controller/dialogs/SimulatorDialog.py
@@ -31,10 +31,16 @@ class SimulatorDialog(QDialog):
open_in_analysis_requested = pyqtSignal(str)
rx_file_saved = pyqtSignal(str)
- def __init__(self, simulator_config, modulators,
- expression_parser, project_manager: ProjectManager, signals: list = None,
- signal_tree_model=None,
- parent=None):
+ def __init__(
+ self,
+ simulator_config,
+ modulators,
+ expression_parser,
+ project_manager: ProjectManager,
+ signals: list = None,
+ signal_tree_model=None,
+ parent=None,
+ ):
super().__init__(parent)
self.ui = Ui_DialogSimulator()
self.ui.setupUi(self)
@@ -48,8 +54,9 @@ def __init__(self, simulator_config, modulators,
self.current_transcript_index = 0
- self.simulator_scene = SimulatorScene(mode=1,
- simulator_config=self.simulator_config)
+ self.simulator_scene = SimulatorScene(
+ mode=1, simulator_config=self.simulator_config
+ )
self.ui.gvSimulator.setScene(self.simulator_scene)
self.project_manager = project_manager
@@ -59,17 +66,22 @@ def __init__(self, simulator_config, modulators,
self.backend_handler = BackendHandler()
if self.rx_needed:
- self.device_settings_rx_widget = DeviceSettingsWidget(project_manager,
- is_tx=False,
- backend_handler=self.backend_handler)
-
- self.sniff_settings_widget = SniffSettingsWidget(self.device_settings_rx_widget.ui.cbDevice.currentText(),
- project_manager,
- signal=None,
- backend_handler=self.backend_handler,
- network_raw_mode=True, signals=signals)
-
- self.device_settings_rx_widget.device = self.sniff_settings_widget.sniffer.rcv_device
+ self.device_settings_rx_widget = DeviceSettingsWidget(
+ project_manager, is_tx=False, backend_handler=self.backend_handler
+ )
+
+ self.sniff_settings_widget = SniffSettingsWidget(
+ self.device_settings_rx_widget.ui.cbDevice.currentText(),
+ project_manager,
+ signal=None,
+ backend_handler=self.backend_handler,
+ network_raw_mode=True,
+ signals=signals,
+ )
+
+ self.device_settings_rx_widget.device = (
+ self.sniff_settings_widget.sniffer.rcv_device
+ )
self.sniff_settings_widget.ui.lineEdit_sniff_OutputFile.hide()
self.sniff_settings_widget.ui.label_sniff_OutputFile.hide()
@@ -77,15 +89,23 @@ def __init__(self, simulator_config, modulators,
self.sniff_settings_widget.ui.checkBox_sniff_Timestamp.hide()
self.sniff_settings_widget.ui.comboBox_sniff_viewtype.hide()
- self.ui.scrollAreaWidgetContentsRX.layout().insertWidget(0, self.device_settings_rx_widget)
- self.ui.scrollAreaWidgetContentsRX.layout().insertWidget(1, self.sniff_settings_widget)
+ self.ui.scrollAreaWidgetContentsRX.layout().insertWidget(
+ 0, self.device_settings_rx_widget
+ )
+ self.ui.scrollAreaWidgetContentsRX.layout().insertWidget(
+ 1, self.sniff_settings_widget
+ )
sniffer = self.sniff_settings_widget.sniffer
- self.scene_manager = SniffSceneManager(np.array([], dtype=sniffer.rcv_device.data_type), parent=self)
+ self.scene_manager = SniffSceneManager(
+ np.array([], dtype=sniffer.rcv_device.data_type), parent=self
+ )
self.ui.graphicsViewPreview.setScene(self.scene_manager.scene)
else:
- self.device_settings_rx_widget = self.sniff_settings_widget = self.scene_manager = None
+ self.device_settings_rx_widget = (
+ self.sniff_settings_widget
+ ) = self.scene_manager = None
self.ui.tabWidgetSimulatorSettings.setTabEnabled(1, False)
self.ui.graphicsViewPreview.hide()
self.ui.btnSaveRX.hide()
@@ -94,17 +114,25 @@ def __init__(self, simulator_config, modulators,
sniffer = None
if self.tx_needed:
- self.device_settings_tx_widget = DeviceSettingsWidget(project_manager, is_tx=True,
- backend_handler=self.backend_handler,
- continuous_send_mode=True)
+ self.device_settings_tx_widget = DeviceSettingsWidget(
+ project_manager,
+ is_tx=True,
+ backend_handler=self.backend_handler,
+ continuous_send_mode=True,
+ )
self.device_settings_tx_widget.ui.spinBoxNRepeat.hide()
self.device_settings_tx_widget.ui.labelNRepeat.hide()
- self.modulation_settings_widget = ModulationSettingsWidget(modulators, signal_tree_model=signal_tree_model,
- parent=None)
+ self.modulation_settings_widget = ModulationSettingsWidget(
+ modulators, signal_tree_model=signal_tree_model, parent=None
+ )
- self.ui.scrollAreaWidgetContentsTX.layout().insertWidget(0, self.device_settings_tx_widget)
- self.ui.scrollAreaWidgetContentsTX.layout().insertWidget(1, self.modulation_settings_widget)
+ self.ui.scrollAreaWidgetContentsTX.layout().insertWidget(
+ 0, self.device_settings_tx_widget
+ )
+ self.ui.scrollAreaWidgetContentsTX.layout().insertWidget(
+ 1, self.modulation_settings_widget
+ )
send_device = self.device_settings_tx_widget.ui.cbDevice.currentText()
sender = EndlessSender(self.backend_handler, send_device)
else:
@@ -113,8 +141,14 @@ def __init__(self, simulator_config, modulators,
sender = None
- self.simulator = Simulator(self.simulator_config, modulators, expression_parser, project_manager,
- sniffer=sniffer, sender=sender)
+ self.simulator = Simulator(
+ self.simulator_config,
+ modulators,
+ expression_parser,
+ project_manager,
+ sniffer=sniffer,
+ sender=sender,
+ )
if self.device_settings_tx_widget:
self.device_settings_tx_widget.device = self.simulator.sender.device
@@ -130,28 +164,48 @@ def __init__(self, simulator_config, modulators,
self.ui.textEditTranscript.setFont(util.get_monospace_font())
- if settings.read('default_view', 0, int) == 1:
+ if settings.read("default_view", 0, int) == 1:
self.ui.radioButtonTranscriptHex.setChecked(True)
def create_connects(self):
if self.rx_needed:
- self.device_settings_rx_widget.selected_device_changed.connect(self.on_selected_rx_device_changed)
- self.device_settings_rx_widget.device_parameters_changed.connect(self.rx_parameters_changed.emit)
+ self.device_settings_rx_widget.selected_device_changed.connect(
+ self.on_selected_rx_device_changed
+ )
+ self.device_settings_rx_widget.device_parameters_changed.connect(
+ self.rx_parameters_changed.emit
+ )
- self.sniff_settings_widget.sniff_parameters_changed.connect(self.sniff_parameters_changed.emit)
+ self.sniff_settings_widget.sniff_parameters_changed.connect(
+ self.sniff_parameters_changed.emit
+ )
self.ui.btnSaveRX.clicked.connect(self.on_btn_save_rx_clicked)
- self.ui.checkBoxCaptureFullRX.clicked.connect(self.on_checkbox_capture_full_rx_clicked)
+ self.ui.checkBoxCaptureFullRX.clicked.connect(
+ self.on_checkbox_capture_full_rx_clicked
+ )
- self.ui.btnTestSniffSettings.clicked.connect(self.on_btn_test_sniff_settings_clicked)
- self.ui.btnOpenInAnalysis.clicked.connect(self.on_btn_open_in_analysis_clicked)
+ self.ui.btnTestSniffSettings.clicked.connect(
+ self.on_btn_test_sniff_settings_clicked
+ )
+ self.ui.btnOpenInAnalysis.clicked.connect(
+ self.on_btn_open_in_analysis_clicked
+ )
if self.tx_needed:
- self.device_settings_tx_widget.selected_device_changed.connect(self.on_selected_tx_device_changed)
- self.device_settings_tx_widget.device_parameters_changed.connect(self.tx_parameters_changed.emit)
-
- self.ui.radioButtonTranscriptBit.clicked.connect(self.on_radio_button_transcript_bit_clicked)
- self.ui.radioButtonTranscriptHex.clicked.connect(self.on_radio_button_transcript_hex_clicked)
+ self.device_settings_tx_widget.selected_device_changed.connect(
+ self.on_selected_tx_device_changed
+ )
+ self.device_settings_tx_widget.device_parameters_changed.connect(
+ self.tx_parameters_changed.emit
+ )
+
+ self.ui.radioButtonTranscriptBit.clicked.connect(
+ self.on_radio_button_transcript_bit_clicked
+ )
+ self.ui.radioButtonTranscriptHex.clicked.connect(
+ self.on_radio_button_transcript_hex_clicked
+ )
self.simulator_scene.selectionChanged.connect(self.update_buttons)
self.simulator_config.items_updated.connect(self.update_buttons)
@@ -169,32 +223,47 @@ def create_connects(self):
def update_buttons(self):
selectable_items = self.simulator_scene.selectable_items()
- all_items_selected = all(item.model_item.logging_active for item in selectable_items)
- any_item_selected = any(item.model_item.logging_active for item in selectable_items)
+ all_items_selected = all(
+ item.model_item.logging_active for item in selectable_items
+ )
+ any_item_selected = any(
+ item.model_item.logging_active for item in selectable_items
+ )
self.ui.btnToggleLog.setEnabled(len(self.simulator_scene.selectedItems()))
self.ui.btnLogAll.setEnabled(not all_items_selected)
self.ui.btnLogNone.setEnabled(any_item_selected)
def __get_full_transcript(self) -> list:
- return self.simulator.transcript.get_for_all_participants(all_rounds=True,
- use_bit=self.ui.radioButtonTranscriptBit.isChecked())
+ return self.simulator.transcript.get_for_all_participants(
+ all_rounds=True, use_bit=self.ui.radioButtonTranscriptBit.isChecked()
+ )
def update_view(self):
- for device_message in filter(None, map(str.rstrip, self.simulator.device_messages())):
+ for device_message in filter(
+ None, map(str.rstrip, self.simulator.device_messages())
+ ):
self.ui.textEditDevices.append(device_message)
- for log_msg in filter(None, map(str.rstrip, self.simulator.read_log_messages())):
+ for log_msg in filter(
+ None, map(str.rstrip, self.simulator.read_log_messages())
+ ):
self.ui.textEditSimulation.append(log_msg)
transcript = self.__get_full_transcript()
- for line in transcript[self.current_transcript_index:]:
+ for line in transcript[self.current_transcript_index :]:
self.ui.textEditTranscript.append(line)
self.current_transcript_index = len(transcript)
- current_repeat = str(self.simulator.current_repeat + 1) if self.simulator.is_simulating else "-"
+ current_repeat = (
+ str(self.simulator.current_repeat + 1)
+ if self.simulator.is_simulating
+ else "-"
+ )
self.ui.lblCurrentRepeatValue.setText(current_repeat)
- current_item = self.simulator.current_item.index() if self.simulator.is_simulating else "-"
+ current_item = (
+ self.simulator.current_item.index() if self.simulator.is_simulating else "-"
+ )
self.ui.lblCurrentItemValue.setText(current_item)
def update_rx_graphics_view(self):
@@ -301,7 +370,9 @@ def on_btn_toggle_clicked(self):
@pyqtSlot()
def on_btn_save_log_clicked(self):
- file_path = QFileDialog.getSaveFileName(self, "Save log", "", "Log file (*.log)")
+ file_path = QFileDialog.getSaveFileName(
+ self, "Save log", "", "Log file (*.log)"
+ )
if file_path[0] == "":
return
@@ -316,7 +387,9 @@ def on_btn_save_log_clicked(self):
@pyqtSlot()
def on_btn_save_transcript_clicked(self):
- file_path = QFileDialog.getSaveFileName(self, "Save transcript", "", "Text file (*.txt)")
+ file_path = QFileDialog.getSaveFileName(
+ self, "Save transcript", "", "Text file (*.txt)"
+ )
if file_path[0] == "":
return
@@ -339,7 +412,9 @@ def on_btn_start_stop_clicked(self):
self.sniff_settings_widget.emit_editing_finished_signals()
self.simulator.sniffer.rcv_device.current_index = 0
- self.simulator.sniffer.rcv_device.resume_on_full_receive_buffer = not self.ui.checkBoxCaptureFullRX.isChecked()
+ self.simulator.sniffer.rcv_device.resume_on_full_receive_buffer = (
+ not self.ui.checkBoxCaptureFullRX.isChecked()
+ )
if self.tx_needed:
self.device_settings_tx_widget.emit_editing_finished_signals()
@@ -372,16 +447,26 @@ def on_selected_tx_device_changed(self):
@pyqtSlot()
def on_btn_test_sniff_settings_clicked(self):
def on_dialog_finished():
- self.device_settings_rx_widget.bootstrap(self.project_manager.simulator_rx_conf)
+ self.device_settings_rx_widget.bootstrap(
+ self.project_manager.simulator_rx_conf
+ )
self.sniff_settings_widget.bootstrap(self.project_manager.device_conf)
self.device_settings_rx_widget.emit_device_parameters_changed()
self.sniff_settings_widget.emit_sniff_parameters_changed()
- psd = ProtocolSniffDialog(self.project_manager, signals=self.sniff_settings_widget.signals, parent=self)
+ psd = ProtocolSniffDialog(
+ self.project_manager,
+ signals=self.sniff_settings_widget.signals,
+ parent=self,
+ )
psd.device_settings_widget.bootstrap(self.project_manager.simulator_rx_conf)
- psd.device_settings_widget.device_parameters_changed.connect(self.rx_parameters_changed.emit)
- psd.sniff_settings_widget.sniff_parameters_changed.connect(self.sniff_parameters_changed.emit)
+ psd.device_settings_widget.device_parameters_changed.connect(
+ self.rx_parameters_changed.emit
+ )
+ psd.sniff_settings_widget.sniff_parameters_changed.connect(
+ self.sniff_parameters_changed.emit
+ )
psd.finished.connect(on_dialog_finished)
psd.ui.btnAccept.hide()
psd.show()
@@ -399,27 +484,39 @@ def __set_rx_scene(self):
return
if self.ui.checkBoxCaptureFullRX.isChecked():
- self.scene_manager = LiveSceneManager(np.array([], dtype=self.simulator.sniffer.rcv_device.data_type),
- parent=self)
+ self.scene_manager = LiveSceneManager(
+ np.array([], dtype=self.simulator.sniffer.rcv_device.data_type),
+ parent=self,
+ )
self.ui.graphicsViewPreview.setScene(self.scene_manager.scene)
else:
- self.scene_manager = SniffSceneManager(np.array([], dtype=self.simulator.sniffer.rcv_device.data_type),
- parent=self)
+ self.scene_manager = SniffSceneManager(
+ np.array([], dtype=self.simulator.sniffer.rcv_device.data_type),
+ parent=self,
+ )
self.ui.graphicsViewPreview.setScene(self.scene_manager.scene)
@pyqtSlot()
def on_checkbox_capture_full_rx_clicked(self):
- self.simulator.sniffer.rcv_device.resume_on_full_receive_buffer = not self.ui.checkBoxCaptureFullRX.isChecked()
+ self.simulator.sniffer.rcv_device.resume_on_full_receive_buffer = (
+ not self.ui.checkBoxCaptureFullRX.isChecked()
+ )
self.__set_rx_scene()
@pyqtSlot()
def on_btn_save_rx_clicked(self):
rx_device = self.simulator.sniffer.rcv_device
- if isinstance(rx_device.data, np.ndarray) or isinstance(rx_device.data, IQArray):
- data = IQArray(rx_device.data[:rx_device.current_index])
- filename = FileOperator.ask_signal_file_name_and_save("simulation_capture", data,
- sample_rate=rx_device.sample_rate, parent=self)
+ if isinstance(rx_device.data, np.ndarray) or isinstance(
+ rx_device.data, IQArray
+ ):
+ data = IQArray(rx_device.data[: rx_device.current_index])
+ filename = FileOperator.ask_signal_file_name_and_save(
+ "simulation_capture",
+ data,
+ sample_rate=rx_device.sample_rate,
+ parent=self,
+ )
if filename:
data.tofile(filename)
self.rx_file_saved.emit(filename)
diff --git a/src/urh/controller/dialogs/SpectrumDialogController.py b/src/urh/controller/dialogs/SpectrumDialogController.py
index ab27115e27..079e9c80e4 100644
--- a/src/urh/controller/dialogs/SpectrumDialogController.py
+++ b/src/urh/controller/dialogs/SpectrumDialogController.py
@@ -10,7 +10,9 @@
class SpectrumDialogController(SendRecvDialog):
def __init__(self, project_manager, parent=None, testing_mode=False):
- super().__init__(project_manager, is_tx=False, parent=parent, testing_mode=testing_mode)
+ super().__init__(
+ project_manager, is_tx=False, parent=parent, testing_mode=testing_mode
+ )
self.graphics_view = self.ui.graphicsViewFFT
self.update_interval = 1
@@ -23,7 +25,9 @@ def __init__(self, project_manager, parent=None, testing_mode=False):
self.ui.btnStart.setToolTip(self.tr("Start"))
self.ui.btnStop.setToolTip(self.tr("Stop"))
- self.scene_manager = FFTSceneManager(parent=self, graphic_view=self.graphics_view)
+ self.scene_manager = FFTSceneManager(
+ parent=self, graphic_view=self.graphics_view
+ )
self.graphics_view.setScene(self.scene_manager.scene)
self.graphics_view.scene_manager = self.scene_manager
@@ -46,9 +50,13 @@ def __init__(self, project_manager, parent=None, testing_mode=False):
def __clear_spectrogram(self):
self.ui.graphicsViewSpectrogram.scene().clear()
window_size = Spectrogram.DEFAULT_FFT_WINDOW_SIZE
- self.ui.graphicsViewSpectrogram.scene().setSceneRect(0, 0, window_size, 20 * window_size)
+ self.ui.graphicsViewSpectrogram.scene().setSceneRect(
+ 0, 0, window_size, 20 * window_size
+ )
self.spectrogram_y_pos = 0
- self.ui.graphicsViewSpectrogram.fitInView(self.ui.graphicsViewSpectrogram.sceneRect())
+ self.ui.graphicsViewSpectrogram.fitInView(
+ self.ui.graphicsViewSpectrogram.sceneRect()
+ )
def __update_spectrogram(self):
spectrogram = Spectrogram(self.device.data)
@@ -60,12 +68,17 @@ def __update_spectrogram(self):
pixmap_item.moveBy(0, self.spectrogram_y_pos)
self.spectrogram_y_pos += pixmap.height()
if self.spectrogram_y_pos >= scene.sceneRect().height():
- scene.setSceneRect(0, 0, Spectrogram.DEFAULT_FFT_WINDOW_SIZE, self.spectrogram_y_pos)
+ scene.setSceneRect(
+ 0, 0, Spectrogram.DEFAULT_FFT_WINDOW_SIZE, self.spectrogram_y_pos
+ )
self.ui.graphicsViewSpectrogram.ensureVisible(pixmap_item)
def _eliminate_graphic_view(self):
super()._eliminate_graphic_view()
- if self.ui.graphicsViewSpectrogram and self.ui.graphicsViewSpectrogram.scene() is not None:
+ if (
+ self.ui.graphicsViewSpectrogram
+ and self.ui.graphicsViewSpectrogram.scene() is not None
+ ):
self.ui.graphicsViewSpectrogram.scene().clear()
self.ui.graphicsViewSpectrogram.scene().setParent(None)
self.ui.graphicsViewSpectrogram.setScene(None)
@@ -75,21 +88,41 @@ def _eliminate_graphic_view(self):
def create_connects(self):
super().create_connects()
self.graphics_view.freq_clicked.connect(self.on_graphics_view_freq_clicked)
- self.graphics_view.wheel_event_triggered.connect(self.on_graphics_view_wheel_event_triggered)
+ self.graphics_view.wheel_event_triggered.connect(
+ self.on_graphics_view_wheel_event_triggered
+ )
- self.device_settings_widget.ui.sliderGain.valueChanged.connect(self.on_slider_gain_value_changed)
+ self.device_settings_widget.ui.sliderGain.valueChanged.connect(
+ self.on_slider_gain_value_changed
+ )
self.device_settings_widget.ui.sliderBasebandGain.valueChanged.connect(
- self.on_slider_baseband_gain_value_changed)
- self.device_settings_widget.ui.sliderIFGain.valueChanged.connect(self.on_slider_if_gain_value_changed)
- self.device_settings_widget.ui.spinBoxFreq.editingFinished.connect(self.on_spinbox_frequency_editing_finished)
-
- self.gain_timer.timeout.connect(self.device_settings_widget.ui.spinBoxGain.editingFinished.emit)
- self.if_gain_timer.timeout.connect(self.device_settings_widget.ui.spinBoxIFGain.editingFinished.emit)
- self.bb_gain_timer.timeout.connect(self.device_settings_widget.ui.spinBoxBasebandGain.editingFinished.emit)
+ self.on_slider_baseband_gain_value_changed
+ )
+ self.device_settings_widget.ui.sliderIFGain.valueChanged.connect(
+ self.on_slider_if_gain_value_changed
+ )
+ self.device_settings_widget.ui.spinBoxFreq.editingFinished.connect(
+ self.on_spinbox_frequency_editing_finished
+ )
+
+ self.gain_timer.timeout.connect(
+ self.device_settings_widget.ui.spinBoxGain.editingFinished.emit
+ )
+ self.if_gain_timer.timeout.connect(
+ self.device_settings_widget.ui.spinBoxIFGain.editingFinished.emit
+ )
+ self.bb_gain_timer.timeout.connect(
+ self.device_settings_widget.ui.spinBoxBasebandGain.editingFinished.emit
+ )
def resizeEvent(self, event: QResizeEvent):
- if self.ui.graphicsViewSpectrogram and self.ui.graphicsViewSpectrogram.sceneRect():
- self.ui.graphicsViewSpectrogram.fitInView(self.ui.graphicsViewSpectrogram.sceneRect())
+ if (
+ self.ui.graphicsViewSpectrogram
+ and self.ui.graphicsViewSpectrogram.sceneRect()
+ ):
+ self.ui.graphicsViewSpectrogram.fitInView(
+ self.ui.graphicsViewSpectrogram.sceneRect()
+ )
def update_view(self):
if super().update_view():
@@ -109,9 +142,13 @@ def update_view(self):
self.__update_spectrogram()
def init_device(self):
- self.device = VirtualDevice(self.backend_handler, self.selected_device_name,
- Mode.spectrum,
- device_ip="192.168.10.2", parent=self)
+ self.device = VirtualDevice(
+ self.backend_handler,
+ self.selected_device_name,
+ Mode.spectrum,
+ device_ip="192.168.10.2",
+ parent=self,
+ )
self._create_device_connects()
@pyqtSlot(QWheelEvent)
@@ -138,7 +175,9 @@ def on_start_clicked(self):
@pyqtSlot()
def on_device_started(self):
- self.ui.graphicsViewSpectrogram.fitInView(self.ui.graphicsViewSpectrogram.scene().sceneRect())
+ self.ui.graphicsViewSpectrogram.fitInView(
+ self.ui.graphicsViewSpectrogram.scene().sceneRect()
+ )
super().on_device_started()
self.device_settings_widget.ui.spinBoxPort.setEnabled(False)
self.device_settings_widget.ui.lineEditIP.setEnabled(False)
diff --git a/src/urh/controller/widgets/ChecksumWidget.py b/src/urh/controller/widgets/ChecksumWidget.py
index 9cac49e4bf..5ecacf6c9f 100644
--- a/src/urh/controller/widgets/ChecksumWidget.py
+++ b/src/urh/controller/widgets/ChecksumWidget.py
@@ -17,15 +17,22 @@
class ChecksumWidget(QWidget):
- SPECIAL_CRCS = OrderedDict([
- ("CC1101", GenericCRC(polynomial="16_standard", start_value=True)),
- ])
-
+ SPECIAL_CRCS = OrderedDict(
+ [
+ ("CC1101", GenericCRC(polynomial="16_standard", start_value=True)),
+ ]
+ )
class RangeTableModel(QAbstractTableModel):
header_labels = ["Start", "End"]
- def __init__(self, checksum_label: ChecksumLabel, message: Message, proto_view: int, parent=None):
+ def __init__(
+ self,
+ checksum_label: ChecksumLabel,
+ message: Message,
+ proto_view: int,
+ parent=None,
+ ):
"""
:param message:
@@ -62,9 +69,16 @@ def data(self, index: QModelIndex, role=Qt.DisplayRole):
if role == Qt.DisplayRole:
data_range = self.checksum_label.data_ranges[i]
if j == 0:
- return self.message.convert_index(data_range[0], 0, self.proto_view, True)[0] + 1
+ return (
+ self.message.convert_index(
+ data_range[0], 0, self.proto_view, True
+ )[0]
+ + 1
+ )
elif j == 1:
- return self.message.convert_index(data_range[1], 0, self.proto_view, True)[0]
+ return self.message.convert_index(
+ data_range[1], 0, self.proto_view, True
+ )[0]
return None
def setData(self, index: QModelIndex, value, role: int = ...):
@@ -81,11 +95,15 @@ def setData(self, index: QModelIndex, value, role: int = ...):
data_range = self.checksum_label.data_ranges[i]
if j == 0:
- converted_index = self.message.convert_index(int_val - 1, self.proto_view, 0, True)[0]
+ converted_index = self.message.convert_index(
+ int_val - 1, self.proto_view, 0, True
+ )[0]
if converted_index < data_range[1]:
data_range[0] = converted_index
elif j == 1:
- converted_index = self.message.convert_index(int_val, self.proto_view, 0, True)[0]
+ converted_index = self.message.convert_index(
+ int_val, self.proto_view, 0, True
+ )[0]
if converted_index > data_range[0]:
data_range[1] = converted_index
@@ -102,21 +120,41 @@ def flags(self, index):
return Qt.ItemIsEditable | Qt.ItemIsEnabled | Qt.ItemIsSelectable
- def __init__(self, checksum_label: ChecksumLabel, message: Message, proto_view: int, parent=None):
+ def __init__(
+ self,
+ checksum_label: ChecksumLabel,
+ message: Message,
+ proto_view: int,
+ parent=None,
+ ):
super().__init__(parent)
self.ui = Ui_ChecksumOptions()
self.ui.setupUi(self)
self.checksum_label = checksum_label
- self.data_range_table_model = self.RangeTableModel(checksum_label, message, proto_view, parent=self)
- self.ui.tableViewDataRanges.setItemDelegateForColumn(0, SpinBoxDelegate(1, 999999, self))
- self.ui.tableViewDataRanges.setItemDelegateForColumn(1, SpinBoxDelegate(1, 999999, self))
- self.ui.tableViewDataRanges.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
+ self.data_range_table_model = self.RangeTableModel(
+ checksum_label, message, proto_view, parent=self
+ )
+ self.ui.tableViewDataRanges.setItemDelegateForColumn(
+ 0, SpinBoxDelegate(1, 999999, self)
+ )
+ self.ui.tableViewDataRanges.setItemDelegateForColumn(
+ 1, SpinBoxDelegate(1, 999999, self)
+ )
+ self.ui.tableViewDataRanges.horizontalHeader().setSectionResizeMode(
+ QHeaderView.Stretch
+ )
self.ui.tableViewDataRanges.setModel(self.data_range_table_model)
self.ui.tableViewDataRanges.setEditTriggers(QAbstractItemView.AllEditTriggers)
self.display_crc_data_ranges_in_table()
- self.ui.comboBoxCRCFunction.addItems([crc_name for crc_name in GenericCRC.DEFAULT_POLYNOMIALS])
- self.ui.comboBoxCRCFunction.addItems([special_crc_name for special_crc_name in self.SPECIAL_CRCS])
- self.ui.lineEditCRCPolynomial.setValidator(QRegExpValidator(QRegExp("[0-9,a-f]*")))
+ self.ui.comboBoxCRCFunction.addItems(
+ [crc_name for crc_name in GenericCRC.DEFAULT_POLYNOMIALS]
+ )
+ self.ui.comboBoxCRCFunction.addItems(
+ [special_crc_name for special_crc_name in self.SPECIAL_CRCS]
+ )
+ self.ui.lineEditCRCPolynomial.setValidator(
+ QRegExpValidator(QRegExp("[0-9,a-f]*"))
+ )
self.ui.comboBoxCategory.clear()
for _, member in self.checksum_label.Category.__members__.items():
self.ui.comboBoxCategory.addItem(member.value)
@@ -135,25 +173,49 @@ def proto_view(self, value):
self.data_range_table_model.update()
def create_connects(self):
- self.ui.comboBoxCRCFunction.currentIndexChanged.connect(self.on_combobox_crc_function_current_index_changed)
+ self.ui.comboBoxCRCFunction.currentIndexChanged.connect(
+ self.on_combobox_crc_function_current_index_changed
+ )
self.ui.btnAddRange.clicked.connect(self.on_btn_add_range_clicked)
self.ui.btnRemoveRange.clicked.connect(self.on_btn_remove_range_clicked)
- self.ui.lineEditCRCPolynomial.editingFinished.connect(self.on_line_edit_crc_polynomial_editing_finished)
- self.ui.lineEditStartValue.editingFinished.connect(self.on_line_edit_start_value_editing_finished)
- self.ui.lineEditFinalXOR.editingFinished.connect(self.on_line_edit_final_xor_editing_finished)
- self.ui.comboBoxCategory.currentIndexChanged.connect(self.on_combobox_category_current_index_changed)
- self.ui.radioButtonWSPAuto.clicked.connect(self.on_radio_button_wsp_auto_clicked)
- self.ui.radioButtonWSPChecksum4.clicked.connect(self.on_radio_button_wsp_checksum4_clicked)
- self.ui.radioButtonWSPChecksum8.clicked.connect(self.on_radio_button_wsp_checksum8_clicked)
- self.ui.radioButtonWSPCRC8.clicked.connect(self.on_radio_button_wsp_crc8_clicked)
+ self.ui.lineEditCRCPolynomial.editingFinished.connect(
+ self.on_line_edit_crc_polynomial_editing_finished
+ )
+ self.ui.lineEditStartValue.editingFinished.connect(
+ self.on_line_edit_start_value_editing_finished
+ )
+ self.ui.lineEditFinalXOR.editingFinished.connect(
+ self.on_line_edit_final_xor_editing_finished
+ )
+ self.ui.comboBoxCategory.currentIndexChanged.connect(
+ self.on_combobox_category_current_index_changed
+ )
+ self.ui.radioButtonWSPAuto.clicked.connect(
+ self.on_radio_button_wsp_auto_clicked
+ )
+ self.ui.radioButtonWSPChecksum4.clicked.connect(
+ self.on_radio_button_wsp_checksum4_clicked
+ )
+ self.ui.radioButtonWSPChecksum8.clicked.connect(
+ self.on_radio_button_wsp_checksum8_clicked
+ )
+ self.ui.radioButtonWSPCRC8.clicked.connect(
+ self.on_radio_button_wsp_crc8_clicked
+ )
self.ui.checkBoxRefIn.clicked.connect(self.on_check_box_ref_in_clicked)
self.ui.checkBoxRefOut.clicked.connect(self.on_check_box_ref_out_clicked)
def set_checksum_ui_elements(self):
if self.checksum_label.is_generic_crc:
- self.ui.lineEditCRCPolynomial.setText(self.checksum_label.checksum.polynomial_as_hex_str)
- self.ui.lineEditStartValue.setText(util.bit2hex(self.checksum_label.checksum.start_value))
- self.ui.lineEditFinalXOR.setText(util.bit2hex(self.checksum_label.checksum.final_xor))
+ self.ui.lineEditCRCPolynomial.setText(
+ self.checksum_label.checksum.polynomial_as_hex_str
+ )
+ self.ui.lineEditStartValue.setText(
+ util.bit2hex(self.checksum_label.checksum.start_value)
+ )
+ self.ui.lineEditFinalXOR.setText(
+ util.bit2hex(self.checksum_label.checksum.final_xor)
+ )
self.ui.checkBoxRefIn.setChecked(self.checksum_label.checksum.lsb_first)
self.ui.checkBoxRefOut.setChecked(self.checksum_label.checksum.reverse_all)
self.__set_crc_function_index()
@@ -162,9 +224,13 @@ def set_checksum_ui_elements(self):
elif self.checksum_label.category == self.checksum_label.Category.wsp:
if self.checksum_label.checksum.mode == WSPChecksum.ChecksumMode.auto:
self.ui.radioButtonWSPAuto.setChecked(True)
- elif self.checksum_label.checksum.mode == WSPChecksum.ChecksumMode.checksum4:
+ elif (
+ self.checksum_label.checksum.mode == WSPChecksum.ChecksumMode.checksum4
+ ):
self.ui.radioButtonWSPChecksum4.setChecked(True)
- elif self.checksum_label.checksum.mode == WSPChecksum.ChecksumMode.checksum8:
+ elif (
+ self.checksum_label.checksum.mode == WSPChecksum.ChecksumMode.checksum8
+ ):
self.ui.radioButtonWSPChecksum8.setChecked(True)
elif self.checksum_label.checksum.mode == WSPChecksum.ChecksumMode.crc8:
self.ui.radioButtonWSPCRC8.setChecked(True)
@@ -202,33 +268,63 @@ def __set_crc_function_index(self):
if not crc_found:
self.__add_and_select_custom_item()
- elif "Custom" in [self.ui.comboBoxCRCFunction.itemText(i) for i in range(self.ui.comboBoxCRCFunction.count())]:
- self.ui.comboBoxCRCFunction.removeItem(self.ui.comboBoxCRCFunction.count() - 1)
-
+ elif "Custom" in [
+ self.ui.comboBoxCRCFunction.itemText(i)
+ for i in range(self.ui.comboBoxCRCFunction.count())
+ ]:
+ self.ui.comboBoxCRCFunction.removeItem(
+ self.ui.comboBoxCRCFunction.count() - 1
+ )
def __set_crc_info_label(self):
crc = self.checksum_label.checksum # type: GenericCRC
- self.ui.label_crc_info.setText("CRC Summary:
" + html.escape(expr) + "" + str(err) + message = ( + "
" + " " * err.offset + "^
" + + html.escape(expr) + + "" + + str(err) + ) else: message = self.formula_help if is_formula else self.rule_condition_help @@ -67,15 +76,18 @@ def validate_expression(self, expr, is_formula=True): def evaluate_node(self, node): if isinstance(node, ast.BinOp): - return self.operators[type(node.op)](self.evaluate_node(node.left), - self.evaluate_node(node.right)) + return self.operators[type(node.op)]( + self.evaluate_node(node.left), self.evaluate_node(node.right) + ) elif isinstance(node, ast.UnaryOp): return self.operators[type(node.op)](self.evaluate_node(node.operand)) elif isinstance(node, ast.Compare): to_string = isinstance(node.comparators[0], ast.Str) - return self.operators[type(node.ops[0])](self.evaluate_attribute_node(node.left, to_string), - self.evaluate_node(node.comparators[0])) + return self.operators[type(node.ops[0])]( + self.evaluate_attribute_node(node.left, to_string), + self.evaluate_node(node.comparators[0]), + ) elif isinstance(node, ast.BoolOp): func = all if isinstance(node.op, ast.And) else any return func(self.evaluate_node(value) for value in node.values) @@ -90,15 +102,25 @@ def evaluate_node(self, node): def evaluate_attribute_node(self, node, to_string=False): identifier = node.value.id + "." + node.attr - if isinstance(self.simulator_config.item_dict[identifier], SimulatorProtocolLabel): + if isinstance( + self.simulator_config.item_dict[identifier], SimulatorProtocolLabel + ): label = self.simulator_config.item_dict[identifier] message = label.parent() start, end = message.get_label_range(label, 2 if to_string else 0, False) - return message.plain_ascii_str[start:end] if to_string else int(message.plain_bits_str[start:end], 2) - elif isinstance(self.simulator_config.item_dict[identifier], SimulatorCounterAction): + return ( + message.plain_ascii_str[start:end] + if to_string + else int(message.plain_bits_str[start:end], 2) + ) + elif isinstance( + self.simulator_config.item_dict[identifier], SimulatorCounterAction + ): return self.simulator_config.item_dict[identifier].value - elif isinstance(self.simulator_config.item_dict[identifier], SimulatorTriggerCommandAction): + elif isinstance( + self.simulator_config.item_dict[identifier], SimulatorTriggerCommandAction + ): return self.simulator_config.item_dict[identifier].return_code def validate_formula_node(self, node): @@ -106,13 +128,17 @@ def validate_formula_node(self, node): return elif isinstance(node, ast.BinOp): if type(node.op) not in self.op_formula: - self.raise_syntax_error("unknown operator", node.lineno, node.col_offset) + self.raise_syntax_error( + "unknown operator", node.lineno, node.col_offset + ) self.validate_formula_node(node.left) self.validate_formula_node(node.right) elif isinstance(node, ast.UnaryOp): if type(node.op) not in self.op_formula: - self.raise_syntax_error("unknown operator", node.lineno, node.col_offset) + self.raise_syntax_error( + "unknown operator", node.lineno, node.col_offset + ) self.validate_formula_node(node.operand) elif isinstance(node, ast.Attribute): @@ -123,7 +149,9 @@ def validate_formula_node(self, node): def validate_condition_node(self, node): if isinstance(node, ast.UnaryOp): if type(node.op) not in self.op_cond: - self.raise_syntax_error("unknown operator", node.lineno, node.col_offset) + self.raise_syntax_error( + "unknown operator", node.lineno, node.col_offset + ) self.validate_condition_node(node.operand) elif isinstance(node, ast.Compare): @@ -131,7 +159,9 @@ def validate_condition_node(self, node): self.raise_syntax_error("", node.lineno, node.col_offset) if type(node.ops[0]) not in self.op_cond: - self.raise_syntax_error("unknown operator", node.lineno, node.col_offset) + self.raise_syntax_error( + "unknown operator", node.lineno, node.col_offset + ) self.validate_compare_nodes(node.left, node.comparators[0]) elif isinstance(node, ast.BoolOp): @@ -142,15 +172,20 @@ def validate_condition_node(self, node): def validate_compare_nodes(self, left, right): if not isinstance(left, ast.Attribute): - self.raise_syntax_error("the left-hand side of a comparison must be a label identifier", - left.lineno, left.col_offset) + self.raise_syntax_error( + "the left-hand side of a comparison must be a label identifier", + left.lineno, + left.col_offset, + ) self.validate_attribute_node(left) if not isinstance(right, (ast.Num, ast.Str, ast.Attribute)): self.raise_syntax_error( "the right-hand side of a comparison must be a number, a string or a label identifier", - right.lineno, right.col_offset) + right.lineno, + right.col_offset, + ) if isinstance(right, ast.Attribute): self.validate_attribute_node(right) @@ -162,20 +197,32 @@ def validate_attribute_node(self, node): identifier = node.value.id + "." + node.attr if not self.is_valid_identifier(identifier): - self.raise_syntax_error("'" + identifier + "' is not a valid label identifier", - node.lineno, node.col_offset) + self.raise_syntax_error( + "'" + identifier + "' is not a valid label identifier", + node.lineno, + node.col_offset, + ) def is_valid_identifier(self, identifier): try: item = self.simulator_config.item_dict[identifier] - return isinstance(item, SimulatorProtocolLabel) or\ - isinstance(item, SimulatorCounterAction) or \ - (isinstance(item, SimulatorTriggerCommandAction) and identifier.endswith("rc")) + return ( + isinstance(item, SimulatorProtocolLabel) + or isinstance(item, SimulatorCounterAction) + or ( + isinstance(item, SimulatorTriggerCommandAction) + and identifier.endswith("rc") + ) + ) except KeyError: return False def get_identifiers(self): - return [identifier for identifier in self.simulator_config.item_dict if self.is_valid_identifier(identifier)] + return [ + identifier + for identifier in self.simulator_config.item_dict + if self.is_valid_identifier(identifier) + ] def raise_syntax_error(self, message, lineno, col_offset): if message == "": diff --git a/src/urh/simulator/SimulatorGotoAction.py b/src/urh/simulator/SimulatorGotoAction.py index a225adb90b..a30ea43fd6 100644 --- a/src/urh/simulator/SimulatorGotoAction.py +++ b/src/urh/simulator/SimulatorGotoAction.py @@ -3,7 +3,11 @@ from urh.simulator.SimulatorCounterAction import SimulatorCounterAction from urh.simulator.SimulatorItem import SimulatorItem from urh.simulator.SimulatorProtocolLabel import SimulatorProtocolLabel -from urh.simulator.SimulatorRule import SimulatorRule, SimulatorRuleCondition, ConditionType +from urh.simulator.SimulatorRule import ( + SimulatorRule, + SimulatorRuleCondition, + ConditionType, +) from urh.simulator.SimulatorTriggerCommandAction import SimulatorTriggerCommandAction @@ -20,7 +24,11 @@ def set_parent(self, value): @property def target(self): - return self.simulator_config.item_dict[self.goto_target] if self.validate() else None + return ( + self.simulator_config.item_dict[self.goto_target] + if self.validate() + else None + ) def validate(self): target = self.simulator_config.item_dict.get(self.goto_target, None) diff --git a/src/urh/simulator/SimulatorMessage.py b/src/urh/simulator/SimulatorMessage.py index 3b056492cb..5d233bbb1a 100644 --- a/src/urh/simulator/SimulatorMessage.py +++ b/src/urh/simulator/SimulatorMessage.py @@ -9,9 +9,19 @@ class SimulatorMessage(Message, SimulatorItem): - def __init__(self, destination: Participant, plain_bits, - pause: int, message_type: MessageType, decoder=None, source=None, timestamp=None): - Message.__init__(self, plain_bits, pause, message_type, decoder=decoder, participant=source) + def __init__( + self, + destination: Participant, + plain_bits, + pause: int, + message_type: MessageType, + decoder=None, + source=None, + timestamp=None, + ): + Message.__init__( + self, plain_bits, pause, message_type, decoder=decoder, participant=source + ) SimulatorItem.__init__(self) if timestamp is not None: self.timestamp = timestamp @@ -56,33 +66,62 @@ def plain_ascii_str(self) -> str: @property def plain_bits_str(self) -> str: - return str(self.send_recv_messages[-1]) if len(self.send_recv_messages) > 0 else str(self) + return ( + str(self.send_recv_messages[-1]) + if len(self.send_recv_messages) > 0 + else str(self) + ) def __delitem__(self, index): removed_labels = self._remove_labels_for_range(index, instant_remove=False) self.simulator_config.delete_items(removed_labels) del self.plain_bits[index] - def to_xml(self, decoders=None, include_message_type=False, write_bits=True) -> ET.Element: - result = ET.Element("simulator_message", - attrib={"destination_id": self.destination.id if self.destination else "", - "repeat": str(self.repeat)}) - - result.append(super().to_xml(decoders, include_message_type, write_bits=write_bits)) + def to_xml( + self, decoders=None, include_message_type=False, write_bits=True + ) -> ET.Element: + result = ET.Element( + "simulator_message", + attrib={ + "destination_id": self.destination.id if self.destination else "", + "repeat": str(self.repeat), + }, + ) + + result.append( + super().to_xml(decoders, include_message_type, write_bits=write_bits) + ) return result - def from_xml(self, tag: ET.Element, participants, decoders=None, message_types=None): + def from_xml( + self, tag: ET.Element, participants, decoders=None, message_types=None + ): super().from_xml(tag, participants, decoders, message_types) - self.destination = Participant.find_matching(tag.get("destination_id", ""), participants) + self.destination = Participant.find_matching( + tag.get("destination_id", ""), participants + ) self.repeat = Formatter.str2val(tag.get("repeat", "1"), int, 1) @classmethod - def new_from_xml(cls, tag: ET.Element, participants, decoders=None, message_types=None): - msg = Message.new_from_xml(tag.find("message"), - participants=participants, - decoders=decoders, - message_types=message_types) - destination = Participant.find_matching(tag.get("destination_id", ""), participants) - return SimulatorMessage(destination, msg.plain_bits, msg.pause, msg.message_type, msg.decoder, msg.participant, - timestamp=msg.timestamp) + def new_from_xml( + cls, tag: ET.Element, participants, decoders=None, message_types=None + ): + msg = Message.new_from_xml( + tag.find("message"), + participants=participants, + decoders=decoders, + message_types=message_types, + ) + destination = Participant.find_matching( + tag.get("destination_id", ""), participants + ) + return SimulatorMessage( + destination, + msg.plain_bits, + msg.pause, + msg.message_type, + msg.decoder, + msg.participant, + timestamp=msg.timestamp, + ) diff --git a/src/urh/simulator/SimulatorProtocolLabel.py b/src/urh/simulator/SimulatorProtocolLabel.py index 97ba1c49a4..bd2d592180 100644 --- a/src/urh/simulator/SimulatorProtocolLabel.py +++ b/src/urh/simulator/SimulatorProtocolLabel.py @@ -10,7 +10,13 @@ class SimulatorProtocolLabel(SimulatorItem): - VALUE_TYPES = ["Constant value", "Live input", "Formula", "External program", "Random value"] + VALUE_TYPES = [ + "Constant value", + "Live input", + "Formula", + "External program", + "Random value", + ] def __init__(self, label: ProtocolLabel): super().__init__() @@ -87,11 +93,16 @@ def validate(self): return result def to_xml(self) -> ET.Element: - result = ET.Element("simulator_label", attrib={"value_type_index": str(self.value_type_index), - "external_program": str(self.external_program), - "formula": str(self.formula), - "random_min": str(self.random_min), - "random_max": str(self.random_max)}) + result = ET.Element( + "simulator_label", + attrib={ + "value_type_index": str(self.value_type_index), + "external_program": str(self.external_program), + "formula": str(self.formula), + "random_min": str(self.random_min), + "random_max": str(self.random_max), + }, + ) result.append(self.label.to_xml()) return result @@ -107,11 +118,17 @@ def from_xml(cls, tag: ET.Element, field_types_by_caption=None): if label_tag is not None: label = ProtocolLabel.from_xml(label_tag, field_types_by_caption) else: - label = ChecksumLabel.from_xml(tag.find("checksum_label"), field_types_by_caption) + label = ChecksumLabel.from_xml( + tag.find("checksum_label"), field_types_by_caption + ) result = SimulatorProtocolLabel(label) - result.value_type_index = Formatter.str2val(tag.get("value_type_index", "0"), int) + result.value_type_index = Formatter.str2val( + tag.get("value_type_index", "0"), int + ) result.external_program = tag.get("external_program", "") result.formula = tag.get("formula", "") result.random_min = Formatter.str2val(tag.get("random_min", "0"), int) - result.random_max = Formatter.str2val(tag.get("random_max", str(label.fuzz_maximum-1)), int) + result.random_max = Formatter.str2val( + tag.get("random_max", str(label.fuzz_maximum - 1)), int + ) return result diff --git a/src/urh/simulator/SimulatorRule.py b/src/urh/simulator/SimulatorRule.py index cc30ab3a22..d292efc65c 100644 --- a/src/urh/simulator/SimulatorRule.py +++ b/src/urh/simulator/SimulatorRule.py @@ -22,7 +22,14 @@ def get_first_applying_condition(self): return next((child for child in self.children if child.condition_applies), None) def next_item(self): - return next((c.children[0] for c in self.children if c.condition_applies and c.child_count()), self.next_sibling()) + return next( + ( + c.children[0] + for c in self.children + if c.condition_applies and c.child_count() + ), + self.next_sibling(), + ) def to_xml(self) -> ET.Element: return ET.Element("simulator_rule") @@ -49,7 +56,9 @@ def condition_applies(self) -> bool: if self.type is ConditionType.ELSE: return True - valid, _, node = self.expression_parser.validate_expression(self.condition, is_formula=False) + valid, _, node = self.expression_parser.validate_expression( + self.condition, is_formula=False + ) assert valid == True and node is not None return self.expression_parser.evaluate_node(node) @@ -63,12 +72,16 @@ def validate(self): if self.type is ConditionType.ELSE: return True - result, _, _ = self.expression_parser.validate_expression(self.condition, is_formula=False) + result, _, _ = self.expression_parser.validate_expression( + self.condition, is_formula=False + ) return result def to_xml(self): - return ET.Element("simulator_rule_condition", attrib={"type": self.type.value, - "condition": self.condition}) + return ET.Element( + "simulator_rule_condition", + attrib={"type": self.type.value, "condition": self.condition}, + ) @classmethod def from_xml(cls, tag: ET.Element): diff --git a/src/urh/simulator/Transcript.py b/src/urh/simulator/Transcript.py index 4353d33f29..0ea85ff7eb 100644 --- a/src/urh/simulator/Transcript.py +++ b/src/urh/simulator/Transcript.py @@ -8,7 +8,9 @@ class Transcript(object): def __init__(self): self.__data = [] - def append(self, source: Participant, destination: Participant, msg: Message, index: int): + def append( + self, source: Participant, destination: Participant, msg: Message, index: int + ): if len(self.__data) == 0: self.__data.append([]) @@ -26,12 +28,20 @@ def get_for_all_participants(self, all_rounds: bool, use_bit=True) -> list: if len(self.__data) == 0: return result - rng = range(0, len(self.__data)) if all_rounds else range(len(self.__data)-1, len(self.__data)) + rng = ( + range(0, len(self.__data)) + if all_rounds + else range(len(self.__data) - 1, len(self.__data)) + ) for i in rng: for source, destination, msg, msg_index in self.__data[i]: data = msg.plain_bits_str if use_bit else msg.plain_hex_str - result.append(self.FORMAT.format(msg_index, source.shortname, destination.shortname, data)) + result.append( + self.FORMAT.format( + msg_index, source.shortname, destination.shortname, data + ) + ) if i != len(self.__data) - 1: result.append("") diff --git a/src/urh/simulator/UnlabeledRangeItem.py b/src/urh/simulator/UnlabeledRangeItem.py index 7ab832ae89..45a52e272e 100644 --- a/src/urh/simulator/UnlabeledRangeItem.py +++ b/src/urh/simulator/UnlabeledRangeItem.py @@ -1,6 +1,7 @@ from PyQt5.QtWidgets import QGraphicsTextItem from PyQt5.QtGui import QFontDatabase + class UnlabeledRangeItem(QGraphicsTextItem): def __init__(self, parent): super().__init__(parent) @@ -8,4 +9,4 @@ def __init__(self, parent): font = QFontDatabase.systemFont(QFontDatabase.FixedFont) font.setPointSize(8) self.setFont(font) - self.setPlainText("...") \ No newline at end of file + self.setPlainText("...") diff --git a/src/urh/ui/ExpressionLineEdit.py b/src/urh/ui/ExpressionLineEdit.py index b2604a63be..3f29095c2d 100644 --- a/src/urh/ui/ExpressionLineEdit.py +++ b/src/urh/ui/ExpressionLineEdit.py @@ -6,7 +6,9 @@ class ExpressionLineEdit(QLineEdit): - fld_abbrev_chars = ".0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ_abcdefghijklmnopqrstuvwxyz" + fld_abbrev_chars = ( + ".0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ_abcdefghijklmnopqrstuvwxyz" + ) def __init__(self, parent=None): super().__init__(parent) @@ -25,7 +27,9 @@ def setValidator(self, validator: RuleExpressionValidator): def on_validation_status_changed(self, status, message): if status == QValidator.Intermediate: col = settings.ERROR_BG_COLOR - bg_string = "background-color: rgba({}, {}, {}, {})".format(col.red(), col.green(), col.blue(), col.alpha()) + bg_string = "background-color: rgba({}, {}, {}, {})".format( + col.red(), col.green(), col.blue(), col.alpha() + ) style_sheet = "QLineEdit {" + bg_string + "}" else: style_sheet = "" @@ -41,14 +45,18 @@ def keyPressEvent(self, event): self.e_completer.setCompletionPrefix(token_word) - if (len(token_word) < 1 or (self.e_completer.completionCount() == 1 and - self.e_completer.currentCompletion() == token_word)): + if len(token_word) < 1 or ( + self.e_completer.completionCount() == 1 + and self.e_completer.currentCompletion() == token_word + ): self.e_completer.popup().hide() return cr = self.cursorRect() - cr.setWidth(self.e_completer.popup().sizeHintForColumn(0) + - self.e_completer.popup().verticalScrollBar().sizeHint().width()) + cr.setWidth( + self.e_completer.popup().sizeHintForColumn(0) + + self.e_completer.popup().verticalScrollBar().sizeHint().width() + ) self.e_completer.complete(cr) @@ -71,4 +79,4 @@ def insert_completion(self, completion_text): start, end = self.get_token_under_cursor() new_text = self.text()[:start] + completion_text + self.text()[end:] self.setText(new_text) - self.setCursorPosition(start + len(completion_text)) \ No newline at end of file + self.setCursorPosition(start + len(completion_text)) diff --git a/src/urh/ui/KillerDoubleSpinBox.py b/src/urh/ui/KillerDoubleSpinBox.py index 6eac29ad04..b458625221 100644 --- a/src/urh/ui/KillerDoubleSpinBox.py +++ b/src/urh/ui/KillerDoubleSpinBox.py @@ -11,6 +11,7 @@ class KillerDoubleSpinBox(QDoubleSpinBox): """ Print values with suffix (G,M,K) """ + def __init__(self, parent=None): super().__init__(parent) @@ -41,12 +42,12 @@ def adjust_step(self): self.setSingleStep(10 ** -(self.decimals())) def textFromValue(self, value: float): - if abs(value) >= 10 ** 9: - result, suffix = super().textFromValue(value / 10 ** 9), "G" - elif abs(value) >= 10 ** 6: - result, suffix = super().textFromValue(value / 10 ** 6), "M" - elif abs(value) >= 10 ** 3: - result, suffix = super().textFromValue(value / 10 ** 3), "K" + if abs(value) >= 10**9: + result, suffix = super().textFromValue(value / 10**9), "G" + elif abs(value) >= 10**6: + result, suffix = super().textFromValue(value / 10**6), "M" + elif abs(value) >= 10**3: + result, suffix = super().textFromValue(value / 10**3), "K" else: result, suffix = super().textFromValue(value), "" @@ -61,11 +62,11 @@ def textFromValue(self, value: float): def valueFromText(self, text: str): if text.endswith("G") or text.endswith("g"): - return QLocale().toDouble(text[:-1])[0] * 10 ** 9 + return QLocale().toDouble(text[:-1])[0] * 10**9 elif text.endswith("M") or text.endswith("m"): - return QLocale().toDouble(text[:-1])[0] * 10 ** 6 + return QLocale().toDouble(text[:-1])[0] * 10**6 elif text.endswith("K") or text.endswith("k"): - return QLocale().toDouble(text[:-1])[0] * 10 ** 3 + return QLocale().toDouble(text[:-1])[0] * 10**3 else: return QLocale().toDouble(text.rstrip(self.suffix()))[0] @@ -74,5 +75,9 @@ def validate(self, inpt: str, pos: int): rx = QRegExp("^(-?[0-9]+)[.]?[0-9]*[kKmMgG]?$") else: rx = QRegExp("^(-?[0-9]+)[.]?[0-9]*[{}]?$".format(self.suffix())) - result = QValidator.Acceptable if rx.exactMatch(inpt.replace(",", ".")) else QValidator.Invalid + result = ( + QValidator.Acceptable + if rx.exactMatch(inpt.replace(",", ".")) + else QValidator.Invalid + ) return result, inpt, pos diff --git a/src/urh/ui/ListWidget.py b/src/urh/ui/ListWidget.py index 38e6cba0c6..82b9d964f0 100644 --- a/src/urh/ui/ListWidget.py +++ b/src/urh/ui/ListWidget.py @@ -24,7 +24,7 @@ def dropEvent(self, event: QDropEvent): index = self.indexFromItem(item).row() self.setCurrentRow(index) else: - self.setCurrentRow(self.count()-1) + self.setCurrentRow(self.count() - 1) def dragEnterEvent(self, event: QDragEnterEvent): self.active_element = self.indexAt(event.pos()).row() @@ -34,8 +34,11 @@ def dragEnterEvent(self, event: QDragEnterEvent): def eventFilter(self, sender, event): if event.type() == QEvent.ChildRemoved: self.internalMove.emit() - elif event.type() == QEvent.KeyPress and event.key() in (Qt.Key_Delete, Qt.Key_Backspace)\ - and self.currentItem() is not None: + elif ( + event.type() == QEvent.KeyPress + and event.key() in (Qt.Key_Delete, Qt.Key_Backspace) + and self.currentItem() is not None + ): item = self.currentRow() item_name = self.currentItem().text() self.active_element_text = item_name @@ -86,7 +89,7 @@ def on_disable_function_triggered(self): item = self.indexAt(self.context_menu_pos).row() item_name = self.item(item).text() if settings.DECODING_DISABLED_PREFIX in item_name: - item_name = item_name[len(settings.DECODING_DISABLED_PREFIX):] + item_name = item_name[len(settings.DECODING_DISABLED_PREFIX) :] else: item_name = settings.DECODING_DISABLED_PREFIX + item_name self.takeItem(item) diff --git a/src/urh/ui/RuleExpressionValidator.py b/src/urh/ui/RuleExpressionValidator.py index 1cbfdbf73e..99e54db0e8 100644 --- a/src/urh/ui/RuleExpressionValidator.py +++ b/src/urh/ui/RuleExpressionValidator.py @@ -1,6 +1,7 @@ from PyQt5.QtGui import QValidator from PyQt5.QtCore import pyqtSignal + class RuleExpressionValidator(QValidator): validation_status_changed = pyqtSignal(QValidator.State, str) @@ -14,4 +15,4 @@ def validate(self, text, pos): state = QValidator.Acceptable if valid else QValidator.Intermediate self.validation_status_changed.emit(state, message) - return (state, text, pos) \ No newline at end of file + return (state, text, pos) diff --git a/src/urh/ui/ScrollArea.py b/src/urh/ui/ScrollArea.py index 7adbea0b75..26623c1ada 100644 --- a/src/urh/ui/ScrollArea.py +++ b/src/urh/ui/ScrollArea.py @@ -2,6 +2,7 @@ from PyQt5.QtGui import QDropEvent, QDragEnterEvent, QWheelEvent from PyQt5.QtWidgets import QScrollArea + class ScrollArea(QScrollArea): files_dropped = pyqtSignal(list) diff --git a/src/urh/ui/SimulatorScene.py b/src/urh/ui/SimulatorScene.py index ccae507498..6016b4ed1b 100644 --- a/src/urh/ui/SimulatorScene.py +++ b/src/urh/ui/SimulatorScene.py @@ -2,14 +2,23 @@ from PyQt5.QtCore import pyqtSignal from PyQt5.QtGui import QDropEvent -from PyQt5.QtWidgets import QGraphicsScene, QGraphicsSceneDragDropEvent, QAbstractItemView +from PyQt5.QtWidgets import ( + QGraphicsScene, + QGraphicsSceneDragDropEvent, + QAbstractItemView, +) from urh.signalprocessing.FieldType import FieldType from urh.signalprocessing.Message import Message from urh.signalprocessing.MessageType import MessageType from urh.signalprocessing.Participant import Participant -from urh.simulator.ActionItem import ActionItem, GotoActionItem, TriggerCommandActionItem, SleepActionItem, \ - CounterActionItem +from urh.simulator.ActionItem import ( + ActionItem, + GotoActionItem, + TriggerCommandActionItem, + SleepActionItem, + CounterActionItem, +) from urh.simulator.GraphicsItem import GraphicsItem from urh.simulator.LabelItem import LabelItem from urh.simulator.MessageItem import MessageItem @@ -21,7 +30,11 @@ from urh.simulator.SimulatorItem import SimulatorItem from urh.simulator.SimulatorMessage import SimulatorMessage from urh.simulator.SimulatorProtocolLabel import SimulatorProtocolLabel -from urh.simulator.SimulatorRule import SimulatorRule, SimulatorRuleCondition, ConditionType +from urh.simulator.SimulatorRule import ( + SimulatorRule, + SimulatorRuleCondition, + ConditionType, +) from urh.simulator.SimulatorSleepAction import SimulatorSleepAction from urh.simulator.SimulatorTriggerCommandAction import SimulatorTriggerCommandAction @@ -37,10 +50,12 @@ class SimulatorScene(QGraphicsScene): SimulatorCounterAction: CounterActionItem, SimulatorSleepAction: SleepActionItem, SimulatorMessage: MessageItem, - SimulatorProtocolLabel: LabelItem + SimulatorProtocolLabel: LabelItem, } - def __init__(self, mode: int, simulator_config: SimulatorConfiguration, parent=None): + def __init__( + self, mode: int, simulator_config: SimulatorConfiguration, parent=None + ): super().__init__(parent) self.mode = mode self.simulator_config = simulator_config @@ -49,7 +64,9 @@ def __init__(self, mode: int, simulator_config: SimulatorConfiguration, parent=N self.participants_dict = {} self.participant_items = [] - self.broadcast_part = self.insert_participant(self.simulator_config.broadcast_part) + self.broadcast_part = self.insert_participant( + self.simulator_config.broadcast_part + ) self.not_assigned_part = self.insert_participant(None) self.update_participants(refresh=False) @@ -65,7 +82,11 @@ def visible_participants(self): @property def visible_participants_without_broadcast(self): - return [part for part in self.participant_items if part.isVisible() and part is not self.broadcast_part] + return [ + part + for part in self.participant_items + if part.isVisible() and part is not self.broadcast_part + ] def create_connects(self): self.simulator_config.participants_changed.connect(self.update_participants) @@ -118,7 +139,7 @@ def on_item_added(self, item: SimulatorItem): self.on_item_added(child) def model_to_scene(self, model_item: SimulatorItem): - if (model_item is None or model_item is self.simulator_config.rootItem): + if model_item is None or model_item is self.simulator_config.rootItem: return None try: @@ -152,7 +173,11 @@ def get_parent_scene_item(self, item: GraphicsItem): def min_items_width(self): width = 0 - items = [item for item in self.items() if isinstance(item, (RuleConditionItem, ActionItem))] + items = [ + item + for item in self.items() + if isinstance(item, (RuleConditionItem, ActionItem)) + ] for item in items: if item.labels_width() > width: @@ -182,7 +207,6 @@ def log_selected_items(self, logging_active: bool): self.log_items(items, logging_active) def log_items(self, items, logging_active: bool): - for item in items: item.model_item.logging_active = logging_active @@ -200,8 +224,11 @@ def log_all_items(self, logging_active: bool): self.log_items(self.selectable_items(), logging_active) def selectable_items(self): - return [item for item in self.items() if isinstance(item, GraphicsItem) and - item.is_selectable()] + return [ + item + for item in self.items() + if isinstance(item, GraphicsItem) and item.is_selectable() + ] def move_items(self, items, ref_item, position): new_pos, new_parent = self.insert_at(ref_item, position) @@ -231,7 +258,10 @@ def update_view(self): def update_participants(self, refresh=True): for participant in list(self.participants_dict): - if participant is None or participant == self.simulator_config.broadcast_part: + if ( + participant is None + or participant == self.simulator_config.broadcast_part + ): continue self.removeItem(self.participants_dict[participant]) @@ -272,22 +302,32 @@ def get_selected_messages(self): :rtype: list[SimulatorMessage] """ - return [item.model_item for item in self.selectedItems() if isinstance(item, MessageItem)] - - def select_messages_with_participant(self, participant: ParticipantItem, from_part=True): + return [ + item.model_item + for item in self.selectedItems() + if isinstance(item, MessageItem) + ] + + def select_messages_with_participant( + self, participant: ParticipantItem, from_part=True + ): messages = self.get_all_message_items() self.clearSelection() for msg in messages: - if ((from_part and msg.source is participant) or - (not from_part and msg.destination is participant)): + if (from_part and msg.source is participant) or ( + not from_part and msg.destination is participant + ): msg.select_all() def arrange_participants(self): messages = self.get_all_message_items() for participant in self.participant_items: - if any(msg.source == participant or msg.destination == participant for msg in messages): + if any( + msg.source == participant or msg.destination == participant + for msg in messages + ): participant.setVisible(True) else: participant.setVisible(False) @@ -304,9 +344,20 @@ def arrange_participants(self): curr_participant = vp[i] participants_left = vp[:i] - items = [msg for msg in messages - if ((msg.source == curr_participant and msg.destination in participants_left) - or (msg.source in participants_left and msg.destination == curr_participant))] + items = [ + msg + for msg in messages + if ( + ( + msg.source == curr_participant + and msg.destination in participants_left + ) + or ( + msg.source in participants_left + and msg.destination == curr_participant + ) + ) + ] x_max = vp[i - 1].x_pos() x_max += (vp[i - 1].width() + curr_participant.width()) / 2 @@ -314,7 +365,11 @@ def arrange_participants(self): for msg in items: x = msg.width() + 30 - x += msg.source.x_pos() if msg.source != curr_participant else msg.destination.x_pos() + x += ( + msg.source.x_pos() + if msg.source != curr_participant + else msg.destination.x_pos() + ) if x > x_max: x_max = x @@ -374,7 +429,11 @@ def insert_at(self, ref_item, position, insert_rule=False): return (insert_position, parent_item) def dropEvent(self, event: QDropEvent): - items = [item for item in self.items(event.scenePos()) if isinstance(item, GraphicsItem) and item.acceptDrops()] + items = [ + item + for item in self.items(event.scenePos()) + if isinstance(item, GraphicsItem) and item.acceptDrops() + ] item = None if len(items) == 0 else items[0] if len(event.mimeData().urls()) > 0: self.files_dropped.emit(event.mimeData().urls()) @@ -403,7 +462,9 @@ def dropEvent(self, event: QDropEvent): """:type: list of ProtocolTreeItem """ for group_node in group_nodes: nodes_to_add.extend(group_node.children) - nodes_to_add.extend([file_node for file_node in file_nodes if file_node not in nodes_to_add]) + nodes_to_add.extend( + [file_node for file_node in file_nodes if file_node not in nodes_to_add] + ) protocols_to_add = [node.protocol for node in nodes_to_add] ref_item = item @@ -454,19 +515,38 @@ def add_trigger_command_action(self, ref_item, position): self.simulator_config.add_items([command_action], pos, parent) return command_action - def add_message(self, plain_bits, pause, message_type, ref_item, position, decoder=None, source=None, - destination=None): - message = self.create_message(destination, plain_bits, pause, message_type, decoder, source) + def add_message( + self, + plain_bits, + pause, + message_type, + ref_item, + position, + decoder=None, + source=None, + destination=None, + ): + message = self.create_message( + destination, plain_bits, pause, message_type, decoder, source + ) pos, parent = self.insert_at(ref_item, position, False) self.simulator_config.add_items([message], pos, parent) return message - def create_message(self, destination, plain_bits, pause, message_type, decoder, source): + def create_message( + self, destination, plain_bits, pause, message_type, decoder, source + ): if destination is None: destination = self.simulator_config.broadcast_part - sim_message = SimulatorMessage(destination=destination, plain_bits=plain_bits, pause=pause, - message_type=MessageType(message_type.name), decoder=decoder, source=source) + sim_message = SimulatorMessage( + destination=destination, + plain_bits=plain_bits, + pause=pause, + message_type=MessageType(message_type.name), + decoder=decoder, + source=source, + ) for lbl in message_type: sim_label = SimulatorProtocolLabel(copy.deepcopy(lbl)) @@ -475,7 +555,9 @@ def create_message(self, destination, plain_bits, pause, message_type, decoder, return sim_message def clear_all(self): - self.simulator_config.delete_items([item for item in self.simulator_config.rootItem.children]) + self.simulator_config.delete_items( + [item for item in self.simulator_config.rootItem.children] + ) def add_protocols(self, ref_item, position, protocols_to_add: list): pos, parent = self.insert_at(ref_item, position) @@ -484,12 +566,14 @@ def add_protocols(self, ref_item, position, protocols_to_add: list): for protocol in protocols_to_add: for msg in protocol.messages: source, destination = self.detect_source_destination(msg) - simulator_msg = self.create_message(destination=destination, - plain_bits=copy.copy(msg.decoded_bits), - pause=0, - message_type=msg.message_type, - decoder=msg.decoder, - source=source) + simulator_msg = self.create_message( + destination=destination, + plain_bits=copy.copy(msg.decoded_bits), + pause=0, + message_type=msg.message_type, + decoder=msg.decoder, + source=source, + ) simulator_msg.timestamp = msg.timestamp messages.append(simulator_msg) @@ -517,12 +601,23 @@ def detect_source_destination(self, message: Message): if message.participant: source = message.participant - dst_address_label = next((lbl for lbl in message.message_type if lbl.field_type and - lbl.field_type.function == FieldType.Function.DST_ADDRESS), None) + dst_address_label = next( + ( + lbl + for lbl in message.message_type + if lbl.field_type + and lbl.field_type.function == FieldType.Function.DST_ADDRESS + ), + None, + ) if dst_address_label: - start, end = message.get_label_range(dst_address_label, view=1, decode=True) + start, end = message.get_label_range( + dst_address_label, view=1, decode=True + ) dst_address = message.decoded_hex_str[start:end] - dst = next((p for p in participants if p.address_hex == dst_address), None) + dst = next( + (p for p in participants if p.address_hex == dst_address), None + ) if dst is not None and dst != source: destination = dst diff --git a/src/urh/ui/actions/ChangeSignalParameter.py b/src/urh/ui/actions/ChangeSignalParameter.py index 6223303225..c2361f5d46 100644 --- a/src/urh/ui/actions/ChangeSignalParameter.py +++ b/src/urh/ui/actions/ChangeSignalParameter.py @@ -7,7 +7,13 @@ class ChangeSignalParameter(QUndoCommand): - def __init__(self, signal: Signal, protocol: ProtocolAnalyzer, parameter_name: str, parameter_value): + def __init__( + self, + signal: Signal, + protocol: ProtocolAnalyzer, + parameter_name: str, + parameter_value, + ): super().__init__() if not hasattr(signal, parameter_name): raise ValueError("signal has no attribute {}".format(parameter_name)) @@ -17,20 +23,36 @@ def __init__(self, signal: Signal, protocol: ProtocolAnalyzer, parameter_name: s self.parameter_value = parameter_value self.orig_value = getattr(self.signal, self.parameter_name) - fmt2 = "d" if isinstance(self.orig_value, int) else ".4n" if isinstance(self.orig_value, float) else "s" - fmt3 = "d" if isinstance(parameter_value, int) else ".4n" if isinstance(parameter_value, float) else "s" + fmt2 = ( + "d" + if isinstance(self.orig_value, int) + else ".4n" + if isinstance(self.orig_value, float) + else "s" + ) + fmt3 = ( + "d" + if isinstance(parameter_value, int) + else ".4n" + if isinstance(parameter_value, float) + else "s" + ) signal_name = signal.name[:10] + "..." if len(signal.name) > 10 else signal.name self.setText( - ("change {0} of {1} from {2:" + fmt2 + "} to {3:" + fmt3 + "}") - .format(parameter_name, signal_name, self.orig_value, parameter_value) + ("change {0} of {1} from {2:" + fmt2 + "} to {3:" + fmt3 + "}").format( + parameter_name, signal_name, self.orig_value, parameter_value + ) ) self.protocol = protocol self.orig_messages = copy.deepcopy(self.protocol.messages) def redo(self): - msg_data = [(msg.decoder, msg.participant, msg.message_type) for msg in self.protocol.messages] + msg_data = [ + (msg.decoder, msg.participant, msg.message_type) + for msg in self.protocol.messages + ] setattr(self.signal, self.parameter_name, self.parameter_value) # Restore msg parameters if len(msg_data) == self.protocol.num_messages: diff --git a/src/urh/ui/actions/DeleteBitsAndPauses.py b/src/urh/ui/actions/DeleteBitsAndPauses.py index 4f04fa7e01..ecbe0263c2 100644 --- a/src/urh/ui/actions/DeleteBitsAndPauses.py +++ b/src/urh/ui/actions/DeleteBitsAndPauses.py @@ -6,11 +6,23 @@ class DeleteBitsAndPauses(QUndoCommand): - def __init__(self, proto_analyzer: ProtocolAnalyzer, start_message: int, end_message:int, - start: int, end: int, view: int, decoded: bool, subprotos=None, update_label_ranges=True): + def __init__( + self, + proto_analyzer: ProtocolAnalyzer, + start_message: int, + end_message: int, + start: int, + end: int, + view: int, + decoded: bool, + subprotos=None, + update_label_ranges=True, + ): super().__init__() - self.sub_protocols = [] if subprotos is None else subprotos # type: list[ProtocolAnalyzer] + self.sub_protocols = ( + [] if subprotos is None else subprotos + ) # type: list[ProtocolAnalyzer] self.view = view self.end = end self.start = start @@ -28,20 +40,34 @@ def __init__(self, proto_analyzer: ProtocolAnalyzer, start_message: int, end_mes self.setText("Delete") def redo(self): - self.saved_messages = copy.deepcopy(self.proto_analyzer.messages[self.start_message:self.end_message+1]) - self.removed_message_indices = self.proto_analyzer.delete_messages(self.start_message, self.end_message, - self.start, self.end, - self.view, self.decoded, self.update_label_ranges) + self.saved_messages = copy.deepcopy( + self.proto_analyzer.messages[self.start_message : self.end_message + 1] + ) + self.removed_message_indices = self.proto_analyzer.delete_messages( + self.start_message, + self.end_message, + self.start, + self.end, + self.view, + self.decoded, + self.update_label_ranges, + ) def undo(self): - for i in reversed(range(self.start_message, self.end_message+1)): + for i in reversed(range(self.start_message, self.end_message + 1)): if i in self.removed_message_indices: - self.proto_analyzer.messages.insert(i, self.saved_messages[i-self.start_message]) + self.proto_analyzer.messages.insert( + i, self.saved_messages[i - self.start_message] + ) else: try: - self.proto_analyzer.messages[i] = self.saved_messages[i-self.start_message] + self.proto_analyzer.messages[i] = self.saved_messages[ + i - self.start_message + ] except IndexError: - self.proto_analyzer.messages.append(self.saved_messages[i-self.start_message]) + self.proto_analyzer.messages.append( + self.saved_messages[i - self.start_message] + ) for sub_protocol in self.sub_protocol_history.keys(): sub_protocol.messages = self.sub_protocol_history[sub_protocol] diff --git a/src/urh/ui/actions/EditSignalAction.py b/src/urh/ui/actions/EditSignalAction.py index d161fc8ddf..3e8fe308d0 100644 --- a/src/urh/ui/actions/EditSignalAction.py +++ b/src/urh/ui/actions/EditSignalAction.py @@ -23,10 +23,18 @@ class EditAction(Enum): class EditSignalAction(QUndoCommand): - def __init__(self, signal: Signal, mode: EditAction, - start: int = 0, end: int = 0, position: int = 0, - data_to_insert: np.ndarray=None, dsp_filter: Filter=None, - protocol: ProtocolAnalyzer=None, cache_qad=True): + def __init__( + self, + signal: Signal, + mode: EditAction, + start: int = 0, + end: int = 0, + position: int = 0, + data_to_insert: np.ndarray = None, + dsp_filter: Filter = None, + protocol: ProtocolAnalyzer = None, + cache_qad=True, + ): """ :param signal: Signal to change @@ -55,24 +63,24 @@ def __init__(self, signal: Signal, mode: EditAction, if self.mode == EditAction.crop: self.setText("Crop Signal") - self.pre_crop_data = self.signal.iq_array[0:self.start] - self.post_crop_data = self.signal.iq_array[self.end:] + self.pre_crop_data = self.signal.iq_array[0 : self.start] + self.post_crop_data = self.signal.iq_array[self.end :] if self.cache_qad: - self.pre_crop_qad = self.signal._qad[0:self.start] - self.post_crop_qad = self.signal._qad[self.end:] + self.pre_crop_qad = self.signal._qad[0 : self.start] + self.post_crop_qad = self.signal._qad[self.end :] elif self.mode == EditAction.mute or self.mode == EditAction.filter: if self.mode == EditAction.mute: self.setText("Mute Signal") elif self.mode == EditAction.filter: self.setText("Filter Signal") - self.orig_data_part = copy.copy(self.signal.iq_array[self.start:self.end]) + self.orig_data_part = copy.copy(self.signal.iq_array[self.start : self.end]) if self.cache_qad and self.signal._qad is not None: - self.orig_qad_part = copy.copy(self.signal._qad[self.start:self.end]) + self.orig_qad_part = copy.copy(self.signal._qad[self.start : self.end]) elif self.mode == EditAction.delete: self.setText("Delete Range") - self.orig_data_part = self.signal.iq_array[self.start:self.end] + self.orig_data_part = self.signal.iq_array[self.start : self.end] if self.cache_qad and self.signal._qad is not None: - self.orig_qad_part = self.signal._qad[self.start:self.end] + self.orig_qad_part = self.signal._qad[self.start : self.end] elif self.mode == EditAction.paste: self.setText("Paste") elif self.mode == EditAction.insert: @@ -89,7 +97,9 @@ def redo(self): keep_msg_indices = {} if self.mode in (EditAction.delete, EditAction.mute) and self.protocol: - removed_msg_indices = self.__find_message_indices_in_sample_range(self.start, self.end) + removed_msg_indices = self.__find_message_indices_in_sample_range( + self.start, self.end + ) if removed_msg_indices: for i in range(self.protocol.num_messages): if i < removed_msg_indices[0]: @@ -100,9 +110,13 @@ def redo(self): keep_msg_indices = {i: i for i in range(self.protocol.num_messages)} elif self.mode == EditAction.crop and self.protocol: removed_left = self.__find_message_indices_in_sample_range(0, self.start) - removed_right = self.__find_message_indices_in_sample_range(self.end, self.signal.num_samples) + removed_right = self.__find_message_indices_in_sample_range( + self.end, self.signal.num_samples + ) last_removed_left = removed_left[-1] if removed_left else -1 - first_removed_right = removed_right[0] if removed_right else self.protocol.num_messages + 1 + first_removed_right = ( + removed_right[0] if removed_right else self.protocol.num_messages + 1 + ) for i in range(self.protocol.num_messages): if last_removed_left < i < first_removed_right: @@ -141,33 +155,41 @@ def undo(self): self.signal.iq_array.insert_subarray(self.start, self.orig_data_part) if self.cache_qad and self.orig_qad_part is not None: try: - self.signal._qad = np.insert(self.signal._qad, self.start, self.orig_qad_part) + self.signal._qad = np.insert( + self.signal._qad, self.start, self.orig_qad_part + ) except ValueError: self.signal._qad = None logger.warning("Could not restore cached qad.") elif self.mode == EditAction.mute or self.mode == EditAction.filter: - self.signal.iq_array[self.start:self.end] = self.orig_data_part + self.signal.iq_array[self.start : self.end] = self.orig_data_part if self.cache_qad and self.orig_qad_part is not None: try: - self.signal._qad[self.start:self.end] = self.orig_qad_part + self.signal._qad[self.start : self.end] = self.orig_qad_part except (ValueError, TypeError): self.signal._qad = None logger.warning("Could not restore cached qad.") elif self.mode == EditAction.crop: self.signal.iq_array = IQArray( - np.concatenate((self.pre_crop_data, self.signal.iq_array.data, self.post_crop_data)) + np.concatenate( + (self.pre_crop_data, self.signal.iq_array.data, self.post_crop_data) + ) ) if self.cache_qad: try: - self.signal._qad = np.concatenate((self.pre_crop_qad, self.signal._qad, self.post_crop_qad)) + self.signal._qad = np.concatenate( + (self.pre_crop_qad, self.signal._qad, self.post_crop_qad) + ) except ValueError: self.signal._qad = None logger.warning("Could not restore cached qad.") elif self.mode == EditAction.paste or self.mode == EditAction.insert: - self.signal.delete_range(self.position, self.position+len(self.data_to_insert)) + self.signal.delete_range( + self.position, self.position + len(self.data_to_insert) + ) self.signal.parameter_cache = self.orig_parameter_cache @@ -191,13 +213,16 @@ def __get_keep_msg_indices_for_paste(self): keep_msg_indices = {i: i for i in range(len(self.orig_messages))} try: - paste_start_index = self.__find_message_indices_in_sample_range(self.position, self.signal.num_samples)[0] + paste_start_index = self.__find_message_indices_in_sample_range( + self.position, self.signal.num_samples + )[0] except IndexError: paste_start_index = 0 try: - paste_end_index = self.__find_message_indices_in_sample_range(self.position + len(self.data_to_insert), - self.signal.num_samples)[0] + paste_end_index = self.__find_message_indices_in_sample_range( + self.position + len(self.data_to_insert), self.signal.num_samples + )[0] except IndexError: paste_end_index = 0 diff --git a/src/urh/ui/actions/Fuzz.py b/src/urh/ui/actions/Fuzz.py index d88b402c7c..2127017a08 100644 --- a/src/urh/ui/actions/Fuzz.py +++ b/src/urh/ui/actions/Fuzz.py @@ -5,7 +5,9 @@ class Fuzz(QUndoCommand): - def __init__(self, proto_analyzer_container: ProtocolAnalyzerContainer, fuz_mode: str): + def __init__( + self, proto_analyzer_container: ProtocolAnalyzerContainer, fuz_mode: str + ): super().__init__() self.proto_analyzer_container = proto_analyzer_container self.fuz_mode = fuz_mode @@ -14,17 +16,23 @@ def __init__(self, proto_analyzer_container: ProtocolAnalyzerContainer, fuz_mode self.added_message_indices = [] def redo(self): - if settings.read('use_default_fuzzing_pause', True, bool): + if settings.read("use_default_fuzzing_pause", True, bool): default_pause = settings.read("default_fuzzing_pause", 10**6, int) else: default_pause = None if self.fuz_mode == "Successive": - added_indices = self.proto_analyzer_container.fuzz_successive(default_pause=default_pause) + added_indices = self.proto_analyzer_container.fuzz_successive( + default_pause=default_pause + ) elif self.fuz_mode == "Concurrent": - added_indices = self.proto_analyzer_container.fuzz_concurrent(default_pause=default_pause) + added_indices = self.proto_analyzer_container.fuzz_concurrent( + default_pause=default_pause + ) elif self.fuz_mode == "Exhaustive": - added_indices = self.proto_analyzer_container.fuzz_exhaustive(default_pause=default_pause) + added_indices = self.proto_analyzer_container.fuzz_exhaustive( + default_pause=default_pause + ) else: added_indices = [] diff --git a/src/urh/ui/actions/InsertBitsAndPauses.py b/src/urh/ui/actions/InsertBitsAndPauses.py index bafa06fc64..9001ce5602 100644 --- a/src/urh/ui/actions/InsertBitsAndPauses.py +++ b/src/urh/ui/actions/InsertBitsAndPauses.py @@ -7,7 +7,12 @@ class InsertBitsAndPauses(QUndoCommand): - def __init__(self, proto_analyzer_container: ProtocolAnalyzerContainer, index: int, pa: ProtocolAnalyzer): + def __init__( + self, + proto_analyzer_container: ProtocolAnalyzerContainer, + index: int, + pa: ProtocolAnalyzer, + ): super().__init__() self.proto_analyzer_container = proto_analyzer_container self.proto_analyzer = pa @@ -19,10 +24,12 @@ def __init__(self, proto_analyzer_container: ProtocolAnalyzerContainer, index: i self.num_messages = 0 def redo(self): - self.proto_analyzer_container.insert_protocol_analyzer(self.index, self.proto_analyzer) + self.proto_analyzer_container.insert_protocol_analyzer( + self.index, self.proto_analyzer + ) self.num_messages += len(self.proto_analyzer.messages) def undo(self): - for i in reversed(range(self.index, self.index+self.num_messages)): + for i in reversed(range(self.index, self.index + self.num_messages)): del self.proto_analyzer_container.messages[i] self.num_messages = 0 diff --git a/src/urh/ui/actions/InsertColumn.py b/src/urh/ui/actions/InsertColumn.py index c7865438c0..78b99baf2b 100644 --- a/src/urh/ui/actions/InsertColumn.py +++ b/src/urh/ui/actions/InsertColumn.py @@ -6,10 +6,14 @@ class InsertColumn(QUndoCommand): - def __init__(self, proto_analyzer: ProtocolAnalyzer, index: int, rows: list, view: int): + def __init__( + self, proto_analyzer: ProtocolAnalyzer, index: int, rows: list, view: int + ): super().__init__() self.proto_analyzer = proto_analyzer - self.index = proto_analyzer.convert_index(index, from_view=view, to_view=0, decoded=False)[0] + self.index = proto_analyzer.convert_index( + index, from_view=view, to_view=0, decoded=False + )[0] self.nbits = 1 if view == 0 else 4 if view == 1 else 8 self.rows = rows diff --git a/src/urh/ui/actions/__init__.py b/src/urh/ui/actions/__init__.py index 3fa5af38d8..813939aaf7 100644 --- a/src/urh/ui/actions/__init__.py +++ b/src/urh/ui/actions/__init__.py @@ -1 +1 @@ -__author__ = 'joe' +__author__ = "joe" diff --git a/src/urh/ui/delegates/CheckBoxDelegate.py b/src/urh/ui/delegates/CheckBoxDelegate.py index f6cbfeb7b8..3547a58073 100644 --- a/src/urh/ui/delegates/CheckBoxDelegate.py +++ b/src/urh/ui/delegates/CheckBoxDelegate.py @@ -1,5 +1,10 @@ from PyQt5.QtCore import QModelIndex, QAbstractItemModel, Qt, pyqtSlot -from PyQt5.QtWidgets import QStyledItemDelegate, QWidget, QStyleOptionViewItem, QCheckBox +from PyQt5.QtWidgets import ( + QStyledItemDelegate, + QWidget, + QStyleOptionViewItem, + QCheckBox, +) class CheckBoxDelegate(QStyledItemDelegate): @@ -7,7 +12,9 @@ def __init__(self, parent=None): super().__init__(parent) self.enabled = True - def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def createEditor( + self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): editor = QCheckBox(parent) editor.stateChanged.connect(self.stateChanged) return editor @@ -18,9 +25,11 @@ def setEditorData(self, editor: QCheckBox, index: QModelIndex): self.enabled = editor.isChecked() editor.blockSignals(False) - def setModelData(self, editor: QCheckBox, model: QAbstractItemModel, index: QModelIndex): + def setModelData( + self, editor: QCheckBox, model: QAbstractItemModel, index: QModelIndex + ): model.setData(index, editor.isChecked(), Qt.EditRole) @pyqtSlot() def stateChanged(self): - self.commitData.emit(self.sender()) \ No newline at end of file + self.commitData.emit(self.sender()) diff --git a/src/urh/ui/delegates/ComboBoxDelegate.py b/src/urh/ui/delegates/ComboBoxDelegate.py index 2f947e65e4..83cf0c2c8a 100644 --- a/src/urh/ui/delegates/ComboBoxDelegate.py +++ b/src/urh/ui/delegates/ComboBoxDelegate.py @@ -2,11 +2,18 @@ from PyQt5.QtCore import QModelIndex, Qt, QAbstractItemModel, pyqtSlot, QRectF from PyQt5.QtGui import QImage, QPainter, QColor, QPixmap -from PyQt5.QtWidgets import QStyledItemDelegate, QWidget, QStyleOptionViewItem, QComboBox +from PyQt5.QtWidgets import ( + QStyledItemDelegate, + QWidget, + QStyleOptionViewItem, + QComboBox, +) class ComboBoxDelegate(QStyledItemDelegate): - def __init__(self, items, colors=None, is_editable=False, return_index=True, parent=None): + def __init__( + self, items, colors=None, is_editable=False, return_index=True, parent=None + ): """ :param items: @@ -25,7 +32,9 @@ def __init__(self, items, colors=None, is_editable=False, return_index=True, par if colors: assert len(items) == len(colors) - def paint(self, painter: QPainter, option: QStyleOptionViewItem, index: QModelIndex): + def paint( + self, painter: QPainter, option: QStyleOptionViewItem, index: QModelIndex + ): if self.colors: try: item = index.model().data(index) @@ -37,13 +46,17 @@ def paint(self, painter: QPainter, option: QStyleOptionViewItem, index: QModelIn rect = QRectF(x + 8, y + h / 2 - 8, 16, 16) painter.fillRect(rect, QColor("black")) rect = rect.adjusted(1, 1, -1, -1) - painter.fillRect(rect, QColor(color.red(), color.green(), color.blue(), 255)) + painter.fillRect( + rect, QColor(color.red(), color.green(), color.blue(), 255) + ) except: super().paint(painter, option, index) else: super().paint(painter, option, index) - def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def createEditor( + self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): editor = QComboBox(parent) if sys.platform == "win32": # Ensure text entries are visible with windows combo boxes @@ -66,7 +79,9 @@ def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QMo rect = img.rect().adjusted(1, 1, -1, -1) for i, item in enumerate(self.items): color = self.colors[i] - painter.fillRect(rect, QColor(color.red(), color.green(), color.blue(), 255)) + painter.fillRect( + rect, QColor(color.red(), color.green(), color.blue(), 255) + ) editor.setItemData(i, QPixmap.fromImage(img), Qt.DecorationRole) del painter @@ -84,13 +99,17 @@ def setEditorData(self, editor: QWidget, index: QModelIndex): pass editor.blockSignals(False) - def setModelData(self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex): + def setModelData( + self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex + ): if self.return_index: model.setData(index, editor.currentIndex(), Qt.EditRole) else: model.setData(index, editor.currentText(), Qt.EditRole) - def updateEditorGeometry(self, editor: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def updateEditorGeometry( + self, editor: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): editor.setGeometry(option.rect) @pyqtSlot() diff --git a/src/urh/ui/delegates/MessageTypeButtonDelegate.py b/src/urh/ui/delegates/MessageTypeButtonDelegate.py index 5eaa3a751a..e5c23c02ff 100644 --- a/src/urh/ui/delegates/MessageTypeButtonDelegate.py +++ b/src/urh/ui/delegates/MessageTypeButtonDelegate.py @@ -17,7 +17,9 @@ def createEditor(self, parent, option, index): button = QPushButton(parent) button.setFlat(True) - num_rules = self.parent().model().get_num_active_rules_of_message_type_at(index.row()) + num_rules = ( + self.parent().model().get_num_active_rules_of_message_type_at(index.row()) + ) if num_rules == 0: icon = QIcon.fromTheme("configure") @@ -51,7 +53,9 @@ def draw_indicator(indicator: int): fw = f.width(indicator_str) fh = f.height() - painter.drawText(math.ceil(w / 2 - fw / 2), math.ceil(h / 2 + fh / 4), indicator_str) + painter.drawText( + math.ceil(w / 2 - fw / 2), math.ceil(h / 2 + fh / 4), indicator_str + ) painter.end() return QIcon(pixmap) diff --git a/src/urh/ui/delegates/ProtocolValueDelegate.py b/src/urh/ui/delegates/ProtocolValueDelegate.py index 00b70cb1b8..e453dc346c 100644 --- a/src/urh/ui/delegates/ProtocolValueDelegate.py +++ b/src/urh/ui/delegates/ProtocolValueDelegate.py @@ -1,6 +1,15 @@ from PyQt5.QtCore import QModelIndex, QAbstractItemModel, Qt -from PyQt5.QtWidgets import QStyledItemDelegate, QWidget, QStyleOptionViewItem, QLineEdit, QHBoxLayout, \ - QCompleter, QLabel, QSpinBox, QDirModel +from PyQt5.QtWidgets import ( + QStyledItemDelegate, + QWidget, + QStyleOptionViewItem, + QLineEdit, + QHBoxLayout, + QCompleter, + QLabel, + QSpinBox, + QDirModel, +) from urh.ui.ExpressionLineEdit import ExpressionLineEdit from urh.ui.RuleExpressionValidator import RuleExpressionValidator @@ -14,7 +23,9 @@ def __init__(self, parent=None): completer.setModel(QDirModel(completer)) self.line_edit_external_program = QLineEdit() self.line_edit_external_program.setCompleter(completer) - self.line_edit_external_program.setPlaceholderText("Type in a path to external program.") + self.line_edit_external_program.setPlaceholderText( + "Type in a path to external program." + ) self.layout = QHBoxLayout() self.layout.setContentsMargins(0, 0, 0, 0) @@ -56,7 +67,9 @@ def __init__(self, controller, parent=None): super().__init__(parent) self.controller = controller - def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def createEditor( + self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): model = index.model() row = index.row() lbl = model.message_type[row] @@ -64,8 +77,12 @@ def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QMo if lbl.value_type_index == 2: line_edit = ExpressionLineEdit(parent) line_edit.setPlaceholderText("(item1.length + 3) ^ 0x12") - line_edit.setCompleter(QCompleter(self.controller.completer_model, line_edit)) - line_edit.setValidator(RuleExpressionValidator(self.controller.sim_expression_parser)) + line_edit.setCompleter( + QCompleter(self.controller.completer_model, line_edit) + ) + line_edit.setValidator( + RuleExpressionValidator(self.controller.sim_expression_parser) + ) line_edit.setToolTip(self.controller.sim_expression_parser.formula_help) return line_edit elif lbl.value_type_index == 3: @@ -91,10 +108,16 @@ def setEditorData(self, editor: QWidget, index: QModelIndex): else: super().setEditorData(editor, index) - def setModelData(self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex): + def setModelData( + self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex + ): if isinstance(editor, ExternalProgramWidget): model.setData(index, editor.line_edit_external_program.text(), Qt.EditRole) elif isinstance(editor, RandomValueWidget): - model.setData(index, [editor.spinbox_random_min.value(), editor.spinbox_random_max.value()], Qt.EditRole) + model.setData( + index, + [editor.spinbox_random_min.value(), editor.spinbox_random_max.value()], + Qt.EditRole, + ) else: super().setModelData(editor, model, index) diff --git a/src/urh/ui/delegates/SectionComboBoxDelegate.py b/src/urh/ui/delegates/SectionComboBoxDelegate.py index 7e1fe8c8bc..1b658e40c3 100644 --- a/src/urh/ui/delegates/SectionComboBoxDelegate.py +++ b/src/urh/ui/delegates/SectionComboBoxDelegate.py @@ -3,14 +3,23 @@ from PyQt5.QtCore import QModelIndex, pyqtSlot, QAbstractItemModel, Qt from PyQt5.QtGui import QPainter, QStandardItem -from PyQt5.QtWidgets import QItemDelegate, QStyleOptionViewItem, QStyle, QComboBox, QStyledItemDelegate, QWidget +from PyQt5.QtWidgets import ( + QItemDelegate, + QStyleOptionViewItem, + QStyle, + QComboBox, + QStyledItemDelegate, + QWidget, +) class SectionItemDelegate(QItemDelegate): def __init__(self, parent=None): super().__init__(parent) - def paint(self, painter: QPainter, option: QStyleOptionViewItem, index: QModelIndex): + def paint( + self, painter: QPainter, option: QStyleOptionViewItem, index: QModelIndex + ): item_type = index.data(Qt.AccessibleDescriptionRole) if item_type == "parent": parent_option = option @@ -57,7 +66,9 @@ def __init__(self, items: OrderedDict, parent=None): super().__init__(parent) self.items = items - def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def createEditor( + self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): editor = SectionComboBox(parent) editor.setItemDelegate(SectionItemDelegate(editor.itemDelegate().parent())) if sys.platform == "win32": @@ -77,10 +88,14 @@ def setEditorData(self, editor: SectionComboBox, index: QModelIndex): editor.setCurrentText(item) editor.blockSignals(False) - def setModelData(self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex): + def setModelData( + self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex + ): model.setData(index, editor.currentText(), Qt.EditRole) - def updateEditorGeometry(self, editor: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def updateEditorGeometry( + self, editor: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): editor.setGeometry(option.rect) @pyqtSlot() diff --git a/src/urh/ui/delegates/SpinBoxDelegate.py b/src/urh/ui/delegates/SpinBoxDelegate.py index 1bb7437baa..8490cd5062 100644 --- a/src/urh/ui/delegates/SpinBoxDelegate.py +++ b/src/urh/ui/delegates/SpinBoxDelegate.py @@ -12,7 +12,9 @@ def __init__(self, minimum, maximum, parent=None, suffix=""): def _get_editor(self, parent) -> QSpinBox: return QSpinBox(parent) - def createEditor(self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex): + def createEditor( + self, parent: QWidget, option: QStyleOptionViewItem, index: QModelIndex + ): editor = self._get_editor(parent) editor.setMinimum(self.minimum) editor.setMaximum(self.maximum) @@ -25,10 +27,12 @@ def setEditorData(self, editor: QWidget, index: QModelIndex): try: editor.setValue(int(index.model().data(index))) except ValueError: - pass # If Label was deleted and UI not updated yet + pass # If Label was deleted and UI not updated yet editor.blockSignals(False) - def setModelData(self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex): + def setModelData( + self, editor: QWidget, model: QAbstractItemModel, index: QModelIndex + ): model.setData(index, editor.value(), Qt.EditRole) @pyqtSlot() diff --git a/src/urh/ui/delegates/__init__.py b/src/urh/ui/delegates/__init__.py index 3fa5af38d8..813939aaf7 100644 --- a/src/urh/ui/delegates/__init__.py +++ b/src/urh/ui/delegates/__init__.py @@ -1 +1 @@ -__author__ = 'joe' +__author__ = "joe" diff --git a/src/urh/ui/painting/FFTSceneManager.py b/src/urh/ui/painting/FFTSceneManager.py index 53831d7864..125c69f518 100644 --- a/src/urh/ui/painting/FFTSceneManager.py +++ b/src/urh/ui/painting/FFTSceneManager.py @@ -16,9 +16,13 @@ def __init__(self, parent, graphic_view=None): self.scene = GridScene(parent=graphic_view) self.scene.setBackgroundBrush(settings.BGCOLOR) - self.peak_item = self.scene.addPath(QPainterPath(), QPen(settings.PEAK_COLOR, 0)) # type: QGraphicsPathItem + self.peak_item = self.scene.addPath( + QPainterPath(), QPen(settings.PEAK_COLOR, 0) + ) # type: QGraphicsPathItem - def show_scene_section(self, x1: float, x2: float, subpath_ranges=None, colors=None): + def show_scene_section( + self, x1: float, x2: float, subpath_ranges=None, colors=None + ): start = int(x1) if x1 > 0 else 0 end = int(x2) if x2 < self.num_samples else self.num_samples paths = path_creator.create_path(np.log10(self.plot_data), start, end) @@ -34,7 +38,11 @@ def show_scene_section(self, x1: float, x2: float, subpath_ranges=None, colors=N def init_scene(self, draw_grid=True): self.scene.draw_grid = draw_grid - self.peak = self.plot_data if len(self.peak) < self.num_samples else np.maximum(self.peak, self.plot_data) + self.peak = ( + self.plot_data + if len(self.peak) < self.num_samples + else np.maximum(self.peak, self.plot_data) + ) self.scene.setSceneRect(0, -5, self.num_samples, 10) def clear_path(self): diff --git a/src/urh/ui/painting/GridScene.py b/src/urh/ui/painting/GridScene.py index 17a8268117..9b4d678b79 100644 --- a/src/urh/ui/painting/GridScene.py +++ b/src/urh/ui/painting/GridScene.py @@ -21,10 +21,18 @@ def __init__(self, parent=None): def drawBackground(self, painter: QPainter, rect: QRectF): if self.draw_grid and len(self.frequencies) > 0: painter.setPen(QPen(painter.pen().color(), 0)) - parent_width = self.parent().width() if hasattr(self.parent(), "width") else 750 - view_rect = self.parent().view_rect() if hasattr(self.parent(), "view_rect") else rect - - font_width = self.font_metrics.width(Formatter.big_value_with_suffix(self.center_freq) + " ") + parent_width = ( + self.parent().width() if hasattr(self.parent(), "width") else 750 + ) + view_rect = ( + self.parent().view_rect() + if hasattr(self.parent(), "view_rect") + else rect + ) + + font_width = self.font_metrics.width( + Formatter.big_value_with_suffix(self.center_freq) + " " + ) x_grid_size = int(view_rect.width() / parent_width * font_width) # x_grid_size = int(0.1 * view_rect.width()) if 0.1 * view_rect.width() > 1 else 1 y_grid_size = 1 @@ -36,14 +44,24 @@ def drawBackground(self, painter: QPainter, rect: QRectF): top = rect.top() - (rect.top() % y_grid_size) bottom = rect.bottom() - (rect.bottom() % y_grid_size) - right_border = int(rect.right()) if rect.right() < len(self.frequencies) else len(self.frequencies) + right_border = ( + int(rect.right()) + if rect.right() < len(self.frequencies) + else len(self.frequencies) + ) scale_x, scale_y = util.calc_x_y_scale(rect, self.parent()) fh = self.font_metrics.height() - x_range = list(range(x_mid, left, -x_grid_size)) + list(range(x_mid, right_border, x_grid_size)) - lines = [QLineF(x, rect.top(), x, bottom-fh*scale_y) for x in x_range] \ - + [QLineF(rect.left(), y, rect.right(), y) for y in np.arange(top, bottom, y_grid_size)] + x_range = list(range(x_mid, left, -x_grid_size)) + list( + range(x_mid, right_border, x_grid_size) + ) + lines = [ + QLineF(x, rect.top(), x, bottom - fh * scale_y) for x in x_range + ] + [ + QLineF(rect.left(), y, rect.right(), y) + for y in np.arange(top, bottom, y_grid_size) + ] pen = painter.pen() pen.setStyle(Qt.DotLine) @@ -63,7 +81,9 @@ def drawBackground(self, painter: QPainter, rect: QRectF): value = Formatter.big_value_with_suffix(self.center_freq + freq, 2) font_width = self.font_metrics.width(value) - painter.drawText(QPointF(x / scale_x - font_width / 2, bottom / scale_y), value) + painter.drawText( + QPointF(x / scale_x - font_width / 2, bottom / scale_y), value + ) def draw_frequency_marker(self, x_pos, frequency): if frequency is None: @@ -86,12 +106,16 @@ def draw_frequency_marker(self, x_pos, frequency): self.frequency_marker[0].setLine(x_pos, y1, x_pos, y2) scale_x, scale_y = util.calc_x_y_scale(self.sceneRect(), self.parent()) - self.frequency_marker[1].setTransform(QTransform.fromScale(scale_x, scale_y), False) - self.frequency_marker[1].setText("Tune to " + Formatter.big_value_with_suffix(frequency, decimals=3)) + self.frequency_marker[1].setTransform( + QTransform.fromScale(scale_x, scale_y), False + ) + self.frequency_marker[1].setText( + "Tune to " + Formatter.big_value_with_suffix(frequency, decimals=3) + ) font_metric = QFontMetrics(self.frequency_marker[1].font()) text_width = font_metric.width("Tune to") * scale_x text_width += (font_metric.width(" ") * scale_x) / 2 - self.frequency_marker[1].setPos(x_pos-text_width, 0.95*y1) + self.frequency_marker[1].setPos(x_pos - text_width, 0.95 * y1) def clear_frequency_marker(self): if self.frequency_marker is not None: @@ -99,7 +123,7 @@ def clear_frequency_marker(self): self.removeItem(self.frequency_marker[1]) self.frequency_marker = None - def get_freq_for_pos(self, x: int) -> float: + def get_freq_for_pos(self, x: int) -> float: try: f = self.frequencies[x] except IndexError: diff --git a/src/urh/ui/painting/LabeledArrow.py b/src/urh/ui/painting/LabeledArrow.py index 79de64160a..68e8b3173c 100644 --- a/src/urh/ui/painting/LabeledArrow.py +++ b/src/urh/ui/painting/LabeledArrow.py @@ -17,11 +17,19 @@ def __init__(self, x1, y1, x2, y2, label): def boundingRect(self): extra = (self.pen().width() + 20) / 2.0 try: - return QRectF(self.line().p1(), QSizeF(self.line().p2().x() - self.line().p1().x(), - self.line().p2().y() - self.line().p1().y())) \ - .normalized().adjusted(-extra, -extra, extra, extra) + return ( + QRectF( + self.line().p1(), + QSizeF( + self.line().p2().x() - self.line().p1().x(), + self.line().p2().y() - self.line().p1().y(), + ), + ) + .normalized() + .adjusted(-extra, -extra, extra, extra) + ) except RuntimeError: - return QRectF(0,0,0,0) + return QRectF(0, 0, 0, 0) def paint(self, painter, QStyleOptionGraphicsItem, QWidget_widget=None): """ @@ -41,12 +49,20 @@ def paint(self, painter, QStyleOptionGraphicsItem, QWidget_widget=None): labelheight = 0.75 * abs(y2 - y1) painter.drawLine(QPointF(x1, y1), QPointF(x1, y1 + labelheight / 2)) - painter.drawLine(QPointF(x1, y1), QPointF(x1 + x_arrowSize / 4, y1 + y_arrowSize / 2)) - painter.drawLine(QPointF(x1, y1), QPointF(x1 - x_arrowSize / 4, y1 + y_arrowSize / 2)) + painter.drawLine( + QPointF(x1, y1), QPointF(x1 + x_arrowSize / 4, y1 + y_arrowSize / 2) + ) + painter.drawLine( + QPointF(x1, y1), QPointF(x1 - x_arrowSize / 4, y1 + y_arrowSize / 2) + ) painter.drawLine(QPointF(x1, y2 - labelheight / 2), QPointF(x1, y2)) - painter.drawLine(QPointF(x1, y2), QPointF(x1 + x_arrowSize / 4, y2 - y_arrowSize / 2)) - painter.drawLine(QPointF(x1, y2), QPointF(x1 - x_arrowSize / 4, y2 - y_arrowSize / 2)) + painter.drawLine( + QPointF(x1, y2), QPointF(x1 + x_arrowSize / 4, y2 - y_arrowSize / 2) + ) + painter.drawLine( + QPointF(x1, y2), QPointF(x1 - x_arrowSize / 4, y2 - y_arrowSize / 2) + ) painter.setRenderHint(QPainter.HighQualityAntialiasing) fm = painter.fontMetrics() @@ -56,11 +72,14 @@ def paint(self, painter, QStyleOptionGraphicsItem, QWidget_widget=None): scale_factor = scale_factor if scale_factor > 0 else 0.0000000000000000001 painter.scale(1, scale_factor) - - # print(y1, y2, pixelsHigh) - painter.drawText(QPointF(x1 - pixelsWide / 2, (1 / scale_factor) * (y1 + y2) / 2 + pixelsHigh / 4), self.label) + painter.drawText( + QPointF( + x1 - pixelsWide / 2, (1 / scale_factor) * (y1 + y2) / 2 + pixelsHigh / 4 + ), + self.label, + ) # painter.drawText(QPointF(x1 - pixelsWide/2, (y1+y2+pixelsHigh)/2), self.label) del painter diff --git a/src/urh/ui/painting/SceneManager.py b/src/urh/ui/painting/SceneManager.py index 2f9c931878..3449574ced 100644 --- a/src/urh/ui/painting/SceneManager.py +++ b/src/urh/ui/painting/SceneManager.py @@ -32,7 +32,9 @@ def num_samples(self): return len(self.plot_data[0]) return len(self.plot_data) - def show_scene_section(self, x1: float, x2: float, subpath_ranges=None, colors=None): + def show_scene_section( + self, x1: float, x2: float, subpath_ranges=None, colors=None + ): """ :param x1: start of section to show :param x2: end of section to show @@ -46,15 +48,28 @@ def show_scene_section(self, x1: float, x2: float, subpath_ranges=None, colors=N if end > start: if isinstance(self.plot_data, list): - paths_i = path_creator.create_path(self.plot_data[0], start=start, end=end, - subpath_ranges=subpath_ranges) - paths_q = path_creator.create_path(self.plot_data[1], start=start, end=end, - subpath_ranges=subpath_ranges) + paths_i = path_creator.create_path( + self.plot_data[0], + start=start, + end=end, + subpath_ranges=subpath_ranges, + ) + paths_q = path_creator.create_path( + self.plot_data[1], + start=start, + end=end, + subpath_ranges=subpath_ranges, + ) self.set_path(paths_i, colors=[settings.LINECOLOR_I] * len(paths_i)) - self.set_path(paths_q, colors=[settings.LINECOLOR_Q] * len(paths_q), run_clear_path=False) + self.set_path( + paths_q, + colors=[settings.LINECOLOR_Q] * len(paths_q), + run_clear_path=False, + ) else: - paths = path_creator.create_path(self.plot_data, start=start, end=end, - subpath_ranges=subpath_ranges) + paths = path_creator.create_path( + self.plot_data, start=start, end=end, subpath_ranges=subpath_ranges + ) self.set_path(paths, colors=colors) def set_path(self, paths: list, colors=None, run_clear_path=True): @@ -63,12 +78,16 @@ def set_path(self, paths: list, colors=None, run_clear_path=True): colors = [settings.LINECOLOR] * len(paths) if colors is None else colors assert len(paths) == len(colors) for path, color in zip(paths, colors): - path_object = self.scene.addPath(path, QPen(color if color else settings.LINECOLOR, 0)) + path_object = self.scene.addPath( + path, QPen(color if color else settings.LINECOLOR, 0) + ) if color: path_object.setZValue(1) def __limit_value(self, val: float) -> int: - return 0 if val < 0 else self.num_samples if val > self.num_samples else int(val) + return ( + 0 if val < 0 else self.num_samples if val > self.num_samples else int(val) + ) def show_full_scene(self): self.show_scene_section(0, self.num_samples) diff --git a/src/urh/ui/painting/Selection.py b/src/urh/ui/painting/Selection.py index de6a92348b..66658d06ee 100644 --- a/src/urh/ui/painting/Selection.py +++ b/src/urh/ui/painting/Selection.py @@ -91,7 +91,9 @@ def end(self): def end(self, value): raise NotImplementedError("Overwrite in subclass") - def _get_selected_edge(self, pos: QPointF, transform: QTransform, horizontal_selection: bool): + def _get_selected_edge( + self, pos: QPointF, transform: QTransform, horizontal_selection: bool + ): x1, x2 = self.x, self.x + self.width y1, y2 = self.y, self.y + self.height x, y = pos.x(), pos.y() diff --git a/src/urh/ui/painting/SignalSceneManager.py b/src/urh/ui/painting/SignalSceneManager.py index d0a312a458..ec9c453144 100644 --- a/src/urh/ui/painting/SignalSceneManager.py +++ b/src/urh/ui/painting/SignalSceneManager.py @@ -8,10 +8,14 @@ class SignalSceneManager(SceneManager): def __init__(self, signal: Signal, parent): super().__init__(parent) self.signal = signal - self.scene_type = 0 # 0 = Analog Signal, 1 = QuadDemodView, 2 = Spectogram, 3 = I/Q view + self.scene_type = ( + 0 # 0 = Analog Signal, 1 = QuadDemodView, 2 = Spectogram, 3 = I/Q view + ) self.mod_type = "ASK" - def show_scene_section(self, x1: float, x2: float, subpath_ranges=None, colors=None): + def show_scene_section( + self, x1: float, x2: float, subpath_ranges=None, colors=None + ): if self.scene_type == 0: self.plot_data = self.signal.real_plot_data elif self.scene_type == 3: @@ -36,7 +40,10 @@ def init_scene(self): self.line_item.setLine(0, 0, 0, 0) # Hide Axis if self.scene_type == 0 or self.scene_type == 3: - self.scene.draw_noise_area(self.signal.noise_min_plot, self.signal.noise_max_plot - self.signal.noise_min_plot) + self.scene.draw_noise_area( + self.signal.noise_min_plot, + self.signal.noise_max_plot - self.signal.noise_min_plot, + ) else: self.scene.draw_sep_area(-self.signal.center_thresholds) diff --git a/src/urh/ui/painting/SniffSceneManager.py b/src/urh/ui/painting/SniffSceneManager.py index 6edee5a853..3bb921c7fd 100644 --- a/src/urh/ui/painting/SniffSceneManager.py +++ b/src/urh/ui/painting/SniffSceneManager.py @@ -12,7 +12,7 @@ def __init__(self, data_array, parent, window_length=5 * 10**6): @property def plot_data(self): - return self.data_array[self.__start:self.end] + return self.data_array[self.__start : self.end] @plot_data.setter def plot_data(self, value): diff --git a/src/urh/ui/painting/SpectrogramScene.py b/src/urh/ui/painting/SpectrogramScene.py index 721b622d52..6692f69523 100644 --- a/src/urh/ui/painting/SpectrogramScene.py +++ b/src/urh/ui/painting/SpectrogramScene.py @@ -8,7 +8,9 @@ def __init__(self, parent=None): super().__init__(parent) self.removeItem(self.selection_area) - self.selection_area = VerticalSelection(0, 0, 0, 0, fillcolor=settings.SELECTION_COLOR, opacity=0.6) + self.selection_area = VerticalSelection( + 0, 0, 0, 0, fillcolor=settings.SELECTION_COLOR, opacity=0.6 + ) self.selection_area.setZValue(1) self.addItem(self.selection_area) diff --git a/src/urh/ui/painting/SpectrogramSceneManager.py b/src/urh/ui/painting/SpectrogramSceneManager.py index ff477d2412..bf6c63ddbf 100644 --- a/src/urh/ui/painting/SpectrogramSceneManager.py +++ b/src/urh/ui/painting/SpectrogramSceneManager.py @@ -21,7 +21,9 @@ def __init__(self, samples, parent): def num_samples(self): return len(self.spectrogram.samples) - def set_parameters(self, samples: np.ndarray, window_size, data_min, data_max) -> bool: + def set_parameters( + self, samples: np.ndarray, window_size, data_min, data_max + ) -> bool: """ Return true if redraw is needed """ @@ -45,11 +47,15 @@ def set_parameters(self, samples: np.ndarray, window_size, data_min, data_max) - return redraw_needed - def show_scene_section(self, x1: float, x2: float, subpath_ranges=None, colors=None): + def show_scene_section( + self, x1: float, x2: float, subpath_ranges=None, colors=None + ): pass def update_scene_rect(self): - self.scene.setSceneRect(0, 0, self.spectrogram.time_bins, self.spectrogram.freq_bins) + self.scene.setSceneRect( + 0, 0, self.spectrogram.time_bins, self.spectrogram.freq_bins + ) def show_full_scene(self): for item in self.scene.items(): diff --git a/src/urh/ui/painting/ZoomableScene.py b/src/urh/ui/painting/ZoomableScene.py index 3dbbe39116..b398f9b3e7 100644 --- a/src/urh/ui/painting/ZoomableScene.py +++ b/src/urh/ui/painting/ZoomableScene.py @@ -3,7 +3,12 @@ import numpy as np from PyQt5.QtCore import QRectF from PyQt5.QtGui import QPen, QFont, QTransform, QFontMetrics -from PyQt5.QtWidgets import QGraphicsScene, QGraphicsRectItem, QGraphicsSceneDragDropEvent, QGraphicsSimpleTextItem +from PyQt5.QtWidgets import ( + QGraphicsScene, + QGraphicsRectItem, + QGraphicsSceneDragDropEvent, + QGraphicsSimpleTextItem, +) from urh import settings from urh.ui.painting.HorizontalSelection import HorizontalSelection @@ -27,8 +32,14 @@ def __init__(self, parent=None): self.ones_arrow = None self.zeros_arrow = None - self.selection_area = HorizontalSelection(0, 0, 0, 0, fillcolor=settings.SELECTION_COLOR, - opacity=settings.SELECTION_OPACITY) + self.selection_area = HorizontalSelection( + 0, + 0, + 0, + 0, + fillcolor=settings.SELECTION_COLOR, + opacity=settings.SELECTION_OPACITY, + ) self.addItem(self.selection_area) @property @@ -43,7 +54,14 @@ def draw_noise_area(self, y, h): area.hide() if self.noise_area is None or self.noise_area.scene() != self: - roi = HorizontalSelection(x, y, w, h, fillcolor=settings.NOISE_COLOR, opacity=settings.NOISE_OPACITY) + roi = HorizontalSelection( + x, + y, + w, + h, + fillcolor=settings.NOISE_COLOR, + opacity=settings.NOISE_OPACITY, + ) self.noise_area = roi self.addItem(self.noise_area) else: @@ -82,13 +100,23 @@ def redraw_legend(self, force_show=False): for i, caption in enumerate(self.captions): caption.show() - scale_x, scale_y = util.calc_x_y_scale(self.separation_areas[i].rect(), self.parent()) + scale_x, scale_y = util.calc_x_y_scale( + self.separation_areas[i].rect(), self.parent() + ) try: - caption.setPos(view_rect.x() + view_rect.width() - fm.width(caption.text()) * scale_x, - self.centers[i] + padding) + caption.setPos( + view_rect.x() + + view_rect.width() + - fm.width(caption.text()) * scale_x, + self.centers[i] + padding, + ) except IndexError: - caption.setPos(view_rect.x() + view_rect.width() - fm.width(caption.text()) * scale_x, - self.centers[i - 1] - padding - fm.height() * scale_y) + caption.setPos( + view_rect.x() + + view_rect.width() + - fm.width(caption.text()) * scale_x, + self.centers[i - 1] - padding - fm.height() * scale_y, + ) caption.setTransform(QTransform.fromScale(scale_x, scale_y), False) @@ -121,7 +149,9 @@ def draw_sep_area(self, centers: np.ndarray, show_symbols=False): for i, area in enumerate(self.separation_areas): area.show() try: - self.separation_areas[i].setRect(x, start, w, abs(start - reversed_centers[i])) + self.separation_areas[i].setRect( + x, start, w, abs(start - reversed_centers[i]) + ) start += abs(start - reversed_centers[i]) except IndexError: self.separation_areas[i].setRect(x, start, w, abs(start - h)) diff --git a/src/urh/ui/ui_advanced_modulation_settings.py b/src/urh/ui/ui_advanced_modulation_settings.py index 33df15565d..388ad581f1 100644 --- a/src/urh/ui/ui_advanced_modulation_settings.py +++ b/src/urh/ui/ui_advanced_modulation_settings.py @@ -43,11 +43,15 @@ def setupUi(self, DialogAdvancedModSettings): self.spinBoxMessageLengthDivisor.setObjectName("spinBoxMessageLengthDivisor") self.verticalLayout_2.addWidget(self.spinBoxMessageLengthDivisor) self.verticalLayout_3.addWidget(self.groupBox_2) - spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_3.addItem(spacerItem) self.buttonBox = QtWidgets.QDialogButtonBox(DialogAdvancedModSettings) self.buttonBox.setOrientation(QtCore.Qt.Horizontal) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setObjectName("buttonBox") self.verticalLayout_3.addWidget(self.buttonBox) @@ -57,9 +61,27 @@ def setupUi(self, DialogAdvancedModSettings): def retranslateUi(self, DialogAdvancedModSettings): _translate = QtCore.QCoreApplication.translate - DialogAdvancedModSettings.setWindowTitle(_translate("DialogAdvancedModSettings", "Advanced Modulation Settings")) - self.groupBox.setTitle(_translate("DialogAdvancedModSettings", "Pause Threshold")) - self.label.setText(_translate("DialogAdvancedModSettings", "
" + + " " * err.offset + + "^
The pause threshold gives you control when to insert a message break.
The pause threshold is the maximum length of consecutive zero bits represented by a pause before a new message begins.
Special value is 0 to disable message breaking completely.
")) - self.spinBoxPauseThreshold.setSpecialValueText(_translate("DialogAdvancedModSettings", "Disable")) - self.groupBox_2.setTitle(_translate("DialogAdvancedModSettings", "Message Length Divisor")) - self.label_2.setText(_translate("DialogAdvancedModSettings", "With the message divisor length you can control the minimum message length in a flexible way. URH will try to demodulate signals in such a way, that the resulting message has a number of bits that is divisble by the configured divisor.
How does the zero padding work? Remaining zero bits are taken from the pause behind the message if possible.
The pause threshold gives you control when to insert a message break.
The pause threshold is the maximum length of consecutive zero bits represented by a pause before a new message begins.
Special value is 0 to disable message breaking completely.
', + ) + ) + self.spinBoxPauseThreshold.setSpecialValueText( + _translate("DialogAdvancedModSettings", "Disable") + ) + self.groupBox_2.setTitle( + _translate("DialogAdvancedModSettings", "Message Length Divisor") + ) + self.label_2.setText( + _translate( + "DialogAdvancedModSettings", + 'With the message divisor length you can control the minimum message length in a flexible way. URH will try to demodulate signals in such a way, that the resulting message has a number of bits that is divisble by the configured divisor.
How does the zero padding work? Remaining zero bits are taken from the pause behind the message if possible.
Set the desired view here.
")) + self.chkBoxShowOnlyDiffs.setText( + _translate("TabAnalysis", "Show only diffs in protocol") + ) + self.cbProtoView.setToolTip( + _translate( + "TabAnalysis", + "Set the desired view here.
", + ) + ) self.cbProtoView.setItemText(0, _translate("TabAnalysis", "Bits")) self.cbProtoView.setItemText(1, _translate("TabAnalysis", "Hex")) self.cbProtoView.setItemText(2, _translate("TabAnalysis", "ASCII")) self.lDecodingErrorsValue.setText(_translate("TabAnalysis", "0 (0.00%)")) - self.chkBoxOnlyShowLabelsInProtocol.setText(_translate("TabAnalysis", "Show only labels in protocol")) + self.chkBoxOnlyShowLabelsInProtocol.setText( + _translate("TabAnalysis", "Show only labels in protocol") + ) self.cbShowDiffs.setText(_translate("TabAnalysis", "Mark diffs in protocol")) - self.btnAnalyze.setToolTip(_translate("TabAnalysis", "Run some automatic analysis on the protocol e.g. assign labels automatically. You can configure which checks to run with the arrow on the right of this button.
")) + self.btnAnalyze.setToolTip( + _translate( + "TabAnalysis", + "Run some automatic analysis on the protocol e.g. assign labels automatically. You can configure which checks to run with the arrow on the right of this button.
", + ) + ) self.btnAnalyze.setText(_translate("TabAnalysis", "Analyze Protocol")) - self.btnSaveProto.setToolTip(_translate("TabAnalysis", "Save current protocol.")) + self.btnSaveProto.setToolTip( + _translate("TabAnalysis", "Save current protocol.") + ) self.lSlash.setText(_translate("TabAnalysis", "/")) self.lblShownRows.setText(_translate("TabAnalysis", "shown: 42/108")) - self.lTime.setToolTip(_translate("TabAnalysis", "The MessageStart is the point in time when a protocol message begins. Additionally the relative time (+ ...) from the previous message is shown.
")) + self.lTime.setToolTip( + _translate( + "TabAnalysis", + 'The MessageStart is the point in time when a protocol message begins. Additionally the relative time (+ ...) from the previous message is shown.
', + ) + ) self.lTime.setText(_translate("TabAnalysis", "0 (+0)")) self.lSearchCurrent.setText(_translate("TabAnalysis", "-")) - self.lblRSSI.setToolTip(_translate("TabAnalysis", "This is the average signal power of the current message. The nearer this value is to zero, the stronger the signal is.
")) + self.lblRSSI.setToolTip( + _translate( + "TabAnalysis", + "This is the average signal power of the current message. The nearer this value is to zero, the stronger the signal is.
", + ) + ) self.lblRSSI.setText(_translate("TabAnalysis", "-∞ dBm")) self.btnNextSearch.setText(_translate("TabAnalysis", ">")) self.btnPrevSearch.setText(_translate("TabAnalysis", "<")) self.lSearchTotal.setText(_translate("TabAnalysis", "-")) - self.label_3.setToolTip(_translate("TabAnalysis", "The Message Start is the point in time when a protocol message begins. Additionally the relative time (+ ...) from the previous message is shown.
")) + self.label_3.setToolTip( + _translate( + "TabAnalysis", + 'The Message Start is the point in time when a protocol message begins. Additionally the relative time (+ ...) from the previous message is shown.
', + ) + ) self.label_3.setText(_translate("TabAnalysis", "Timestamp:")) self.btnSearchSelectFilter.setText(_translate("TabAnalysis", "Search")) self.btnLoadProto.setToolTip(_translate("TabAnalysis", "Load a protocol.")) self.btnLoadProto.setText(_translate("TabAnalysis", "...")) - self.lblClearAlignment.setText(_translate("TabAnalysis", "")) - self.lineEditSearch.setPlaceholderText(_translate("TabAnalysis", "Enter pattern here")) + self.lblClearAlignment.setText( + _translate( + "TabAnalysis", + '', + ) + ) + self.lineEditSearch.setPlaceholderText( + _translate("TabAnalysis", "Enter pattern here") + ) self.lBits.setText(_translate("TabAnalysis", "Bit:")) self.lHex.setText(_translate("TabAnalysis", "Hex:")) self.lDecimal.setText(_translate("TabAnalysis", "Decimal:")) self.lNumSelectedColumns.setText(_translate("TabAnalysis", "0")) - self.lColumnsSelectedText.setText(_translate("TabAnalysis", "column(s) selected")) + self.lColumnsSelectedText.setText( + _translate("TabAnalysis", "column(s) selected") + ) self.label.setText(_translate("TabAnalysis", "Message types")) self.lblLabelValues.setText(_translate("TabAnalysis", "Labels for message")) - self.btnAddMessagetype.setToolTip(_translate("TabAnalysis", "Add a new message type")) - self.btnAddMessagetype.setText(_translate("TabAnalysis", "Add new message type")) + self.btnAddMessagetype.setToolTip( + _translate("TabAnalysis", "Add a new message type") + ) + self.btnAddMessagetype.setText( + _translate("TabAnalysis", "Add new message type") + ) + + from urh.ui.views.LabelValueTableView import LabelValueTableView from urh.ui.views.MessageTypeTableView import MessageTypeTableView from urh.ui.views.ProtocolTableView import ProtocolTableView diff --git a/src/urh/ui/ui_checksum_options_widget.py b/src/urh/ui/ui_checksum_options_widget.py index 281139ec56..582adb59b5 100644 --- a/src/urh/ui/ui_checksum_options_widget.py +++ b/src/urh/ui/ui_checksum_options_widget.py @@ -66,7 +66,9 @@ def setupUi(self, ChecksumOptions): self.btnRemoveRange.setIcon(icon) self.btnRemoveRange.setObjectName("btnRemoveRange") self.verticalLayout.addWidget(self.btnRemoveRange) - spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout.addItem(spacerItem) self.horizontalLayout.addLayout(self.verticalLayout) self.gridLayout_2.addWidget(self.groupBox, 7, 0, 1, 2) @@ -104,7 +106,7 @@ def setupUi(self, ChecksumOptions): self.verticalLayout_2 = QtWidgets.QVBoxLayout(self.page_wsp) self.verticalLayout_2.setObjectName("verticalLayout_2") self.label_6 = QtWidgets.QLabel(self.page_wsp) - self.label_6.setAlignment(QtCore.Qt.AlignJustify|QtCore.Qt.AlignVCenter) + self.label_6.setAlignment(QtCore.Qt.AlignJustify | QtCore.Qt.AlignVCenter) self.label_6.setWordWrap(True) self.label_6.setObjectName("label_6") self.verticalLayout_2.addWidget(self.label_6) @@ -120,18 +122,24 @@ def setupUi(self, ChecksumOptions): self.radioButtonWSPCRC8 = QtWidgets.QRadioButton(self.page_wsp) self.radioButtonWSPCRC8.setObjectName("radioButtonWSPCRC8") self.verticalLayout_2.addWidget(self.radioButtonWSPCRC8) - spacerItem1 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_2.addItem(spacerItem1) self.stackedWidget.addWidget(self.page_wsp) self.verticalLayout_3.addWidget(self.stackedWidget) - spacerItem2 = QtWidgets.QSpacerItem(20, 107, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem2 = QtWidgets.QSpacerItem( + 20, 107, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_3.addItem(spacerItem2) self.scrollArea.setWidget(self.scrollAreaWidgetContents) self.gridLayout.addWidget(self.scrollArea, 1, 0, 1, 2) self.retranslateUi(ChecksumOptions) self.stackedWidget.setCurrentIndex(0) - ChecksumOptions.setTabOrder(self.comboBoxCRCFunction, self.lineEditCRCPolynomial) + ChecksumOptions.setTabOrder( + self.comboBoxCRCFunction, self.lineEditCRCPolynomial + ) ChecksumOptions.setTabOrder(self.lineEditCRCPolynomial, self.lineEditStartValue) ChecksumOptions.setTabOrder(self.lineEditStartValue, self.lineEditFinalXOR) ChecksumOptions.setTabOrder(self.lineEditFinalXOR, self.tableViewDataRanges) @@ -139,27 +147,57 @@ def setupUi(self, ChecksumOptions): ChecksumOptions.setTabOrder(self.btnAddRange, self.radioButtonWSPAuto) ChecksumOptions.setTabOrder(self.radioButtonWSPAuto, self.btnRemoveRange) ChecksumOptions.setTabOrder(self.btnRemoveRange, self.radioButtonWSPChecksum4) - ChecksumOptions.setTabOrder(self.radioButtonWSPChecksum4, self.radioButtonWSPChecksum8) - ChecksumOptions.setTabOrder(self.radioButtonWSPChecksum8, self.radioButtonWSPCRC8) + ChecksumOptions.setTabOrder( + self.radioButtonWSPChecksum4, self.radioButtonWSPChecksum8 + ) + ChecksumOptions.setTabOrder( + self.radioButtonWSPChecksum8, self.radioButtonWSPCRC8 + ) def retranslateUi(self, ChecksumOptions): _translate = QtCore.QCoreApplication.translate - ChecksumOptions.setWindowTitle(_translate("ChecksumOptions", "Configure Checksum")) + ChecksumOptions.setWindowTitle( + _translate("ChecksumOptions", "Configure Checksum") + ) self.label_4.setText(_translate("ChecksumOptions", "Checksum category:")) self.comboBoxCategory.setItemText(0, _translate("ChecksumOptions", "CRC")) - self.comboBoxCategory.setItemText(1, _translate("ChecksumOptions", "Wireless Short Packet Checksum")) + self.comboBoxCategory.setItemText( + 1, _translate("ChecksumOptions", "Wireless Short Packet Checksum") + ) self.label_5.setText(_translate("ChecksumOptions", "CRC polynomial (hex):")) - self.groupBox.setTitle(_translate("ChecksumOptions", "Configure data ranges for CRC")) + self.groupBox.setTitle( + _translate("ChecksumOptions", "Configure data ranges for CRC") + ) self.btnAddRange.setText(_translate("ChecksumOptions", "...")) self.btnRemoveRange.setText(_translate("ChecksumOptions", "...")) self.label_3.setText(_translate("ChecksumOptions", "CRC function:")) self.label_2.setText(_translate("ChecksumOptions", "Final XOR (hex):")) self.label.setText(_translate("ChecksumOptions", "Start value (hex):")) - self.label_crc_info.setText(_translate("ChecksumOptions", "Order=17
Length of checksum=16
start value length =16
final XOR length = 16
Polynomial = x1 + 4
")) - self.checkBoxRefIn.setText(_translate("ChecksumOptions", "RefIn (Reflect input)")) - self.checkBoxRefOut.setText(_translate("ChecksumOptions", "RefOut (Reflect output)")) - self.label_6.setText(_translate("ChecksumOptions", "The Wireless Short Packet (WSP) standard uses three different checksums. URH can automatically detect the used checksum algorithm from the message. However, you can enforce the usage of a certain checksum if you need to.
With Automatic setting, checksums are chosen by these rules:
1) 4 Bit Checksum - For Switch Telegram (RORG=5 or 6 and STATUS = 0x20 or 0x30)
2) 8 Bit Checksum: STATUS bit 27 = 0
3) 8 Bit CRC: STATUS bit 27 = 1
")) - self.radioButtonWSPAuto.setText(_translate("ChecksumOptions", "Automatic (recommended)")) - self.radioButtonWSPChecksum4.setText(_translate("ChecksumOptions", "Force Checksum4")) - self.radioButtonWSPChecksum8.setText(_translate("ChecksumOptions", "Force Checksum8")) + self.label_crc_info.setText( + _translate( + "ChecksumOptions", + 'Order=17
Length of checksum=16
start value length =16
final XOR length = 16
Polynomial = x1 + 4
', + ) + ) + self.checkBoxRefIn.setText( + _translate("ChecksumOptions", "RefIn (Reflect input)") + ) + self.checkBoxRefOut.setText( + _translate("ChecksumOptions", "RefOut (Reflect output)") + ) + self.label_6.setText( + _translate( + "ChecksumOptions", + 'The Wireless Short Packet (WSP) standard uses three different checksums. URH can automatically detect the used checksum algorithm from the message. However, you can enforce the usage of a certain checksum if you need to.
With Automatic setting, checksums are chosen by these rules:
1) 4 Bit Checksum - For Switch Telegram (RORG=5 or 6 and STATUS = 0x20 or 0x30)
2) 8 Bit Checksum: STATUS bit 27 = 0
3) 8 Bit CRC: STATUS bit 27 = 1
', + ) + ) + self.radioButtonWSPAuto.setText( + _translate("ChecksumOptions", "Automatic (recommended)") + ) + self.radioButtonWSPChecksum4.setText( + _translate("ChecksumOptions", "Force Checksum4") + ) + self.radioButtonWSPChecksum8.setText( + _translate("ChecksumOptions", "Force Checksum8") + ) self.radioButtonWSPCRC8.setText(_translate("ChecksumOptions", "Force CRC8")) diff --git a/src/urh/ui/ui_costa.py b/src/urh/ui/ui_costa.py index a5a8a59e18..72e4237e09 100644 --- a/src/urh/ui/ui_costa.py +++ b/src/urh/ui/ui_costa.py @@ -16,7 +16,9 @@ def setupUi(self, DialogCosta): self.verticalLayout = QtWidgets.QVBoxLayout(DialogCosta) self.verticalLayout.setObjectName("verticalLayout") self.label = QtWidgets.QLabel(DialogCosta) - self.label.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignTop) + self.label.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignTop + ) self.label.setWordWrap(True) self.label.setObjectName("label") self.verticalLayout.addWidget(self.label) @@ -32,10 +34,14 @@ def setupUi(self, DialogCosta): self.verticalLayout.addLayout(self.horizontalLayout) self.buttonBox = QtWidgets.QDialogButtonBox(DialogCosta) self.buttonBox.setOrientation(QtCore.Qt.Horizontal) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setObjectName("buttonBox") self.verticalLayout.addWidget(self.buttonBox) - spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout.addItem(spacerItem) self.retranslateUi(DialogCosta) @@ -45,5 +51,10 @@ def setupUi(self, DialogCosta): def retranslateUi(self, DialogCosta): _translate = QtCore.QCoreApplication.translate DialogCosta.setWindowTitle(_translate("DialogCosta", "Configure Costas Loop")) - self.label.setText(_translate("DialogCosta", "URH uses a Costas loop for PSK demodulation. Configure the loop bandwidth below.")) + self.label.setText( + _translate( + "DialogCosta", + "URH uses a Costas loop for PSK demodulation. Configure the loop bandwidth below.", + ) + ) self.labelLoopBandwidth.setText(_translate("DialogCosta", "Loop Bandwidth:")) diff --git a/src/urh/ui/ui_csv_wizard.py b/src/urh/ui/ui_csv_wizard.py index 97b04b119d..1c23beb1af 100644 --- a/src/urh/ui/ui_csv_wizard.py +++ b/src/urh/ui/ui_csv_wizard.py @@ -89,14 +89,18 @@ def setupUi(self, DialogCSVImport): self.gridLayout.addWidget(self.label_4, 3, 0, 1, 2) self.buttonBox = QtWidgets.QDialogButtonBox(DialogCSVImport) self.buttonBox.setOrientation(QtCore.Qt.Horizontal) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setObjectName("buttonBox") self.gridLayout.addWidget(self.buttonBox, 9, 2, 1, 1) self.groupBoxFilePreview = QtWidgets.QGroupBox(DialogCSVImport) self.groupBoxFilePreview.setObjectName("groupBoxFilePreview") self.verticalLayout = QtWidgets.QVBoxLayout(self.groupBoxFilePreview) self.verticalLayout.setObjectName("verticalLayout") - self.plainTextEditFilePreview = QtWidgets.QPlainTextEdit(self.groupBoxFilePreview) + self.plainTextEditFilePreview = QtWidgets.QPlainTextEdit( + self.groupBoxFilePreview + ) self.plainTextEditFilePreview.setUndoRedoEnabled(False) self.plainTextEditFilePreview.setReadOnly(True) self.plainTextEditFilePreview.setObjectName("plainTextEditFilePreview") @@ -115,26 +119,53 @@ def setupUi(self, DialogCSVImport): self.buttonBox.rejected.connect(DialogCSVImport.reject) DialogCSVImport.setTabOrder(self.lineEditFilename, self.btnChooseFile) DialogCSVImport.setTabOrder(self.btnChooseFile, self.plainTextEditFilePreview) - DialogCSVImport.setTabOrder(self.plainTextEditFilePreview, self.comboBoxCSVSeparator) + DialogCSVImport.setTabOrder( + self.plainTextEditFilePreview, self.comboBoxCSVSeparator + ) DialogCSVImport.setTabOrder(self.comboBoxCSVSeparator, self.btnAddSeparator) DialogCSVImport.setTabOrder(self.btnAddSeparator, self.spinBoxIDataColumn) DialogCSVImport.setTabOrder(self.spinBoxIDataColumn, self.spinBoxQDataColumn) - DialogCSVImport.setTabOrder(self.spinBoxQDataColumn, self.spinBoxTimestampColumn) - DialogCSVImport.setTabOrder(self.spinBoxTimestampColumn, self.tableWidgetPreview) + DialogCSVImport.setTabOrder( + self.spinBoxQDataColumn, self.spinBoxTimestampColumn + ) + DialogCSVImport.setTabOrder( + self.spinBoxTimestampColumn, self.tableWidgetPreview + ) def retranslateUi(self, DialogCSVImport): _translate = QtCore.QCoreApplication.translate DialogCSVImport.setWindowTitle(_translate("DialogCSVImport", "CSV Import")) - self.labelFileNotFound.setText(_translate("DialogCSVImport", "Could not open the selected file.
")) + self.labelFileNotFound.setText( + _translate( + "DialogCSVImport", + 'Could not open the selected file.
', + ) + ) self.comboBoxCSVSeparator.setItemText(0, _translate("DialogCSVImport", ",")) self.comboBoxCSVSeparator.setItemText(1, _translate("DialogCSVImport", ";")) - self.btnAddSeparator.setToolTip(_translate("DialogCSVImport", "Add a custom separator.")) + self.btnAddSeparator.setToolTip( + _translate("DialogCSVImport", "Add a custom separator.") + ) self.btnAddSeparator.setText(_translate("DialogCSVImport", "...")) - self.spinBoxTimestampColumn.setToolTip(_translate("DialogCSVImport", "If your dataset contains timestamps URH will calculate the sample rate from them. You can manually edit the sample rate after import in the signal details.
")) - self.spinBoxTimestampColumn.setSpecialValueText(_translate("DialogCSVImport", "Not present")) + self.spinBoxTimestampColumn.setToolTip( + _translate( + "DialogCSVImport", + "If your dataset contains timestamps URH will calculate the sample rate from them. You can manually edit the sample rate after import in the signal details.
", + ) + ) + self.spinBoxTimestampColumn.setSpecialValueText( + _translate("DialogCSVImport", "Not present") + ) self.label.setText(_translate("DialogCSVImport", "I Data Column:")) - self.spinBoxQDataColumn.setSpecialValueText(_translate("DialogCSVImport", "Not present")) - self.label_3.setToolTip(_translate("DialogCSVImport", "If your dataset contains timestamps URH will calculate the sample rate from them. You can manually edit the sample rate after import in the signal details.
")) + self.spinBoxQDataColumn.setSpecialValueText( + _translate("DialogCSVImport", "Not present") + ) + self.label_3.setToolTip( + _translate( + "DialogCSVImport", + "If your dataset contains timestamps URH will calculate the sample rate from them. You can manually edit the sample rate after import in the signal details.
", + ) + ) self.label_3.setText(_translate("DialogCSVImport", "Timestamp Column:")) self.label_2.setText(_translate("DialogCSVImport", "Q Data Column:")) self.groupBox.setTitle(_translate("DialogCSVImport", "Preview")) @@ -146,6 +177,10 @@ def retranslateUi(self, DialogCSVImport): item.setText(_translate("DialogCSVImport", "Q")) self.btnChooseFile.setText(_translate("DialogCSVImport", "...")) self.label_4.setText(_translate("DialogCSVImport", "CSV Separator:")) - self.groupBoxFilePreview.setTitle(_translate("DialogCSVImport", "File Content (at most 100 rows)")) + self.groupBoxFilePreview.setTitle( + _translate("DialogCSVImport", "File Content (at most 100 rows)") + ) self.label_5.setText(_translate("DialogCSVImport", "File to import:")) - self.btnAutoDefault.setText(_translate("DialogCSVImport", "Prevent Dialog From Close with Enter")) + self.btnAutoDefault.setText( + _translate("DialogCSVImport", "Prevent Dialog From Close with Enter") + ) diff --git a/src/urh/ui/ui_decoding.py b/src/urh/ui/ui_decoding.py index ba29dc1dfd..17b273b866 100644 --- a/src/urh/ui/ui_decoding.py +++ b/src/urh/ui/ui_decoding.py @@ -29,7 +29,9 @@ def setupUi(self, Decoder): self.saveas = QtWidgets.QPushButton(Decoder) self.saveas.setObjectName("saveas") self.horizontalLayout_2.addWidget(self.saveas) - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_2.addItem(spacerItem) self.verticalLayout_4.addLayout(self.horizontalLayout_2) self.horizontalLayout = QtWidgets.QHBoxLayout() @@ -40,10 +42,14 @@ def setupUi(self, Decoder): self.label_8.setObjectName("label_8") self.verticalLayout_2.addWidget(self.label_8) self.basefunctions = QtWidgets.QListWidget(Decoder) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.basefunctions.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.basefunctions.sizePolicy().hasHeightForWidth() + ) self.basefunctions.setSizePolicy(sizePolicy) self.basefunctions.setDragEnabled(True) self.basefunctions.setDragDropMode(QtWidgets.QAbstractItemView.DragOnly) @@ -53,10 +59,14 @@ def setupUi(self, Decoder): self.label_9.setObjectName("label_9") self.verticalLayout_2.addWidget(self.label_9) self.additionalfunctions = QtWidgets.QListWidget(Decoder) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.additionalfunctions.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.additionalfunctions.sizePolicy().hasHeightForWidth() + ) self.additionalfunctions.setSizePolicy(sizePolicy) self.additionalfunctions.setDragEnabled(True) self.additionalfunctions.setDragDropMode(QtWidgets.QAbstractItemView.DragOnly) @@ -75,7 +85,9 @@ def setupUi(self, Decoder): self.label.setObjectName("label") self.verticalLayout.addWidget(self.label) self.decoderchain = ListWidget(Decoder) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.decoderchain.sizePolicy().hasHeightForWidth()) @@ -93,16 +105,22 @@ def setupUi(self, Decoder): self.verticalLayout_3 = QtWidgets.QVBoxLayout() self.verticalLayout_3.setObjectName("verticalLayout_3") self.gb_infoandoptions = QtWidgets.QGroupBox(Decoder) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.gb_infoandoptions.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.gb_infoandoptions.sizePolicy().hasHeightForWidth() + ) self.gb_infoandoptions.setSizePolicy(sizePolicy) self.gb_infoandoptions.setObjectName("gb_infoandoptions") self.verticalLayout_5 = QtWidgets.QVBoxLayout(self.gb_infoandoptions) self.verticalLayout_5.setObjectName("verticalLayout_5") self.info = QtWidgets.QLabel(self.gb_infoandoptions) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.info.sizePolicy().hasHeightForWidth()) @@ -111,12 +129,16 @@ def setupUi(self, Decoder): font.setItalic(True) self.info.setFont(font) self.info.setTextFormat(QtCore.Qt.PlainText) - self.info.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignTop) + self.info.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignTop + ) self.info.setWordWrap(True) self.info.setObjectName("info") self.verticalLayout_5.addWidget(self.info) self.optionWidget = QtWidgets.QStackedWidget(self.gb_infoandoptions) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.optionWidget.sizePolicy().hasHeightForWidth()) @@ -158,8 +180,12 @@ def setupUi(self, Decoder): self.label_10.setObjectName("label_10") self.gridLayout.addWidget(self.label_10, 0, 1, 1, 1) self.substitution = QtWidgets.QTableWidget(self.page_substitution) - self.substitution.setVerticalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) - self.substitution.setHorizontalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) + self.substitution.setVerticalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) + self.substitution.setHorizontalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) self.substitution.setObjectName("substitution") self.substitution.setColumnCount(0) self.substitution.setRowCount(0) @@ -193,7 +219,9 @@ def setupUi(self, Decoder): self.btnChooseEncoder.setObjectName("btnChooseEncoder") self.horizontalLayout_4.addWidget(self.btnChooseEncoder) self.verticalLayout_6.addLayout(self.horizontalLayout_4) - spacerItem1 = QtWidgets.QSpacerItem(20, 158, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 158, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_6.addItem(spacerItem1) self.optionWidget.addWidget(self.page_external) self.page_data_whitening = QtWidgets.QWidget() @@ -281,7 +309,9 @@ def setupUi(self, Decoder): self.verticalLayout_4.addLayout(self.horizontalLayout) self.gridLayout_2 = QtWidgets.QGridLayout() self.gridLayout_2.setObjectName("gridLayout_2") - spacerItem2 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem2 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_2.addItem(spacerItem2, 0, 1, 1, 1) self.inpt = QtWidgets.QLineEdit(Decoder) self.inpt.setInputMethodHints(QtCore.Qt.ImhDigitsOnly) @@ -309,7 +339,9 @@ def setupUi(self, Decoder): self.gridLayout_2.addWidget(self.label_3, 3, 0, 1, 1) self.verticalLayout_4.addLayout(self.gridLayout_2) self.decoding_errors_label = QtWidgets.QLabel(Decoder) - self.decoding_errors_label.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter) + self.decoding_errors_label.setAlignment( + QtCore.Qt.AlignRight | QtCore.Qt.AlignTrailing | QtCore.Qt.AlignVCenter + ) self.decoding_errors_label.setObjectName("decoding_errors_label") self.verticalLayout_4.addWidget(self.decoding_errors_label) @@ -319,25 +351,44 @@ def setupUi(self, Decoder): def retranslateUi(self, Decoder): _translate = QtCore.QCoreApplication.translate Decoder.setWindowTitle(_translate("Decoder", "Decoding")) - self.combobox_decodings.setItemText(0, _translate("Decoder", "Non Return to Zero (NRZ)")) + self.combobox_decodings.setItemText( + 0, _translate("Decoder", "Non Return to Zero (NRZ)") + ) self.combobox_decodings.setItemText(1, _translate("Decoder", "Empty")) self.delete_decoding.setText(_translate("Decoder", "Delete")) self.saveas.setText(_translate("Decoder", "Save as...")) self.label_8.setText(_translate("Decoder", "Base Functions")) self.label_9.setText(_translate("Decoder", "Additional Functions")) self.label.setText(_translate("Decoder", "Your Decoding")) - self.gb_infoandoptions.setTitle(_translate("Decoder", "Information and Options")) - self.info.setText(_translate("Decoder", "Please drag functions from the categories base and additional to the decoding process (Decoder). You can reorder functions by drag and drop and remove functions by dropping them outside the Decoder box. Click on every function for detailed information.")) + self.gb_infoandoptions.setTitle( + _translate("Decoder", "Information and Options") + ) + self.info.setText( + _translate( + "Decoder", + "Please drag functions from the categories base and additional to the decoding process (Decoder). You can reorder functions by drag and drop and remove functions by dropping them outside the Decoder box. Click on every function for detailed information.", + ) + ) self.label_5.setText(_translate("Decoder", "Number of redundant bits")) - self.label_6.setText(_translate("Decoder", "Carrier (\'1_\' -> 1_1_1_...)")) + self.label_6.setText(_translate("Decoder", "Carrier ('1_' -> 1_1_1_...)")) self.label_10.setText(_translate("Decoder", "Rows")) self.label_11.setText(_translate("Decoder", "Decoder")) self.btnChooseDecoder.setText(_translate("Decoder", "...")) self.label_12.setText(_translate("Decoder", "Encoder")) self.btnChooseEncoder.setText(_translate("Decoder", "...")) - self.label_13.setText(_translate("Decoder", "Synchronization bytes (hex coded)")) - self.label_14.setText(_translate("Decoder", "Data whitening polynomial (LFSR, hex, w/o first bit)")) - self.datawhitening_overwrite_crc.setText(_translate("Decoder", "Overwrite CRC16 field with correct value when encoding")) + self.label_13.setText( + _translate("Decoder", "Synchronization bytes (hex coded)") + ) + self.label_14.setText( + _translate( + "Decoder", "Data whitening polynomial (LFSR, hex, w/o first bit)" + ) + ) + self.datawhitening_overwrite_crc.setText( + _translate( + "Decoder", "Overwrite CRC16 field with correct value when encoding" + ) + ) self.cutmark.setText(_translate("Decoder", "1010")) self.label_15.setText(_translate("Decoder", "Sequence")) self.rB_delbefore.setText(_translate("Decoder", "&Cut before")) @@ -345,13 +396,25 @@ def retranslateUi(self, Decoder): self.rB_delbeforepos.setText(_translate("Decoder", "Cut before")) self.rB_delafterpos.setText(_translate("Decoder", "Cut after")) self.label_16.setText(_translate("Decoder", "Position (in bit)")) - self.label_17.setText(_translate("Decoder", "Maximum (<=) length of 1-sequence for: Low (0)")) - self.label_18.setText(_translate("Decoder", "Minimum (>=) length of 1-sequence for: High (1)")) - self.label_19.setText(_translate("Decoder", "Number of 0s between 1-sequences (just for encoding)")) + self.label_17.setText( + _translate("Decoder", "Maximum (<=) length of 1-sequence for: Low (0)") + ) + self.label_18.setText( + _translate("Decoder", "Minimum (>=) length of 1-sequence for: High (1)") + ) + self.label_19.setText( + _translate( + "Decoder", "Number of 0s between 1-sequences (just for encoding)" + ) + ) self.btnAddtoYourDecoding.setText(_translate("Decoder", "Add to Your Decoding")) self.combobox_signals.setItemText(0, _translate("Decoder", "Test")) self.label_2.setText(_translate("Decoder", "Signal {0,1}:")) self.label_3.setText(_translate("Decoder", "Decoded Bits:")) - self.decoding_errors_label.setText(_translate("Decoder", "[Decoding Errors = 0]")) + self.decoding_errors_label.setText( + _translate("Decoder", "[Decoding Errors = 0]") + ) + + from urh.ui.ListWidget import ListWidget from urh.ui.views.ZoomableGraphicView import ZoomableGraphicView diff --git a/src/urh/ui/ui_filter_bandwidth_dialog.py b/src/urh/ui/ui_filter_bandwidth_dialog.py index 6e5e3d059c..8052e41d8f 100644 --- a/src/urh/ui/ui_filter_bandwidth_dialog.py +++ b/src/urh/ui/ui_filter_bandwidth_dialog.py @@ -16,22 +16,32 @@ def setupUi(self, DialogFilterBandwidth): self.verticalLayout.setSpacing(7) self.verticalLayout.setObjectName("verticalLayout") self.labelExplanation = QtWidgets.QLabel(DialogFilterBandwidth) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.labelExplanation.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.labelExplanation.sizePolicy().hasHeightForWidth() + ) self.labelExplanation.setSizePolicy(sizePolicy) - self.labelExplanation.setAlignment(QtCore.Qt.AlignJustify|QtCore.Qt.AlignTop) + self.labelExplanation.setAlignment(QtCore.Qt.AlignJustify | QtCore.Qt.AlignTop) self.labelExplanation.setWordWrap(True) self.labelExplanation.setObjectName("labelExplanation") self.verticalLayout.addWidget(self.labelExplanation) self.gridLayout = QtWidgets.QGridLayout() self.gridLayout.setObjectName("gridLayout") - self.doubleSpinBoxCustomBandwidth = QtWidgets.QDoubleSpinBox(DialogFilterBandwidth) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed) + self.doubleSpinBoxCustomBandwidth = QtWidgets.QDoubleSpinBox( + DialogFilterBandwidth + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.doubleSpinBoxCustomBandwidth.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.doubleSpinBoxCustomBandwidth.sizePolicy().hasHeightForWidth() + ) self.doubleSpinBoxCustomBandwidth.setSizePolicy(sizePolicy) self.doubleSpinBoxCustomBandwidth.setDecimals(4) self.doubleSpinBoxCustomBandwidth.setMinimum(0.0001) @@ -89,7 +99,9 @@ def setupUi(self, DialogFilterBandwidth): self.radioButtonVery_Narrow.setObjectName("radioButtonVery_Narrow") self.gridLayout.addWidget(self.radioButtonVery_Narrow, 2, 0, 1, 1) self.label = QtWidgets.QLabel(DialogFilterBandwidth) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label.sizePolicy().hasHeightForWidth()) @@ -113,7 +125,9 @@ def setupUi(self, DialogFilterBandwidth): self.verticalLayout.addLayout(self.gridLayout) self.buttonBox = QtWidgets.QDialogButtonBox(DialogFilterBandwidth) self.buttonBox.setOrientation(QtCore.Qt.Horizontal) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setCenterButtons(True) self.buttonBox.setObjectName("buttonBox") self.verticalLayout.addWidget(self.buttonBox) @@ -127,24 +141,59 @@ def setupUi(self, DialogFilterBandwidth): def retranslateUi(self, DialogFilterBandwidth): _translate = QtCore.QCoreApplication.translate - DialogFilterBandwidth.setWindowTitle(_translate("DialogFilterBandwidth", "Configure Filter Bandwidth")) - self.labelExplanation.setText(_translate("DialogFilterBandwidth", "To separate the frequency bands from each other a bandpass filter is used. You can configure the bandwidth of this filter here. The bandwidth determines the length N of the filter kernel. Decreasing the bandwidth will increase the accuracy of the filter, at cost of higher computation time.")) + DialogFilterBandwidth.setWindowTitle( + _translate("DialogFilterBandwidth", "Configure Filter Bandwidth") + ) + self.labelExplanation.setText( + _translate( + "DialogFilterBandwidth", + "To separate the frequency bands from each other a bandpass filter is used. You can configure the bandwidth of this filter here. The bandwidth determines the length N of the filter kernel. Decreasing the bandwidth will increase the accuracy of the filter, at cost of higher computation time.", + ) + ) self.radioButtonCustom.setText(_translate("DialogFilterBandwidth", "Custom")) - self.labelMediumKernelLength.setText(_translate("DialogFilterBandwidth", "TextLabel")) + self.labelMediumKernelLength.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) self.radioButtonWide.setText(_translate("DialogFilterBandwidth", "Wide")) - self.labelVeryWideKernelLength.setText(_translate("DialogFilterBandwidth", "TextLabel")) + self.labelVeryWideKernelLength.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) self.radioButtonNarrow.setText(_translate("DialogFilterBandwidth", "Narrow")) - self.labelWideBandwidth.setText(_translate("DialogFilterBandwidth", "TextLabel")) - self.labelVeryNarrowKernelLength.setText(_translate("DialogFilterBandwidth", "TextLabel")) - self.labelBandwidthCaption.setText(_translate("DialogFilterBandwidth", "Bandwidth (Hz)")) - self.labelNarrowBandwidth.setText(_translate("DialogFilterBandwidth", "TextLabel")) - self.labelNarrowKernelLength.setText(_translate("DialogFilterBandwidth", "TextLabel")) - self.labelVeryNarrowBandwidth.setText(_translate("DialogFilterBandwidth", "TextLabel")) - self.labelKernelLengthCaption.setText(_translate("DialogFilterBandwidth", "Kernel Length N")) - self.labelWideKernelLength.setText(_translate("DialogFilterBandwidth", "TextLabel")) - self.radioButtonVery_Wide.setText(_translate("DialogFilterBandwidth", "Very Wide")) - self.radioButtonVery_Narrow.setText(_translate("DialogFilterBandwidth", "Very Narrow")) + self.labelWideBandwidth.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) + self.labelVeryNarrowKernelLength.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) + self.labelBandwidthCaption.setText( + _translate("DialogFilterBandwidth", "Bandwidth (Hz)") + ) + self.labelNarrowBandwidth.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) + self.labelNarrowKernelLength.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) + self.labelVeryNarrowBandwidth.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) + self.labelKernelLengthCaption.setText( + _translate("DialogFilterBandwidth", "Kernel Length N") + ) + self.labelWideKernelLength.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) + self.radioButtonVery_Wide.setText( + _translate("DialogFilterBandwidth", "Very Wide") + ) + self.radioButtonVery_Narrow.setText( + _translate("DialogFilterBandwidth", "Very Narrow") + ) self.label.setText(_translate("DialogFilterBandwidth", "Choose ")) - self.labelVeryWideBandwidth.setText(_translate("DialogFilterBandwidth", "TextLabel")) + self.labelVeryWideBandwidth.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) self.radioButtonMedium.setText(_translate("DialogFilterBandwidth", "Medium")) - self.labelMediumBandwidth.setText(_translate("DialogFilterBandwidth", "TextLabel")) + self.labelMediumBandwidth.setText( + _translate("DialogFilterBandwidth", "TextLabel") + ) diff --git a/src/urh/ui/ui_filter_dialog.py b/src/urh/ui/ui_filter_dialog.py index 67579252c8..ce5ab27cd6 100644 --- a/src/urh/ui/ui_filter_dialog.py +++ b/src/urh/ui/ui_filter_dialog.py @@ -25,11 +25,15 @@ def setupUi(self, FilterDialog): self.gridLayout.addWidget(self.radioButtonCustomTaps, 9, 0, 1, 1) self.buttonBox = QtWidgets.QDialogButtonBox(FilterDialog) self.buttonBox.setOrientation(QtCore.Qt.Horizontal) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setCenterButtons(True) self.buttonBox.setObjectName("buttonBox") self.gridLayout.addWidget(self.buttonBox, 15, 0, 1, 2) - spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout.addItem(spacerItem, 16, 0, 1, 1) self.line = QtWidgets.QFrame(FilterDialog) self.line.setFrameShape(QtWidgets.QFrame.HLine) @@ -91,11 +95,35 @@ def retranslateUi(self, FilterDialog): _translate = QtCore.QCoreApplication.translate FilterDialog.setWindowTitle(_translate("FilterDialog", "Configure filter")) self.radioButtonCustomTaps.setText(_translate("FilterDialog", "Custom taps:")) - self.radioButtonMovingAverage.setText(_translate("FilterDialog", "Moving average")) - self.label_4.setText(_translate("FilterDialog", "A DC correction filter will remove the DC component (mean value) of the signal and center it around zero.")) - self.lineEditCustomTaps.setToolTip(_translate("FilterDialog", "You can configure custom filter taps here either explicit using [0.1, 0.2, 0.3] or with python programming shortcuts like [0.1] * 3 + [0.2] * 4 will result in [0.1, 0.1, 0.1, 0.2, 0.2, 0.2, 0.2]
")) + self.radioButtonMovingAverage.setText( + _translate("FilterDialog", "Moving average") + ) + self.label_4.setText( + _translate( + "FilterDialog", + "A DC correction filter will remove the DC component (mean value) of the signal and center it around zero.", + ) + ) + self.lineEditCustomTaps.setToolTip( + _translate( + "FilterDialog", + 'You can configure custom filter taps here either explicit using [0.1, 0.2, 0.3] or with python programming shortcuts like [0.1] * 3 + [0.2] * 4 will result in [0.1, 0.1, 0.1, 0.2, 0.2, 0.2, 0.2]
', + ) + ) self.lineEditCustomTaps.setText(_translate("FilterDialog", "[0.1]*10")) self.label.setText(_translate("FilterDialog", "Number of taps:")) - self.label_3.setText(_translate("FilterDialog", "You can imagine taps as weighting factors applied to n samples of the signal whereby n is the number of taps. By default we use 10 taps with each tap set to 0.1 producing a moving average filter.")) - self.label_2.setText(_translate("FilterDialog", "These n weighted samples get summed up to produce the output of the filter. In DSP terms you configure the impulse response of the filter here.")) - self.radioButtonDCcorrection.setText(_translate("FilterDialog", "DC correction")) + self.label_3.setText( + _translate( + "FilterDialog", + "You can imagine taps as weighting factors applied to n samples of the signal whereby n is the number of taps. By default we use 10 taps with each tap set to 0.1 producing a moving average filter.", + ) + ) + self.label_2.setText( + _translate( + "FilterDialog", + "These n weighted samples get summed up to produce the output of the filter. In DSP terms you configure the impulse response of the filter here.", + ) + ) + self.radioButtonDCcorrection.setText( + _translate("FilterDialog", "DC correction") + ) diff --git a/src/urh/ui/ui_fuzzing.py b/src/urh/ui/ui_fuzzing.py index bab9a49cd5..2fe82559ab 100644 --- a/src/urh/ui/ui_fuzzing.py +++ b/src/urh/ui/ui_fuzzing.py @@ -15,10 +15,14 @@ def setupUi(self, FuzzingDialog): self.gridLayout_5 = QtWidgets.QGridLayout(FuzzingDialog) self.gridLayout_5.setObjectName("gridLayout_5") self.spinBoxFuzzMessage = QtWidgets.QSpinBox(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxFuzzMessage.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxFuzzMessage.sizePolicy().hasHeightForWidth() + ) self.spinBoxFuzzMessage.setSizePolicy(sizePolicy) self.spinBoxFuzzMessage.setMaximum(999999999) self.spinBoxFuzzMessage.setObjectName("spinBoxFuzzMessage") @@ -34,10 +38,14 @@ def setupUi(self, FuzzingDialog): self.btnAddFuzzingValues.setObjectName("btnAddFuzzingValues") self.gridLayout_5.addWidget(self.btnAddFuzzingValues, 9, 1, 1, 1) self.stackedWidgetLabels = QtWidgets.QStackedWidget(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.stackedWidgetLabels.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.stackedWidgetLabels.sizePolicy().hasHeightForWidth() + ) self.stackedWidgetLabels.setSizePolicy(sizePolicy) self.stackedWidgetLabels.setObjectName("stackedWidgetLabels") self.pageAddRangeLabel = QtWidgets.QWidget() @@ -47,7 +55,9 @@ def setupUi(self, FuzzingDialog): self.verticalLayout_3.setSpacing(6) self.verticalLayout_3.setObjectName("verticalLayout_3") self.lStart = QtWidgets.QLabel(self.pageAddRangeLabel) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lStart.sizePolicy().hasHeightForWidth()) @@ -55,7 +65,9 @@ def setupUi(self, FuzzingDialog): self.lStart.setObjectName("lStart") self.verticalLayout_3.addWidget(self.lStart) self.lEnd = QtWidgets.QLabel(self.pageAddRangeLabel) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lEnd.sizePolicy().hasHeightForWidth()) @@ -63,7 +75,9 @@ def setupUi(self, FuzzingDialog): self.lEnd.setObjectName("lEnd") self.verticalLayout_3.addWidget(self.lEnd) self.lStep = QtWidgets.QLabel(self.pageAddRangeLabel) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lStep.sizePolicy().hasHeightForWidth()) @@ -86,10 +100,14 @@ def setupUi(self, FuzzingDialog): self.checkBoxUpperBound.setObjectName("checkBoxUpperBound") self.verticalLayout_4.addWidget(self.checkBoxUpperBound) self.lNumberBoundaries = QtWidgets.QLabel(self.pageAddBoundariesLabel) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lNumberBoundaries.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lNumberBoundaries.sizePolicy().hasHeightForWidth() + ) self.lNumberBoundaries.setSizePolicy(sizePolicy) self.lNumberBoundaries.setObjectName("lNumberBoundaries") self.verticalLayout_4.addWidget(self.lNumberBoundaries) @@ -117,7 +135,9 @@ def setupUi(self, FuzzingDialog): self.lPreBits.setObjectName("lPreBits") self.horizontalLayout_2.addWidget(self.lPreBits) self.lFuzzedBits = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lFuzzedBits.sizePolicy().hasHeightForWidth()) @@ -130,19 +150,27 @@ def setupUi(self, FuzzingDialog): self.lFuzzedBits.setObjectName("lFuzzedBits") self.horizontalLayout_2.addWidget(self.lFuzzedBits) self.lPostBits = QtWidgets.QLabel(FuzzingDialog) - self.lPostBits.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter) + self.lPostBits.setAlignment( + QtCore.Qt.AlignRight | QtCore.Qt.AlignTrailing | QtCore.Qt.AlignVCenter + ) self.lPostBits.setObjectName("lPostBits") self.horizontalLayout_2.addWidget(self.lPostBits) - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_2.addItem(spacerItem) self.gridLayout_5.addLayout(self.horizontalLayout_2, 1, 1, 1, 1) self.verticalLayout = QtWidgets.QVBoxLayout() self.verticalLayout.setObjectName("verticalLayout") self.lFuzzedValues = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lFuzzedValues.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lFuzzedValues.sizePolicy().hasHeightForWidth() + ) self.lFuzzedValues.setSizePolicy(sizePolicy) font = QtGui.QFont() font.setBold(True) @@ -162,20 +190,30 @@ def setupUi(self, FuzzingDialog): self.btnDelRow.setObjectName("btnDelRow") self.gridLayout_4.addWidget(self.btnDelRow, 1, 1, 1, 1) self.tblFuzzingValues = FuzzingTableView(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.tblFuzzingValues.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.tblFuzzingValues.sizePolicy().hasHeightForWidth() + ) self.tblFuzzingValues.setSizePolicy(sizePolicy) self.tblFuzzingValues.setAlternatingRowColors(True) - self.tblFuzzingValues.setVerticalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) - self.tblFuzzingValues.setHorizontalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) + self.tblFuzzingValues.setVerticalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) + self.tblFuzzingValues.setHorizontalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) self.tblFuzzingValues.setShowGrid(False) self.tblFuzzingValues.setObjectName("tblFuzzingValues") self.tblFuzzingValues.horizontalHeader().setHighlightSections(False) self.tblFuzzingValues.verticalHeader().setHighlightSections(False) self.gridLayout_4.addWidget(self.tblFuzzingValues, 0, 0, 4, 1) - spacerItem1 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_4.addItem(spacerItem1, 3, 1, 1, 1) self.btnAddRow = QtWidgets.QToolButton(FuzzingDialog) icon = QtGui.QIcon.fromTheme("list-add") @@ -247,7 +285,9 @@ def setupUi(self, FuzzingDialog): self.stackedWidgetSpinboxes.addWidget(self.pageAddRandom) self.gridLayout_5.addWidget(self.stackedWidgetSpinboxes, 7, 1, 2, 1) self.lSourceBlock = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lSourceBlock.sizePolicy().hasHeightForWidth()) @@ -255,15 +295,21 @@ def setupUi(self, FuzzingDialog): self.lSourceBlock.setObjectName("lSourceBlock") self.gridLayout_5.addWidget(self.lSourceBlock, 1, 0, 1, 1) self.lFuzzingReferenceBlock = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lFuzzingReferenceBlock.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lFuzzingReferenceBlock.sizePolicy().hasHeightForWidth() + ) self.lFuzzingReferenceBlock.setSizePolicy(sizePolicy) self.lFuzzingReferenceBlock.setObjectName("lFuzzingReferenceBlock") self.gridLayout_5.addWidget(self.lFuzzingReferenceBlock, 2, 0, 1, 1) self.lFuzzingEnd = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lFuzzingEnd.sizePolicy().hasHeightForWidth()) @@ -274,48 +320,68 @@ def setupUi(self, FuzzingDialog): self.lStrategy.setObjectName("lStrategy") self.gridLayout_5.addWidget(self.lStrategy, 6, 0, 1, 1) self.comboBoxFuzzingLabel = QtWidgets.QComboBox(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.comboBoxFuzzingLabel.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.comboBoxFuzzingLabel.sizePolicy().hasHeightForWidth() + ) self.comboBoxFuzzingLabel.setSizePolicy(sizePolicy) self.comboBoxFuzzingLabel.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.comboBoxFuzzingLabel.setEditable(True) self.comboBoxFuzzingLabel.setObjectName("comboBoxFuzzingLabel") self.gridLayout_5.addWidget(self.comboBoxFuzzingLabel, 0, 1, 1, 1) self.spinBoxFuzzingEnd = QtWidgets.QSpinBox(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxFuzzingEnd.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxFuzzingEnd.sizePolicy().hasHeightForWidth() + ) self.spinBoxFuzzingEnd.setSizePolicy(sizePolicy) self.spinBoxFuzzingEnd.setMinimum(1) self.spinBoxFuzzingEnd.setMaximum(999999999) self.spinBoxFuzzingEnd.setObjectName("spinBoxFuzzingEnd") self.gridLayout_5.addWidget(self.spinBoxFuzzingEnd, 4, 1, 1, 1) self.spinBoxFuzzingStart = QtWidgets.QSpinBox(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxFuzzingStart.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxFuzzingStart.sizePolicy().hasHeightForWidth() + ) self.spinBoxFuzzingStart.setSizePolicy(sizePolicy) self.spinBoxFuzzingStart.setMinimum(1) self.spinBoxFuzzingStart.setMaximum(999999999) self.spinBoxFuzzingStart.setObjectName("spinBoxFuzzingStart") self.gridLayout_5.addWidget(self.spinBoxFuzzingStart, 3, 1, 1, 1) self.lFuzzingStart = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lFuzzingStart.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lFuzzingStart.sizePolicy().hasHeightForWidth() + ) self.lFuzzingStart.setSizePolicy(sizePolicy) self.lFuzzingStart.setObjectName("lFuzzingStart") self.gridLayout_5.addWidget(self.lFuzzingStart, 3, 0, 1, 1) self.lFuzzingLabel = QtWidgets.QLabel(FuzzingDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lFuzzingLabel.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lFuzzingLabel.sizePolicy().hasHeightForWidth() + ) self.lFuzzingLabel.setSizePolicy(sizePolicy) self.lFuzzingLabel.setObjectName("lFuzzingLabel") self.gridLayout_5.addWidget(self.lFuzzingLabel, 0, 0, 1, 1) @@ -323,23 +389,39 @@ def setupUi(self, FuzzingDialog): self.retranslateUi(FuzzingDialog) self.stackedWidgetLabels.setCurrentIndex(0) self.stackedWidgetSpinboxes.setCurrentIndex(0) - self.comboBoxStrategy.currentIndexChanged['int'].connect(self.stackedWidgetLabels.setCurrentIndex) - self.comboBoxStrategy.currentIndexChanged['int'].connect(self.stackedWidgetSpinboxes.setCurrentIndex) + self.comboBoxStrategy.currentIndexChanged["int"].connect( + self.stackedWidgetLabels.setCurrentIndex + ) + self.comboBoxStrategy.currentIndexChanged["int"].connect( + self.stackedWidgetSpinboxes.setCurrentIndex + ) def retranslateUi(self, FuzzingDialog): _translate = QtCore.QCoreApplication.translate FuzzingDialog.setWindowTitle(_translate("FuzzingDialog", "Fuzzing")) - self.comboBoxStrategy.setItemText(0, _translate("FuzzingDialog", "Add Range of Values")) - self.comboBoxStrategy.setItemText(1, _translate("FuzzingDialog", "Add Boundaries")) - self.comboBoxStrategy.setItemText(2, _translate("FuzzingDialog", "Add Random Values from Range")) - self.comboBoxStrategy.setItemText(3, _translate("FuzzingDialog", "Add De Bruijn Sequence")) - self.btnAddFuzzingValues.setText(_translate("FuzzingDialog", "Add to Fuzzed Values")) + self.comboBoxStrategy.setItemText( + 0, _translate("FuzzingDialog", "Add Range of Values") + ) + self.comboBoxStrategy.setItemText( + 1, _translate("FuzzingDialog", "Add Boundaries") + ) + self.comboBoxStrategy.setItemText( + 2, _translate("FuzzingDialog", "Add Random Values from Range") + ) + self.comboBoxStrategy.setItemText( + 3, _translate("FuzzingDialog", "Add De Bruijn Sequence") + ) + self.btnAddFuzzingValues.setText( + _translate("FuzzingDialog", "Add to Fuzzed Values") + ) self.lStart.setText(_translate("FuzzingDialog", "Start (Decimal):")) self.lEnd.setText(_translate("FuzzingDialog", "End (Decimal):")) self.lStep.setText(_translate("FuzzingDialog", "Step (Decimal):")) self.checkBoxLowerBound.setText(_translate("FuzzingDialog", "Lower Bound")) self.checkBoxUpperBound.setText(_translate("FuzzingDialog", "Upper Bound")) - self.lNumberBoundaries.setText(_translate("FuzzingDialog", "Values per Boundary:")) + self.lNumberBoundaries.setText( + _translate("FuzzingDialog", "Values per Boundary:") + ) self.lRandomMin.setText(_translate("FuzzingDialog", "Range Minimum:")) self.lRandomMax.setText(_translate("FuzzingDialog", "Range Maximum:")) self.lNumRandom.setText(_translate("FuzzingDialog", "Number Values:")) @@ -347,17 +429,37 @@ def retranslateUi(self, FuzzingDialog): self.lFuzzedBits.setText(_translate("FuzzingDialog", "1010")) self.lPostBits.setText(_translate("FuzzingDialog", "010101")) self.lFuzzedValues.setText(_translate("FuzzingDialog", "Fuzzed Values")) - self.chkBRemoveDuplicates.setText(_translate("FuzzingDialog", "Remove Duplicates")) - self.btnDelRow.setToolTip(_translate("FuzzingDialog", "Remove selected values or last value if nothing is selected.")) + self.chkBRemoveDuplicates.setText( + _translate("FuzzingDialog", "Remove Duplicates") + ) + self.btnDelRow.setToolTip( + _translate( + "FuzzingDialog", + "Remove selected values or last value if nothing is selected.", + ) + ) self.btnDelRow.setText(_translate("FuzzingDialog", "...")) self.btnAddRow.setToolTip(_translate("FuzzingDialog", "Add a new value.")) self.btnAddRow.setText(_translate("FuzzingDialog", "...")) - self.btnRepeatValues.setToolTip(_translate("FuzzingDialog", "Repeat selected values or all values if nothing is selected.")) + self.btnRepeatValues.setToolTip( + _translate( + "FuzzingDialog", + "Repeat selected values or all values if nothing is selected.", + ) + ) self.btnRepeatValues.setText(_translate("FuzzingDialog", "...")) self.lSourceBlock.setText(_translate("FuzzingDialog", "Source Message:")) - self.lFuzzingReferenceBlock.setText(_translate("FuzzingDialog", "Message to fuzz:")) - self.lFuzzingEnd.setText(_translate("FuzzingDialog", "Fuzzing Label End Index:")) + self.lFuzzingReferenceBlock.setText( + _translate("FuzzingDialog", "Message to fuzz:") + ) + self.lFuzzingEnd.setText( + _translate("FuzzingDialog", "Fuzzing Label End Index:") + ) self.lStrategy.setText(_translate("FuzzingDialog", "Strategy:")) - self.lFuzzingStart.setText(_translate("FuzzingDialog", "Fuzzing Label Start Index:")) + self.lFuzzingStart.setText( + _translate("FuzzingDialog", "Fuzzing Label Start Index:") + ) self.lFuzzingLabel.setText(_translate("FuzzingDialog", "Fuzzing Label:")) + + from urh.ui.views.FuzzingTableView import FuzzingTableView diff --git a/src/urh/ui/ui_generator.py b/src/urh/ui/ui_generator.py index ed40fd8a6e..825c8935f9 100644 --- a/src/urh/ui/ui_generator.py +++ b/src/urh/ui/ui_generator.py @@ -27,14 +27,16 @@ def setupUi(self, GeneratorTab): self.verticalLayout_2 = QtWidgets.QVBoxLayout(self.scrollAreaWidgetContents) self.verticalLayout_2.setObjectName("verticalLayout_2") self.splitter = QtWidgets.QSplitter(self.scrollAreaWidgetContents) - self.splitter.setStyleSheet("QSplitter::handle:horizontal {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -"image: url(:/icons/icons/splitter_handle_vertical.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:horizontal {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + "image: url(:/icons/icons/splitter_handle_vertical.svg);\n" + "}" + ) self.splitter.setOrientation(QtCore.Qt.Horizontal) self.splitter.setHandleWidth(6) self.splitter.setObjectName("splitter") @@ -44,7 +46,9 @@ def setupUi(self, GeneratorTab): self.verticalLayout.setContentsMargins(11, 11, 11, 11) self.verticalLayout.setObjectName("verticalLayout") self.tabWidget = QtWidgets.QTabWidget(self.layoutWidget_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.tabWidget.sizePolicy().hasHeightForWidth()) @@ -59,10 +63,14 @@ def setupUi(self, GeneratorTab): self.verticalLayout_4.setContentsMargins(0, 0, 0, 0) self.verticalLayout_4.setObjectName("verticalLayout_4") self.treeProtocols = GeneratorTreeView(self.tab_proto) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.treeProtocols.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.treeProtocols.sizePolicy().hasHeightForWidth() + ) self.treeProtocols.setSizePolicy(sizePolicy) self.treeProtocols.setObjectName("treeProtocols") self.treeProtocols.header().setDefaultSectionSize(57) @@ -74,12 +82,17 @@ def setupUi(self, GeneratorTab): self.gridLayout_5.setContentsMargins(0, 0, 0, 0) self.gridLayout_5.setObjectName("gridLayout_5") self.lWPauses = GeneratorListWidget(self.tab_pauses) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lWPauses.sizePolicy().hasHeightForWidth()) self.lWPauses.setSizePolicy(sizePolicy) - self.lWPauses.setEditTriggers(QtWidgets.QAbstractItemView.DoubleClicked|QtWidgets.QAbstractItemView.EditKeyPressed) + self.lWPauses.setEditTriggers( + QtWidgets.QAbstractItemView.DoubleClicked + | QtWidgets.QAbstractItemView.EditKeyPressed + ) self.lWPauses.setProperty("showDropIndicator", False) self.lWPauses.setDragDropMode(QtWidgets.QAbstractItemView.NoDragDrop) self.lWPauses.setObjectName("lWPauses") @@ -92,12 +105,18 @@ def setupUi(self, GeneratorTab): self.verticalLayout_9.setSpacing(6) self.verticalLayout_9.setObjectName("verticalLayout_9") self.listViewProtoLabels = GeneratorListView(self.tab_fuzzing) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.listViewProtoLabels.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.listViewProtoLabels.sizePolicy().hasHeightForWidth() + ) self.listViewProtoLabels.setSizePolicy(sizePolicy) - self.listViewProtoLabels.setEditTriggers(QtWidgets.QAbstractItemView.EditKeyPressed) + self.listViewProtoLabels.setEditTriggers( + QtWidgets.QAbstractItemView.EditKeyPressed + ) self.listViewProtoLabels.setObjectName("listViewProtoLabels") self.verticalLayout_9.addWidget(self.listViewProtoLabels) self.groupBox = QtWidgets.QGroupBox(self.tab_fuzzing) @@ -105,10 +124,14 @@ def setupUi(self, GeneratorTab): self.horizontalLayout_6 = QtWidgets.QHBoxLayout(self.groupBox) self.horizontalLayout_6.setObjectName("horizontalLayout_6") self.stackedWidgetFuzzing = QtWidgets.QStackedWidget(self.groupBox) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.stackedWidgetFuzzing.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.stackedWidgetFuzzing.sizePolicy().hasHeightForWidth() + ) self.stackedWidgetFuzzing.setSizePolicy(sizePolicy) self.stackedWidgetFuzzing.setObjectName("stackedWidgetFuzzing") self.pageFuzzingUI = QtWidgets.QWidget() @@ -177,10 +200,14 @@ def setupUi(self, GeneratorTab): self.lEncoding.setObjectName("lEncoding") self.modulationLayout_2.addWidget(self.lEncoding, 0, 0, 1, 1) self.lEncodingValue = QtWidgets.QLabel(self.layoutWidget_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lEncodingValue.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lEncodingValue.sizePolicy().hasHeightForWidth() + ) self.lEncodingValue.setSizePolicy(sizePolicy) self.lEncodingValue.setObjectName("lEncodingValue") self.modulationLayout_2.addWidget(self.lEncodingValue, 0, 1, 1, 1) @@ -216,10 +243,14 @@ def setupUi(self, GeneratorTab): self.cBoxModulations.addItem("") self.gridLayout_6.addWidget(self.cBoxModulations, 2, 1, 1, 1) self.prBarGeneration = QtWidgets.QProgressBar(self.layoutWidget_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.prBarGeneration.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.prBarGeneration.sizePolicy().hasHeightForWidth() + ) self.prBarGeneration.setSizePolicy(sizePolicy) self.prBarGeneration.setProperty("value", 0) self.prBarGeneration.setObjectName("prBarGeneration") @@ -257,8 +288,12 @@ def setupUi(self, GeneratorTab): self.tableMessages.setDefaultDropAction(QtCore.Qt.CopyAction) self.tableMessages.setAlternatingRowColors(True) self.tableMessages.setSelectionBehavior(QtWidgets.QAbstractItemView.SelectItems) - self.tableMessages.setVerticalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) - self.tableMessages.setHorizontalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) + self.tableMessages.setVerticalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) + self.tableMessages.setHorizontalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) self.tableMessages.setShowGrid(False) self.tableMessages.setObjectName("tableMessages") self.tableMessages.horizontalHeader().setHighlightSections(False) @@ -270,7 +305,9 @@ def setupUi(self, GeneratorTab): self.btnNetworkSDRSend.setCheckable(True) self.btnNetworkSDRSend.setObjectName("btnNetworkSDRSend") self.gridLayout_2.addWidget(self.btnNetworkSDRSend, 2, 0, 1, 1) - spacerItem = QtWidgets.QSpacerItem(38, 22, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 38, 22, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_2.addItem(spacerItem, 2, 4, 1, 2) self.cbViewType = QtWidgets.QComboBox(self.layoutWidget) self.cbViewType.setObjectName("cbViewType") @@ -279,7 +316,9 @@ def setupUi(self, GeneratorTab): self.cbViewType.addItem("") self.gridLayout_2.addWidget(self.cbViewType, 2, 7, 1, 1) self.lViewType = QtWidgets.QLabel(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lViewType.sizePolicy().hasHeightForWidth()) @@ -295,10 +334,14 @@ def setupUi(self, GeneratorTab): self.horizontalLayout.setContentsMargins(-1, 0, -1, 0) self.horizontalLayout.setObjectName("horizontalLayout") self.labelGeneratedData = QtWidgets.QLabel(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.labelGeneratedData.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.labelGeneratedData.sizePolicy().hasHeightForWidth() + ) self.labelGeneratedData.setSizePolicy(sizePolicy) font = QtGui.QFont() font.setBold(True) @@ -308,7 +351,9 @@ def setupUi(self, GeneratorTab): self.labelGeneratedData.setObjectName("labelGeneratedData") self.horizontalLayout.addWidget(self.labelGeneratedData) self.btnSave = QtWidgets.QToolButton(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.btnSave.sizePolicy().hasHeightForWidth()) @@ -318,7 +363,9 @@ def setupUi(self, GeneratorTab): self.btnSave.setObjectName("btnSave") self.horizontalLayout.addWidget(self.btnSave) self.btnOpen = QtWidgets.QToolButton(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.btnOpen.sizePolicy().hasHeightForWidth()) @@ -347,20 +394,56 @@ def setupUi(self, GeneratorTab): def retranslateUi(self, GeneratorTab): _translate = QtCore.QCoreApplication.translate GeneratorTab.setWindowTitle(_translate("GeneratorTab", "Form")) - self.treeProtocols.setToolTip(_translate("GeneratorTab", "Drag&Drop Protocols to the table on the right to fill the generation table.
")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_proto), _translate("GeneratorTab", "Protocols")) - self.lWPauses.setToolTip(_translate("GeneratorTab", "The pauses will be added automatically when you drag a protocol from the tree above to the table on the right.
You can see the position of each pause by selecting it. There will be drawn a line in the table indicating the position of the pause.
Use context menu or double click to edit a pauses\' length.
")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_pauses), _translate("GeneratorTab", "Pauses")) - self.groupBox.setTitle(_translate("GeneratorTab", "Add fuzzing values to generated data")) + self.treeProtocols.setToolTip( + _translate( + "GeneratorTab", + "Drag&Drop Protocols to the table on the right to fill the generation table.
", + ) + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_proto), + _translate("GeneratorTab", "Protocols"), + ) + self.lWPauses.setToolTip( + _translate( + "GeneratorTab", + 'The pauses will be added automatically when you drag a protocol from the tree above to the table on the right.
You can see the position of each pause by selecting it. There will be drawn a line in the table indicating the position of the pause.
Use context menu or double click to edit a pauses\' length.
', + ) + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_pauses), + _translate("GeneratorTab", "Pauses"), + ) + self.groupBox.setTitle( + _translate("GeneratorTab", "Add fuzzing values to generated data") + ) self.btnFuzz.setText(_translate("GeneratorTab", "Fuzz")) - self.rBSuccessive.setToolTip(_translate("GeneratorTab", "For multiple labels per message the fuzzed values are inserted one-by-one.
")) + self.rBSuccessive.setToolTip( + _translate( + "GeneratorTab", + 'For multiple labels per message the fuzzed values are inserted one-by-one.
', + ) + ) self.rBSuccessive.setText(_translate("GeneratorTab", "S&uccessive")) - self.rbConcurrent.setToolTip(_translate("GeneratorTab", "For multiple labels per message the labels are fuzzed at the same time.
")) + self.rbConcurrent.setToolTip( + _translate( + "GeneratorTab", + 'For multiple labels per message the labels are fuzzed at the same time.
', + ) + ) self.rbConcurrent.setText(_translate("GeneratorTab", "&Concurrent")) - self.rBExhaustive.setToolTip(_translate("GeneratorTab", "For multiple labels per message the fuzzed values are inserted in all possible combinations.
")) + self.rBExhaustive.setToolTip( + _translate( + "GeneratorTab", + 'For multiple labels per message the fuzzed values are inserted in all possible combinations.
', + ) + ) self.rBExhaustive.setText(_translate("GeneratorTab", "E&xhaustive")) self.progressBarFuzzing.setFormat(_translate("GeneratorTab", "%v/%m")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_fuzzing), _translate("GeneratorTab", "Fuzzing")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_fuzzing), + _translate("GeneratorTab", "Fuzzing"), + ) self.lCarrierFreqValue.setText(_translate("GeneratorTab", "TextLabel")) self.lModType.setText(_translate("GeneratorTab", "Modulation Type:")) self.lModTypeValue.setText(_translate("GeneratorTab", "TextLabel")) @@ -382,24 +465,48 @@ def retranslateUi(self, GeneratorTab): self.btnSend.setText(_translate("GeneratorTab", "Send data...")) self.btnEditModulation.setText(_translate("GeneratorTab", "Edit ...")) self.lModulation.setText(_translate("GeneratorTab", "Modulation:")) - self.btnGenerate.setToolTip(_translate("GeneratorTab", "Generate the complex file of the modulated signal, after tuning all parameters above.")) + self.btnGenerate.setToolTip( + _translate( + "GeneratorTab", + "Generate the complex file of the modulated signal, after tuning all parameters above.", + ) + ) self.btnGenerate.setText(_translate("GeneratorTab", "Generate file...")) - self.btnNetworkSDRSend.setToolTip(_translate("GeneratorTab", "Send encoded data to your external application via TCP.
")) + self.btnNetworkSDRSend.setToolTip( + _translate( + "GeneratorTab", + 'Send encoded data to your external application via TCP.
', + ) + ) self.btnNetworkSDRSend.setText(_translate("GeneratorTab", "Send via Network")) self.cbViewType.setItemText(0, _translate("GeneratorTab", "Bit")) self.cbViewType.setItemText(1, _translate("GeneratorTab", "Hex")) self.cbViewType.setItemText(2, _translate("GeneratorTab", "ASCII")) self.lViewType.setText(_translate("GeneratorTab", "Viewtype:")) - self.btnRfCatSend.setToolTip(_translate("GeneratorTab", "Send encoded data via RfCat.
Hit again for stopping the sending process. Note that you can set the number of repetitions (from 1 to infinite) in:
Edit->Options->Device->\'Device sending repetitions\'
")) + self.btnRfCatSend.setToolTip( + _translate( + "GeneratorTab", + 'Send encoded data via RfCat.
Hit again for stopping the sending process. Note that you can set the number of repetitions (from 1 to infinite) in:
Edit->Options->Device->\'Device sending repetitions\'
', + ) + ) self.btnRfCatSend.setText(_translate("GeneratorTab", "Send via RfCat")) self.labelGeneratedData.setText(_translate("GeneratorTab", "Generated Data")) - self.btnSave.setToolTip(_translate("GeneratorTab", "Save current fuzz profile.")) + self.btnSave.setToolTip( + _translate("GeneratorTab", "Save current fuzz profile.") + ) self.btnSave.setText(_translate("GeneratorTab", "...")) self.btnOpen.setToolTip(_translate("GeneratorTab", "Load a fuzz profile.")) self.btnOpen.setText(_translate("GeneratorTab", "...")) - self.lEstimatedTime.setToolTip(_translate("GeneratorTab", "The estimated average time is based on the average number of bits per message and average sample rate, you set for the modulations.
")) + self.lEstimatedTime.setToolTip( + _translate( + "GeneratorTab", + "The estimated average time is based on the average number of bits per message and average sample rate, you set for the modulations.
", + ) + ) self.lEstimatedTime.setText(_translate("GeneratorTab", "Estimated Time: ")) self.btnFZSave.setText(_translate("GeneratorTab", "Save as FlipperZero SubGHz")) + + from urh.ui.ElidedLabel import ElidedLabel from urh.ui.GeneratorListWidget import GeneratorListWidget from urh.ui.views.GeneratorListView import GeneratorListView diff --git a/src/urh/ui/ui_main.py b/src/urh/ui/ui_main.py index 3defb740b8..4634c24e3e 100644 --- a/src/urh/ui/ui_main.py +++ b/src/urh/ui/ui_main.py @@ -14,7 +14,11 @@ def setupUi(self, MainWindow): MainWindow.setObjectName("MainWindow") MainWindow.resize(798, 469) icon = QtGui.QIcon() - icon.addPixmap(QtGui.QPixmap(":/icons/icons/appicon.png"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon.addPixmap( + QtGui.QPixmap(":/icons/icons/appicon.png"), + QtGui.QIcon.Normal, + QtGui.QIcon.Off, + ) MainWindow.setWindowIcon(icon) MainWindow.setTabShape(QtWidgets.QTabWidget.Rounded) MainWindow.setDockNestingEnabled(False) @@ -23,14 +27,16 @@ def setupUi(self, MainWindow): self.verticalLayout_4 = QtWidgets.QVBoxLayout(self.centralwidget) self.verticalLayout_4.setObjectName("verticalLayout_4") self.splitter = QtWidgets.QSplitter(self.centralwidget) - self.splitter.setStyleSheet("QSplitter::handle:horizontal {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -"image: url(:/icons/icons/splitter_handle_vertical.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:horizontal {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + "image: url(:/icons/icons/splitter_handle_vertical.svg);\n" + "}" + ) self.splitter.setOrientation(QtCore.Qt.Horizontal) self.splitter.setHandleWidth(6) self.splitter.setObjectName("splitter") @@ -43,17 +49,23 @@ def setupUi(self, MainWindow): self.horizontalLayout_3 = QtWidgets.QHBoxLayout() self.horizontalLayout_3.setObjectName("horizontalLayout_3") self.lnEdtTreeFilter = QtWidgets.QLineEdit(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lnEdtTreeFilter.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lnEdtTreeFilter.sizePolicy().hasHeightForWidth() + ) self.lnEdtTreeFilter.setSizePolicy(sizePolicy) self.lnEdtTreeFilter.setAcceptDrops(False) self.lnEdtTreeFilter.setInputMethodHints(QtCore.Qt.ImhDialableCharactersOnly) self.lnEdtTreeFilter.setClearButtonEnabled(True) self.lnEdtTreeFilter.setObjectName("lnEdtTreeFilter") self.horizontalLayout_3.addWidget(self.lnEdtTreeFilter) - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_3.addItem(spacerItem) self.btnFileTreeGoUp = QtWidgets.QToolButton(self.layoutWidget) icon = QtGui.QIcon.fromTheme("go-up") @@ -62,7 +74,9 @@ def setupUi(self, MainWindow): self.horizontalLayout_3.addWidget(self.btnFileTreeGoUp) self.verticalLayout_3.addLayout(self.horizontalLayout_3) self.fileTree = DirectoryTreeView(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(10) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.fileTree.sizePolicy().hasHeightForWidth()) @@ -79,10 +93,14 @@ def setupUi(self, MainWindow): self.fileTree.header().setStretchLastSection(False) self.verticalLayout_3.addWidget(self.fileTree) self.tabWidget_Project = QtWidgets.QTabWidget(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.tabWidget_Project.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.tabWidget_Project.sizePolicy().hasHeightForWidth() + ) self.tabWidget_Project.setSizePolicy(sizePolicy) self.tabWidget_Project.setStyleSheet("QTabWidget::pane { border: 0; }") self.tabWidget_Project.setObjectName("tabWidget_Project") @@ -107,7 +125,9 @@ def setupUi(self, MainWindow): self.tabWidget_Project.addTab(self.tabDescription, "") self.verticalLayout_3.addWidget(self.tabWidget_Project) self.tabWidget = QtWidgets.QTabWidget(self.splitter) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(1) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.tabWidget.sizePolicy().hasHeightForWidth()) @@ -194,7 +214,9 @@ def setupUi(self, MainWindow): self.actionShow_Confirm_Close_Dialog = QtWidgets.QAction(MainWindow) self.actionShow_Confirm_Close_Dialog.setCheckable(True) self.actionShow_Confirm_Close_Dialog.setChecked(False) - self.actionShow_Confirm_Close_Dialog.setObjectName("actionShow_Confirm_Close_Dialog") + self.actionShow_Confirm_Close_Dialog.setObjectName( + "actionShow_Confirm_Close_Dialog" + ) self.actionTest = QtWidgets.QAction(MainWindow) self.actionTest.setObjectName("actionTest") self.actionHold_Shift_to_Drag = QtWidgets.QAction(MainWindow) @@ -232,7 +254,9 @@ def setupUi(self, MainWindow): self.actionSeperate_Protocols_in_Compare_Frame = QtWidgets.QAction(MainWindow) self.actionSeperate_Protocols_in_Compare_Frame.setCheckable(True) self.actionSeperate_Protocols_in_Compare_Frame.setChecked(True) - self.actionSeperate_Protocols_in_Compare_Frame.setObjectName("actionSeperate_Protocols_in_Compare_Frame") + self.actionSeperate_Protocols_in_Compare_Frame.setObjectName( + "actionSeperate_Protocols_in_Compare_Frame" + ) self.actionOpenArchive = QtWidgets.QAction(MainWindow) self.actionOpenArchive.setObjectName("actionOpenArchive") self.actionOpen = QtWidgets.QAction(MainWindow) @@ -247,7 +271,9 @@ def setupUi(self, MainWindow): self.actionShow_only_Compare_Frame = QtWidgets.QAction(MainWindow) self.actionShow_only_Compare_Frame.setCheckable(True) self.actionShow_only_Compare_Frame.setChecked(True) - self.actionShow_only_Compare_Frame.setObjectName("actionShow_only_Compare_Frame") + self.actionShow_only_Compare_Frame.setObjectName( + "actionShow_only_Compare_Frame" + ) self.actionConfigurePlugins = QtWidgets.QAction(MainWindow) self.actionConfigurePlugins.setIconVisibleInMenu(True) self.actionConfigurePlugins.setObjectName("actionConfigurePlugins") @@ -255,10 +281,16 @@ def setupUi(self, MainWindow): self.actionSort_Frames_by_Name.setObjectName("actionSort_Frames_by_Name") self.actionConvert_Folder_to_Project = QtWidgets.QAction(MainWindow) self.actionConvert_Folder_to_Project.setIconVisibleInMenu(True) - self.actionConvert_Folder_to_Project.setObjectName("actionConvert_Folder_to_Project") + self.actionConvert_Folder_to_Project.setObjectName( + "actionConvert_Folder_to_Project" + ) self.actionDecoding = QtWidgets.QAction(MainWindow) icon1 = QtGui.QIcon() - icon1.addPixmap(QtGui.QPixmap(":/icons/icons/decoding.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon1.addPixmap( + QtGui.QPixmap(":/icons/icons/decoding.svg"), + QtGui.QIcon.Normal, + QtGui.QIcon.Off, + ) self.actionDecoding.setIcon(icon1) self.actionDecoding.setObjectName("actionDecoding") self.actionRecord = QtWidgets.QAction(MainWindow) @@ -268,7 +300,11 @@ def setupUi(self, MainWindow): self.actionRecord.setObjectName("actionRecord") self.actionSpectrum_Analyzer = QtWidgets.QAction(MainWindow) icon2 = QtGui.QIcon() - icon2.addPixmap(QtGui.QPixmap(":/icons/icons/spectrum.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon2.addPixmap( + QtGui.QPixmap(":/icons/icons/spectrum.svg"), + QtGui.QIcon.Normal, + QtGui.QIcon.Off, + ) self.actionSpectrum_Analyzer.setIcon(icon2) self.actionSpectrum_Analyzer.setIconVisibleInMenu(True) self.actionSpectrum_Analyzer.setObjectName("actionSpectrum_Analyzer") @@ -283,7 +319,11 @@ def setupUi(self, MainWindow): self.actionNew_Project.setObjectName("actionNew_Project") self.actionSniff_protocol = QtWidgets.QAction(MainWindow) icon3 = QtGui.QIcon() - icon3.addPixmap(QtGui.QPixmap(":/icons/icons/sniffer.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon3.addPixmap( + QtGui.QPixmap(":/icons/icons/sniffer.svg"), + QtGui.QIcon.Normal, + QtGui.QIcon.Off, + ) self.actionSniff_protocol.setIcon(icon3) self.actionSniff_protocol.setObjectName("actionSniff_protocol") self.actionProject_settings = QtWidgets.QAction(MainWindow) @@ -317,11 +357,15 @@ def setupUi(self, MainWindow): self.actionAuto_detect_new_signals = QtWidgets.QAction(MainWindow) self.actionAuto_detect_new_signals.setCheckable(True) self.actionAuto_detect_new_signals.setChecked(True) - self.actionAuto_detect_new_signals.setObjectName("actionAuto_detect_new_signals") + self.actionAuto_detect_new_signals.setObjectName( + "actionAuto_detect_new_signals" + ) self.actionAutomaticNoiseThreshold = QtWidgets.QAction(MainWindow) self.actionAutomaticNoiseThreshold.setCheckable(True) self.actionAutomaticNoiseThreshold.setChecked(True) - self.actionAutomaticNoiseThreshold.setObjectName("actionAutomaticNoiseThreshold") + self.actionAutomaticNoiseThreshold.setObjectName( + "actionAutomaticNoiseThreshold" + ) self.action1NoiseThreshold = QtWidgets.QAction(MainWindow) self.action1NoiseThreshold.setCheckable(True) self.action1NoiseThreshold.setObjectName("action1NoiseThreshold") @@ -380,17 +424,42 @@ def retranslateUi(self, MainWindow): MainWindow.setWindowTitle(_translate("MainWindow", "Universal Radio Hacker")) self.lnEdtTreeFilter.setPlaceholderText(_translate("MainWindow", "Filter")) self.btnFileTreeGoUp.setText(_translate("MainWindow", "...")) - self.tabWidget_Project.setTabText(self.tabWidget_Project.indexOf(self.tabParticipants), _translate("MainWindow", "Participants")) - self.tabWidget_Project.setTabText(self.tabWidget_Project.indexOf(self.tabDescription), _translate("MainWindow", "Description")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_interpretation), _translate("MainWindow", "Interpretation")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_protocol), _translate("MainWindow", "Analysis")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_generator), _translate("MainWindow", "Generator")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_simulator), _translate("MainWindow", "Simulator")) - self.labelNonProjectMode.setText(_translate("MainWindow", "Warning: You are running URH in non project mode. All your settings will be lost after closing the program. If you want to keep your settings create a project via File -> New Project. Don\'t show this hint
")) + self.tabWidget_Project.setTabText( + self.tabWidget_Project.indexOf(self.tabParticipants), + _translate("MainWindow", "Participants"), + ) + self.tabWidget_Project.setTabText( + self.tabWidget_Project.indexOf(self.tabDescription), + _translate("MainWindow", "Description"), + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_interpretation), + _translate("MainWindow", "Interpretation"), + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_protocol), + _translate("MainWindow", "Analysis"), + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_generator), + _translate("MainWindow", "Generator"), + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_simulator), + _translate("MainWindow", "Simulator"), + ) + self.labelNonProjectMode.setText( + _translate( + "MainWindow", + 'Warning: You are running URH in non project mode. All your settings will be lost after closing the program. If you want to keep your settings create a project via File -> New Project. Don\'t show this hint
', + ) + ) self.menuFile.setTitle(_translate("MainWindow", "Fi&le")) self.menuImport.setTitle(_translate("MainWindow", "Import")) self.menuEdit.setTitle(_translate("MainWindow", "Edi&t")) - self.menuDefault_noise_threshold.setTitle(_translate("MainWindow", "Default noise threshold")) + self.menuDefault_noise_threshold.setTitle( + _translate("MainWindow", "Default noise threshold") + ) self.menuHelp.setTitle(_translate("MainWindow", "Hel&p")) self.actionFSK.setText(_translate("MainWindow", "Undo")) self.actionOOK.setText(_translate("MainWindow", "Redo")) @@ -399,43 +468,75 @@ def retranslateUi(self, MainWindow): self.actionAuto_Fit_Y.setText(_translate("MainWindow", "&Auto Fit Y")) self.actionUndo.setText(_translate("MainWindow", "&Undo")) self.actionRedo.setText(_translate("MainWindow", "&Redo")) - self.actionShow_Confirm_Close_Dialog.setText(_translate("MainWindow", "&Show Confirm Close Dialog")) + self.actionShow_Confirm_Close_Dialog.setText( + _translate("MainWindow", "&Show Confirm Close Dialog") + ) self.actionTest.setText(_translate("MainWindow", "test")) - self.actionHold_Shift_to_Drag.setText(_translate("MainWindow", "&Hold Shift to Drag")) + self.actionHold_Shift_to_Drag.setText( + _translate("MainWindow", "&Hold Shift to Drag") + ) self.actionDocumentation.setText(_translate("MainWindow", "&Documentation")) - self.actionAbout_AutomaticHacker.setText(_translate("MainWindow", "&About Universal Radio Hacker...")) + self.actionAbout_AutomaticHacker.setText( + _translate("MainWindow", "&About Universal Radio Hacker...") + ) self.actionOpenSignal.setText(_translate("MainWindow", "&Signal")) self.actionOpenProtocol.setText(_translate("MainWindow", "&Protocol")) - self.actionShow_Compare_Frame.setText(_translate("MainWindow", "Show &Compare Frame")) + self.actionShow_Compare_Frame.setText( + _translate("MainWindow", "Show &Compare Frame") + ) self.actionCloseAllFiles.setText(_translate("MainWindow", "&Close all files")) self.actionSaveAllSignals.setText(_translate("MainWindow", "&Save all signals")) - self.actionSeperate_Protocols_in_Compare_Frame.setText(_translate("MainWindow", "Separate &Protocols in Compare Frame")) + self.actionSeperate_Protocols_in_Compare_Frame.setText( + _translate("MainWindow", "Separate &Protocols in Compare Frame") + ) self.actionOpenArchive.setText(_translate("MainWindow", "&Archive")) self.actionOpen.setText(_translate("MainWindow", "&Open...")) self.actionOpen_Folder.setText(_translate("MainWindow", "Open &Folder..")) - self.actionShow_only_Compare_Frame.setText(_translate("MainWindow", "Show Compare Frame only")) + self.actionShow_only_Compare_Frame.setText( + _translate("MainWindow", "Show Compare Frame only") + ) self.actionConfigurePlugins.setText(_translate("MainWindow", "Configure...")) - self.actionSort_Frames_by_Name.setText(_translate("MainWindow", "Sort &Frames by Name")) - self.actionConvert_Folder_to_Project.setText(_translate("MainWindow", "Conv&ert Folder to Project")) + self.actionSort_Frames_by_Name.setText( + _translate("MainWindow", "Sort &Frames by Name") + ) + self.actionConvert_Folder_to_Project.setText( + _translate("MainWindow", "Conv&ert Folder to Project") + ) self.actionDecoding.setText(_translate("MainWindow", "&Decoding...")) self.actionRecord.setText(_translate("MainWindow", "&Record signal...")) - self.actionSpectrum_Analyzer.setText(_translate("MainWindow", "Spectrum &Analyzer...")) + self.actionSpectrum_Analyzer.setText( + _translate("MainWindow", "Spectrum &Analyzer...") + ) self.actionOptions.setText(_translate("MainWindow", "&Options...")) self.actionNew_Project.setText(_translate("MainWindow", "&New Project..")) - self.actionSniff_protocol.setText(_translate("MainWindow", "Sn&iff protocol...")) - self.actionProject_settings.setText(_translate("MainWindow", "&Project settings...")) + self.actionSniff_protocol.setText( + _translate("MainWindow", "Sn&iff protocol...") + ) + self.actionProject_settings.setText( + _translate("MainWindow", "&Project settings...") + ) self.actionSave_project.setText(_translate("MainWindow", "Sa&ve project")) self.actionFullscreen_mode.setText(_translate("MainWindow", "&Fullscreen mode")) self.actionOpen_directory.setText(_translate("MainWindow", "Open &folder...")) self.actionAbout_Qt.setText(_translate("MainWindow", "About &Qt")) self.actionShowFileTree.setText(_translate("MainWindow", "&Show file tree")) - self.actionSamples_from_csv.setText(_translate("MainWindow", "IQ samples from csv")) + self.actionSamples_from_csv.setText( + _translate("MainWindow", "IQ samples from csv") + ) self.actionClose_project.setText(_translate("MainWindow", "Close project")) - self.actionAuto_detect_new_signals.setText(_translate("MainWindow", "Auto detect signals on loading")) - self.actionAutomaticNoiseThreshold.setText(_translate("MainWindow", "Automatic")) + self.actionAuto_detect_new_signals.setText( + _translate("MainWindow", "Auto detect signals on loading") + ) + self.actionAutomaticNoiseThreshold.setText( + _translate("MainWindow", "Automatic") + ) self.action1NoiseThreshold.setText(_translate("MainWindow", "1%")) self.action5NoiseThreshold.setText(_translate("MainWindow", "5%")) self.action10NoiseThreshold.setText(_translate("MainWindow", "10%")) - self.action100NoiseThreshold.setText(_translate("MainWindow", "100% (disables demodulation)")) + self.action100NoiseThreshold.setText( + _translate("MainWindow", "100% (disables demodulation)") + ) + + from urh.ui.views.DirectoryTreeView import DirectoryTreeView from . import urh_rc diff --git a/src/urh/ui/ui_messagetype_options.py b/src/urh/ui/ui_messagetype_options.py index 47ea4b71d6..c35f880073 100644 --- a/src/urh/ui/ui_messagetype_options.py +++ b/src/urh/ui/ui_messagetype_options.py @@ -35,7 +35,9 @@ def setupUi(self, DialogMessageType): self.rbAssignAutomatically = QtWidgets.QRadioButton(DialogMessageType) self.rbAssignAutomatically.setObjectName("rbAssignAutomatically") self.gridLayout.addWidget(self.rbAssignAutomatically, 0, 1, 1, 1) - spacerItem = QtWidgets.QSpacerItem(20, 145, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 145, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout.addItem(spacerItem, 4, 2, 1, 1) self.btnAddRule = QtWidgets.QToolButton(DialogMessageType) icon = QtGui.QIcon.fromTheme("list-add") @@ -43,7 +45,9 @@ def setupUi(self, DialogMessageType): self.btnAddRule.setObjectName("btnAddRule") self.gridLayout.addWidget(self.btnAddRule, 2, 2, 1, 1) self.buttonBox = QtWidgets.QDialogButtonBox(DialogMessageType) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setObjectName("buttonBox") self.gridLayout.addWidget(self.buttonBox, 5, 0, 1, 2) @@ -52,12 +56,22 @@ def setupUi(self, DialogMessageType): def retranslateUi(self, DialogMessageType): _translate = QtCore.QCoreApplication.translate DialogMessageType.setWindowTitle(_translate("DialogMessageType", "Dialog")) - self.cbRulesetMode.setItemText(0, _translate("DialogMessageType", "All rules must apply (AND)")) - self.cbRulesetMode.setItemText(1, _translate("DialogMessageType", "At least one rule must apply (OR)")) - self.cbRulesetMode.setItemText(2, _translate("DialogMessageType", "No rule must apply (NOR)")) + self.cbRulesetMode.setItemText( + 0, _translate("DialogMessageType", "All rules must apply (AND)") + ) + self.cbRulesetMode.setItemText( + 1, _translate("DialogMessageType", "At least one rule must apply (OR)") + ) + self.cbRulesetMode.setItemText( + 2, _translate("DialogMessageType", "No rule must apply (NOR)") + ) self.btnRemoveRule.setToolTip(_translate("DialogMessageType", "Remove ruleset")) self.btnRemoveRule.setText(_translate("DialogMessageType", "...")) - self.rbAssignManually.setText(_translate("DialogMessageType", "Assi&gn manually")) - self.rbAssignAutomatically.setText(_translate("DialogMessageType", "Assign a&utomatically")) + self.rbAssignManually.setText( + _translate("DialogMessageType", "Assi&gn manually") + ) + self.rbAssignAutomatically.setText( + _translate("DialogMessageType", "Assign a&utomatically") + ) self.btnAddRule.setToolTip(_translate("DialogMessageType", "Add ruleset")) self.btnAddRule.setText(_translate("DialogMessageType", "...")) diff --git a/src/urh/ui/ui_modulation.py b/src/urh/ui/ui_modulation.py index d2ed49469f..eb9f329fc3 100644 --- a/src/urh/ui/ui_modulation.py +++ b/src/urh/ui/ui_modulation.py @@ -13,7 +13,11 @@ def setupUi(self, DialogModulation): DialogModulation.setObjectName("DialogModulation") DialogModulation.resize(977, 1041) icon = QtGui.QIcon() - icon.addPixmap(QtGui.QPixmap(":/icons/icons/modulation.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon.addPixmap( + QtGui.QPixmap(":/icons/icons/modulation.svg"), + QtGui.QIcon.Normal, + QtGui.QIcon.Off, + ) DialogModulation.setWindowIcon(icon) self.verticalLayout = QtWidgets.QVBoxLayout(DialogModulation) self.verticalLayout.setObjectName("verticalLayout") @@ -21,8 +25,12 @@ def setupUi(self, DialogModulation): self.gridLayout_5.setObjectName("gridLayout_5") self.comboBoxCustomModulations = QtWidgets.QComboBox(DialogModulation) self.comboBoxCustomModulations.setEditable(True) - self.comboBoxCustomModulations.setInsertPolicy(QtWidgets.QComboBox.InsertAtCurrent) - self.comboBoxCustomModulations.setSizeAdjustPolicy(QtWidgets.QComboBox.AdjustToContents) + self.comboBoxCustomModulations.setInsertPolicy( + QtWidgets.QComboBox.InsertAtCurrent + ) + self.comboBoxCustomModulations.setSizeAdjustPolicy( + QtWidgets.QComboBox.AdjustToContents + ) self.comboBoxCustomModulations.setObjectName("comboBoxCustomModulations") self.comboBoxCustomModulations.addItem("") self.gridLayout_5.addWidget(self.comboBoxCustomModulations, 0, 0, 1, 1) @@ -54,7 +62,9 @@ def setupUi(self, DialogModulation): self.label_5.setObjectName("label_5") self.gridLayout_7.addWidget(self.label_5, 2, 0, 1, 1) self.lEqual = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lEqual.sizePolicy().hasHeightForWidth()) @@ -73,7 +83,9 @@ def setupUi(self, DialogModulation): self.label_6.setFont(font) self.label_6.setObjectName("label_6") self.gridLayout_7.addWidget(self.label_6, 4, 0, 1, 1) - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_7.addItem(spacerItem, 8, 1, 1, 1) self.label_7 = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) font = QtGui.QFont() @@ -82,9 +94,13 @@ def setupUi(self, DialogModulation): self.label_7.setFont(font) self.label_7.setObjectName("label_7") self.gridLayout_7.addWidget(self.label_7, 8, 0, 1, 1) - spacerItem1 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem1 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_7.addItem(spacerItem1, 2, 3, 1, 1) - spacerItem2 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem2 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_7.addItem(spacerItem2, 4, 1, 1, 1) self.label_4 = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) font = QtGui.QFont() @@ -93,10 +109,14 @@ def setupUi(self, DialogModulation): self.label_4.setFont(font) self.label_4.setObjectName("label_4") self.gridLayout_7.addWidget(self.label_4, 0, 0, 1, 1) - self.gVOriginalSignal = ZoomAndDropableGraphicView(self.scrollAreaWidgetContents_2) + self.gVOriginalSignal = ZoomAndDropableGraphicView( + self.scrollAreaWidgetContents_2 + ) self.gVOriginalSignal.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) self.gVOriginalSignal.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOn) - self.gVOriginalSignal.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.HighQualityAntialiasing) + self.gVOriginalSignal.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.HighQualityAntialiasing + ) self.gVOriginalSignal.setDragMode(QtWidgets.QGraphicsView.NoDrag) self.gVOriginalSignal.setObjectName("gVOriginalSignal") self.gridLayout_7.addWidget(self.gVOriginalSignal, 9, 1, 1, 3) @@ -110,10 +130,14 @@ def setupUi(self, DialogModulation): self.gridLayout_4 = QtWidgets.QGridLayout(self.scrollAreaWidgetContents_5) self.gridLayout_4.setObjectName("gridLayout_4") self.lCurrentSearchResult = QtWidgets.QLabel(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lCurrentSearchResult.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lCurrentSearchResult.sizePolicy().hasHeightForWidth() + ) self.lCurrentSearchResult.setSizePolicy(sizePolicy) self.lCurrentSearchResult.setMinimumSize(QtCore.QSize(0, 0)) self.lCurrentSearchResult.setMaximumSize(QtCore.QSize(16777215, 16777215)) @@ -121,20 +145,28 @@ def setupUi(self, DialogModulation): self.lCurrentSearchResult.setObjectName("lCurrentSearchResult") self.gridLayout_4.addWidget(self.lCurrentSearchResult, 3, 1, 1, 2) self.cbShowDataBitsOnly = QtWidgets.QCheckBox(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.cbShowDataBitsOnly.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.cbShowDataBitsOnly.sizePolicy().hasHeightForWidth() + ) self.cbShowDataBitsOnly.setSizePolicy(sizePolicy) self.cbShowDataBitsOnly.setMinimumSize(QtCore.QSize(0, 0)) self.cbShowDataBitsOnly.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.cbShowDataBitsOnly.setObjectName("cbShowDataBitsOnly") self.gridLayout_4.addWidget(self.cbShowDataBitsOnly, 2, 0, 1, 5) self.btnSearchPrev = QtWidgets.QPushButton(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.btnSearchPrev.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.btnSearchPrev.sizePolicy().hasHeightForWidth() + ) self.btnSearchPrev.setSizePolicy(sizePolicy) self.btnSearchPrev.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.btnSearchPrev.setText("") @@ -143,20 +175,28 @@ def setupUi(self, DialogModulation): self.btnSearchPrev.setObjectName("btnSearchPrev") self.gridLayout_4.addWidget(self.btnSearchPrev, 3, 0, 1, 1) self.lTotalSearchresults = QtWidgets.QLabel(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lTotalSearchresults.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lTotalSearchresults.sizePolicy().hasHeightForWidth() + ) self.lTotalSearchresults.setSizePolicy(sizePolicy) self.lTotalSearchresults.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.lTotalSearchresults.setAlignment(QtCore.Qt.AlignCenter) self.lTotalSearchresults.setObjectName("lTotalSearchresults") self.gridLayout_4.addWidget(self.lTotalSearchresults, 3, 4, 1, 1) self.treeViewSignals = ModulatorTreeView(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.treeViewSignals.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.treeViewSignals.sizePolicy().hasHeightForWidth() + ) self.treeViewSignals.setSizePolicy(sizePolicy) self.treeViewSignals.setProperty("showDropIndicator", True) self.treeViewSignals.setDragEnabled(True) @@ -165,7 +205,9 @@ def setupUi(self, DialogModulation): self.treeViewSignals.setObjectName("treeViewSignals") self.gridLayout_4.addWidget(self.treeViewSignals, 0, 0, 1, 6) self.lSlash = QtWidgets.QLabel(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lSlash.sizePolicy().hasHeightForWidth()) @@ -174,10 +216,14 @@ def setupUi(self, DialogModulation): self.lSlash.setObjectName("lSlash") self.gridLayout_4.addWidget(self.lSlash, 3, 3, 1, 1) self.btnSearchNext = QtWidgets.QPushButton(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.btnSearchNext.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.btnSearchNext.sizePolicy().hasHeightForWidth() + ) self.btnSearchNext.setSizePolicy(sizePolicy) self.btnSearchNext.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.btnSearchNext.setText("") @@ -186,10 +232,14 @@ def setupUi(self, DialogModulation): self.btnSearchNext.setObjectName("btnSearchNext") self.gridLayout_4.addWidget(self.btnSearchNext, 3, 5, 1, 1) self.chkBoxLockSIV = QtWidgets.QCheckBox(self.scrollAreaWidgetContents_5) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.chkBoxLockSIV.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.chkBoxLockSIV.sizePolicy().hasHeightForWidth() + ) self.chkBoxLockSIV.setSizePolicy(sizePolicy) self.chkBoxLockSIV.setObjectName("chkBoxLockSIV") self.gridLayout_4.addWidget(self.chkBoxLockSIV, 1, 0, 1, 5) @@ -197,26 +247,38 @@ def setupUi(self, DialogModulation): self.gridLayout_7.addWidget(self.scrollArea_5, 9, 0, 1, 1) self.horizontalLayout = QtWidgets.QHBoxLayout() self.horizontalLayout.setObjectName("horizontalLayout") - self.lSamplesInViewModulatedText = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + self.lSamplesInViewModulatedText = QtWidgets.QLabel( + self.scrollAreaWidgetContents_2 + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesInViewModulatedText.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesInViewModulatedText.sizePolicy().hasHeightForWidth() + ) self.lSamplesInViewModulatedText.setSizePolicy(sizePolicy) self.lSamplesInViewModulatedText.setObjectName("lSamplesInViewModulatedText") self.horizontalLayout.addWidget(self.lSamplesInViewModulatedText) self.lSamplesInViewModulated = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesInViewModulated.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesInViewModulated.sizePolicy().hasHeightForWidth() + ) self.lSamplesInViewModulated.setSizePolicy(sizePolicy) self.lSamplesInViewModulated.setObjectName("lSamplesInViewModulated") self.horizontalLayout.addWidget(self.lSamplesInViewModulated) self.label_9 = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) self.label_9.setObjectName("label_9") self.horizontalLayout.addWidget(self.label_9) - self.lModulatedSelectedSamples = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) + self.lModulatedSelectedSamples = QtWidgets.QLabel( + self.scrollAreaWidgetContents_2 + ) self.lModulatedSelectedSamples.setObjectName("lModulatedSelectedSamples") self.horizontalLayout.addWidget(self.lModulatedSelectedSamples) self.gridLayout_7.addLayout(self.horizontalLayout, 6, 1, 1, 1) @@ -230,20 +292,28 @@ def setupUi(self, DialogModulation): self.gridLayout_2 = QtWidgets.QGridLayout(self.scrollAreaWidgetContents_3) self.gridLayout_2.setObjectName("gridLayout_2") self.spinBoxSampleRate = KillerDoubleSpinBox(self.scrollAreaWidgetContents_3) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxSampleRate.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxSampleRate.sizePolicy().hasHeightForWidth() + ) self.spinBoxSampleRate.setSizePolicy(sizePolicy) self.spinBoxSampleRate.setDecimals(10) self.spinBoxSampleRate.setMinimum(0.001) self.spinBoxSampleRate.setMaximum(999999999.0) self.spinBoxSampleRate.setObjectName("spinBoxSampleRate") self.gridLayout_2.addWidget(self.spinBoxSampleRate, 2, 1, 1, 1) - spacerItem3 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem3 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_2.addItem(spacerItem3, 3, 0, 1, 1) self.label_3 = QtWidgets.QLabel(self.scrollAreaWidgetContents_3) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label_3.sizePolicy().hasHeightForWidth()) @@ -251,28 +321,40 @@ def setupUi(self, DialogModulation): self.label_3.setObjectName("label_3") self.gridLayout_2.addWidget(self.label_3, 2, 0, 1, 1) self.label = QtWidgets.QLabel(self.scrollAreaWidgetContents_3) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label.sizePolicy().hasHeightForWidth()) self.label.setSizePolicy(sizePolicy) self.label.setObjectName("label") self.gridLayout_2.addWidget(self.label, 1, 0, 1, 1) - self.spinBoxSamplesPerSymbol = QtWidgets.QSpinBox(self.scrollAreaWidgetContents_3) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + self.spinBoxSamplesPerSymbol = QtWidgets.QSpinBox( + self.scrollAreaWidgetContents_3 + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxSamplesPerSymbol.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxSamplesPerSymbol.sizePolicy().hasHeightForWidth() + ) self.spinBoxSamplesPerSymbol.setSizePolicy(sizePolicy) self.spinBoxSamplesPerSymbol.setMinimum(1) self.spinBoxSamplesPerSymbol.setMaximum(999999) self.spinBoxSamplesPerSymbol.setObjectName("spinBoxSamplesPerSymbol") self.gridLayout_2.addWidget(self.spinBoxSamplesPerSymbol, 1, 1, 1, 1) self.linEdDataBits = QtWidgets.QLineEdit(self.scrollAreaWidgetContents_3) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.linEdDataBits.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.linEdDataBits.sizePolicy().hasHeightForWidth() + ) self.linEdDataBits.setSizePolicy(sizePolicy) self.linEdDataBits.setObjectName("linEdDataBits") self.gridLayout_2.addWidget(self.linEdDataBits, 0, 0, 1, 2) @@ -288,18 +370,26 @@ def setupUi(self, DialogModulation): self.gridLayout = QtWidgets.QGridLayout(self.scrollAreaWidgetContents) self.gridLayout.setObjectName("gridLayout") self.lCarrierFreq = QtWidgets.QLabel(self.scrollAreaWidgetContents) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lCarrierFreq.sizePolicy().hasHeightForWidth()) self.lCarrierFreq.setSizePolicy(sizePolicy) self.lCarrierFreq.setObjectName("lCarrierFreq") self.gridLayout.addWidget(self.lCarrierFreq, 0, 0, 1, 1) - self.doubleSpinBoxCarrierFreq = KillerDoubleSpinBox(self.scrollAreaWidgetContents) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + self.doubleSpinBoxCarrierFreq = KillerDoubleSpinBox( + self.scrollAreaWidgetContents + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.doubleSpinBoxCarrierFreq.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.doubleSpinBoxCarrierFreq.sizePolicy().hasHeightForWidth() + ) self.doubleSpinBoxCarrierFreq.setSizePolicy(sizePolicy) self.doubleSpinBoxCarrierFreq.setSuffix("") self.doubleSpinBoxCarrierFreq.setDecimals(10) @@ -308,18 +398,26 @@ def setupUi(self, DialogModulation): self.doubleSpinBoxCarrierFreq.setObjectName("doubleSpinBoxCarrierFreq") self.gridLayout.addWidget(self.doubleSpinBoxCarrierFreq, 0, 1, 1, 1) self.label_2 = QtWidgets.QLabel(self.scrollAreaWidgetContents) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label_2.sizePolicy().hasHeightForWidth()) self.label_2.setSizePolicy(sizePolicy) self.label_2.setObjectName("label_2") self.gridLayout.addWidget(self.label_2, 1, 0, 1, 1) - self.doubleSpinBoxCarrierPhase = QtWidgets.QDoubleSpinBox(self.scrollAreaWidgetContents) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + self.doubleSpinBoxCarrierPhase = QtWidgets.QDoubleSpinBox( + self.scrollAreaWidgetContents + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.doubleSpinBoxCarrierPhase.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.doubleSpinBoxCarrierPhase.sizePolicy().hasHeightForWidth() + ) self.doubleSpinBoxCarrierPhase.setSizePolicy(sizePolicy) self.doubleSpinBoxCarrierPhase.setDecimals(3) self.doubleSpinBoxCarrierPhase.setMaximum(360.0) @@ -327,19 +425,27 @@ def setupUi(self, DialogModulation): self.gridLayout.addWidget(self.doubleSpinBoxCarrierPhase, 1, 1, 1, 1) self.btnAutoDetect = QtWidgets.QPushButton(self.scrollAreaWidgetContents) self.btnAutoDetect.setEnabled(False) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.btnAutoDetect.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.btnAutoDetect.sizePolicy().hasHeightForWidth() + ) self.btnAutoDetect.setSizePolicy(sizePolicy) self.btnAutoDetect.setObjectName("btnAutoDetect") self.gridLayout.addWidget(self.btnAutoDetect, 2, 0, 1, 2) - spacerItem4 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem4 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout.addItem(spacerItem4, 3, 0, 1, 1) self.scrollArea_2.setWidget(self.scrollAreaWidgetContents) self.gridLayout_7.addWidget(self.scrollArea_2, 1, 0, 1, 1) self.lPlus = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lPlus.sizePolicy().hasHeightForWidth()) @@ -352,7 +458,9 @@ def setupUi(self, DialogModulation): self.lPlus.setObjectName("lPlus") self.gridLayout_7.addWidget(self.lPlus, 2, 2, 1, 1) self.gVCarrier = ZoomableGraphicView(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.gVCarrier.sizePolicy().hasHeightForWidth()) @@ -360,14 +468,20 @@ def setupUi(self, DialogModulation): self.gVCarrier.setAcceptDrops(False) self.gVCarrier.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) self.gVCarrier.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOn) - self.gVCarrier.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.HighQualityAntialiasing) + self.gVCarrier.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.HighQualityAntialiasing + ) self.gVCarrier.setDragMode(QtWidgets.QGraphicsView.NoDrag) self.gVCarrier.setObjectName("gVCarrier") self.gridLayout_7.addWidget(self.gVCarrier, 1, 1, 1, 3) - spacerItem5 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem5 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_7.addItem(spacerItem5, 2, 1, 1, 1) self.gVModulated = ZoomableGraphicView(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.gVModulated.sizePolicy().hasHeightForWidth()) @@ -375,12 +489,16 @@ def setupUi(self, DialogModulation): self.gVModulated.setAcceptDrops(False) self.gVModulated.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) self.gVModulated.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOn) - self.gVModulated.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.HighQualityAntialiasing) + self.gVModulated.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.HighQualityAntialiasing + ) self.gVModulated.setDragMode(QtWidgets.QGraphicsView.NoDrag) self.gVModulated.setObjectName("gVModulated") self.gridLayout_7.addWidget(self.gVModulated, 5, 1, 1, 3) self.gVData = ZoomableGraphicView(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.gVData.sizePolicy().hasHeightForWidth()) @@ -388,7 +506,9 @@ def setupUi(self, DialogModulation): self.gVData.setAcceptDrops(False) self.gVData.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) self.gVData.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOn) - self.gVData.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.HighQualityAntialiasing) + self.gVData.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.HighQualityAntialiasing + ) self.gVData.setDragMode(QtWidgets.QGraphicsView.NoDrag) self.gVData.setObjectName("gVData") self.gridLayout_7.addWidget(self.gVData, 3, 1, 1, 3) @@ -418,7 +538,9 @@ def setupUi(self, DialogModulation): self.lGaussBT = QtWidgets.QLabel(self.scrollAreaWidgetContents_4) self.lGaussBT.setObjectName("lGaussBT") self.gridLayout_3.addWidget(self.lGaussBT, 3, 0, 1, 1) - self.spinBoxGaussFilterWidth = QtWidgets.QDoubleSpinBox(self.scrollAreaWidgetContents_4) + self.spinBoxGaussFilterWidth = QtWidgets.QDoubleSpinBox( + self.scrollAreaWidgetContents_4 + ) self.spinBoxGaussFilterWidth.setMinimum(0.01) self.spinBoxGaussFilterWidth.setMaximum(100.0) self.spinBoxGaussFilterWidth.setSingleStep(0.01) @@ -428,19 +550,29 @@ def setupUi(self, DialogModulation): self.labelBitsPerSymbol = QtWidgets.QLabel(self.scrollAreaWidgetContents_4) self.labelBitsPerSymbol.setObjectName("labelBitsPerSymbol") self.gridLayout_3.addWidget(self.labelBitsPerSymbol, 1, 0, 1, 1) - spacerItem6 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem6 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_3.addItem(spacerItem6, 5, 0, 1, 1) - spacerItem7 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem7 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_3.addItem(spacerItem7, 5, 1, 1, 1) self.lineEditParameters = QtWidgets.QLineEdit(self.scrollAreaWidgetContents_4) self.lineEditParameters.setClearButtonEnabled(False) self.lineEditParameters.setObjectName("lineEditParameters") self.gridLayout_3.addWidget(self.lineEditParameters, 2, 1, 1, 1) - self.comboBoxModulationType = QtWidgets.QComboBox(self.scrollAreaWidgetContents_4) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + self.comboBoxModulationType = QtWidgets.QComboBox( + self.scrollAreaWidgetContents_4 + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.comboBoxModulationType.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.comboBoxModulationType.sizePolicy().hasHeightForWidth() + ) self.comboBoxModulationType.setSizePolicy(sizePolicy) self.comboBoxModulationType.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.comboBoxModulationType.setObjectName("comboBoxModulationType") @@ -454,12 +586,18 @@ def setupUi(self, DialogModulation): self.gridLayout_3.addWidget(self.labelParameters, 2, 0, 1, 1) self.scrollArea_4.setWidget(self.scrollAreaWidgetContents_4) self.gridLayout_7.addWidget(self.scrollArea_4, 5, 0, 1, 1) - spacerItem8 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem8 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_7.addItem(spacerItem8, 4, 3, 1, 1) - spacerItem9 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem9 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout_7.addItem(spacerItem9, 8, 3, 1, 1) self.lEqual_qm = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lEqual_qm.sizePolicy().hasHeightForWidth()) @@ -473,27 +611,43 @@ def setupUi(self, DialogModulation): self.gridLayout_7.addWidget(self.lEqual_qm, 8, 2, 1, 1) self.horizontalLayout_2 = QtWidgets.QHBoxLayout() self.horizontalLayout_2.setObjectName("horizontalLayout_2") - self.lSamplesInViewOrigSignalText = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred) + self.lSamplesInViewOrigSignalText = QtWidgets.QLabel( + self.scrollAreaWidgetContents_2 + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesInViewOrigSignalText.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesInViewOrigSignalText.sizePolicy().hasHeightForWidth() + ) self.lSamplesInViewOrigSignalText.setSizePolicy(sizePolicy) self.lSamplesInViewOrigSignalText.setObjectName("lSamplesInViewOrigSignalText") self.horizontalLayout_2.addWidget(self.lSamplesInViewOrigSignalText) - self.lSamplesInViewOrigSignal = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + self.lSamplesInViewOrigSignal = QtWidgets.QLabel( + self.scrollAreaWidgetContents_2 + ) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesInViewOrigSignal.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesInViewOrigSignal.sizePolicy().hasHeightForWidth() + ) self.lSamplesInViewOrigSignal.setSizePolicy(sizePolicy) self.lSamplesInViewOrigSignal.setObjectName("lSamplesInViewOrigSignal") self.horizontalLayout_2.addWidget(self.lSamplesInViewOrigSignal) self.label_10 = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) self.label_10.setObjectName("label_10") self.horizontalLayout_2.addWidget(self.label_10) - self.lOriginalSignalSamplesSelected = QtWidgets.QLabel(self.scrollAreaWidgetContents_2) - self.lOriginalSignalSamplesSelected.setObjectName("lOriginalSignalSamplesSelected") + self.lOriginalSignalSamplesSelected = QtWidgets.QLabel( + self.scrollAreaWidgetContents_2 + ) + self.lOriginalSignalSamplesSelected.setObjectName( + "lOriginalSignalSamplesSelected" + ) self.horizontalLayout_2.addWidget(self.lOriginalSignalSamplesSelected) self.gridLayout_7.addLayout(self.horizontalLayout_2, 10, 1, 1, 1) self.gridLayout_7.setRowStretch(1, 1) @@ -506,15 +660,21 @@ def setupUi(self, DialogModulation): self.retranslateUi(DialogModulation) DialogModulation.setTabOrder(self.btnAddModulation, self.scrollArea_2) DialogModulation.setTabOrder(self.scrollArea_2, self.doubleSpinBoxCarrierFreq) - DialogModulation.setTabOrder(self.doubleSpinBoxCarrierFreq, self.doubleSpinBoxCarrierPhase) + DialogModulation.setTabOrder( + self.doubleSpinBoxCarrierFreq, self.doubleSpinBoxCarrierPhase + ) DialogModulation.setTabOrder(self.doubleSpinBoxCarrierPhase, self.btnAutoDetect) DialogModulation.setTabOrder(self.btnAutoDetect, self.scrollArea_3) DialogModulation.setTabOrder(self.scrollArea_3, self.linEdDataBits) DialogModulation.setTabOrder(self.linEdDataBits, self.spinBoxSamplesPerSymbol) - DialogModulation.setTabOrder(self.spinBoxSamplesPerSymbol, self.spinBoxSampleRate) + DialogModulation.setTabOrder( + self.spinBoxSamplesPerSymbol, self.spinBoxSampleRate + ) DialogModulation.setTabOrder(self.spinBoxSampleRate, self.scrollArea_4) DialogModulation.setTabOrder(self.scrollArea_4, self.comboBoxModulationType) - DialogModulation.setTabOrder(self.comboBoxModulationType, self.spinBoxBitsPerSymbol) + DialogModulation.setTabOrder( + self.comboBoxModulationType, self.spinBoxBitsPerSymbol + ) DialogModulation.setTabOrder(self.spinBoxBitsPerSymbol, self.lineEditParameters) DialogModulation.setTabOrder(self.lineEditParameters, self.spinBoxGaussBT) DialogModulation.setTabOrder(self.spinBoxGaussBT, self.spinBoxGaussFilterWidth) @@ -529,51 +689,97 @@ def setupUi(self, DialogModulation): DialogModulation.setTabOrder(self.cbShowDataBitsOnly, self.btnSearchPrev) DialogModulation.setTabOrder(self.btnSearchPrev, self.btnSearchNext) DialogModulation.setTabOrder(self.btnSearchNext, self.btnRemoveModulation) - DialogModulation.setTabOrder(self.btnRemoveModulation, self.comboBoxCustomModulations) + DialogModulation.setTabOrder( + self.btnRemoveModulation, self.comboBoxCustomModulations + ) DialogModulation.setTabOrder(self.comboBoxCustomModulations, self.scrollArea) def retranslateUi(self, DialogModulation): _translate = QtCore.QCoreApplication.translate DialogModulation.setWindowTitle(_translate("DialogModulation", "Modulation")) - self.comboBoxCustomModulations.setItemText(0, _translate("DialogModulation", "My Modulation")) + self.comboBoxCustomModulations.setItemText( + 0, _translate("DialogModulation", "My Modulation") + ) self.btnAddModulation.setText(_translate("DialogModulation", "...")) self.btnRemoveModulation.setText(_translate("DialogModulation", "...")) self.label_5.setText(_translate("DialogModulation", "Data (raw bits)")) self.label_6.setText(_translate("DialogModulation", "Modulation")) - self.label_7.setText(_translate("DialogModulation", "Original Signal (drag&drop)")) + self.label_7.setText( + _translate("DialogModulation", "Original Signal (drag&drop)") + ) self.label_4.setText(_translate("DialogModulation", "Carrier")) self.lCurrentSearchResult.setText(_translate("DialogModulation", "-")) - self.cbShowDataBitsOnly.setText(_translate("DialogModulation", "Show Only Data Sequence\n" -"(10)")) + self.cbShowDataBitsOnly.setText( + _translate("DialogModulation", "Show Only Data Sequence\n" "(10)") + ) self.lTotalSearchresults.setText(_translate("DialogModulation", "-")) self.lSlash.setText(_translate("DialogModulation", "/")) - self.chkBoxLockSIV.setText(_translate("DialogModulation", "Lock view to original signal")) - self.lSamplesInViewModulatedText.setText(_translate("DialogModulation", "Samples in View:")) - self.lSamplesInViewModulated.setToolTip(_translate("DialogModulation", "Shown Samples in View:
Red - if samples in view differ from original signal
Normal - if samples in view are equal to the original signal
")) - self.lSamplesInViewModulated.setText(_translate("DialogModulation", "101010121")) + self.chkBoxLockSIV.setText( + _translate("DialogModulation", "Lock view to original signal") + ) + self.lSamplesInViewModulatedText.setText( + _translate("DialogModulation", "Samples in View:") + ) + self.lSamplesInViewModulated.setToolTip( + _translate( + "DialogModulation", + 'Shown Samples in View:
Red - if samples in view differ from original signal
Normal - if samples in view are equal to the original signal
', + ) + ) + self.lSamplesInViewModulated.setText( + _translate("DialogModulation", "101010121") + ) self.label_9.setText(_translate("DialogModulation", "Samples selected:")) self.lModulatedSelectedSamples.setText(_translate("DialogModulation", "0")) self.label_3.setText(_translate("DialogModulation", "Sample Rate (Sps):")) self.label.setText(_translate("DialogModulation", "Samples per Symbol:")) - self.linEdDataBits.setPlaceholderText(_translate("DialogModulation", "Enter Data Bits here")) + self.linEdDataBits.setPlaceholderText( + _translate("DialogModulation", "Enter Data Bits here") + ) self.lCarrierFreq.setText(_translate("DialogModulation", "Frequency:")) self.label_2.setText(_translate("DialogModulation", "Phase:")) self.doubleSpinBoxCarrierPhase.setSuffix(_translate("DialogModulation", "°")) - self.btnAutoDetect.setToolTip(_translate("DialogModulation", "Auto detect the frequency based on the original signal. You have to select a signal (bottom of this window) to use this feature.
Select a signal by dragging it from the tree and dropping it on the graphics pane to the right.
")) - self.btnAutoDetect.setText(_translate("DialogModulation", "Auto detect from original signal")) + self.btnAutoDetect.setToolTip( + _translate( + "DialogModulation", + 'Auto detect the frequency based on the original signal. You have to select a signal (bottom of this window) to use this feature.
Select a signal by dragging it from the tree and dropping it on the graphics pane to the right.
', + ) + ) + self.btnAutoDetect.setText( + _translate("DialogModulation", "Auto detect from original signal") + ) self.lGaussWidth.setText(_translate("DialogModulation", "Gauss filter width:")) self.lGaussBT.setText(_translate("DialogModulation", "Gauss BT:")) - self.labelBitsPerSymbol.setText(_translate("DialogModulation", "Bits per Symbol:")) - self.comboBoxModulationType.setItemText(0, _translate("DialogModulation", "Amplitude Shift Keying (ASK)")) - self.comboBoxModulationType.setItemText(1, _translate("DialogModulation", "Frequency Shift Keying (FSK)")) - self.comboBoxModulationType.setItemText(2, _translate("DialogModulation", "Gaussian Frequency Shift Keying (GFSK)")) - self.comboBoxModulationType.setItemText(3, _translate("DialogModulation", "Phase Shift Keying (PSK)")) + self.labelBitsPerSymbol.setText( + _translate("DialogModulation", "Bits per Symbol:") + ) + self.comboBoxModulationType.setItemText( + 0, _translate("DialogModulation", "Amplitude Shift Keying (ASK)") + ) + self.comboBoxModulationType.setItemText( + 1, _translate("DialogModulation", "Frequency Shift Keying (FSK)") + ) + self.comboBoxModulationType.setItemText( + 2, _translate("DialogModulation", "Gaussian Frequency Shift Keying (GFSK)") + ) + self.comboBoxModulationType.setItemText( + 3, _translate("DialogModulation", "Phase Shift Keying (PSK)") + ) self.labelParameters.setText(_translate("DialogModulation", "Parameters:")) - self.lSamplesInViewOrigSignalText.setText(_translate("DialogModulation", "Samples in View:")) - self.lSamplesInViewOrigSignal.setToolTip(_translate("DialogModulation", "Shown Samples in View:
Red - if samples in view differ from original signal
Normal - if samples in view are equal to the original signal
")) + self.lSamplesInViewOrigSignalText.setText( + _translate("DialogModulation", "Samples in View:") + ) + self.lSamplesInViewOrigSignal.setToolTip( + _translate( + "DialogModulation", + 'Shown Samples in View:
Red - if samples in view differ from original signal
Normal - if samples in view are equal to the original signal
', + ) + ) self.lSamplesInViewOrigSignal.setText(_translate("DialogModulation", "0")) self.label_10.setText(_translate("DialogModulation", "Samples selected:")) self.lOriginalSignalSamplesSelected.setText(_translate("DialogModulation", "0")) + + from urh.ui.KillerDoubleSpinBox import KillerDoubleSpinBox from urh.ui.views.ModulatorTreeView import ModulatorTreeView from urh.ui.views.ZoomAndDropableGraphicView import ZoomAndDropableGraphicView diff --git a/src/urh/ui/ui_modulation_parameters_dialog.py b/src/urh/ui/ui_modulation_parameters_dialog.py index 62d1f9b6e2..61a6e79f6d 100644 --- a/src/urh/ui/ui_modulation_parameters_dialog.py +++ b/src/urh/ui/ui_modulation_parameters_dialog.py @@ -28,7 +28,9 @@ def setupUi(self, DialogModulationParameters): self.tblSymbolParameters.verticalHeader().setVisible(False) self.verticalLayout.addWidget(self.tblSymbolParameters) self.buttonBox = QtWidgets.QDialogButtonBox(DialogModulationParameters) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setObjectName("buttonBox") self.verticalLayout.addWidget(self.buttonBox) @@ -36,7 +38,9 @@ def setupUi(self, DialogModulationParameters): def retranslateUi(self, DialogModulationParameters): _translate = QtCore.QCoreApplication.translate - DialogModulationParameters.setWindowTitle(_translate("DialogModulationParameters", "Modulation Parameters")) + DialogModulationParameters.setWindowTitle( + _translate("DialogModulationParameters", "Modulation Parameters") + ) item = self.tblSymbolParameters.horizontalHeaderItem(0) item.setText(_translate("DialogModulationParameters", "Symbol")) item = self.tblSymbolParameters.horizontalHeaderItem(1) diff --git a/src/urh/ui/ui_modulation_settings_widget.py b/src/urh/ui/ui_modulation_settings_widget.py index 2d9256a99a..f2b4d0714d 100644 --- a/src/urh/ui/ui_modulation_settings_widget.py +++ b/src/urh/ui/ui_modulation_settings_widget.py @@ -20,20 +20,22 @@ def setupUi(self, ModulationSettings): font.setBold(True) font.setWeight(75) self.groupBoxSniffSettings.setFont(font) - self.groupBoxSniffSettings.setStyleSheet("QGroupBox\n" -"{\n" -"border: none;\n" -"}\n" -"\n" -"QGroupBox::title {\n" -" subcontrol-origin: margin;\n" -"}\n" -"QGroupBox::indicator:unchecked {\n" -" image: url(:/icons/icons/collapse.svg)\n" -"}\n" -"QGroupBox::indicator:checked {\n" -" image: url(:/icons/icons/uncollapse.svg)\n" -"}") + self.groupBoxSniffSettings.setStyleSheet( + "QGroupBox\n" + "{\n" + "border: none;\n" + "}\n" + "\n" + "QGroupBox::title {\n" + " subcontrol-origin: margin;\n" + "}\n" + "QGroupBox::indicator:unchecked {\n" + " image: url(:/icons/icons/collapse.svg)\n" + "}\n" + "QGroupBox::indicator:checked {\n" + " image: url(:/icons/icons/uncollapse.svg)\n" + "}" + ) self.groupBoxSniffSettings.setFlat(True) self.groupBoxSniffSettings.setCheckable(True) self.groupBoxSniffSettings.setObjectName("groupBoxSniffSettings") @@ -56,10 +58,14 @@ def setupUi(self, ModulationSettings): self.gridLayout = QtWidgets.QGridLayout() self.gridLayout.setObjectName("gridLayout") self.labelCarrierFrequencyValue = QtWidgets.QLabel(self.frame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.labelCarrierFrequencyValue.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.labelCarrierFrequencyValue.sizePolicy().hasHeightForWidth() + ) self.labelCarrierFrequencyValue.setSizePolicy(sizePolicy) self.labelCarrierFrequencyValue.setObjectName("labelCarrierFrequencyValue") self.gridLayout.addWidget(self.labelCarrierFrequencyValue, 0, 1, 1, 1) @@ -112,33 +118,61 @@ def setupUi(self, ModulationSettings): self.btnConfigurationDialog.setIcon(icon) self.btnConfigurationDialog.setObjectName("btnConfigurationDialog") self.verticalLayout_2.addWidget(self.btnConfigurationDialog) - spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_2.addItem(spacerItem) self.gridLayout_3.addWidget(self.frame, 0, 0, 1, 1) self.verticalLayout.addWidget(self.groupBoxSniffSettings) self.retranslateUi(ModulationSettings) - self.groupBoxSniffSettings.toggled['bool'].connect(self.frame.setVisible) - ModulationSettings.setTabOrder(self.groupBoxSniffSettings, self.comboBoxModulationProfiles) - ModulationSettings.setTabOrder(self.comboBoxModulationProfiles, self.btnConfigurationDialog) + self.groupBoxSniffSettings.toggled["bool"].connect(self.frame.setVisible) + ModulationSettings.setTabOrder( + self.groupBoxSniffSettings, self.comboBoxModulationProfiles + ) + ModulationSettings.setTabOrder( + self.comboBoxModulationProfiles, self.btnConfigurationDialog + ) def retranslateUi(self, ModulationSettings): _translate = QtCore.QCoreApplication.translate ModulationSettings.setWindowTitle(_translate("ModulationSettings", "Form")) - self.groupBoxSniffSettings.setTitle(_translate("ModulationSettings", "Modulation settings")) - self.labelModulationProfile.setText(_translate("ModulationSettings", "Choose profile:")) - self.labelCarrierFrequencyValue.setText(_translate("ModulationSettings", "TextLabel")) + self.groupBoxSniffSettings.setTitle( + _translate("ModulationSettings", "Modulation settings") + ) + self.labelModulationProfile.setText( + _translate("ModulationSettings", "Choose profile:") + ) + self.labelCarrierFrequencyValue.setText( + _translate("ModulationSettings", "TextLabel") + ) self.labelSampleRate.setText(_translate("ModulationSettings", "Sample Rate:")) - self.labelCarrierFrequency.setText(_translate("ModulationSettings", "Carrier Frequency:")) - self.labelSamplesPerSymbol.setText(_translate("ModulationSettings", "Samples per Symbol:")) + self.labelCarrierFrequency.setText( + _translate("ModulationSettings", "Carrier Frequency:") + ) + self.labelSamplesPerSymbol.setText( + _translate("ModulationSettings", "Samples per Symbol:") + ) self.labelSampleRateValue.setText(_translate("ModulationSettings", "TextLabel")) - self.labelModulationType.setText(_translate("ModulationSettings", "Modulation type:")) - self.labelSamplesPerSymbolValue.setText(_translate("ModulationSettings", "TextLabel")) - self.labelModulationTypeValue.setText(_translate("ModulationSettings", "TextLabel")) - self.labelParameters.setText(_translate("ModulationSettings", "Amplitudes in %:")) + self.labelModulationType.setText( + _translate("ModulationSettings", "Modulation type:") + ) + self.labelSamplesPerSymbolValue.setText( + _translate("ModulationSettings", "TextLabel") + ) + self.labelModulationTypeValue.setText( + _translate("ModulationSettings", "TextLabel") + ) + self.labelParameters.setText( + _translate("ModulationSettings", "Amplitudes in %:") + ) self.labelParameterValues.setText(_translate("ModulationSettings", "0/100")) self.label.setText(_translate("ModulationSettings", "Bits per Symbol:")) self.labelBitsPerSymbol.setText(_translate("ModulationSettings", "1")) - self.btnConfigurationDialog.setText(_translate("ModulationSettings", "Open modulation configuration dialog...")) + self.btnConfigurationDialog.setText( + _translate("ModulationSettings", "Open modulation configuration dialog...") + ) + + from urh.ui.ElidedLabel import ElidedLabel from . import urh_rc diff --git a/src/urh/ui/ui_options.py b/src/urh/ui/ui_options.py index 99052ed25a..94d77335ee 100644 --- a/src/urh/ui/ui_options.py +++ b/src/urh/ui/ui_options.py @@ -44,17 +44,31 @@ def setupUi(self, DialogOptions): self.groupBoxModulationAccuracy.setObjectName("groupBoxModulationAccuracy") self.verticalLayout_7 = QtWidgets.QVBoxLayout(self.groupBoxModulationAccuracy) self.verticalLayout_7.setObjectName("verticalLayout_7") - self.radioButtonLowModulationAccuracy = QtWidgets.QRadioButton(self.groupBoxModulationAccuracy) - self.radioButtonLowModulationAccuracy.setObjectName("radioButtonLowModulationAccuracy") + self.radioButtonLowModulationAccuracy = QtWidgets.QRadioButton( + self.groupBoxModulationAccuracy + ) + self.radioButtonLowModulationAccuracy.setObjectName( + "radioButtonLowModulationAccuracy" + ) self.verticalLayout_7.addWidget(self.radioButtonLowModulationAccuracy) - self.radioButtonMediumModulationAccuracy = QtWidgets.QRadioButton(self.groupBoxModulationAccuracy) - self.radioButtonMediumModulationAccuracy.setObjectName("radioButtonMediumModulationAccuracy") + self.radioButtonMediumModulationAccuracy = QtWidgets.QRadioButton( + self.groupBoxModulationAccuracy + ) + self.radioButtonMediumModulationAccuracy.setObjectName( + "radioButtonMediumModulationAccuracy" + ) self.verticalLayout_7.addWidget(self.radioButtonMediumModulationAccuracy) - self.radioButtonHighModulationAccuracy = QtWidgets.QRadioButton(self.groupBoxModulationAccuracy) - self.radioButtonHighModulationAccuracy.setObjectName("radioButtonHighModulationAccuracy") + self.radioButtonHighModulationAccuracy = QtWidgets.QRadioButton( + self.groupBoxModulationAccuracy + ) + self.radioButtonHighModulationAccuracy.setObjectName( + "radioButtonHighModulationAccuracy" + ) self.verticalLayout_7.addWidget(self.radioButtonHighModulationAccuracy) self.verticalLayout_9.addWidget(self.groupBoxModulationAccuracy) - spacerItem = QtWidgets.QSpacerItem(20, 500, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 500, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_9.addItem(spacerItem) self.tabWidget.addTab(self.tabGeneration, "") self.tabView = QtWidgets.QWidget() @@ -67,10 +81,14 @@ def setupUi(self, DialogOptions): self.label_7.setObjectName("label_7") self.horizontalLayout_2.addWidget(self.label_7) self.comboBoxDefaultView = QtWidgets.QComboBox(self.tabView) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.comboBoxDefaultView.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.comboBoxDefaultView.sizePolicy().hasHeightForWidth() + ) self.comboBoxDefaultView.setSizePolicy(sizePolicy) self.comboBoxDefaultView.setObjectName("comboBoxDefaultView") self.comboBoxDefaultView.addItem("") @@ -79,7 +97,9 @@ def setupUi(self, DialogOptions): self.horizontalLayout_2.addWidget(self.comboBoxDefaultView) self.verticalLayout.addLayout(self.horizontalLayout_2) self.checkBoxShowConfirmCloseDialog = QtWidgets.QCheckBox(self.tabView) - self.checkBoxShowConfirmCloseDialog.setObjectName("checkBoxShowConfirmCloseDialog") + self.checkBoxShowConfirmCloseDialog.setObjectName( + "checkBoxShowConfirmCloseDialog" + ) self.verticalLayout.addWidget(self.checkBoxShowConfirmCloseDialog) self.checkBoxHoldShiftToDrag = QtWidgets.QCheckBox(self.tabView) self.checkBoxHoldShiftToDrag.setObjectName("checkBoxHoldShiftToDrag") @@ -127,15 +147,27 @@ def setupUi(self, DialogOptions): self.groupBoxSpectrogramColormap.setObjectName("groupBoxSpectrogramColormap") self.verticalLayout_2 = QtWidgets.QVBoxLayout(self.groupBoxSpectrogramColormap) self.verticalLayout_2.setObjectName("verticalLayout_2") - self.scrollAreaSpectrogramColormap = QtWidgets.QScrollArea(self.groupBoxSpectrogramColormap) + self.scrollAreaSpectrogramColormap = QtWidgets.QScrollArea( + self.groupBoxSpectrogramColormap + ) self.scrollAreaSpectrogramColormap.setWidgetResizable(True) - self.scrollAreaSpectrogramColormap.setObjectName("scrollAreaSpectrogramColormap") + self.scrollAreaSpectrogramColormap.setObjectName( + "scrollAreaSpectrogramColormap" + ) self.scrollAreaWidgetSpectrogramColormapContents = QtWidgets.QWidget() - self.scrollAreaWidgetSpectrogramColormapContents.setGeometry(QtCore.QRect(0, 0, 762, 466)) - self.scrollAreaWidgetSpectrogramColormapContents.setObjectName("scrollAreaWidgetSpectrogramColormapContents") - self.verticalLayout_4 = QtWidgets.QVBoxLayout(self.scrollAreaWidgetSpectrogramColormapContents) + self.scrollAreaWidgetSpectrogramColormapContents.setGeometry( + QtCore.QRect(0, 0, 762, 466) + ) + self.scrollAreaWidgetSpectrogramColormapContents.setObjectName( + "scrollAreaWidgetSpectrogramColormapContents" + ) + self.verticalLayout_4 = QtWidgets.QVBoxLayout( + self.scrollAreaWidgetSpectrogramColormapContents + ) self.verticalLayout_4.setObjectName("verticalLayout_4") - self.scrollAreaSpectrogramColormap.setWidget(self.scrollAreaWidgetSpectrogramColormapContents) + self.scrollAreaSpectrogramColormap.setWidget( + self.scrollAreaWidgetSpectrogramColormapContents + ) self.verticalLayout_2.addWidget(self.scrollAreaSpectrogramColormap) self.verticalLayout.addWidget(self.groupBoxSpectrogramColormap) self.tabWidget.addTab(self.tabView, "") @@ -161,11 +193,15 @@ def setupUi(self, DialogOptions): self.btnRemoveLabeltype.setIcon(icon) self.btnRemoveLabeltype.setObjectName("btnRemoveLabeltype") self.verticalLayout_3.addWidget(self.btnRemoveLabeltype) - spacerItem1 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_3.addItem(spacerItem1) self.horizontalLayout_3.addLayout(self.verticalLayout_3) self.verticalLayout_5.addLayout(self.horizontalLayout_3) - spacerItem2 = QtWidgets.QSpacerItem(20, 203, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem2 = QtWidgets.QSpacerItem( + 20, 203, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_5.addItem(spacerItem2) self.tabWidget.addTab(self.tabFieldtypes, "") self.tab_plugins = QtWidgets.QWidget() @@ -234,7 +270,9 @@ def setupUi(self, DialogOptions): self.btnRebuildNative.setObjectName("btnRebuildNative") self.gridLayout_5.addWidget(self.btnRebuildNative, 4, 0, 1, 1) self.labelRebuildNativeStatus = QtWidgets.QLabel(self.groupBoxNativeOptions) - self.labelRebuildNativeStatus.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignVCenter) + self.labelRebuildNativeStatus.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignVCenter + ) self.labelRebuildNativeStatus.setObjectName("labelRebuildNativeStatus") self.gridLayout_5.addWidget(self.labelRebuildNativeStatus, 4, 2, 1, 1) self.verticalLayout_8.addWidget(self.groupBoxNativeOptions) @@ -292,59 +330,194 @@ def retranslateUi(self, DialogOptions): _translate = QtCore.QCoreApplication.translate DialogOptions.setWindowTitle(_translate("DialogOptions", "Options")) self.labelFuzzingSamples.setText(_translate("DialogOptions", "Samples")) - self.checkBoxDefaultFuzzingPause.setToolTip(_translate("DialogOptions", "If you disable the default pause, the pause of the fuzzed message will be used.
")) - self.checkBoxDefaultFuzzingPause.setText(_translate("DialogOptions", "Use a default pause for fuzzed messages")) - self.checkBoxMultipleModulations.setText(_translate("DialogOptions", "Enable modulation profiles")) - self.groupBoxModulationAccuracy.setTitle(_translate("DialogOptions", "Modulation Accuracy")) - self.radioButtonLowModulationAccuracy.setText(_translate("DialogOptions", "Low (2x8 bit) - Recommended for HackRF and RTL-SDR")) - self.radioButtonMediumModulationAccuracy.setText(_translate("DialogOptions", "Medium (2x16 bit) - Recommended for BladeRF, PlutoSDR and SDRPlay")) - self.radioButtonHighModulationAccuracy.setText(_translate("DialogOptions", "High (2x32 bit) - Recommended if you are not sure what to choose")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tabGeneration), _translate("DialogOptions", "Generation")) + self.checkBoxDefaultFuzzingPause.setToolTip( + _translate( + "DialogOptions", + "If you disable the default pause, the pause of the fuzzed message will be used.
", + ) + ) + self.checkBoxDefaultFuzzingPause.setText( + _translate("DialogOptions", "Use a default pause for fuzzed messages") + ) + self.checkBoxMultipleModulations.setText( + _translate("DialogOptions", "Enable modulation profiles") + ) + self.groupBoxModulationAccuracy.setTitle( + _translate("DialogOptions", "Modulation Accuracy") + ) + self.radioButtonLowModulationAccuracy.setText( + _translate( + "DialogOptions", "Low (2x8 bit) - Recommended for HackRF and RTL-SDR" + ) + ) + self.radioButtonMediumModulationAccuracy.setText( + _translate( + "DialogOptions", + "Medium (2x16 bit) - Recommended for BladeRF, PlutoSDR and SDRPlay", + ) + ) + self.radioButtonHighModulationAccuracy.setText( + _translate( + "DialogOptions", + "High (2x32 bit) - Recommended if you are not sure what to choose", + ) + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tabGeneration), + _translate("DialogOptions", "Generation"), + ) self.label_7.setText(_translate("DialogOptions", "Default View:")) self.comboBoxDefaultView.setItemText(0, _translate("DialogOptions", "Bit")) self.comboBoxDefaultView.setItemText(1, _translate("DialogOptions", "Hex")) self.comboBoxDefaultView.setItemText(2, _translate("DialogOptions", "ASCII")) - self.checkBoxShowConfirmCloseDialog.setText(_translate("DialogOptions", "Show \"confirm close\" dialog")) - self.checkBoxHoldShiftToDrag.setToolTip(_translate("DialogOptions", "If checked, you need to hold the Shift key to drag with the mouse inside graphic views like the drawn signal in Interpretation tab, while making a selection with the mouse does not require holding any buttons.
If unchecked, this is inverted: Hold shift to make a selection, and drag by default.
")) - self.checkBoxHoldShiftToDrag.setText(_translate("DialogOptions", "Hold shift to drag")) - self.checkBoxPauseTime.setText(_translate("DialogOptions", "Show pauses as time")) + self.checkBoxShowConfirmCloseDialog.setText( + _translate("DialogOptions", 'Show "confirm close" dialog') + ) + self.checkBoxHoldShiftToDrag.setToolTip( + _translate( + "DialogOptions", + 'If checked, you need to hold the Shift key to drag with the mouse inside graphic views like the drawn signal in Interpretation tab, while making a selection with the mouse does not require holding any buttons.
If unchecked, this is inverted: Hold shift to make a selection, and drag by default.
', + ) + ) + self.checkBoxHoldShiftToDrag.setText( + _translate("DialogOptions", "Hold shift to drag") + ) + self.checkBoxPauseTime.setText( + _translate("DialogOptions", "Show pauses as time") + ) self.checkBoxAlignLabels.setText(_translate("DialogOptions", "Align on labels")) - self.labelFontSize.setText(_translate("DialogOptions", "Application font size (restart for full effect):
")) + self.labelFontSize.setText( + _translate( + "DialogOptions", + 'Application font size (restart for full effect):
', + ) + ) self.doubleSpinBoxFontSize.setSuffix(_translate("DialogOptions", "pt")) - self.label_9.setText(_translate("DialogOptions", "Choose application theme (requires restart):")) - self.comboBoxTheme.setItemText(0, _translate("DialogOptions", "native look (default)")) + self.label_9.setText( + _translate("DialogOptions", "Choose application theme (requires restart):") + ) + self.comboBoxTheme.setItemText( + 0, _translate("DialogOptions", "native look (default)") + ) self.comboBoxTheme.setItemText(1, _translate("DialogOptions", "fallback theme")) - self.comboBoxTheme.setItemText(2, _translate("DialogOptions", "fallback theme (dark)")) - self.labelIconTheme.setText(_translate("DialogOptions", "Choose icon theme (requires restart):")) - self.comboBoxIconTheme.setItemText(0, _translate("DialogOptions", "bundled icons (default)")) - self.comboBoxIconTheme.setItemText(1, _translate("DialogOptions", "native icon theme")) - self.groupBoxSpectrogramColormap.setTitle(_translate("DialogOptions", "Spectrogram Colormap")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tabView), _translate("DialogOptions", "View")) + self.comboBoxTheme.setItemText( + 2, _translate("DialogOptions", "fallback theme (dark)") + ) + self.labelIconTheme.setText( + _translate("DialogOptions", "Choose icon theme (requires restart):") + ) + self.comboBoxIconTheme.setItemText( + 0, _translate("DialogOptions", "bundled icons (default)") + ) + self.comboBoxIconTheme.setItemText( + 1, _translate("DialogOptions", "native icon theme") + ) + self.groupBoxSpectrogramColormap.setTitle( + _translate("DialogOptions", "Spectrogram Colormap") + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tabView), _translate("DialogOptions", "View") + ) self.btnAddLabelType.setText(_translate("DialogOptions", "...")) self.btnRemoveLabeltype.setText(_translate("DialogOptions", "...")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tabFieldtypes), _translate("DialogOptions", "Fieldtypes")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_plugins), _translate("DialogOptions", "Plugins")) - self.labelInfoDeviceTable.setText(_translate("DialogOptions", "Use the checkboxes in the table below to choose device backends and enable or disable devices. Disabled devices will not show up in device related dialogs such as send or receive.
")) - self.labelDeviceMissingInfo.setText(_translate("DialogOptions", "Missing a native backend? Perform a health check! If GNU Radio backend is not available double check the GNU Radio settings below.
")) - self.groupBoxNativeOptions.setTitle(_translate("DialogOptions", "Native options")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tabFieldtypes), + _translate("DialogOptions", "Fieldtypes"), + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_plugins), + _translate("DialogOptions", "Plugins"), + ) + self.labelInfoDeviceTable.setText( + _translate( + "DialogOptions", + "Use the checkboxes in the table below to choose device backends and enable or disable devices. Disabled devices will not show up in device related dialogs such as send or receive.
", + ) + ) + self.labelDeviceMissingInfo.setText( + _translate( + "DialogOptions", + 'Missing a native backend? Perform a health check! If GNU Radio backend is not available double check the GNU Radio settings below.
', + ) + ) + self.groupBoxNativeOptions.setTitle( + _translate("DialogOptions", "Native options") + ) self.labelLibDirs.setText(_translate("DialogOptions", "Library directories:")) - self.lineEditIncludeDirs.setPlaceholderText(_translate("DialogOptions", "Comma separated list of additional include directories")) - self.labelNativeRebuildInfo.setText(_translate("DialogOptions", "You can rebuild the native device extensions here. This is useful, when you installed a device driver afterwards or your drivers are stored in an unusual location.")) - self.lineEditLibDirs.setPlaceholderText(_translate("DialogOptions", "Comma separated list of additional library directories")) - self.labelIncludeDirs.setText(_translate("DialogOptions", "Include directories:")) + self.lineEditIncludeDirs.setPlaceholderText( + _translate( + "DialogOptions", + "Comma separated list of additional include directories", + ) + ) + self.labelNativeRebuildInfo.setText( + _translate( + "DialogOptions", + "You can rebuild the native device extensions here. This is useful, when you installed a device driver afterwards or your drivers are stored in an unusual location.", + ) + ) + self.lineEditLibDirs.setPlaceholderText( + _translate( + "DialogOptions", + "Comma separated list of additional library directories", + ) + ) + self.labelIncludeDirs.setText( + _translate("DialogOptions", "Include directories:") + ) self.btnViewBuildLog.setText(_translate("DialogOptions", "View log")) - self.btnRebuildNative.setToolTip(_translate("DialogOptions", "Rebuild the native device extensions. You need to restart URH after this, to use new extensions.
")) + self.btnRebuildNative.setToolTip( + _translate( + "DialogOptions", + "Rebuild the native device extensions. You need to restart URH after this, to use new extensions.
", + ) + ) self.btnRebuildNative.setText(_translate("DialogOptions", "Rebuild")) - self.labelRebuildNativeStatus.setText(_translate("DialogOptions", "RebuildChoose a python interpreter which has access to your GNU Radio installation, that is, you can do python -c "import gnuradio" with it on the command line.
")) + self.labelRebuildNativeStatus.setText( + _translate( + "DialogOptions", + "RebuildChoose a python interpreter which has access to your GNU Radio installation, that is, you can do python -c "import gnuradio" with it on the command line.
', + ) + ) self.label.setText(_translate("DialogOptions", "Python Interpreter:")) - self.lineEditGRPythonInterpreter.setToolTip(_translate("DialogOptions", "Choose a python interpreter which has access to your GNU Radio installation, that is, you can do python -c "import gnuradio" with it on the command line.
")) - self.lineEditGRPythonInterpreter.setPlaceholderText(_translate("DialogOptions", "Enter python interpreter path e.g. /usr/bin/python")) + self.lineEditGRPythonInterpreter.setToolTip( + _translate( + "DialogOptions", + 'Choose a python interpreter which has access to your GNU Radio installation, that is, you can do python -c "import gnuradio" with it on the command line.
', + ) + ) + self.lineEditGRPythonInterpreter.setPlaceholderText( + _translate( + "DialogOptions", "Enter python interpreter path e.g. /usr/bin/python" + ) + ) self.btnChooseGRPythonInterpreter.setText(_translate("DialogOptions", "...")) - self.label_8.setText(_translate("DialogOptions", "Default sending repititions:")) - self.spinBoxNumSendingRepeats.setSpecialValueText(_translate("DialogOptions", "Infinite")) - self.label_5.setText(_translate("DialogOptions", "Use this percentage of available RAM for buffer allocation:")) + self.label_8.setText( + _translate("DialogOptions", "Default sending repititions:") + ) + self.spinBoxNumSendingRepeats.setSpecialValueText( + _translate("DialogOptions", "Infinite") + ) + self.label_5.setText( + _translate( + "DialogOptions", + "Use this percentage of available RAM for buffer allocation:", + ) + ) self.doubleSpinBoxRAMThreshold.setSuffix(_translate("DialogOptions", "%")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tabDevices), _translate("DialogOptions", "Device")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tabDevices), + _translate("DialogOptions", "Device"), + ) + + from urh.ui.KillerDoubleSpinBox import KillerDoubleSpinBox diff --git a/src/urh/ui/ui_plugins.py b/src/urh/ui/ui_plugins.py index ddc4040651..6efa1878f3 100644 --- a/src/urh/ui/ui_plugins.py +++ b/src/urh/ui/ui_plugins.py @@ -22,10 +22,14 @@ def setupUi(self, FramePlugins): self.label.setObjectName("label") self.verticalLayout_2.addWidget(self.label) self.listViewPlugins = QtWidgets.QListView(FramePlugins) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.MinimumExpanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.MinimumExpanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.listViewPlugins.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.listViewPlugins.sizePolicy().hasHeightForWidth() + ) self.listViewPlugins.setSizePolicy(sizePolicy) self.listViewPlugins.setObjectName("listViewPlugins") self.verticalLayout_2.addWidget(self.listViewPlugins) @@ -33,7 +37,9 @@ def setupUi(self, FramePlugins): self.verticalLayout = QtWidgets.QVBoxLayout() self.verticalLayout.setObjectName("verticalLayout") self.label_2 = QtWidgets.QLabel(FramePlugins) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label_2.sizePolicy().hasHeightForWidth()) @@ -41,10 +47,14 @@ def setupUi(self, FramePlugins): self.label_2.setObjectName("label_2") self.verticalLayout.addWidget(self.label_2) self.txtEditPluginDescription = QtWidgets.QTextEdit(FramePlugins) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.txtEditPluginDescription.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.txtEditPluginDescription.sizePolicy().hasHeightForWidth() + ) self.txtEditPluginDescription.setSizePolicy(sizePolicy) self.txtEditPluginDescription.setReadOnly(True) self.txtEditPluginDescription.setPlaceholderText("") @@ -53,10 +63,14 @@ def setupUi(self, FramePlugins): self.horizontalLayout.addLayout(self.verticalLayout) self.verticalLayout_3.addLayout(self.horizontalLayout) self.groupBoxSettings = QtWidgets.QGroupBox(FramePlugins) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.groupBoxSettings.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.groupBoxSettings.sizePolicy().hasHeightForWidth() + ) self.groupBoxSettings.setSizePolicy(sizePolicy) self.groupBoxSettings.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.groupBoxSettings.setObjectName("groupBoxSettings") diff --git a/src/urh/ui/ui_project.py b/src/urh/ui/ui_project.py index 75377c8c98..f47597a5b8 100644 --- a/src/urh/ui/ui_project.py +++ b/src/urh/ui/ui_project.py @@ -25,7 +25,9 @@ def setupUi(self, ProjectDialog): self.lblName = QtWidgets.QLabel(ProjectDialog) self.lblName.setObjectName("lblName") self.verticalLayout.addWidget(self.lblName) - spacerItem = QtWidgets.QSpacerItem(17, 10, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed) + spacerItem = QtWidgets.QSpacerItem( + 17, 10, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed + ) self.verticalLayout.addItem(spacerItem) self.gridLayout = QtWidgets.QGridLayout() self.gridLayout.setObjectName("gridLayout") @@ -43,14 +45,18 @@ def setupUi(self, ProjectDialog): self.lblNewPath = QtWidgets.QLabel(ProjectDialog) self.lblNewPath.setObjectName("lblNewPath") self.gridLayout.addWidget(self.lblNewPath, 1, 3, 1, 1) - spacerItem1 = QtWidgets.QSpacerItem(20, 57, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 57, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout.addItem(spacerItem1, 15, 4, 1, 2) self.txtEdDescription = QtWidgets.QPlainTextEdit(ProjectDialog) self.txtEdDescription.setObjectName("txtEdDescription") self.gridLayout.addWidget(self.txtEdDescription, 10, 3, 1, 1) self.tblParticipants = ParticipantTableView(ProjectDialog) self.tblParticipants.setAlternatingRowColors(True) - self.tblParticipants.setSelectionMode(QtWidgets.QAbstractItemView.ExtendedSelection) + self.tblParticipants.setSelectionMode( + QtWidgets.QAbstractItemView.ExtendedSelection + ) self.tblParticipants.setObjectName("tblParticipants") self.tblParticipants.horizontalHeader().setCascadingSectionResizes(False) self.tblParticipants.horizontalHeader().setDefaultSectionSize(100) @@ -117,7 +123,9 @@ def setupUi(self, ProjectDialog): self.label_2.setObjectName("label_2") self.gridLayout.addWidget(self.label_2, 3, 0, 1, 2) self.label = QtWidgets.QLabel(ProjectDialog) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label.sizePolicy().hasHeightForWidth()) @@ -138,7 +146,9 @@ def setupUi(self, ProjectDialog): self.label_4.setObjectName("label_4") self.gridLayout.addWidget(self.label_4, 16, 0, 1, 2) self.label_8 = QtWidgets.QLabel(ProjectDialog) - self.label_8.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignVCenter) + self.label_8.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignVCenter + ) self.label_8.setObjectName("label_8") self.gridLayout.addWidget(self.label_8, 10, 0, 1, 2) self.label_12 = QtWidgets.QLabel(ProjectDialog) @@ -166,7 +176,9 @@ def setupUi(self, ProjectDialog): self.btnDown.setObjectName("btnDown") self.gridLayout.addWidget(self.btnDown, 14, 4, 1, 1) self.buttonBox = QtWidgets.QDialogButtonBox(ProjectDialog) - self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.Ok) + self.buttonBox.setStandardButtons( + QtWidgets.QDialogButtonBox.Cancel | QtWidgets.QDialogButtonBox.Ok + ) self.buttonBox.setObjectName("buttonBox") self.gridLayout.addWidget(self.buttonBox, 17, 3, 1, 1) self.verticalLayout.addLayout(self.gridLayout) @@ -181,36 +193,65 @@ def setupUi(self, ProjectDialog): ProjectDialog.setTabOrder(self.txtEdDescription, self.tblParticipants) ProjectDialog.setTabOrder(self.tblParticipants, self.btnAddParticipant) ProjectDialog.setTabOrder(self.btnAddParticipant, self.btnRemoveParticipant) - ProjectDialog.setTabOrder(self.btnRemoveParticipant, self.lineEditBroadcastAddress) + ProjectDialog.setTabOrder( + self.btnRemoveParticipant, self.lineEditBroadcastAddress + ) def retranslateUi(self, ProjectDialog): _translate = QtCore.QCoreApplication.translate - ProjectDialog.setWindowTitle(_translate("ProjectDialog", "Create a new project")) + ProjectDialog.setWindowTitle( + _translate("ProjectDialog", "Create a new project") + ) self.lNewProject.setText(_translate("ProjectDialog", "New Project")) self.lblName.setText(_translate("ProjectDialog", "Note: A new directory will be created.
")) + self.lblNewPath.setText( + _translate( + "ProjectDialog", + 'Note: A new directory will be created.
', + ) + ) self.label_3.setText(_translate("ProjectDialog", "Default frequency:")) - self.lineEditBroadcastAddress.setToolTip(_translate("ProjectDialog", "Enter the broadcast address of your protocol in hex. If you do not know what to enter here, just leave the default.
")) + self.lineEditBroadcastAddress.setToolTip( + _translate( + "ProjectDialog", + 'Enter the broadcast address of your protocol in hex. If you do not know what to enter here, just leave the default.
', + ) + ) self.lineEditBroadcastAddress.setText(_translate("ProjectDialog", "ffff")) self.label_10.setText(_translate("ProjectDialog", "Default bandwidth:")) - self.btnAddParticipant.setToolTip(_translate("ProjectDialog", "Add participant")) + self.btnAddParticipant.setToolTip( + _translate("ProjectDialog", "Add participant") + ) self.btnAddParticipant.setText(_translate("ProjectDialog", "...")) self.btnSelectPath.setText(_translate("ProjectDialog", "...")) self.label_6.setText(_translate("ProjectDialog", "Hz")) self.label_2.setText(_translate("ProjectDialog", "Default sample rate:")) self.label.setText(_translate("ProjectDialog", "Choose a path:")) - self.btnRemoveParticipant.setToolTip(_translate("ProjectDialog", "Remove participant")) + self.btnRemoveParticipant.setToolTip( + _translate("ProjectDialog", "Remove participant") + ) self.btnRemoveParticipant.setText(_translate("ProjectDialog", "...")) self.label_4.setText(_translate("ProjectDialog", "Broadcast address (hex):")) self.label_8.setText(_translate("ProjectDialog", "Description:")) self.label_12.setText(_translate("ProjectDialog", "Hz")) self.label_11.setText(_translate("ProjectDialog", "Default gain:")) - self.btnUp.setToolTip(_translate("ProjectDialog", "Move selected participants up")) + self.btnUp.setToolTip( + _translate("ProjectDialog", "Move selected participants up") + ) self.btnUp.setText(_translate("ProjectDialog", "...")) - self.lOpenSpectrumAnalyzer.setText(_translate("ProjectDialog", "Tip: Open spectrum analyzer to find these values.
")) + self.lOpenSpectrumAnalyzer.setText( + _translate( + "ProjectDialog", + 'Tip: Open spectrum analyzer to find these values.
', + ) + ) self.label_9.setText(_translate("ProjectDialog", "Participants:")) - self.btnDown.setToolTip(_translate("ProjectDialog", "Move selected participants down")) + self.btnDown.setToolTip( + _translate("ProjectDialog", "Move selected participants down") + ) self.btnDown.setText(_translate("ProjectDialog", "...")) + + from urh.ui.KillerDoubleSpinBox import KillerDoubleSpinBox from urh.ui.views.ParticipantTableView import ParticipantTableView diff --git a/src/urh/ui/ui_properties_dialog.py b/src/urh/ui/ui_properties_dialog.py index f01e4dbc3e..89d3bddb87 100644 --- a/src/urh/ui/ui_properties_dialog.py +++ b/src/urh/ui/ui_properties_dialog.py @@ -15,14 +15,16 @@ def setupUi(self, DialogLabels): self.verticalLayout_3 = QtWidgets.QVBoxLayout(DialogLabels) self.verticalLayout_3.setObjectName("verticalLayout_3") self.splitter = QtWidgets.QSplitter(DialogLabels) - self.splitter.setStyleSheet("QSplitter::handle:vertical {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -" image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:vertical {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + " image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" + "}" + ) self.splitter.setOrientation(QtCore.Qt.Vertical) self.splitter.setHandleWidth(6) self.splitter.setChildrenCollapsible(False) @@ -32,10 +34,18 @@ def setupUi(self, DialogLabels): self.verticalLayout = QtWidgets.QVBoxLayout(self.groupBoxSettings) self.verticalLayout.setObjectName("verticalLayout") self.tblViewProtoLabels = ProtocolLabelTableView(self.groupBoxSettings) - self.tblViewProtoLabels.setSelectionMode(QtWidgets.QAbstractItemView.ExtendedSelection) - self.tblViewProtoLabels.setSelectionBehavior(QtWidgets.QAbstractItemView.SelectItems) - self.tblViewProtoLabels.setVerticalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) - self.tblViewProtoLabels.setHorizontalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) + self.tblViewProtoLabels.setSelectionMode( + QtWidgets.QAbstractItemView.ExtendedSelection + ) + self.tblViewProtoLabels.setSelectionBehavior( + QtWidgets.QAbstractItemView.SelectItems + ) + self.tblViewProtoLabels.setVerticalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) + self.tblViewProtoLabels.setHorizontalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) self.tblViewProtoLabels.setShowGrid(False) self.tblViewProtoLabels.setObjectName("tblViewProtoLabels") self.verticalLayout.addWidget(self.tblViewProtoLabels) @@ -53,14 +63,18 @@ def setupUi(self, DialogLabels): self.cbProtoView.addItem("") self.cbProtoView.addItem("") self.horizontalLayout_2.addWidget(self.cbProtoView) - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_2.addItem(spacerItem) self.verticalLayout.addLayout(self.horizontalLayout_2) self.groupBoxAdvancedSettings = QtWidgets.QGroupBox(self.splitter) self.groupBoxAdvancedSettings.setObjectName("groupBoxAdvancedSettings") self.verticalLayout_2 = QtWidgets.QVBoxLayout(self.groupBoxAdvancedSettings) self.verticalLayout_2.setObjectName("verticalLayout_2") - self.tabWidgetAdvancedSettings = QtWidgets.QTabWidget(self.groupBoxAdvancedSettings) + self.tabWidgetAdvancedSettings = QtWidgets.QTabWidget( + self.groupBoxAdvancedSettings + ) self.tabWidgetAdvancedSettings.setObjectName("tabWidgetAdvancedSettings") self.verticalLayout_2.addWidget(self.tabWidgetAdvancedSettings) self.verticalLayout_3.addWidget(self.splitter) @@ -72,13 +86,23 @@ def setupUi(self, DialogLabels): def retranslateUi(self, DialogLabels): _translate = QtCore.QCoreApplication.translate - DialogLabels.setWindowTitle(_translate("DialogLabels", "Manage Protocol Labels")) - self.groupBoxSettings.setTitle(_translate("DialogLabels", "Protocol Label Settings")) - self.label.setText(_translate("DialogLabels", "Start/End values refer to view type:")) + DialogLabels.setWindowTitle( + _translate("DialogLabels", "Manage Protocol Labels") + ) + self.groupBoxSettings.setTitle( + _translate("DialogLabels", "Protocol Label Settings") + ) + self.label.setText( + _translate("DialogLabels", "Start/End values refer to view type:") + ) self.cbProtoView.setItemText(0, _translate("DialogLabels", "Bits")) self.cbProtoView.setItemText(1, _translate("DialogLabels", "Hex")) self.cbProtoView.setItemText(2, _translate("DialogLabels", "ASCII")) - self.groupBoxAdvancedSettings.setTitle(_translate("DialogLabels", "Advanced Settings")) + self.groupBoxAdvancedSettings.setTitle( + _translate("DialogLabels", "Advanced Settings") + ) self.btnConfirm.setText(_translate("DialogLabels", "Confirm")) + + from urh.ui.views.ProtocolLabelTableView import ProtocolLabelTableView from . import urh_rc diff --git a/src/urh/ui/ui_send_recv.py b/src/urh/ui/ui_send_recv.py index 40a04c52ec..fd48345b3e 100644 --- a/src/urh/ui/ui_send_recv.py +++ b/src/urh/ui/ui_send_recv.py @@ -17,14 +17,16 @@ def setupUi(self, SendRecvDialog): self.verticalLayout = QtWidgets.QVBoxLayout(SendRecvDialog) self.verticalLayout.setObjectName("verticalLayout") self.splitter = QtWidgets.QSplitter(SendRecvDialog) - self.splitter.setStyleSheet("QSplitter::handle:horizontal {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -"image: url(:/icons/icons/splitter_handle_vertical.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:horizontal {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + "image: url(:/icons/icons/splitter_handle_vertical.svg);\n" + "}" + ) self.splitter.setOrientation(QtCore.Qt.Horizontal) self.splitter.setObjectName("splitter") self.scrollArea = QtWidgets.QScrollArea(self.splitter) @@ -37,10 +39,7 @@ def setupUi(self, SendRecvDialog): self.verticalLayout_8 = QtWidgets.QVBoxLayout(self.scrollAreaWidgetContents_2) self.verticalLayout_8.setObjectName("verticalLayout_8") self.groupBox = QtWidgets.QGroupBox(self.scrollAreaWidgetContents_2) - self.groupBox.setStyleSheet("QGroupBox\n" -"{\n" -" border: none;\n" -"}") + self.groupBox.setStyleSheet("QGroupBox\n" "{\n" " border: none;\n" "}") self.groupBox.setTitle("") self.groupBox.setObjectName("groupBox") self.gridLayout_2 = QtWidgets.QGridLayout(self.groupBox) @@ -67,26 +66,38 @@ def setupUi(self, SendRecvDialog): self.lTimeText.setObjectName("lTimeText") self.gridLayout_2.addWidget(self.lTimeText, 12, 0, 1, 1) self.lSamplesCapturedText = QtWidgets.QLabel(self.groupBox) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesCapturedText.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesCapturedText.sizePolicy().hasHeightForWidth() + ) self.lSamplesCapturedText.setSizePolicy(sizePolicy) self.lSamplesCapturedText.setObjectName("lSamplesCapturedText") self.gridLayout_2.addWidget(self.lSamplesCapturedText, 5, 0, 1, 1) self.lSignalSizeText = QtWidgets.QLabel(self.groupBox) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSignalSizeText.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSignalSizeText.sizePolicy().hasHeightForWidth() + ) self.lSignalSizeText.setSizePolicy(sizePolicy) self.lSignalSizeText.setObjectName("lSignalSizeText") self.gridLayout_2.addWidget(self.lSignalSizeText, 9, 0, 1, 1) self.lSamplesCaptured = QtWidgets.QLabel(self.groupBox) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesCaptured.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesCaptured.sizePolicy().hasHeightForWidth() + ) self.lSamplesCaptured.setSizePolicy(sizePolicy) font = QtGui.QFont() font.setBold(True) @@ -104,7 +115,9 @@ def setupUi(self, SendRecvDialog): self.lTime.setObjectName("lTime") self.gridLayout_2.addWidget(self.lTime, 15, 0, 1, 2) self.lSignalSize = QtWidgets.QLabel(self.groupBox) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lSignalSize.sizePolicy().hasHeightForWidth()) @@ -128,10 +141,14 @@ def setupUi(self, SendRecvDialog): self.lblCurrentRepeatValue.setObjectName("lblCurrentRepeatValue") self.gridLayout_2.addWidget(self.lblCurrentRepeatValue, 17, 0, 1, 1) self.labelReceiveBufferFull = QtWidgets.QLabel(self.groupBox) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.labelReceiveBufferFull.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.labelReceiveBufferFull.sizePolicy().hasHeightForWidth() + ) self.labelReceiveBufferFull.setSizePolicy(sizePolicy) font = QtGui.QFont() font.setBold(True) @@ -142,7 +159,9 @@ def setupUi(self, SendRecvDialog): self.gridLayout_2.addWidget(self.labelReceiveBufferFull, 8, 0, 1, 1) self.horizontalLayout = QtWidgets.QHBoxLayout() self.horizontalLayout.setObjectName("horizontalLayout") - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout.addItem(spacerItem) self.btnStart = QtWidgets.QToolButton(self.groupBox) self.btnStart.setMinimumSize(QtCore.QSize(64, 64)) @@ -176,10 +195,14 @@ def setupUi(self, SendRecvDialog): self.btnClear.setToolButtonStyle(QtCore.Qt.ToolButtonTextUnderIcon) self.btnClear.setObjectName("btnClear") self.horizontalLayout.addWidget(self.btnClear) - spacerItem1 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem1 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout.addItem(spacerItem1) self.gridLayout_2.addLayout(self.horizontalLayout, 1, 0, 1, 2) - spacerItem2 = QtWidgets.QSpacerItem(20, 10, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed) + spacerItem2 = QtWidgets.QSpacerItem( + 20, 10, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed + ) self.gridLayout_2.addItem(spacerItem2, 2, 0, 1, 1) self.verticalLayout_8.addWidget(self.groupBox) self.txtEditErrors = QtWidgets.QTextEdit(self.scrollAreaWidgetContents_2) @@ -199,8 +222,12 @@ def setupUi(self, SendRecvDialog): self.verticalLayout_2 = QtWidgets.QVBoxLayout(self.page_receive) self.verticalLayout_2.setObjectName("verticalLayout_2") self.graphicsViewReceive = LiveGraphicView(self.page_receive) - self.graphicsViewReceive.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) - self.graphicsViewReceive.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAsNeeded) + self.graphicsViewReceive.setVerticalScrollBarPolicy( + QtCore.Qt.ScrollBarAlwaysOff + ) + self.graphicsViewReceive.setHorizontalScrollBarPolicy( + QtCore.Qt.ScrollBarAsNeeded + ) self.graphicsViewReceive.setObjectName("graphicsViewReceive") self.verticalLayout_2.addWidget(self.graphicsViewReceive) self.stackedWidget.addWidget(self.page_receive) @@ -210,7 +237,9 @@ def setupUi(self, SendRecvDialog): self.verticalLayout_3.setObjectName("verticalLayout_3") self.graphicsViewSend = EditableGraphicView(self.page_send) self.graphicsViewSend.setMouseTracking(True) - self.graphicsViewSend.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.TextAntialiasing) + self.graphicsViewSend.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.TextAntialiasing + ) self.graphicsViewSend.setTransformationAnchor(QtWidgets.QGraphicsView.NoAnchor) self.graphicsViewSend.setResizeAnchor(QtWidgets.QGraphicsView.NoAnchor) self.graphicsViewSend.setObjectName("graphicsViewSend") @@ -227,7 +256,9 @@ def setupUi(self, SendRecvDialog): self.verticalLayout_6 = QtWidgets.QVBoxLayout(self.page_continuous_send) self.verticalLayout_6.setObjectName("verticalLayout_6") self.graphicsViewContinuousSend = LiveGraphicView(self.page_continuous_send) - self.graphicsViewContinuousSend.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.TextAntialiasing) + self.graphicsViewContinuousSend.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.TextAntialiasing + ) self.graphicsViewContinuousSend.setObjectName("graphicsViewContinuousSend") self.verticalLayout_6.addWidget(self.graphicsViewContinuousSend) self.stackedWidget.addWidget(self.page_continuous_send) @@ -239,11 +270,19 @@ def setupUi(self, SendRecvDialog): self.graphicsViewFFT.setObjectName("graphicsViewFFT") self.verticalLayout_7.addWidget(self.graphicsViewFFT) self.graphicsViewSpectrogram = QtWidgets.QGraphicsView(self.page_spectrum) - self.graphicsViewSpectrogram.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAsNeeded) - self.graphicsViewSpectrogram.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOff) - self.graphicsViewSpectrogram.setRenderHints(QtGui.QPainter.SmoothPixmapTransform|QtGui.QPainter.TextAntialiasing) + self.graphicsViewSpectrogram.setVerticalScrollBarPolicy( + QtCore.Qt.ScrollBarAsNeeded + ) + self.graphicsViewSpectrogram.setHorizontalScrollBarPolicy( + QtCore.Qt.ScrollBarAlwaysOff + ) + self.graphicsViewSpectrogram.setRenderHints( + QtGui.QPainter.SmoothPixmapTransform | QtGui.QPainter.TextAntialiasing + ) self.graphicsViewSpectrogram.setCacheMode(QtWidgets.QGraphicsView.CacheNone) - self.graphicsViewSpectrogram.setViewportUpdateMode(QtWidgets.QGraphicsView.MinimalViewportUpdate) + self.graphicsViewSpectrogram.setViewportUpdateMode( + QtWidgets.QGraphicsView.MinimalViewportUpdate + ) self.graphicsViewSpectrogram.setObjectName("graphicsViewSpectrogram") self.verticalLayout_7.addWidget(self.graphicsViewSpectrogram) self.verticalLayout_7.setStretch(0, 1) @@ -300,13 +339,21 @@ def retranslateUi(self, SendRecvDialog): _translate = QtCore.QCoreApplication.translate SendRecvDialog.setWindowTitle(_translate("SendRecvDialog", "Record Signal")) self.progressBarMessage.setFormat(_translate("SendRecvDialog", "%v/%m")) - self.labelCurrentMessage.setText(_translate("SendRecvDialog", "Current message:")) - self.lReceiveBufferFullText.setText(_translate("SendRecvDialog", "Receive buffer full:")) + self.labelCurrentMessage.setText( + _translate("SendRecvDialog", "Current message:") + ) + self.lReceiveBufferFullText.setText( + _translate("SendRecvDialog", "Receive buffer full:") + ) self.progressBarSample.setFormat(_translate("SendRecvDialog", "%v/%m")) self.lSamplesSentText.setText(_translate("SendRecvDialog", "Current sample:")) self.lTimeText.setText(_translate("SendRecvDialog", "Time (in seconds):")) - self.lSamplesCapturedText.setText(_translate("SendRecvDialog", "Samples captured:")) - self.lSignalSizeText.setText(_translate("SendRecvDialog", "Signal size (in MiB):")) + self.lSamplesCapturedText.setText( + _translate("SendRecvDialog", "Samples captured:") + ) + self.lSignalSizeText.setText( + _translate("SendRecvDialog", "Signal size (in MiB):") + ) self.lSamplesCaptured.setText(_translate("SendRecvDialog", "0")) self.lTime.setText(_translate("SendRecvDialog", "0")) self.lSignalSize.setText(_translate("SendRecvDialog", "0")) @@ -320,10 +367,23 @@ def retranslateUi(self, SendRecvDialog): self.btnSave.setText(_translate("SendRecvDialog", "Save...")) self.btnClear.setToolTip(_translate("SendRecvDialog", "Clear")) self.btnClear.setText(_translate("SendRecvDialog", "Clear")) - self.label_7.setText(_translate("SendRecvDialog", "Hint: You can edit the raw signal before sending.")) - self.btnAccept.setToolTip(_translate("SendRecvDialog", "Accept the sniffed data and load it into Analysis tab.
")) - self.btnAccept.setText(_translate("SendRecvDialog", "Accept data (Open in Analysis)")) + self.label_7.setText( + _translate( + "SendRecvDialog", "Hint: You can edit the raw signal before sending." + ) + ) + self.btnAccept.setToolTip( + _translate( + "SendRecvDialog", + 'Accept the sniffed data and load it into Analysis tab.
', + ) + ) + self.btnAccept.setText( + _translate("SendRecvDialog", "Accept data (Open in Analysis)") + ) self.label_y_scale.setText(_translate("SendRecvDialog", "Y-Scale")) + + from urh.ui.views.EditableGraphicView import EditableGraphicView from urh.ui.views.LiveGraphicView import LiveGraphicView from . import urh_rc diff --git a/src/urh/ui/ui_send_recv_device_settings.py b/src/urh/ui/ui_send_recv_device_settings.py index 7188ffd99b..622bdb6497 100644 --- a/src/urh/ui/ui_send_recv_device_settings.py +++ b/src/urh/ui/ui_send_recv_device_settings.py @@ -21,20 +21,22 @@ def setupUi(self, FormDeviceSettings): font.setBold(True) font.setWeight(75) self.groupBoxDeviceSettings.setFont(font) - self.groupBoxDeviceSettings.setStyleSheet("QGroupBox\n" -"{\n" -"border: none;\n" -"}\n" -"\n" -"QGroupBox::title {\n" -" subcontrol-origin: margin;\n" -"}\n" -"QGroupBox::indicator:unchecked {\n" -" image: url(:/icons/icons/collapse.svg)\n" -"}\n" -"QGroupBox::indicator:checked {\n" -" image: url(:/icons/icons/uncollapse.svg)\n" -"}") + self.groupBoxDeviceSettings.setStyleSheet( + "QGroupBox\n" + "{\n" + "border: none;\n" + "}\n" + "\n" + "QGroupBox::title {\n" + " subcontrol-origin: margin;\n" + "}\n" + "QGroupBox::indicator:unchecked {\n" + " image: url(:/icons/icons/collapse.svg)\n" + "}\n" + "QGroupBox::indicator:checked {\n" + " image: url(:/icons/icons/uncollapse.svg)\n" + "}" + ) self.groupBoxDeviceSettings.setFlat(True) self.groupBoxDeviceSettings.setCheckable(True) self.groupBoxDeviceSettings.setObjectName("groupBoxDeviceSettings") @@ -101,7 +103,9 @@ def setupUi(self, FormDeviceSettings): self.sliderGain.setObjectName("sliderGain") self.gridLayout_5.addWidget(self.sliderGain, 0, 0, 1, 1) self.spinBoxGain = QtWidgets.QSpinBox(self.frame_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.MinimumExpanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.MinimumExpanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.spinBoxGain.sizePolicy().hasHeightForWidth()) @@ -122,10 +126,14 @@ def setupUi(self, FormDeviceSettings): self.sliderIFGain.setObjectName("sliderIFGain") self.gridLayout_7.addWidget(self.sliderIFGain, 0, 0, 1, 1) self.spinBoxIFGain = QtWidgets.QSpinBox(self.frame_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.MinimumExpanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.MinimumExpanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxIFGain.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxIFGain.sizePolicy().hasHeightForWidth() + ) self.spinBoxIFGain.setSizePolicy(sizePolicy) self.spinBoxIFGain.setObjectName("spinBoxIFGain") self.gridLayout_7.addWidget(self.spinBoxIFGain, 0, 1, 1, 1) @@ -139,7 +147,9 @@ def setupUi(self, FormDeviceSettings): self.comboBoxDeviceIdentifier.setObjectName("comboBoxDeviceIdentifier") self.gridLayout.addWidget(self.comboBoxDeviceIdentifier, 1, 1, 1, 1) self.cbDevice = QtWidgets.QComboBox(self.frame_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.cbDevice.sizePolicy().hasHeightForWidth()) @@ -164,10 +174,14 @@ def setupUi(self, FormDeviceSettings): self.sliderBasebandGain.setObjectName("sliderBasebandGain") self.gridLayout_8.addWidget(self.sliderBasebandGain, 0, 0, 1, 1) self.spinBoxBasebandGain = QtWidgets.QSpinBox(self.frame_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.MinimumExpanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.MinimumExpanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxBasebandGain.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxBasebandGain.sizePolicy().hasHeightForWidth() + ) self.spinBoxBasebandGain.setSizePolicy(sizePolicy) self.spinBoxBasebandGain.setObjectName("spinBoxBasebandGain") self.gridLayout_8.addWidget(self.spinBoxBasebandGain, 0, 1, 1, 1) @@ -231,13 +245,17 @@ def setupUi(self, FormDeviceSettings): self.labelChannel.setObjectName("labelChannel") self.gridLayout.addWidget(self.labelChannel, 3, 0, 1, 1) self.btnLockBWSR = QtWidgets.QToolButton(self.frame_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.btnLockBWSR.sizePolicy().hasHeightForWidth()) self.btnLockBWSR.setSizePolicy(sizePolicy) icon = QtGui.QIcon() - icon.addPixmap(QtGui.QPixmap(":/icons/icons/lock.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon.addPixmap( + QtGui.QPixmap(":/icons/icons/lock.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off + ) self.btnLockBWSR.setIcon(icon) self.btnLockBWSR.setIconSize(QtCore.QSize(16, 16)) self.btnLockBWSR.setCheckable(True) @@ -257,11 +275,15 @@ def setupUi(self, FormDeviceSettings): self.verticalLayout.addWidget(self.groupBoxDeviceSettings) self.retranslateUi(FormDeviceSettings) - self.groupBoxDeviceSettings.toggled['bool'].connect(self.frame_2.setVisible) + self.groupBoxDeviceSettings.toggled["bool"].connect(self.frame_2.setVisible) FormDeviceSettings.setTabOrder(self.groupBoxDeviceSettings, self.cbDevice) FormDeviceSettings.setTabOrder(self.cbDevice, self.comboBoxDeviceIdentifier) - FormDeviceSettings.setTabOrder(self.comboBoxDeviceIdentifier, self.btnRefreshDeviceIdentifier) - FormDeviceSettings.setTabOrder(self.btnRefreshDeviceIdentifier, self.lineEditSubdevice) + FormDeviceSettings.setTabOrder( + self.comboBoxDeviceIdentifier, self.btnRefreshDeviceIdentifier + ) + FormDeviceSettings.setTabOrder( + self.btnRefreshDeviceIdentifier, self.lineEditSubdevice + ) FormDeviceSettings.setTabOrder(self.lineEditSubdevice, self.comboBoxChannel) FormDeviceSettings.setTabOrder(self.comboBoxChannel, self.comboBoxAntenna) FormDeviceSettings.setTabOrder(self.comboBoxAntenna, self.lineEditIP) @@ -275,63 +297,206 @@ def setupUi(self, FormDeviceSettings): FormDeviceSettings.setTabOrder(self.spinBoxGain, self.sliderIFGain) FormDeviceSettings.setTabOrder(self.sliderIFGain, self.spinBoxIFGain) FormDeviceSettings.setTabOrder(self.spinBoxIFGain, self.sliderBasebandGain) - FormDeviceSettings.setTabOrder(self.sliderBasebandGain, self.spinBoxBasebandGain) - FormDeviceSettings.setTabOrder(self.spinBoxBasebandGain, self.spinBoxFreqCorrection) - FormDeviceSettings.setTabOrder(self.spinBoxFreqCorrection, self.comboBoxDirectSampling) + FormDeviceSettings.setTabOrder( + self.sliderBasebandGain, self.spinBoxBasebandGain + ) + FormDeviceSettings.setTabOrder( + self.spinBoxBasebandGain, self.spinBoxFreqCorrection + ) + FormDeviceSettings.setTabOrder( + self.spinBoxFreqCorrection, self.comboBoxDirectSampling + ) FormDeviceSettings.setTabOrder(self.comboBoxDirectSampling, self.spinBoxNRepeat) FormDeviceSettings.setTabOrder(self.spinBoxNRepeat, self.checkBoxDCCorrection) def retranslateUi(self, FormDeviceSettings): _translate = QtCore.QCoreApplication.translate FormDeviceSettings.setWindowTitle(_translate("FormDeviceSettings", "Form")) - self.groupBoxDeviceSettings.setTitle(_translate("FormDeviceSettings", "Device settings")) + self.groupBoxDeviceSettings.setTitle( + _translate("FormDeviceSettings", "Device settings") + ) self.labelAntenna.setText(_translate("FormDeviceSettings", "Antenna:")) - self.labelBasebandGain.setToolTip(_translate("FormDeviceSettings", "The baseband gain is applied to the baseband signal in your software defined radio. The baseband signal is at low frequency and gathered from the RF signal by complex downsampling.
")) - self.labelBasebandGain.setText(_translate("FormDeviceSettings", "Baseband gain:")) - self.lineEditSubdevice.setToolTip(_translate("FormDeviceSettings", "Configure the subdevice of your USRP. For example, B:0 to select a WBX on slot B. You can learn more at http://files.ettus.com/manual/page_configuration.html#config_subdev.
")) - self.labelSubdevice.setToolTip(_translate("FormDeviceSettings", "Configure the subdevice of your USRP. For example, B:0 to select a WBX on slot B. You can learn more at http://files.ettus.com/manual/page_configuration.html#config_subdev.
")) - self.labelSubdevice.setText(_translate("FormDeviceSettings", "Subdevice:
")) + self.labelBasebandGain.setToolTip( + _translate( + "FormDeviceSettings", + 'The baseband gain is applied to the baseband signal in your software defined radio. The baseband signal is at low frequency and gathered from the RF signal by complex downsampling.
', + ) + ) + self.labelBasebandGain.setText( + _translate("FormDeviceSettings", "Baseband gain:") + ) + self.lineEditSubdevice.setToolTip( + _translate( + "FormDeviceSettings", + 'Configure the subdevice of your USRP. For example, B:0 to select a WBX on slot B. You can learn more at http://files.ettus.com/manual/page_configuration.html#config_subdev.
', + ) + ) + self.labelSubdevice.setToolTip( + _translate( + "FormDeviceSettings", + 'Configure the subdevice of your USRP. For example, B:0 to select a WBX on slot B. You can learn more at http://files.ettus.com/manual/page_configuration.html#config_subdev.
', + ) + ) + self.labelSubdevice.setText( + _translate( + "FormDeviceSettings", + "Subdevice:
", + ) + ) self.lineEditIP.setText(_translate("FormDeviceSettings", "127.0.0.1")) - self.btnRefreshDeviceIdentifier.setToolTip(_translate("FormDeviceSettings", "Automatically detect connected SDRs of the above configured type. There is no need to press this button if you have only one SDR of a certain type attached.
")) + self.btnRefreshDeviceIdentifier.setToolTip( + _translate( + "FormDeviceSettings", + 'Automatically detect connected SDRs of the above configured type. There is no need to press this button if you have only one SDR of a certain type attached.
', + ) + ) self.btnRefreshDeviceIdentifier.setText(_translate("FormDeviceSettings", "...")) - self.labelDirectSampling.setToolTip(_translate("FormDeviceSettings", "Set the direct sampling mode. If you do not know what to choose here, just set it to disabled. The native backend is recommended, when using this setting.
")) - self.labelDirectSampling.setText(_translate("FormDeviceSettings", "Direct sampling:")) + self.labelDirectSampling.setToolTip( + _translate( + "FormDeviceSettings", + 'Set the direct sampling mode. If you do not know what to choose here, just set it to disabled. The native backend is recommended, when using this setting.
', + ) + ) + self.labelDirectSampling.setText( + _translate("FormDeviceSettings", "Direct sampling:") + ) self.labelBandwidth.setText(_translate("FormDeviceSettings", "Bandwidth (Hz):")) - self.sliderGain.setToolTip(_translate("FormDeviceSettings", "The gain (more exactly RF gain) is the gain applied to the RF signal. This amplifies the high frequent signal arriving at the antenna of your Software Defined Radio.
")) - self.spinBoxGain.setToolTip(_translate("FormDeviceSettings", "The gain (more exactly RF gain) is the gain applied to the RF signal. This amplifies the high frequent signal arriving at the antenna of your Software Defined Radio.
")) - self.labelSampleRate.setText(_translate("FormDeviceSettings", "Sample rate (Sps):")) - self.sliderIFGain.setToolTip(_translate("FormDeviceSettings", "The IF Gain is applied to the Intermediate Frequency signal in your Software Defined Radio. An IF signal has a lower frequency than the high frequent RF signal, so signal processing can be applied more efficiently.
")) - self.spinBoxIFGain.setToolTip(_translate("FormDeviceSettings", "The IF Gain is applied to the Intermediate Frequency signal in your Software Defined Radio. An IF signal has a lower frequency than the high frequent RF signal, so signal processing can be applied more efficiently.
")) - self.labelFreqCorrection.setToolTip(_translate("FormDeviceSettings", "Set the frequency correction in ppm. If you do not know what to enter here, just leave it to one.
")) - self.labelFreqCorrection.setText(_translate("FormDeviceSettings", "Frequency correction:")) - self.comboBoxDeviceIdentifier.setToolTip(_translate("FormDeviceSettings", "You can enter a device identifier here if you have multiple SDRs of the same type attached to separate them. There is no need to configure this value otherwise. URH will automatically select an attached SDR of the configured type if you leave this value empty.
")) + self.sliderGain.setToolTip( + _translate( + "FormDeviceSettings", + "The gain (more exactly RF gain) is the gain applied to the RF signal. This amplifies the high frequent signal arriving at the antenna of your Software Defined Radio.
", + ) + ) + self.spinBoxGain.setToolTip( + _translate( + "FormDeviceSettings", + "The gain (more exactly RF gain) is the gain applied to the RF signal. This amplifies the high frequent signal arriving at the antenna of your Software Defined Radio.
", + ) + ) + self.labelSampleRate.setText( + _translate("FormDeviceSettings", "Sample rate (Sps):") + ) + self.sliderIFGain.setToolTip( + _translate( + "FormDeviceSettings", + "The IF Gain is applied to the Intermediate Frequency signal in your Software Defined Radio. An IF signal has a lower frequency than the high frequent RF signal, so signal processing can be applied more efficiently.
", + ) + ) + self.spinBoxIFGain.setToolTip( + _translate( + "FormDeviceSettings", + "The IF Gain is applied to the Intermediate Frequency signal in your Software Defined Radio. An IF signal has a lower frequency than the high frequent RF signal, so signal processing can be applied more efficiently.
", + ) + ) + self.labelFreqCorrection.setToolTip( + _translate( + "FormDeviceSettings", + 'Set the frequency correction in ppm. If you do not know what to enter here, just leave it to one.
', + ) + ) + self.labelFreqCorrection.setText( + _translate("FormDeviceSettings", "Frequency correction:") + ) + self.comboBoxDeviceIdentifier.setToolTip( + _translate( + "FormDeviceSettings", + 'You can enter a device identifier here if you have multiple SDRs of the same type attached to separate them. There is no need to configure this value otherwise. URH will automatically select an attached SDR of the configured type if you leave this value empty.
', + ) + ) self.cbDevice.setItemText(0, _translate("FormDeviceSettings", "USRP")) self.cbDevice.setItemText(1, _translate("FormDeviceSettings", "HackRF")) - self.spinBoxNRepeat.setSpecialValueText(_translate("FormDeviceSettings", "Infinite")) - self.sliderBasebandGain.setToolTip(_translate("FormDeviceSettings", "The baseband gain is applied to the baseband signal in your software defined radio. The baseband signal is at low frequency and gathered from the RF signal by complex downsampling.
")) - self.spinBoxBasebandGain.setToolTip(_translate("FormDeviceSettings", "The baseband gain is applied to the baseband signal in your software defined radio. The baseband signal is at low frequency and gathered from the RF signal by complex downsampling.
")) + self.spinBoxNRepeat.setSpecialValueText( + _translate("FormDeviceSettings", "Infinite") + ) + self.sliderBasebandGain.setToolTip( + _translate( + "FormDeviceSettings", + 'The baseband gain is applied to the baseband signal in your software defined radio. The baseband signal is at low frequency and gathered from the RF signal by complex downsampling.
', + ) + ) + self.spinBoxBasebandGain.setToolTip( + _translate( + "FormDeviceSettings", + 'The baseband gain is applied to the baseband signal in your software defined radio. The baseband signal is at low frequency and gathered from the RF signal by complex downsampling.
', + ) + ) self.labelPort.setText(_translate("FormDeviceSettings", "Port number:")) - self.labelGain.setToolTip(_translate("FormDeviceSettings", "The gain (more exactly RF gain) is the gain applied to the RF signal. This amplifies the high frequent signal arriving at the antenna of your Software Defined Radio.
")) + self.labelGain.setToolTip( + _translate( + "FormDeviceSettings", + "The gain (more exactly RF gain) is the gain applied to the RF signal. This amplifies the high frequent signal arriving at the antenna of your Software Defined Radio.
", + ) + ) self.labelGain.setText(_translate("FormDeviceSettings", "Gain:")) - self.checkBoxDCCorrection.setToolTip(_translate("FormDeviceSettings", "Apply DC correction during recording, that is, ensure the captured signal has a mean value of zero.")) - self.checkBoxDCCorrection.setText(_translate("FormDeviceSettings", "Apply DC correction")) - self.labelDeviceIdentifier.setToolTip(_translate("FormDeviceSettings", "You can enter a device identifier here if you have multiple SDRs of the same type attached to separate them. There is no need to configure this value otherwise. URH will automatically select an attached SDR of the configured type if you leave this value empty.
")) - self.labelDeviceIdentifier.setText(_translate("FormDeviceSettings", "Device Identifier:")) + self.checkBoxDCCorrection.setToolTip( + _translate( + "FormDeviceSettings", + "Apply DC correction during recording, that is, ensure the captured signal has a mean value of zero.", + ) + ) + self.checkBoxDCCorrection.setText( + _translate("FormDeviceSettings", "Apply DC correction") + ) + self.labelDeviceIdentifier.setToolTip( + _translate( + "FormDeviceSettings", + 'You can enter a device identifier here if you have multiple SDRs of the same type attached to separate them. There is no need to configure this value otherwise. URH will automatically select an attached SDR of the configured type if you leave this value empty.
', + ) + ) + self.labelDeviceIdentifier.setText( + _translate("FormDeviceSettings", "Device Identifier:") + ) self.labelFreq.setText(_translate("FormDeviceSettings", "Frequency (Hz):")) self.labelIP.setText(_translate("FormDeviceSettings", "IP address:")) - self.spinBoxFreqCorrection.setToolTip(_translate("FormDeviceSettings", "Set the frequency correction in ppm. If you do not know what to enter here, just leave it to one.
")) + self.spinBoxFreqCorrection.setToolTip( + _translate( + "FormDeviceSettings", + 'Set the frequency correction in ppm. If you do not know what to enter here, just leave it to one.
', + ) + ) self.labelNRepeat.setText(_translate("FormDeviceSettings", "Repeat:")) - self.comboBoxDirectSampling.setToolTip(_translate("FormDeviceSettings", "Set the direct sampling mode. If you do not know what to choose here, just set it to disabled. The native backend is recommended, when using this setting.
")) - self.labelDCCorrection.setToolTip(_translate("FormDeviceSettings", "Apply DC correction during recording, that is, ensure the captured signal has a mean value of zero.")) - self.labelDCCorrection.setText(_translate("FormDeviceSettings", "DC correction:")) - self.labelIFGain.setToolTip(_translate("FormDeviceSettings", "The IF Gain is applied to the Intermediate Frequency signal in your Software Defined Radio. An IF signal has a lower frequency than the high frequent RF signal, so signal processing can be applied more efficiently.
")) + self.comboBoxDirectSampling.setToolTip( + _translate( + "FormDeviceSettings", + 'Set the direct sampling mode. If you do not know what to choose here, just set it to disabled. The native backend is recommended, when using this setting.
', + ) + ) + self.labelDCCorrection.setToolTip( + _translate( + "FormDeviceSettings", + "Apply DC correction during recording, that is, ensure the captured signal has a mean value of zero.", + ) + ) + self.labelDCCorrection.setText( + _translate("FormDeviceSettings", "DC correction:") + ) + self.labelIFGain.setToolTip( + _translate( + "FormDeviceSettings", + "The IF Gain is applied to the Intermediate Frequency signal in your Software Defined Radio. An IF signal has a lower frequency than the high frequent RF signal, so signal processing can be applied more efficiently.
", + ) + ) self.labelIFGain.setText(_translate("FormDeviceSettings", "IF Gain:")) self.labelChannel.setText(_translate("FormDeviceSettings", "Channel:")) self.btnLockBWSR.setText(_translate("FormDeviceSettings", "...")) self.label_3.setText(_translate("FormDeviceSettings", "Device:")) - self.labelBiasTee.setToolTip(_translate("FormDeviceSettings", "Enable the bias tee of your SDR, if you have an external LNA connected. Leave this disabled if you have no external LNA attached.
")) + self.labelBiasTee.setToolTip( + _translate( + "FormDeviceSettings", + "Enable the bias tee of your SDR, if you have an external LNA connected. Leave this disabled if you have no external LNA attached.
", + ) + ) self.labelBiasTee.setText(_translate("FormDeviceSettings", "Bias Tee:")) - self.checkBoxBiasTee.setToolTip(_translate("FormDeviceSettings", "Enable the bias tee of your SDR, if you have an external LNA connected. Leave this disabled if you have no external LNA attached.
")) - self.checkBoxBiasTee.setText(_translate("FormDeviceSettings", "Enable Bias Tee")) + self.checkBoxBiasTee.setToolTip( + _translate( + "FormDeviceSettings", + "Enable the bias tee of your SDR, if you have an external LNA connected. Leave this disabled if you have no external LNA attached.
", + ) + ) + self.checkBoxBiasTee.setText( + _translate("FormDeviceSettings", "Enable Bias Tee") + ) + + from urh.ui.KillerDoubleSpinBox import KillerDoubleSpinBox from . import urh_rc diff --git a/src/urh/ui/ui_send_recv_sniff_settings.py b/src/urh/ui/ui_send_recv_sniff_settings.py index 843e885899..62b3f47392 100644 --- a/src/urh/ui/ui_send_recv_sniff_settings.py +++ b/src/urh/ui/ui_send_recv_sniff_settings.py @@ -20,20 +20,22 @@ def setupUi(self, SniffSettings): font.setBold(True) font.setWeight(75) self.groupBoxSniffSettings.setFont(font) - self.groupBoxSniffSettings.setStyleSheet("QGroupBox\n" -"{\n" -"border: none;\n" -"}\n" -"\n" -"QGroupBox::title {\n" -" subcontrol-origin: margin;\n" -"}\n" -"QGroupBox::indicator:unchecked {\n" -" image: url(:/icons/icons/collapse.svg)\n" -"}\n" -"QGroupBox::indicator:checked {\n" -" image: url(:/icons/icons/uncollapse.svg)\n" -"}") + self.groupBoxSniffSettings.setStyleSheet( + "QGroupBox\n" + "{\n" + "border: none;\n" + "}\n" + "\n" + "QGroupBox::title {\n" + " subcontrol-origin: margin;\n" + "}\n" + "QGroupBox::indicator:unchecked {\n" + " image: url(:/icons/icons/collapse.svg)\n" + "}\n" + "QGroupBox::indicator:checked {\n" + " image: url(:/icons/icons/uncollapse.svg)\n" + "}" + ) self.groupBoxSniffSettings.setFlat(True) self.groupBoxSniffSettings.setCheckable(True) self.groupBoxSniffSettings.setObjectName("groupBoxSniffSettings") @@ -53,10 +55,14 @@ def setupUi(self, SniffSettings): self.horizontalLayout_3 = QtWidgets.QHBoxLayout() self.horizontalLayout_3.setObjectName("horizontalLayout_3") self.spinbox_sniff_Noise = QtWidgets.QDoubleSpinBox(self.frame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinbox_sniff_Noise.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinbox_sniff_Noise.sizePolicy().hasHeightForWidth() + ) self.spinbox_sniff_Noise.setSizePolicy(sizePolicy) self.spinbox_sniff_Noise.setDecimals(4) self.spinbox_sniff_Noise.setMaximum(1.0) @@ -89,16 +95,22 @@ def setupUi(self, SniffSettings): self.spinbox_sniff_SamplesPerSymbol = QtWidgets.QSpinBox(self.frame) self.spinbox_sniff_SamplesPerSymbol.setMinimum(1) self.spinbox_sniff_SamplesPerSymbol.setMaximum(999999999) - self.spinbox_sniff_SamplesPerSymbol.setObjectName("spinbox_sniff_SamplesPerSymbol") + self.spinbox_sniff_SamplesPerSymbol.setObjectName( + "spinbox_sniff_SamplesPerSymbol" + ) self.gridLayout.addWidget(self.spinbox_sniff_SamplesPerSymbol, 4, 1, 1, 1) self.label_sniff_viewtype = QtWidgets.QLabel(self.frame) self.label_sniff_viewtype.setObjectName("label_sniff_viewtype") self.gridLayout.addWidget(self.label_sniff_viewtype, 9, 0, 1, 1) self.lineEdit_sniff_OutputFile = QtWidgets.QLineEdit(self.frame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lineEdit_sniff_OutputFile.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lineEdit_sniff_OutputFile.sizePolicy().hasHeightForWidth() + ) self.lineEdit_sniff_OutputFile.setSizePolicy(sizePolicy) self.lineEdit_sniff_OutputFile.setReadOnly(False) self.lineEdit_sniff_OutputFile.setClearButtonEnabled(True) @@ -115,10 +127,14 @@ def setupUi(self, SniffSettings): self.horizontalLayout_2 = QtWidgets.QHBoxLayout() self.horizontalLayout_2.setObjectName("horizontalLayout_2") self.comboBox_sniff_signal = QtWidgets.QComboBox(self.frame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.comboBox_sniff_signal.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.comboBox_sniff_signal.sizePolicy().hasHeightForWidth() + ) self.comboBox_sniff_signal.setSizePolicy(sizePolicy) self.comboBox_sniff_signal.setObjectName("comboBox_sniff_signal") self.horizontalLayout_2.addWidget(self.comboBox_sniff_signal) @@ -132,10 +148,14 @@ def setupUi(self, SniffSettings): self.horizontalLayout_4 = QtWidgets.QHBoxLayout() self.horizontalLayout_4.setObjectName("horizontalLayout_4") self.spinbox_sniff_Center = QtWidgets.QDoubleSpinBox(self.frame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinbox_sniff_Center.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinbox_sniff_Center.sizePolicy().hasHeightForWidth() + ) self.spinbox_sniff_Center.setSizePolicy(sizePolicy) self.spinbox_sniff_Center.setDecimals(4) self.spinbox_sniff_Center.setMinimum(-3.14) @@ -166,10 +186,14 @@ def setupUi(self, SniffSettings): self.label_sniff_Noise.setObjectName("label_sniff_Noise") self.gridLayout.addWidget(self.label_sniff_Noise, 1, 0, 1, 1) self.comboBox_sniff_encoding = QtWidgets.QComboBox(self.frame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.comboBox_sniff_encoding.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.comboBox_sniff_encoding.sizePolicy().hasHeightForWidth() + ) self.comboBox_sniff_encoding.setSizePolicy(sizePolicy) self.comboBox_sniff_encoding.setObjectName("comboBox_sniff_encoding") self.gridLayout.addWidget(self.comboBox_sniff_encoding, 8, 1, 1, 1) @@ -188,41 +212,76 @@ def setupUi(self, SniffSettings): self.verticalLayout.addWidget(self.groupBoxSniffSettings) self.retranslateUi(SniffSettings) - self.groupBoxSniffSettings.toggled['bool'].connect(self.frame.setVisible) + self.groupBoxSniffSettings.toggled["bool"].connect(self.frame.setVisible) SniffSettings.setTabOrder(self.groupBoxSniffSettings, self.spinbox_sniff_Noise) - SniffSettings.setTabOrder(self.spinbox_sniff_Noise, self.spinbox_sniff_SamplesPerSymbol) - SniffSettings.setTabOrder(self.spinbox_sniff_SamplesPerSymbol, self.spinbox_sniff_ErrorTolerance) - SniffSettings.setTabOrder(self.spinbox_sniff_ErrorTolerance, self.combox_sniff_Modulation) - SniffSettings.setTabOrder(self.combox_sniff_Modulation, self.comboBox_sniff_encoding) - SniffSettings.setTabOrder(self.comboBox_sniff_encoding, self.comboBox_sniff_viewtype) - SniffSettings.setTabOrder(self.comboBox_sniff_viewtype, self.checkBox_sniff_Timestamp) - SniffSettings.setTabOrder(self.checkBox_sniff_Timestamp, self.lineEdit_sniff_OutputFile) + SniffSettings.setTabOrder( + self.spinbox_sniff_Noise, self.spinbox_sniff_SamplesPerSymbol + ) + SniffSettings.setTabOrder( + self.spinbox_sniff_SamplesPerSymbol, self.spinbox_sniff_ErrorTolerance + ) + SniffSettings.setTabOrder( + self.spinbox_sniff_ErrorTolerance, self.combox_sniff_Modulation + ) + SniffSettings.setTabOrder( + self.combox_sniff_Modulation, self.comboBox_sniff_encoding + ) + SniffSettings.setTabOrder( + self.comboBox_sniff_encoding, self.comboBox_sniff_viewtype + ) + SniffSettings.setTabOrder( + self.comboBox_sniff_viewtype, self.checkBox_sniff_Timestamp + ) + SniffSettings.setTabOrder( + self.checkBox_sniff_Timestamp, self.lineEdit_sniff_OutputFile + ) def retranslateUi(self, SniffSettings): _translate = QtCore.QCoreApplication.translate SniffSettings.setWindowTitle(_translate("SniffSettings", "Form")) - self.groupBoxSniffSettings.setTitle(_translate("SniffSettings", "Sniff settings")) + self.groupBoxSniffSettings.setTitle( + _translate("SniffSettings", "Sniff settings") + ) self.label_sniff_Center.setText(_translate("SniffSettings", "Center:")) - self.checkBoxAdaptiveNoise.setToolTip(_translate("SniffSettings", "With adaptive noise URH will update the noise level automatically during RX. This is helpful in a dynamic environment where noise differs in time.
")) + self.checkBoxAdaptiveNoise.setToolTip( + _translate( + "SniffSettings", + "With adaptive noise URH will update the noise level automatically during RX. This is helpful in a dynamic environment where noise differs in time.
", + ) + ) self.checkBoxAdaptiveNoise.setText(_translate("SniffSettings", "Adaptive")) self.label_sniff_Modulation.setText(_translate("SniffSettings", "Modulation:")) self.label_sniff_Signal.setText(_translate("SniffSettings", "Use values from:")) self.combox_sniff_Modulation.setItemText(0, _translate("SniffSettings", "ASK")) self.combox_sniff_Modulation.setItemText(1, _translate("SniffSettings", "FSK")) self.combox_sniff_Modulation.setItemText(2, _translate("SniffSettings", "PSK")) - self.label_sniff_Tolerance.setText(_translate("SniffSettings", "Error Tolerance:")) + self.label_sniff_Tolerance.setText( + _translate("SniffSettings", "Error Tolerance:") + ) self.label_sniff_viewtype.setText(_translate("SniffSettings", "View:")) - self.lineEdit_sniff_OutputFile.setPlaceholderText(_translate("SniffSettings", "None")) - self.label_sniff_BitLength.setText(_translate("SniffSettings", "Samples per Symbol:")) + self.lineEdit_sniff_OutputFile.setPlaceholderText( + _translate("SniffSettings", "None") + ) + self.label_sniff_BitLength.setText( + _translate("SniffSettings", "Samples per Symbol:") + ) self.btn_sniff_use_signal.setText(_translate("SniffSettings", "Use")) - self.label_sniff_OutputFile.setText(_translate("SniffSettings", "Write bitstream to file:")) + self.label_sniff_OutputFile.setText( + _translate("SniffSettings", "Write bitstream to file:") + ) self.checkBoxAutoCenter.setText(_translate("SniffSettings", "Automatic")) self.label_sniff_encoding.setText(_translate("SniffSettings", "Encoding:")) self.comboBox_sniff_viewtype.setItemText(0, _translate("SniffSettings", "Bit")) self.comboBox_sniff_viewtype.setItemText(1, _translate("SniffSettings", "Hex")) - self.comboBox_sniff_viewtype.setItemText(2, _translate("SniffSettings", "ASCII")) - self.checkBox_sniff_Timestamp.setText(_translate("SniffSettings", "Show Timestamp")) + self.comboBox_sniff_viewtype.setItemText( + 2, _translate("SniffSettings", "ASCII") + ) + self.checkBox_sniff_Timestamp.setText( + _translate("SniffSettings", "Show Timestamp") + ) self.label_sniff_Noise.setText(_translate("SniffSettings", "Noise:")) self.labelCenterSpacing.setText(_translate("SniffSettings", "Center Spacing:")) self.labelBitsPerSymbol.setText(_translate("SniffSettings", "Bits per Symbol:")) + + from . import urh_rc diff --git a/src/urh/ui/ui_signal_details.py b/src/urh/ui/ui_signal_details.py index 885bdb6004..2595d369f7 100644 --- a/src/urh/ui/ui_signal_details.py +++ b/src/urh/ui/ui_signal_details.py @@ -16,13 +16,15 @@ def setupUi(self, SignalDetails): self.verticalLayout.setObjectName("verticalLayout") self.gridLayout = QtWidgets.QGridLayout() self.gridLayout.setObjectName("gridLayout") - spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout.addItem(spacerItem, 2, 2, 1, 1) self.dsb_sample_rate = KillerDoubleSpinBox(SignalDetails) self.dsb_sample_rate.setWrapping(False) self.dsb_sample_rate.setProperty("showGroupSeparator", False) self.dsb_sample_rate.setMinimum(0.01) - self.dsb_sample_rate.setMaximum(1e+33) + self.dsb_sample_rate.setMaximum(1e33) self.dsb_sample_rate.setProperty("value", 1000000.0) self.dsb_sample_rate.setObjectName("dsb_sample_rate") self.gridLayout.addWidget(self.dsb_sample_rate, 5, 1, 1, 1) @@ -30,12 +32,18 @@ def setupUi(self, SignalDetails): self.label.setObjectName("label") self.gridLayout.addWidget(self.label, 0, 0, 1, 1) self.lblFile = QtWidgets.QLabel(SignalDetails) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lblFile.sizePolicy().hasHeightForWidth()) self.lblFile.setSizePolicy(sizePolicy) - self.lblFile.setTextInteractionFlags(QtCore.Qt.LinksAccessibleByMouse|QtCore.Qt.TextSelectableByKeyboard|QtCore.Qt.TextSelectableByMouse) + self.lblFile.setTextInteractionFlags( + QtCore.Qt.LinksAccessibleByMouse + | QtCore.Qt.TextSelectableByKeyboard + | QtCore.Qt.TextSelectableByMouse + ) self.lblFile.setObjectName("lblFile") self.gridLayout.addWidget(self.lblFile, 1, 1, 1, 1) self.label_2 = QtWidgets.QLabel(SignalDetails) @@ -54,12 +62,18 @@ def setupUi(self, SignalDetails): self.label_3.setObjectName("label_3") self.gridLayout.addWidget(self.label_3, 2, 0, 1, 1) self.lblName = QtWidgets.QLabel(SignalDetails) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lblName.sizePolicy().hasHeightForWidth()) self.lblName.setSizePolicy(sizePolicy) - self.lblName.setTextInteractionFlags(QtCore.Qt.LinksAccessibleByMouse|QtCore.Qt.TextSelectableByKeyboard|QtCore.Qt.TextSelectableByMouse) + self.lblName.setTextInteractionFlags( + QtCore.Qt.LinksAccessibleByMouse + | QtCore.Qt.TextSelectableByKeyboard + | QtCore.Qt.TextSelectableByMouse + ) self.lblName.setObjectName("lblName") self.gridLayout.addWidget(self.lblName, 0, 1, 1, 1) self.lblFileSize = QtWidgets.QLabel(SignalDetails) @@ -78,7 +92,9 @@ def setupUi(self, SignalDetails): self.lDuration.setObjectName("lDuration") self.gridLayout.addWidget(self.lDuration, 6, 1, 1, 1) self.verticalLayout.addLayout(self.gridLayout) - spacerItem1 = QtWidgets.QSpacerItem(20, 135, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 135, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout.addItem(spacerItem1) self.retranslateUi(SignalDetails) @@ -99,4 +115,6 @@ def retranslateUi(self, SignalDetails): self.lFileCreated.setText(_translate("SignalDetails", "TextLabel")) self.label_7.setText(_translate("SignalDetails", "Duration:")) self.lDuration.setText(_translate("SignalDetails", "42s")) + + from urh.ui.KillerDoubleSpinBox import KillerDoubleSpinBox diff --git a/src/urh/ui/ui_signal_frame.py b/src/urh/ui/ui_signal_frame.py index 6f172b4b72..55a70adeba 100644 --- a/src/urh/ui/ui_signal_frame.py +++ b/src/urh/ui/ui_signal_frame.py @@ -13,7 +13,9 @@ class Ui_SignalFrame(object): def setupUi(self, SignalFrame): SignalFrame.setObjectName("SignalFrame") SignalFrame.resize(1057, 652) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Maximum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(SignalFrame.sizePolicy().hasHeightForWidth()) @@ -35,10 +37,14 @@ def setupUi(self, SignalFrame): self.gridLayout_2.setSizeConstraint(QtWidgets.QLayout.SetFixedSize) self.gridLayout_2.setObjectName("gridLayout_2") self.lSignalViewText = QtWidgets.QLabel(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSignalViewText.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSignalViewText.sizePolicy().hasHeightForWidth() + ) self.lSignalViewText.setSizePolicy(sizePolicy) font = QtGui.QFont() font.setUnderline(False) @@ -59,13 +65,19 @@ def setupUi(self, SignalFrame): self.btnSaveSignal.setIcon(icon) self.btnSaveSignal.setObjectName("btnSaveSignal") self.gridLayout.addWidget(self.btnSaveSignal, 0, 3, 1, 1) - spacerItem = QtWidgets.QSpacerItem(10, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 10, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.gridLayout.addItem(spacerItem, 0, 2, 1, 1) self.btnCloseSignal = QtWidgets.QToolButton(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.btnCloseSignal.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.btnCloseSignal.sizePolicy().hasHeightForWidth() + ) self.btnCloseSignal.setSizePolicy(sizePolicy) self.btnCloseSignal.setMinimumSize(QtCore.QSize(24, 24)) self.btnCloseSignal.setMaximumSize(QtCore.QSize(24, 24)) @@ -75,7 +87,9 @@ def setupUi(self, SignalFrame): self.btnCloseSignal.setObjectName("btnCloseSignal") self.gridLayout.addWidget(self.btnCloseSignal, 0, 9, 1, 1) self.lSignalTyp = QtWidgets.QLabel(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Fixed, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lSignalTyp.sizePolicy().hasHeightForWidth()) @@ -83,7 +97,9 @@ def setupUi(self, SignalFrame): self.lSignalTyp.setObjectName("lSignalTyp") self.gridLayout.addWidget(self.lSignalTyp, 0, 1, 1, 1) self.lSignalNr = QtWidgets.QLabel(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Maximum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lSignalNr.sizePolicy().hasHeightForWidth()) @@ -124,29 +140,41 @@ def setupUi(self, SignalFrame): self.spinBoxTolerance.setObjectName("spinBoxTolerance") self.gridLayout_2.addWidget(self.spinBoxTolerance, 8, 1, 1, 1) self.lSamplesPerSymbol = QtWidgets.QLabel(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lSamplesPerSymbol.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lSamplesPerSymbol.sizePolicy().hasHeightForWidth() + ) self.lSamplesPerSymbol.setSizePolicy(sizePolicy) self.lSamplesPerSymbol.setTextInteractionFlags(QtCore.Qt.LinksAccessibleByMouse) self.lSamplesPerSymbol.setObjectName("lSamplesPerSymbol") self.gridLayout_2.addWidget(self.lSamplesPerSymbol, 5, 0, 1, 1) self.lErrorTolerance = QtWidgets.QLabel(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lErrorTolerance.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lErrorTolerance.sizePolicy().hasHeightForWidth() + ) self.lErrorTolerance.setSizePolicy(sizePolicy) self.lErrorTolerance.setMinimumSize(QtCore.QSize(0, 0)) self.lErrorTolerance.setMaximumSize(QtCore.QSize(16777215, 16777215)) self.lErrorTolerance.setObjectName("lErrorTolerance") self.gridLayout_2.addWidget(self.lErrorTolerance, 8, 0, 1, 1) self.lCenterOffset = QtWidgets.QLabel(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lCenterOffset.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lCenterOffset.sizePolicy().hasHeightForWidth() + ) self.lCenterOffset.setSizePolicy(sizePolicy) self.lCenterOffset.setMinimumSize(QtCore.QSize(0, 0)) self.lCenterOffset.setMaximumSize(QtCore.QSize(16777215, 16777215)) @@ -157,7 +185,9 @@ def setupUi(self, SignalFrame): self.labelModulation.setObjectName("labelModulation") self.gridLayout_2.addWidget(self.labelModulation, 10, 0, 1, 1) self.cbSignalView = QtWidgets.QComboBox(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.cbSignalView.sizePolicy().hasHeightForWidth()) @@ -184,10 +214,14 @@ def setupUi(self, SignalFrame): self.spinBoxCenterSpacing.setObjectName("spinBoxCenterSpacing") self.gridLayout_2.addWidget(self.spinBoxCenterSpacing, 4, 1, 1, 1) self.lineEditSignalName = QtWidgets.QLineEdit(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lineEditSignalName.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lineEditSignalName.sizePolicy().hasHeightForWidth() + ) self.lineEditSignalName.setSizePolicy(sizePolicy) self.lineEditSignalName.setMinimumSize(QtCore.QSize(214, 0)) self.lineEditSignalName.setMaximumSize(QtCore.QSize(16777215, 16777215)) @@ -207,7 +241,9 @@ def setupUi(self, SignalFrame): icon = QtGui.QIcon.fromTheme("configure") self.btnAdvancedModulationSettings.setIcon(icon) self.btnAdvancedModulationSettings.setIconSize(QtCore.QSize(16, 16)) - self.btnAdvancedModulationSettings.setObjectName("btnAdvancedModulationSettings") + self.btnAdvancedModulationSettings.setObjectName( + "btnAdvancedModulationSettings" + ) self.horizontalLayout_5.addWidget(self.btnAdvancedModulationSettings) self.gridLayout_2.addLayout(self.horizontalLayout_5, 10, 1, 1, 1) self.chkBoxSyncSelection = QtWidgets.QCheckBox(SignalFrame) @@ -230,10 +266,14 @@ def setupUi(self, SignalFrame): self.spinBoxSamplesPerSymbol.setObjectName("spinBoxSamplesPerSymbol") self.gridLayout_2.addWidget(self.spinBoxSamplesPerSymbol, 5, 1, 1, 1) self.btnAutoDetect = QtWidgets.QToolButton(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.btnAutoDetect.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.btnAutoDetect.sizePolicy().hasHeightForWidth() + ) self.btnAutoDetect.setSizePolicy(sizePolicy) self.btnAutoDetect.setIconSize(QtCore.QSize(16, 16)) self.btnAutoDetect.setCheckable(False) @@ -248,10 +288,14 @@ def setupUi(self, SignalFrame): self.line.setObjectName("line") self.gridLayout_2.addWidget(self.line, 15, 0, 1, 2) self.sliderSpectrogramMin = QtWidgets.QSlider(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.sliderSpectrogramMin.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.sliderSpectrogramMin.sizePolicy().hasHeightForWidth() + ) self.sliderSpectrogramMin.setSizePolicy(sizePolicy) self.sliderSpectrogramMin.setMinimum(-150) self.sliderSpectrogramMin.setMaximum(10) @@ -262,10 +306,14 @@ def setupUi(self, SignalFrame): self.labelFFTWindowSize.setObjectName("labelFFTWindowSize") self.gridLayout_2.addWidget(self.labelFFTWindowSize, 20, 0, 1, 1) self.sliderSpectrogramMax = QtWidgets.QSlider(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.sliderSpectrogramMax.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.sliderSpectrogramMax.sizePolicy().hasHeightForWidth() + ) self.sliderSpectrogramMax.setSizePolicy(sizePolicy) self.sliderSpectrogramMax.setMinimum(-150) self.sliderSpectrogramMax.setMaximum(10) @@ -284,13 +332,19 @@ def setupUi(self, SignalFrame): self.labelNoise = QtWidgets.QLabel(SignalFrame) self.labelNoise.setObjectName("labelNoise") self.gridLayout_2.addWidget(self.labelNoise, 2, 0, 1, 1) - spacerItem1 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_2.addItem(spacerItem1, 14, 0, 1, 1) self.sliderFFTWindowSize = QtWidgets.QSlider(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.sliderFFTWindowSize.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.sliderFFTWindowSize.sizePolicy().hasHeightForWidth() + ) self.sliderFFTWindowSize.setSizePolicy(sizePolicy) self.sliderFFTWindowSize.setMinimum(6) self.sliderFFTWindowSize.setMaximum(15) @@ -302,19 +356,23 @@ def setupUi(self, SignalFrame): self.gridLayout_2.addWidget(self.lBitsPerSymbol, 11, 0, 1, 1) self.horizontalLayout.addLayout(self.gridLayout_2) self.splitter = QtWidgets.QSplitter(SignalFrame) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.splitter.sizePolicy().hasHeightForWidth()) self.splitter.setSizePolicy(sizePolicy) - self.splitter.setStyleSheet("QSplitter::handle:vertical {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -" image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:vertical {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + " image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" + "}" + ) self.splitter.setFrameShape(QtWidgets.QFrame.NoFrame) self.splitter.setLineWidth(1) self.splitter.setOrientation(QtCore.Qt.Vertical) @@ -340,7 +398,9 @@ def setupUi(self, SignalFrame): self.horizontalLayout_6.setObjectName("horizontalLayout_6") self.gvSignal = EpicGraphicView(self.pageSignal) self.gvSignal.setEnabled(True) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.gvSignal.sizePolicy().hasHeightForWidth()) @@ -357,14 +417,21 @@ def setupUi(self, SignalFrame): self.gvSignal.setVerticalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOn) self.gvSignal.setHorizontalScrollBarPolicy(QtCore.Qt.ScrollBarAlwaysOn) self.gvSignal.setInteractive(False) - self.gvSignal.setRenderHints(QtGui.QPainter.Antialiasing|QtGui.QPainter.TextAntialiasing) + self.gvSignal.setRenderHints( + QtGui.QPainter.Antialiasing | QtGui.QPainter.TextAntialiasing + ) self.gvSignal.setDragMode(QtWidgets.QGraphicsView.NoDrag) self.gvSignal.setCacheMode(QtWidgets.QGraphicsView.CacheNone) self.gvSignal.setTransformationAnchor(QtWidgets.QGraphicsView.NoAnchor) self.gvSignal.setResizeAnchor(QtWidgets.QGraphicsView.NoAnchor) - self.gvSignal.setViewportUpdateMode(QtWidgets.QGraphicsView.MinimalViewportUpdate) + self.gvSignal.setViewportUpdateMode( + QtWidgets.QGraphicsView.MinimalViewportUpdate + ) self.gvSignal.setRubberBandSelectionMode(QtCore.Qt.ContainsItemShape) - self.gvSignal.setOptimizationFlags(QtWidgets.QGraphicsView.DontClipPainter|QtWidgets.QGraphicsView.DontSavePainterState) + self.gvSignal.setOptimizationFlags( + QtWidgets.QGraphicsView.DontClipPainter + | QtWidgets.QGraphicsView.DontSavePainterState + ) self.gvSignal.setObjectName("gvSignal") self.horizontalLayout_6.addWidget(self.gvSignal) self.stackedWidget.addWidget(self.pageSignal) @@ -381,8 +448,13 @@ def setupUi(self, SignalFrame): self.gvSpectrogram.setRenderHints(QtGui.QPainter.TextAntialiasing) self.gvSpectrogram.setCacheMode(QtWidgets.QGraphicsView.CacheNone) self.gvSpectrogram.setTransformationAnchor(QtWidgets.QGraphicsView.NoAnchor) - self.gvSpectrogram.setViewportUpdateMode(QtWidgets.QGraphicsView.MinimalViewportUpdate) - self.gvSpectrogram.setOptimizationFlags(QtWidgets.QGraphicsView.DontClipPainter|QtWidgets.QGraphicsView.DontSavePainterState) + self.gvSpectrogram.setViewportUpdateMode( + QtWidgets.QGraphicsView.MinimalViewportUpdate + ) + self.gvSpectrogram.setOptimizationFlags( + QtWidgets.QGraphicsView.DontClipPainter + | QtWidgets.QGraphicsView.DontSavePainterState + ) self.gvSpectrogram.setObjectName("gvSpectrogram") self.horizontalLayout_4.addWidget(self.gvSpectrogram) self.stackedWidget.addWidget(self.pageSpectrogram) @@ -395,14 +467,18 @@ def setupUi(self, SignalFrame): font.setPointSize(12) self.labelLoadingAutoInterpretation.setFont(font) self.labelLoadingAutoInterpretation.setWordWrap(True) - self.labelLoadingAutoInterpretation.setObjectName("labelLoadingAutoInterpretation") + self.labelLoadingAutoInterpretation.setObjectName( + "labelLoadingAutoInterpretation" + ) self.verticalLayout_2.addWidget(self.labelLoadingAutoInterpretation) self.stackedWidget.addWidget(self.pageLoading) self.horizontalLayout_2.addWidget(self.stackedWidget) self.verticalLayout_5 = QtWidgets.QVBoxLayout() self.verticalLayout_5.setObjectName("verticalLayout_5") self.lYScale = QtWidgets.QLabel(self.layoutWidget) - self.lYScale.setLocale(QtCore.QLocale(QtCore.QLocale.English, QtCore.QLocale.UnitedStates)) + self.lYScale.setLocale( + QtCore.QLocale(QtCore.QLocale.English, QtCore.QLocale.UnitedStates) + ) self.lYScale.setObjectName("lYScale") self.verticalLayout_5.addWidget(self.lYScale) self.sliderYScale = QtWidgets.QSlider(self.layoutWidget) @@ -417,10 +493,14 @@ def setupUi(self, SignalFrame): self.horizontalLayout_3 = QtWidgets.QHBoxLayout() self.horizontalLayout_3.setObjectName("horizontalLayout_3") self.btnShowHideStartEnd = QtWidgets.QToolButton(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.btnShowHideStartEnd.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.btnShowHideStartEnd.sizePolicy().hasHeightForWidth() + ) self.btnShowHideStartEnd.setSizePolicy(sizePolicy) self.btnShowHideStartEnd.setAutoFillBackground(False) self.btnShowHideStartEnd.setStyleSheet("") @@ -452,7 +532,9 @@ def setupUi(self, SignalFrame): self.labelRSSI = QtWidgets.QLabel(self.layoutWidget) self.labelRSSI.setObjectName("labelRSSI") self.horizontalLayout_3.addWidget(self.labelRSSI) - spacerItem2 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem2 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_3.addItem(spacerItem2) self.btnFilter = QtWidgets.QToolButton(self.layoutWidget) icon = QtGui.QIcon.fromTheme("view-filter") @@ -481,10 +563,14 @@ def setupUi(self, SignalFrame): self.spinBoxSelectionEnd.setMaximum(99999999) self.spinBoxSelectionEnd.setObjectName("spinBoxSelectionEnd") self.additionalInfos.addWidget(self.spinBoxSelectionEnd) - spacerItem3 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem3 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.additionalInfos.addItem(spacerItem3) self.lZoomText = QtWidgets.QLabel(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Minimum) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Minimum + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.lZoomText.sizePolicy().hasHeightForWidth()) @@ -496,7 +582,9 @@ def setupUi(self, SignalFrame): font.setUnderline(False) self.lZoomText.setFont(font) self.lZoomText.setTextFormat(QtCore.Qt.PlainText) - self.lZoomText.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignVCenter) + self.lZoomText.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignVCenter + ) self.lZoomText.setObjectName("lZoomText") self.additionalInfos.addWidget(self.lZoomText) self.spinBoxXZoom = QtWidgets.QSpinBox(self.layoutWidget) @@ -504,7 +592,9 @@ def setupUi(self, SignalFrame): self.spinBoxXZoom.setMaximum(999999999) self.spinBoxXZoom.setObjectName("spinBoxXZoom") self.additionalInfos.addWidget(self.spinBoxXZoom) - spacerItem4 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem4 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.additionalInfos.addItem(spacerItem4) self.lSamplesInView = QtWidgets.QLabel(self.layoutWidget) self.lSamplesInView.setObjectName("lSamplesInView") @@ -520,7 +610,9 @@ def setupUi(self, SignalFrame): self.additionalInfos.addWidget(self.lSamplesViewText) self.verticalLayout.addLayout(self.additionalInfos) self.txtEdProto = TextEditProtocolView(self.splitter) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.MinimumExpanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.MinimumExpanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.txtEdProto.sizePolicy().hasHeightForWidth()) @@ -547,8 +639,12 @@ def setupUi(self, SignalFrame): SignalFrame.setTabOrder(self.spinBoxSamplesPerSymbol, self.spinBoxTolerance) SignalFrame.setTabOrder(self.spinBoxTolerance, self.cbModulationType) SignalFrame.setTabOrder(self.cbModulationType, self.spinBoxBitsPerSymbol) - SignalFrame.setTabOrder(self.spinBoxBitsPerSymbol, self.btnAdvancedModulationSettings) - SignalFrame.setTabOrder(self.btnAdvancedModulationSettings, self.btnShowHideStartEnd) + SignalFrame.setTabOrder( + self.spinBoxBitsPerSymbol, self.btnAdvancedModulationSettings + ) + SignalFrame.setTabOrder( + self.btnAdvancedModulationSettings, self.btnShowHideStartEnd + ) SignalFrame.setTabOrder(self.btnShowHideStartEnd, self.btnAutoDetect) SignalFrame.setTabOrder(self.btnAutoDetect, self.txtEdProto) SignalFrame.setTabOrder(self.txtEdProto, self.cbSignalView) @@ -569,7 +665,12 @@ def retranslateUi(self, SignalFrame): _translate = QtCore.QCoreApplication.translate SignalFrame.setWindowTitle(_translate("SignalFrame", "Frame")) self.lSignalViewText.setText(_translate("SignalFrame", "Signal view:")) - self.spinBoxBitsPerSymbol.setToolTip(_translate("SignalFrame", "Higher order modulations can carry multiple bits with each symbol. Configure how many bits are represented by a symbol. (Default = Binary modulation with one bit per symbol)
")) + self.spinBoxBitsPerSymbol.setToolTip( + _translate( + "SignalFrame", + 'Higher order modulations can carry multiple bits with each symbol. Configure how many bits are represented by a symbol. (Default = Binary modulation with one bit per symbol)
', + ) + ) self.btnSaveSignal.setText(_translate("SignalFrame", "...")) self.btnCloseSignal.setText(_translate("SignalFrame", "X")) self.lSignalTyp.setText(_translate("SignalFrame", "For higher order modulations (> 1 Bits/Symbol), there are multiple centers. We assume that the spacing between all possible symbols is constant. Therefore you configure the spacing between centers.
")) + self.lCenterSpacing.setToolTip( + _translate( + "SignalFrame", + 'For higher order modulations (> 1 Bits/Symbol), there are multiple centers. We assume that the spacing between all possible symbols is constant. Therefore you configure the spacing between centers.
', + ) + ) self.lCenterSpacing.setText(_translate("SignalFrame", "Center spacing:")) - self.spinBoxTolerance.setToolTip(_translate("SignalFrame", "This is the error tolerance for determining the pulse lengths in the demodulated signal.
Example: Say, we are reading a ones pulse and the tolerance value was set to 5. Then 5 errors (which must follow sequentially) are accepted.
Tune this value if you have spiky data after demodulation.
")) - self.lSamplesPerSymbol.setToolTip(_translate("SignalFrame", "This is the length of one symbol in samples. For binary modulations (default) this is the bit length.
")) + self.spinBoxTolerance.setToolTip( + _translate( + "SignalFrame", + 'This is the error tolerance for determining the pulse lengths in the demodulated signal.
Example: Say, we are reading a ones pulse and the tolerance value was set to 5. Then 5 errors (which must follow sequentially) are accepted.
Tune this value if you have spiky data after demodulation.
', + ) + ) + self.lSamplesPerSymbol.setToolTip( + _translate( + "SignalFrame", + 'This is the length of one symbol in samples. For binary modulations (default) this is the bit length.
', + ) + ) self.lSamplesPerSymbol.setText(_translate("SignalFrame", "Samples/Symbol:")) - self.lErrorTolerance.setToolTip(_translate("SignalFrame", "This is the error tolerance for determining the pulse lengths in the demodulated signal.
Example: Say, we are reading a ones pulse and the tolerance value was set to 5. Then 5 errors (which must follow sequentially) are accepted.
Tune this value if you have spiky data after demodulation.
")) + self.lErrorTolerance.setToolTip( + _translate( + "SignalFrame", + 'This is the error tolerance for determining the pulse lengths in the demodulated signal.
Example: Say, we are reading a ones pulse and the tolerance value was set to 5. Then 5 errors (which must follow sequentially) are accepted.
Tune this value if you have spiky data after demodulation.
', + ) + ) self.lErrorTolerance.setText(_translate("SignalFrame", "Error tolerance:")) - self.lCenterOffset.setToolTip(_translate("SignalFrame", "This is the threshold used for determining if a bit is one or zero. You can set it here or grab the middle of the area in Quadrature Demod View.
")) + self.lCenterOffset.setToolTip( + _translate( + "SignalFrame", + 'This is the threshold used for determining if a bit is one or zero. You can set it here or grab the middle of the area in Quadrature Demod View.
', + ) + ) self.lCenterOffset.setText(_translate("SignalFrame", "Center:")) - self.labelModulation.setToolTip(_translate("SignalFrame", "Choose signals modulation:
Choose signals modulation:
Choose the view of your signal. Analog, Demodulated, Spectrogram or I/Q view.
The quadrature demodulation uses a threshold of magnitudes, to suppress noise. All samples with a magnitude lower than this threshold will be eliminated after demodulation.
Tune this value by selecting a noisy area and mark it as noise using context menu.
Signal colors:
I => blue
Q => black
Choose the view of your signal. Analog, Demodulated, Spectrogram or I/Q view.
The quadrature demodulation uses a threshold of magnitudes, to suppress noise. All samples with a magnitude lower than this threshold will be eliminated after demodulation.
Tune this value by selecting a noisy area and mark it as noise using context menu.
Signal colors:
I => blue
Q => black
Set the noise magnitude of your signal. You can tune this value to mute noise in your signal and reveal the true data.
")) - self.spinBoxCenterSpacing.setToolTip(_translate("SignalFrame", "For higher order modulations (> 1 Bits/Symbol), there are multiple centers. We assume that the spacing between all possible symbols is constant. Therefore you configure the spacing between centers.
")) + self.spinBoxNoiseTreshold.setToolTip( + _translate( + "SignalFrame", + 'Set the noise magnitude of your signal. You can tune this value to mute noise in your signal and reveal the true data.
', + ) + ) + self.spinBoxCenterSpacing.setToolTip( + _translate( + "SignalFrame", + 'For higher order modulations (> 1 Bits/Symbol), there are multiple centers. We assume that the spacing between all possible symbols is constant. Therefore you configure the spacing between centers.
', + ) + ) self.lineEditSignalName.setText(_translate("SignalFrame", "SignalName")) - self.cbModulationType.setToolTip(_translate("SignalFrame", "Choose signals modulation:
Choose signals modulation:
This is the length of one symbol in samples. For binary modulations (default) this is the bit length.
")) - self.btnAutoDetect.setToolTip(_translate("SignalFrame", "Automatically detect center, bit length and tolerance. You can also choose to additionally detect the noise and modulation when clicking this button.
")) + self.spinBoxSamplesPerSymbol.setToolTip( + _translate( + "SignalFrame", + 'This is the length of one symbol in samples. For binary modulations (default) this is the bit length.
', + ) + ) + self.btnAutoDetect.setToolTip( + _translate( + "SignalFrame", + 'Automatically detect center, bit length and tolerance. You can also choose to additionally detect the noise and modulation when clicking this button.
', + ) + ) self.btnAutoDetect.setText(_translate("SignalFrame", "Autodetect parameters")) self.labelFFTWindowSize.setText(_translate("SignalFrame", "FFT window size:")) - self.spinBoxCenterOffset.setToolTip(_translate("SignalFrame", "This is the threshold used for determining if a bit is one or zero. You can set it here or grab the middle of the area in Quadrature Demod View.
")) - self.labelNoise.setToolTip(_translate("SignalFrame", "Set the noise magnitude of your signal. You can tune this value to mute noise in your signal and reveal the true data.
")) + self.spinBoxCenterOffset.setToolTip( + _translate( + "SignalFrame", + 'This is the threshold used for determining if a bit is one or zero. You can set it here or grab the middle of the area in Quadrature Demod View.
', + ) + ) + self.labelNoise.setToolTip( + _translate( + "SignalFrame", + 'Set the noise magnitude of your signal. You can tune this value to mute noise in your signal and reveal the true data.
', + ) + ) self.labelNoise.setText(_translate("SignalFrame", "Noise:")) - self.lBitsPerSymbol.setToolTip(_translate("SignalFrame", "Higher order modulations can carry multiple bits with each symbol. Configure how many bits are represented by a symbol. (Default = Binary modulation with one bit per symbol)
")) + self.lBitsPerSymbol.setToolTip( + _translate( + "SignalFrame", + 'Higher order modulations can carry multiple bits with each symbol. Configure how many bits are represented by a symbol. (Default = Binary modulation with one bit per symbol)
', + ) + ) self.lBitsPerSymbol.setText(_translate("SignalFrame", "Bits/Symbol:")) - self.labelLoadingAutoInterpretation.setText(_translate("SignalFrame", "Running automatic detecting of demodulation parameters.
You can disable this behaviour for newly loaded signals by unchecking Edit -> Auto detect signals on loading.
")) + self.labelLoadingAutoInterpretation.setText( + _translate( + "SignalFrame", + 'Running automatic detecting of demodulation parameters.
You can disable this behaviour for newly loaded signals by unchecking Edit -> Auto detect signals on loading.
', + ) + ) self.lYScale.setText(_translate("SignalFrame", "Y-Scale")) self.btnShowHideStartEnd.setText(_translate("SignalFrame", "-")) - self.lNumSelectedSamples.setToolTip(_translate("SignalFrame", "Number of currently selected samples.")) + self.lNumSelectedSamples.setToolTip( + _translate("SignalFrame", "Number of currently selected samples.") + ) self.lNumSelectedSamples.setText(_translate("SignalFrame", "0")) - self.lTextSelectedSamples.setToolTip(_translate("SignalFrame", "Number of currently selected samples.")) + self.lTextSelectedSamples.setToolTip( + _translate("SignalFrame", "Number of currently selected samples.") + ) self.lTextSelectedSamples.setText(_translate("SignalFrame", "selected")) self.lDuration.setText(_translate("SignalFrame", "42 µs")) - self.labelRSSI.setToolTip(_translate("SignalFrame", "This is the average signal power of the selection. The closer this value is to zero, the stronger the selected signal is.
")) + self.labelRSSI.setToolTip( + _translate( + "SignalFrame", + "This is the average signal power of the selection. The closer this value is to zero, the stronger the selected signal is.
", + ) + ) self.labelRSSI.setText(_translate("SignalFrame", "0,434 dBm")) self.btnFilter.setText(_translate("SignalFrame", "Filter (moving average)")) self.lStart.setText(_translate("SignalFrame", "Start:")) self.lEnd.setText(_translate("SignalFrame", "End:")) - self.lZoomText.setToolTip(_translate("SignalFrame", "Current (relative) Zoom. Standard is 100%, if you zoom in, this factor increases. You can directly set a value in the spinbox or use the mousewheel to zoom.
")) + self.lZoomText.setToolTip( + _translate( + "SignalFrame", + 'Current (relative) Zoom. Standard is 100%, if you zoom in, this factor increases. You can directly set a value in the spinbox or use the mousewheel to zoom.
', + ) + ) self.lZoomText.setText(_translate("SignalFrame", "X-Zoom:")) - self.spinBoxXZoom.setToolTip(_translate("SignalFrame", "Current (relative) Zoom. Standard is 100%, if you zoom in, this factor increases. You can directly set a value in the spinbox or use the mousewheel to zoom.
")) + self.spinBoxXZoom.setToolTip( + _translate( + "SignalFrame", + 'Current (relative) Zoom. Standard is 100%, if you zoom in, this factor increases. You can directly set a value in the spinbox or use the mousewheel to zoom.
', + ) + ) self.spinBoxXZoom.setSuffix(_translate("SignalFrame", "%")) self.lSamplesInView.setText(_translate("SignalFrame", "0")) self.lStrich.setText(_translate("SignalFrame", "/")) self.lSamplesTotal.setText(_translate("SignalFrame", "0")) self.lSamplesViewText.setText(_translate("SignalFrame", "Samples in view")) + + from urh.ui.views.EpicGraphicView import EpicGraphicView from urh.ui.views.SpectrogramGraphicView import SpectrogramGraphicView from urh.ui.views.TextEditProtocolView import TextEditProtocolView diff --git a/src/urh/ui/ui_simulator.py b/src/urh/ui/ui_simulator.py index 8159c3953b..7c7c69f998 100644 --- a/src/urh/ui/ui_simulator.py +++ b/src/urh/ui/ui_simulator.py @@ -26,14 +26,16 @@ def setupUi(self, SimulatorTab): self.verticalLayout_5 = QtWidgets.QVBoxLayout(self.scrollAreaWidgetContents) self.verticalLayout_5.setObjectName("verticalLayout_5") self.splitterLeftRight = QtWidgets.QSplitter(self.scrollAreaWidgetContents) - self.splitterLeftRight.setStyleSheet("QSplitter::handle:horizontal {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -"image: url(:/icons/icons/splitter_handle_vertical.svg);\n" -"}") + self.splitterLeftRight.setStyleSheet( + "QSplitter::handle:horizontal {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:0, y2:1, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + "image: url(:/icons/icons/splitter_handle_vertical.svg);\n" + "}" + ) self.splitterLeftRight.setOrientation(QtCore.Qt.Horizontal) self.splitterLeftRight.setHandleWidth(6) self.splitterLeftRight.setObjectName("splitterLeftRight") @@ -61,10 +63,14 @@ def setupUi(self, SimulatorTab): self.label_4.setObjectName("label_4") self.gridLayout.addWidget(self.label_4, 0, 0, 1, 1) self.spinBoxNRepeat = QtWidgets.QSpinBox(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxNRepeat.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxNRepeat.sizePolicy().hasHeightForWidth() + ) self.spinBoxNRepeat.setSizePolicy(sizePolicy) self.spinBoxNRepeat.setMaximum(9999999) self.spinBoxNRepeat.setObjectName("spinBoxNRepeat") @@ -73,10 +79,14 @@ def setupUi(self, SimulatorTab): self.label_3.setObjectName("label_3") self.gridLayout.addWidget(self.label_3, 1, 0, 1, 1) self.spinBoxTimeout = QtWidgets.QSpinBox(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.spinBoxTimeout.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.spinBoxTimeout.sizePolicy().hasHeightForWidth() + ) self.spinBoxTimeout.setSizePolicy(sizePolicy) self.spinBoxTimeout.setMinimum(1) self.spinBoxTimeout.setMaximum(9999999) @@ -86,10 +96,14 @@ def setupUi(self, SimulatorTab): self.label_7.setObjectName("label_7") self.gridLayout.addWidget(self.label_7, 2, 0, 1, 1) self.comboBoxError = QtWidgets.QComboBox(self.layoutWidget) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.comboBoxError.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.comboBoxError.sizePolicy().hasHeightForWidth() + ) self.comboBoxError.setSizePolicy(sizePolicy) self.comboBoxError.setObjectName("comboBoxError") self.comboBoxError.addItem("") @@ -112,14 +126,16 @@ def setupUi(self, SimulatorTab): self.btnStartSim.setObjectName("btnStartSim") self.verticalLayout_3.addWidget(self.btnStartSim) self.splitter = QtWidgets.QSplitter(self.splitterLeftRight) - self.splitter.setStyleSheet("QSplitter::handle:vertical {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -"image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:vertical {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + "image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" + "}" + ) self.splitter.setOrientation(QtCore.Qt.Vertical) self.splitter.setHandleWidth(6) self.splitter.setObjectName("splitter") @@ -129,7 +145,9 @@ def setupUi(self, SimulatorTab): self.verticalLayout_2.setContentsMargins(11, 11, 11, 11) self.verticalLayout_2.setObjectName("verticalLayout_2") self.tabWidget = QtWidgets.QTabWidget(self.layoutWidget_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.tabWidget.sizePolicy().hasHeightForWidth()) @@ -145,7 +163,9 @@ def setupUi(self, SimulatorTab): self.verticalLayout.setSpacing(7) self.verticalLayout.setObjectName("verticalLayout") self.gvSimulator = SimulatorGraphicsView(self.tab) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.gvSimulator.sizePolicy().hasHeightForWidth()) @@ -159,14 +179,22 @@ def setupUi(self, SimulatorTab): self.verticalLayout_6.setContentsMargins(0, 0, 0, 0) self.verticalLayout_6.setObjectName("verticalLayout_6") self.tblViewMessage = SimulatorMessageTableView(self.tab_2) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.tblViewMessage.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.tblViewMessage.sizePolicy().hasHeightForWidth() + ) self.tblViewMessage.setSizePolicy(sizePolicy) self.tblViewMessage.setAlternatingRowColors(True) - self.tblViewMessage.setVerticalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) - self.tblViewMessage.setHorizontalScrollMode(QtWidgets.QAbstractItemView.ScrollPerPixel) + self.tblViewMessage.setVerticalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) + self.tblViewMessage.setHorizontalScrollMode( + QtWidgets.QAbstractItemView.ScrollPerPixel + ) self.tblViewMessage.setShowGrid(False) self.tblViewMessage.setObjectName("tblViewMessage") self.tblViewMessage.horizontalHeader().setHighlightSections(False) @@ -175,13 +203,17 @@ def setupUi(self, SimulatorTab): self.horizontalLayout_3 = QtWidgets.QHBoxLayout() self.horizontalLayout_3.setObjectName("horizontalLayout_3") self.lNumSelectedColumns = QtWidgets.QLabel(self.tab_2) - self.lNumSelectedColumns.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignVCenter) + self.lNumSelectedColumns.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignVCenter + ) self.lNumSelectedColumns.setObjectName("lNumSelectedColumns") self.horizontalLayout_3.addWidget(self.lNumSelectedColumns) self.lColumnsSelectedText = QtWidgets.QLabel(self.tab_2) self.lColumnsSelectedText.setObjectName("lColumnsSelectedText") self.horizontalLayout_3.addWidget(self.lColumnsSelectedText) - spacerItem = QtWidgets.QSpacerItem(138, 33, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem = QtWidgets.QSpacerItem( + 138, 33, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_3.addItem(spacerItem) self.label_5 = QtWidgets.QLabel(self.tab_2) self.label_5.setObjectName("label_5") @@ -224,7 +256,9 @@ def setupUi(self, SimulatorTab): self.btnDown.setIcon(icon) self.btnDown.setObjectName("btnDown") self.verticalLayout_9.addWidget(self.btnDown) - spacerItem1 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_9.addItem(spacerItem1) self.horizontalLayout.addLayout(self.verticalLayout_9) self.tabWidget.addTab(self.tabParticipants, "") @@ -235,10 +269,14 @@ def setupUi(self, SimulatorTab): self.verticalLayout_4.setContentsMargins(11, 11, 11, 11) self.verticalLayout_4.setObjectName("verticalLayout_4") self.lblMsgFieldsValues = QtWidgets.QLabel(self.layoutWidget_3) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Fixed + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.lblMsgFieldsValues.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.lblMsgFieldsValues.sizePolicy().hasHeightForWidth() + ) self.lblMsgFieldsValues.setSizePolicy(sizePolicy) font = QtGui.QFont() font.setBold(True) @@ -262,9 +300,13 @@ def setupUi(self, SimulatorTab): self.goto_combobox = QtWidgets.QComboBox(self.page_goto_action) self.goto_combobox.setObjectName("goto_combobox") self.verticalLayout_7.addWidget(self.goto_combobox, 0, 1, 1, 1) - spacerItem2 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem2 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.verticalLayout_7.addItem(spacerItem2, 0, 2, 1, 1) - spacerItem3 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem3 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_7.addItem(spacerItem3, 1, 0, 1, 3) self.detail_view_widget.addWidget(self.page_goto_action) self.page_message = QtWidgets.QWidget() @@ -272,7 +314,9 @@ def setupUi(self, SimulatorTab): self.gridLayout_6 = QtWidgets.QGridLayout(self.page_message) self.gridLayout_6.setObjectName("gridLayout_6") self.label_10 = QtWidgets.QLabel(self.page_message) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label_10.sizePolicy().hasHeightForWidth()) @@ -288,12 +332,16 @@ def setupUi(self, SimulatorTab): self.tblViewFieldValues.verticalHeader().setVisible(False) self.gridLayout_6.addWidget(self.tblViewFieldValues, 2, 2, 1, 1) self.label_11 = QtWidgets.QLabel(self.page_message) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Preferred + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.label_11.sizePolicy().hasHeightForWidth()) self.label_11.setSizePolicy(sizePolicy) - self.label_11.setAlignment(QtCore.Qt.AlignLeading|QtCore.Qt.AlignLeft|QtCore.Qt.AlignTop) + self.label_11.setAlignment( + QtCore.Qt.AlignLeading | QtCore.Qt.AlignLeft | QtCore.Qt.AlignTop + ) self.label_11.setObjectName("label_11") self.gridLayout_6.addWidget(self.label_11, 2, 0, 1, 1) self.spinBoxRepeat = QtWidgets.QSpinBox(self.page_message) @@ -314,7 +362,9 @@ def setupUi(self, SimulatorTab): self.label_12 = QtWidgets.QLabel(self.page_rule) self.label_12.setObjectName("label_12") self.gridLayout_3.addWidget(self.label_12, 0, 0, 1, 1) - spacerItem4 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem4 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_3.addItem(spacerItem4, 1, 0, 1, 2) self.ruleCondLineEdit = ExpressionLineEdit(self.page_rule) self.ruleCondLineEdit.setObjectName("ruleCondLineEdit") @@ -324,7 +374,9 @@ def setupUi(self, SimulatorTab): self.page_ext_prog_action.setObjectName("page_ext_prog_action") self.gridLayout_9 = QtWidgets.QGridLayout(self.page_ext_prog_action) self.gridLayout_9.setObjectName("gridLayout_9") - self.checkBoxPassTranscriptSTDIN = QtWidgets.QCheckBox(self.page_ext_prog_action) + self.checkBoxPassTranscriptSTDIN = QtWidgets.QCheckBox( + self.page_ext_prog_action + ) self.checkBoxPassTranscriptSTDIN.setObjectName("checkBoxPassTranscriptSTDIN") self.gridLayout_9.addWidget(self.checkBoxPassTranscriptSTDIN, 2, 0, 1, 4) self.label_14 = QtWidgets.QLabel(self.page_ext_prog_action) @@ -337,7 +389,9 @@ def setupUi(self, SimulatorTab): self.btnChooseCommand = QtWidgets.QToolButton(self.page_ext_prog_action) self.btnChooseCommand.setObjectName("btnChooseCommand") self.gridLayout_9.addWidget(self.btnChooseCommand, 1, 2, 1, 2) - spacerItem5 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem5 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.gridLayout_9.addItem(spacerItem5, 4, 0, 1, 4) self.label_18 = QtWidgets.QLabel(self.page_ext_prog_action) self.label_18.setWordWrap(True) @@ -360,7 +414,9 @@ def setupUi(self, SimulatorTab): self.doubleSpinBoxSleep.setObjectName("doubleSpinBoxSleep") self.horizontalLayout_2.addWidget(self.doubleSpinBoxSleep) self.verticalLayout_10.addLayout(self.horizontalLayout_2) - spacerItem6 = QtWidgets.QSpacerItem(20, 231, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem6 = QtWidgets.QSpacerItem( + 20, 231, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_10.addItem(spacerItem6) self.detail_view_widget.addWidget(self.page_sleep) self.page = QtWidgets.QWidget() @@ -389,7 +445,9 @@ def setupUi(self, SimulatorTab): self.label_17.setWordWrap(True) self.label_17.setObjectName("label_17") self.verticalLayout_11.addWidget(self.label_17) - spacerItem7 = QtWidgets.QSpacerItem(20, 36, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem7 = QtWidgets.QSpacerItem( + 20, 36, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_11.addItem(spacerItem7) self.detail_view_widget.addWidget(self.page) self.verticalLayout_4.addWidget(self.detail_view_widget) @@ -404,53 +462,101 @@ def setupUi(self, SimulatorTab): def retranslateUi(self, SimulatorTab): _translate = QtCore.QCoreApplication.translate SimulatorTab.setWindowTitle(_translate("SimulatorTab", "Form")) - self.label.setText(_translate("SimulatorTab", "Protocols (Drag&Drop to Flow Graph):")) + self.label.setText( + _translate("SimulatorTab", "Protocols (Drag&Drop to Flow Graph):") + ) self.label_6.setText(_translate("SimulatorTab", "Simulate these participants:")) - self.label_4.setText(_translate("SimulatorTab", "Repeat simulation this often:")) + self.label_4.setText( + _translate("SimulatorTab", "Repeat simulation this often:") + ) self.spinBoxNRepeat.setSpecialValueText(_translate("SimulatorTab", "Infinite")) self.label_3.setText(_translate("SimulatorTab", "Timeout:")) self.spinBoxTimeout.setSuffix(_translate("SimulatorTab", "ms")) - self.label_7.setText(_translate("SimulatorTab", "In case of an overdue response:")) - self.comboBoxError.setItemText(0, _translate("SimulatorTab", "Resend last message")) + self.label_7.setText( + _translate("SimulatorTab", "In case of an overdue response:") + ) + self.comboBoxError.setItemText( + 0, _translate("SimulatorTab", "Resend last message") + ) self.comboBoxError.setItemText(1, _translate("SimulatorTab", "Stop simulation")) - self.comboBoxError.setItemText(2, _translate("SimulatorTab", "Restart simulation")) + self.comboBoxError.setItemText( + 2, _translate("SimulatorTab", "Restart simulation") + ) self.label_8.setText(_translate("SimulatorTab", "Maximum retries:")) self.btnStartSim.setText(_translate("SimulatorTab", "Simulate...")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab), _translate("SimulatorTab", "Flow Graph")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab), _translate("SimulatorTab", "Flow Graph") + ) self.lNumSelectedColumns.setText(_translate("SimulatorTab", "0")) - self.lColumnsSelectedText.setText(_translate("SimulatorTab", "column(s) selected")) + self.lColumnsSelectedText.setText( + _translate("SimulatorTab", "column(s) selected") + ) self.label_5.setText(_translate("SimulatorTab", "Viewtype:")) self.cbViewType.setItemText(0, _translate("SimulatorTab", "Bit")) self.cbViewType.setItemText(1, _translate("SimulatorTab", "Hex")) self.cbViewType.setItemText(2, _translate("SimulatorTab", "ASCII")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_2), _translate("SimulatorTab", "Messages")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_2), _translate("SimulatorTab", "Messages") + ) self.btnAddParticipant.setToolTip(_translate("SimulatorTab", "Add participant")) self.btnAddParticipant.setText(_translate("SimulatorTab", "Add")) - self.btnRemoveParticipant.setToolTip(_translate("SimulatorTab", "Remove participant")) + self.btnRemoveParticipant.setToolTip( + _translate("SimulatorTab", "Remove participant") + ) self.btnRemoveParticipant.setText(_translate("SimulatorTab", "Remove")) - self.btnUp.setToolTip(_translate("SimulatorTab", "Move selected participants up")) + self.btnUp.setToolTip( + _translate("SimulatorTab", "Move selected participants up") + ) self.btnUp.setText(_translate("SimulatorTab", "...")) - self.btnDown.setToolTip(_translate("SimulatorTab", "Move selected participants down")) + self.btnDown.setToolTip( + _translate("SimulatorTab", "Move selected participants down") + ) self.btnDown.setText(_translate("SimulatorTab", "...")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tabParticipants), _translate("SimulatorTab", "Participants")) - self.lblMsgFieldsValues.setText(_translate("SimulatorTab", "Detail view for item")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tabParticipants), + _translate("SimulatorTab", "Participants"), + ) + self.lblMsgFieldsValues.setText( + _translate("SimulatorTab", "Detail view for item") + ) self.label_9.setText(_translate("SimulatorTab", "Goto:")) self.label_10.setText(_translate("SimulatorTab", "Copies:")) self.label_11.setText(_translate("SimulatorTab", "Labels:")) self.label_2.setText(_translate("SimulatorTab", "Coding:")) self.lblEncodingDecoding.setText(_translate("SimulatorTab", "-")) self.label_12.setText(_translate("SimulatorTab", "Condition:")) - self.ruleCondLineEdit.setPlaceholderText(_translate("SimulatorTab", "not (item1.crc == 0b1010 and item2.length >=3)")) - self.checkBoxPassTranscriptSTDIN.setText(_translate("SimulatorTab", "Pass transcript to STDIN")) + self.ruleCondLineEdit.setPlaceholderText( + _translate("SimulatorTab", "not (item1.crc == 0b1010 and item2.length >=3)") + ) + self.checkBoxPassTranscriptSTDIN.setText( + _translate("SimulatorTab", "Pass transcript to STDIN") + ) self.label_14.setText(_translate("SimulatorTab", "Command:")) - self.lineEditTriggerCommand.setPlaceholderText(_translate("SimulatorTab", "Path [+arguments] to external command e.g. mail or sendsms")) + self.lineEditTriggerCommand.setPlaceholderText( + _translate( + "SimulatorTab", + "Path [+arguments] to external command e.g. mail or sendsms", + ) + ) self.btnChooseCommand.setText(_translate("SimulatorTab", "...")) - self.label_18.setText(_translate("SimulatorTab", "You can access the return code of this item in formulas and rules using the item identifier followed by .rc e.g. item5.rc.
")) + self.label_18.setText( + _translate( + "SimulatorTab", + 'You can access the return code of this item in formulas and rules using the item identifier followed by .rc e.g. item5.rc.
', + ) + ) self.label_13.setText(_translate("SimulatorTab", "Sleep for:")) self.doubleSpinBoxSleep.setSuffix(_translate("SimulatorTab", "s")) self.label_15.setText(_translate("SimulatorTab", "Start:")) self.label_16.setText(_translate("SimulatorTab", "Step:")) - self.label_17.setText(_translate("SimulatorTab", "This counter will increase by step each time it gets hit during simulation. It will preserve it\'s value during simulation repeats and retries. To reset all counters stop the simulation and start it again.
Access the value of this counter using item<Number>.counter_value in Formulas or as parameter in external programs e.g. external_py -c item5.counter_value. The value of this counter will be inserted during simulation time.
")) + self.label_17.setText( + _translate( + "SimulatorTab", + 'This counter will increase by step each time it gets hit during simulation. It will preserve it\'s value during simulation repeats and retries. To reset all counters stop the simulation and start it again.
Access the value of this counter using item<Number>.counter_value in Formulas or as parameter in external programs e.g. external_py -c item5.counter_value. The value of this counter will be inserted during simulation time.
', + ) + ) + + from urh.ui.ExpressionLineEdit import ExpressionLineEdit from urh.ui.views.GeneratorTreeView import GeneratorTreeView from urh.ui.views.ParticipantTableView import ParticipantTableView diff --git a/src/urh/ui/ui_simulator_dialog.py b/src/urh/ui/ui_simulator_dialog.py index fa0124373e..0fb285826c 100644 --- a/src/urh/ui/ui_simulator_dialog.py +++ b/src/urh/ui/ui_simulator_dialog.py @@ -56,14 +56,22 @@ def setupUi(self, DialogSimulator): self.scrollAreaWidgetContentsRX.setObjectName("scrollAreaWidgetContentsRX") self.verticalLayout_6 = QtWidgets.QVBoxLayout(self.scrollAreaWidgetContentsRX) self.verticalLayout_6.setObjectName("verticalLayout_6") - self.btnTestSniffSettings = QtWidgets.QPushButton(self.scrollAreaWidgetContentsRX) + self.btnTestSniffSettings = QtWidgets.QPushButton( + self.scrollAreaWidgetContentsRX + ) icon = QtGui.QIcon() - icon.addPixmap(QtGui.QPixmap(":/icons/icons/sniffer.svg"), QtGui.QIcon.Normal, QtGui.QIcon.Off) + icon.addPixmap( + QtGui.QPixmap(":/icons/icons/sniffer.svg"), + QtGui.QIcon.Normal, + QtGui.QIcon.Off, + ) self.btnTestSniffSettings.setIcon(icon) self.btnTestSniffSettings.setAutoDefault(False) self.btnTestSniffSettings.setObjectName("btnTestSniffSettings") self.verticalLayout_6.addWidget(self.btnTestSniffSettings) - spacerItem = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_6.addItem(spacerItem) self.scrollAreaRX.setWidget(self.scrollAreaWidgetContentsRX) self.verticalLayout_5.addWidget(self.scrollAreaRX) @@ -82,7 +90,9 @@ def setupUi(self, DialogSimulator): self.scrollAreaWidgetContentsTX.setObjectName("scrollAreaWidgetContentsTX") self.verticalLayout_8 = QtWidgets.QVBoxLayout(self.scrollAreaWidgetContentsTX) self.verticalLayout_8.setObjectName("verticalLayout_8") - spacerItem1 = QtWidgets.QSpacerItem(20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding) + spacerItem1 = QtWidgets.QSpacerItem( + 20, 40, QtWidgets.QSizePolicy.Minimum, QtWidgets.QSizePolicy.Expanding + ) self.verticalLayout_8.addItem(spacerItem1) self.scrollAreaTX.setWidget(self.scrollAreaWidgetContentsTX) self.verticalLayout_7.addWidget(self.scrollAreaTX) @@ -102,20 +112,22 @@ def setupUi(self, DialogSimulator): self.verticalLayout_15 = QtWidgets.QVBoxLayout(self.tab_simulation) self.verticalLayout_15.setObjectName("verticalLayout_15") self.groupBoxSimulationStatus = QtWidgets.QGroupBox(self.tab_simulation) - self.groupBoxSimulationStatus.setStyleSheet("QGroupBox\n" -"{\n" -"border: none;\n" -"}\n" -"\n" -"QGroupBox::title {\n" -" subcontrol-origin: margin;\n" -"}\n" -"QGroupBox::indicator:unchecked {\n" -" image: url(:/icons/icons/collapse.svg)\n" -"}\n" -"QGroupBox::indicator:checked {\n" -" image: url(:/icons/icons/uncollapse.svg)\n" -"}") + self.groupBoxSimulationStatus.setStyleSheet( + "QGroupBox\n" + "{\n" + "border: none;\n" + "}\n" + "\n" + "QGroupBox::title {\n" + " subcontrol-origin: margin;\n" + "}\n" + "QGroupBox::indicator:unchecked {\n" + " image: url(:/icons/icons/collapse.svg)\n" + "}\n" + "QGroupBox::indicator:checked {\n" + " image: url(:/icons/icons/uncollapse.svg)\n" + "}" + ) self.groupBoxSimulationStatus.setCheckable(True) self.groupBoxSimulationStatus.setObjectName("groupBoxSimulationStatus") self.verticalLayout_12 = QtWidgets.QVBoxLayout(self.groupBoxSimulationStatus) @@ -165,20 +177,22 @@ def setupUi(self, DialogSimulator): self.verticalLayout_12.addWidget(self.frame) self.verticalLayout_15.addWidget(self.groupBoxSimulationStatus) self.groupBoxRXStatus = QtWidgets.QGroupBox(self.tab_simulation) - self.groupBoxRXStatus.setStyleSheet("QGroupBox\n" -"{\n" -"border: none;\n" -"}\n" -"\n" -"QGroupBox::title {\n" -" subcontrol-origin: margin;\n" -"}\n" -"QGroupBox::indicator:unchecked {\n" -" image: url(:/icons/icons/collapse.svg)\n" -"}\n" -"QGroupBox::indicator:checked {\n" -" image: url(:/icons/icons/uncollapse.svg)\n" -"}") + self.groupBoxRXStatus.setStyleSheet( + "QGroupBox\n" + "{\n" + "border: none;\n" + "}\n" + "\n" + "QGroupBox::title {\n" + " subcontrol-origin: margin;\n" + "}\n" + "QGroupBox::indicator:unchecked {\n" + " image: url(:/icons/icons/collapse.svg)\n" + "}\n" + "QGroupBox::indicator:checked {\n" + " image: url(:/icons/icons/uncollapse.svg)\n" + "}" + ) self.groupBoxRXStatus.setCheckable(True) self.groupBoxRXStatus.setObjectName("groupBoxRXStatus") self.verticalLayout_14 = QtWidgets.QVBoxLayout(self.groupBoxRXStatus) @@ -196,7 +210,9 @@ def setupUi(self, DialogSimulator): self.checkBoxCaptureFullRX = QtWidgets.QCheckBox(self.frame_2) self.checkBoxCaptureFullRX.setObjectName("checkBoxCaptureFullRX") self.horizontalLayout_5.addWidget(self.checkBoxCaptureFullRX) - spacerItem2 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem2 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_5.addItem(spacerItem2) self.btnSaveRX = QtWidgets.QToolButton(self.frame_2) icon = QtGui.QIcon.fromTheme("document-save") @@ -227,7 +243,9 @@ def setupUi(self, DialogSimulator): self.radioButtonTranscriptHex = QtWidgets.QRadioButton(self.tab) self.radioButtonTranscriptHex.setObjectName("radioButtonTranscriptHex") self.horizontalLayout_3.addWidget(self.radioButtonTranscriptHex) - spacerItem3 = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum) + spacerItem3 = QtWidgets.QSpacerItem( + 40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum + ) self.horizontalLayout_3.addItem(spacerItem3) self.btnOpenInAnalysis = QtWidgets.QPushButton(self.tab) self.btnOpenInAnalysis.setObjectName("btnOpenInAnalysis") @@ -261,8 +279,8 @@ def setupUi(self, DialogSimulator): self.retranslateUi(DialogSimulator) self.tabWidgetSimulatorSettings.setCurrentIndex(3) self.tabWidget.setCurrentIndex(0) - self.groupBoxSimulationStatus.toggled['bool'].connect(self.frame.setVisible) - self.groupBoxRXStatus.toggled['bool'].connect(self.frame_2.setVisible) + self.groupBoxSimulationStatus.toggled["bool"].connect(self.frame.setVisible) + self.groupBoxRXStatus.toggled["bool"].connect(self.frame_2.setVisible) def retranslateUi(self, DialogSimulator): _translate = QtCore.QCoreApplication.translate @@ -270,31 +288,73 @@ def retranslateUi(self, DialogSimulator): self.btnLogAll.setText(_translate("DialogSimulator", "Log all")) self.btnLogNone.setText(_translate("DialogSimulator", "Log none")) self.btnToggleLog.setText(_translate("DialogSimulator", "Toggle selected")) - self.tabWidgetSimulatorSettings.setTabText(self.tabWidgetSimulatorSettings.indexOf(self.tabLog), _translate("DialogSimulator", "Log settings")) - self.btnTestSniffSettings.setText(_translate("DialogSimulator", "Test sniffer settings")) - self.tabWidgetSimulatorSettings.setTabText(self.tabWidgetSimulatorSettings.indexOf(self.tabRX), _translate("DialogSimulator", "RX settings")) - self.tabWidgetSimulatorSettings.setTabText(self.tabWidgetSimulatorSettings.indexOf(self.tabTX), _translate("DialogSimulator", "TX settings")) - self.groupBoxSimulationStatus.setTitle(_translate("DialogSimulator", "Simulation Status")) + self.tabWidgetSimulatorSettings.setTabText( + self.tabWidgetSimulatorSettings.indexOf(self.tabLog), + _translate("DialogSimulator", "Log settings"), + ) + self.btnTestSniffSettings.setText( + _translate("DialogSimulator", "Test sniffer settings") + ) + self.tabWidgetSimulatorSettings.setTabText( + self.tabWidgetSimulatorSettings.indexOf(self.tabRX), + _translate("DialogSimulator", "RX settings"), + ) + self.tabWidgetSimulatorSettings.setTabText( + self.tabWidgetSimulatorSettings.indexOf(self.tabTX), + _translate("DialogSimulator", "TX settings"), + ) + self.groupBoxSimulationStatus.setTitle( + _translate("DialogSimulator", "Simulation Status") + ) self.label.setText(_translate("DialogSimulator", "Current iteration:")) self.lblCurrentRepeatValue.setText(_translate("DialogSimulator", "0")) self.label_2.setText(_translate("DialogSimulator", "Current item:")) self.lblCurrentItemValue.setText(_translate("DialogSimulator", "0")) self.btnSaveLog.setText(_translate("DialogSimulator", "...")) self.groupBoxRXStatus.setTitle(_translate("DialogSimulator", "RX Status")) - self.checkBoxCaptureFullRX.setText(_translate("DialogSimulator", "Capture complete RX")) + self.checkBoxCaptureFullRX.setText( + _translate("DialogSimulator", "Capture complete RX") + ) self.btnSaveRX.setToolTip(_translate("DialogSimulator", "Save current capture")) self.btnSaveRX.setText(_translate("DialogSimulator", "Save")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_simulation), _translate("DialogSimulator", "Status")) - self.textEditTranscript.setPlaceholderText(_translate("DialogSimulator", "Here you will find all messages that were sent and received during simulation.")) - self.radioButtonTranscriptBit.setText(_translate("DialogSimulator", "Bit &view")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_simulation), + _translate("DialogSimulator", "Status"), + ) + self.textEditTranscript.setPlaceholderText( + _translate( + "DialogSimulator", + "Here you will find all messages that were sent and received during simulation.", + ) + ) + self.radioButtonTranscriptBit.setText( + _translate("DialogSimulator", "Bit &view") + ) self.radioButtonTranscriptHex.setText(_translate("DialogSimulator", "Hex view")) - self.btnOpenInAnalysis.setText(_translate("DialogSimulator", "Open in Analysis")) + self.btnOpenInAnalysis.setText( + _translate("DialogSimulator", "Open in Analysis") + ) self.btnSaveTranscript.setText(_translate("DialogSimulator", "...")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab), _translate("DialogSimulator", "Messages")) - self.textEditDevices.setPlaceholderText(_translate("DialogSimulator", "After simulation start you will see the log messages of your configured SDRs here.")) - self.tabWidget.setTabText(self.tabWidget.indexOf(self.tab_device), _translate("DialogSimulator", "Devices")) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab), _translate("DialogSimulator", "Messages") + ) + self.textEditDevices.setPlaceholderText( + _translate( + "DialogSimulator", + "After simulation start you will see the log messages of your configured SDRs here.", + ) + ) + self.tabWidget.setTabText( + self.tabWidget.indexOf(self.tab_device), + _translate("DialogSimulator", "Devices"), + ) self.btnStartStop.setText(_translate("DialogSimulator", "Start")) - self.tabWidgetSimulatorSettings.setTabText(self.tabWidgetSimulatorSettings.indexOf(self.tabSimulation), _translate("DialogSimulator", "Simulation")) + self.tabWidgetSimulatorSettings.setTabText( + self.tabWidgetSimulatorSettings.indexOf(self.tabSimulation), + _translate("DialogSimulator", "Simulation"), + ) + + from urh.ui.views.LiveGraphicView import LiveGraphicView from urh.ui.views.LoggingGraphicsView import LoggingGraphicsView from . import urh_rc diff --git a/src/urh/ui/ui_tab_interpretation.py b/src/urh/ui/ui_tab_interpretation.py index fc596b81c0..905660da0b 100644 --- a/src/urh/ui/ui_tab_interpretation.py +++ b/src/urh/ui/ui_tab_interpretation.py @@ -17,7 +17,9 @@ def setupUi(self, Interpretation): self.horizontalLayout.setSpacing(0) self.horizontalLayout.setObjectName("horizontalLayout") self.scrollArea = ScrollArea(Interpretation) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) sizePolicy.setHeightForWidth(self.scrollArea.sizePolicy().hasHeightForWidth()) @@ -31,10 +33,14 @@ def setupUi(self, Interpretation): self.scrollArea.setObjectName("scrollArea") self.scrlAreaSignals = QtWidgets.QWidget() self.scrlAreaSignals.setGeometry(QtCore.QRect(0, 0, 631, 561)) - sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding) + sizePolicy = QtWidgets.QSizePolicy( + QtWidgets.QSizePolicy.Preferred, QtWidgets.QSizePolicy.Expanding + ) sizePolicy.setHorizontalStretch(0) sizePolicy.setVerticalStretch(0) - sizePolicy.setHeightForWidth(self.scrlAreaSignals.sizePolicy().hasHeightForWidth()) + sizePolicy.setHeightForWidth( + self.scrlAreaSignals.sizePolicy().hasHeightForWidth() + ) self.scrlAreaSignals.setSizePolicy(sizePolicy) self.scrlAreaSignals.setAutoFillBackground(True) self.scrlAreaSignals.setStyleSheet("") @@ -43,14 +49,16 @@ def setupUi(self, Interpretation): self.verticalLayout.setContentsMargins(0, 0, 0, 0) self.verticalLayout.setObjectName("verticalLayout") self.splitter = QtWidgets.QSplitter(self.scrlAreaSignals) - self.splitter.setStyleSheet("QSplitter::handle:vertical {\n" -"margin: 4px 0px;\n" -" background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" -"stop:0 rgba(255, 255, 255, 0), \n" -"stop:0.5 rgba(100, 100, 100, 100), \n" -"stop:1 rgba(255, 255, 255, 0));\n" -" image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" -"}") + self.splitter.setStyleSheet( + "QSplitter::handle:vertical {\n" + "margin: 4px 0px;\n" + " background-color: qlineargradient(x1:0, y1:0, x2:1, y2:0, \n" + "stop:0 rgba(255, 255, 255, 0), \n" + "stop:0.5 rgba(100, 100, 100, 100), \n" + "stop:1 rgba(255, 255, 255, 0));\n" + " image: url(:/icons/icons/splitter_handle_horizontal.svg);\n" + "}" + ) self.splitter.setOrientation(QtCore.Qt.Vertical) self.splitter.setHandleWidth(6) self.splitter.setObjectName("splitter") @@ -75,6 +83,13 @@ def setupUi(self, Interpretation): def retranslateUi(self, Interpretation): _translate = QtCore.QCoreApplication.translate Interpretation.setWindowTitle(_translate("Interpretation", "Form")) - self.labelGettingStarted.setText(_translate("Interpretation", "Open a file or record a new signal using the File menu to get started.
")) + self.labelGettingStarted.setText( + _translate( + "Interpretation", + "Open a file or record a new signal using the File menu to get started.
", + ) + ) + + from urh.ui.ScrollArea import ScrollArea from . import urh_rc diff --git a/src/urh/ui/urh_rc.py b/src/urh/ui/urh_rc.py index d7cadd4166..82874cfbb7 100644 --- a/src/urh/ui/urh_rc.py +++ b/src/urh/ui/urh_rc.py @@ -13158,7 +13158,7 @@ \x00\x00\x01\x71\x40\xc9\x02\x46\ " -qt_version = [int(v) for v in QtCore.qVersion().split('.')] +qt_version = [int(v) for v in QtCore.qVersion().split(".")] if qt_version < [5, 8, 0]: rcc_version = 1 qt_resource_struct = qt_resource_struct_v1 @@ -13166,10 +13166,17 @@ rcc_version = 2 qt_resource_struct = qt_resource_struct_v2 + def qInitResources(): - QtCore.qRegisterResourceData(rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data) + QtCore.qRegisterResourceData( + rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data + ) + def qCleanupResources(): - QtCore.qUnregisterResourceData(rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data) + QtCore.qUnregisterResourceData( + rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data + ) + qInitResources() diff --git a/src/urh/ui/views/DirectoryTreeView.py b/src/urh/ui/views/DirectoryTreeView.py index d4ca336e3d..24829a3800 100644 --- a/src/urh/ui/views/DirectoryTreeView.py +++ b/src/urh/ui/views/DirectoryTreeView.py @@ -23,11 +23,17 @@ def create_directory(self): return model = self.model().sourceModel() - dir_name, ok = QInputDialog.getText(self, self.tr("Create Directory"), self.tr("Directory name")) + dir_name, ok = QInputDialog.getText( + self, self.tr("Create Directory"), self.tr("Directory name") + ) if ok and len(dir_name) > 0: if not model.mkdir(index, dir_name).isValid(): - QMessageBox.information(self, self.tr("Create Directory"), self.tr("Failed to create the directory")) + QMessageBox.information( + self, + self.tr("Create Directory"), + self.tr("Failed to create the directory"), + ) def remove(self): index = self.model().mapToSource(self.currentIndex()) # type: QModelIndex @@ -41,16 +47,23 @@ def remove(self): ok = model.remove(index) if not ok: - QMessageBox.information(self, self.tr("Remove"), - self.tr("Failed to remove {0}".format(model.fileName(index)))) + QMessageBox.information( + self, + self.tr("Remove"), + self.tr("Failed to remove {0}".format(model.fileName(index))), + ) def create_context_menu(self) -> QMenu: menu = QMenu(self) index = self.model().mapToSource(self.currentIndex()) # type: QModelIndex if index.isValid(): - current_index_info = self.model().sourceModel().fileInfo(index) # type: QFileInfo + current_index_info = ( + self.model().sourceModel().fileInfo(index) + ) # type: QFileInfo if current_index_info.isDir(): - if os.path.isfile(os.path.join(current_index_info.filePath(), settings.PROJECT_FILE)): + if os.path.isfile( + os.path.join(current_index_info.filePath(), settings.PROJECT_FILE) + ): open_action = menu.addAction("Open project") open_action.setIcon(QIcon(":/icons/icons/appicon.png")) else: @@ -68,7 +81,9 @@ def create_context_menu(self) -> QMenu: menu.addSeparator() open_in_explorer_action = menu.addAction("Open in file manager...") - open_in_explorer_action.triggered.connect(self.on_open_explorer_action_triggered) + open_in_explorer_action.triggered.connect( + self.on_open_explorer_action_triggered + ) return menu diff --git a/src/urh/ui/views/EditableGraphicView.py b/src/urh/ui/views/EditableGraphicView.py index 51024f1dc7..653f0350a2 100644 --- a/src/urh/ui/views/EditableGraphicView.py +++ b/src/urh/ui/views/EditableGraphicView.py @@ -62,7 +62,9 @@ def __init__(self, parent=None): self.delete_action.setIcon(QIcon.fromTheme("edit-delete")) self.addAction(self.delete_action) - self.save_as_action = QAction(self.tr("Save Signal as..."), self) # type: QAction + self.save_as_action = QAction( + self.tr("Save Signal as..."), self + ) # type: QAction self.save_as_action.setIcon(QIcon.fromTheme("document-save-as")) self.save_as_action.setShortcut(QKeySequence.SaveAs) self.save_as_action.triggered.connect(self.save_as_clicked.emit) @@ -84,7 +86,9 @@ def __init__(self, parent=None): self.insert_sine_action.triggered.connect(self.on_insert_sine_action_triggered) self.insert_sine_plugin = InsertSinePlugin() - self.insert_sine_plugin.insert_sine_wave_clicked.connect(self.on_insert_sine_wave_clicked) + self.insert_sine_plugin.insert_sine_wave_clicked.connect( + self.on_insert_sine_wave_clicked + ) def init_undo_stack(self, undo_stack): self.undo_stack = undo_stack @@ -183,13 +187,17 @@ def create_context_menu(self): none_participant_action = participant_menu.addAction("None") none_participant_action.setCheckable(True) none_participant_action.setActionGroup(participant_group) - none_participant_action.triggered.connect(self.on_none_participant_action_triggered) + none_participant_action.triggered.connect( + self.on_none_participant_action_triggered + ) if selected_msg and selected_msg.participant is None: none_participant_action.setChecked(True) for participant in self.participants: - pa = participant_menu.addAction(participant.name + " (" + participant.shortname + ")") + pa = participant_menu.addAction( + participant.name + " (" + participant.shortname + ")" + ) pa.setCheckable(True) pa.setActionGroup(participant_group) if selected_msg and selected_msg.participant == participant: @@ -227,7 +235,9 @@ def clear_horizontal_selection(self): def toggle_symbol_legend(self): if self.scene_type == 1 and self.signal is not None: - self.scene().always_show_symbols_legend = self.show_symbol_legend_action.isChecked() + self.scene().always_show_symbols_legend = ( + self.show_symbol_legend_action.isChecked() + ) self.scene().draw_sep_area(-self.signal.center_thresholds) @pyqtSlot() @@ -242,10 +252,12 @@ def on_insert_sine_action_triggered(self): logger.critical("No data to insert a sine wave to") return - dialog = self.insert_sine_plugin.get_insert_sine_dialog(original_data=original_data, - position=self.paste_position, - sample_rate=self.sample_rate, - num_samples=num_samples) + dialog = self.insert_sine_plugin.get_insert_sine_dialog( + original_data=original_data, + position=self.paste_position, + sample_rate=self.sample_rate, + num_samples=num_samples, + ) dialog.show() @pyqtSlot() @@ -253,26 +265,38 @@ def on_insert_sine_wave_clicked(self): if self.insert_sine_plugin.complex_wave is not None and self.signal is not None: self.clear_horizontal_selection() - insert_action = EditSignalAction(signal=self.signal, protocol=self.protocol, - data_to_insert=self.insert_sine_plugin.complex_wave, - position=self.paste_position, - mode=EditAction.insert, cache_qad=self.cache_qad) + insert_action = EditSignalAction( + signal=self.signal, + protocol=self.protocol, + data_to_insert=self.insert_sine_plugin.complex_wave, + position=self.paste_position, + mode=EditAction.insert, + cache_qad=self.cache_qad, + ) self.undo_stack.push(insert_action) @pyqtSlot() def on_copy_action_triggered(self): if self.something_is_selected: - self.stored_item = self.signal.iq_array[int(self.selection_area.start):int(self.selection_area.end)] + self.stored_item = self.signal.iq_array[ + int(self.selection_area.start) : int(self.selection_area.end) + ] @pyqtSlot() def on_paste_action_triggered(self): if self.stored_item is not None: # paste_position is set in ContextMenuEvent self.clear_horizontal_selection() - paste_action = EditSignalAction(signal=self.signal, protocol=self.protocol, - start=self.selection_area.start, end=self.selection_area.end, - data_to_insert=self.stored_item, position=self.paste_position, - mode=EditAction.paste, cache_qad=self.cache_qad) + paste_action = EditSignalAction( + signal=self.signal, + protocol=self.protocol, + start=self.selection_area.start, + end=self.selection_area.end, + data_to_insert=self.stored_item, + position=self.paste_position, + mode=EditAction.paste, + cache_qad=self.cache_qad, + ) self.undo_stack.push(paste_action) @pyqtSlot() @@ -280,9 +304,14 @@ def on_delete_action_triggered(self): if self.something_is_selected: start, end = self.selection_area.start, self.selection_area.end self.clear_horizontal_selection() - del_action = EditSignalAction(signal=self.signal, protocol=self.protocol, - start=start, end=end, - mode=EditAction.delete, cache_qad=self.cache_qad) + del_action = EditSignalAction( + signal=self.signal, + protocol=self.protocol, + start=start, + end=end, + mode=EditAction.delete, + cache_qad=self.cache_qad, + ) self.undo_stack.push(del_action) @pyqtSlot() @@ -290,21 +319,33 @@ def on_crop_action_triggered(self): if self.something_is_selected: start, end = self.selection_area.start, self.selection_area.end self.clear_horizontal_selection() - crop_action = EditSignalAction(signal=self.signal, protocol=self.protocol, - start=start, end=end, - mode=EditAction.crop, cache_qad=self.cache_qad) + crop_action = EditSignalAction( + signal=self.signal, + protocol=self.protocol, + start=start, + end=end, + mode=EditAction.crop, + cache_qad=self.cache_qad, + ) self.undo_stack.push(crop_action) @pyqtSlot() def on_mute_action_triggered(self): - mute_action = EditSignalAction(signal=self.signal, protocol=self.protocol, - start=self.selection_area.start, end=self.selection_area.end, - mode=EditAction.mute, cache_qad=self.cache_qad) + mute_action = EditSignalAction( + signal=self.signal, + protocol=self.protocol, + start=self.selection_area.start, + end=self.selection_area.end, + mode=EditAction.mute, + cache_qad=self.cache_qad, + ) self.undo_stack.push(mute_action) @pyqtSlot() def on_create_action_triggered(self): - self.create_clicked.emit(int(self.selection_area.start), int(self.selection_area.end)) + self.create_clicked.emit( + int(self.selection_area.start), int(self.selection_area.end) + ) @pyqtSlot() def on_none_participant_action_triggered(self): diff --git a/src/urh/ui/views/EpicGraphicView.py b/src/urh/ui/views/EpicGraphicView.py index 3d71fcbc94..e348cfab9c 100644 --- a/src/urh/ui/views/EpicGraphicView.py +++ b/src/urh/ui/views/EpicGraphicView.py @@ -12,6 +12,7 @@ class EpicGraphicView(EditableGraphicView): """ Tied to Signal Frame (Interpretation) """ + save_clicked = pyqtSignal() def __init__(self, parent=None): @@ -44,8 +45,10 @@ def sample_rate(self, value): @property def selected_messages(self): if self.something_is_selected and self.protocol: - sb, _, eb, _ = self.protocol.get_bitseq_from_selection(self.selection_area.start, abs(self.selection_area.width)) - return self.protocol.messages[sb:eb + 1] + sb, _, eb, _ = self.protocol.get_bitseq_from_selection( + self.selection_area.start, abs(self.selection_area.width) + ) + return self.protocol.messages[sb : eb + 1] else: return [] @@ -68,8 +71,11 @@ def _get_sub_path_ranges_and_colors(self, start: float, end: float): if message.bit_sample_pos[-2] < start: continue - color = None if message.participant is None else settings.PARTICIPANT_COLORS[ - message.participant.color_index] + color = ( + None + if message.participant is None + else settings.PARTICIPANT_COLORS[message.participant.color_index] + ) if color is None: continue @@ -87,7 +93,9 @@ def _get_sub_path_ranges_and_colors(self, start: float, end: float): break # Data part of the message - sub_path_ranges.append((message.bit_sample_pos[0], message.bit_sample_pos[-2] + 1)) + sub_path_ranges.append( + (message.bit_sample_pos[0], message.bit_sample_pos[-2] + 1) + ) colors.append(color) start = message.bit_sample_pos[-2] + 1 @@ -103,4 +111,3 @@ def _get_sub_path_ranges_and_colors(self, start: float, end: float): @pyqtSlot() def on_save_action_triggered(self): self.save_clicked.emit() - diff --git a/src/urh/ui/views/GeneratorTableView.py b/src/urh/ui/views/GeneratorTableView.py index 133260b5d4..eb06389714 100644 --- a/src/urh/ui/views/GeneratorTableView.py +++ b/src/urh/ui/views/GeneratorTableView.py @@ -1,6 +1,16 @@ from PyQt5.QtCore import Qt, QRect, pyqtSignal, pyqtSlot -from PyQt5.QtGui import QDragMoveEvent, QDragEnterEvent, QPainter, QBrush, QColor, QPen, QDropEvent, QDragLeaveEvent, \ - QContextMenuEvent, QIcon +from PyQt5.QtGui import ( + QDragMoveEvent, + QDragEnterEvent, + QPainter, + QBrush, + QColor, + QPen, + QDropEvent, + QDragLeaveEvent, + QContextMenuEvent, + QIcon, +) from PyQt5.QtWidgets import QActionGroup, QInputDialog from PyQt5.QtWidgets import QHeaderView, QAbstractItemView, QStyleOption, QMenu @@ -34,26 +44,44 @@ def dragMoveEvent(self, event: QDragMoveEvent): pos = event.pos() row = self.rowAt(pos.y()) - index = self.model().createIndex(row, 0) # this always get the default 0 column index + index = self.model().createIndex( + row, 0 + ) # this always get the default 0 column index rect = self.visualRect(index) rect_left = self.visualRect(index.sibling(index.row(), 0)) - rect_right = self.visualRect(index.sibling(index.row(), - self.horizontalHeader().logicalIndex( - self.model().columnCount() - 1))) # in case section has been moved + rect_right = self.visualRect( + index.sibling( + index.row(), + self.horizontalHeader().logicalIndex(self.model().columnCount() - 1), + ) + ) # in case section has been moved self.drop_indicator_position = self.position(event.pos(), rect) if self.drop_indicator_position == self.AboveItem: - self.drop_indicator_rect = QRect(rect_left.left(), rect_left.top(), rect_right.right() - rect_left.left(), 0) + self.drop_indicator_rect = QRect( + rect_left.left(), + rect_left.top(), + rect_right.right() - rect_left.left(), + 0, + ) event.accept() elif self.drop_indicator_position == self.BelowItem: - self.drop_indicator_rect = QRect(rect_left.left(), rect_left.bottom(), rect_right.right() - rect_left.left(), - 0) + self.drop_indicator_rect = QRect( + rect_left.left(), + rect_left.bottom(), + rect_right.right() - rect_left.left(), + 0, + ) event.accept() elif self.drop_indicator_position == self.OnItem: - self.drop_indicator_rect = QRect(rect_left.left(), rect_left.bottom(), rect_right.right() - rect_left.left(), - 0) + self.drop_indicator_rect = QRect( + rect_left.left(), + rect_left.bottom(), + rect_right.right() - rect_left.left(), + 0, + ) event.accept() else: self.drop_indicator_rect = QRect() @@ -62,23 +90,38 @@ def dragMoveEvent(self, event: QDragMoveEvent): self.viewport().update() def __rect_for_row(self, row): - index = self.model().createIndex(row, 0) # this always get the default 0 column index + index = self.model().createIndex( + row, 0 + ) # this always get the default 0 column index # rect = self.visualRect(index) rect_left = self.visualRect(index.sibling(index.row(), 0)) - rect_right = self.visualRect(index.sibling(index.row(), - self.horizontalHeader().logicalIndex( - self.model().columnCount() - 1))) # in case section has been moved - return QRect(rect_left.left(), rect_left.bottom(), rect_right.right() - rect_left.left(), 0) + rect_right = self.visualRect( + index.sibling( + index.row(), + self.horizontalHeader().logicalIndex(self.model().columnCount() - 1), + ) + ) # in case section has been moved + return QRect( + rect_left.left(), + rect_left.bottom(), + rect_right.right() - rect_left.left(), + 0, + ) def dropEvent(self, event: QDropEvent): self.drag_active = False row = self.rowAt(event.pos().y()) - index = self.model().createIndex(row, 0) # this always get the default 0 column index + index = self.model().createIndex( + row, 0 + ) # this always get the default 0 column index rect = self.visualRect(index) drop_indicator_position = self.position(event.pos(), rect) if row == -1: row = self.model().row_count - 1 - elif drop_indicator_position == self.BelowItem or drop_indicator_position == self.OnItem: + elif ( + drop_indicator_position == self.BelowItem + or drop_indicator_position == self.OnItem + ): row += 1 self.model().dropped_row = row @@ -164,7 +207,9 @@ def create_context_menu(self) -> QMenu: self.encoding_actions = {} if not self.selection_is_empty: - selected_encoding = self.model().protocol.messages[self.selected_rows[0]].decoder + selected_encoding = ( + self.model().protocol.messages[self.selected_rows[0]].decoder + ) for i in self.selected_rows: if self.model().protocol.messages[i].decoder != selected_encoding: selected_encoding = None @@ -185,7 +230,9 @@ def create_context_menu(self) -> QMenu: ea.triggered.connect(self.on_encoding_action_triggered) menu.addSeparator() - de_bruijn_action = menu.addAction("Generate De Bruijn Sequence from Selection") + de_bruijn_action = menu.addAction( + "Generate De Bruijn Sequence from Selection" + ) de_bruijn_action.triggered.connect(self.on_de_bruijn_action_triggered) return menu @@ -201,7 +248,9 @@ def on_clear_action_triggered(self): @pyqtSlot() def on_encoding_action_triggered(self): for row in self.selected_rows: - self.model().protocol.messages[row].decoder = self.encoding_actions[self.sender()] + self.model().protocol.messages[row].decoder = self.encoding_actions[ + self.sender() + ] self.encodings_updated.emit() @pyqtSlot() @@ -217,7 +266,12 @@ def on_de_bruijn_action_triggered(self): @pyqtSlot() def on_add_message_action_triggered(self): row = self.rowAt(self.context_menu_pos.y()) - num_bits, ok = QInputDialog.getInt(self, self.tr("How many bits shall the new message have?"), - self.tr("Number of bits:"), 42, 1) + num_bits, ok = QInputDialog.getInt( + self, + self.tr("How many bits shall the new message have?"), + self.tr("Number of bits:"), + 42, + 1, + ) if ok: self.model().add_empty_row_behind(row, num_bits) diff --git a/src/urh/ui/views/GeneratorTreeView.py b/src/urh/ui/views/GeneratorTreeView.py index 7c56715b81..6742736116 100644 --- a/src/urh/ui/views/GeneratorTreeView.py +++ b/src/urh/ui/views/GeneratorTreeView.py @@ -16,4 +16,4 @@ def model(self) -> GeneratorTreeModel: return super().model() def selectionModel(self) -> QItemSelectionModel: - return super().selectionModel() \ No newline at end of file + return super().selectionModel() diff --git a/src/urh/ui/views/LabelValueTableView.py b/src/urh/ui/views/LabelValueTableView.py index f46b8a8bd9..b6d235ae19 100644 --- a/src/urh/ui/views/LabelValueTableView.py +++ b/src/urh/ui/views/LabelValueTableView.py @@ -17,13 +17,30 @@ class LabelValueTableView(QTableView): def __init__(self, parent=None): super().__init__(parent) - self.setItemDelegateForColumn(1, ComboBoxDelegate([""] * len(settings.LABEL_COLORS), - colors=settings.LABEL_COLORS, - parent=self)) - self.setItemDelegateForColumn(2, ComboBoxDelegate(ProtocolLabel.DISPLAY_FORMATS, parent=self)) - - orders = OrderedDict([("Big Endian (BE)", [bo + "/BE" for bo in ProtocolLabel.DISPLAY_BIT_ORDERS]), - ("Little Endian (LE)", [bo + "/LE" for bo in ProtocolLabel.DISPLAY_BIT_ORDERS])]) + self.setItemDelegateForColumn( + 1, + ComboBoxDelegate( + [""] * len(settings.LABEL_COLORS), + colors=settings.LABEL_COLORS, + parent=self, + ), + ) + self.setItemDelegateForColumn( + 2, ComboBoxDelegate(ProtocolLabel.DISPLAY_FORMATS, parent=self) + ) + + orders = OrderedDict( + [ + ( + "Big Endian (BE)", + [bo + "/BE" for bo in ProtocolLabel.DISPLAY_BIT_ORDERS], + ), + ( + "Little Endian (LE)", + [bo + "/LE" for bo in ProtocolLabel.DISPLAY_BIT_ORDERS], + ), + ] + ) self.setItemDelegateForColumn(3, SectionComboBoxDelegate(orders, parent=self)) @@ -54,16 +71,22 @@ def create_context_menu(self): if len(self.model().controller.proto_analyzer.message_types) > 1: msg_type_menu = menu.addMenu("Copy to message type") - for i, message_type in enumerate(self.model().controller.proto_analyzer.message_types): + for i, message_type in enumerate( + self.model().controller.proto_analyzer.message_types + ): if message_type != self.model().controller.active_message_type: msg_type_action = msg_type_menu.addAction(message_type.name) msg_type_action.setData(i) - msg_type_action.triggered.connect(self.on_copy_to_msg_type_action_triggered) + msg_type_action.triggered.connect( + self.on_copy_to_msg_type_action_triggered + ) menu.addAction(self.del_rows_action) menu.addSeparator() configure_field_types_action = menu.addAction("Configure field types...") - configure_field_types_action.triggered.connect(self.configure_field_types_action_triggered.emit) + configure_field_types_action.triggered.connect( + self.configure_field_types_action_triggered.emit + ) return menu @@ -86,4 +109,6 @@ def on_edit_label_action_triggered(self): @pyqtSlot() def on_copy_to_msg_type_action_triggered(self): min_row, max_row = self.selected_min_max_row - self.model().add_labels_to_message_type(min_row, max_row, int(self.sender().data())) + self.model().add_labels_to_message_type( + min_row, max_row, int(self.sender().data()) + ) diff --git a/src/urh/ui/views/LoggingGraphicsView.py b/src/urh/ui/views/LoggingGraphicsView.py index d6e7b2d88c..d980ce70b1 100644 --- a/src/urh/ui/views/LoggingGraphicsView.py +++ b/src/urh/ui/views/LoggingGraphicsView.py @@ -5,7 +5,6 @@ class LoggingGraphicsView(QGraphicsView): - def __init__(self, parent=None): super().__init__(parent) @@ -13,11 +12,17 @@ def __init__(self, parent=None): self.log_selected_action = QAction(self.tr("Log selected items"), self) self.log_selected_action.setShortcut(QKeySequence("L")) - self.log_selected_action.triggered.connect(self.on_log_selected_action_triggered) + self.log_selected_action.triggered.connect( + self.on_log_selected_action_triggered + ) - self.do_not_log_selected_action = QAction(self.tr("Do not log selected items"), self) + self.do_not_log_selected_action = QAction( + self.tr("Do not log selected items"), self + ) self.do_not_log_selected_action.setShortcut(QKeySequence("N")) - self.do_not_log_selected_action.triggered.connect(self.on_do_not_log_selected_action_triggered) + self.do_not_log_selected_action.triggered.connect( + self.on_do_not_log_selected_action_triggered + ) self.select_all_action = QAction(self.tr("Select all"), self) self.select_all_action.setShortcut(QKeySequence.SelectAll) @@ -38,9 +43,12 @@ def create_context_menu(self): menu.addAction(self.log_selected_action) menu.addAction(self.do_not_log_selected_action) - SimulatorGraphicsView.add_select_actions_to_menu(menu, self.scene(), - select_to_trigger=self.on_select_to_action_triggered, - select_from_trigger=self.on_select_from_action_triggered) + SimulatorGraphicsView.add_select_actions_to_menu( + menu, + self.scene(), + select_to_trigger=self.on_select_to_action_triggered, + select_from_trigger=self.on_select_from_action_triggered, + ) return menu @@ -48,7 +56,9 @@ def on_select_from_action_triggered(self): self.scene().select_messages_with_participant(self.sender().data()) def on_select_to_action_triggered(self): - self.scene().select_messages_with_participant(self.sender().data(), from_part=False) + self.scene().select_messages_with_participant( + self.sender().data(), from_part=False + ) def on_log_selected_action_triggered(self): self.scene().log_selected_items(True) diff --git a/src/urh/ui/views/MessageTypeTableView.py b/src/urh/ui/views/MessageTypeTableView.py index 0cb1805393..b7843312e3 100644 --- a/src/urh/ui/views/MessageTypeTableView.py +++ b/src/urh/ui/views/MessageTypeTableView.py @@ -52,9 +52,13 @@ def create_context_menu(self): menu.addAction(self.del_rows_action) menu.addSeparator() - update_message_types_action = menu.addAction("Update automatically assigned message types") + update_message_types_action = menu.addAction( + "Update automatically assigned message types" + ) update_message_types_action.setIcon(QIcon.fromTheme("view-refresh")) - update_message_types_action.triggered.connect(self.auto_message_type_update_triggered.emit) + update_message_types_action.triggered.connect( + self.auto_message_type_update_triggered.emit + ) menu.addSeparator() show_all_action = menu.addAction("Show all message types") @@ -77,9 +81,13 @@ def delete_rows(self): @pyqtSlot() def on_show_all_action_triggered(self): for i in range(self.model().rowCount()): - self.model().setData(self.model().index(i, 0), Qt.Checked, role=Qt.CheckStateRole) + self.model().setData( + self.model().index(i, 0), Qt.Checked, role=Qt.CheckStateRole + ) @pyqtSlot() def on_hide_all_action_triggered(self): for i in range(self.model().rowCount()): - self.model().setData(self.model().index(i, 0), Qt.Unchecked, role=Qt.CheckStateRole) + self.model().setData( + self.model().index(i, 0), Qt.Unchecked, role=Qt.CheckStateRole + ) diff --git a/src/urh/ui/views/ModulatorTreeView.py b/src/urh/ui/views/ModulatorTreeView.py index 7d73c48f38..ca5cea6051 100644 --- a/src/urh/ui/views/ModulatorTreeView.py +++ b/src/urh/ui/views/ModulatorTreeView.py @@ -18,4 +18,4 @@ def selectionModel(self) -> QItemSelectionModel: def selectionChanged(self, QItemSelection, QItemSelection_1): self.selection_changed.emit() - super().selectionChanged(QItemSelection, QItemSelection_1) \ No newline at end of file + super().selectionChanged(QItemSelection, QItemSelection_1) diff --git a/src/urh/ui/views/ParticipantTableView.py b/src/urh/ui/views/ParticipantTableView.py index 96047aa089..d2230dcc97 100644 --- a/src/urh/ui/views/ParticipantTableView.py +++ b/src/urh/ui/views/ParticipantTableView.py @@ -24,7 +24,9 @@ def selected_columns(self) -> (int, int): if selection.isEmpty(): return 0, self.model().columnCount() - 1 - return min([rng.left() for rng in selection]), max([rng.right() for rng in selection]) + return min([rng.left() for rng in selection]), max( + [rng.right() for rng in selection] + ) def select(self, row_1, col_1, row_2, col_2): selection = QItemSelection() @@ -52,9 +54,13 @@ def create_context_menu(self): menu.addAction(self.remove_action) menu.addSeparator() - move_up = menu.addAction(QIcon.fromTheme("go-up"), "Move selected participants up") + move_up = menu.addAction( + QIcon.fromTheme("go-up"), "Move selected participants up" + ) move_up.triggered.connect(self.on_move_up_action_triggered) - move_down = menu.addAction(QIcon.fromTheme("go-down"), "Move selected participants down") + move_down = menu.addAction( + QIcon.fromTheme("go-down"), "Move selected participants down" + ) move_down.triggered.connect(self.on_move_down_action_triggered) return menu @@ -72,9 +78,14 @@ def refresh_participant_table(self): for row in range(n): self.closePersistentEditor(self.model().index(row, 3)) - self.setItemDelegateForColumn(2, ComboBoxDelegate([""] * len(settings.PARTICIPANT_COLORS), - colors=settings.PARTICIPANT_COLORS, - parent=self)) + self.setItemDelegateForColumn( + 2, + ComboBoxDelegate( + [""] * len(settings.PARTICIPANT_COLORS), + colors=settings.PARTICIPANT_COLORS, + parent=self, + ), + ) self.setItemDelegateForColumn(3, ComboBoxDelegate(items, parent=self)) for row in range(n): @@ -94,7 +105,7 @@ def on_move_up_action_triggered(self): col_start, col_end = self.selected_columns start, end = self.model().move_up(self.selectionModel().selection()) if start is not None and end is not None: - self.select(start-1, col_start, end-1, col_end) + self.select(start - 1, col_start, end - 1, col_end) @pyqtSlot() def on_move_down_action_triggered(self): diff --git a/src/urh/ui/views/ProtocolLabelTableView.py b/src/urh/ui/views/ProtocolLabelTableView.py index 6a680660d2..2834789d48 100644 --- a/src/urh/ui/views/ProtocolLabelTableView.py +++ b/src/urh/ui/views/ProtocolLabelTableView.py @@ -33,8 +33,11 @@ def create_context_menu(self): if isinstance(self.model(), SimulatorMessageFieldModel): value_type_group = QActionGroup(self) value_type_menu = menu.addMenu("Set value type") - labels = [self.model().message_type[i] for i in self.selected_rows - if not self.model().message_type[i].is_checksum_label] + labels = [ + self.model().message_type[i] + for i in self.selected_rows + if not self.model().message_type[i].is_checksum_label + ] for i, value_type in enumerate(SimulatorProtocolLabel.VALUE_TYPES): va = value_type_menu.addAction(value_type) @@ -61,4 +64,3 @@ def on_set_value_type_action_triggered(self): assert isinstance(self.model(), SimulatorMessageFieldModel) value_type_index = self.sender().data() self.model().set_value_type_index(self.selected_rows, value_type_index) - diff --git a/src/urh/ui/views/ProtocolTableView.py b/src/urh/ui/views/ProtocolTableView.py index 33c3040c85..528a880ae4 100644 --- a/src/urh/ui/views/ProtocolTableView.py +++ b/src/urh/ui/views/ProtocolTableView.py @@ -53,7 +53,9 @@ def selected_messages(self): rows = set(i.row() for i in self.selectionModel().selectedIndexes()) return [messages[i] for i in rows] - def selectionChanged(self, selection_1: QItemSelection, selection_2: QItemSelection): + def selectionChanged( + self, selection_1: QItemSelection, selection_2: QItemSelection + ): self.selection_changed.emit() super().selectionChanged(selection_1, selection_2) @@ -70,7 +72,11 @@ def dropEvent(self, event: QDropEvent): def create_context_menu(self): menu = super().create_context_menu() row = self.rowAt(self.context_menu_pos.y()) - cols = [index.column() for index in self.selectionModel().selectedIndexes() if index.row() == row] + cols = [ + index.column() + for index in self.selectionModel().selectedIndexes() + if index.row() == row + ] cols.sort() pos = self.context_menu_pos @@ -113,10 +119,15 @@ def create_context_menu(self): new_message_type_action = message_type_menu.addAction("Create new") new_message_type_action.setIcon(QIcon.fromTheme("list-add")) - new_message_type_action.triggered.connect(self.on_new_message_type_action_triggered) - - if self.model().participants and self.model().protocol and not self.selection_is_empty: - + new_message_type_action.triggered.connect( + self.on_new_message_type_action_triggered + ) + + if ( + self.model().participants + and self.model().protocol + and not self.selection_is_empty + ): participant_group = QActionGroup(self) participant_menu_str = self.tr("Participant") if selected_participant is None: @@ -129,13 +140,17 @@ def create_context_menu(self): none_participant_action = participant_menu.addAction("None") none_participant_action.setCheckable(True) none_participant_action.setActionGroup(participant_group) - none_participant_action.triggered.connect(self.on_none_participant_action_triggered) + none_participant_action.triggered.connect( + self.on_none_participant_action_triggered + ) if selected_participant is None: none_participant_action.setChecked(True) for participant in self.model().participants: - pa = participant_menu.addAction(participant.name + " (" + participant.shortname + ")") + pa = participant_menu.addAction( + participant.name + " (" + participant.shortname + ")" + ) pa.setCheckable(True) pa.setActionGroup(participant_group) if selected_participant == participant: @@ -152,23 +167,31 @@ def create_context_menu(self): menu.addAction(self.hide_row_action) hidden_rows = self.model().hidden_rows if len(hidden_rows) > 0: - show_row_action = menu.addAction(self.tr("Show all rows (reset {0:d} hidden)".format(len(hidden_rows)))) + show_row_action = menu.addAction( + self.tr("Show all rows (reset {0:d} hidden)".format(len(hidden_rows))) + ) show_row_action.triggered.connect(self.on_show_row_action_triggered) if self.model().refindex != -1: menu.addAction(self.ref_message_action) if not self.model().is_writeable: - show_interpretation_action = menu.addAction(self.tr("Show selection in Interpretation")) + show_interpretation_action = menu.addAction( + self.tr("Show selection in Interpretation") + ) show_interpretation_action.setIcon(QIcon.fromTheme("zoom-select")) - show_interpretation_action.triggered.connect(self.on_show_in_interpretation_action_triggered) + show_interpretation_action.triggered.connect( + self.on_show_in_interpretation_action_triggered + ) if self.model().is_writeable: writeable_action = menu.addAction(self.tr("Writeable")) writeable_action.setCheckable(True) writeable_action.setChecked(True) else: - writeable_action = menu.addAction(self.tr("Writeable (decouples from signal)")) + writeable_action = menu.addAction( + self.tr("Writeable (decouples from signal)") + ) writeable_action.setCheckable(True) writeable_action.setChecked(False) @@ -180,8 +203,13 @@ def create_context_menu(self): for plugin in self.controller.plugin_manager.protocol_plugins: if plugin.enabled: - act = plugin.get_action(self, undo_stack, self.selection_range(), - self.controller.proto_analyzer, view) + act = plugin.get_action( + self, + undo_stack, + self.selection_range(), + self.controller.proto_analyzer, + view, + ) if act is not None: menu.addAction(act) @@ -257,7 +285,9 @@ def on_participant_action_triggered(self): @pyqtSlot() def on_message_type_action_triggered(self): - self.messagetype_selected.emit(self.message_type_actions[self.sender()], self.selected_messages) + self.messagetype_selected.emit( + self.message_type_actions[self.sender()], self.selected_messages + ) @pyqtSlot() def on_new_message_type_action_triggered(self): diff --git a/src/urh/ui/views/ProtocolTreeView.py b/src/urh/ui/views/ProtocolTreeView.py index b72ad5bd30..38e7e2ef1d 100644 --- a/src/urh/ui/views/ProtocolTreeView.py +++ b/src/urh/ui/views/ProtocolTreeView.py @@ -1,7 +1,7 @@ from PyQt5.QtCore import QItemSelection, pyqtSlot from PyQt5.QtCore import pyqtSignal, QItemSelectionModel, Qt from PyQt5.QtGui import QContextMenuEvent, QDropEvent, QIcon -from PyQt5.QtWidgets import QTreeView, QAbstractItemView, QMenu +from PyQt5.QtWidgets import QTreeView, QAbstractItemView, QMenu from urh.models.ProtocolTreeModel import ProtocolTreeModel @@ -35,8 +35,13 @@ def create_context_menu(self): new_group_action.triggered.connect(self.on_new_group_action_triggered) item = self.model().getItem(self.indexAt(self.context_menu_pos)) - selected_items = [self.model().getItem(index) for index in self.selectionModel().selectedIndexes()] - selected_protocols = [item.protocol for item in selected_items if not item.is_group] + selected_items = [ + self.model().getItem(index) + for index in self.selectionModel().selectedIndexes() + ] + selected_protocols = [ + item.protocol for item in selected_items if not item.is_group + ] self.move_to_group_actions.clear() if item.is_group: @@ -58,14 +63,18 @@ def create_context_menu(self): for i in other_groups: group_name = self.model().rootItem.child(i).data() move_to_group_action = move_to_group_menu.addAction(group_name) - move_to_group_action.triggered.connect(self.on_move_to_group_action_triggered) + move_to_group_action.triggered.connect( + self.on_move_to_group_action_triggered + ) self.move_to_group_actions[move_to_group_action] = i if item != self.model().rootItem: menu.addSeparator() sort_group_elements_action = menu.addAction("Sort Group Elements") sort_group_elements_action.setIcon(QIcon.fromTheme("view-sort-ascending")) - sort_group_elements_action.triggered.connect(self.on_sort_group_elements_action_triggered) + sort_group_elements_action.triggered.connect( + self.on_sort_group_elements_action_triggered + ) return menu @@ -93,14 +102,22 @@ def on_new_group_action_triggered(self): @pyqtSlot() def on_move_to_group_action_triggered(self): - selected_items = [self.model().getItem(index) for index in self.selectionModel().selectedIndexes()] + selected_items = [ + self.model().getItem(index) + for index in self.selectionModel().selectedIndexes() + ] i = self.move_to_group_actions[self.sender()] self.model().move_to_group(selected_items, i) @pyqtSlot() def on_close_action_triggered(self): - selected_items = [self.model().getItem(index) for index in self.selectionModel().selectedIndexes()] - selected_protocols = [item.protocol for item in selected_items if not item.is_group] + selected_items = [ + self.model().getItem(index) + for index in self.selectionModel().selectedIndexes() + ] + selected_protocols = [ + item.protocol for item in selected_items if not item.is_group + ] self.close_wanted.emit(selected_protocols) @pyqtSlot() diff --git a/src/urh/ui/views/SelectableGraphicView.py b/src/urh/ui/views/SelectableGraphicView.py index bead32d6fb..f808c064f4 100644 --- a/src/urh/ui/views/SelectableGraphicView.py +++ b/src/urh/ui/views/SelectableGraphicView.py @@ -59,11 +59,15 @@ def has_horizontal_selection(self) -> bool: @property def hold_shift_to_drag(self) -> bool: - return settings.read('hold_shift_to_drag', True, type=bool) + return settings.read("hold_shift_to_drag", True, type=bool) @property def something_is_selected(self) -> bool: - return hasattr(self, "selection_area") and self.selection_area is not None and not self.selection_area.is_empty + return ( + hasattr(self, "selection_area") + and self.selection_area is not None + and not self.selection_area.is_empty + ) def is_pos_in_separea(self, pos: QPoint): """ @@ -106,9 +110,12 @@ def mousePressEvent(self, event: QMouseEvent): cursor = self.cursor().shape() has_shift_modifier = event.modifiers() == Qt.ShiftModifier - is_in_shift_mode = (has_shift_modifier and self.hold_shift_to_drag) \ - or (not has_shift_modifier and not self.hold_shift_to_drag) \ - and cursor != Qt.SplitHCursor and cursor != Qt.SplitVCursor + is_in_shift_mode = ( + (has_shift_modifier and self.hold_shift_to_drag) + or (not has_shift_modifier and not self.hold_shift_to_drag) + and cursor != Qt.SplitHCursor + and cursor != Qt.SplitVCursor + ) if event.buttons() == Qt.LeftButton and is_in_shift_mode: self.setCursor(Qt.ClosedHandCursor) @@ -118,7 +125,10 @@ def mousePressEvent(self, event: QMouseEvent): self.separation_area_moving = True self.setCursor(Qt.SplitVCursor) - elif self.selection_area.is_empty or self.selection_area.selected_edge is None: + elif ( + self.selection_area.is_empty + or self.selection_area.selected_edge is None + ): # Create new selection self.mouse_press_pos = event.pos() self.mouse_pos = event.pos() @@ -137,11 +147,15 @@ def mouseMoveEvent(self, event: QMouseEvent): if self.grab_start is not None: move_x = self.grab_start.x() - event.pos().x() - self.horizontalScrollBar().setValue(self.horizontalScrollBar().value() + move_x) + self.horizontalScrollBar().setValue( + self.horizontalScrollBar().value() + move_x + ) if self.move_y_with_drag: move_y = self.grab_start.y() - event.pos().y() - self.verticalScrollBar().setValue(self.verticalScrollBar().value() + move_y) + self.verticalScrollBar().setValue( + self.verticalScrollBar().value() + move_y + ) self.grab_start = event.pos() return @@ -150,8 +164,14 @@ def mouseMoveEvent(self, event: QMouseEvent): y_sep = self.mapToScene(event.pos()).y() y = self.sceneRect().y() h = self.sceneRect().height() - if y < y_sep < y + h and hasattr(self, "signal") and self.signal is not None: - self.scene().draw_sep_area(-self.signal.get_thresholds_for_center(-y_sep), show_symbols=True) + if ( + y < y_sep < y + h + and hasattr(self, "signal") + and self.signal is not None + ): + self.scene().draw_sep_area( + -self.signal.get_thresholds_for_center(-y_sep), show_symbols=True + ) elif self.is_pos_in_separea(self.mapToScene(event.pos())): self.setCursor(Qt.SplitVCursor) elif cursor == Qt.SplitVCursor and self.has_horizontal_selection: @@ -161,8 +181,9 @@ def mouseMoveEvent(self, event: QMouseEvent): pos = self.mapToScene(event.pos()) roi_edge = self.selection_area.get_selected_edge(pos, self.transform()) if roi_edge is None: - if (cursor == Qt.SplitHCursor and self.has_horizontal_selection) \ - or (cursor == Qt.SplitVCursor and not self.has_horizontal_selection): + if (cursor == Qt.SplitHCursor and self.has_horizontal_selection) or ( + cursor == Qt.SplitVCursor and not self.has_horizontal_selection + ): self.unsetCursor() return elif roi_edge == 0 or roi_edge == 1: @@ -172,7 +193,6 @@ def mouseMoveEvent(self, event: QMouseEvent): self.setCursor(Qt.SplitVCursor) if event.buttons() == Qt.LeftButton and self.selection_area.resizing: - if self.selection_area.selected_edge == 0: start = self.mapToScene(event.pos()) self.__set_selection_area(x=start.x(), y=start.y()) @@ -226,7 +246,11 @@ def mouseReleaseEvent(self, event: QMouseEvent): self.grab_start = None self.setCursor(Qt.OpenHandCursor) - elif self.separation_area_moving and hasattr(self, "signal") and self.signal is not None: + elif ( + self.separation_area_moving + and hasattr(self, "signal") + and self.signal is not None + ): y_sep = self.mapToScene(event.pos()).y() y = self.sceneRect().y() h = self.sceneRect().height() @@ -252,15 +276,23 @@ def refresh_selection_area(self): This happens e.g. when switching from Signal View to Quad Demod view :return: """ - self.__set_selection_area(x=self.selection_area.x, y=self.selection_area.y, - w=self.selection_area.width, h=self.selection_area.height) + self.__set_selection_area( + x=self.selection_area.x, + y=self.selection_area.y, + w=self.selection_area.width, + h=self.selection_area.height, + ) def set_vertical_selection(self, y=None, h=None): self.selection_area.setX(self.sceneRect().x()) self.selection_area.width = self.sceneRect().width() if y is not None: - y = util.clip(y, self.sceneRect().y(), self.sceneRect().y() + self.sceneRect().height()) + y = util.clip( + y, + self.sceneRect().y(), + self.sceneRect().y() + self.sceneRect().height(), + ) self.selection_area.setY(y) if h is not None: @@ -279,7 +311,9 @@ def set_horizontal_selection(self, x=None, w=None): self.selection_area.height = self.view_rect().height() if x is not None: - x = util.clip(x, self.sceneRect().x(), self.sceneRect().x() + self.sceneRect().width()) + x = util.clip( + x, self.sceneRect().x(), self.sceneRect().x() + self.sceneRect().width() + ) self.selection_area.setX(x) if w is not None: @@ -309,14 +343,18 @@ def emit_selection_size_changed(self): self.selection_height_changed.emit(int(self.selection_area.height)) def emit_selection_start_end_changed(self): - self.sel_area_start_end_changed.emit(self.selection_area.start, self.selection_area.end) + self.sel_area_start_end_changed.emit( + self.selection_area.start, self.selection_area.end + ) def view_rect(self) -> QRectF: """ Return the boundaries of the view in scene coordinates """ top_left = self.mapToScene(0, 0) - bottom_right = self.mapToScene(self.viewport().width() - 1, self.viewport().height() - 1) + bottom_right = self.mapToScene( + self.viewport().width() - 1, self.viewport().height() - 1 + ) return QRectF(top_left, bottom_right) def eliminate(self): diff --git a/src/urh/ui/views/SimulatorGraphicsView.py b/src/urh/ui/views/SimulatorGraphicsView.py index 5106a68531..49ba227898 100644 --- a/src/urh/ui/views/SimulatorGraphicsView.py +++ b/src/urh/ui/views/SimulatorGraphicsView.py @@ -2,7 +2,14 @@ from PyQt5.QtCore import Qt, pyqtSlot, pyqtSignal from PyQt5.QtGui import QKeySequence, QIcon -from PyQt5.QtWidgets import QGraphicsView, QAction, QActionGroup, QMenu, QAbstractItemView, QInputDialog +from PyQt5.QtWidgets import ( + QGraphicsView, + QAction, + QActionGroup, + QMenu, + QAbstractItemView, + QInputDialog, +) from urh.signalprocessing.MessageType import MessageType from urh.simulator.GraphicsItem import GraphicsItem @@ -40,7 +47,9 @@ def __init__(self, parent=None): self.delete_action.setShortcutContext(Qt.WidgetWithChildrenShortcut) self.addAction(self.select_all_action) - self.copy_action = QAction(self.tr("Copy selected items"), self) # type: QAction + self.copy_action = QAction( + self.tr("Copy selected items"), self + ) # type: QAction self.copy_action.setShortcut(QKeySequence.Copy) self.copy_action.triggered.connect(self.on_copy_action_triggered) self.copy_action.setShortcutContext(Qt.WidgetWithChildrenShortcut) @@ -59,9 +68,13 @@ def scene(self) -> SimulatorScene: @pyqtSlot() def on_add_message_action_triggered(self): - num_bits, ok = QInputDialog.getInt(self, - self.tr("How many bits shall the new message have?"), - self.tr("Number of bits:"), 42, 1) + num_bits, ok = QInputDialog.getInt( + self, + self.tr("How many bits shall the new message have?"), + self.tr("Number of bits:"), + 42, + 1, + ) if ok: self.add_empty_message(num_bits) @@ -77,44 +90,64 @@ def add_empty_message(self, num_bits): else: position = QAbstractItemView.BelowItem - message = self.scene().add_message(plain_bits=[0] * num_bits, - pause=0, - message_type=message_type, - ref_item=ref_item, - position=position) + message = self.scene().add_message( + plain_bits=[0] * num_bits, + pause=0, + message_type=message_type, + ref_item=ref_item, + position=position, + ) self.jump_to_item(message) @pyqtSlot() def on_add_rule_action_triggered(self): - rule = self.scene().add_rule(self.context_menu_item, QAbstractItemView.BelowItem) + rule = self.scene().add_rule( + self.context_menu_item, QAbstractItemView.BelowItem + ) if_cond = rule.children[0] self.jump_to_item(if_cond) @pyqtSlot() def on_add_goto_action_triggered(self): ref_item = self.context_menu_item - position = QAbstractItemView.OnItem if isinstance(ref_item, RuleConditionItem) else QAbstractItemView.BelowItem + position = ( + QAbstractItemView.OnItem + if isinstance(ref_item, RuleConditionItem) + else QAbstractItemView.BelowItem + ) ga = self.scene().add_goto_action(ref_item, position) self.jump_to_item(ga) @pyqtSlot() def on_add_sleep_action_triggered(self): ref_item = self.context_menu_item - position = QAbstractItemView.OnItem if isinstance(ref_item, RuleConditionItem) else QAbstractItemView.BelowItem + position = ( + QAbstractItemView.OnItem + if isinstance(ref_item, RuleConditionItem) + else QAbstractItemView.BelowItem + ) sa = self.scene().add_sleep_action(ref_item, position) self.jump_to_item(sa) @pyqtSlot() def on_add_counter_action_triggered(self): ref_item = self.context_menu_item - position = QAbstractItemView.OnItem if isinstance(ref_item, RuleConditionItem) else QAbstractItemView.BelowItem + position = ( + QAbstractItemView.OnItem + if isinstance(ref_item, RuleConditionItem) + else QAbstractItemView.BelowItem + ) ca = self.scene().add_counter_action(ref_item, position) self.jump_to_item(ca) @pyqtSlot() def on_trigger_command_action_triggered(self): ref_item = self.context_menu_item - position = QAbstractItemView.OnItem if isinstance(ref_item, RuleConditionItem) else QAbstractItemView.BelowItem + position = ( + QAbstractItemView.OnItem + if isinstance(ref_item, RuleConditionItem) + else QAbstractItemView.BelowItem + ) pa = self.scene().add_trigger_command_action(ref_item, position) self.jump_to_item(pa) @@ -180,7 +213,9 @@ def on_select_from_action_triggered(self): self.scene().select_messages_with_participant(self.sender().data()) def on_select_to_action_triggered(self): - self.scene().select_messages_with_participant(self.sender().data(), from_part=False) + self.scene().select_messages_with_participant( + self.sender().data(), from_part=False + ) def create_context_menu(self): menu = QMenu() @@ -206,17 +241,23 @@ def create_context_menu(self): add_counter_action = action_menu.addAction("Counter") add_counter_action.triggered.connect(self.on_add_counter_action_triggered) trigger_command_action = action_menu.addAction("Trigger command") - trigger_command_action.triggered.connect(self.on_trigger_command_action_triggered) + trigger_command_action.triggered.connect( + self.on_trigger_command_action_triggered + ) if isinstance(self.context_menu_item, RuleConditionItem): menu.addSeparator() add_else_if_cond_action = menu.addAction("Add else if block") - add_else_if_cond_action.triggered.connect(self.on_add_else_if_cond_action_triggered) + add_else_if_cond_action.triggered.connect( + self.on_add_else_if_cond_action_triggered + ) if not self.context_menu_item.parentItem().has_else_condition: add_else_cond_action = menu.addAction("Add else block") - add_else_cond_action.triggered.connect(self.on_add_else_cond_action_triggered) + add_else_cond_action.triggered.connect( + self.on_add_else_cond_action_triggered + ) menu.addSeparator() menu.addAction(self.copy_action) @@ -237,8 +278,12 @@ def create_context_menu(self): va.setActionGroup(value_type_group) va.setData(i) - if all(lbl.value_type_index == i for msg in messages for lbl in msg.message_type - if not lbl.is_checksum_label): + if all( + lbl.value_type_index == i + for msg in messages + for lbl in msg.message_type + if not lbl.is_checksum_label + ): va.setChecked(True) va.triggered.connect(self.on_set_value_type_action_triggered) @@ -285,24 +330,35 @@ def create_context_menu(self): swap_part_action.triggered.connect(self.on_swap_part_action_triggered) swap_part_action.setIcon(QIcon.fromTheme("object-flip-horizontal")) - pause_action = menu.addAction("Set subsequent pause ({} samples)".format(self.context_menu_item.model_item.pause)) + pause_action = menu.addAction( + "Set subsequent pause ({} samples)".format( + self.context_menu_item.model_item.pause + ) + ) pause_action.triggered.connect(self.on_pause_action_triggered) menu.addSeparator() if len(self.scene().get_all_message_items()) > 1: consolidate_messages_action = menu.addAction("Consolidate messages") - consolidate_messages_action.triggered.connect(self.on_consolidate_messages_action_triggered) + consolidate_messages_action.triggered.connect( + self.on_consolidate_messages_action_triggered + ) - if len([item for item in self.scene().items() if isinstance(item, GraphicsItem)]): + if len( + [item for item in self.scene().items() if isinstance(item, GraphicsItem)] + ): # menu.addAction(self.select_all_action) clear_all_action = menu.addAction("Clear all") clear_all_action.triggered.connect(self.on_clear_all_action_triggered) clear_all_action.setIcon(QIcon.fromTheme("edit-clear")) - self.add_select_actions_to_menu(menu, self.scene(), - select_to_trigger=self.on_select_to_action_triggered, - select_from_trigger=self.on_select_from_action_triggered) + self.add_select_actions_to_menu( + menu, + self.scene(), + select_to_trigger=self.on_select_to_action_triggered, + select_from_trigger=self.on_select_from_action_triggered, + ) return menu @@ -332,7 +388,11 @@ def jump_to_scene_item(self, item): item.setSelected(True) def contextMenuEvent(self, event): - items = [item for item in self.items(event.pos()) if isinstance(item, GraphicsItem) and item.is_selectable()] + items = [ + item + for item in self.items(event.pos()) + if isinstance(item, GraphicsItem) and item.is_selectable() + ] self.context_menu_item = None if len(items) == 0 else items[0] menu = self.create_context_menu() menu.exec_(event.globalPos()) @@ -349,15 +409,22 @@ def keyPressEvent(self, event): @pyqtSlot() def on_pause_action_triggered(self): - p = self.context_menu_item.model_item.pause if isinstance(self.context_menu_item, MessageItem) else 0 - pause, ok = QInputDialog.getInt(self, self.tr("Enter new pause"), - self.tr("Pause in samples:"), p, 0) + p = ( + self.context_menu_item.model_item.pause + if isinstance(self.context_menu_item, MessageItem) + else 0 + ) + pause, ok = QInputDialog.getInt( + self, self.tr("Enter new pause"), self.tr("Pause in samples:"), p, 0 + ) if ok: for msg in self.scene().get_selected_messages(): msg.pause = pause @classmethod - def add_select_actions_to_menu(cls, menu, scene: SimulatorScene, select_to_trigger, select_from_trigger): + def add_select_actions_to_menu( + cls, menu, scene: SimulatorScene, select_to_trigger, select_from_trigger + ): if len(scene.visible_participants) == 0: return @@ -387,5 +454,6 @@ def on_paste_action_triggered(self): assert isinstance(item, GraphicsItem) parent = item.model_item.parent() pos = parent.child_count() if parent is not None else 0 - self.scene().simulator_config.add_items([copy.deepcopy(item.model_item)], pos, parent) - + self.scene().simulator_config.add_items( + [copy.deepcopy(item.model_item)], pos, parent + ) diff --git a/src/urh/ui/views/SimulatorLabelTableView.py b/src/urh/ui/views/SimulatorLabelTableView.py index f49f08f428..bdf6d173d3 100644 --- a/src/urh/ui/views/SimulatorLabelTableView.py +++ b/src/urh/ui/views/SimulatorLabelTableView.py @@ -13,7 +13,6 @@ def __init__(self, parent=None): self.setMouseTracking(True) self.clicked.connect(self.on_clicked) - def model(self) -> SimulatorMessageFieldModel: return super().model() diff --git a/src/urh/ui/views/SimulatorMessageTableView.py b/src/urh/ui/views/SimulatorMessageTableView.py index bc2b8ab1fd..d389f65d6c 100644 --- a/src/urh/ui/views/SimulatorMessageTableView.py +++ b/src/urh/ui/views/SimulatorMessageTableView.py @@ -21,7 +21,9 @@ def __init__(self, parent=None): def _insert_column(self, pos): view_type = self.model().proto_view - index = self.model().protocol.convert_index(pos, from_view=view_type, to_view=0, decoded=False)[0] + index = self.model().protocol.convert_index( + pos, from_view=view_type, to_view=0, decoded=False + )[0] nbits = 1 if view_type == 0 else 4 if view_type == 1 else 8 for row in self.selected_rows: msg = self.model().protocol.messages[row] @@ -53,8 +55,10 @@ def create_context_menu(self) -> QMenu: selected_encoding = self.selected_message.decoder - if not all(self.model().protocol.messages[i].decoder is selected_encoding - for i in self.selected_rows): + if not all( + self.model().protocol.messages[i].decoder is selected_encoding + for i in self.selected_rows + ): selected_encoding = None encoding_group = QActionGroup(self) @@ -72,10 +76,14 @@ def create_context_menu(self) -> QMenu: ea.triggered.connect(self.on_encoding_action_triggered) if settings.read("multiple_modulations", False, bool): - selected_modulation = self.model().protocol.messages[self.selected_rows[0]].modulator_index - - if not all(self.model().protocol.messages[i].modulator_index == selected_modulation - for i in self.selected_rows): + selected_modulation = ( + self.model().protocol.messages[self.selected_rows[0]].modulator_index + ) + + if not all( + self.model().protocol.messages[i].modulator_index == selected_modulation + for i in self.selected_rows + ): selected_modulation = -1 modulation_group = QActionGroup(self) @@ -93,7 +101,9 @@ def create_context_menu(self) -> QMenu: ma.triggered.connect(self.on_modulation_action_triggered) open_modulator_dialog_action = modulation_menu.addAction(self.tr("...")) - open_modulator_dialog_action.triggered.connect(self.on_open_modulator_dialog_action_triggered) + open_modulator_dialog_action.triggered.connect( + self.on_open_modulator_dialog_action_triggered + ) return menu diff --git a/src/urh/ui/views/SpectrogramGraphicView.py b/src/urh/ui/views/SpectrogramGraphicView.py index 387a197dcb..e10746ca0b 100644 --- a/src/urh/ui/views/SpectrogramGraphicView.py +++ b/src/urh/ui/views/SpectrogramGraphicView.py @@ -21,7 +21,9 @@ def __init__(self, parent=None): super().__init__(parent) self.move_y_with_drag = True - self.scene_manager = SpectrogramSceneManager(np.zeros(1, dtype=np.complex64), parent=self) + self.scene_manager = SpectrogramSceneManager( + np.zeros(1, dtype=np.complex64), parent=self + ) self.setScene(self.scene_manager.scene) @property @@ -54,16 +56,22 @@ def create_context_menu(self): filter_bw = Filter.read_configured_filter_bw() text = self.tr("Apply bandpass filter (filter bw={0:n})".format(filter_bw)) create_from_frequency_selection = menu.addAction(text) - create_from_frequency_selection.triggered.connect(self.on_create_from_frequency_selection_triggered) + create_from_frequency_selection.triggered.connect( + self.on_create_from_frequency_selection_triggered + ) create_from_frequency_selection.setIcon(QIcon.fromTheme("view-filter")) try: - cancel_button = " or ".join(k.toString() for k in QKeySequence.keyBindings(QKeySequence.Cancel)) + cancel_button = " or ".join( + k.toString() for k in QKeySequence.keyBindings(QKeySequence.Cancel) + ) except Exception as e: logger.debug("Error reading cancel button: " + str(e)) cancel_button = "Esc" - create_from_frequency_selection.setToolTip("You can abort filtering with {}.".format(cancel_button)) + create_from_frequency_selection.setToolTip( + "You can abort filtering with {}.".format(cancel_button) + ) configure_filter_bw = menu.addAction(self.tr("Configure filter bandwidth...")) configure_filter_bw.triggered.connect(self.on_configure_filter_bw_triggered) @@ -91,7 +99,9 @@ def auto_fit_view(self): def emit_selection_start_end_changed(self): h = self.sceneRect().height() - self.sel_area_start_end_changed.emit(h - self.selection_area.end, h - self.selection_area.start) + self.sel_area_start_end_changed.emit( + h - self.selection_area.end, h - self.selection_area.start + ) @pyqtSlot() def on_create_from_frequency_selection_triggered(self): @@ -110,7 +120,7 @@ def on_configure_filter_bw_triggered(self): @pyqtSlot() def on_export_fta_action_triggered(self): - if not(self.scene_manager and self.scene_manager.spectrogram): + if not (self.scene_manager and self.scene_manager.spectrogram): return self.export_fta_wanted.emit() diff --git a/src/urh/ui/views/TableView.py b/src/urh/ui/views/TableView.py index 00e8b44eea..acc99d95ff 100644 --- a/src/urh/ui/views/TableView.py +++ b/src/urh/ui/views/TableView.py @@ -21,8 +21,10 @@ def __init__(self, parent=None): self.use_header_colors = False self.original_font_size = self.font().pointSize() - self.original_header_font_sizes = {"vertical": self.verticalHeader().font().pointSize(), - "horizontal": self.horizontalHeader().font().pointSize()} + self.original_header_font_sizes = { + "vertical": self.verticalHeader().font().pointSize(), + "horizontal": self.horizontalHeader().font().pointSize(), + } self.zoom_in_action = QAction(self.tr("Zoom in"), self) self.zoom_in_action.setShortcut(QKeySequence.ZoomIn) @@ -40,7 +42,9 @@ def __init__(self, parent=None): self.zoom_original_action = QAction(self.tr("Zoom original"), self) self.zoom_original_action.setShortcut(QKeySequence(Qt.CTRL + Qt.Key_0)) - self.zoom_original_action.triggered.connect(self.on_zoom_original_action_triggered) + self.zoom_original_action.triggered.connect( + self.on_zoom_original_action_triggered + ) self.zoom_original_action.setShortcutContext(Qt.WidgetWithChildrenShortcut) self.zoom_original_action.setIcon(QIcon.fromTheme("zoom-original")) self.addAction(self.zoom_original_action) @@ -51,11 +55,19 @@ def _add_insert_column_menu(self, menu): column_menu = menu.addMenu("Insert column") insert_column_left_action = column_menu.addAction("on the left") - insert_column_left_action.triggered.connect(self.on_insert_column_left_action_triggered) - insert_column_left_action.setIcon(QIcon.fromTheme("edit-table-insert-column-left")) + insert_column_left_action.triggered.connect( + self.on_insert_column_left_action_triggered + ) + insert_column_left_action.setIcon( + QIcon.fromTheme("edit-table-insert-column-left") + ) insert_column_right_action = column_menu.addAction("on the right") - insert_column_right_action.setIcon(QIcon.fromTheme("edit-table-insert-column-right")) - insert_column_right_action.triggered.connect(self.on_insert_column_right_action_triggered) + insert_column_right_action.setIcon( + QIcon.fromTheme("edit-table-insert-column-right") + ) + insert_column_right_action.triggered.connect( + self.on_insert_column_right_action_triggered + ) def selectionModel(self) -> QItemSelectionModel: return super().selectionModel() @@ -126,8 +138,10 @@ def create_context_menu(self) -> QMenu: if self.context_menu_pos is None: return menu - selected_label_index = self.model().get_selected_label_index(row=self.rowAt(self.context_menu_pos.y()), - column=self.columnAt(self.context_menu_pos.x())) + selected_label_index = self.model().get_selected_label_index( + row=self.rowAt(self.context_menu_pos.y()), + column=self.columnAt(self.context_menu_pos.x()), + ) if self.model().row_count > 0: if selected_label_index == -1: @@ -137,7 +151,9 @@ def create_context_menu(self) -> QMenu: label_action = menu.addAction("Edit label...") label_action.setIcon(QIcon.fromTheme("configure")) - label_action.triggered.connect(self.on_create_or_edit_label_action_triggered) + label_action.triggered.connect( + self.on_create_or_edit_label_action_triggered + ) menu.addSeparator() zoom_menu = menu.addMenu("Zoom font size") @@ -180,7 +196,9 @@ def resize_vertical_header(self): num_rows = self.model().rowCount() if self.isVisible() and num_rows > 0: hd = self.model().headerData - max_len = np.max([len(str(hd(i, Qt.Vertical, Qt.DisplayRole))) for i in range(num_rows)]) + max_len = np.max( + [len(str(hd(i, Qt.Vertical, Qt.DisplayRole))) for i in range(num_rows)] + ) w = (self.font().pointSize() + 2) * max_len # https://github.com/jopohl/urh/issues/182 @@ -216,8 +234,10 @@ def keyPressEvent(self, event: QKeyEvent): self.model().insert_column(start, list(range(min_row, max_row + 1))) - if event.key() not in (Qt.Key_Right, Qt.Key_Left, Qt.Key_Up, Qt.Key_Down) \ - or event.modifiers() == Qt.ShiftModifier: + if ( + event.key() not in (Qt.Key_Right, Qt.Key_Left, Qt.Key_Up, Qt.Key_Down) + or event.modifiers() == Qt.ShiftModifier + ): super().keyPressEvent(event) return @@ -314,8 +334,10 @@ def on_insert_column_right_action_triggered(self): @pyqtSlot() def on_create_or_edit_label_action_triggered(self): - selected_label_index = self.model().get_selected_label_index(row=self.rowAt(self.context_menu_pos.y()), - column=self.columnAt(self.context_menu_pos.x())) + selected_label_index = self.model().get_selected_label_index( + row=self.rowAt(self.context_menu_pos.y()), + column=self.columnAt(self.context_menu_pos.x()), + ) if selected_label_index == -1: min_row, max_row, start, end = self.selection_range() self.create_label_triggered.emit(min_row, start, end) diff --git a/src/urh/ui/views/TextEditProtocolView.py b/src/urh/ui/views/TextEditProtocolView.py index 0afb55d909..4d92b9f1eb 100644 --- a/src/urh/ui/views/TextEditProtocolView.py +++ b/src/urh/ui/views/TextEditProtocolView.py @@ -17,7 +17,7 @@ def __init__(self, parent=None): @property def selected_text(self): - return self.textCursor().selectedText().replace('\u2028', '\n') + return self.textCursor().selectedText().replace("\u2028", "\n") def keyPressEvent(self, event: QKeyEvent): if event.key() == Qt.Key_Delete: @@ -98,8 +98,8 @@ def create_context_menu(self) -> QMenu: cursor = self.textCursor() if self.participants and self.messages and not cursor.selection().isEmpty(): self.selected_messages = [] - start_msg = self.toPlainText()[0:cursor.selectionStart()].count("\n") - end_msg = self.toPlainText()[0:cursor.selectionEnd()].count("\n") + 1 + start_msg = self.toPlainText()[0 : cursor.selectionStart()].count("\n") + end_msg = self.toPlainText()[0 : cursor.selectionEnd()].count("\n") + 1 for i in range(start_msg, end_msg): self.selected_messages.append(self.messages[i]) @@ -113,13 +113,17 @@ def create_context_menu(self) -> QMenu: none_participant_action = participant_menu.addAction("None") none_participant_action.setCheckable(True) none_participant_action.setActionGroup(participant_group) - none_participant_action.triggered.connect(self.on_none_participant_action_triggered) + none_participant_action.triggered.connect( + self.on_none_participant_action_triggered + ) if selected_msg and selected_msg.participant is None: none_participant_action.setChecked(True) for participant in self.participants: - pa = participant_menu.addAction(participant.name + " (" + participant.shortname + ")") + pa = participant_menu.addAction( + participant.name + " (" + participant.shortname + ")" + ) pa.setCheckable(True) pa.setActionGroup(participant_group) if selected_msg and selected_msg.participant == participant: @@ -134,7 +138,9 @@ def create_context_menu(self) -> QMenu: menu.addSeparator() - line_wrap_action = menu.addAction("Linewrap (may take a while for long protocols)") + line_wrap_action = menu.addAction( + "Linewrap (may take a while for long protocols)" + ) line_wrap_action.setCheckable(True) line_wrap = self.lineWrapMode() == QTextEdit.WidgetWidth line_wrap_action.setChecked(line_wrap) diff --git a/src/urh/ui/views/ZoomAndDropableGraphicView.py b/src/urh/ui/views/ZoomAndDropableGraphicView.py index 49aede97df..c6ae1ba072 100644 --- a/src/urh/ui/views/ZoomAndDropableGraphicView.py +++ b/src/urh/ui/views/ZoomAndDropableGraphicView.py @@ -65,9 +65,11 @@ def auto_fit_view(self): plot_min, plot_max = util.minmax(self.signal.real_plot_data) data_min, data_max = IQArray.min_max_for_dtype(self.signal.real_plot_data.dtype) - self.scale(1, (data_max - data_min) / (plot_max-plot_min)) + self.scale(1, (data_max - data_min) / (plot_max - plot_min)) - self.centerOn(self.view_rect().x() + self.view_rect().width() / 2, self.y_center) + self.centerOn( + self.view_rect().x() + self.view_rect().width() / 2, self.y_center + ) def eliminate(self): # Do _not_ call eliminate() for self.signal and self.proto_analyzer diff --git a/src/urh/ui/views/ZoomableGraphicView.py b/src/urh/ui/views/ZoomableGraphicView.py index 600819e715..d18a2ac4c6 100644 --- a/src/urh/ui/views/ZoomableGraphicView.py +++ b/src/urh/ui/views/ZoomableGraphicView.py @@ -38,7 +38,9 @@ def __init__(self, parent=None): self.zoom_original_action = QAction(self.tr("Zoom original"), self) self.zoom_original_action.setShortcut(QKeySequence(Qt.CTRL + Qt.Key_0)) - self.zoom_original_action.triggered.connect(self.on_zoom_original_action_triggered) + self.zoom_original_action.triggered.connect( + self.on_zoom_original_action_triggered + ) self.zoom_original_action.setShortcutContext(Qt.WidgetWithChildrenShortcut) self.zoom_original_action.setIcon(QIcon.fromTheme("zoom-original")) self.addAction(self.zoom_original_action) @@ -60,7 +62,9 @@ def y_center(self): else: return -self.signal.center except Exception as e: - logger.error("Could not access y_center property: {0}. Falling back to 0".format(e)) + logger.error( + "Could not access y_center property: {0}. Falling back to 0".format(e) + ) return 0 def create_context_menu(self): @@ -97,7 +101,9 @@ def zoom(self, factor, zoom_to_mouse_cursor=True, cursor_pos=None): factor = self.view_rect().width() / self.MINIMUM_VIEW_WIDTH if zoom_to_mouse_cursor: - pos = self.mapFromGlobal(QCursor.pos()) if cursor_pos is None else cursor_pos + pos = ( + self.mapFromGlobal(QCursor.pos()) if cursor_pos is None else cursor_pos + ) else: pos = None old_pos = self.mapToScene(pos) if pos is not None else None @@ -134,14 +140,19 @@ def auto_fit_view(self): if abs(h_tar) > 0: self.scale(1, h_view / h_tar) - self.centerOn(self.view_rect().x() + self.view_rect().width() / 2, self.y_center) + self.centerOn( + self.view_rect().x() + self.view_rect().width() / 2, self.y_center + ) def show_full_scene(self, reinitialize=False): y_factor = self.transform().m22() self.resetTransform() # Use full self.width() here to enable show_full_scene when view_rect not yet set e.g. in Record Signal Dialog - x_factor = self.width() / ( - self.sceneRect().width() * self.scene_x_zoom_stretch) if self.sceneRect().width() else 1 + x_factor = ( + self.width() / (self.sceneRect().width() * self.scene_x_zoom_stretch) + if self.sceneRect().width() + else 1 + ) self.scale(x_factor, y_factor) self.centerOn(0, self.y_center) @@ -172,7 +183,9 @@ def redraw_view(self, reinitialize=False): vr = self.view_rect() start, end = vr.x(), vr.x() + vr.width() - self.scene_manager.show_scene_section(start, end, *self._get_sub_path_ranges_and_colors(start, end)) + self.scene_manager.show_scene_section( + start, end, *self._get_sub_path_ranges_and_colors(start, end) + ) if self.scene_type == 1: self.scene().redraw_legend() diff --git a/src/urh/ui/views/__init__.py b/src/urh/ui/views/__init__.py index 3fa5af38d8..813939aaf7 100644 --- a/src/urh/ui/views/__init__.py +++ b/src/urh/ui/views/__init__.py @@ -1 +1 @@ -__author__ = 'joe' +__author__ = "joe" diff --git a/src/urh/ui/xtra_icons_rc.py b/src/urh/ui/xtra_icons_rc.py index 1d893be3c9..d13b052843 100644 --- a/src/urh/ui/xtra_icons_rc.py +++ b/src/urh/ui/xtra_icons_rc.py @@ -60374,18 +60374,25 @@ \x00\x00\x01\x63\xee\xee\x7d\xe3\ " -qt_version = QtCore.qVersion().split('.') -if qt_version < ['5', '8', '0']: +qt_version = QtCore.qVersion().split(".") +if qt_version < ["5", "8", "0"]: rcc_version = 1 qt_resource_struct = qt_resource_struct_v1 else: rcc_version = 2 qt_resource_struct = qt_resource_struct_v2 + def qInitResources(): - QtCore.qRegisterResourceData(rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data) + QtCore.qRegisterResourceData( + rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data + ) + def qCleanupResources(): - QtCore.qUnregisterResourceData(rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data) + QtCore.qUnregisterResourceData( + rcc_version, qt_resource_struct, qt_resource_name, qt_resource_data + ) + qInitResources() diff --git a/src/urh/util/Errors.py b/src/urh/util/Errors.py index d0bab9dc89..34d82a4c88 100644 --- a/src/urh/util/Errors.py +++ b/src/urh/util/Errors.py @@ -12,9 +12,13 @@ class Errors: def generic_error(title: str, msg: str, detailed_msg: str = None): w = QWidget() if detailed_msg: - msg = "Error: " + msg.replace("\n", - "{1} |
{1} |
Expected: | {1} |
Got: | {2} |
Expected: | {1} |
Got: | {2} |