parser = [
+ const HTMLTextNodeParser(),
+ const HTMLBulletedListNodeParser(),
+ const HTMLNumberedListNodeParser(),
+ const HTMLTodoListNodeParser(),
+ const HTMLQuoteNodeParser(),
+ const HTMLHeadingNodeParser(),
+ const HTMLImageNodeParser(),
+ ];
+ group('html_text_node_parser.dart', () {
+ const text = 'Welcome to AppFlowy';
+
+ test('heading style', () {
+ for (var i = 1; i <= 6; i++) {
+ final node = headingNode(
+ level: i,
+ attributes: {
+ 'delta': (Delta()..insert(text)).toJson(),
+ },
+ );
+ expect(
+ const HTMLHeadingNodeParser()
+ .transformNodeToHTMLString(node, encodeParsers: parser),
+ 'Welcome to AppFlowy',
+ );
+ }
+ });
+
+ test('bulleted list style', () {
+ final node = bulletedListNode(
+ attributes: {
+ 'delta': (Delta()..insert(text)).toJson(),
+ },
+ );
+ expect(
+ const HTMLBulletedListNodeParser()
+ .transformNodeToHTMLString(node, encodeParsers: parser),
+ '',
+ );
+ });
+
+ test('numbered list style', () {
+ final node = numberedListNode(
+ attributes: {
+ 'delta': (Delta()..insert(text)).toJson(),
+ },
+ );
+ expect(
+ const HTMLNumberedListNodeParser()
+ .transformNodeToHTMLString(node, encodeParsers: parser),
+ '- Welcome to AppFlowy
',
+ );
+ });
+
+ test('todo list style', () {
+ final checkedNode = todoListNode(
+ checked: true,
+ attributes: {
+ 'delta': (Delta()..insert(text)).toJson(),
+ },
+ );
+ final uncheckedNode = todoListNode(
+ checked: false,
+ attributes: {
+ 'delta': (Delta()..insert(text)).toJson(),
+ },
+ );
+ expect(
+ const HTMLTodoListNodeParser()
+ .transformNodeToHTMLString(checkedNode, encodeParsers: parser),
+ 'Welcome to AppFlowy
',
+ );
+ expect(
+ const HTMLTodoListNodeParser()
+ .transformNodeToHTMLString(uncheckedNode, encodeParsers: parser),
+ 'Welcome to AppFlowy
',
+ );
+ });
+
+ test('quote style', () {
+ final node = quoteNode(
+ attributes: {
+ 'delta': (Delta()..insert(text)).toJson(),
+ },
+ );
+ expect(
+ const HTMLQuoteNodeParser()
+ .transformNodeToHTMLString(node, encodeParsers: parser),
+ 'Welcome to AppFlowy
',
+ );
+ });
+
+ test('fallback', () {
+ final node = paragraphNode(
+ attributes: {
+ 'delta': (Delta()
+ ..insert(
+ text,
+ attributes: {
+ 'bold': true,
+ },
+ ))
+ .toJson(),
+ },
+ );
+ expect(
+ const HTMLTextNodeParser()
+ .transformNodeToHTMLString(node, encodeParsers: parser),
+ "Welcome to AppFlowy
",
+ );
+ });
+ });
+}
diff --git a/test/plugins/html/html_document_test.dart b/test/plugins/html/html_document_test.dart
index 6a2de5608..10c4de99b 100644
--- a/test/plugins/html/html_document_test.dart
+++ b/test/plugins/html/html_document_test.dart
@@ -1,5 +1,5 @@
-import 'package:flutter_test/flutter_test.dart';
import 'package:appflowy_editor/appflowy_editor.dart';
+import 'package:flutter_test/flutter_test.dart';
void main() {
group('html_document_test.dart tests', () {
@@ -8,20 +8,20 @@ void main() {
expect(document.toJson(), data);
});
test('nestedhtmlToDocument()', () {
- final document = htmlToDocument(nestedhtml);
+ final document = htmlToDocument(nestedHTML);
expect(document.toJson(), nestedDelta);
});
});
group('document_html_test.dart tests', () {
- test('documentToHtml()', () {
+ test('documentToHTML()', () {
final document = documentToHTML(Document.fromJson(data));
expect(document, rawHTML);
});
- test('nesteddocumentToHtml()', () {
+ test('nesteddocumentToHTML()', () {
final document = documentToHTML(Document.fromJson(nestedDelta));
- expect(document, nestedhtml);
+ expect(document, nestedHTML);
});
});
}
@@ -297,10 +297,10 @@ const data = {
}
};
const rawHTML =
- '''AppFlowyEditor
👋 Welcome to AppFlowy Editor
AppFlowy Editor is a highly customizable rich-text editor
Here is an example your you can give a try
Span element
Span element two
Span element three
This is an anchor tag!
Features!
- [x] Customizable
- [x] Test-covered
- [ ] more to come!
- First item
- Second item
- List element
This is a quote!
Code block
Italic one
Italic two
Bold tag
You can also use AppFlowy Editor as a component to build your own app.
Awesome features
If you have questions or feedback, please submit an issue on Github or join the community along with 1000+ builders!
''';
+ '''AppFlowyEditor
👋 Welcome to AppFlowy Editor
AppFlowy Editor is a highly customizable rich-text editor
Here is an example your you can give a try
Span element
Span element two
Span element three
This is an anchor tag!
Features!
This is a quote!
Code block
Italic one
Italic two
Bold tag
You can also use AppFlowy Editor as a component to build your own app.
Awesome features
If you have questions or feedback, please submit an issue on Github or join the community along with 1000+ builders!
''';
-const nestedhtml =
- '''Welcome to the playground
In case you were wondering what the black box at the bottom is – it's the debug view, showing the current state of the editor. You can disable it by pressing on the settings control in the bottom-left of your screen and toggling the debug view setting. The playground is a demo environment built with @lexical/react
. Try typing in some text with different formats.
\t
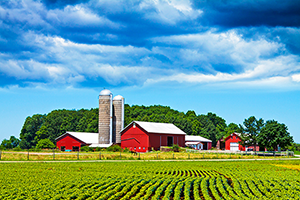
Make sure to check out the various plugins in the toolbar. You can also use #hashtags or @-mentions too!
If you'd like to find out more about Lexical, you can:
Lastly, we're constantly adding cool new features to this playground. So make sure you check back here when you next get a chance 🙂.
''';
+const nestedHTML =
+ '''Welcome to the playground
In case you were wondering what the black box at the bottom is – it's the debug view, showing the current state of the editor. You can disable it by pressing on the settings control in the bottom-left of your screen and toggling the debug view setting. The playground is a demo environment built with @lexical/react
. Try typing in some text with different formats.
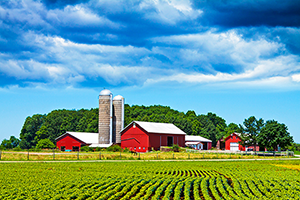
Make sure to check out the various plugins in the toolbar. You can also use #hashtags or @-mentions too!
If you'd like to find out more about Lexical, you can:
- Playground code can be found here.
- Playground code can be found here.
Lastly, we're constantly adding cool new features to this playground. So make sure you check back here when you next get a chance 🙂.
''';
const nestedDelta = {
'document': {
'type': 'page',
@@ -329,7 +329,11 @@ const nestedDelta = {
{'insert': '. Try typing in '},
{
'insert': 'some text',
- 'attributes': {'bold': true}
+ 'attributes': {
+ 'bold': true,
+ "italic": true,
+ 'href': 'https://appflowy.io'
+ }
},
{'insert': ' with '},
{
@@ -340,19 +344,11 @@ const nestedDelta = {
]
}
},
- {
- 'type': 'paragraph',
- 'data': {
- 'delta': [
- {'insert': '\t'}
- ]
- }
- },
{
'type': 'image',
'data': {
'url': 'https://richtexteditor.com/images/editor-image.png',
- 'align': 'center'
+ 'align': 'center',
}
},
{
@@ -401,15 +397,11 @@ const nestedDelta = {
'type': 'image',
'data': {
'url': 'https://richtexteditor.com/images/editor-image.png',
- 'align': 'center'
+ 'align': 'center',
}
}
],
- 'data': {
- 'delta': [
- {'insert': '\t'}
- ]
- }
+ 'data': {'delta': []}
},
{
'type': 'bulleted_list',