diff --git a/README.md b/README.md
index 7c787c3..0e63f1c 100644
--- a/README.md
+++ b/README.md
@@ -1,51 +1,29 @@
-`#php` `#basics` `#master-in-software-development`
+# PHP-basics :technologist:
+_The goal of this project is to tough the PHP at the first time and to see the basics concept of this programming language. There are eleven files that are separated according to the topics._
-# PHP Basics
+## Resources :pushpin:
+- XAMPP
+- VisualStudio Code
+- PHP v.8.1
+- GIT
-
-
-
+## How to see the code? :globe_with_meridians:
+1. Open the XAMPP folder
+2. Find "htdocs"
+3. Inside of "htdocs" create a new folder where you will store your files
+4. In my case it is "PHPBasicsWorkShop"
+5. Open XAMPP and "start" Apache
+6. Open **localhost** in your navegater and after slash-simbol put the name of your folder from the previous step
+7. If everything is ok, you will see the next page=>
-> In this project you will learn the basic notions of the famous PHP language which is so used in the world of web development.
->
-> What distinguishes PHP from other languages such as Javascript is that the code is executed on the server, generating HTML and sending it to the client.
+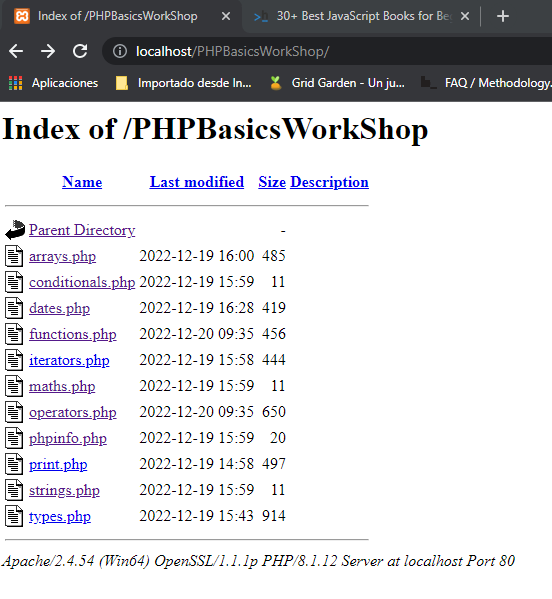
-## Index
+## Sources of information :bulb:
-- [Requirements](#requirements)
-- [Repository](#repository)
-- [Technologies used](#technologies-used)
-- [Project delivery](#project-delivery)
-- [Resources](#resources)
+PHP official [website](https://www.php.net/)
-## Requirements
+Youtube content: PHP classes, XAMPP, web servers
-- Learn the basics to program in PHP
-- Understand what a server-side language is and what it is used for
-
-## Repository
-
-First of all you must fork this project into your GitHub account.
-
-To create a fork on GitHub is as easy as clicking the “fork” button on the repository page.
-
-
-
-## Technologies used
-
-\* PHP
-
-## Project delivery
-
-To deliver this project you must send a Pull Request as explained in the Students Handbook. Remember that the PR title must be with the format
-- Solution: + NAME AND SURNAME or TEAM NAMES AND SURNAMES.
-- For example: "Solution: Josep Riera", "Solution: Josep Riera, Toni Suárez, Marta Vázquez"
-
-## Resources
-
-- [What can PHP do?](https://www.php.net/manual/es/intro-whatcando.php)
-- [Sample guide for README](https://gist.github.com/Villanuevand/6386899f70346d4580c723232524d35a)
-- [XAMPP](https://www.apachefriends.org/es/index.html)
-- [How to install XAMPP on Windows](https://www.youtube.com/watch?v=h6DEDm7C37A)
-- [What is a web server?](https://www.youtube.com/watch?v=Yt1nesKi5Ec)
-- [Web server basics](https://www.youtube.com/watch?v=3VqfpVKvlxQ)
+### Author :pencil2:
+This is a study project made for [Assembler Institute of Technology](https://assemblerinstitute.com/)
+ by student [**Iuliia Shikhanova**](https://github.com/IuliiaNova)
diff --git a/arrays.php b/arrays.php
new file mode 100644
index 0000000..4f9a7c5
--- /dev/null
+++ b/arrays.php
@@ -0,0 +1,31 @@
+
";
+ #obtain the length of an array
+ var_dump(count($cars));
+
+ #combination of two arrays
+
+ $result = array_combine($nombres, $numbers);
+
+ print_r($result);
+ echo "
";
+
+ #Execute the function that once is given an array return the last element of it
+ echo end($nombres);
+ echo "
";
+
+#Execute the function that once is given an array add a new element to the array in question
+
+ array_push($nombres, "Olga", "Katy");
+ print_r($nombres);
+?>
diff --git a/conditionals.php b/conditionals.php
new file mode 100644
index 0000000..291dda4
--- /dev/null
+++ b/conditionals.php
@@ -0,0 +1,47 @@
+
";
+}else echo "We are not on Monday
";
+
+if($month == "October"){
+ echo "We are in October
";
+}else echo "We are in " . date("F") . "
";
+
+// Doble condition
+
+$minute = 9;
+if($minute < 10){
+ echo "the current minute is less than 10
";
+}elseif($minute>15){
+ echo "the current minute is more than 15";
+}else echo "does not meet any conditions";
+
+// Switch
+$today = "friday";
+
+switch($today){
+ case 'Monday':
+ echo "Let start the week
";
+ break;
+
+ case 'Wednesday':
+ echo "The are just three days more to finish the week!
";
+ break;
+
+ case 'friday':
+ echo "The last working day
";
+ break;
+
+ case 'Sunday':
+ echo "Tomorrow we will start again
";
+ break;
+
+ default: echo "nothing to do";
+}
+
+
+?>
\ No newline at end of file
diff --git a/dates.php b/dates.php
new file mode 100644
index 0000000..c79e7e5
--- /dev/null
+++ b/dates.php
@@ -0,0 +1,26 @@
+setDate(2022, 12, 20)
+ ->setTime(12, 00)
+ ->setTimezone(new DateTimeZone('America/New_York'))
+ ->format("Y-m-d\\TH:i:sP");
+echo "
";
+
+// date
+echo "Today is " . date("Y-m-d") . "
";
+
+// day
+echo "Today is " . date("l") . "
";
+
+//month in numerical format (1-12)
+echo "Today is " . date("m") . "
";
+
+//current minute with leading zeros (00 - 59)
+echo "Now is " . date("i") . "
";
+
+?>
+
+
+
+
diff --git a/functions.php b/functions.php
new file mode 100644
index 0000000..1402a45
--- /dev/null
+++ b/functions.php
@@ -0,0 +1,37 @@
+
";
+
+function multOfBoth(int $a, int $b){
+ return $a * $b;
+}
+echo multOfBoth(10,7);
+echo "
";
+
+function divOfBoth(int $a, int $b){
+ return $a / $b;
+}
+echo divOfBoth(70,7);
+echo "
";
+
+
+function all(int $a,int $b, int $c, int $d){
+ return $a + $b * $c / $d;
+}
+echo all(70, 7, 8, 6);
+echo "
";
+
+
+$x=100;
+$y=60;
+echo "The sum of x and y is : ". ($x+$y) ."
";
+echo "The difference between x and y is : ". ($x-$y) ."
";
+echo "Multiplication of x and y : ". ($x*$y) ."
";
+echo "Division of x and y : ". ($x/$y) ."
";
+echo "Modulus of x and y : " . ($x%$y) ."
";
+?>
+
diff --git a/iterators.php b/iterators.php
new file mode 100644
index 0000000..2df19ed
--- /dev/null
+++ b/iterators.php
@@ -0,0 +1,36 @@
+
";
+
+// For
+
+$i = 0;
+for ($i=0; $i < 10; $i++){
+ echo $i;
+ }
+ echo "
";
+
+// While
+$y = 0;
+while ($y < 10){
+ $y++;
+ echo "While: $y";
+}
+echo "
";
+
+// Do-while que imprime 012345678910
+$x = 0;
+do{
+ $x++;
+ echo "Do-While: $x";
+ } while ($x<10);
+
+?>
\ No newline at end of file
diff --git a/maths.php b/maths.php
new file mode 100644
index 0000000..3bb2c8f
--- /dev/null
+++ b/maths.php
@@ -0,0 +1,39 @@
+
";
+var_dump(abs(5));
+echo"
";
+var_dump(abs(-5));
+echo"
";
+
+//Define a variable whose value is the result of the function that returns a rounded value to the next highest integer.
+
+echo ceil(4.3); // 5
+echo"
";
+echo ceil(9.999); // 10
+echo"
";
+echo ceil(-3.14);
+echo"
";
+
+//Define a variable whose value is the result of the function that returns the highest value of a series of values that are received by parameter.
+echo max(2, 3, 1, 6, 7);
+echo"
";
+echo max(array(2, 4, 5));
+echo"
";
+
+//Define a variable whose value is the result of the function that returns the lowest value of a series of values that are received by parameter.
+echo min(2, 3, 1, 6, 7);
+echo"
";
+echo min(array(2, 4, 5));
+echo"
";
+
+//Define a variable whose value is the result of the function that returns a random number
+echo rand() . "\n";
+echo rand() . "\n";
+echo rand(5, 15);
+echo"
";
+
+?>
+
diff --git a/operators.php b/operators.php
new file mode 100644
index 0000000..7a93050
--- /dev/null
+++ b/operators.php
@@ -0,0 +1,56 @@
+
";
+var_dump(1 - 1);
+echo "
";
+var_dump(1 * 2);
+echo "
";
+var_dump(10 / 5);
+echo "
";
+var_dump(10 % 1);
+echo "
";
+
+// Create a usage example for comparison operators: ==,! =, <,>, <=,> =
+var_dump(1 == TRUE);
+var_dump(0 != FALSE);
+var_dump(10 > 9);
+var_dump(7 < 8);
+echo "
";
+$hi = (object) ["hi" => "hi"];
+$biy = (object) ["biy" => "biy"];
+echo $hi <=> $biy;
+echo "
";
+
+// Create an example of use for logical operators: &&, And; ||, Or; ! (NOT); Xor
+
+$o = true && false;
+var_dump($o);
+$y = false && true;
+var_dump($y);
+$z = true && true;
+var_dump($z);
+$r = false && false;
+var_dump($r);
+$q = true || false;
+var_dump($q);
+$l = false || true;
+var_dump($l);
+$d = true || true;
+var_dump($d);
+$s = false || false;
+var_dump($s);
+echo "
";
+
+
+$var='10am';
+if($var !='12pm'){
+echo 'Get To Work';
+}else{
+echo 'Break Time!';
+}
+?>
+
+
+
diff --git a/phpinfo.php b/phpinfo.php
new file mode 100644
index 0000000..5299cc6
--- /dev/null
+++ b/phpinfo.php
@@ -0,0 +1,3 @@
+
\ No newline at end of file
diff --git a/print.php b/print.php
new file mode 100644
index 0000000..e9f2eee
--- /dev/null
+++ b/print.php
@@ -0,0 +1,22 @@
+ Mi codigo PHP - print ";
+
+//Echo permite imprimir varios resultadoe
+
+echo " Mi primer codigo PHP - echo
", " Mi segundo codigo PHP - echo
";
+
+// imprimen los detalles de una variable
+
+$letKnow = array( 5, 0.0, "Hola", false, '' );
+print_r($letKnow);
+echo "
";
+
+// imprimen los detalles de una variable con el typo de datos
+
+var_dump($letKnow);
+
+?>
+
diff --git a/strings.php b/strings.php
new file mode 100644
index 0000000..3d9d8bf
--- /dev/null
+++ b/strings.php
@@ -0,0 +1,67 @@
+
';
+
+$name = 'Iuliia';
+
+echo "My name is $name";
+echo "
";
+
+// Concatenate a previously declared variable in a text string
+
+$a = "Hello ";
+$b = $a . "World!"; // now $b contains "Hello World!"
+$a .= "World!";
+echo "$a";
+echo "
";
+
+
+//Execute the function that allows you to replace text in a string
+
+// Provides: You should eat pizza, beer, and ice cream every day
+$phrase = "You should eat fruits, vegetables, and fiber every day.";
+$healthy = array("fruits", "vegetables", "fiber");
+$yummy = array("pizza", "beer", "ice cream");
+
+$newphrase = str_replace($healthy, $yummy, $phrase);
+
+echo "$newphrase";
+echo "
";
+
+
+//Execute the function that allows you to write a text N times
+
+echo str_repeat("Say Hi ", 5);
+echo "
";
+
+//Execute the function that allows to obtain the length of a text string
+$howLong = 'Hola Iuliia';
+echo strlen($howLong);
+echo "
";
+
+//Executes the function that allows to obtain the position of the first occurrence of a text within a text string
+
+echo strpos("I love php, I love php too!","love");
+echo "
";
+
+//Execute the function that allows a text string to be capitalized
+$hola = "Mary Had A Little Lamb and She LOVED It So";
+$hola = strtoupper($hola);
+echo $hola;
+echo "
";
+
+// Execute the function that allows you to transform a text string to lowercase
+
+$adios = "Mary Had A Little Lamb and She LOVED It So";
+$adios = strtolower($adios);
+echo $adios;
+echo "
";
+
+// Execute the function that allows to obtain a text substring from a given position
+$rest = substr("abcdef", -1); // returns "f"
+$rest = substr("abcdef", 2);
+echo "$rest";
+
+echo "
";
+
+?>
\ No newline at end of file
diff --git a/types.php b/types.php
new file mode 100644
index 0000000..82f504b
--- /dev/null
+++ b/types.php
@@ -0,0 +1,61 @@
+
";
+
+#Integer
+
+$numero = 25;
+echo "Esto es variable integer: $numero";
+echo "
";
+
+#Float
+
+$numeroFloat = 25.67;
+echo "Esto es variable float: $numeroFloat";
+echo "
";
+
+
+#NULL
+
+$myNull = null;
+echo "Esto es variable NULL: $myNull";
+echo "
";
+
+
+#Variavle boolean
+$boleanaOne = true;
+echo "Esto es boleana true: $boleanaOne";
+echo "
";
+
+$boleanaTwo = false;
+echo "Esto es boleana false: $boleanaTwo";
+echo "
";
+
+#Array
+
+$colores = array("red", "blue", "green");
+echo "Esto es array: $colores[0]";
+echo "
";
+print_r ($colores);
+echo "
";
+
+#Objeto
+
+$frutas = (object)["fruta1"=>"pera", "fruta2"=>"platano", "fruta3"=>"manzana"];
+echo "Esto es un variable-objeto: $frutas->fruta2";
+echo "
";
+
+?>
+
+
+
+
+
+
+
+
+